- A Brief Intro To C++
- The Timeline Of C++
- Importance Of C++
- Versions Of C++ Language
- Comparison With Other Popular Programming Languages
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Variables In C++?
- Declaration & Definition Of Variables In C++
- Variable Initialization In C++
- Rules & Regulations For Naming Variables In C++ Language
- Different Types Of Variables In C++
- Different Types of Variable Initialization In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Primitive Data Types In C++?
- Derived Data Types In C++
- User-Defined Data Types In C++
- Abstract Data Types In C++
- Data Type Modifiers In C++
- Declaring Variables With Auto Keyword
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- Structure Of C++ Program: Components
- Compilation & Execution Of C++ Programs | Step-by-Step Explanation
- Structure Of C++ Program With Example
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What is Typedef in C++?
- The Role & Applications of Typedef in C++
- Basic Syntax for typedef in C++
- How Does typedef Work in C++?
- How to Use Typedef in C++ With Examples? (Multiple Data Types)
- The Difference Between #define & Typedef in C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Strings In C++?
- Types Of Strings In C++
- How To Declare & Initialize C-Style Strings In C++ Programs?
- How To Declare & Initialize Strings In C++ Using String Keyword?
- List Of String Functions In C++
- Operations On Strings Using String Functions In C++
- Concatenation Of Strings In C++
- How To Convert Int To Strings In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is String Concatenation In C++?
- How To Concatenate Two Strings In C++ Using The ‘+' Operator?
- String Concatenation Using The strcat( ) Function
- Concatenation Of Two Strings In C++ Using Loops
- String Concatenation Using The append() Function
- C++ String Concatenation Using The Inheritance Of Class
- Concatenate Two Strings In C++ With The Friend and strcat() Functions
- Why Do We Need To Concatenate Two Strings?
- How To Reverse Concatenation Of Strings In C++?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is Find In String C++?
- What Is A Substring?
- How To Find A Substring In A String In C++?
- How To Find A Character In String C++?
- Find All Substrings From A Given String In C++
- Index Substring In String In C++ From A Specific Start To A Specific Length
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Pointers In C++?
- Pointer Declaration In C++
- How To Initialize And Use Pointers In C++?
- Different Types Of Pointers In C++
- References & Pointers In C++
- Arrays And Pointers In C++
- String Literals & Pointers In C++
- Pointers To Pointers In C++ (Double Pointers)
- Arithmetic Operation On Pointers In C++
- Advantages Of Pointers In C++
- Some Common Mistakes To Avoid With Pointers In Cpp
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- Understanding Pointers In C++
- What Is Pointer To Object In C++?
- Declaration And Use Of Object Pointers In C++
- Advantages Of Pointer To Object In C++
- Pointer To Objects In C++ With Arrow Operator
- An Array Of Objects Using Pointers In C++
- Base Class Pointer For Derived Class Object In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is 'This' Pointer In C++?
- Defining ‘this’ Pointer In C++
- Example Of 'this' Pointer In C++
- Describing The Constness Of 'this' Pointer In C++
- Important Uses Of 'this' Pointer In C++
- Method Chaining Using 'this' Pointer In C++
- C++ Programs To Show Application Of 'This' Pointer
- How To Delete The ‘this’ Pointer In C++?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What is Reference?
- What is Pointer?
- Comparison Table Of C++ Pointer Vs. Reference
- Differences Between Reference And Pointer: A Detailed Explanation
- Why Are References Less Powerful Than Pointers?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- How To Declare A 2D Array In C++?
- C++ Multi-Dimensional Arrays
- Ways To Initialize A 2D Array In C++
- Methods To Dynamically Allocate A 2D Array In C++
- Accessing/ Referencing Two-Dimensional Array Elements
- How To Initialize A Two-Dimensional Integer Array In C++?
- How To Initialize A Two-Dimensional Character Array?
- How To Enter Data In Two-Dimensional Array In C++?
- Conclusion
- Frequently Asked Questions
- What Are Arrays Of Strings In C++?
- Different Ways To Create String Arrays In C++
- How To Access The Elements Of A String Array In C++?
- How To Convert Char Array To String?
- Conclusion
- Frequently Asked Questions
- What is Memory Allocation in C++?
- The “new" Operator In C++
- The "delete" Operator In C++
- Dynamic Memory Allocation In C++ | Arrays
- Dynamic Memory Allocation In C++ | Objects
- Deallocation Of Dynamic Memory
- Dynamic Memory Allocation In C++ | Uses
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is A Substring In C++ (Substr C++)?
- Example For Substr In C++
- Points To Remember For Substr In C++
- Important Applications Of substr() Function
- How to Get a Substring Before a Character?
- Print All Substrings Of A Given String
- Print Sum Of All Substrings Of A String Representing A Number
- Print Minimum Value Of All Substrings Of A String Representing A Number
- Print Maximum Value Of All Substrings Of A String Representing A Number
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is Operator In C++?
- Types Of Operators In C++ With Examples
- What Are Arithmetic Operators In C++?
- What Are Assignment Operators In C++?
- What Are Relational Operators In C++?
- What Are Logical Operators In C++?
- What Are Bitwise Operators In C++?
- What Is Ternary/ Conditional Operator In C++?
- Miscellaneous Operators In C++
- Precedence & Associativity Of Operators In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is The New Operator In C++?
- Example To Understand New Operator In C++
- The Grammar Elements Of The New Operator In C++
- Storage Space Allocation
- How Does The C++ New Operator Works?
- What Happens When Enough Memory In The Program Is Not Available?
- Initializing Objects Allocated With New Operator In C++
- Lifetime Of Objects Allocated With The New Operator In C++
- What Is The Delete Operator In C++?
- Difference Between New And Delete Operator In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- Types Of Overloading In C++
- What Is Operator Overloading In C++?
- How To Overload An Operator In C++?
- Overloadable & Non-overloadable Operators In C++
- Unary Operator Overloading In C++
- Binary Operator Overloading In C++
- Special Operator Overloading In C++
- Rules For Operator Overloading In C++
- Advantages And Disadvantages Of Operator Overloading In C++
- Function Overloading In C++
- What Is the Difference Between Operator Functions and Normal Functions?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Operators In C++?
- Introduction To Logical Operators In C++
- Types Of Logical Operators In C++ With Example Program
- Logical AND (&&) Operator In C++
- Logical NOT(!) Operator In C++
- Logical Operator Precedence And Associativity In C++
- Relation Between Conditional Statements And Logical Operators In C++
- C++ Relational Operators
- Conclusion
- Frequently Asked Important Interview Questions:
- Test Your Skills: Quiz Time
- Different Type Of C++ Bitwise Operators
- C++ Bitwise AND Operator
- C++ Bitwise OR Operator
- C++ Bitwise XOR Operator
- Bitwise Left Shift Operator In C++
- Bitwise Right Shift Operator In C++
- Bitwise NOT Operator
- What Is The Meaning Of Set Bit In C++?
- What Does Clear Bit Mean?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- Types of Comments in C++
- Single Line Comment In C++
- Multi-Line Comment In C++
- How Do Compilers Process Comments In C++?
- C- Style Comments In C++
- How To Use Comment In C++ For Debugging Purposes?
- When To Use Comments While Writing Codes?
- Why Do We Use Comments In Codes?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Storage Classes In Cpp?
- What Is The Scope Of Variables?
- What Are Lifetime And Visibility Of Variables In C++?
- Types of Storage Classes in C++
- Automatic Storage Class In C++
- Register Storage Class In C++
- Static Storage Class In C++
- External Storage Class In C++
- Mutable Storage Class In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- Decision Making Statements In C++
- Types Of Conditional Statements In C++
- If-Else Statement In C++
- If-Else-If Ladder Statement In C++
- Nested If Statements In C++
- Alternatives To Conditional If-Else In C++
- Switch Case Statement In C++
- Jump Statements & If-Else In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is A Switch Statement/ Switch Case In C++?
- Rules Of Switch Case In C++
- How Does Switch Case In C++ Work?
- The break Keyword In Switch Case C++
- The default Keyword In C++ Switch Case
- Switch Case Without Break And Default
- Advantages & Disadvantages of C++ Switch Case
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is A For Loop In C++?
- Syntax Of For Loop In C++
- How Does A For Loop In C++ Work?
- Examples Of For Loop Program In C++
- Ranged Based For Loop In C++
- Nested For Loop In C++
- Infinite For Loop In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is A While Loop In C++?
- Parts Of The While Loop In C++
- C++ While Loop Program Example
- How Does A While Loop In C++ Work?
- What Is Pre-checking Process Or Entry-controlled Loop?
- When Are While Loops In C++ Useful?
- Example C++ While Loop Program
- What Are Nested While Loops In C++?
- Infinite While Loop In C++
- Alternatives To While Loop In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Loops & Its Types In C++?
- What Is A Do-While Loop In C++?
- Do-While Loop Example In C++ To Print Numbers
- How Does A Do-While Loop In C++ Work?
- Various Components Of The Do-While Loop In C++
- Example 2: Adding User-Input Positive Numbers With Do-While Loop
- C++ Nested Do-While Loop
- C++ Infinitive Do-while Loop
- What is the Difference Between While Loop and Do While Loop in C++?
- When To Use A Do-While Loop?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are 2D Vectors In C++?
- How To Declare 2D Vector In C++?
- How To Initialize 2D Vector In C++?
- C++ Program Examples For 2D Vectors
- How To Access & Modify 2D Vector Elements In C++?
- Methods To Traverse, Manipulate & Print 2D Vectors In C++
- Adding Elements To 2-D Vector Using push_back() Function
- Removing Elements From Vector In C++ Using pop_back() Function
- Creating 2D Vector In C++ With User Input For Size Of Column & Row
- Advantages of 2D Vectors Over Traditional Arrays
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- How To Print A Vector In C++ By Overloading Left Shift (<<) Operator?
- How To Print Vector In C++ Using Range-Based For-Loop?
- Print Vector In C++ With Comma Separator
- Printing Vector In C++ Using Indices (Square Brackets/ Double Brackets & at() Function)
- How To Print A Vector In C++ Using std::copy?
- How To Print A Vector In C++ Using for_each() Function?
- Printing C++ Vector Using The Lambda Function
- How To Print Vector In C++ Using Iterators?
- Conclusion
- Frequently Asked Questions
- Definition Of C++ Find In Vector
- Using The std::find() Function
- How Does find() In Vector C++ Function Work?
- Finding An Element By Custom Comparator Using std::find_if() Function
- Use std::find_if() With std::distance()
- Element Find In Vector C++ Using For Loop
- Using The find_if_not Function
- Find Elements With The Linear Search Approach
- Conclusion
- Frequently Asked Questions
- What Is Sort() Function In C++?
- Sort() Function In C++ From Standard Template Library
- Exceptions Of Sort() Function/ Algorithm In C++
- The Stable Sort() Function In C++
- Partial Sort() Function In C++
- Sorting In Ascending Order With Sort() Function In C++
- Sorting In Descending Order With Sort Function In C++
- Sorting In Desired Order With Custom Comparator Function & Sort Function In C++
- Sorting Elements In Desired Order Using Lambda Expression & Sort Function In C++
- Types of Sorting Algorithms In C++
- Advanced Sorting Algorithms In C++
- How Does the Sort() Function Algorithm Work In C++?
- Conclusion
- Frequently Asked Questions
- What Is Function Overloading In C++?
- Ways Of Function Overloading In C++
- Function Overloading In C++ Using Different Types Of Parameters
- Function Overloading In C++ With Different Number Of Parameters
- Function Overloading In C++ Using Different Sequence Of Parameters
- How Does Function Overloading In C++ Work?
- Rules Of Function Overloading In C++
- Why Is Function Overloading Used?
- Types Of Function Overloading Based On Time Of Resolution
- Causes Of Function Overloading In C++
- Ambiguity & Function Overloading In C++
- Advantages Of Function Overloading In C++
- Disadvantages Of Function Overloading In C++
- Operator Overloading In C++
- Function Overriding In C++
- Difference Between Function Overriding & Function Overloading In C++
- Conclusion
- Frequently Asked Questions
- What Is An Inline Function In C++?
- How To Define The Inline Function In C++?
- How Does Inline Function In C++ Work?
- The Need For An Inline Function In C++
- Can The Compiler Ignore/ Reject Inline Function In C++ Programs?
- Normal Function Vs. Inline Function In C++
- Classes & Inline Function In C++
- Understanding Inline, __inline, And __forceinline Functions In C++
- When To Use An Inline Function In C++?
- Advantages Of Inline Function In C++
- Disadvantages Of Inline Function In C++
- Why Not Use Macros Instead Of An Inline Function In C++?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is Static Data Member In C++?
- How To Declare Static Data Members In C++?
- How To Initialize/ Define Static Data Member In C++?
- Ways To Access A Static Data Member In C++
- What Are Static Member Functions In C++?
- Example Of Member Function & Static Data Member In C++
- Practical Applications Of Static Data Member In C++
- Conclusion
- Frequently Asked Questions
- What Is A Constant In C++?
- Ways To Define Constant In C++
- What Are Literals In C++?
- Pointer To A Constant In C++
- Constant Function Arguments In C++
- Constant Member Function Of Class In C++
- Constant Data Members In C++
- Object Constant In C++
- Conclusion
- Frequently Asked Questions(FAQ)
- What Is Friend Function In C++?
- Declaration Of Friend Function In C++ With Example
- Characteristics Of Friend Function In C++
- Global Friend Function In C++ (Global Function As Friend Function )
- Member Function Of Another Class As Friend Function In C++
- Function Overloading Using Friend Function In C++
- Advantages & Disadvantages Of Friend Function in C++
- What Is A C++ Friend Class?
- A Function Friendly To Multiple Classes
- C++ Friend Class Vs. Friend Function In C++
- Some Important Points About Friend Functions And Classes In C++
- Conclusion
- Frequently Asked Questions
- What Is Function Overriding In C++?
- The Working Mechanism Of Function Overriding In C++
- Real-Life Example Of Function Overriding In C++
- Accessing Overriding Function In C++
- Accessing Overridden Function In C++
- Function Call Binding With Class Objects | Function Overriding In C++
- Function Call Binding With Base Class Pointers | Function Overriding In C++
- Advantages Of Function Overriding In C++
- Variations In Function Overriding In C++
- Function Overloading In C++
- Function Overloading Vs Function Overriding In C++
- Conclusion
- Frequently Asked Questions
- Errors In C++
- What Is Exception Handling In C++?
- Exception Handling In C++ Program Example
- C++ Exception Handling: Basic Keywords
- The Need For C++ Exception Handling
- C++ Standard Exceptions
- C++ Exception Classes
- User-Defined Exceptions In C++
- Advantages & Disadvantages Of C++ Exception Handling
- Conclusion
- Frequently Asked Questions
- What Are Templates In C++ & How Do They Work?
- Types Of Templates In C++
- What Are Function Templates In C++?
- C++ Template Functions With Multiple Parameters
- C++ Template Function Overloading
- What Are Class Templates In C++?
- Defining A Class Member Outside C++ Template Class
- C++ Template Class With Multiple Parameters
- What Is C++ Template Specialization?
- How To Specify Default Arguments For Templates In C++?
- Advantages Of C++ Templates
- Disadvantages Of C++ Templates
- Difference Between Function Overloading And Templates In C++
- Conclusion
- Frequently Asked Questions
- Structure
- Structure Declaration
- Initialization of Structure
- Copying and Comparing Structures
- Array of Structures
- Nested Structures
- Pointer to a Structure
- Structure as Function Argument
- Self Referential Structures
- Class
- Object Declaration
- Accessing Class Members
- Similarities between Structure and Class
- Which One Should You Choose?
- Key Difference Between a Structure and Class
- Summing Up
- Test Your Skills: Quiz Time
- What Is A Class And Object In C++?
- What Is An Object In C++?
- How To Create A Class & Object In C++? With Example
- Access Modifiers & Class/ Object In C++
- Member Functions Of A Class In C++
- How To Access Data Members And Member Functions?
- Significance Of Class & Object In C++
- What Are Constructors In C++ & Its Types?
- What Is A Destructor Of Class In C++?
- An Array Of Objects In C++
- Object In C++ As Function Arguments
- The this (->) Pointer & Classes In C++
- The Need For Semicolons At The End Of A Class In C++
- Difference Between Structure & Class In C++
- Conclusion
- Frequently Asked Questions
- What Are Static Members In C++?
- Static Member Functions in C++
- Ways To Call Static Member Function In C++
- Properties Of Static Member Function In C++
- Need Of Static Member Functions In C++
- Regular Member Function Vs. Static Member Function In C++
- Limitations Of Static Member Functions In C++
- Conclusion
- Frequently Asked Questions
- What Is Constructor In C++?
- Characteristics Of A Constructor In C++
- Types Of Constructors In C++
- Default Constructor In C++
- Parameterized Constructor In C++
- Copy Constructor In C++
- Dynamic Constructor In C++
- Benefits Of Using Constructor In C++
- How Does Constructor In C++ Differ From Normal Member Function?
- Constructor Overloading In C++
- Constructor For Array Of Objects In C++
- Constructor In C++ With Default Arguments
- Initializer List For Constructor In C++
- Dynamic Initialization Using Constructor In C++
- Conclusion
- Frequently Asked Questions
- What Is A Constructor In C++?
- What Is Constructor Overloading In C++?
- Dеclaration Of Constructor Ovеrloading In C++
- Condition For Constructor Overloading In C++
- How Constructor Ovеrloading In C++ Works?
- Examples Of Constructor Overloading In C++
- Lеgal & Illеgal Constructor Ovеrloading In C++
- Types Of Constructors In C++
- Characteristics Of Constructors In C++
- Advantage Of Constructor Overloading In C++
- Disadvantage Of Constructor Overloading In C++
- Conclusion
- Frеquеntly Askеd Quеstions
- What Is A Destructor In C++?
- Rules For Defining A Destructor In C++
- When Is A Destructor in C++ Called?
- Order Of Destruction In C++
- Default Destructor & User-Defined Destructor In C++
- Virtual Destructor In C++
- Pure Virtual Destructor In C++
- Key Properties Of Destructor In C++ You Must Know
- Explicit Destructor Calls In C++
- Destructor Overloading In C++
- Difference Between Normal Member Function & Destructor In C++
- Important Uses Of Destructor In C++
- Conclusion
- Frequently Asked Questions
- What Is A Constructor In C++?
- What Is A Destructor In C++?
- Difference Between Constructor And Destructor In C++
- Constructor In C++ | A Brief Explanation
- Destructor In C++ | A Brief Explanation
- Difference Between Constructor And Destructor In C++ Explained
- Order Of Calling Constructor And Destructor In C++ Classes
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is Type Conversion In C++?
- What Is Type Casting In C++?
- Types Of Type Conversion In C++
- Implicit Type Conversion (Coercion) In C++
- Explicit Type Conversion (Casting) In C++
- Advantages Of Type Conversion In C++
- Disadvantages Of Type Conversion In C++
- Difference Between Type Casting & Type Conversion In C++
- Application Of Type Casting In C++
- Conclusion
- Frequently Asked Questions
- What Is A Copy Constructor In C++?
- Characteristics Of Copy Constructors In C++
- Types Of Copy Constructors In C++
- When Do We Call The Copy Constructor In C++?
- When Is A User-Defined Copy Constructor Needed In C++?
- Types Of Constructor Copies In C++
- Can We Make The Copy Constructor In C++ Private?
- Assignment Operator Vs Copy Constructor In C++
- Example Of Class Where A Copy Constructor Is Essential
- Uses Of Copy Constructors In C++
- Conclusion
- Frequently Asked Questions
- Why Do You Need Object-Oriented Programming (OOP) In C++?
- OOPs Concepts In C++ With Examples
- The Class OOPs Concept In C++
- The Object OOPs Concept In C++
- The Inheritance OOPs Concept In C++
- Polymorphism OOPs Concept In C++
- Abstraction OOPs Concept In C++
- Encapsulation OOPs Concept In C++
- Other Features Of OOPs In C++
- Benefits Of OOP In C++ Over Procedural-Oriented Programming
- Disadvantages Of OOPS Concept In C++
- Why Is C++ A Partial OOP Language?
- Conclusion
- Frequently Asked Questions
- Introduction To Abstraction In C++
- Types Of Abstraction In C++
- What Is Data Abstraction In C++?
- Understanding Data Abstraction In C++ Using Real Life Example
- Ways Of Achieving Data Abstraction In C++
- What Is An Abstract Class?
- Advantages Of Data Abstraction In C++
- Use Cases Of Data Abstraction In C++
- Encapsulation Vs. Abstraction In C++
- Conclusion
- Frequently Asked Questions
- What Is Encapsulation In C++?
- How Does Encapsulation Work In C++?
- Types Of Encapsulation In C++
- Why Do We Need Encapsulation In C++?
- Implementation Of Encapsulation In C++
- Access Specifiers & Encapsulation In C++
- Role Of Access Specifiers In Encapsulation In C++
- Member Functions & Encapsulation In C++
- Data Hiding & Encapsulation In C++
- Features Of Encapsulation In C++
- Advantages & Disadvantages Of Encapsulation In C++
- Difference Between Abstraction and Encapsulation In C++
- Conclusion
- Frequently Asked Questions
- What Is Inheritance In C++?
- What Are Child And Parent Classes?
- Syntax And Structure Of Inheritance In C++
- Implementing Inheritance In C++
- Importance Of Inheritance In C++
- Types Of Inheritance In C++
- Visibility Modes Of Inheritance In C++
- Access Modifiers & Inheritance In C++
- How To Make A Private Member Inheritable?
- Member Function Overriding In Inheritance In C++
- The Diamond Problem | Inheritance In C++ & Ambiguity
- Ways To Avoid Ambiguity Inheritance In C++
- Why & When To Use Inheritance In C++?
- Advantages Of Inheritance In C++
- The Disadvantages Of Inheritance In C++
- Conclusion
- Frequently Asked Questions
- What Is Hybrid Inheritance In C++?
- Importance Of Hybrid Inheritance In Object Oriented Programming
- Example Of Hybrid Inheritance In C++: Using Single and Multiple Inheritance
- Example Of Hybrid Inheritance In C++: Using Multilevel and Hierarchical Inheritance
- Real-World Applications Of Hybrid Inheritance In C++
- Conclusion
- Frequently Asked Questions
- What Is Multiple Inheritance In C++?
- Examples Of Multiple Inheritance In C++
- Ambiguity Problem In Multiple Inheritance In C++
- Ambiguity Resolution In Multiple Inheritance In C++
- The Diamond Problem In Multiple Inheritance In C++
- Visibility Modes In Multiple Inheritance In C++
- Advantages & Disadvantages Of Multiple Inheritance In C++
- Multiple Inheritance Vs. Multilevel Inheritance In C++
- Conclusion
- Frequently Asked Questions
- What Is Multilevel Inheritance In C++?
- Block Diagram For Multilevel Inheritance In C++
- Multilevel Inheritance In C++ Example
- Constructor & Multilevel Inheritance In C++
- Use Cases Of Multilevel Inheritance In C++
- Multiple Vs Multilevel Inheritance In C++
- Advantages & Disadvantages Of Multilevel Inheritance In C++
- Conclusion
- Frequently Asked Questions
- What Is Hierarchical Inheritance In C++?
- Example 1: Hierarchical Inheritance In C++
- Example 2: Hierarchical Inheritance In C++
- Impact of Visibility Modes In Hierarchical Inheritance In C++
- Advantages And Disadvantages Of Hierarchical Inheritance In C++
- Use Cases Of Hierarchical Inheritance In C++
- Conclusion
- Frequently Asked Questions
- What Are Access Specifiers In C++?
- Types Of Access Specifiers In C++
- Public Access Specifiers In C++
- Private Access Specifier In C++
- Protected Access Specifier In C++
- The Need For Access Specifiers In C++
- Combined Example For All Access Specifiers In C++
- Best Practices For Using Access Specifiers In C++
- Why Can't Private Members Be Accessed From Outside A Class?
- Conclusion
- Frequently Asked Questions
- What Is The Diamond Problem In C++?
- Example Of The Diamond Problem In C++
- Resolution Of The Diamond Problem In C++
- Virtual Inheritance To Resolve Diamond Problem In C++
- Scope Resolution Operator To Resolve Diamond Problem In C++
- Conclusion
- Frequently Asked Questions
Data Types In C++ | All 4 Categories Explained With Code Examples
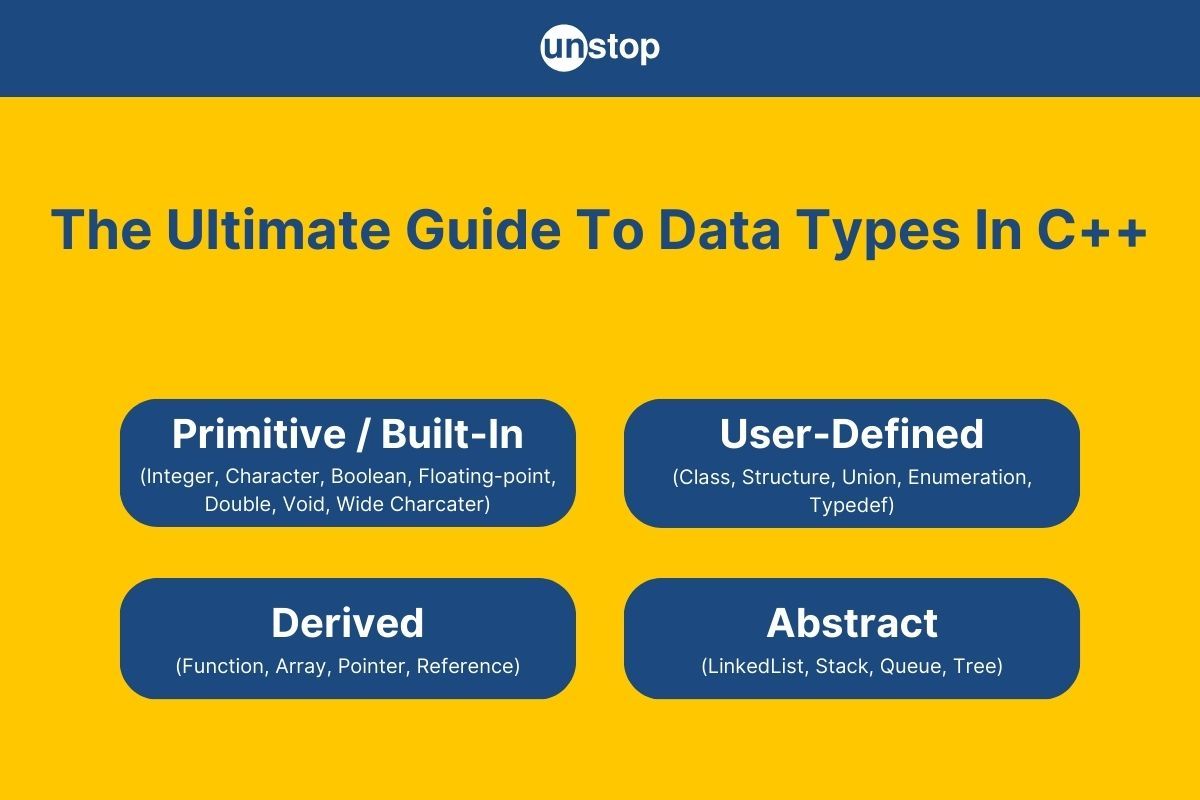
One of the fundamental concepts of any programming language is data types. What is that? In simple terms, it refers to the type of data stored in a variable. There are a wide variety of data types in C++ programming that are used for different purposes and intentions. This type not only determines the type of data but also the memory size/ space it takes. For example, the integer data type takes 4 bytes of space in memory.
All the data types in C++ can be classified into 4 categories (or types): primitive/ built-in types, derived data types, user-defined types, and abstract data types. In this article, we will discuss the categories of data types in Cpp in detail, as well as their subtypes, with the help of code examples.
What Are Primitive Data Types In C++?
Primitive data types, also referred to as built-in data types in C++, are ones that are predefined in a language. In this section, we will look at these common data types in C++ programming language (and their type sizes) that represent basic values, such as integers, characters, and floating-point numbers. We have explained all these basic types in detail, followed by a sample C++ program.
The Integer Data Type In C++
The int keyword represents the integer data type/ variable type that stores integral values without decimal values. The size of an integer type depends on the platform and generally varies between 2 bytes and 4 bytes of memory space. The range of values is (-)2,147,483,648 to 2,147,483,647.
Syntax:
int myInteger = 42;
The Unsigned Integer Data Type In C++
This is similar to int, but the difference is that type unsigned can only represent non-negative numeric values without decimal places. The format specifier for this type of unsigned int is %u. It also takes up to 4 bytes of memory space and has a range of 0 to 4,294,967,295.
Syntax:
unsigned int myUnsignedInteger = 123;
The Character Data Type (char) In C++
This fundamental type is represented by the keyword char. It refers to a single character data type/ alphabetical character value. When we initialize a variable with a char data type, we must enclose the respective characters in single quotes. The size of the char variable is typically 1 byte of memory space, and it can represent 256 different characters.
Syntax:
char myChar = 'A';
The Unsigned Character Data Type In C++
This is similar to a single char, but the type unsigned only represents non-negative values. And it takes up 1 byte of memory space.
Syntax:
unsigned char myUnsignedChar = 'X';
The Float Type/ Floating-Point Data Type In C++
The float data type refers to a floating-point value, i.e., a value that has a whole integer part with a single precision of approximately 6 decimal digits. A float variable data type generally occupies 4 bytes of space in memory.
Syntax:
float myFloat = 3.14f;
The Double Data Type In C++
The type double represents a floating-point number with double precision of approximately 15 decimal digits. This can be considered an extension of the floating-point type and is hence also referred to as double floating or double-precision floating-point value. The size of this double variable is typically 8 bytes of memory space.
Syntax:
double myDouble = 2.71828;
The Long Double Type In C++
The long double keyword represents a floating-point number with quadruple precision. The size of this data type is at least 8 bytes while going up to 16 bytes on certain systems and compilers.
Syntax:
long double myLongDouble = 3.14159265358979323846L;
The Bool Type Data Type In C++
This data type represents a Boolean variable or boolean values, i.e., either true or false. In terms of numbers, a true equates to 1, and a false equates to 0. The size of a boolean data type is typically 1 byte.
Syntax:
bool myBool = true;
The Long Data Type IN C++
Represents integer values that require more storage space than int data type (since it is longer). Typically its size is 4 bytes, but it can go up to 8 bytes depending on the system and compiler used.
Syntax:
long myLong = 123456L;
The Unsigned Long Data Type In C++
This is similar to long, but the type unsigned can represent only non-negative values. It also takes 4 bytes of memory and can go to 8 bytes depending on the system and compiler used. It ranges from 0 to 4,294,967,295.
Syntax:
unsigned long myUnsignedLong = 987654UL;
The Long Long Type In C++
This represents integer values that require more storage space than even the long int data type. It’s typically 8 bytes in size, and ranges from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807.
Syntax:
long long myLongLong = 9223372036854775807LL;
The Unsigned Long Long Type In C++
This is similar to long long, with the type unsigned indicating only non-negative values. It takes up 8 bytes of memory and has a range of 0 to 18,446,744,073,709,551,615.
Syntax:
unsigned long long myUnsignedLongLong = 18446744073709551615ULL;
The Short Data Type In C++
This represents integers that require less storage space than int (since it is shorter). It takes up to 2 bytes of memory and has a range of -32,768 to 32,767.
Syntax:
short myShort = 32767;
The Unsigned Short Data Type In C++
This is similar to short, but it can represent only non-negative values as indicated by the unsigned keyword. It also takes up 2 bytes of memory while the range values are 0 to 65,535.
Syntax:
unsigned short myUnsignedShort = 65535;
The Wide Character Type In C++
A wide character data type, represented by wchar_t notation, refers to characters that require more storage than the typical 8-bit (1 byte) char type, allowing for a larger set of characters to be represented. It typically takes 2 bytes of space but can go up to 4 bytes as well.
It is essential for handling extended character sets that include characters from various languages and symbols beyond what the normal 8-bit data type (char) can represent.
The Void/ Empty Data Type In C++
The void data types are special types that represent the absence of a specific type. That is, the values stored in void type variables lack a particular type. They are mainly used as return types in functions (called void functions) and to declare pointers of type void.
Syntax:
void myFunction() {
// Function with no return type
}
Example For Primitive Data Types In C++
These primitive or built-in datatypes can be used in combinations such as long double, short int, short double, etc. The simple C program below shows all these standard data types along with their sizes in C++.
Code Example:
#include<iostream>
using namespace std;
int main(){
cout<<" size of int(in bytes):"<<sizeof(int)<<endl;
cout<<" size of unsigned int(in bytes):"<<sizeof(unsigned int)<<endl;
cout<<" size of char(in bytes):"<<sizeof(char)<<endl;
cout<<" size of unsigned char(in bytes):"<<sizeof(unsigned char)<<endl;
cout<<" size of float(in bytes):"<<sizeof(float)<<endl;
cout<<" size of double(in bytes):"<<sizeof(double)<<endl;
cout<<" size of long double(in bytes):"<<sizeof(long double)<<endl;
cout<<" size of bool(in bytes):"<<sizeof(bool)<<endl;
cout<<" size of long(in bytes):"<<sizeof(long)<<endl;
cout<<" size of unsigned long(in bytes):"<<sizeof(unsigned long)<<endl;
cout<<" size of long long(in bytes):"<<sizeof(long long)<<endl;
cout<<" size of unsigned long long(in bytes):"<<sizeof(unsigned long long)<<endl;
cout<<" size of short(in bytes):"<<sizeof(short)<<endl;
cout<<" size of unsigned short(in bytes):"<<sizeof(unsigned short)<<endl;
}
Output:
size of int(in bytes):4
size of unsigned int(in bytes):4
size of char(in bytes):1
size of unsigned char(in bytes):1
size of float(in bytes):4
size of double(in bytes):8
size of long double(in bytes):16
size of bool(in bytes):1
size of long(in bytes):8
size of unsigned long(in bytes):8
size of long long(in bytes):8
size of unsigned long long(in bytes):8
size of short(in bytes):2
size of unsigned short(in bytes):2
Explanation:
We begin the C++ program example by including the <iostream> header file needed for input/ output operations and also declare the usage of namespace std.
- Inside the main() function, we use a series of cout statements to print the sizes (in bytes) of different data types.
- The cout statements include a formatted string where the program fills in the sizes using the sizeof() operator. This operator returns the size in bytes of the specified data type.
- The data types include int, unsigned int, char, unsigned char, float, double, long double, bool, long, unsigned long, long long, unsigned long long, short, and unsigned short.
- The output of the program will display the sizes of each data type in bytes.
Derived Data Types In C++
The data types that are defined with the help of the fundamental data types (referred to as base types) are called derived data types. There are four derived data types in C++, namely arrays, pointers, function types, and references.
Function Types In C++
A function is a block of code that defines how to perform a specific task/ operation on the input values it takes. They provide a way to modularize code by grouping a set of instructions under a single name. We can then use a function to perform the specific task repeatedly, thus improving code organization, readability, and reusability.
Syntax:
returnType functionName(parameterType1 parameterName1, parameterType2 parameterName2, ...) {
// Function body
return returnValue;} // Return statement (if applicable)
Here,
- The returnType refers to the data type of the return value, and the return keyword indicates that it is the return statement.
- The functionName refers to the identifier/ name of the function, and the curly braces contain the lines of code that make up its body.
- ParamterType1, paramterType2, and so on refer to the data type of the parameters the function takes and their names are given by parameterName.
Code Example:
#include <iostream>
// Function declaration
int addNumbers(int a, int b);
int main() {
// Function call
int result = addNumbers(5, 7);
// Output the result
std::cout << "Sum: " << result << std::endl;
return 0;
}
// Function definition
int addNumbers(int a, int b) {
// Function body
int sum = a + b;
// Return statement
return sum;
}
Output:
Sum: 12
Explanation:
- We begin the C code by declaring a function named addNumbers(). This function takes two integer parameters (a and b), calculates the sum of them, and returns the result.
- Inside the main() function, we call the addNumbers() function with the values 5 and 7 as arguments.
- This call invokes the function and stores the total/ sum in the variable result.
- We then print the result to the console using the cout statement, as mentioned in the code comments.
- The main() function terminates with a return 0 statement indicating successful completion.
- Outside of the main(), we define the function, which uses the addition arithmetic operator to calculate the sum and return the same.
Also Read- Function Prototype In C++ | Definition, Purpose & More (+Examples)
C++ Array
An array is a collection of elements of the same data type stored in contiguous memory locations. The elements are accessed by their position, which is specified by an index value. IN an array data type, the index starts from 0, i.e., the 1st element is at index 0, and so on.
The size of an array is fixed at compile-time and can’t be changed during program execution. Array input and output are usually dealt with with the help of control statements.
Syntax:
DataType arrayName[Size];
Here,
- DataType refers to the type of the values stored inside the array, which can be integers, characters, strings (interestingly, a string is an array of characters), etc.
- The arrayName refers to the name we give to the array, and the size refers to the number of elements inside the array.
Below is an example that shows the implementation of the array in a C++ program.
Code Example:
#include<iostream>
using namespace std;
int main(){
int arr[10];//declaring an array of size 10
for(inti=0; i<10; i++){
arr[i] = i+1;//storing the numbers in the array
}
for(inti=0; i<10; i++){
cout<<arr[i]<<" ";//printing the numbers in the array
}
}
Output:
1 2 3 4 5 6 7 8 9 10
Explanation:
We begin the example C program above by including essential header files and indicating the usage of the namespace.
- Inside the main() function, we declare an integer array arr of size 10, meaning it can store up to 10 integer values.
- Then, we create a for loop to initialize the array. The loop control variable i is initially set to 0, and loop continues iterations will it remains less than 10.
- In every iteration, the loop accesses the array element at ith position and assigns a value i+1 to it. It then increments the value of the control variable 1 and continues iteration.
- By the end of this loop, the array will be initialized with numbers from 1 to 10.
- We use another for loop containing cout statements to traverse through the array and print its elements separated by white space.
C++ - Pointer Data Type
A pointer is a derived data type that stores the memory address of another variable. It allows us to indirectly access a variable by manipulating the address stored in the pointer. They are often used for dynamic memory allocation at run-time and are instrumental in implementing abstract data types such as linked lists, trees, stacks, queues, etc.
Syntax:
dataType* pointerName;
Here,
- dataType refers to the type of the variable whose location is being pointed to.
- The pointerName refers to the name we give to the pointer being declared.
Code Example:
#include<iostream>
using namespace std;
int main(){
int n=10; // declaring & initializing integer variable
int* ptr=&n; // declaring & initializing pointer variable to store address of the integer variable
cout<<"The value of n: "<<n<<endl;
cout<<"The address of n: "<<&n<<endl;
cout<<"The value of ptr (address stored in it): "<<ptr<<endl;
cout<<"The value of *ptr(value at the address stored in ptr): "<<*ptr<<endl;
cout<<"The address of ptr: "<<&ptr<<endl;
cout<<"The size of ptr: "<<sizeof(ptr)<<endl;
}
Output:
The value of n: 10
The address of n: 0x7ffd775961bc
The value of ptr (address stored in it): 0x7ffd775961bc
The value of *ptr(value at the address stored in ptr): 10
The address of ptr: 0x7ffd775961c0
The size of ptr: 8
Explanation:
- In the main() function of the example above, we first declare an integer variable n and initialize it with the value 10.
- Next, we declare a pointer variable ptr and initialize it with the address of the integer variable n using the address of/ reference operator.
- We then use a series of cout statements to print the following-
- The value is stored in variable n and its address, which we get using the address-of operator.
- Then, we print the value stored in pointer ptr (which is the address of n) and the data at the address stored in ptr (which is the value of n).
- Similarly, we print the address of the ptr variable and the size of the pointer using the sizeof() operator.
- As shown in the output, the pointer variable ptr points to the data stored inside it, which in this case is n.
Also, read- Dynamic Memory Allocation In C++ Explained In Detail (With Examples)
User-Defined Data Types In C++
User-defined data types are the ones that a user creates using predefined or built-in data types, for their specific purpose. There are several ways to define custom data types in C++ that allow programmers to cater to more complex data types than those provided by primitive data types. This includes structures, unions, enumerations, classes, and typedef.
Enumerations Data Type In C++
Enumerations are user-defined data types that consist of a set of name constants or enumerators. They are often used to represent a set of related values that have a common meaning. In C++, they are defined using the enum keyword, and each value is assigned an integer by default. They are used to improve the readability and maintainability of the code.
Syntax:
enum EnumName {
EnumValue1,
EnumValue2,
// ... additional values
};
Here,
- The enum keyword indicates that the data type of the variable is an enumeration.
- EnumName refers to the name of the variable, and EnumValue refers to the respective instances of the variable type/ values we assign to them.
Here is an example code that shows how enumerators work in C++.
Code Example:
#include<iostream>
using namespace std;
int main(){
enum dayofweek{monday,tuesday,wednesday,thursday,friday,saturday,sunday};
enum dayofweek day1=monday;
enum dayofweek day2=tuesday;
enum dayofweek day3=wednesday;
enum dayofweek day4=thursday;
enum dayofweek day5=friday;
enum dayofweek day6=saturday;
enum dayofweek day7=sunday;
cout<<day1<<""<<day2<<""<<day3<<""<<day4<<""<<day5<<""<<day6<<""<<day7<<endl;
}
Output:
0 1 2 3 4 5 6
Explanation:
- We define an enumeration (enum) named dayofweek inside the main() function. This enum variable represents the days of the week.
- Next, we create 7 instances of the enum variable, one for each day of the week, i.e., day1, day2, so on, and assign values corresponding to the days.
- Then, we use a series of cout statements to print the numeric values assigned to each day of the week using the enum instances.
Structures Data Types In C++
The C++ structures are user-defined data types that group together variables of different data types under a single name (or single type, since structure is also a type). They are often used to represent real-world entities that have multiple attributes. This way they help organize data and improve the readability, maintenance, and modularity of code.
Syntax:
struct StructName {
dataType1 member1;
dataType2 member2;
// ... additional members
};
Here,
- The struct keyword indicates that we are defining a structure data type variable whose name is given by StructName.
- The dataType and member indicate the type of the data member and its name, respectively.
Look at the example below to understand how structures work in C++ programs.
Code Example:
#include<iostream>
using namespace std;
struct Employee{
char name[30];
char hiredate[30];
float salary;
};
int main(){
struct Employee emp={"Shivi","31/04/2024",80000.00};
cout<<"The Employee Details Are-"<<endl;
cout<<"Name of employee:"<<emp.name<<endl;
cout<<"Hiring date: "<<emp.hiredate<<endl;
cout<<"Salary: "<<emp.salary<<endl;
}
Output:
The Employee Details Are-
Name of employee:Shivi
Hiring date: 31/04/2024
Salary: 80000
Explanation:
- We begin by defining a structure called employee, which contains three data members/ components that represent the details of an employee. They are-
- A character variable name to store the name of the employee, which can be up to 30 characters.
- Another character variable hiredate to store the date of hiring of the employee, which has a size limit of 30 characters.
- And a float-type variable salary to store the employee's salary.
- Then, inside the main() function, we create an instance of the Employee struct called emp and initialize it with specific values.
- Finally, we use cout statements to print the details of the employee, including their name, hiring date, and salary, to the console.
Union Data Types In C++
Unions are user-defined data types that allow programmers to store different data types in the same memory location. This means that since they share the memory, you can access only one element/ member of the union at a given point in time. Also, the size of the union is dependent on the largest member attribute in terms of memory usage.
Syntax:
union UnionName {
dataType1 member1;
dataType2 member2;
// ... additional members
};
Here,
- The union keyword indicates that we are defining a variable/ element of union data type whose name is given by UnionName.
- The terms member and dataType refer to the name of the data variable and its data type, respectively.
Below is an example that shows how unions are used in C++ programs.
Code Example:
#include<iostream>
using namespace std;
union Data{
int i;
float f;
double d;
};
int main(){
Data var1,var2,var3;
var1.i=10;
var2.f=3.14;
var3.d=8.76837;
cout<<"Integer value:"<<var1.i<<endl;
cout<<"Float value:"<<var2.f<<endl;
cout<<"Double value:"<<var3.d<<endl;
}
Output:
Integer value:10
Float value:3.14
Double value:8.76837
Explanation:
We begin the sample C code by including the needed header file and using the namespace.
- We define a union named Data to represent a collection of different data types (int, float, double) sharing the same memory location.
- Then, inside the main() function, we declare 3 instances of the Data union, i.e., var1, var2, and var3.
- Next, we initialize these members of the union with values 10, 3.14, and 8.76837. Since all members share the same memory, assigning a value to one member may affect the value of another member.
- Finally, we use cout statements to print the values of the union members based on their respective data types.
- This demonstrates the use of a union, where different types of data can be stored in the same memory location.
Classes In C++
These are not the traditional C++ data types per se. Instead, classes are user-defined data types that consist of data members (variables) and member functions (functions) that can be accessed through objects. Objects are instances of the class and are used to access the data members and member functions of the class.
The concept of classes and objects is of an integral part of object-oriented programming that forms the basis for other OOPs concepts like inheritance in C++.
Syntax:
class ClassName {
private:
DataType1 member1;
//More data memebers
public:
ClassName();
//memberFunctions()};
Here,
- The class keyword indicates we are defining a class with the name ClassName.
- Next, the terms private and public are the access specifiers that control the accessibility of class members, i.e., who and from where can access the respective data members.
- The className() is the default class constructor which initializes the data members.
- The term memberFunction() refers to the functions/ methods that are a part of the class.
Below is an example to show how classes and objects work in C++.
Code Example:
#include<iostream>
using namespace std;
class Square {
public:
int side;
int area() {
return side * side;}
};
int main() {
Square s; // Creating an object of the Square class
s.side = 5; // Assigning a value to the data member 'side' using the object
cout << "Area of square of side 5 is " << s.area(); // Calling the member function 'area' using the object
}
Output:
Area of square of side 5 is 25
Explanation:
- We define a class named Square encapsulating data members (side of integer type) and a member function (area) that calculates and returns the square of the side.
- Then, in the main() function, we create an object s of the Square class and then use the dot operator to initialize the side data member with the value of 5.
- We then use a cout statement to print the area of the square by calling the area() function on the side variable.
Also Read- OOPs Concept In C++ | A Detailed Guide With Codes & Explanations
Typedef Data Types In C++
In C++, the typedef keyword is used to create an alias (alternate name) for existing data types. This can make code more readable and manageable, especially when dealing with complex or lengthy type declarations. The typedef keyword simplifies the process of defining custom names for various data types.
Here's the general syntax for using typedef:
typedef existing_type new_type_name;
Below is an example to illustrate the usage of typedef in C++.
Code Example:
#include<iostream>
using namespace std;
// Original type declaration
typedef int Integer;
int main() {
// Using the typedef alias
Integer myNumber = 42;
// Original type usage
int anotherNumber = 24;
cout << "Using typedef: " << myNumber << endl;
cout << "Without typedef: " << anotherNumber << endl;
return 0;
}
Output:
Using typedef: 42
Without typedef: 24
Explanation:
- We begin by declaring a new data type by using the typedef keyword.
- Integer becomes an alias for the existing type int.
- In the main() function, we declare a variable myNumber using the Integer alias.
- Then, we declare another variable, anotherNumber, using the original type int.
- Both variables, myNumber and anotherNumber, are essentially of the same type (int).
- The cout statements print the values of the two reference variables, demonstrating the use of typedef for code readability.
- The return 0 statement indicates successful program execution.
Abstract Data Types In C++
Abstract data types are defined by their behaviour rather than their implementation because they are not data types in the traditional sense but data structures. In other words, they are used to represent complex data structures such as linked lists, stacks, queues, and trees. Programmers define their own data types with specific behaviour and individual characters. Listed below are some of the most used abstract data types in C++.
Linked List In C++
It’s a data structure in which elements are stored in a sequence, and each element points to the next. The first element is called the head, and the last element points to null. Linked lists are useful for dynamic data structures where the size is not known beforehand.
Syntax:
struct Node {
int data;
Node* next;
};
class LinkedList {
private:
Node* head;
public:
LinkedList();
void insertFront(int value);
void display();
};
Here’s an example of the implementation of a linked list in C++.
Code Example:
#include<iostream>
using namespace std;
struct Node{
int data;
Node* next;
};
void printlist(Node* node){
while(node!=NULL){
cout<<node->data<<" ";
node = node->next;}
}
int main(){
Node* head=NULL;
Node* second=NULL;
Node* third=NULL;
head = new Node();
second = new Node();
third = new Node();
head->data=1;
head->next = second;
second->data=2;
second->next = third;
third->data=3;
third->next = NULL;
printlist(head);
}
Output:
1 2 3
Explanation:
- We begin by defining a structure named Node that represents a node in a linked list. Each node contains an integer data value and a pointer next to the next node in the list.
- Next, we define a function printlist that takes a pointer to the head of a linked list as a parameter and prints the elements of the list using a while loop.
- In the main() function, we declare three nodes (head, second, and third) using the Node structure. Memory is allocated for each node using the new keyword.
- We then assign values and pointers to each node to create a simple linked list: 1 -> 2 -> 3 -> NULL.
- Then, we call the printlist() function with the head of the list as an argument to print the elements of the linked list.
Stacks Data Types In C++
This is a data structure where elements are stacked one on top of the other. They follow a last-in-first-out (LIFO) order, meaning, its elements are inserted and removed from the top end. Stacks are useful in situations where the most recently added element is the most important. For example, function calls are stored in a call stack and popped off the stack after the function returns control.
Syntax:
#include<stack>
int main() {
std::stack<int> myStack;
myStack.push(1);
myStack.pop();
return 0;
}
Here’s an example of a basic implementation of a stack.
Code Example:
#include<iostream>
#include<stack>
using namespace std;
int main(){
stack<int>s;
s.push(1);
s.push(2);
s.push(3);
while(!s.empty()){
cout<<s.top()<<endl;
s.pop();}
}
Output:
3
2
1
Explanation:
- In the main() function, we declare a stack of integers named s using the stack template class.
- We then use the push() function to push the values 1, 2, and 3 onto the top of the stack.
- Next, we create a while loop to iterate through the elements in the stack. It first checks if the stack is not empty using the logical NOT operator and the empty() function.
- If the condition is false, i.e., the stack is not empty, the loop prints the top element using the cout statement and removes it from the stack using the pop() function.
- If the condition is true, i.e., the stack is empty, then the loop terminates.
Queues Data Types In C++
It is a linear data structure that follows a first-in-first-out (FIFO) order, where elements are inserted at the rear end and elements are removed from the front end. Queues are useful in situations where a group of elements need to be processed in the order they were received.
Syntax:
#include<queue>
int main() {
std::queue<int> myQueue;
myQueue.push(1);
myQueue.pop();
return 0;
}
Here’s an example of how queues work in C++.
Code Example:
#include<iostream>
#include<queue>
using namespace std;
int main(){
queue<int>q;
q.push(1);
q.push(2);
q.push(3);
while(!q.empty()){
cout<<q.front()<<endl;
q.pop();
}
}
Output:
1
2
3
Explanation:
- The code includes two header files: <iostream> for input and output operations and <queue> for using the queue data structure.
- In the main() function, we declare a queue of integers named q using the queue template class.
- We then use the push() function to push the values 1, 2, and 3 onto the back of the queue.
- Next, we create a while loop to iterate through the elements in the queue as long as it is not empty (i.e., !q.empty()).
- Inside the loop, the front element of the queue is printed using cout statements.
- After that, we use the pop() method to remove the front element from the queue.
- The loop continues until the queue becomes empty, printing the front element at each iteration.
Trees Data Structure/ Data Type In C++
It is a data structure that consists of nodes connected by edges. Each node can have multiple child nodes, except for the leaf nodes, which have no children. Trees are useful in situations where hierarchical relationships need to be represented.
Syntax:
struct TreeNode {
int data;
TreeNode* left;
TreeNode* right;
};
TreeNode* createSampleTree();
int main() {
TreeNode* myTree = createSampleTree();
return 0;
}
Given below is an implementation of the tree in C++.
Code Example:
#include<iostream>
using namespace std;
struct Node {
int data;
Node* left;
Node* right;
};
void printTree(Node* node){
if(node==NULL)
return;
cout<<node->data<<endl;
printTree(node->left);
printTree(node->right);}
int main(){
Node* root=NULL;
Node* leftChild=NULL;
Node* rightChild=NULL;
root = new Node();
leftChild = new Node();
rightChild = new Node();
root->data=1;
root->left=leftChild;
root->right=rightChild;
leftChild->data=2;
leftChild->left=NULL;
leftChild->right=NULL;
rightChild->data=3;
rightChild->left=NULL;
rightChild->right=NULL;
printTree(root);
}
Output:
1
2
3
Explanation:
- We define a structure named Node representing a node in a binary tree. Each node contains an integer data value and pointers to its left and right children.
- Next, we define a recursive function, printTree, that traverses a binary tree in a pre-order fashion and prints the data of each node.
- In the main() function, we create a simple binary tree with three nodes, i.e., root, left child, and right child.
- Next, we assign integer values to each node in the tree (1 for the root, 2 for the left child, and 3 for the right child).
- Then, we call the printTree function to traverse and print the binary tree in pre-order.
Data Type Modifiers In C++
In C++, data type modifiers are keywords that modify the properties of basic data types or fine-tune the characteristics to better suit the requirements of your program. Here are some commonly used data type modifiers in C++:
- Signed: This is the default modifier for integer types. It allows both positive and negative values. E.g., signed int temperature = -10.
- Unsigned: This modifier is used when only non-negative values are needed. It effectively doubles the positive range. E.g., unsigned int count = 100.
- Short: It reduces the size of an integer, allocating less memory. It is typically used when memory efficiency is crucial. E.g., short int smallNumber = 32767.
- Long: It increases the size of an integer, providing a larger range of values. E.g., long int bigNumber = 1234567890.
- Float: Represents single-precision floating-point numbers. E.g., float myFloat = 3.14159f.
- Double: Represents double-precision floating-point numbers (larger range and precision compared to float). E.g., double myDouble = 3.14159265358979.
- Long Double: Represents extended precision floating-point numbers. E.g., long double myLongDouble = 3.14159265358979323846L.
Declaring Variables With Auto Keyword
In C++, the auto keyword is used for type inference, allowing the compiler to automatically deduce the data type of a variable based on its initializer. This feature was introduced in C++11 and is part of modern C++ programming. Using auto can simplify code, especially when dealing with complex or lengthy type names.
The Syntax to Declare Variables Using the auto Keyword:
auto variable_name = initial_value;
Here, as must have noticed we have used the auto keyword instead of the data type. This signals the compiler to look at the initial_value and on basis of that decide/ assign the data type of the variable known as variable_name.
Code Example:
#include<iostream>
#include<vector>
using namespace std;
int main() {
// Using auto with primitive type
auto x = 42; // Compiler deduces x is of type int
// Using auto with a more complex type (vector)
vector<int> numbers = {1, 2, 3, 4, 5};
auto iterator = numbers.begin(); // Compiler deduces iterator is of type vector<int>::iterator
// Using auto with a lambda function
auto add = [](int a, int b) { return a + b; }; // Compiler deduces add is of type a lambda function
cout << "x: " << x << endl;
cout << "First element: " << *iterator << endl;
cout << "Sum: " << add(3, 5) << endl;
return 0;
}
Output:
x: 42
First element: 1
Sum: 8
Explanation:
We begin by including the header file for input and output operations: #include<iostream> and the <vector> header for using the vector container.
- Inside the main() function, we then declare a variable x with the auto keyword and initialize it with the value 42. The compiler deduces that x is of type int.
- Next, we declare an integer vector called numbers and initialize it.
- Then, we declare a variable iterator and initialize it with the iterator pointing to the beginning of a vector. The compiler deduces that the iterator is of type vector.
- We then declare a variable add and initialize it with the lambda() function, i.e., auto add = [](int a, int b) { return a + b; }. The compiler deduces the type of add based on the lambda function's signature.
- Lastly, we use cout statements to print the values of x, the first element of the vector, and the result of the lambda function.
Conclusion
C++ offers a wide range of data types to choose from, including primitive, derived, user-defined, and abstract data types. It also provides data type modifiers to specify additional constraints about the behaviour of variables and pointers in C++ programs. Understanding the various data types in C++ and their uses is crucial for effective and efficient programming. Selecting the appropriate data type for a given problem can help optimize the code for both memory usage and runtime efficiency. A solid understanding of data types in C++ is an essential component of becoming a proficient C++ programmer.
Also read- 51 C++ Interview Questions For Freshers & Experienced (With Answers)
Frequently Asked Questions
Q. What is the difference between primitive and derived data types in C++?
Primitive data types are the fundamental data types in C++, such as int, char, float, double, bool, etc. In comparison, derived data types are built on top of primitive data types, such as arrays and pointers.
Q. What are abstract data types in C++?
Abstract data types (ADT) are data types that are defined on the basis of the behavior they exhibit and not on the basis of their implementation. ADTs don't specify the implementation of the data structure but rather the actions performed on it. Examples of abstract data types in C++ are linked lists, trees, stacks, and queues.
Q. What is the size of integer data types in C++?
The correct size of an integer data type in C++ varies depending on the compiler and architecture being used. They vary from 4 bytes to 8 bytes. However, in general, they are 4 bytes.
Q. What is the difference between signed and unsigned data types in C++?
Signed data types can hold both positive and negative values, so their C++ range is from -2147483648 to 2147483647. In comparison, unsigned data types can only hold non-negative values, and their range is from 0 to 4294967295.
Q. What are user-defined data types in C++?
User-defined data types are custom-defined data types implemented by the programmer to create more complex data structures than those provided by primitive data types, such as structures, classes, enumerations, and unions. They are created using one or more primitive data types.
Q. What are pointers in C++?
A pointer is a derived data type that stores the memory address of another variable. It allows us to indirectly access a variable by manipulating the address stored in the pointer. They are often used to dynamically allocate memory at run-time and are instrumental in implementing abstract data types such as linked lists, trees, stacks, queues, etc.
Q. What are classes in C++?
Classes are user-defined data types that contain data members and member functions that operate on the data members. Objects are instances of a class and are used to access the data members and member functions of the class.
Q. What are data type modifiers in C++?
Data type modifiers are used to modify the behavior or properties of the basic data types in C++. The C++ language provides data type modifiers to specify additional constraints about the behavior of variables and pointers in C++ programs.
Q. What is the difference between a stack and a queue in C++?
Stacks are last in, first out(LIFO) data structures, while queues are first in, first out(FIFO) data structures. Stacks are used for expression evaluation and function call management. Queues are used for tasks like process scheduling and data buffering.
Q. What is the importance of data types in C++?
There are a wide range of data types to choose from, including primitive, derived, user-defined, and abstract data types. It is important for one to understand these different data types in C++ and to write effective and efficient code.
Test Your Skills: Quiz Time
You might also be interested in reading the following:
- The 'this' Pointer In C++ | Declaration, Constness, Applications & More!
- C++ Type Conversion & Type Casting Demystified (With Examples)
- Storage Classes In C++ & Its Types Explained (With Examples)
- Pointer To Object In C++ | Simplified Explanation & Examples!
- Array Of Objects In C++ | A Complete Guide To Creation (Using Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Ujjwal Sharma 2 weeks ago
Kartik Deshmukh 4 weeks ago
Ishita Pahuja 1 month ago
Ruchita Kamble Kamble 1 month ago
Himanshu Soni 1 month ago
Muskan Kela 1 month ago