Table of content:
- What Are Logical Operators In C?
- The AND (&&) Logical Operators In C
- The OR (||) Logical Operator In C
- The NOT (!) Logical Operator In C
- The XOR (^) Logical Operator In C
- Implementation Of Logical Operators In C
- Short-Circuiting With Logical Operators In C
- Conclusion
- Frequently Asked Questions
Logical Operators In C (AND, OR, NOT, XOR) With Code Examples
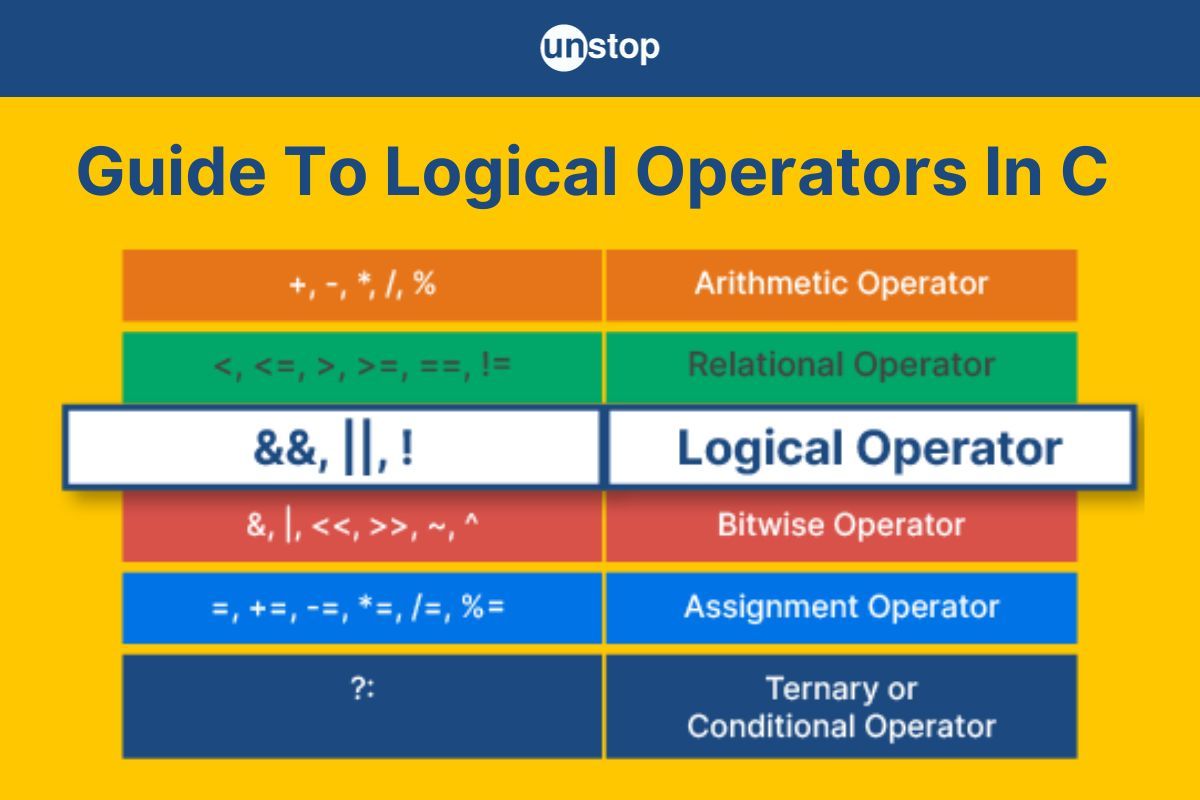
There are a variety of tools and constructs in programming that facilitate the decision-making process and control the program flow. This includes operators- symbols denoting the mathematical or logical computation to be performed on one or more operands/ data values. Several kinds of operators are available in the C like arithmetic, relational, logical, bitwise, etc.
In this article, we will explore logical operators in C language, their types, usage, etc., with proper code examples. Logical operators are crucial in evaluating conditions and making informed choices within your code.
What Are Logical Operators In C?
Logical operators in C programming language are used to perform logical operations/comparisons on two or more boolean expressions or operands. By boolean expression we mean that the expressions evaluate to a boolean value.
The outcome of the logical comparison is also always a boolean value, i.e., True or False. There are mainly four logical operators in C, including:
- Logical AND (&&)
- Logical OR (||)
- Logical NOT (!)
- Logical XOR (^)
These operators are used in powerful decision-making operations involving various conditional if statements. We will discuss all these operators individually in the sections ahead.
The AND (&&) Logical Operators In C
The logical AND operator in C is denoted by the double ampersand symbol (&&). It is a binary operator that takes two boolean expressions as operands and returns a boolean value.
- When both the boolean expressions are true, the operator returns true and false otherwise.
- The logical AND operator in C is mostly used in decision-making statements to check if multiple conditions are true before executing a block/ lines of code.
Syntax Of AND Logical Operator In C:
expression1 && expression2
Here,
- The expression1 and expression2 refer to the two operands or conditions.
- The logical AND operator (&&) is placed in the middle, showcasing that it's comparing the two operands/ expressions.
Note- The result of the logical-AND operation will be a boolean value. The truth table for the logical AND operator in C is as follows:
expression1 |
expression2 |
expression1&&expression2 |
false |
false |
false |
false |
true |
false |
true |
false |
false |
true |
true |
true |
Let's look at a simple C program example illustrating how this logical operator works.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKCgppbnQgYWdlID0gMjU7CmNoYXIgZ2VuZGVyID0gJ00nOwoKCmlmIChhZ2UgPj0gMTggJiYgZ2VuZGVyID09ICdNJykgewpwcmludGYoIllvdSBhcmUgYSBtYWxlIGFkdWx0LlxuIik7Cn0gZWxzZSB7CnByaW50ZigiWW91IGFyZSBub3QgYSBtYWxlIGFkdWx0LlxuIik7fQoKcmV0dXJuIDA7Cn0=
Output:
You are a male adult.
Explanation:
In the simple C code example above,
- We begin by including the header file for input/ output operations and define the main() function, which is the entry point for the program's execution.
- Inside the main(), we declare two variables, i.e., age (integer type) and gender (character type) and initialize them with values 25 and 'M', respectively.
- Then, we initiate an if-else conditional statement to check if a person is a male adult based on age and gender.
- In the statement condition, we use relational operators to see if the age is greater than or equal to 18 (i.e., age >= 18) and gender is equal to male (i.e., gender == 'M').
- Then, we use the logical AND operator (&&) to connect the entire condition, and the program checks the result of individual conditions/ operands.
- If both conditions are true, the whole logical condition is evaluated as true, and the code inside the if-block is executed. It contains a printf() function to display the string literal- 'You are a male adult'.
- Inside the literal/ formatted string, we have a newline escape sequence (\n) that shifts the cursor to the next line.
- If either of the conditions is false, the whole statement condition is evaluated as false as per the logical AND operator, and the code inside the else block is executed. It prints the string/ message- 'You are not a male adult'.
- The program ends with a return 0 statement, indicating successful execution without any errors.
The OR (||) Logical Operator In C
The binary logical OR operator (||) takes two operands and analyzes the relations between them.
- This logical operator in C returns true if at least one of the expressions/ operands is evaluated to be true. In all other situations, it returns false.
- This operator is employed in control statements/ structures, notably the if conditional statements and while loops, to test multiple conditions at one point in time.
In simple terms, the logical OR operator (||) is used in C programs to evaluate the two expressions on either side, returning a boolean value, i.e., true or false, depending on the results of each.
Syntax Of OR Logical Operator In C:
expression1 || expression2
Here,
- The expressions, i.e., expression1 and 2, are the two operands/ expressions.
- The logical OR (||) operator tests the expressions depending on the condition.
Note- This type of operator returns true even if at least one of the expressions comes to be true. The return value for the logical OR operator in C is summarized in the truth table below.
expression1 |
expression2 |
expression1|| expression2 |
false |
false |
false |
false |
true |
true |
true |
false |
true |
true |
true |
true |
One needs to understand how to use the looping statement and if-statements with logical operators like OR, etc., to write and run reliable C programs. Let's look at a C code example showcasing the implementation of the OR logical operator.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKCmludCBudW07CnByaW50ZigiRW50ZXIgYSBudW1iZXI6ICIpOwpzY2FuZigiJWQiLCAmbnVtKTsKCmlmIChudW0gJSAzID09IDAgfHwgbnVtICUgNSA9PSAwKSB7CnByaW50ZigiJWQgaXMgZGl2aXNpYmxlIGJ5IDMgb3IgNS4iLCBudW0pOwp9IGVsc2UgewpwcmludGYoIiVkIGlzIG5vdCBkaXZpc2libGUgYnkgMyBvciA1LiIsIG51bSk7fQoKcmV0dXJuIDA7Cn0=
Output:
Enter a number: 40
40 is divisible by 3 or 5.
Explanation:
In this C program example, we test two conditions and perform the logical-OR operation to see if the given number is divisible by 3 or 5.
- We begin by including essential header files and initiating the main() function, which is the program's entry point for execution.
- Inside main(), we then declare an integer data type variable num and prompt the user to input a value using the printf() function.
- We then read input with scanf() function and store in the respective variable using address-of/ reference operator (&).
- Next, we set up an if-else conditional statement to check if the num is divisible by 3 or 5.
- For this, we first have a combination of conditions that use the equal to relational operator to see if the modulus (i.e., remainder) of division by either 3 or 5 is equal to zero.
- We then use the logical OR operator to compare the evaluation/ result of the two conditions.
- If either condition is true, the entire condition evaluates to true, and the if-block is executed. It prints a message indicating that the number is divisible by 3 or 5. Here, we use the %d format specifier is the placeholder for the integer value.
- If neither condition is true, the whole logical condition evaluates to false and the else-block is executed. It prints a message indicating that the number is not divisible by 3 or 5.
- Since in this example, the modulus of num and 5 is zero here, the OR operator returns true, and the first message is printed to the console.
- Finally, the program returns 0 to signal successful execution and ends.
Check out this amazing course to become the best version of the C programmer you can be.
The NOT (!) Logical Operator In C
In C programming, the logical NOT operator is a unary operator that takes a single operand and reverses its truth value.
- It is denoted by the exclamation mark, i.e., the (!) symbol and is used to negate a logical expression.
- If the expression is true, the logical NOT operator will return false.
- If the expression is false, then this type of operator returns true.
Syntax For NOT Logical Operator In C:
!expression
Here,
- The expression can be any logical expression that evaluates to either true or false.
- After applying the logical NOT operator (!) to the expression, the resulting value will be the opposite of the original value of the expression.
Common applications of this operator include checking whether a variable is equal to a specific value or reversing the outcome of a comparison operation. It can be used to write effective programs in C language. The truth table for this unary operator/ OR logical operator in C is as follows:
expression |
!expression |
false |
true |
true |
false |
Below is an example C program illustrating the use of this logical operator.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKaW50IG51bTsKcHJpbnRmKCJFbnRlciBhIG51bWJlcjogIik7CnNjYW5mKCIlZCIsICZudW0pOwoKCmlmICghKG51bSA8IDApKSB7CnByaW50ZigiJWQgaXMgbm90IG5lZ2F0aXZlLiIsIG51bSk7Cn0gZWxzZSB7CnByaW50ZigiJWQgaXMgbmVnYXRpdmUuIiwgbnVtKTt9CgpyZXR1cm4gMDsKfQ==
Output:
Enter a number: -7
-7 is negative.
Explanation
In the example C code, we check if the user input number is greater than zero using the logical NOT operator.
- Inside the main() function, we declare an integer variable with the name/ identifier num.
- We then prompt the user to input a value using the printf() function and use the standard library function scanf() to read an integer value from the user. The inputted value is stored in the num variable.
- Next, we define an if-else conditional statement to check if the condition if the value of num is not less than 0 (i.e., !(num < 0)) using a relational operator and logical NOT. In other words, it checks if the number is greater than or equal to 0.
- If the condition is true, it prints a message indicating that the number is not negative.
- If the condition is false, it prints a message indicating that the number is negative.
- Finally, the program returns 0 to signal successful execution and ends.
Hone your coding skills with the 100-Day Coding Sprint at Unstop and claim bragging rights now!
The XOR (^) Logical Operator In C
In C, the XOR logical operator is represented by the hat sign (^) and is used to perform the exclusive OR operation between two operands.
- The XOR operation evaluates to true (1) if the operands have opposite truth values (one operand is true and the other is false) and false (0) if the operands have the same truth value (both true or both false).
- The XOR operator is commonly used in C to manipulate bits in binary numbers, particularly for encryption and error detection tasks.
Syntax:
expression1 ^ expression2
Here,
- Both expression1 and expression2 refer to the two operands, which should be of the data type that can be represented in binary (such as integers or characters).
- This is because the XOR operation is performed on the binary representation of these values.
The return values for the logical XOR operator in C, depending upon the evaluation of the operands, are given in the truth table as follows:
expression1 |
expression2 |
expression1 ^ expression2 |
false |
false |
false |
false |
true |
true |
true |
false |
true |
true |
true |
false |
Look at the sample C program below to understand the implementation fo this logical operator.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKaW50IHggPSA1OyAvLyBCaW5hcnkgcmVwcmVzZW50YXRpb246IDAxMDEKaW50IHkgPSAxMDsgLy8gQmluYXJ5IHJlcHJlc2VudGF0aW9uOiAxMDEwCgppbnQgcmVzdWx0ID0geCBeIHk7IC8vIEJpbmFyeSByZXByZXNlbnRhdGlvbjogMTExMQpwcmludGYoInggWE9SIHkgPSAlZCIsIHJlc3VsdCk7CgpyZXR1cm4gMDsKfQ==
Output
x XOR y = 15
Explanation
In the sample C code, we perform a logical XOR operation between two integer values, x and y.
- We include the <stdio.h> header and define the main() function.
- Inside the main, we declare two integer variables, x and y, and assign them values 5 and 10, respectively.
- The binary representation of x is 0101, and the binary representation of y is 1010.
- We then perform a logical XOR operation between x and y, and the result is stored in the result variable.
- The XOR operation combines the bits from x and y by taking the XOR of each pair of corresponding bits, resulting in the binary representation 1111. (Since 0 and 1 give 1, 1 and 0 give 1, and so on.)
- Next, we use the printf() function to display the result of the XOR operation, which is the decimal value 15 (result).
- Finally, the program returns 0 to signal successful execution and ends.
Implementation Of Logical Operators In C
Thus far, we have discussed all four logical operators in C individually. Let's look at programming scenario in which we apply all four logical operators in C and observe their logical outcomes. The C program sample below illustrates the same.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpewppbnQgYSA9IDU7CmludCBiID0gNzsKaW50IGMgPSAxMTsKaW50IHJlc3VsdDsKCi8vIFVzaW5nIHRoZSBBTkQgb3BlcmF0b3IgdG8gY2hlY2sgaWYgYSBhbmQgYiBhcmUgb2RkIG9yIGV2ZW4KaWYgKGEgJSAyID09IDAgJiYgYiAlIDIgPT0gMCkgewpwcmludGYoIkJvdGggYSBhbmQgYiBhcmUgZXZlblxuIik7Cn1lbHNlewpwcmludGYoIkJvdGggYSBhbmQgYiBhcmUgb2RkXG4iKTt9CgovLyBVc2luZyB0aGUgT1Igb3BlcmF0b3IgdG8gY2hlY2sgaWYgYiBpcyBncmVhdGVyIHRoYW4gMTAgb3IgYyBpcyBsZXNzIHRoYW4gMTIKaWYgKGIgPiAxMCB8fCBjIDwgMTIpIHsKcHJpbnRmKCJiIGlzIGdyZWF0ZXIgdGhhbiAxMCBvciBjIGlzIGxlc3MgdGhhbiAxMlxuIik7fQoKLy8gVXNpbmcgdGhlIE5PVCBvcGVyYXRvciB0byBjaGVjayBpZiBiIGlzIG5vdCBlcXVhbCB0byA3CmlmICghKGIgPT0gNykpIHsKcHJpbnRmKCJiIGlzIG5vdCBlcXVhbCB0byA3XG4iKTsKfWVsc2V7CnByaW50ZigiYiBpcyBlcXVhbCB0byA3XG4iKTt9CgovLyBVc2luZyB0aGUgWE9SIG9wZXJhdG9yIHRvIHBlcmZvcm0gdGhlIGV4Y2x1c2l2ZSBPUiBvcGVyYXRpb24gYmV0d2VlbiBiIGFuZCBjCnJlc3VsdCA9IGIgXiBjOwpwcmludGYoImIgWE9SIGMgPSAlZFxuIiwgcmVzdWx0KTsKCnJldHVybiAwOwp9
Output
Both a and b are odd
b is greater than 10 or c is less than 12
b is equal to 7
b XOR c = 12
Explanation:
We again begin the C code sample above by including the <stdio.h> library.
- Inside the main() function, we declare three integer variables, i.e., a, b, and c, and initialize them with values 5, 7, and 11, respectively.
- We also declare a fourth integer variable called result, where we will store the output of the logical XOR operation.
- Next, we start applying the logical operators in C one by one.
- First, we have an if-else statement that applies the logical AND operator (as mentioned in the code comments) to determine if a and b are odd (not divisible by 2) or even (divisible by 2).
- Here, we have two expressions with relational operators. The left one checks if the modulus of a by 2 is zero, and the right one checks if the modulus of b by 2 is zero.
- Then, using the AND operator, if both conditions are true, the entire logical condition returns true, and the code inside the first curly brackets is executed.
- If either condition is false, the entire logical condition returns false and the else block is executed.
- Next, we use an if-statement and logical operator OR to check if b is greater than 10 or c is less than 12.
- Here, the left-side operand/condition/expression checks if b<10, and the right-hand operand checks if c<12.
- If either expression is true, the whole logical expression returns True and the if-block (marked by if keyword) is executed. It prints the string message- 'b is greater than 10 or c is less than 12'.
- If both expressions return false, the whole logical expression returns False, and the program moves to the next line of code.
- Then, we have an if-else conditional statement which checks if b is not equal to 7. It uses the logical NOT operator (!) as follows-
- The if condition checks if b is not equal to 7, i.e., (!(b == 7)).
- If the condition is true, the code in the if-block is executed printing the message- "b is not equal to 7".
- If the condition is false, then the else block is executed printing the message- "b is equal to 7".
- Finally, we perform the logical XOR operation between b and c and store the result in the result variable.
- The binary representation of b (7) is 0111, and the binary representation of c (11) is 1011.
- The XOR operation combines the bits, resulting in the binary representation 1100, whose decimal representation is 12.
- The program terminates with a return 0 statement, indicating successful execution without any errors.
Also Read: Compilation In C | Detail Explanation Using Diagrams & Examples
Short-Circuiting With Logical Operators In C
In C programming language, short-circuiting refers to a behaviour of logical operators (&& and ||) where the second operand/ condition of the whole logical expression is evaluated only if it is necessary to determine the result of the expression.
- For example, when using the AND (&&) logical operator in C programs, there is no need to evaluate the second operand if the first operand evaluates to false. This is because, in this case, if either of the expressions/ conditions is false, the entire expression must be false.
- Similalry, in case of OR logical operator, if either conditions/ operands are true then the entire expression must be true. Therefore, there will be no need to evaluate the second operand if the first operand is evaluated to be true.
- This optimization is called short-circuiting because it can result in fewer operations being performed and, therefore, shorter execution time.
Short-circuiting can be particularly useful when evaluating expressions that involve complex conditions/ extensive computations, function calls, or I/O operations. It can also be useful in cases where evaluating a particular operand would result in an error or exception.
Looking for mentors? Find the perfect mentor from select experienced coding & software experts here.
Conclusion
Logical operators in C are fundamental components that allow developers to create conditional statements and perform logical comparisons.
- There are four primary logical operators in C: logical AND (&&), logical OR (||), logical NOT (!), and logical XOR (^).
- These operators let programmers combine multiple conditions or complex expressions and evaluate them as a single boolean value.
- Logical operators are crucial in regulating program flow by writing effective code that helps in decision-making based on true or false conditions.
The short-circuit evaluation feature of logical operators can be used to optimize code performance by only evaluating necessary operands. In summary, logical operators in C are integral for making logical judgments and managing program flow.
Also read- 100+ Top C Interview Questions With Answers (2023)
Frequently Asked Questions
Q1. What are the primary types of operators in C?
There are seven primary types of operators in C programming, including:
- Arithmetic operators (+, -, *, /, %): These operators are used when conducting mathematical/ arithmetic operations like addition, multiplication, subtraction etc.
- Relational operators (<, >, <=, >=, ==): These are used to compare two values, and depending on the outcome, the operator returns true or false, i.e., a Boolean value.
- Logical operators (&&, AND, ||, OR): The logical operators in C are frequently employed to carry out logical operations and return a boolean value.
- Bitwise operators (AND, OR, XOR): They perform operations on the binary representation of variables, i.e., at the bit-level.
- Assignment operators (=, +=, -+, /+): As the name suggests, these operators are used to assign values to variables.
- Conditional operator (? :): Conditional operator, also known as ternary operator, is a shorthand way of writing conditional statements.
- Increment & Decrement Operators (++, ---): These operators help increase or decrease the value of operands by a predefined number.
Q. What are logical operators in C? Explain with an example.
Logical operators in C programming are used to evaluate multiple conditions or expressions and return a boolean value (true or false) based on the result. Logical operators are used in many different areas of programming, like in conditional statements and loops. In C, we have four major logical operators to make programming more concise, including:
Logical AND (&&) Operator: This binary operator takes two boolean expressions as operands and returns a boolean value. When both the boolean expressions are true, they return true and false otherwise.
Syntax: expression1 && expression2
Logical OR(||) Operator: Also a binary operator, this logical operator in C receives two operands and returns true if at least one evaluates to true. It returns false in all other cases. In other words, the logical OR operator (||) in C works by evaluating the two expressions on either side of the operator and returning a boolean value.
Syntax: expression1 || expression2
Logical NOT (!) Operator: The logical NOT operator in C is used to reverse the truth value of a logical expression, denoted by the exclamation mark (!). If the single expression is true, then the logical NOT operator will make it false. Alternatively, if the expression is false, the logical NOT operator will make it true.
Syntax: !expression
Logical XOR (^) Operator: The XOR logical operator performs the exclusive OR operation between two operands. The XOR operation results in true (1) if the operands have opposite truth values (one operand is true and the other is false). The result is false (0) if the operands have the same truth value (both true or both false).
Syntax: expression1 ^ expression2
Here is an example of the AND logical operators in C-
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKaW50IG51bTsKcHJpbnRmKCJFbnRlciBhbiBpbnRlZ2VyOiAiKTsKc2NhbmYoIiVkIiwgJm51bSk7CgppZiAobnVtID4gMCAmJiBudW0gJSAyID09IDApIHsKcHJpbnRmKCIlZCBpcyBhIHBvc2l0aXZlIGFuZCBldmVuIG51bWJlci5cbiIsIG51bSk7Cn0gZWxzZSB7CnByaW50ZigiJWQgaXMgbm90IGEgcG9zaXRpdmUgYW5kIGV2ZW4gbnVtYmVyLlxuIiwgbnVtKTt9CgpyZXR1cm4gMDsKfQ==
Output
Enter an integer: 10
10 is a positive and even number.
Explanation:
Here, we have used the logical AND operator to check whether the given number is positive and even or not. We have taken the input as 10, as 10 is greater than 0 and divisible by 2, so it has been printed that 10 is a positive and even number.
Q. What is the difference between bitwise operators and logical operators in C?
Bitwise operators perform bit-level manipulations on integer operands, often used in low-level programming. Meanwhile, logical operators in C are used to evaluate Boolean expressions and control the flow of a program. The table below highlights the key differences between the two:
Basis | Logical Operators | Bitwise Operators |
---|---|---|
Operators | AND (&&), OR (||), NOT (!) | AND (&), OR (|), XOR (^), Complement (^) |
Operands | Operates on Boolean expressions (true/false) | Operate on individual bits of integer data types/ binary representation |
Return Value | Boolean (0 for false, 1 for true) | Integer value with manipulated bits |
Usage | Used in control flow statements (if, while, etc.) | Used for low-level operations, such as bit manipulation |
Operation Level | Evaluates entire expressions logically | Manipulates individual bits in binary representation |
Common Use Cases | Combine conditions, invert conditions | Masking bits, toggling bits, shifting bits |
Q. What are the operators in C? Explain.
To begin with, an operator is a symbol signifying a mathematical/ logical operation that has to be performed on one or more values. These values are known as operands. We use operators to manipulate data. There are many kinds of operators in the C programming language, including-
Arithmetic Operators | +, -, *, /, % |
Relational Operators | <, >, <=,>=, == |
Logical Operators | AND (&&), OR (||), NOT (!), XOR (^) |
Bitwise Operators | AND (&), OR (|), XOR (^), Complement (~) |
Assignment Operators | =, +=, -=, *=, /=, %= |
Shift Operators | <<, >> |
Size-of Operator | sizeof() |
Special Operators | Comma (,), Address-of (&), |
Pointer Operator | * |
Q. What is the NOT logical operator in C?
The NOT logical operator in C is the exclamation symbol (!). It is used to invert the Boolean value of an expression. If an expression evaluates to true, applying the NOT operator will make it false, and if it evaluates to false, the NOT operator will make it true. For example, if x is true, then !x will be false, and vice versa. This operator is commonly used in conditions to reverse the outcome of a Boolean expression.
Q. Why are logical operators in C important?
Logical operators in C are essential because they allow programmers to create conditional statements and perform logical comparisons between variables or expressions.
- These operators play a crucial role in decision-making and regulating the flow of a program.
- By combining multiple conditions, logical operators determine whether a compound relational expression is true or false, enabling specific blocks of code to run based on the result.
- Additionally, the short-circuiting feature of logical operators can improve program efficiency by avoiding unnecessary evaluation process.
In essence, you can execute logical decisions and regular program flow effectively using logical operators in C.
You might also be interested in reading the following:
- Pointers In C | Ultimate Guide With Easy Explanations (+Examples)
- Type Casting In C | Types, Cast Functions, & More (+Code Examples)
- Arrays In C | Declare, Initialize, Manipulate & More (+Code Examples)
- Reverse A String In C | 10 Different Ways With Detailed Examples
- Understanding Constant In C: How To Define, Syntax, and Types
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment