Table of content:
- Why Find Length Of A String In Python?
- How To Find Length Of A String In Python
- The len() Function To Find Length Of A String In Python
- Find Python String Length Using For Loop & in Operator
- The join() & count() Method To Find Python String Length
- The str.count() Method To Find Length Of A String In Python
- The str.__len__() Method To Find Length Of A String In Python
- The enumerate() Method To Find Length Of A String In Python
- Using Recursion To Find Length Of A String In Python
- The sum() Method To Find Length Of A String In Python
- The collections.Counter() Method To Find Length Of A String In Python
- The sys.getsizeof() Method To Find Python String Length
- Handling Special Cases When Finding Python String Length
- Conclusion
- Frequently Asked Questions
Find Length Of A String In Python | 10 Ways With Code Examples
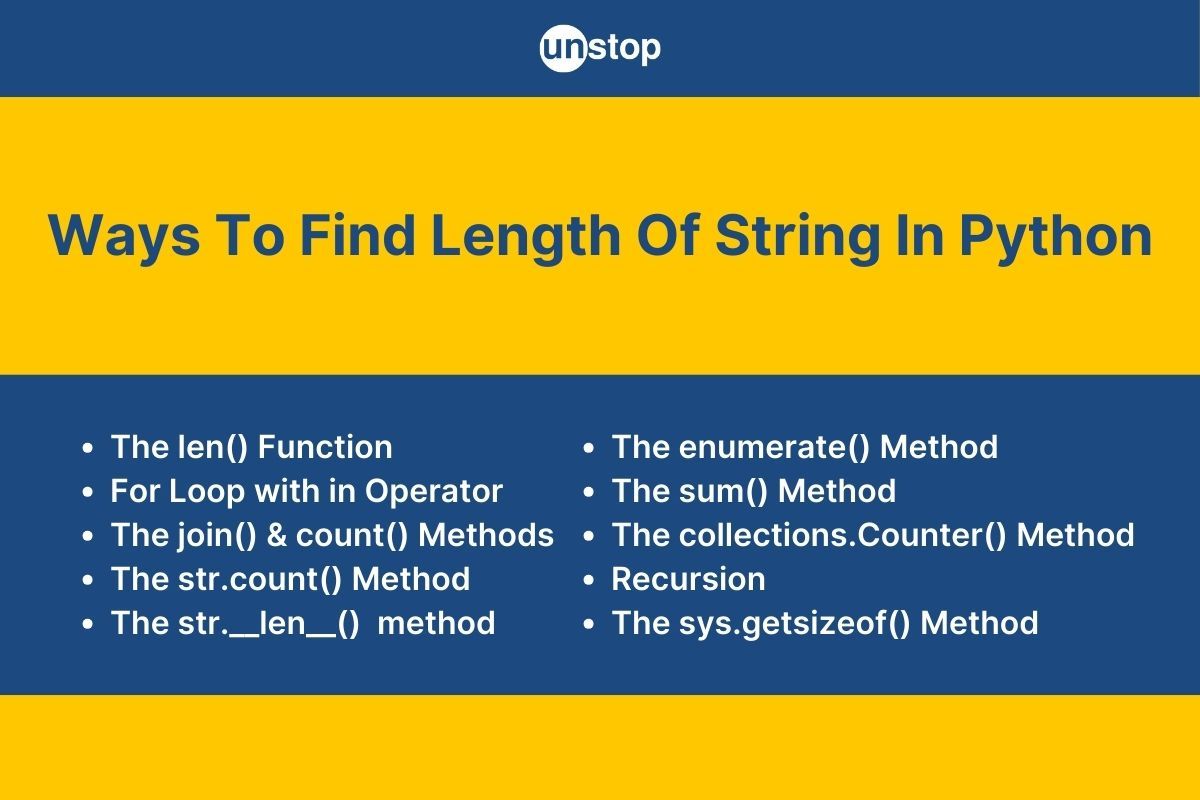
Strings are everywhere in Python, whether you're crafting a tweet, analyzing text data, or simply taking user input. But have you ever wondered how social media platforms limit your tweets to 280 characters? Behind the scenes, they rely on finding the length of your input string to enforce the limit. Finding the length of a string is also important in scenarios like limiting input size, processing text, or validating data.
In this article, we'll explore various ways to find the length of a string in Python, starting from the simplest built-in functions to creative alternatives, with examples. Let’s get started!
Why Find Length Of A String In Python?
The length of a string is simply the number of characters it contains, including spaces, punctuation, and special characters. Knowing the length of a string in Python programming is fundamental for many reasons, like:
- Validating Input Length: You often need to ensure the input is within a certain size limit, for example, in forms, passwords, or usernames.
- Text Processing: When analyzing text data, splitting strings, or extracting substrings, string length is often the starting point.
- Storage Constraints: APIs, databases, or file systems may impose restrictions on the size of the text being sent or stored.
- Truncation and Formatting: Whether for social media posts or UI elements, it's essential to truncate strings without cutting off critical parts.
- Automation and Parsing: Scripts that process or scrape data rely on the precise string length for slicing and data extraction.
In short, whether you're working on small scripts or building complex applications, finding string length is a foundational skill in Python programming that saves time and ensures accuracy.
How To Find Length Of A String In Python
Python provides multiple ways to find the length of a string, ranging from the straightforward len() function to more creative methods like loops or recursion. Whether you're a beginner or an advanced programmer, you'll find a method that suits your style or specific use case. Here’s a quick preview of the methods we’ll cover:
- Using the len() function
- Iterating with a for loop and the in operator
- Combining join() and count() methods
- Utilizing str.count()
- The str.__len__() method
- The enumerate() method
- Recursion
- The sys.getsizeof() method
Let’s dive into the most popular method first: the len() function.
Also read: Find Length Of List In Python | 8 Ways (+Examples) & Analysis
The len() Function To Find Length Of A String In Python
The len() function is the simplest and most widely used way to find the length of a string in Python. It is a built-in function that takes a string (or any iterable) as an argument and returns the total number of characters, including spaces and special characters, in that string.
How It Works: The len() function internally calls the string object's __len__() method, which is a built-in dunder (double underscore) method in Python.
- This method is implemented by all iterable objects, including strings, lists, and tuples, to return their length.
- When you pass a string to len(), it delegates the operation to str.__len__(), which efficiently counts and returns the number of characters in the string.
Syntax:
len(object)
Here,
- Parameter: The function takes one parameter, i.e., the object. It is the string or iterable whose length you want to determine.
- Return Value: The function returns an integer representing the total number of elements in the object.
The simple Python program example below illustrates how to use the len() function to find the length of a string.
Code Example:
IyBDcmVhdGluZyBhIHN0cmluZwpteV9zdHJpbmcgPSAiUHl0aG9uIGlzIGZ1biEiCgojIFVzaW5nIGxlbigpIHRvIGZpbmQgdGhlIGxlbmd0aCBvZiB0aGUgc3RyaW5nCmxlbmd0aCA9IGxlbihteV9zdHJpbmcpCgpwcmludCgiVGhlIGxlbmd0aCBvZiB0aGUgc3RyaW5nIGlzOiIsIGxlbmd0aCk=
Output:
The length of the string is: 14
Code Explanation:
In the simple Python code example:
- We create a variable my_string, which stores the string "Python is fun!".
- Then, we call the len() function, passing the string variable as an argument.
- The function calculates the total number of characters in the string, including spaces and the exclamation mark.
- We store the outcome in the length variable and display it to the console using the print() function.
The len() function is your go-to method for quickly and efficiently finding the length of any string in Python. Simple, isn’t it?
Find Python String Length Using For Loop & in Operator
Using a for loop along with the in operator is a manual approach to finding the length of a string. This method iterates through each character in the string and counts them one by one. Although not as straightforward as len(), it’s a useful exercise for understanding loops and iteration in Python. The basic Python program example below illustrates this approach to finding the length of a string.
Code Example:
I0NyZWF0aW5nIGEgc3RyaW5nIGFuZCBhIGxlbmd0aCB2YXJpYWJsZQpteV9zdHJpbmcgPSAiVW5zdG9wIGlzIGF3ZXNvbWUiCmxlbmd0aCA9IDAKCiNVc2luZyBmb3IgbG9vcCB0byBmaW5kIHRoZSBsZW5ndGggb2YgYSBzdHJpbmcKZm9yIGNoYXIgaW4gbXlfc3RyaW5nOgogIGxlbmd0aCArPSAxCgpwcmludCgiVGhlIGxlbmd0aCBvZiB0aGUgc3RyaW5nIGlzOiIsIGxlbmd0aCk=
Output:
The length of the string is: 18
Code Explanation:
In the basic Python code example:
- We define a variable my_string and store the value "Unstop is awesome" in it, and a counter variable length is initialized to 0.
- Then, we use a for loop to iterate through the string, counting every character in it.
- It goes through each character in the string, with char representing the current character during each iteration.
- In every iteration, we increment the length variable by 1 for each character encountered.
- After the loop is complete, length holds the total number of characters in the string, which we then print to the console.
This method is particularly helpful in scenarios where you need to process each character of the string while calculating its length.
Check out this amazing course to become the best version of the Python programmer you can be.
The join() & count() Method To Find Python String Length
Using the join() method along with the count() method is a more creative way to calculate the length of a string. The idea here is to join the string with a unique character and then count how many times that character appears. While this approach is a bit unconventional, it highlights Python’s flexibility in handling strings.
How It Works:
- The join() method combines the string into one unified string using an empty string ("") as the delimiter.
- The count() method is then used to count how many times the empty string appears, which effectively gives us the length of the string.
While this method is more complex than using len(), it demonstrates the power of Python’s string methods when combined. The Python program example below illustrates the same.
Code Example:
I0NyZWF0aW5nIGEgc3RyaW5nCm15X3N0cmluZyA9ICJVbnN0b3Agcm9ja3MhIgoKI1VzaW5nIGpvaW4oKSBhbmQgY291bnQoKSB0byBmaW5kIHRoZSBsZW5ndGggb2YgYSBzdHJpbmcKbGVuZ3RoID0gIiIuam9pbihteV9zdHJpbmcpLmNvdW50KCIiKQoKcHJpbnQoIlRoZSBsZW5ndGggb2YgdGhlIHN0cmluZyBpczoiLCBsZW5ndGgp
Output:
The length of the string is: 13
Code Explanation:
In the Python code example:
- We define a string variable my_string and assign the value “Unstop rocks!” to it.
- Then, we use the join() and count methods to calculate the length of this string.
- Here, the join() method is used with an empty string ("") to combine the string characters into one continuous sequence.
- The count() method counts the number of times the empty string appears, which equals the total number of characters in the original string.
- We finally print the outcome to the console, which we stored in the length variable.
The str.count() Method To Find Length Of A String In Python
The str.count() method can also be used to find the length of a string, though it's typically used for counting occurrences of a specific character. By counting the empty string (""), you can effectively determine the length of the string, as it counts all characters, including spaces and special characters.
Code Example:
I0NyZWF0aW5nIGEgc3RyaW5nCm15X3N0cmluZyA9ICJIZWxsbywgV29ybGQhIgoKI1VzaW5nIHN0ci5jb3VudCgpIHRvIGZpbmQgdGhlIGxlbmd0aCBvZiBhIHN0cmluZwpsZW5ndGggPSBteV9zdHJpbmcuY291bnQoIiIpIC0gMQoKcHJpbnQoIlRoZSBsZW5ndGggb2YgdGhlIHN0cmluZyBpczoiLCBsZW5ndGgp
Output:
The length of the string is: 13
Code Explanation:
In the example Python program:
- We define a string my_string and assign the value “Hello, World!” to it.
- Then, we call the count("") method on the string. It counts the occurrences of an empty string within the string.
- This count includes the characters, and Python returns one extra count, so we subtract 1 to get the correct length.
- We store the outcome in the variable length, i.e., 13, which we then print out.
The str.__len__() Method To Find Length Of A String In Python
The str.__len__() method is the internal dunder (double underscore) method that the len() function calls when determining the length of a string. While you can access it directly, it’s not commonly used in regular practice since the len() function in Python is more intuitive and convenient.
Code Example:
I0NyZWF0aW5nIGEgc3RyaW5nCm15X3N0cmluZyA9ICJQeXRob24gcHJvZ3JhbW1pbmchIgoKI1VzaW5nIHN0ci5fX2xlbl9fKCkgdG8gZmluZCB0aGUgbGVuZ3RoIG9mIGEgc3RyaW5nCmxlbmd0aCA9IG15X3N0cmluZy5fX2xlbl9fKCkKCnByaW50KCJUaGUgbGVuZ3RoIG9mIHRoZSBzdHJpbmcgaXM6IiwgbGVuZ3RoKQ==
Output:
The length of the string is: 19
Code Explanation:
In the example Python code:
- We create a string variable with the value “Python programming!”.
- Then, we call the __len__() method directly on the string object my_string. This is the internal method behind Python’s len() function.
- The function counts the characters in the string, and the outcome, i.e., 19, is stored in the length variable.
- Finally, we print it as the final output.
The enumerate() Method To Find Length Of A String In Python
The enumerate() method is primarily used for iterating over iterable objects like strings while keeping track of the index of each element. Though it’s not typically used to find string lengths, you can creatively combine it with a loop to count the number of iterations, which gives you the string's length. The sample Python program below illustrates how this works.
Code Example:
I0NyZWF0aW5nIHN0cmluZwpteV9zdHJpbmcgPSAiVW5zdG9wIHJvY2tzISIKCiNVc2luZyBlbnVtZXJhdGUoKSB0byBmaW5kIHRoZSBsZW5ndGggb2YgdGhlIHN0cmluZwpsZW5ndGggPSBzdW0oMSBmb3IgXyBpbiBlbnVtZXJhdGUobXlfc3RyaW5nKSkKCnByaW50KCJUaGUgbGVuZ3RoIG9mIHRoZSBzdHJpbmcgaXM6IiwgbGVuZ3RoKQ==
Output:
The length of the string is: 13
Code Explanation:
- We create a string object and then use the enumerate() function inside a loop to find its length.
- Here, the for loop iterates through the string, and the enumerate() function is used as a generator expression. It generates index-value pairs for each character in the string, but we don’t need the actual character, so we use an underscore (_) as a placeholder.
- After the loop iterations, the sum() function adds 1 for each iteration, counting how many characters are in the string.
- Finally, we print the total count, 13, as the length of the string.
Looking for guidance? Find the perfect mentor from select experienced coding & software development experts here.
Using Recursion To Find Length Of A String In Python
Recursion is a technique where a function calls itself to solve smaller instances of a problem until it reaches a base case. You can use recursion to find the length of a string by reducing the string one character at a time and counting the steps until the string is empty. The sample Python code below illustrates how to define a recursive function and then use it to find the length of a string.
Code Example:
IyBEZWZpbmluZyBhIGZ1bmN0aW9uIHRoYXQgdXNlcyByZWN1cnNpb24gdG8gZmluZCB0aGUgbGVuZ3RoIG9mIGEgc3RyaW5nCmRlZiBmaW5kX2xlbmd0aChzdHJpbmcpOgogICAgaWYgc3RyaW5nID09ICIiOiAjIEJhc2UgY2FzZTogZW1wdHkgc3RyaW5nCiAgICAgICAgcmV0dXJuIDAKICAgIHJldHVybiAxICsgZmluZF9sZW5ndGgoc3RyaW5nWzE6XSkgIyBSZWN1cnNpdmUgY2FsbAoKI0NyZWF0aW5nIGEgc3RyaW5nIGFuZCBjYWxsaW5nIHRoZSByZWN1cnNpdmUgZnVuY3Rpb24KbXlfc3RyaW5nID0gIlJlY3Vyc2l2ZSBsZW5ndGghIgpsZW5ndGggPSBmaW5kX2xlbmd0aChteV9zdHJpbmcpCgpwcmludCgiVGhlIGxlbmd0aCBvZiB0aGUgc3RyaW5nIGlzOiIsIGxlbmd0aCk=
Output:
The length of the string is: 17
Code Explanation:
- We first define a function called find_length that takes a string as input. Inside:
- We have the base case, where we check for empty strings using an if-statement.
- If the string is empty, the function returns 0, indicating the end of recursion.
- Then, we have the recursive case, which is implemented if the string is non-empty.
- In this case, the function adds 1 (for the first character) and makes a recursive call with the rest of the string using the slicing notation, i.e., string[1:].
- In the main part of the program, we create a string object, my_string, with the value “Recursive length”.
- Then, we call the recursive function on the string and store the outcome in the length variable.
- After the function completes all recursive calls, it returns the total count, 17, which we print to the console.
The sum() Method To Find Length Of A String In Python
Using the sum() function is a creative way to determine the length of a string. By iterating over the string and summing up a value (like 1) for each character, you can calculate the string's length without directly using len().
Code Example:
IyBDcmVhdGluZyBhIHN0cmluZyBhbmQgdXNpbmcgc3VtKCkgdG8gZmluZCBpdHMgbGVuZ3RoCm15X3N0cmluZyA9ICJTdW0gaXQgdXAhIgpsZW5ndGggPSBzdW0oMSBmb3IgXyBpbiBteV9zdHJpbmcpCgpwcmludCgiVGhlIGxlbmd0aCBvZiB0aGUgc3RyaW5nIGlzOiIsIGxlbmd0aCk=
Output:
The length of the string is: 10
Code Explanation:
In the Python code sample:
- We create a string object and call the sum() function on it.
- The sum() function iterates through the string using a generator expression (1 for _ in my_string), which produces a 1 for every character in the string.
- After the iterations, the sum() function adds up all the 1s generated, effectively counting the number of characters.
- We store the outcome, i.e., 10, in the variable length and print the string's length.
The collections.Counter() Method To Find Length Of A String In Python
The collections.Counter() class counts the occurrences of each character in a string. While its primary purpose is to count frequency, you can sum up the values of the character counts to calculate the total length of the string.
Code Example:
I0ltcG9ydGluZyBDb3VudGVyIGNsYXNzIGZyb20gY29sbGVjdGlvbnMgbW9kdWxlCmZyb20gY29sbGVjdGlvbnMgaW1wb3J0IENvdW50ZXIKCiNVc2luZyBjb2xsZWN0aW9ucy5Db3VudGVyKCkgdG8gZmluZCB0aGUgbGVuZ3RoIG9mIGEgc3RyaW5nCm15X3N0cmluZyA9ICJDb3VudCBtZSBpbiEiCmNoYXJfY291bnQgPSBDb3VudGVyKG15X3N0cmluZykKbGVuZ3RoID0gc3VtKGNoYXJfY291bnQudmFsdWVzKCkpCgpwcmludCgiVGhlIGxlbmd0aCBvZiB0aGUgc3RyaW5nIGlzOiIsIGxlbmd0aCk=
Output:
The length of the string is: 12
Code Explanation:
- We first import the Counter class from the collections module to access the Counter() method.
- The Counter class creates a dictionary-like object where keys are characters, and values are their frequencies in the string.
- Then, we create a string object, my_string, and call the Counter() method on it, storing the outcome in the char_count variable.
- After that, we use the values() method to retrieve the character frequencies, which we then sum using the sum() function to compute the total character count.
- The outcome of this operation is stored in the length variable, i.e., 12, which we then print as the string's length.
The sys.getsizeof() Method To Find Python String Length
The sys.getsizeof() method is typically used to get the memory size of an object in bytes, but it can be indirectly applied to measure the approximate length of a string. This method includes additional overhead for object metadata, so it’s not ideal for precise length calculations. The Python program sample below demonstrates how to use this function for string length.
Code Example:
I0ltcG9ydGluZyB0aGUgc3lzIG1vZHVsZQppbXBvcnQgc3lzCgojVXNpbmcgc3lzLmdldHNpemVvZigpIHRvIGZpbmQgdGhlIGxlbmd0aCBvZiBhIHN0cmluZwpteV9zdHJpbmcgPSAiUHl0aG9uIG1lbW9yeSEiCm1lbW9yeV9zaXplID0gc3lzLmdldHNpemVvZihteV9zdHJpbmcpCgpwcmludCgiVGhlIG1lbW9yeSBzaXplIG9mIHRoZSBzdHJpbmcgaW4gYnl0ZXMgaXM6IiwgbWVtb3J5X3NpemUp
Output:
The memory size of the string in bytes is: 65
Code Explanation:
- We begin by importing the sys module of Python library to access the getsizeof() method.
- Then, we create a string object and call the sys.getsizeof() method.
- The method calculates the memory size of my_string, including both the string data and Python's internal metadata.
- The size in bytes is returned and printed. Note that this includes additional memory overhead and does not represent the character count.
Handling Special Cases When Finding Python String Length
While finding the length of a string might seem straightforward, special cases can arise that impact accuracy or interpretation. Let’s look at some of these cases, their challenges, and how to handle them effectively.
1. Empty Strings
An empty string ("") has a length of 0. This scenario often occurs with uninitialized variables, user input, or placeholder values in data processing.
Why It’s Important:
- Ignoring empty strings in datasets can lead to errors, such as division by zero in averaging calculations.
- They can also indicate missing or invalid data, requiring special handling.
How To Handle: Always validate string inputs, especially in user-facing applications or data processing pipelines.
Code Example:
ZGVmIHByb2Nlc3Nfc3RyaW5nKGlucHV0X3N0cmluZyk6CiAgICBpZiBsZW4oaW5wdXRfc3RyaW5nKSA9PSAwOgogICAgICByZXR1cm4gIklucHV0IGlzIGVtcHR5ISIKICAgIHJldHVybiBmIlByb2Nlc3NlZCBzdHJpbmc6IHtpbnB1dF9zdHJpbmd9IgoKcHJpbnQocHJvY2Vzc19zdHJpbmcoIiIpKSAjIE91dHB1dDogSW5wdXQgaXMgZW1wdHkh
Code Explanation:
Here, we define a function that first checks if the string is empty using the len() to find the length and compare it to 0 using the relational operator. If the string is empty, the function returns a string message to that effect, allowing the program to run without raising an error.
2. Strings With Only Whitespace
Whitespace characters (spaces, tabs, newlines) are valid characters, but they can misrepresent the actual content of a string. In this context, strings that appear empty may consist solely of whitespace characters (e.g., spaces, tabs, newlines) and can be misleading. Hence, you must deal with whitespaces appropriately.
Why It’s Important:
- These strings can bypass basic empty string checks and cause validation errors.
- Whitespace-only strings might carry unintended data or formatting issues, especially in user inputs or text processing.
How To Handle: Use str.strip() to trim whitespace and compare the result to an empty string to identify true content.
Code Example:
ZGVmIHZhbGlkYXRlX2lucHV0KHVzZXJfaW5wdXQpOgogICAgdHJpbW1lZF9pbnB1dCA9IHVzZXJfaW5wdXQuc3RyaXAoKQogICAgaWYgbGVuKHRyaW1tZWRfaW5wdXQpID09IDA6CiAgICAgICAgcmV0dXJuICJJbnB1dCBpcyBvbmx5IHdoaXRlc3BhY2UhIgogICAgcmV0dXJuIGYiVmFsaWQgaW5wdXQ6IHt0cmltbWVkX2lucHV0fSIKCnByaW50KHZhbGlkYXRlX2lucHV0KCIgICAiKSkgIyBPdXRwdXQ6IElucHV0IGlzIG9ubHkgd2hpdGVzcGFjZSE=
Code Explanation:
The validate_input function uses .strip() to remove leading and trailing spaces before calculating the string’s length. This ensures that whitespace-only strings are correctly identified and processed.
3. Strings With Special Characters
Special characters include punctuation marks, symbols, and even emojis. Python treats these as single characters, but their byte representation varies. For example, emojis may occupy multiple bytes due to their Unicode representation. This calls for handling string length calculation appropriately.
Why It’s Important:
- Multibyte characters (e.g., emojis) might exceed length limits when stored in specific encodings.
- Applications with strict character limits (like tweets) may interpret lengths differently.
How To Handle: Use Python's unicodedata module or string encoding (str.encode()) to analyze byte lengths if needed.
Code Example:
I0ltcG9ydGluZyBtb2R1bGUKaW1wb3J0IHVuaWNvZGVkYXRhCgojU3RyaW5nIHdpdGggZW1vamkKc3BlY2lhbF9zdHJpbmcgPSAiSGVsbG8g8J+YiiEiCgpwcmludCgiQ2hhcmFjdGVyIGNvdW50OiIsIGxlbihzcGVjaWFsX3N0cmluZykpICMgT3V0cHV0OiA5CnByaW50KCJCeXRlIHNpemUgKFVURi04KToiLCBsZW4oc3BlY2lhbF9zdHJpbmcuZW5jb2RlKCd1dGYtOCcpKSkgIyBPdXRwdXQ6IDEy
Code Explanation:
The len() function counts visible characters, while the .encode('utf-8') method reveals the byte size of the string. This distinction is vital in encoding-sensitive applications.
4. Multiline Strings
Multiline strings (""" or ''') span multiple lines and contain visible characters as well as invisible newline characters (\n) in their length. You must accurately measure visible characters without counting newline characters when calculating the length of a string in Python.
Why It’s Important:
- Inconsistent newline handling can affect text analytics or visualization.
- Misinterpreted lengths can skew data processing, especially when extracting visible content.
How To Handle: Replace or strip newline characters when measuring text without formatting.
Code Example:
bXVsdGlsaW5lX3N0cmluZyA9ICIiIkhlbGxvLApXb3JsZCEiIiIKCnByaW50KCJMZW5ndGggd2l0aCBuZXdsaW5lczoiLCBsZW4obXVsdGlsaW5lX3N0cmluZykpICMgT3V0cHV0OiAxMwpwcmludCgiTGVuZ3RoIHdpdGhvdXQgbmV3bGluZXM6IiwgbGVuKG11bHRpbGluZV9zdHJpbmcucmVwbGFjZSgnXG4nLCAnJykpKSAjIE91dHB1dDogMTE=
Code Explanation:
Replacing newline characters ensures only visible characters are counted, making this approach suitable for analytics or display-focused applications.
5. Unicode Strings
Unicode characters are common in multilingual text and each character/symbol may occupy multiple bytes. But Python counts them as single characters when using len(), hence the need to adjust our length measuring mechanisms.
Why It’s Important:
- Misinterpreting lengths in multilingual text can result in incomplete processing or layout issues.
- For efficiency, applications must distinguish between character count and memory size.
How To Handle: Use encoding (str.encode()) to determine byte size or libraries like unicodedata for precise Unicode handling.
Code Example:
I0NyZWF0aW5nIGEgc3RyaW5nIGluIEhpbmRpIGxhbmdhdWdlCnVuaWNvZGVfc3RyaW5nID0gIuCkqOCkruCkuOCljeCkpOClhyIKCiNVc2luZyB0aGUgbGVuKCkgZnVuY3Rpb24gdG8gZ2V0IHRoZSBzdHJpbmcgbGVuZ3RoCnByaW50KCJDaGFyYWN0ZXIgY291bnQ6IiwgbGVuKHVuaWNvZGVfc3RyaW5nKSkgIyBPdXRwdXQ6IDYKCiNVc2luZyAuZW5jb2RlKCkgaW5zaWRlIGxlbigpIHRvIGdldCB0aGUgc3RyaW5nIGxlbmd0aApwcmludCgiQnl0ZSBzaXplIChVVEYtOCk6IiwgbGVuKHVuaWNvZGVfc3RyaW5nLmVuY29kZSgndXRmLTgnKSkpICMgT3V0cHV0OiAxOA==
Code Explanation:
The len() function counts characters, while .encode('utf-8') calculates the memory footprint, enabling proper handling of Unicode text in storage or transmission.
Ready to upskill your Python game? Dive deeper into actual problem statements and elevate your coding game with hands-on practice!
Conclusion
Understanding how to find the length of a string in Python is fundamental to effectively working with text-based data. In this article, we've explored various methods to calculate string length, each with its unique use cases and strengths:
- The len() function is the simplest and most efficient method for most situations.
- Alternative methods like using a for loop or str.count() offer more creative ways to calculate length in specific scenarios, though they may not be as efficient.
- Special cases, including empty strings, whitespace, special characters, and Unicode, require careful consideration to ensure that string length calculations remain accurate and meaningful.
By mastering these techniques and handling edge cases properly, you’ll be better equipped to handle string data in real-world Python applications, ensuring accurate data processing, user input validation, and robust functionality across various platforms.
Frequently Asked Questions
Q1. Does len() work on strings in Python?
Yes, the len() function in Python is specifically designed to work with strings. It returns the number of characters in a string, including letters, digits, spaces, and special characters.
Code Example:
dGV4dCA9ICJIZWxsbywgV29ybGQhIgpsZW5ndGggPSBsZW4odGV4dCkKcHJpbnQobGVuZ3RoKSAjIE91dHB1dDogMTM=
In this example, len(text) returns 13 because there are 13 characters in the string "Hello, World!".
Q2. What is str() in Python?
The str() function in Python is a built-in function that converts the specified value into a string. This is particularly useful when you need to concatenate non-string data types with strings.
Code Example:
bnVtYmVyID0gNDIKdGV4dCA9IHN0cihudW1iZXIpCnByaW50KHRleHQpICNPdXRwdXQ6ICI0MiI=
Here, str(number) converts the integer 42 into the string "42", allowing it to be concatenated with other strings.
Q3. What is string length()?
It seems there might be a slight confusion in this question. If you're referring to the len() function, it is used to determine the length of a string in Python. The len() function returns the number of items (characters) in an object, and when applied to a string, it returns the number of characters in that string.
Code Example:
dGV4dCA9ICJQeXRob24iCmxlbmd0aCA9IGxlbih0ZXh0KQpwcmludChsZW5ndGgpICMgT3V0cHV0OiA2
In this example, len(text) returns 6 because there are 6 characters in the string "Python".
Q4. How to Handle Errors When Getting String Length?
When using the len() function, it's important to ensure that the object is a string to avoid errors. If there's a possibility that the object might not be a string, you can handle such cases using a try-except block.
Code Example:
ZGVmIGdldF9zdHJpbmdfbGVuZ3RoKG9iaik6CiAgICB0cnk6CiAgICAgICAgcmV0dXJuIGxlbihvYmopCiAgICBleGNlcHQgVHlwZUVycm9yOgogICAgICAgIHJldHVybiAiUHJvdmlkZWQgb2JqZWN0IGlzIG5vdCBhIHN0cmluZy4iCgojIFVzYWdlCnJlc3VsdCA9IGdldF9zdHJpbmdfbGVuZ3RoKCJIZWxsbyIpCnByaW50KHJlc3VsdCkgIyBPdXRwdXQ6IDUKCnJlc3VsdCA9IGdldF9zdHJpbmdfbGVuZ3RoKDEyMykKcHJpbnQocmVzdWx0KSAjIE91dHB1dDogUHJvdmlkZWQgb2JqZWN0IGlzIG5vdCBhIHN0cmluZy4=
In this function, get_string_length, we attempt to get the length of the object. If the object is not a string, a TypeError is raised, and we handle it by returning a custom error message.
Q5. How can I find the longest and shortest strings in a list?
To find the longest and shortest strings in a list, you can use the max() and min() functions with the key parameter set to len, which allows you to compare the strings based on their lengths.
Code Example:
c3RyaW5ncyA9IFsiYXBwbGUiLCAiYmFuYW5hIiwgImNoZXJyeSIsICJkYXRlIl0KbG9uZ2VzdCA9IG1heChzdHJpbmdzLCBrZXk9bGVuKQpzaG9ydGVzdCA9IG1pbihzdHJpbmdzLCBrZXk9bGVuKQoKcHJpbnQoZiJMb25nZXN0IHN0cmluZzoge2xvbmdlc3R9IikgIyBPdXRwdXQ6IGJhbmFuYQpwcmludChmIlNob3J0ZXN0IHN0cmluZzoge3Nob3J0ZXN0fSIpICMgT3V0cHV0OiBkYXRl
In this example:
- We use the max() function to find the longest string. The max(strings, key=len) returns the string with the maximum length, which is "banana".
- Similarly, min(strings, key=len) returns the string with the minimum length, which is "date".
By now, you must be able to write a program to find the length of a string in Python. Here are a few more interesting topics you will like:
- Python String.Replace() And 8 Other Ways Explained (+Examples)
- f-string In Python | Syntax & Usage Explained (+Code Examples)
- How To Reverse A String In Python? 10 Easy Ways With Examples
- Python List insert() Method Explained With Detailed Code Examples
- List Comprehension In Python | A Complete Guide With Example Codes
- What Is High-Level Language? Key Features, Types & More (Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment