- How To Take Input In Java?
- The Scanner Class | How To Take Input In Java
- How To Take Input In Java Using BufferedReader
- How To Take Input In Java Using The Console Class
- Comparison Of Different Ways To Take Input In Java
- Conclusion
- Frequently Asked Questions
How To Take Input In Java | 3 Methods Explained (+Code Examples)
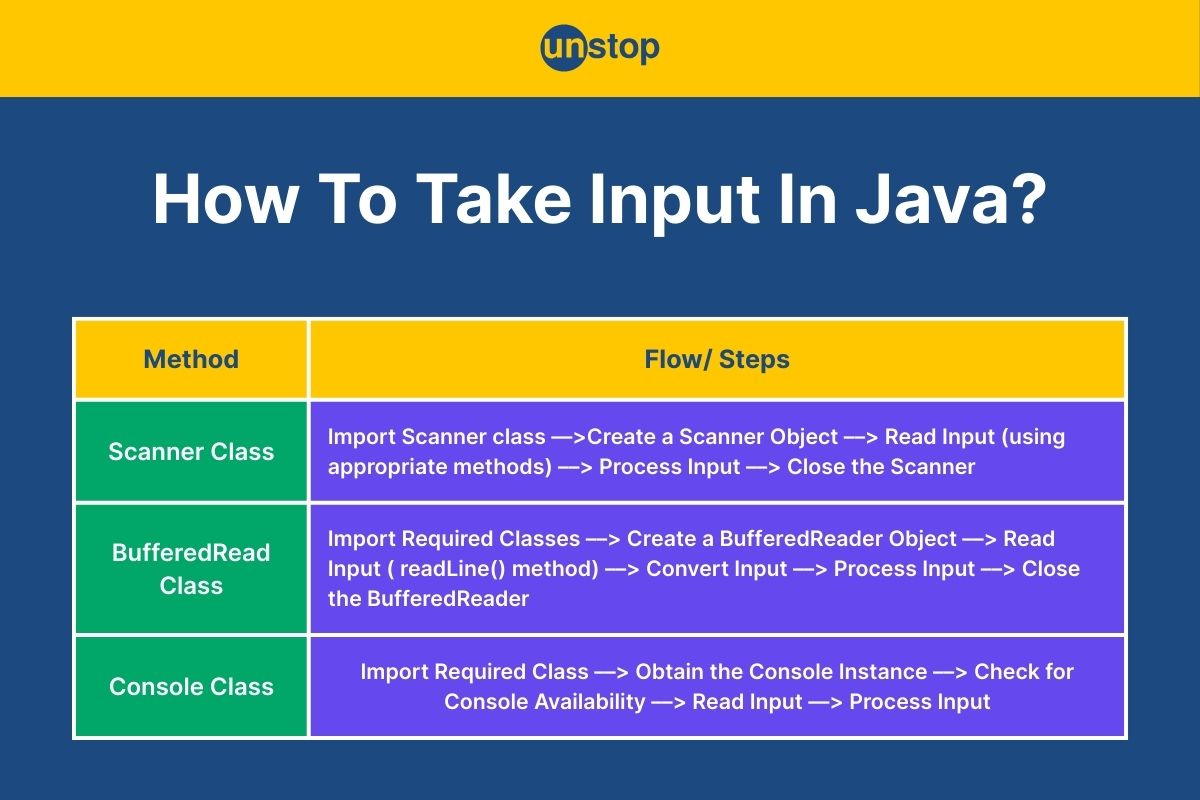
Java is not just a language for building backend systems or mobile apps, it’s also a go-to tool for creating interactive, user-driven programs. Whether you’re developing a simple command-line tool or a complex graphical application, handling user input is essential.
In this article, we’ll explore how to take input in Java by walking you through various methods and explaining their unique advantages and limitations.
How To Take Input In Java?
At its core, taking user input in Java programming transforms static programs into dynamic, interactive experiences. By reading input from the user, your program can react to real-time data, making it adaptable and more user-friendly.
Hence, it is essential for you to know how to take input in Java programs. There are several methods for this, each designed for different scenarios:
- The Scanner Class: A part of the java.util package, the Scanner class is one of the most popular and beginner-friendly ways to take input in Java. It is widely used for parsing primitive types and strings from various input sources, including user input from the console. Its ease of use and flexibility make it a favorite among new and experienced developers alike.
- BufferedReader: Found in the java.io package, BufferedReader reads text from an input stream efficiently, making it suitable for handling large inputs. In other words, BufferedReader, when used in combination with an InputStreamReader (from the java.io package), offers a more traditional way to read input.
- The Console Class: This class provides a simple interface for console input and output. It’s particularly useful for scenarios like password entry (using readPassword()), as it disables echoing on the screen. However, note that it might not work in every IDE environment.
Each of these methods has its own set of trade-offs. We will discuss them in greater detail in the following sections, discussing how each of these methods to take input in Java works with examples and discuss the best practices for using them in your projects.
The Scanner Class | How To Take Input In Java
The Scanner class (from the java.util package) is a versatile and easy-to-use tool for reading user input in Java. It converts raw input from the standard input stream (System.in) into tokens and primitive types using regular expressions.
It offers a straightforward and flexible way to read user input in Java. It parses primitive types and strings using regular expressions and is particularly well-suited for console-based applications.
How To Take Input In Java Using Scanner?
Let’s break down the steps to take user input in Java programming language using this class.
- Import the Class: Begin by importing java.util.Scanner to gain access to the Scanner class.
- Create a Scanner Object: Instantiate a Scanner object by wrapping System.in the standard input stream.
- Read Input: Use the appreciate methods such as nextInt(), nextLine(), or nextDouble() to take the input, depending on the data type. We will discuss the various methods later.
- Process Input: Optionally process or validate the input as required.
- Close the Scanner: It's good practice to close the scanner to free up resources once input is no longer needed. For this, you must call the method scanner.close() to release the underlying resources once input is complete.
Examples Of How To Take Input In Java Programs Using Scanner Class (Single & Multiple)
Example 1– Reading a Single Input: In the simple Java program example below, we have demonstrated how to take a single input using the Scanner class.
Code Example:
import java.util.Scanner;
public class SingleInputExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in); // Create scanner object
System.out.print("Enter your age: ");
int age = scanner.nextInt(); // Read an integer input
System.out.println("Your age is: " + age);
scanner.close(); // Close the scanner
}
}
Output:
Enter your age: 25
Your age is: 25
Code Explanation:
We begin the Java code example by importing the java.util.Scanner package to get access to the Scanner class and its methods to take input.
- Then we define a SingleInputExample class and then define the main() method, which serves as the program's entry point.
- Inside main(), we create an object of the Scanner class with System.in as its source, linking it to the standard input (typically the keyboard).
- We then use the System.out.print() method to display a prompt asking the user to enter their age.
- When the user inputs a number, we use the nextInt() method from the Scanner class to read the input. This method converts the token into an integer, which we store in the integer variable age.
- Next, we use the System.out.println() method to display the age along with a message, connecting them using string concatenation.
- Finally, we call the method close() on the Scanner object to release the system resources.
Example 2– Reading Multiple Inputs: The example Java program below illustrates how to take multiple user inputs using the class.
Code Example:
import java.util.Scanner;
public class MultipleInputExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in); // Create scanner object
System.out.print("Enter your name: ");
String name = scanner.nextLine(); // Read a string input
System.out.print("Enter your salary: ");
double salary = scanner.nextDouble(); // Read a double input
System.out.println("Name: " + name + ", Salary: " + salary);
scanner.close(); // Close the scanner
}
}
Output:
Enter your name: Amita
Enter your salary: 50000.0
Name: Amita, Salary: 50000.0
Code Explanation:
As in the previous example, we start by importing java.util.Scanner, defining the MultipleInputExample class and creating the main() method.
- Inside main(), we create a Scanner object linked to System.in.
- We then prompt the user to enter their name using System.out.print().
- Then, we use the nextLine() method to read the entire line of input, which we store in the variable name.
- Next, we prompt the user again to enter their salary. This time, we use the nextDouble() method to read and convert the input into a double value, storing it in the variable salary.
- We display both the name and the salary using the System.out.println() method.
- Finally, as before, we call the close() method on the Scanner object to free up the system resources.
Scanner Methods To Take Various Types Of Input In Java
The table below lists all the key Scanner methods used to take input in Java, along with their purpose and the type of value each returns.
Method |
Description |
Return Type |
next() |
Reads the next complete token (word) |
String |
nextLine() |
Reads the rest of the current line |
String |
nextInt() |
Scans the next token as an integer |
int |
nextDouble() |
Scans the next token as a double |
double |
nextFloat() |
Scans the next token as a float |
float |
nextBoolean() |
Scans the next token as a boolean |
boolean |
nextLong() |
Scans the next token as a long |
long |
nextShort() |
Scans the next token as a short |
short |
nextByte() |
Scans the next token as a byte |
byte |
hasNext() |
Returns true if there is another token available |
boolean |
hasNextLine() |
Returns true if there is another line of input |
boolean |
hasNextInt() |
Returns true if the next token can be interpreted as an int |
boolean |
hasNextDouble() |
Returns true if the next token can be interpreted as a double |
boolean |
useDelimiter(String regex) |
Sets the delimiter pattern for splitting input tokens |
Scanner |
Notes:
- Methods prefixed with hasNext (or hasNextInt, etc.) are useful for checking whether there is more input available before attempting to read it.
- The useDelimiter method allows you to customize how the input is tokenized, which can be particularly useful when dealing with non-standard input formats.
Advantages and Disadvantages of Using Scanner
Advantages |
Disadvantages |
|
|
This section provides a comprehensive overview of the Scanner class, showcasing its ease of use through detailed code examples, a breakdown of its methods, and a balanced look at its pros and cons.
Want to learn all about Java programming? Check out this amazing course and fast-track your journey to becoming a Java developer.
How To Take Input In Java Using BufferedReader
BufferedReader is a class in the java.io package that reads text from a character input stream, buffering characters for efficient reading. Although not as widely recommended for simple user input as the Scanner class, BufferedReader remains useful, especially in scenarios requiring high performance or when working with legacy code.
It's particularly useful for reading large files or when you need to process input line by line. Unlike Scanner, BufferedReader reads input only as strings, so you must manually convert the input to other data types as needed (e.g., using Integer.parseInt() for integers).
Steps to Take Input Using BufferedReader
Let’s break down the steps to take user input in Java using BufferedReader.
- Import Required Classes: Import java.io.BufferedReader, java.io.InputStreamReader, and java.io.IOException to handle input and potential exceptions.
- Create a BufferedReader Object: Instantiate a BufferedReader object by wrapping an InputStreamReader(System.in) around the standard input stream.
- Read Input: Use the readLine() method to read the input from the user as a String.
- Convert Input: Convert the string input to the desired data type using methods like Integer.parseInt() or Double.parseDouble() if needed.
- Process Input: Optionally process or validate the input as required.
- Close the BufferedReader: It's good practice to close the BufferedReader to free up resources after completing the input operations. For this, you must use the reader.close() method once input is complete.
Examples Of How To Take Input In Java Programs Using BufferedReader (Single & Multiple)
Example 1: Reading a Single Input using BufferedReader
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
public class SingleInputExampleBuffered {
public static void main(String[] args) throws IOException {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
System.out.print("Enter your age: ");
int age = Integer.parseInt(reader.readLine());
System.out.println("Your age is: " + age);
reader.close();
}
}
Output:
Enter your age: 29
Your age is: 29
Code Explanation:
We begin by importing the required packages: BufferedReader, InputStreamReader, and IOException.
- Then, we define the SingleInputExampleBuffered class and the main() method, which throws IOException to handle any potential input/output errors.
- Inside main(), we create a BufferedReader object by wrapping an InputStreamReader around System.in, thereby linking it to the standard input.
- Next, we prompt the user to enter their age using System.out.print().
- Then, we use the readLine() method to read the entire line of input as a string.
- We then convert this string to an integer using Integer.parseInt() and store the result in the variable age.
- Next, we display the age using System.out.println(), concatenating the string message with the integer value.
- Finally, we close the BufferedReader using reader.close() to free the associated system resources.
Example 2: Reading Multiple Inputs using BufferedReader
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
public class MultipleInputExampleBuffered {
public static void main(String[] args) throws IOException {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
System.out.print("Enter your name: ");
String name = reader.readLine();
System.out.print("Enter your salary: ");
double salary = Double.parseDouble(reader.readLine());
System.out.println("Name: " + name + ", Salary: " + salary);
reader.close();
}
}
Output:
Enter your name: Aashi
Enter your salary: 65000
Name: Aashi, Salary: 65000.0
Code Explanation:
As in the previous example, we start by importing the necessary packages and defining the MultipleInputExampleBuffered class along with the main() method (declared with throws IOException).
- Inside main(), we create a BufferedReader object connected to System.in.
- We then prompt the user to enter their name using System.out.print().
- Then, we capture the entire input line as a string using the readLine() method and store it in the variable name.
- Next, we prompt the user for their salary. We read this input as a string using readLine() and then converted it to a double using Double.parseDouble(). Then, we store the value in the variable salary.
- After that, we display the captured name and salary using System.out.println(), concatenating them into formatted output.
- Finally, we close the BufferedReader with reader.close() to release system resources.
Common Methods To Take Input In Java Using BufferedReader
The BufferedReader class in Java provides several methods to facilitate efficient reading of text from character-input streams. Below is a table summarizing some of its commonly used methods:
Method |
Description |
read() |
Reads a single character and returns it as an integer. Returns -1 at the end of the stream. |
readLine() |
Reads a line of text and returns it as a String. Returns null at the end of the stream. |
ready() |
Checks if the stream is ready to be read. Returns a boolean. |
mark(int limit) |
Marks the present position in the stream, allowing a subsequent call to reset() to return to this position. |
reset() |
Resets the stream to the most recent mark. |
skip(long n) |
Skips n characters in the stream. |
close() |
Closes the stream and releases any system resources associated with it. |
Note: The mark() and reset() methods are useful for scenarios where you need to revisit a specific point in the stream.
Advantages & Disadvantages Of Taking Input In Java Using BufferedReader
Advantages |
Disadvantages |
|
|
In summary, BufferedReader is a powerful tool for efficient reading of text data in Java, particularly suited for handling large volumes of input. However, developers should be mindful of the need for manual parsing and exception handling.
Top the leaderboard while polishing your coding skills now. How? Participate in the 100-Day Coding Sprint at Unstop.
How To Take Input In Java Using The Console Class
The Console class, introduced in Java 6, provides methods to access the character-based console device associated with the current Java virtual machine. It's particularly useful for reading input and writing output in a console-based application. One of its key features is the ability to read passwords securely without echoing the characters to the console.
Steps To Take Input Using The Console Class
- Import Required Class: Import java.io.Console to utilize the Console class.
- Obtain the Console Instance: Use System.console() to retrieve the unique Console instance associated with the JVM.
- Check for Console Availability: Ensure that the console is available; System.console() returns null if no console is present.
- Read Input: Utilize methods like readLine() for reading string input or readPassword() for reading password input without echoing characters.
- Process Input: Convert or validate the input as required for your application.
Example: Reading User Credentials Using the Console Class
import java.io.Console;
public class ConsoleInputExample {
public static void main(String[] args) {
// Obtain the Console instance
Console console = System.console();
// Check if console is available
if (console != null) {
// Read username
String username = console.readLine("Enter your username: ");
// Read password without echoing
char[] passwordArray = console.readPassword("Enter your password: ");
String password = new String(passwordArray);
// Display the entered information
console.printf("Username: %s%n", username);
console.printf("Password: %s%n", password);
} else {
System.out.println("No console available");
}
}
}
Output:
Enter your username: Aakash
Enter your password:Username: Aakash
Password: ******
(Here, ****** represents the hidden input; the actual password will not be displayed on the screen.)
Code Explanation:
We begin by importing the Console class from the java.io package to access console-specific methods.
- Then, we define a class and the main() method. Within main(), we retrieve the console instance using System.console().
- We then verify if the console is available by checking if the retrieved console instance is not null. If it's null, we print "No console available" and terminate the program.
- If the console is available, we prompt the user to enter their username using console.readLine(), which reads a line of text from the user.
- We then prompt the user to enter their password using console.readPassword(). This method reads the password without echoing the characters to the console, enhancing security.
- The password is returned as a character array for security reasons. We convert this array to a String object for easier manipulation.
- Finally, we display the entered username and password using console.printf().
Note: You may not get the output as mentioned above. In other words, the System.console() method may return null in environments where a dedicated system console is not available, such as when running Java programs inside an IDE like Eclipse, IntelliJ, or VS Code. This is because these IDEs redirect standard input and output, preventing System.console() from accessing the system’s native console. As a result, calling console.readLine() or console.readPassword() in such environments can cause a NullPointerException.
What to do?
If you're using an IDE and find that System.console() is returning null, you have two options:
- Run the program in a terminal: Execute your Java program directly from the command line (e.g., Command Prompt, Terminal, or PowerShell). This ensures that the console instance is properly obtained.
- Use Scanner instead: The Scanner class works consistently across both IDEs and terminals, making it a more flexible alternative for reading user input.
Common Console Class Methods To Take Input In Java
The Console class offers several methods for interacting with the console:
Method |
Description |
readLine() |
Reads a single line of text from the console. |
readPassword() |
Reads a password or sensitive data without echoing the input. |
printf() |
Writes a formatted string to the console. |
format() |
An alias for printf(), writes a formatted string to the console. |
flush() |
Flushes the console output stream. |
writer() |
Retrieves the PrintWriter object associated with the console. |
reader() |
Retrieves the Reader object associated with the console. |
Advantages & Disadvantages Of Taking Input In Java Using Console Class
Advantages |
Disadvantages |
|
|
In summary, the Console class is a useful tool for console-based applications, especially when handling secure user input. However, developers should account for its limited availability in non-interactive environments and plan accordingly.
Comparison Of Different Ways To Take Input In Java
Choosing the right input method in Java depends on your use case. The table below compares Scanner, BufferedReader, and Console to help you decide which one best fits your needs.
Feature |
Scanner |
BufferedReader |
Console |
Package |
java.util |
java.io |
java.io |
Ease of Use |
Easy |
Moderate (requires type conversion) |
Moderate (limited availability) |
Reads Data As |
Different data types (nextInt(), nextDouble(), etc.) |
Strings (manual conversion needed) |
Strings (manual conversion needed) |
Handles Whitespace |
Yes, supports token-based reading |
Reads entire line as a string |
Reads entire line as a string |
Supports Password Input |
No |
No |
Yes (readPassword() hides input) |
Performance |
Slightly slower (due to parsing overhead) |
Faster for large inputs |
Depends on system console availability |
Best Use Case |
General-purpose input handling |
High-performance input processing |
Secure credential input |
Each method has its advantages and trade-offs, so understanding their strengths can help you write more efficient and user-friendly Java programs.
Feel like you need guidance to progress as a Java developer? You can now select an expert to be your mentor here.
Conclusion
Taking user input in Java is an essential skill for building interactive programs. Throughout this article, we explored how to take input in Java programs in different ways, including:
- For Most General Use Cases → Use Scanner
- Best for handling different data types effortlessly.
- Easy to use but slightly slower due to internal parsing.
- For Large Input or Performance-Critical Applications → Use BufferedReader
- Reads input more efficiently, especially for large text-based data.
- Requires manual parsing for non-string data types.
- For Secure Input (e.g., Passwords) → Use Console
- Only works in environments with a system console (won’t work in some IDEs).
- Offers readPassword() to safely handle sensitive input.
Choosing the right method to take input in Java depends on the use case. Scanner is ideal for most applications, BufferedReader is preferred for performance-critical cases, and Console is suitable for command-line utilities. By understanding these techniques, you can effectively manage user input in Java, ensuring seamless interaction between the user and your program.
Frequently Asked Questions
Q1. What is the best way to take user input in Java?
The Scanner class is the most commonly used method for taking user input in Java. It provides built-in methods to read different data types easily.
Q2. Why is BufferedReader used instead of Scanner?
BufferedReader is used when performance is a priority, especially for reading large amounts of input. Unlike Scanner, it reads input as a whole line and requires manual type conversion.
Q3. Why does System.console() return null?
System.console() returns null when the program is run in an environment that does not support a console, such as an IDE or non-interactive shell. It works properly when executed in a command-line interface.
Q4. Do I need to close Scanner or BufferedReader?
Yes, you should close Scanner and BufferedReader when input is no longer needed to free up system resources. However, avoid closing Scanner if it's reading from System.in, as it will close the input stream.
Q5. Can I take multiple inputs in a single line using Scanner?
Yes, you can take multiple inputs in a single line using Scanner by calling methods like nextInt(), nextDouble(), or next() consecutively. You can also use nextLine() and split() to handle multiple inputs in one go.
By now you must know all about how to take input in Java programs. Here are a few more topics you must explore:
- Operators In Java | 9 Types, Precedence & More (+ Code Examples)
- Java Keywords | List Of 54 Keywords With Description +Code Examples
- Control Statements In Java | Types & Applications (+Code Examples)
- Identifiers In Java | Types, Conventions, Errors & More (+Examples)
- Switch Statement In Java | Working, Uses & More (+Code Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Pranathi Nakkitla 1 month ago