- How To Convert String To List In Python? (List Of Methods)
- Using split() To Convert A String To A List In Python
- Using list() To Convert A String To A List In Python
- Using List Comprehension To Convert A String To A List
- Using map() To Convert A String To A List In Python
- Using ast.literal_eval() To Convert A String To A List In Python
- Using Regular Expressions To Convert A String To A List
- Using JSON Parsing To Convert A String To A List In Python
- Using String Slicing To Convert A String To A List In Python
- Using enumerate() to Convert a String to a List In Python
- Handling Edge Cases When Converting A String To A List In Python
- Performance Comparison Of Ways To Convert String To List In Python
- Conclusion
- Frequently Asked Questions
How To Convert String To List In Python? 9 Ways With Code Examples
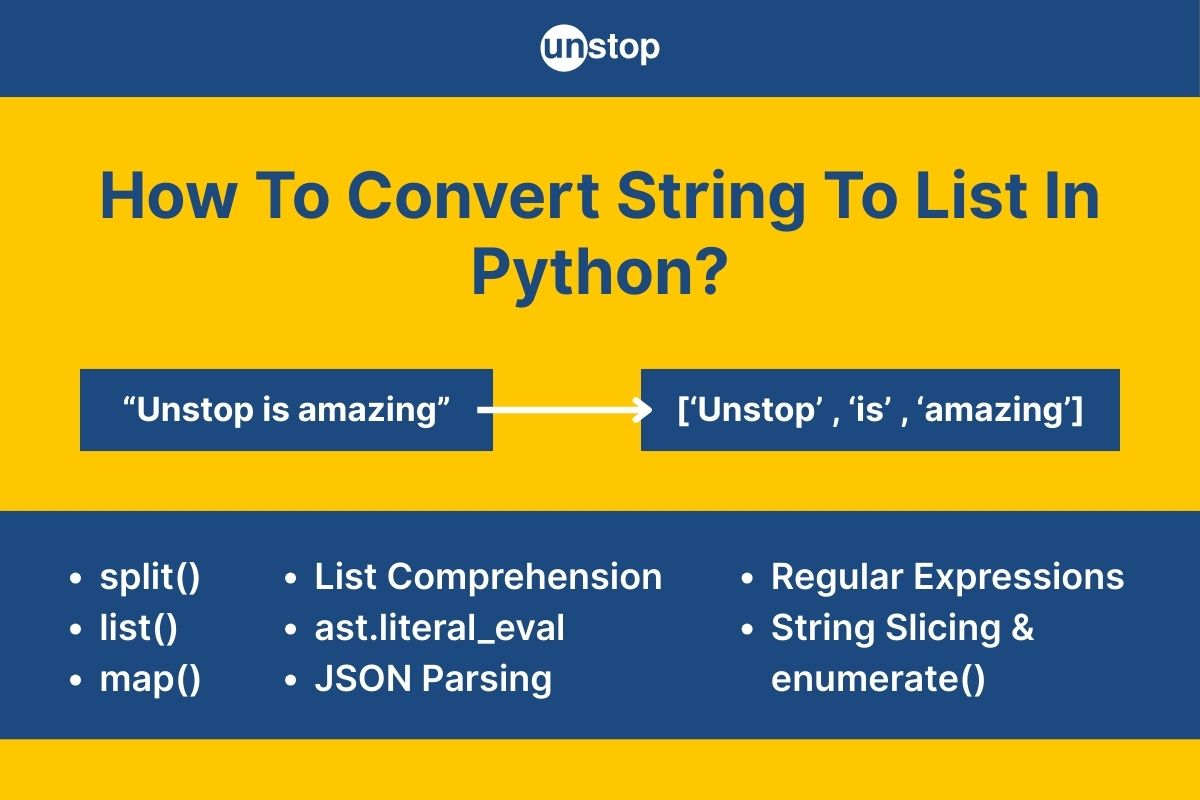
Converting a string into a list is a fundamental task in Python programming. Whether you're parsing data from an external source, processing user input, or transforming data for further analysis, being able to convert strings into lists unlocks a wide range of possibilities.
In this article, we will discuss how to convert string to list in Python using several techniques, starting from the most straightforward methods like split() and list() to more advanced approaches such as list comprehensions, map(), safe evaluation with ast.literal_eval, regular expressions, and JSON parsing. We'll also discuss practical use cases, performance implications, and common pitfalls of these methods of string to list conversion.
How To Convert String To List In Python? (List Of Methods)
Python language offers a variety of methods to transform a string into a list, each suited to different needs:
- split(): This method breaks a string into a list of substrings based on a specified delimiter (defaulting to whitespace). It’s ideal for splitting sentences into words or comma-separated values into individual elements.
- list(): The built-in Python function list() converts a string directly into a list of characters. This is a straightforward approach when you need to work with individual characters.
- List Comprehension: They allow you to iterate over a string and create a list based on specific conditions or transformations for more customized conversion logic.
- map(): Similar to list comprehension, map() applies a given function to every element in the string, which can then be collected into a list.
- ast.literal_eval: When the string represents a Python literal (like a list written as a string), ast.literal_eval safely evaluates it into the actual list structure.
- Regular Expressions: For strings in Python programs with non-uniform delimiters or more complex patterns, regular expressions provide a powerful way to extract list elements.
- JSON Parsing: If your string is formatted as a JSON array, json.loads() can convert it directly into a list.
- String Slicing and enumerate(): In some specialized cases, slicing or using enumerate() might be useful–especially when precise control over indexes or characters is needed.
In the upcoming sections, we will explain each of these Python list functions and techniques with detailed code examples and practical insights so you can choose the one that best fits your specific scenario.
Using split() To Convert String To List In Python
The split() method is one of Python’s simplest and most efficient ways to convert a string into a list. It works by dividing the string at each occurrence of a specified delimiter and returning a list of substrings. If no delimiter is provided, split() defaults to using any whitespace (spaces, tabs, or newlines) as the separator.
How It Works:
- Default Behavior: When called without any arguments, split() removes any leading or trailing whitespace and then splits the string into words wherever it finds one or more whitespace characters.
- Custom Delimiters: By specifying a delimiter (for example, a comma or hyphen), you can control how the string is split. This is particularly useful when dealing with CSV data or any structured text.
Code Examples To Convert String To List Using split() In Python
Code Example–Splitting a Sentence into Words:
#Creating a string
text = "Python makes string manipulation simple."
#Using split to break sentence basis whitespaces
words = text.split()
print(words)
Output:
['Python', 'makes', 'string', 'manipulation', 'simple.']
Code Explanation:
We begin the Python example program by creating a variable text and assigning a string value “Python makes string manipulation simple” to it.
- Then, we call the function split() on the text string and store the outcome in the variable words.
- The function splits the string into individual words after the occurrence of whitespace and returns a list of strings.
- Finally, we use the print() function in Python to display the outcome to the console.
Code Example–Splitting a Comma-Separated String:
#Creating cvs data type string
csv_data = "apple,banana,cherry,dragonfruit"
#Using split() to convert it into a list, with the comma as a delimiter
fruits = csv_data.split(',')
print(fruits)
Output:
['apple', 'banana', 'cherry', 'dragonfruit']
Code Explanation:
Here, the delimiter is a comma, so split(',') separates the string wherever it finds a comma.
Code Example–Handling Extra Whitespace:
messy_text = " hello world "
words = messy_text.split()
print(words)
Output:
['hello', 'world']
Code Explanation:
Even though there are extra spaces at the beginning, end, and between words, the default behavior of split() removes these extra whitespaces and returns only the meaningful words.
Quick Knowledge Check!
Using list() To Convert String To List In Python
The built-in list() function converts any iterable into a list. When applied to a string, it treats the string as a sequence of characters and returns a list where each element is one character from the original string.
How It Works:
The list() function iterates over the string, retrieving each character in order, and collects them into a new list. This means every character, including spaces, punctuation, or any special symbols, becomes a separate element in the resulting list.
Code Example:
text = "Python"
chars = list(text)
print(chars)
Output:
['P', 'y', 't', 'h', 'o', 'n']
Code Explanation:
In the simple Python program example, we begin by creating a variable text and assigning it the string "Python".
- Next, we call list(text), which iterates through each character in text and collects them into a new list.
- The resulting list, stored in the variable chars, is then printed to the console, showing each character as an individual element.
Also read: Python IDLE | The Ultimate Beginner's Guide With Images & Codes!
Quick Knowledge Check!
Using List Comprehension To Convert String To List
List comprehension provides a concise way to create lists by iterating over an iterable, in this case, a string. When you use list comprehension with a string, it goes through each character and builds a new list from the results.
How It Works: The list comprehension iterates over every character in the string and collects each one into a list. This method is particularly flexible, allowing you to apply conditions or transformations to each element if needed.
Code Example:
text = "Python"
chars = [char for char in text]
print(chars)
Output:
['P', 'y', 't', 'h', 'o', 'n']
Code Explanation:
In the basic Python program example, we begin by creating a variable text and assigning it the string "Python".
- Next, we use a list comprehension to iterate over each character in text, with the expression simply returning the character itself.
- The resulting list, stored in the variable chars, contains each character from the string as a separate element, which we then print to the console.
Quick Knowledge Check!
Using map() To Convert String To List In Python
The map() function applies a specified function to each element of an iterable, in this case, a string. It returns a map object that can be converted into a list.
How It Works: The map() takes two parameters: a function and an iterable.
- It applies the function to every element of the iterable.
- Here, we use a simple lambda function that returns each character as-is.
Code Example:
text = "Python"
# Apply a lambda function that returns each character
chars = list(map(lambda x: x, text))
print(chars)
Output:
['P', 'y', 't', 'h', 'o', 'n']
Code Explanation:
We start by creating a variable text and assign it the string "Python".
- Next, we call map() with a lambda function that returns each character unchanged and pass the text string as the iterable.
- The map() function applies this lambda function to every character in the string, producing a map object.
- We then convert this map object into a list using the list() function, storing the result in chars.
- Finally, printing chars outputs the list of individual characters from the original string.
Looking for guidance? Find the perfect mentor from select experienced coding & software development experts here.
Using ast.literal_eval() To Convert String To List In Python
The ast.literal_eval() function safely evaluates a string containing a Python literal (like a list, tuple, or dict) and converts it into the corresponding Python data structure.
How It Works: The ast.literal_eval() parses the string and evaluates it as a Python literal. Unlike eval(), it only allows a restricted set of safe literals, which makes it a secure option when converting strings to data structures.
Code Example:
import ast
string_list = "[1, 2, 3, 4, 5]"
list_result = ast.literal_eval(string_list)
print(list_result)
Output:
[1, 2, 3, 4, 5]
Code Explanation:
We begin by importing the ast module in Python.
- Next, we create a variable string_list that holds a string representing a list.
- We then pass this string to ast.literal_eval(), which safely evaluates the string and converts it into a Python list.
- The resulting list is stored in the variable list_result and printed to the console.
Using Regular Expressions To Convert String To List
Regular expressions offer a powerful way to extract specific patterns from a string. This method is particularly useful when you need to extract only certain elements–like numbers–from a string that may contain other characters.
How It Works: A regular expression searches the string for patterns (for example, sequences of digits).
- The re.findall() function returns all non-overlapping matches as a list of strings.
- If needed, you can further process these strings (such as converting them to integers).
Code Example:
import re
string_list = "[1, 2, 3, 4, 5]"
parts = re.findall(r'\d+', string_list)
list_result = [int(x) for x in parts]
print(list_result)
Output:
[1, 2, 3, 4, 5]
Code Explanation:
We start by importing the re module from the Python library, which provides support for regular expressions.
- Then, we create variable string_list containing a string representing a list of numbers.
- Next, we use re.findall(r'\d+', string_list) to search for one or more consecutive digits in the string.
- This returns a list of strings (e.g., ['1', '2', '3', '4', '5']).
- We then use a list comprehension to convert each string in this list to an integer, storing the final list in list_result.
- Finally, we print list_result to display the converted list.
Also read: Convert Int To String In Python | Learn 6 Methods With Examples
Using JSON Parsing To Convert String To List In Python
JSON parsing offers a straightforward way to convert a string formatted as a JSON array into a Python list. This method is particularly useful when working with data exchanged between systems, as JSON is a common format for data serialization.
How It Works: The json.loads() function parses the JSON-formatted string and converts it into the corresponding Python data structure, in this case, a list.
Code Example:
import json
string_list = '["apple", "banana", "cherry"]'
list_result = json.loads(string_list)
print(list_result)
Output:
['apple', 'banana', 'cherry']
Code Explanation:
We start by importing the json module and then create a variable string_list containing a JSON-formatted string representing a list.
- We then call json.loads(string_list), which parses the string and converts it into a Python list.
- The resulting list is stored in the variable list_result, which is printed to the console.
Using String Slicing To Convert String To List In Python
String slicing allows you to extract specific parts of a string. When combined with list comprehension, slicing can convert a string into a list of substrings based on a defined interval.
How It Works:
The slicing operation text[start:end] extracts a portion of the string from the start index up to, but not including, the end index. By iterating over the string with an appropriate step, you can create a list where each element is a substring (for example, a single character or a fixed-length group of characters).
Code Example (Extracting Individual Characters):
text = "Python"
#Using list comprehension with slicing
chars = [text[i:i+1] for i in range(len(text))]
print(chars)
Output:
['P', 'y', 't', 'h', 'o', 'n']
Code Explanation:
We begin by defining the variable text with the string "Python".
- Then, we use a list comprehension that iterates over each index i in the range of the string's length.
- The slicing text[i:i+1] extracts one character at a time.
- The resulting list, stored in variable chars, containing each individual character from the original string, which is printed to the console.
Code Example (Extracting Substrings of Fixed Length):
text = "PythonProgramming"
# Extract substrings of 2 characters each
substrings = [text[i:i+2] for i in range(0, len(text), 2)]
print(substrings)
Output:
['Py', 'th', 'on', 'Pr', 'og', 'ra', 'mm', 'in', 'g']
Explanation:
Here, we use slicing with a step of 2 in the list comprehension.
- For each iteration, text[i:i+2] extracts a substring of two characters.
- The resulting list, substrings, contains pairs of characters from the original string, which is then printed to the console.
Also read: Python List Slice Technique | Syntax & Use Cases (+Code Examples)
Using enumerate() To Convert String To List In Python
The enumerate() function returns an iterator that produces pairs of each element’s index and the element itself from the given string. While it’s typically used when you need both the index and the value, it can also be used in a list comprehension to create a list of characters.
How It Works: The enumerate() function iterates over the string and returns a tuple (index, character) for each character. In our example, we use a list comprehension to extract only the character from each tuple.
Code Example:
text = "Python"
result = [char for idx, char in enumerate(text)]
print(result)
Output:
['P', 'y', 't', 'h', 'o', 'n']
Code Explanation:
We start by assigning the string "Python" to the variable text.
- Then, using enumerate(text), we iterate over each character along with its index.
- In the list comprehension, we ignore the index (idx) and collect only the character (char).
- This results in a list of individual characters, which is then printed to the console.
Handling Edge Cases When Converting A String To A List In Python
When converting strings to lists, several edge cases may arise that could lead to unexpected behavior or errors. It’s important to consider these cases and handle them appropriately.
Common Edge Cases
- Empty Strings: An empty string ("") should ideally return an empty list. Some methods (like split()) handle this gracefully, while others might require additional checks.
- Strings with Only Delimiters or Whitespace: If a string contains only delimiters or whitespace, the resulting list might include empty strings. It’s useful to filter these out if they’re not needed.
- Irregular Formatting: Strings that have inconsistent delimiters, extra spaces, or misplaced punctuation may not split into the expected elements. In these cases, preprocessing the string (e.g., using strip()) can help.
- Invalid Literal Representations: When using methods like ast.literal_eval(), ensure that the string accurately represents a valid Python literal to avoid exceptions.
Example: Handling An Empty String & Filtering Out Empty Elements
def safe_split(text, delimiter=None):
# If the text is empty, return an empty list
if not text:
return []
# Split the text using the provided delimiter (or default whitespace)
parts = text.split(delimiter)
# Filter out any empty strings that may occur due to extra delimiters or spaces
return [part for part in parts if part]
# Examples
print(safe_split(""))
print(safe_split(" "))
print(safe_split("apple,,banana,,cherry", ","))
Output:
[]
[]
['apple', 'banana', 'cherry']
Code Explanation:
In the example, we define a function named safe_split() to handle empty strings/elements. Inside:
- We first use an if-statement to check if the input text is empty, in which case we return an empty list immediately.
- If the input is not empty then we use the split() function on text with the specified delimiter (or default whitespace if none is provided).
- Finally, we use a list comprehension to filter out any empty strings from the resulting list.
- In the main part, we test this function by calling it with variety of inputs and printing the results.
- As shown in the output, this approach helps ensure that your conversion handles edge cases gracefully.
- You can do the same in cases where you take user input in Python programs.
Ready to upskill your Python game? Dive deeper into actual problem statements and elevate your coding game with hands-on practice!
Performance Comparison Of Ways To Convert String To List In Python
Below is a comparison table summarizing the key performance aspects and readability of each conversion method. Note that for most typical use cases, these methods operate in O(n) time complexity, where n is the length of the string. However, factors like overhead, internal operations, and the specific use case can affect real-world performance.
Method |
Time Complexity |
Memory Usage |
Readability |
split() |
O(n) |
Low |
High |
list() |
O(n) |
Low |
High |
List Comprehension |
O(n) |
Low |
High |
map() |
O(n) |
Low |
Medium |
ast.literal_eval() |
O(n) |
Medium |
Medium (for literals) |
Regular Expressions |
O(n)–O(n²)* |
Medium |
Low (complex regex) |
json.loads() |
O(n) |
Low |
High (for JSON data) |
String Slicing |
O(n) |
Low |
Medium |
enumerate() |
O(n) |
Low |
High (when indexing) |
Note:
- Regular expressions can have variable performance based on pattern complexity and the string’s structure.
- For small strings, these differences are usually negligible. For larger datasets, selecting the most efficient method based on your specific requirements can make a noticeable difference.
This table provides a high-level overview. In practice, performance testing with your actual data is recommended to choose the optimal method for your scenario.
Check out this amazing course to become the best version of Python programmer you can be.
Conclusion
Converting a string to a list is a common and versatile operation in Python, with multiple methods available to suit various needs.
- You can break a sentence into words using split() or obtain a list of individual characters with list().
- Alternatively, you can apply custom logic via list comprehensions or map(), safely evaluate literal representations using ast.literal_eval(), or extract data with regular expressions or JSON parsing.
- By understanding how each approach works and the trade-offs in terms of readability and performance, you can choose the optimal technique for your project.
- Remember to consider potential edge cases, such as empty strings or inconsistent formatting, and test your code with real data to ensure it behaves as expected.
In summary, mastering these conversion techniques will not only simplify your text-processing tasks but also help you write more efficient, readable, and robust Python code. Happy coding!
Frequently Asked Questions
Q1: What are the most common methods to convert a string to a list in Python?
The most frequently used methods include using split() for delimiter-based splitting, list() for converting a string into a list of characters, list comprehensions for custom conversions, and json.loads() or ast.literal_eval() for strings that represent literal Python data structures.
Q2: When should I use split() versus list() or list comprehension?
Use split() when you need to break a string into substrings based on a delimiter (or whitespace by default). Use list() when you need a simple list of individual characters. List comprehensions are best when you require additional processing or filtering while converting the string.
Q3: Are there any security concerns with using eval() for conversion?
Yes. Although eval() can convert strings into Python objects, it executes the string as code, which can be dangerous if the input is not trusted. Prefer ast.literal_eval() for safely evaluating literals.
Q4: How do I handle edge cases, like empty strings or strings with only delimiters?
You can address edge cases by checking if the input string is empty before conversion and by filtering out any empty elements after splitting. For example, a helper function can verify that the string isn’t empty and remove any unwanted empty strings from the result.
Q5: Which method is most efficient for large strings?
Efficiency depends on the use case. For large, well-formatted strings, using split() or list() is typically fast and memory-efficient. For more complex cases (e.g., with irregular delimiters), you might need regular expressions or JSON parsing, though these can introduce extra overhead. Profiling with your actual data is recommended for the best choice.
- 40+ Python String Methods Explained | List, Syntax & Code Examples
- Find Length Of List In Python | 8 Ways (+Examples) & Analysis
- How To Convert Python List To String? 8 Ways Explained (+Examples)
- Find Area Of Triangle In Python In 8 Ways (Explained With Examples)
- Remove Item From Python List | 5 Ways & Comparison (+Code Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
ARUN MISHRA 1 week ago
Mulagala Sravani 1 week ago
Sounak Nandi 1 week ago
Sunita Bhat 1 week ago
Anshika Kumari 1 week ago