Java Programming Language Table of content:
Main Method In Java | Breakdown, Rules & Variations (+Examples)
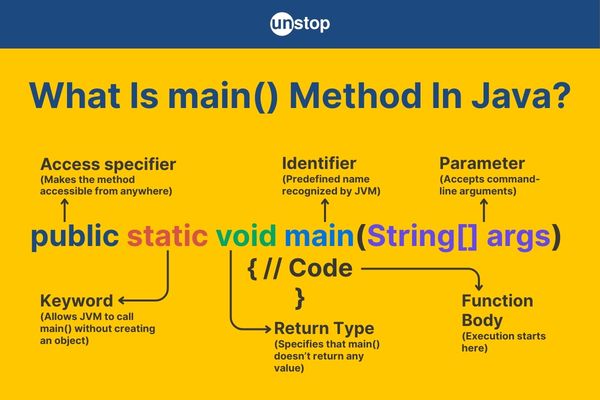
Imagine writing a heartfelt letter but forgetting to mention the recipient’s name. No matter how well-written it is, no one knows whom it’s for! That’s exactly how Java programs feel without the main() method—confused and directionless.
The main() method in Java is where the magic begins. It's the official entry point for any standalone Java application, acting as the bridge between your code and the Java Virtual Machine (JVM). Without it, your program won’t execute, no matter how brilliant your logic is.
In this article, we’ll break down the main() method in Java, its structure/components, and more.
What Is main() Method In Java?
The main() method in Java is the designated entry point for program execution. When you run a Java application, the Java Virtual Machine (JVM) searches for this method to kick-start the execution.
Key Points About Java main() Method:
- It must be public, static, and void to be recognized by the JVM.
- It is placed inside a class since Java follows an object-oriented approach.
- The parameter String[] args allows command-line arguments to be passed when executing the program.
- Without a proper main() method, your Java application won’t run successfully.
For example, a simple Java program always includes the main() method:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, Java!");
}
}
Importance Of main() Method In Java Applications
- Program Entry Point: The main() method is the first thing the JVM looks for when running a program.
- Standard Convention: Following this structure ensures consistency across Java applications.
- Command-Line Interaction: The String[] args parameter enables command-line arguments for flexibility.
- Foundation for Larger Programs: Even complex Java applications start with main() as an entry point before branching into other methods and classes.
Syntax Of main() Method In Java
The main() method in Java programming language follows a specific structure that the JVM recognizes to start program execution. Here’s its standard syntax:
public static void main(String[] args) {
// Code execution starts here
}
Let's break down its components:
Component |
Meaning |
public |
Makes the method accessible to the JVM from anywhere. |
static |
Allows JVM to call main() without creating an object. |
void |
Specifies that the method doesn’t return any value. |
main |
The predefined name is recognized by the JVM as the entry point. |
String[] args |
Accepts command-line arguments as an array of strings. |
Look at the labeled diagram of the syntax of the main() method in Java, given in the banner image at the top, to get a better idea. Each component plays a crucial role, and we will discuss each of these in detail in the following sections.
public Specifier – Main Method In Java
An access specifier determines the visibility of a method or variables in Java programs. The main() method uses the public access specifier, ensuring that it is accessible to the Java Virtual Machine (JVM) from anywhere. Since the JVM needs to call main() from outside the class, making it public is essential.
Why Is main() Public?
- JVM Accessibility: Since the JVM calls main(), it must be publicly accessible from outside the class.
- Prevents Access Issues: If main() were private or protected, the JVM wouldn’t be able to execute it, leading to an error.
- Standard Convention: Java follows strict conventions, and making main() public ensures uniformity across all applications.
What Happens If main() Is Not Public?
Let’s see an example where main() is declared as private (filename– Demo.java):
Error Output:
Error: Main method not found in class Demo, please define the main method as:
public static void main(String[] args)
Explanation:
- Since main() is private, the JVM cannot access it, resulting in an error.
- To fix this, we must declare main() as public.
static Keyword – Main Method In Java
The static keyword in Java allows a method or variable to belong to the class itself rather than requiring an instance of the class. In the main() method, static plays a crucial role in enabling the JVM to execute the method without creating an object of the class.
Why Is main() Static?
- No Object Creation Needed: Since main() is a static method, the JVM can call it directly without needing to instantiate the class.
- Saves Memory & Execution Time: Avoiding object creation reduces memory usage and speeds up execution.
- Prevents Unnecessary Overhead: If main() were non-static, every execution would require an object, which is inefficient.
What Happens If main() Is Not Static?
Let’s see an example where the main() method in Java program is declared without static:
Error Output:
Error: Main method is not static in class Demo, please define the main method as:
public static void main(String[] args)
Explanation:
- Since main() is an instance method here, the JVM cannot call it without an object.
- This violates Java’s execution model, causing an error.
- To fix this, we must declare main() as static.
Want to expand your knowledge of Java programming? Check out this amazing course and fast-track your journey to becoming a Java developer.
void Return Type Of Main Method In Java
In Java, the void keyword indicates that a method does not return any value. Since the main() method serves as the program’s entry point rather than producing a result, it is declared with void keyword for its return type.
Why Is main() Void?
- No Value Needs to Be Returned: The main() method is executed by the JVM to start the program, but it doesn’t return any result to the JVM.
- Follows Java's Convention: Standard Java applications follow this structure, ensuring consistency across different programs.
- Prevents Unexpected Behavior: If main() returned a value, there would be ambiguity about what the JVM should do with it.
What Happens If main() Has a Return Type?
Let’s modify the main() method in Java to return an int data type value and see what happens:
Error Output:
Error: Main method must return void in class Demo, please define the main method as:
public static void main(String[] args)
Explanation:
- The JVM expects main() to have a void return type.
- Returning a value disrupts the standard method signature, causing an error.
- To fix this, main() must be declared as void.
The main Identifier – Main Method In Java
In Java, main is not a keyword but an identifier—a name assigned to the method that serves as the entry point for program execution. The Java Virtual Machine (JVM) specifically looks for a method named main with the correct signature to start execution.
Why Must It Be Named main?
- JVM Recognition: The JVM is programmed to look for public static void main(String[] args). Any other name will cause an error.
- Standard Convention: Using main ensures uniformity across Java applications.
- Not a Reserved Keyword: Since main is just an identifier, it can technically be used as a variable or method name elsewhere in the program.
What Happens If We Change the Name?
Let’s modify the name of the main() method in Java to start and see how it impacts execution:
Error Output:
Error: Main method not found in class Demo, please define the main method as:
public static void main(String[] args)
Explanation:
- The JVM does not recognize start() as the entry point.
- It strictly looks for main() with the exact method signature.
- To fix this, we must name the method main.
String[] args In Main Method In Java
In Java, String[] args is a parameter passed to the main() method that allows users to provide command-line arguments when running a program. These arguments are received as an array of String values, which can be accessed inside the program.
Why Does main() Method In Java Have String[] args?
- User Input at Runtime: Allows users to pass values when executing the program without modifying the code.
- Enhances Flexibility: Programs can behave differently based on the provided arguments.
- Handles External Data: Useful in real-world applications, such as configuring settings, specifying file paths, or passing inputs to scripts.
How Command-Line Arguments Work
When running a Java program from the terminal, we can pass arguments after the class name. For example, consider this Java program:
If we compile and run it using:
javac Demo.java
java Demo Hello World
Output:
First argument: Hello
Second argument: World
Breaking It Down:
- args[0] stores "Hello", and args[1] stores "World".
- The values are passed when executing the program.
- If no arguments are provided, trying to access args[0] or args[1] will cause an ArrayIndexOutOfBoundsException.
What If We Don’t Use String[] args?
If we modify the main() method in Java to remove String[] args here is what happens:
Error Output:
Error: Main method not found in class Demo, please define the main method as:
public static void main(String[] args)
Explanation:
- The JVM requires the method signature to include String[] args, even if we don’t use it.
- If no arguments are needed, we can simply ignore the args array inside the method.
Here is your chance to top the leaderboard while polishing your coding skills: Participate in the 100-Day Coding Sprint at Unstop.
The Role Of Java Virtual Machine (JVM)
The Java Virtual Machine (JVM) is responsible for executing Java programs, and it plays a crucial role in identifying and invoking the main() method. Without the JVM, Java code is just text—it's the JVM that translates it into machine-executable instructions.
How Does The JVM Identify main()?
When a Java program is executed:
- Class Loading: The JVM loads the class that contains the main() method.
- Method Lookup: It searches for public static void main(String[] args).
- Execution Start: Once found, execution begins inside the main() method in Java programs.
If the JVM does not find a properly defined main() method, it throws an error:
Error: Main method not found in class Demo, please define the main method as:
public static void main(String[] args)
JVM’s Role In Java Execution
- Compilation & Interpretation: Java code is compiled into bytecode (.class files) and executed by the JVM.
- Memory Management: The JVM handles memory allocation, garbage collection, and stack/heap management.
- Platform Independence: The JVM ensures Java runs on any system with a compatible JVM, making Java a "write once, run anywhere" language.
JVM & The main() Method In Java– A Visual Flow
Running Java Programs Without The Main Method
The main() method is the entry point of a Java application, but are there ways to run Java programs without it? The short answer: Yes, but with limitations.
1. Using Static Blocks (Before Java 7)
Before Java 7, Java features allowed code execution inside a static block without needing the main() method.
Output:
Executed without main!
Why Does This Work?
- The JVM executes static blocks when the class is loaded.
- System.exit(0) prevents the JVM from searching for main().
Why Doesn't This Work Anymore?
Since Java 7, the JVM strictly requires the main() method to exist, and missing it results in an error:
Error: Main method not found in class Demo, please define the main method as:
public static void main(String[] args)
2. Using JavaFX Or Servlets
JavaFX applications and web-based servlets don’t always require the main() method to be there in Java programs. This is because:
- JavaFX applications use the Application class’s start() method instead.
- Servlets are executed by a web server, not the JVM’s main() method.
3. Using JNI (Java Native Interface) Or Custom JVM Launchers
Advanced developers can manipulate Java execution using JNI or custom class loaders, but these are not standard approaches for beginners.
This shows that the main() method is mandatory in standard Java applications. Workarounds like static blocks were possible before Java 7 but are now restricted. Also, there are some specialized Java applications (JavaFX, servlets) that don’t require the main() method in Java programs for successful execution.
Variations In Declaration Of Main Method In Java
While public static void main(String[] args) is the standard method signature, Java allows some variations in its declaration without breaking execution.
1. Changing Parameter Name
The parameter name args can be changed to anything as long as the type remains String[].
Output:
This still works!
✅ Why does this work? The JVM looks for String[] as the parameter type, not the variable name.
2. Using varargs (String... args) Instead Of String[] args
Java supports varargs (...), allowing main() to be written as:
Output:
Varargs main method!
✅ Why does this work? String... args is equivalent to String[] args in Java.
3. Reordering Access Specifiers
The order of public and static does not matter.
Output:
Access specifier order changed!
✅ Why does this work? Java doesn’t enforce a strict order for public and static, as long as both are present.
4. Using final, synchronized, or strictfp Modifiers
Java allows some additional modifiers with main(), though they are rarely used.
Output:
Final main method!
✅ Why does this work? The JVM ignores final, synchronized, and strictfp when looking for main().
Overloading The Main Method In Java
Java allows method overloading, meaning multiple methods can share the same name but differ in parameters. This applies to the main() method in Java as well, but with a catch—the JVM will always call the standard main() method (public static void main(String[] args)) to start execution.
Overloaded main() Methods
Output:
Standard main() called
Overloaded main() called with num: 10
✅ Why does this work?
- The JVM starts execution with the standard main() method.
- The overloaded main(int num) method is explicitly called inside the standard main().
Can The JVM Call An Overloaded main() Directly?
No. The JVM only looks for public static void main(String[] args). Any overloaded main() will be ignored unless it is explicitly called within the standard main() method.
Why Overload main() Method In Java Programs?
- Organizing Code – We can use overloaded main() methods to separate logic.
- Handling Different Inputs – Overloaded methods can process different argument types.
- Interview Trick Questions – Knowing that the JVM only calls public static void main(String[] args) helps avoid confusion.
Need more guidance on how to become a Java developer? You can now select an expert to be your mentor here.
Conclusion
The main() method in Java is the entry point of any standalone Java application, serving as the first method executed by the JVM. It follows a specific signature—public static void main(String[] args)—to ensure consistency across Java programs.
Each component of the main() method in Java has a role:
- public ensures accessibility.
- static allows execution without an object.
- void means no return value.
- main is the recognized identifier.
- String[] args handles command-line arguments.
The JVM strictly requires the main() method, and failing to define it correctly results in an error. However, some variations in declaration are allowed, such as changing parameter names, using String... args, or modifying access specifier order.
It is also possible to overload the main() method in Java, but only the standard method is called by the JVM. Understanding the main() method is crucial for writing Java applications, as it dictates how programs begin execution.
Frequently Asked Questions
Q1. Can we have multiple main() methods in a Java program?
Yes, you can use multiple main() methods in Java programs through method overloading. However, the JVM only calls the standard public static void main(String[] args) automatically. The other main() methods need to be explicitly called within the program.
Q2. What happens if we remove static from main()?
Removing static keywords from the main() method in Java causes a runtime error because the JVM would need to create an object before calling main(). But since no constructor is provided for automatic instantiation, it will lead to error in execution.
Q3. Is main() mandatory in Java?
Yes, the main() method is mandatory in all standalone Java applications. However, Java frameworks like JavaFX, servlets, and Spring Boot can run without a main() method, relying on different entry points.
Q4. Can we write main() inside an interface?
No, you cannot write the main() method inside interfaces because they cannot have static methods before Java 8. Even in Java 8+, a static method inside an interface is not considered a valid entry point for program execution.
Q5. Why is String[] args used instead of String args[]?
Both notations– String[] args and String args[]– of the main() method in Java are valid. But String[] args is preferred as it clearly signifies an array of Strings.
Do check the following out for more interesting Java topic reads:
- Operators In Java | 9 Types, Precedence & More (+ Code Examples)
- One Dimensional Array In Java | Operations & More (+Code Examples)
- How To Install Java For Windows, MacOS, And Linux? With Examples
- Method Overriding In Java | Rules, Use-Cases & More (+Examples)
- Advantages And Disadvantages of Java Programming Language