- Data Types In C
- The Different Data Types In C Language
- Primary Data Types In C
- Derived Data Types in C
- User-Defined Data Types In C
- Enumerated Data Types In C (With Example)
- Void Data Types In C (With Example)
- Data Type Modifiers In C
- Conclusion
- Frequently Asked Questions
Data Types In C | A Comprehensive Guide (With Examples)
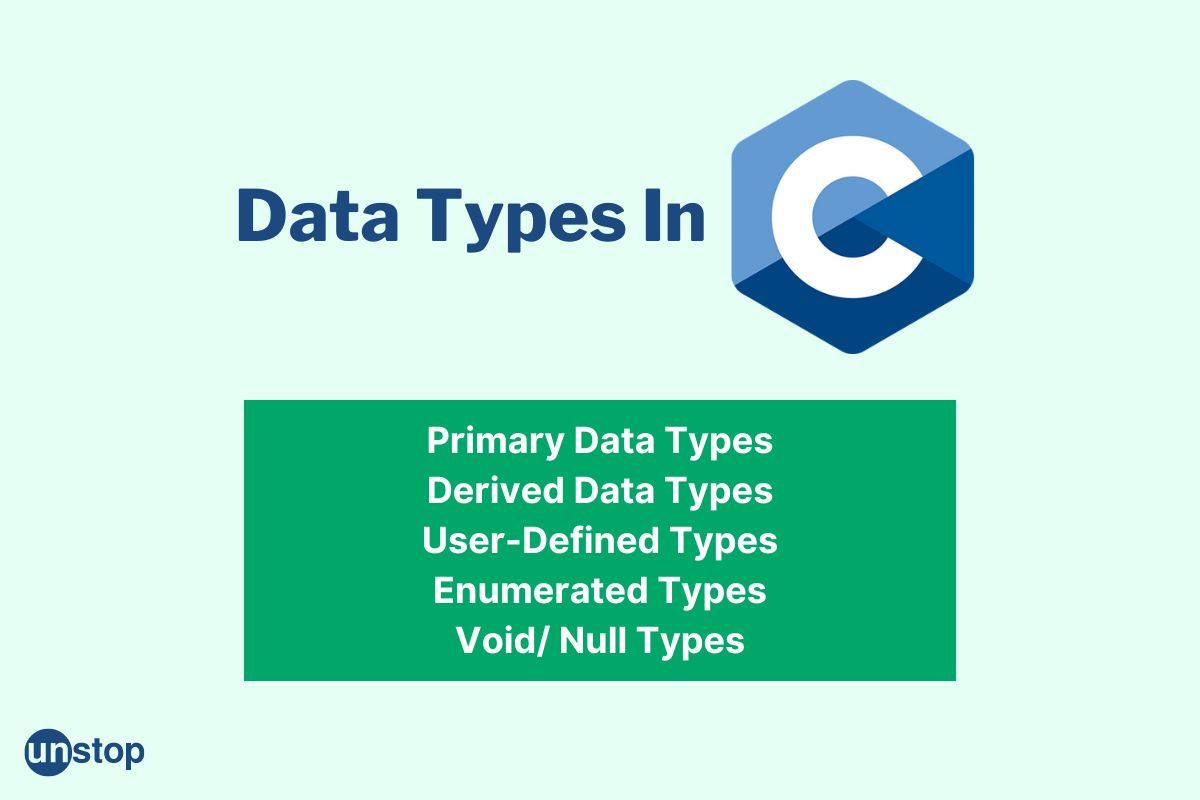
Data types are fundamental in computer programming because they enable a programmer to determine the type of data that is being utilized and saved precisely. Some typical examples are- integers (whole numbers), floating point numbers (numbers with decimals), strings, etc. The storage method and processing instructions differ for every data type. For instance, the text is shown differently than numbers or pictures. In this article, we will discuss data types in C in proper detail, along with examples to help understand the concept and implement it properly.
The primary reason why you should know about and specify data types properly is that it helps a program access and manipulate information more effectively based on the respective data type.
Data Types In C
Data types in C can be divided into 2 main categories- basic data types and derived. Basic C programming language data types are built-in datatypes that store fundamental information such as numbers, letters, and text. Some of the commonly used basic data types in C are char (character), int (integer), float (floating point number), and double(double precision floating point).
Derived or compound C data types entail grouping simple elements to form a complex type. The most common of these are arrays, structures, and unions, which aggregate one or more of the aforementioned primitive components to create new objects with enhanced functionality.
The Different Data Types In C Language
There are 4 different types of data types in C, they are-
- Primitive Data Types: This data type includes- integer data type (int), character data type, i.e., character or small integer value (char), floating-point data type, i.e., containing fractional part (float), and double data type (double), which is the same as float data type, but it occupies twice the storage space.
- Enumerated Data Types: This data type consists of named integral constants represented by identifiers. For example, false/true for boolean types or days in a week like Monday/Tuesday, etc.
- Derived Data Types: These are those data types that are derived from the other basic data types in C. Some common examples of the same are Arrays (i.e., a collection of elements having the same data type stored at contiguous memory locations), Pointers (that store address to a memory location that holds some particular value), Structure (struct, i.e., a user-defined composite datatype containing fields each having different datatypes), and Union contains set of variables sharing common storage area.
- User-Defined Data Types: These are similar to derived data types in that they are a combination of or are derived from other basic data types. But one major difference between derived and user-defined data types is that the latter is created by users themselves.
- Void Data Type/ Null Pointer: This is the data type used when the pointer not pointing to any valid location.
Primary Data Types In C
As we've mentioned above, int, float, double, char, and void are all primary data types. These are the fundamental data types in C used to declare variables. They can hold different values like integer values, fractional values, or characters. Variables declared with primary data type can store only one value at a time and cannot be divided into smaller parts. The size and range of these primary data types are specific for every implementation of the C compiler, depending on machine architecture, etc.
In the table below, we have specified the various primitive data types along with specifier, range, and memory occupied.
Data Types In C |
Format Specifier |
Range |
Memory Size (in bytes) |
unsigned short int |
%hu |
0 to 65,535 |
2 |
short int |
%hd |
-32,768 to 32,767 |
2 |
usigned int |
%u |
0 to 4,294,967,295 |
4 |
Int |
%d |
-2,147,483,648 to 2,147,483,647 |
4 |
long int |
%ld |
-2,147,483,648 to 2,147,483,647 |
4 |
unsigned long int |
%lu |
0 to 4,294,967,295 |
4 |
long long int |
%lld |
-(2^63) to (2^63)-1 |
8 |
unsigned long long int |
%llu |
0 to 18,446,744,073,709,551,615 |
8 |
signed char
|
%c |
-128 to 127 |
1 |
unsigned char |
%c |
0 to 255 |
1 |
float |
%f |
1.2E-38 to 3.4E+38 |
4 |
double |
%lf |
1.7E-308 to 1.7E+308 |
8 |
long double |
%lf |
|
16 |
Integer Data Type in C (With Example)
The integer data type in C is one of the most common data types and is represented by int. It refers to a whole number that can be either positive or negative. Although the implementation (different compilers have different sizes) determines the memory size of an int, they are commonly either 16-bit or 32-bit signed integers. A 16-bit signed integer ranges from -32768 to 32767, and a 32-bit ranges up to 2147483647
The syntax of int data type:
int var1; //creating an integer type variable named 'var1'
Example of integer data type in C:
#include <stdio.h>
#include <limits.h>
int main ( )
{
int minIntValue;
minIntValue = INT_MIN ;
printf("Minimum limit for Integer data stores : %d \n",minIntValue);
return 0;
}
Output:
Minimum limit for Integer data stores: -2147483648
Code Explanation:
The first line includes the standard input/output library, which allows for printing results (printf). The second line includes limits.h, necessary for accessing constants related to integer types such as INT_MIN (the minimum value allowed by an int type). Then it declares a variable named minIntValue of type int and sets its value equal to INT_MIN, using the constant defined in limits.h. Finally, the print() function prints out this result on the screen so that it can be observed by anyone running the program.
Float Data Type in C (With Example)
The float data type allows programs to represent real numbers with decimals like 3.141592 in the program’s codebase instead of integers like 3 only. The float data type size is 4 bytes, i.e., it occupies 4 bytes of memory. It ranges between +/- 3.4E-38F up until +/- 1 .7E+308F depending on the system’s architecture design choice when compiled into an executable file format suitable for processor execution by CPU core( s ) installed inside the computer unit.
The syntax of float data type:
float var2; // creating a float type variable named ‘var2’
Example of floating-point type in C:
#include <stdio.h>
int main()
{
float a = 10.20;
printf("Value of Float variable is : %f\n", a);
return 0;
}
Output:
Value of Float variable is: 10.200000
Code Explanation:
The printf() statement is a standard library function in C that prints the string passed to it along with other optional parameters. It does so according to the format of its first argument, which contains directives describing what should be printed and where. The %f directive is used for printing floating-point numbers and stands for "floating point". The variable 'a' is declared before being passed as an argument so that its value can be printed on the screen.
This declares a float type variable 'a' and assigns the value 10.20 to it. And the printf() statement is used for printing the contents of the variable on the screen.
Double Data Type in C
The double data type in C is a 64-bit floating point number. This means it can represent decimal values with double precision or greater precision than the 32-bit float. It is better suited for larger numbers or calculations involving fractional parts such as 3/2 (1.5). Here, numbers are written using scientific notation; 1e+06 represents one million.
The syntax of double data type:
double var3; //creating a double type variable named 'var3'
Example of double data type in C:
#include <stdio.h>
int main()
{
double d = 5.16;
printf("The Double Value is %f",d);
return 0;
}
Output:
The Double Value is 5.160000
Code Explanation:
Here is an explanation of the code-
- The first line includes the standard input-output library, which contains functions for taking inputs and giving outputs.
- In the second line, a main method is declared, which serves as an entry point of execution for any program written in C language.
- Inside this function, two operations declare a variable ‘d’ with datatype double and assign it value 3.14.
- It then prints out its content stored in the d variable on the console window by printf.
- Finally, the return statement terminates the execution flow from the main() function.
Character Data Type in C (With Example)
The character data type is represented by the char keyword and is used to store a variable with only a single character. The size of the char data type is 1 byte (i.e., 8 bits). It has two subtypes- signed char and unsigned char. The range for these is -127 to 128 and 0 to 255, respectively.
The syntax for a character data type in C:
char var_name;
Example for character data type:
#include <stdio.h>
int main()
{
char var;
printf("Enter a character: ");
scanf("%c", &var);
printf("The character is %c\n", var);
}
Output Window:
Input:
U
Output:
The character is U
Explanation:
The program explains how to declare and use a char type in C. It starts by declaring the character variable, char var, and then prompts the user to enter a character. After that, it reads the character entered and prints out the same in the output window.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Derived Data Types in C
Derived data types in C are created from basic or primitive data types. There are five subtypes of derived/ user-defined types in C; they are as follows:
- Array Type: They are a collection of data stored together as a single unit and indexed according to the associated value or index. Here, one or more elements can be arranged under a common name.
- Pointers: They reference other variables by storing their address in memory. Note that multiple pointers can point to the same object, but they will always have separate addresses
- Structures Types: They are composed of many fields declared within the same block of memory that may contain different types or values. Structure types are accessed via dot notation (e.g., struct_name .field_name).
- Unions: They allow users to combine two different sets of values into one variable, with only one set being active at any given time due to limited resources.
- Functions: These allow grouping logic statements representing calculations or sequences, which can then be referred to through their name without having re-write every time it needs to use
Array Type In C
An array in C is a collection of elements identified by an index number. It allows us to store multiple values under a single variable name and access data quickly using index numbers. Arrays represent lists or sequences of related data items with common properties such as size, type, or range of valid values. The elements stored in an array can be any type (i.e., they can be numbers, strings, etc.). But note that all of the elements must be of the same type for an individual array declaration.
Syntax:
<data_type> <array_name>[<array_size>] = {element1, element2, ..};
Code Example:
#include <stdio.h>
int main()
{
int arr[5] = { 11, 22, 33, 44, 55 };
printf("The numbers in the Array are: \n");
for (int i= 0; i< 5;i++)
{
printf("%d\n",arr[i]);
}
return 0;
}
Output:
The numbers in the Array are:
11
22
33
44
55
Explanation:
This program declares and initializes an array with the size of 5, containing values from 10 to 50. It then uses a for loop that iterates through the array using an index variable (i) to print out each array element on its line. The printf function is used to output each value within the brackets [] when called by its corresponding index number in arr[i]. After all, elements are printed out, 0 is returned as indicated by ‘return 0,’ signaling the successful execution of code thus far before termination.
Pointer Data Type
A pointer is a variable in the C programming language that stores the address of another variable. It makes memory management more effective and can be used to transport data between programs or directly access memory (for example, when creating low-level graphics programs). Programs can run at lower levels than they otherwise could, thanks to pointers with a wide range of uses.
Syntax:
pointer_variable = &data;
*pointer_variable;
Code Example:
#include<stdio.h>
int main()
{
int x=85;
int* ptr;
ptr = &x;
printf("Value at Address %p is: %d\n",ptr , *ptr);
printf("Address of Value Stored by Pointer Variable :%p\n",&ptr);
return 0;
}
Output:
Value at Address 0x7fffd6aeb8ac is: 85
Address of Value Stored by Pointer Variable :0x7fffd6aeb8b0
Explanation:
The above code is a C program that demonstrates the use of pointers.
- It declares an integer variable x and assigns it a value of 85.
- It then creates another variable, ptr, which holds the address of x using the ‘&’ operator.
- Finally, it prints out both values stored in ptr (the address) and *ptr (the actual content at that memory location, i.e., 85).
Structure Type
In C, the structure data type is a collection of one or more variables grouped together with a single given name. It can contain elements of different data types like int, float, char, pointer, etc.
Syntax:
struct <structure_name> { datatype member1; datatype member2; ... .. . };
Code Example:
#include <stdio.h>
struct Employee
{
int id;
char name[25];
};
void main()
{
struct Employee emp1;
printf("Enter Id and Name");
scanf("%d %s", &emp1.id, emp1.name);
printf("Entered Data is \nId = %d\nName = %s",emp1.id, emp1.name);
}
Output:
Enter Id and Name
1 John
Entered Data is
Id = 1
Name = John
Explanation:
This program declares a structure 'Employee' and defines two member variables (id, name) of type int and char array, respectively. It then creates an object emp1 for the structure and reads data from the user into it using scanf(). Finally, it prints this read data on the console output window with the printf() function.
Union Type
The union data type in C is a user-defined datatype that allows users to store different data types in the exact memory location. It is similar to a structure but with one important difference, i.e., only one of its members can be active and contain valid information at any given point in time.
This makes unions extremely useful for saving space when storing large amounts of data in many different types. Unions allow multiple variables to share the same address. Here each variable will interpret the contents differently depending on its declared type, enabling efficient use of memory resources by reusing an allocated region for other purposes as needed.
Syntax:
union union_name { //declaring members of the union member1; member2; ... } variable_name;
Code Example:
#include<stdio.h>
union Data
{
int x;
float y;
}
data;
int main()
{
data.x = 20;
printf("data.x: %d\n", data.x);
data.y = 5.5f ;
printf("data.y: %f", data.y);
return 0;
}
Output:
data.x: 20
data.y: 5.500000
Explanation:
This program demonstrates how to use a union in C. The code first defines the data structure 'Data,' which contains two members, x (an int) and y (a float). It then creates an instance of that structure named 'data.' In this example, the variable is assigned 10 for its integer value and 5.5f for its float value. These values are accessed separately through separate printf statements - one for each union member. Finally, it returns 0 to indicate normal termination of execution.
Function Data Type
A function data type in the C programming language is the information that specifies the kinds of values it can accept, handle, and return. To put it another way, it explains how to use a specific function within your code. Examples of C functions are printf(), scanf(), malloc(), etc. Each of these functions has a specific data type associated with it based on the parameters it accepts as input or returns as a result.
Syntax:
datatype (*function_name)(parameters) = &real_function;
Code Example:
#include<stdio.h>
int add(int a, int b)
{
return (a+b);
}
int main() {
int x=20;
int y=25;
printf("Sum = %d", add(x,y));
return 0;
}
Output:
Sum = 45
Explanation:
The program is a simple C program that defines and calls a function to add two integers. The main() function declares two integer variables, x, and y, with assigned values of 10 and 5, respectively. It then prints the result of calling the add() function on these two integers by passing them as parameters. The add()function takes two integer arguments and returns their sum, printed out via printf(). Finally, 0 is returned from main(), signifying the normal termination of this program.
User-Defined Data Types In C
User-defined data types in C represent abstract data elements using precisely defined structures. These user-defined data types can store collections of related items and are typically used as a type definition for larger projects or libraries. The structure, for example, is created with the struct keyword and contains one or more members, each having a name and associated value.
Structs can also be derived from existing ones allowing complex relationships between different pieces of information within your codebase (i.e., inheritance). User-defined data types provide an essential tool in object-oriented programming, allowing developers to define their proprietary objects that will be accessible throughout the application’s lifetime.
Syntax:
struct defined_type // Defines a new user-defined data type called defined_type
{ // Begin the structure definition.
member1; // Member 1 of your new data type, e.g., an int, float or char*
member2; // Member 2 of your new data type, e.g., an int, float or char*. . . . etc... } ; /// End of structure declaration
Code Example:
#include <stdio.h>
struct Student {
int roll;
char name[20];
float marks;
};
struct Student stuArr[10] = {
{1, "John", 88.3},
{2, "Harry", 75.4},
{3, "Mike", 64.9 },
{4,"Emma", 95.7},
{5, "James", 68.8 }
};
void printStudent(int roll) {
for (int i = 0; i < 10; ++i) {
if (stuArr[i].roll == roll) {
printf("Roll: %d\nName: %s\nMarks: %f\n", stuArr[i].roll, stuArr[i].name, stuArr[i].marks);
break;
}
}
}
// Main Function
int main(void) {
printStudent(4);
return 0;
}
Output:
Roll: 4
Name: Emma
Marks: 95.699997
Explanation:
This program is an example of user-defined data types in C.
- The code creates a struct called Student which includes three members, roll (an integer), name (a character array), and marks (a float).
- A static array of 10 students with their corresponding information are then defined.
- Finally, the printStudent() function prints out the information for any given student determined by its ‘roll’ number passed as an argument to this function.
Enumerated Data Types In C (With Example)
Enumerated data types in C define variables that can only take on predefined values. These values are stored as constants, and the variable must be assigned one or more of these constant values upon its declaration. This is useful when working with sequences such as days of the week, months in a year, etc. This is because it avoids writing out all possible combinations whenever there is an assignment. Enumeration types also improve readability since their code appears logically grouped instead of scattered throughout your program.
Syntax:
enum identifier {Enumerator = constant, Enumerator = constant ... } variablename;
Code Example:
#include<stdio.h>
int main()
{
enum Days {Sun, Mon, Tue, Wed, Thu , Fri , Sat};
enum Days day1;
day1 = Mon;
printf("%d",day1);
return 0;
}
Output:
1
Explanation:
The main() function is declared where program execution begins, and all other code must be nested inside this function’s curly braces.
- An enumerated type (enum) called Days is then created after ‘int main()’ that has seven constant values assigned accordingly: Sun, Mon, Tue...Sat (each assigned an integer value in sequence).
- Then one variable, day1 of enum type Days, is declared outside any loop or conditional statement.
- Finally, it initialized by assigning it one of those constants days before printing its value on-screen using printf().
- The return 0; statement, at last, ensures execution exits gracefully without errors if none were encountered during runtime.
Void Data Types In C (With Example)
In C programming, the void data type is an empty data type with no value and cannot be directly assigned to a variable. It is commonly used in function declarations as a return type indicating that the function does not return any values and that it simply performs some task without producing any results. Void functions are sometimes referred to as "procedures," They may take parameters but do not have a defined set of output values.
Syntax:
void <variable_name>;
Code Example:
#include <stdio.h>
void func(); // Declaring void function
int main()
{
printf("Hello Unstop!\n");
func();
return 0;
}
void func()
{
printf("This is a Void Type Function.");
}
Output:
Hello Unstop!
This is a Void Type Function.
Explanation:
This is a simple example of using the void data type in C programming language.
- It includes two parts: first, it declares a function called func() and specifies that it has no parameters or return types.
- Second, the main program calls this function by invoking its name.
- Inside func(), we have only one line of code, which prints out an informational message to the console window.
- When run, this program will print "Hello Unstop!" followed by "This is a Void Type Function."
Uses Of Void Data Type In C
- The void data type can be used as a function return type. Such functions do not return any value to the calling program.
- It is also used in the header of a C function that requires no arguments, i.e., no parameters are passed during its invocation or call from another part of the code.
- A void pointer (void *) can point to any type, thus allowing for generic programming in C language by providing flexibility when dealing with types and implicitly casting them upon dereferencing.
- Void data types are mainly used in system calls like open(), and close(), which don’t require user input but only perform some action without returning anything meaningful
Data Type Modifiers In C
Modifiers are used to alter the behavior of existing data types. That is, they can be used to increase or decrease the range of possible values assigned to certain variables within your program code. These modifiers are short (s), long (l), signed, and unsigned.
The short modifier reduces the size of the data type in C, while the long modifier increases it. They work with the int data type, i.e., short int for 16-bit and long int for 32-bits, thus altering the range of integers held in memory locations. The unsigned modifiers prevent negative values and allow the storage of twice as many positive numbers in contrast to their signed counterparts.
- Short int: A short integer is a data type representing an integer number between -32768 and 32767 in memory. The size of the short integer typically ranges from 8 to 16 bits (2^8 to 2^16); however exact size depends on the computer architecture. It occupies less space but has a limited range compared with other types, such as long, signed, or unsigned integers.
- Long Int: A long integer is a data type representing an integer number between −231 and 231−1 in memory, greater than what shorts can store – up to 65,535 (-32,768 and 32,767 for shorts). This means more precision when representing large numbers. Again its size varies depending on the computer architecture ranging from 16-64 bits (2^16 to 2^64), usually occupying twice as much space as shorts.
- Signed: Signed refers to whether or not we are storing values and their sign information (+ve/-ve). That is, it allows for the value to be both negative and positive. Note that negative numbers need two's complement representations for efficient storage/computation purposes involving addition/subtraction etc., and will hence need extra bit space.
- Unsigned: Unsigned refers to the lack of sign information, i.e., we only store numeric values, and no sign bit is used for them. If we want our variable to store only positive numbers, then unsigned should be part of the modifier list.
Linking of Data Modifiers
Now both modifiers "Signed" & "Unsigned" can appear with anyone in four original modifiers that are short int, long int, signed, and/or Unsigned, e.g., "long unsigned int" or "short unsigned int," etc. All in all, it depends upon the user as to how much memory space one needs (also provides precision when needed) and whether the value will be +ve/-ve or not.
Modifiers can also help to ensure compatibility between different systems. For example, two programs are compiled on different operating systems with varying sizes of integers and ranges. In that case, the same variable may not have the same value when accessing it in both environments. Modifiers allow us to control how much data is stored so that values read from one system will be correctly converted for another system.
Syntax of Modifiers In C:
<modifier> <data type> <variable name>
E.g.- unsigned short int count;
Code Example:
#include <stdio.h>
int main()
{
short int a;
long int b;
signed c;
unsigned d;
printf("Size of short integer : %zu\n", sizeof(a));
printf("Size of long integer : %zu\n", sizeof(b));
printf("Size of Signed Integer : %zu\n",sizeof(c));
printf("Size of Unsigned Integer : %zu\n",sizeof(d));
}
Output:
Size of short integer : 2
Size of long integer : 8
Size of Signed Integer : 4
Size of Unsigned Integer : 4
Explanation:
In the example above, we are trying to find the size of different types of integers.
- The first line includes the header file <stdio.h>, which contains functions for input and output operations such as printf().
- The next lines declare four integer variables 'a, 'b,' 'c,' and 'd' with different data types, i.e., short int, long int, signed, and unsigned.
- Then the program uses the printf() function to display the size (in bytes) of each variable on the console screen using the %zu specifier, which is used for printing size_t values returned by the size of the operator in the C language.
- Finally, it returns 0, indicating the successful execution of a program.
Conclusion
Data types are essential in computer programming, enabling programmers to store and manipulate information accurately. The C language provides four primary data types (int, float, double, and char) along with other derived/composite ones such as arrays, pointers, structures & unions, plus void/null pointers for empty reference locations commonly used when creating advanced applications. Data type modifiers like signed or unsigned can be applied to the variables declared to control their size & range of acceptable values, which helps improve portability between different systems. Understanding how different data types work is key to writing efficient code that runs consistently regardless of underlying architecture design specs.
Frequently Asked Questions
Q. What are the basic data types in C?
The basic/ primary data types in C are as follows:
- Integer (int): Stores whole numbers without decimals.
- Character (char): Stores single characters enclosed within single quotes (' ').
- Floating Point (float): Stores decimal numbers.
- Double Precision Floating Point Number: Stores more precise decimal numbers than float-type variables.
- Void: Special data type that has no value; used to indicate the absence of a value or as a placeholder for future values.
Q. What is the enumerated data type in the C programming language?
Enumerated data types in C programming language define variables that can only take one value out of a set of values. Enumeration constants are named integer constants associated with an enumerated data type. Common examples include Boolean values such as true and false, days of the week (Monday, Tuesday, etc.), or even more complex structures such as card suits in a deck of cards (Clubs, Diamonds, etc.).
Q. What is the difference between signed and unsigned datatypes?
Signed datatypes can represent positive and negative values, whereas unsigned ones can only represent positive values. Signed variables use the most significant bit (MSB) to store sign information (whether a given number is positive or negative).
Unsigned variables do not include this additional MSB bit, so one more is available for storing numerical data. This means that an unsigned variable has a broader range of possible numbers than its signed counterpart – making it capable of representing larger numerical values within the same amount of memory space.
Q. What is the use of the Void data type in C?
The Void data type in C is a special data type for pointer types, where no value can be stored. The main use of the Void data type is to indicate that a function does not return any value or represent an incomplete definition. It can also be used in place of other more specific user-defined generic types and when casting functions, allowing you to pass arguments through without specifying their exact types.
Q. Can we create our own datatypes in C?
Yes, C allows for the creation of custom data types. The two most common ways are via a typedef or struct. A typedef creates an alias of an existing data type, for example, typedef int MyInt. With structs, you can create fully customized data types that contain multiple elements, for example, struct {int x; char y[100]; float z}.
Q. What is the difference between the data types and variables?
Datatypes are the different data types that can be stored and manipulated in a programming language. Some common data types in C are numbers, strings (text), booleans (true/false values), and objects. Variables, on the other hand, are any named information used to store a value or set of values for later use.
In other words, variables refer to memory locations (or containers) that hold some given value that is changeable during runtime. So, variables have datatypes but don’t directly define them. That is, they take their type from what has been assigned to them at variable declaration.
You might also be interested in reading the following:
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment