Table of content:
- What is Reference?
- What is Pointer?
- Comparison Table Of C++ Pointer Vs. Reference
- Differences Between Reference And Pointer: A Detailed Explanation
- Why Are References Less Powerful Than Pointers?
- Conclusion
- Frequently Asked Questions
Difference Between Pointer And Reference In C++ (With Examples)
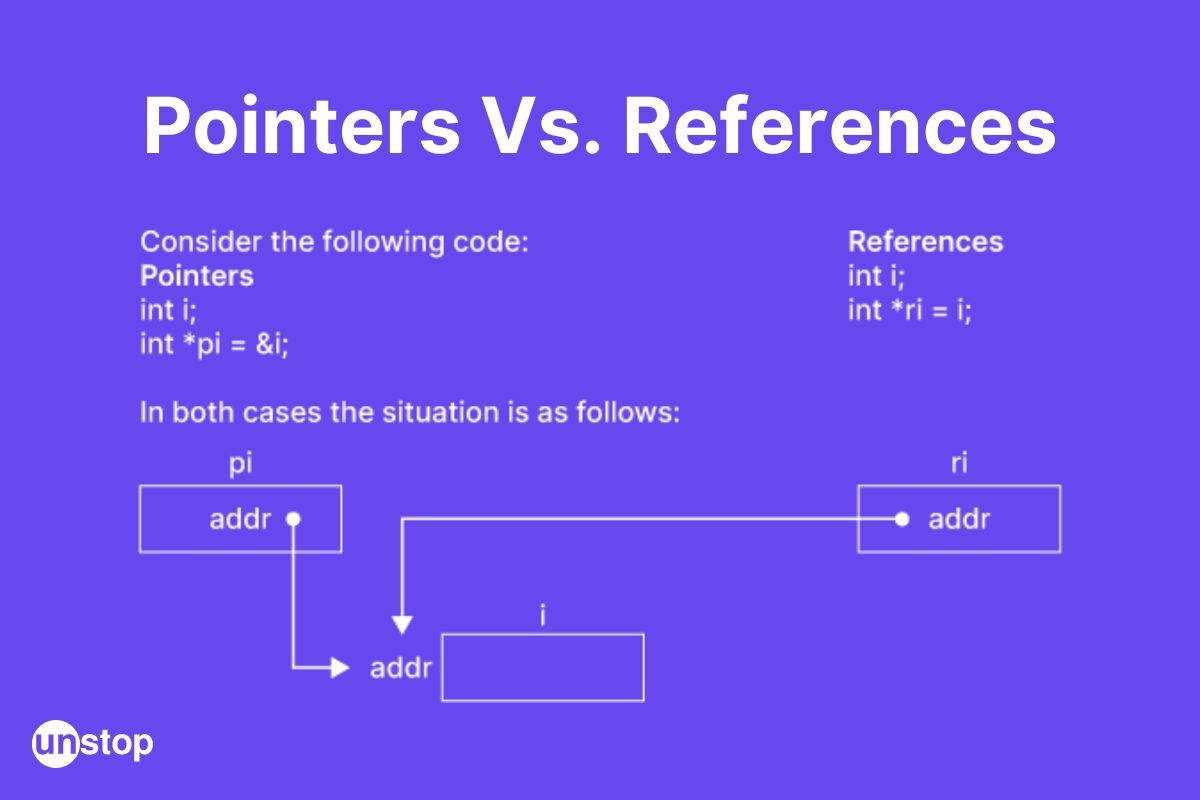
References and pointers are two very important concepts for those who want to write efficient, correct code. In this article, we will discuss the difference between pointer and reference in C++ in complete detail to help you understand the nuances and write better codes. But first, let's take a look at the basics of the concepts themselves.
What is Reference?
A reference is an alias for an already existing variable. After the reference variable is initialized, it is possible to use a reference name to refer to that variable. Reference in C++ is created by storing the address of another variable. A reference variable can be considered a constant pointer with automatic indirection, i.e., the compiler will apply an indirection operator (*) for you. The declaration of a reference variable contains the base type followed by the ‘&’ sign and then the variable name.
The Syntax for Reference in C++
<data-type> &<reference-variable-name> = <existing variable of same type>
In the syntax above, the data type on the right side should be the same as the assignment operator. For example-
int &a = i; //for reference we use & sign
Code Example
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluKCl7CgppbnQgdGVtcCA9IDEwOyAvL2luaXRpYWxpemluZyB0ZW1wIG5hbWVkIHZhcmlhYmxlCgppbnQgJmEgPSB0ZW1wOyAvL2NyZWF0aW5nIGEgcmVmZXJlbmNlIHZhcmlhYmxlIHRvIGl0wqAKCmNvdXQ8PCJUaGUgdmFsdWUgb2YgdGVtcCBpczoiPDxhOwoKcmV0dXJuIDA7Cgp9
Output:
The value of temp is: 10;
Explanation:
- We started by declaring the header file, then moved to the main function.
- In the main function, we first declare an int variable temp and assign it a value of 10.
- Then we declared a reference to temp and named it 'a'.
- In the next line, when we print the value of 'a', it shows the value of the variable where it is referencing.
What is Pointer?
A pointer is a programming language object that stores the address of another variable. In other words, it stores the direct address of the memory location. A pointer should also be declared before it is used, unlike other variables. It can be dereferenced with the help of the '*' operator to access the memory location to which the pointer points.
The size of the int pointer is 4 bytes. (which can be determined using the operation sizeof())
The syntax for pointer in C++
The declaration of the pointer variable contains the base data type followed by the ‘*’ sign. For example,
int *a; //
Here we use a '*' operator for defining a pointer
Code Example
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdXNpbmcgbmFtZXNwYWNlIHN0ZDsKCmludCBtYWluKCl7CgppbnQgdGVtcCA9IDEwOyAvL2luaXRpYWxpemluZyB0ZW1wIG5hbWVkIHZhcmlhYmxlCgppbnQgKnB0ciA9ICZ0ZW1wOyAvL2Fzc2lnbmluZyB0aGUgYWRkcmVzcyB0byBwb2ludGVyIHB0csKgCgpjb3V0PDwiVGhlIHZhbHVlIG9mIHRlbXAgaXM6Ijw8YTsKCnJldHVybiAwOwoKfQ==
Output:
The value of temp is: 10;
Explanation:
In the C++ program above,
- We started by declaring the header file, then move to the main function.
- In the main function, we first declare an int variable temp and assign it a value of 10.
- Then we create a pointer variable 'ptr', which stores/points to the address of the temp variable (i.e., we have used &temp, which indicates the address of the temp variable).
- In the next line, when we print the value of 'ptr', it shows the value of the variable where it is pointing.
To know about the history and timeline of C++ read- History Of C++ | Detailed Explanation (With Timeline Infographic)
Comparison Table Of C++ Pointer Vs. Reference
Reference | Pointers |
Reference must have a data type. |
A pointer can have a void pointer also. |
The reference variable can not be reassigned. |
The pointer variable can be reassigned. |
There is no need to dereference these. The variable name is simply used in case of reference in C++ |
We must deference a pointer. For this, the pointer '*' operator is used. |
References have to be initialized at the time of declaration only. |
Pointers can be initialized after declaration also. However, this sometimes makes wild pointers (i.e., uninitialized pointers that point to arbitrary memory locations). |
There cannot be multiple indirection levels in references. For example, a reference to a reference is not possible. |
Pointer allows multiple levels of indirection. For example, double pointer (which is pointer to pointer) |
The reference variable does not have its own address and shares the same address as the original variable. |
The pointer variable in C++ has its own address and also occupies space in the stack. |
Running any arithmetic operation on the reference will result in a compiler time error. |
Arithmetic operations such as addition, multiplication, and subtraction are possible on pointers. |
The reference variable is used to return the address of the address it is referring to. The address can be retrieved using the (&) operator. |
The pointer variable returns the value whose address it is pointing to. Value can be retrieved using the (*) operator. |
This variable is referenced by the method pass-by-value. |
The pointer does it work by the method known as pass-by-reference. |
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Differences Between Reference And Pointer: A Detailed Explanation
Some of the key differences between Pointers and references are given below-
1. Methos of Initialization
The initialization of a pointer in C++ is as given below:
//at the time of declaration only
int a = 10;
int *ptr = &a;
//after the declaration
int *ptr;
ptr = &a;
The way to initialize the reference variable is as shown below-
//here we have to initialize and declare at the same time only
int a = 10;
int &ref = a;
2. Functionality
A pointer holds the address of the variable to which it is pointing to, while a reference variable is an alias for a variable that already exists.
3. Operator
The pointer operator, i.e., the operator used to declare the pointer is the star operator (*), while the reference operator is an ampersand, i.e., (&).
Example for the pointer:
int a = 10;
int *ptr = &a; // use the * operator for declaring the pointer in C++
Example for reference:
int a = 10;
int &ref = a; //uses the & operator for reference
4. Null value
In C++, we can have a NULL pointer, which can be used in logical operations. However, we cannot have NULL references.
Code Example:
I2luY2x1ZGU8c3RkaW8uaD4KCnVzaW5nIG5hbWVzcGFjZSBzdGQ7CgppbnQgbWFpbigpewoKaW50ICpwdHIgPSBOVUxMOwoKY291dDw8IlRoZSB2YWx1ZSBvZiBOVUxMIFBvaW50ZXIgaXM6Ijw8cHRyOwoKcmV0dXJuIDA7Cgp9
Output:
The value of NULL pointer is: 0
Here we have created a NULL pointer in C++ 'ptr' and assigned it to NULL. When we print the value of NULL it shows '0'.
5. Memory Address
A pointer has its own memory address and size in the stack. In contrast, a reference does not have its own separate memory location. Instead, it shares with the variable memory only but takes some space on the stack.
Example:
int *ptr = a;
cout<<"Address of a pointer ptr:"<<&ptr<<endl;
cout<<"Address of variable where Pointer points:" << &a<endl;
Explanation:
The first line will print the address of the pointer, while the other line prints the address of the variable. You will observe that both addresses are different
6. Dereferencing
A pointer variable can be dereferenced with the help of the '*' operator. In contrast, the reference variable requires no operator for dereferencing. This is because it can be used like a normal variable.
7. Reassignments
Reassignment is the property of a variable to reassign its value again. A pointer can be re-assigned, i.e., a pointer can be re-assigned again to a different variable. This property of the pointer makes it useful in many data structures, such as a Linked list, trees, etc.
Code Example:
I2luY2x1ZGU8c3RkaW8uaD4KCnVzaW5nIG5hbWVzcGFjZSBzdGQ7CgppbnQgbWFpbigpewoKaW50IGEgPSAxMDsKCmludCAqcHRyID0gJmE7Cgpjb3V0PDwiVGhlIHZhbHVlIG9mIHBvaW50ZXIgaXM6IiA8PHB0cjsKCmludCBiID0gMjA7CgpwdHIgPSAmYjsgLy9yZS1hc3NpZ24gdmFsdWUKCmNvdXQ8PCJ0aGUgdmFsdWUgb2YgcG9pbnRlciBpczoiPDxwdHI7CgpyZXR1cm4gMDsKCn0=
Output:
The value of pointer is: 10
The value of pointer is: 20
Explanation:
- Initially, we started the code by including the header file, and then we start the main function.
- We declare and initialize an int variable 'a' and assign it a value of 10.
- Next, a pointer is initialized and assigned to 'a'.
- Using the cout statement, we print the value of the pointer, which came out to be 10.
- Then again, we declare and initialize a different variable b, and assign a value of 20.
- Next, we create a pointer that points to b, and using the cout statement, we print the value of the pointer again, which comes out to be 20.
So we can see that we can re-assigned the value of the pointer. While on the other hand, references cannot be re-assigned. That is, it has to be assigned at the time of initialization only.
Example:
int a =10;
int &ref = a;
int b = 20;
int &ref = b; // this line will throw an error stating "Multiple declarations is not allowed"
The example above throws the error as the reassignment operation is not valid in references.
8. Arithmetic operations
Various arithmetic operations can be performed on the pointer, which is also known as pointer arithmetic. For example, increment/decrement, addition, subtraction, etc. But there are no such arithmetic operations in reference.
9. Indirection
You can have multiple levels of indirection in a pointer, such as a pointer to a pointer (known as a double pointer) and others. In contrast, references only offer one level of indirection.
Code Example for indirection in pointers:
I2luY2x1ZGU8c3RkaW8uaD4KdXNpbmcgbmFtZXNwYWNlIHN0ZDsKaW50IG1haW4oKXsKaW50IGEgPSAxMDsKaW50ICpwdHIgPSAmYTsKaW50ICoqcHRyMiA9ICZwdHI7CnJldHVybiAwOwp9
Code Explanation:
Here, we first declare a pointer name as ptr and assign it to a. Then, we declare a pointer to the pointer which is ptr2 which is pointing to ptr. This is known as the level of indirection.
10. Usage
Pointer variables should be used when the possibility of referring to nothing exists or when it is required to refer to different things at different times. In comparison, the usage of references is valid only when there is any object to refer to.
11. Application/ Use Cases
The concept of pointer needs to be understood completely before using the data structures such as linked lists, trees, etc.
A reference variable can be used in the function parameters, function return value, and return type to define user interfaces. In many cases, it can be used as an alternative to a pointer also.
Why Are References Less Powerful Than Pointers?
The reasons why a reference is not as powerful as a pointer are-
- NULL declaration: Reference cannot be NULL, unlike a pointer, which can have a NULL value. Null pointers indicate that they are pointing to nothing, which is helpful in many cases.
- Initialization: A reference variable has to be initialized and declared at the same time.
- Reassignment: Unlike pointers, reference variables cannot reassign to a new value after they have been declared.
Conclusion
In the end, we come to conclude that the pointer and reference are related to dynamic memory allocation in C++. The main difference between the both is that the pointer holds the address of another variable to which it is pointing to, while the reference is just an alias to access the existing variable.
Secondly, we can conclude that references are less powerful as compared to pointers but are easy and safer to use than pointers.
Frequently Asked Questions
Q. What is the most basic difference between a pointer and a reference?
Some of the most basic differences between the two are-
- Reference declaration and initialization must happen at the same time, while the pointer declaration and initialization can happen separately.
- Reference cannot be declared as NULL, while a pointer can be declared as NULL.
- Another important difference is reassignment. While a pointer value can be reassigned, the same does not hold true for a reference. That is, a reference cannot be reassigned, and it becomes fixed as soon as it is assigned to a variable.
Q. How can you reseat a reference to make it refer to a different object?
We cannot, in fact, reseat a reference to make it refer to a different object. Unlike a pointer, once a reference is bound to an object, it can not be “reseated” to another object. The reference isn’t a separate object, i.e., it has no identity. Taking the address of a reference gives you the address of the referent. Remember- the reference is its referent.
Q.Why does C++ have both pointers and references?
C++ inherited the concept of the pointer from C language without causing serious compatibility problems. In C++, we introduce a new concept of reference to support function overloading.
Q.When should I use references, and when should I use pointers?
References are generally preferred over pointers when you don't need to change the object being referred to. Still, there are some points to keep in mind-
Use references in C++:
- In function parameters and return type
Use pointer:
- In the implementation of the data structure, such as linked lists, trees, etc. This is because we might have to change the value of the pointer where it is pointing.
- If pointer arithmetic or passing a NULL pointer is needed. For example, for arrays.
We are sure that you must know the difference between pointer and reference by now. Here are a few more articles that you might be interested in reading:
- 51 C++ Interview Questions For Freshers & Experienced (With Answers)
- Typedef In C++ | Syntax, Application & How To Use It (With Examples)
- Difference Between C And C++| Features | Application & More!
- The 'this' Pointer In C++ | Declaration, Constness, Applications & More!
- Find In Strings C++ | Examples To Find Substrings, Character & More!
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment