Most Common Programming Interview Questions With Answers 2023
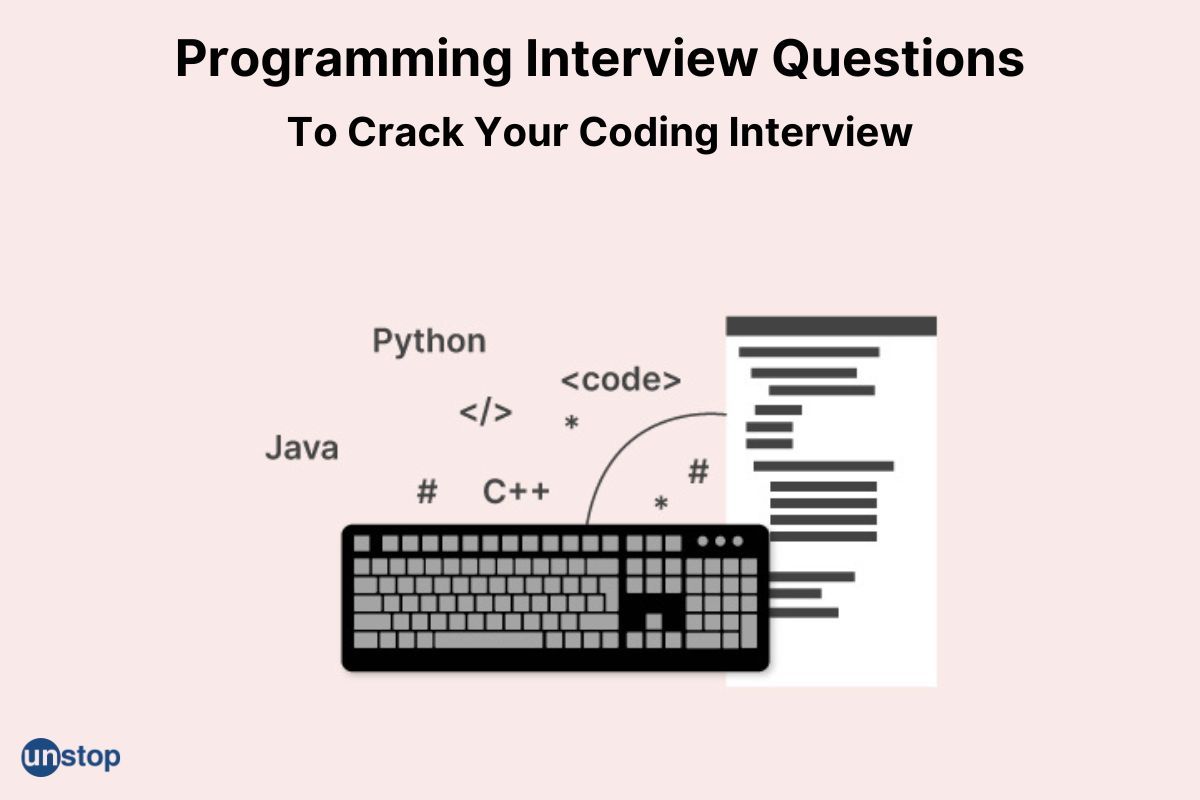
Programming concepts are the foundation of many software and applications around us. With knowledge of array data structure, strings, linked list data structure, binary trees, binary search trees, etc. one can develop real-world solutions to complex problems. Furthermore, they hold key importance in programming jobs or technical interviews as recruiters often ask different coding questions – ranging from basic to advanced theoretical concepts.
Following are some common coding questions, conceptual interview questions, trial questions, advanced questions, and theoretical questions for programming job interviews and technical interviews.
List Of Programming Interview Questions
1. What is computer programming?
Computer programming is the process of writing instructions that tell a computer how to perform tasks. It involves using a programming language, creating algorithms and solving problems. Computer programming aims to create software or applications capable of performing specific tasks efficiently and automatically with minimal user input.
2. What are the different types of errors?
The different types of errors typically found in computer programs include
- Logic Errors: This refers to an inconsistency in the program's flow which may result from incorrect ordering of operations.
- Runtime Errors: These occur during execution due to invalid values provided at run time, such as division by zero.
- Compilation Error: This occurs before execution starts due to improper use of keywords or incorrect data types declared.
- Semantic Error: A semantic error is a code error that produces a result that isn't what the programmer intended. The mistakes in understanding the language cause semantic errors and can be hard to spot, as they don't generate a compiler or runtime warning or error message.
- Syntax Errors: These occur when the text does not conform to proper coding conventions, for example, missing punctuation marks or closing quotation marks, etc.
3. Write a function to check whether a string is a palindrome or not.
Below is a function written in Java to check whether a string is a palindrome or not:
Here's an explanation of the code:
The above code starts by initializing two integer variables, i and j. Integer variable i is set to point to the beginning of the String, while j points to its end. A while loop then iterates through each character in the String, starting with the first one pointed at by i and ending up at one before the middle (pointed at by j).
It compares characters from both ends using the charAt() method, which returns a character from a particular index position provided as an argument. If a mismatch is found, then it immediately terminates loop execution, returning false and signifying that the given String was not palindromic. Still, if the whole iteration completes without any discrepancy between characters from opposite sides, it means all matched, confirming that this indeed was Palindrome. Thus, the function would return true instead.
4. Write a function to reverse a number.
The function takes the integer as input and returns the reverse of it. The approach is to keep modding the number i.e. applying the modulo operation with ten until it becomes 0. With every iteration, the last digit is added at the beginning of the new reversed_number variable. Eventually, once all digits have been accounted for in the reverse form, this becomes our return value.
Here's the code or function to reverse a number C:
5. Write the function to sort the array using the bubble sort algorithm.
Bubble Sort compares each element in the array to its adjacent elements and swaps them if they are out of order.
The code below, in Java, describes an implementation of bubble sort on an array of integers, where it starts from the beginning of an array and swaps two elements if they are out of order until it reaches the end:
This code is an example of a bubble sort algorithm. Here, the outer loop (for loop i) iterates through the entire array, while the inner loop (for loop j) starts at index 0 and compares each element to its right neighbour until it reaches the end of the list - i elements from the last item's position. If two elements are found to be out of order, they are swapped using a temporary variable 'temp.' This process is repeated until no more swaps are needed, ensuring all items within one iteration have been sorted correctly before moving on with the next iteration set up for loops mentioned earlier.
6. Write a recursive function to find the middle element of an array of integers.
Written below is a recursive function that can help find the middle element of an array of integers in C++:
This code creates a linked list and finds the middle data element in the given linked list. It also provides some functions such as incrementing the count, pushing an entry to stack, etc. Function 'push()' takes two parameters, head_ref, which refers to the pointer, and the new_data integer element that needs to be pushed on top of the stack. Then it allocates memory for a node using a new operator and assigns its value as entered by the user. Then the next points toward earlier blocks stored in a heap. Function 'get middle ()' takes one parameter, i.e., pointer head, and it stores the first address of do mid variable for further use. The function counts the number of nodes iteratively. If the condition becomes true when count mod 2 is not equal to zero, then mid=mid->next so that we can reach at our desired middle position. At last, the function returns data inside a middle node.
7. Write a recursive function to count all the leaf nodes in a given tree structure.
A recursive function to count all the leaf nodes of a given tree structure can be defined as follows:
1. Start from the root node in the given tree.
2. Check if it is a leaf node, i.e., having no child nodes, then increment the counter value by one and return this value back upwards through the recursion stack until you get back to starting point (root).
3. If not, traverse each child downwards using recursion, counting their respective leaves and accumulating the same into the final result, returning it upon getting up at every level until you reach starting point(root).
The code below is in C++:
This program initializes a tree with nodes 1, 2, 3 and 4. The function CountLeafNodes() is used to count the number of leaf nodes or end nodes in the tree that have no children. This function takes one parameter, a pointer to the Node root, which points to the head node of the tree. If this parameter is NULL, 0 will be returned, indicating no leaf node present, thus ending recursion. Else, if the left & right children are null (node->left ==NULL && node ->right==NULL), it returns 1 as there exists one leaf node here at this point. Otherwise, it will return a sum of leaves from both subtrees i:e= return countLeafNodes(root ->left)+countLeafNodes(root —>right). Finally, the count statement prints out the total number of Leaf Nodes after calling the above-mentioned function, taking root as its argument.
8. Write the function to sort the array using the insertion sort algorithm.
Below is a function written in C to sort the array using the insertion sort algorithm:
This code is an example of Insertion Sort. It is a sorting algorithm that sorts the given array by iterating through each element in the array and comparing it with its preceding elements. If any preceding elements are greater than themselves, they get swapped to maintain sorted order until all elements have been compared and properly ordered from smallest to largest (or vice versa, depending on user preference). This function takes two parameters, an integer array "arr" and int n representing the size of the said array as input. It also utilizes three variables; key, which will store value during comparisons for every iteration, i which starts at 1 since we assume index 0 counts as 'sorted,' and j, assigned at one less than i so our loop can start with correct comparison points while moving larger values up ahead within arr if needed, once comparison has been done(j--). Then after looping through each element, including the key, we pass on newly shifted positions/values into their respective places using [ j+1 ]=key.
9. Write the function to perform the postorder traversal of a tree.
The function in Python to perform the post-order traversal algorithm is as follows:
This code is an implementation of a post-order traversal algorithm for binary trees. The algorithm uses two stacks, s1 and s2, to traverse the tree correctly (left child first, then right child). First, it pushes the root node onto stack 1 - this will be popped later. Then we enter our while loop, which runs as long as stack 1 has elements. Inside the loop, we pop a node from stack 1 and append it to stack 2 - this corresponds to going down one level of the tree. Then if there are any nodes, children of that node (right or left) are pushed onto Stack 1 so they can be visited at another iteration of our loop when their parent has been processed already. At last, outside this while loop, all nodes stored on the second stack (s2) can be retrieved by popping them out with proper PostOrder Traversal i.e., left child, then right child followed by its parent node. So printing these nodes using the print statement gives us the expected output.
10. Write a program to sort an array of integers using Quick Sort?
Quick Sort is an efficient divide-and-conquer algorithm for sorting an array of integers. The basic idea behind the quick sort algorithm is to partition the array into two parts based on a pivot element, then recursively apply this process until the entire array has been sorted.
To begin, select any element of the array as a "pivot" and reorder all elements such that those smaller than or equal to it appear before it in its subarray and others greater than or equal appear after it in its subarray (the so-called partition step). Then repeat this process with each individual partition until no further partitions can be made!
Here's an example code in Python demonstrating how one might implement Quick Sort:
11. How can we use Hash Table to store and retrieve data efficiently in C++?
A Hash Table is a data structure that efficiently stores and retrieves items based on the key associated with each item. In C++, we can create a bucket of the array (where each bucket contains several elements) and use hash functions to determine which bucket a given element goes into. Then we store all our keys in the same way; the lookup time for any key will be O(1). Hash tables also support efficient insertion and deletion operations, thus making them ideal for implementing dynamic sets or maps.
12. What is the order of precedence for arithmetic operators in Java?
The order of precedence for operators in Java follows standard evaluation rules:
1) Parentheses
2) Exponentiation
3) Multiplication/Division
4) Addition/Subtraction
5) Relational Operators
6) Logical NOT
7) Logical AND
8) Logical OR
9) Assignment Operator
10) Ternary Operator
For example, in the expression 8 + 3 * 4 / 2 - 5, the multiplication and division operations would be completed first (3 x 4 = 12 &; 12/2=6), leaving us with 8+6-5. Then addition and subtraction are performed next (8+ 6 = 14 &; 14 – 5 = 9). The final answer is, therefore, 9.
13. Explain how you would declare and initialize a character array with 10 elements in Python?
To declare and initialize a character array with 10 elements in Python, one would write code like this:
char_arr = ['a', 'b,' 'c," d',' e," f,' 'go,' 'h,' 'i',' j']
For example, the following program prints all of the elements in a character array:
14. Write a program to implement Merge Sort Algorithm on an array of integers.
The below code is in C++ to implement Merge Sort Algorithm on an array of integers:
This program implements the Merge Sort algorithm, which uses a divide-and-conquer approach to sort an array. The program starts by asking for user input (the array size and its elements). It then calls the mergesort() function, passing in parameters like a leftmost index of the array, the rightmost index of the array, and the middle element. This function further divides the unsorted portion into two subarrays until there’s only one element left in each subarray. At this point, merge() is called to combine these already sorted parts in ascending order. Finally, printArray() prints out all elements inside A[].
15. Implement the Binary Search Algorithm to search for an element in a sorted one-dimensional array.
Here is the pseudocode for the binary search algorithm.
1. Start with the middle element of the array;
2. Compare it to an item in question;
3. If they are not equal, divide the array into two parts depending on whether it is greater or less than the target number and repeat step 1 considering only one part;
4. Repeat until either item is found or the entire section has been searched without success (return -1).
Here's an example of the code in Python:
The BinarySearch function uses a while loop to check whether the starting index is less than or equal to the ending index of the list array. Inside the while loop, the middlemen variable stores the middle element of the sorted one-dimensional array. It then checks if it is equal to the target using an IF statement and returns true if so. Else, it will divide into two parts depending on whether its greater or lesser than the target number (else if - else statements), assign those values respectively, and reassign Midindex accordingly with new position values before iterating again in a cycle until either item is found or entire section is searched through without success (-1).
16. Write code to traverse and print values of all elements stored in a multi-dimensional array.
For traversing and printing values of all elements in a multi-dimensional array, we can use nested loops. Below is the code to traverse and print values of all elements stored in a multi-dimensional array in JavaScript:
The code above is used to traverse and print values of all elements stored in a multi-dimensional array. It uses a nested loop structure, with the outer loop responsible for iterating through each element of the array (which represents another smaller array) and an inner loop that iterates through each value in the smaller sub-arrays. We then use console.log() for each iteration to log out the current value at every index within these arrays.
17. Develop an iterative quicksort algorithm using loops instead of recursion for sorting an array of elements in increasing order.
The Iterative QuickSort algorithm uses loops instead of recursion to sort an array of elements in increasing order. First, the given array is pushed onto a stack. Then a while loop iterates over the stack until it becomes empty. For each iteration, the top element from the stack is popped and stored as subpar.
Below is a code in JavaScript to develop an iterative quicksort algorithm using loops instead of recursion for sorting an array of elements in increasing order:
This code implements an iterative quicksort algorithm using loops instead of recursion for sorting an array of elements in increasing order. The function takes the given array as its argument and stores it in a stack variable. It then creates two sub-arrays called leftSubArray and rightSubArray, which will be used to store values that are less than or greater than the pivot element. A loop is used to go through each element of the subArr parameter (excluding the first one) and push them into either left or right arrays respectively, depending upon their value compared with the pivot. Finally, we add these three variables, i.e., rightSubArray, [pivot], and leftSub Array, onto our stack before returning arr at the end.
18. Write a recursive solution to traverse a tree data structure using an artificial language.
Below is the code for a recursive solution to traverse a tree data structure using an artificial language. The code is written in Pseudocode.
function traverseTree(node) print node.value
for each child in node.children
traverseTree(child)
end for
end function
This code recursively traverses a tree data structure by first printing out the current node's value, then iterating through all its children and performing the same operation on each one. This process continues until it reaches any leaf nodes (nodes with no further children), stopping and returning to the calling function.
19. How can we perform dynamic memory allocation in machine language?
Dynamic memory allocation in machine language can be performed using the stack mechanism. This is achieved by pushing values onto a virtual "stack" and then popping them off it as needed, to allocate memory for different variables or data structures. The processors typically have predefined instructions that allow for pushing and popping values from the stack. Additionally, registers can also be used to reserve space for dynamic memory allocation.
20. What is the most efficient way of storing and retrieving data from a non-contiguous memory location in an artificial language?
The most effective method for storing and retrieving data from a non-contiguous memory region in an artificial language is to use a hash table or dynamic array. Hash tables are effective for quickly gaining access to non-sequential things since they use keys to store and retrieve values. Dynamic arrays are collections of elements with changeable sizes that are kept in close proximity in memory, making it simple to retrieve any element by indexing it into the proper location within the array.
21. How can logical operators be used to improve user experience in loops?
Logical operators can be used in combination with loops to introduce conditions inside the loop. This allows us to apply different logic depending on whether certain criteria are met or not. For example, a loop could check if an item from an array matches some condition and only perform operations when that specific item is found. Using this type of logic makes it easier for users to find relevant data faster by avoiding iterations over all elements in the array. This, in turn, improves the user experience by providing faster results.
22. What are the common causes of logical errors when working with string constants?
When working with string constants, common causes of logical mistakes include incorrect syntax, typos in variable names or values, forgetting to declare variables before use, incorrect string concatenation, and attempting to compare two different types (for example, trying to compare an integer and a string).
23. How do temp variables help optimize code within a loop statement?
Temp variables help optimize code within a loop statement by storing intermediate values needed more than once. This saves time and memory as the value does not need to be calculated or looked up multiple times. Instead, it is stored in the temp variable and accessed whenever needed. This can improve the efficiency of a loop and make it run faster.
Here is a program example of using temp variables to optimize code within a loop statement:
24. Write a program to calculate the number of vowels in a string.
This program determines how many vowels are present in a given string. Each character in the string is iterated using a loop, and then the character's vowel status is determined. If so, it adds one to the counter variable. The total number of vowels in the string is then printed. The program is created in C#.
This code is a C# program determining how many vowels are in a given string. It starts by defining and initializing a variable that contains the string, setting an integer counter to 0, and then running a for each loop to determine if any of the ten possible vowel combinations including upper and lowercase variations match the characters in the given string. The counter is raised by one if one is discovered. After all, letters have been processed, the total number of vowels is presented on the screen for viewing purposes.
25. Write a program to check if a given number is an Armstrong number.
An Armstrong number is a special number in which the sum of its digits, each raised to the power of the total number, i.e.(sum = d^n), equals itself. The code is written in C to check if a given number is an Armstrong number:
The code first takes in the input number from the user and stores it in a variable called num. The same value is also copied to another variable called dig for later use. A while loop iterates through every individual digit of this number by performing a modulus ten operation on it, extracting each of its digits starting from the rightmost one and raising them to power three and summing up with previous values stored in a sum, which is initially 0 at the start of program execution. So when we finish iteration over all get digits, if final summation values match the original input value, i.e. dig ==sum, then we know that the given number is Armstrong else not.
26. Write a program to understand the time complexity to segregate odd and even nodes in a linked list.
This program seeks to understand the time complexity of segregating odd and even nodes in a linked list. In this algorithm, we traverse through the list with two pointers (one for odd-numbered elements and one for even-numbered elements) and rearrange them such that all the odd-numbered elements come before all the even-numbered ones. The following code is written in Java:
The above code segregates the odd and even nodes in a linked list. It uses two pointers, one for pointing at the current node with an odd-valued data element and another for pointing at the current node with an even-valued data element. The pointer pointed by secondHalfStartpoint stores the address of the first encountered even valued element, which will form the start point or head of the new segregated list formed from all even valued elements. A while loop keeps running until either of these pointers becomes null after traversing through the whole list containing both odd and even elements. In each iteration, it changes the next link accordingly such that the linked list only contains odd or even values according to its natural order manner, i.e., odd followed by even if no other condition break exists like null, etc.
27. Write a program to calculate the factorial of a number.
The product of all positive integers that are less than or equal to a certain number is known as the factorial. To determine the factorial of a given number, see the below code in C:
The program is written to calculate the factorial of a given input integer. It starts by declaring two integers, i and n. Then it prints out an instruction for the user to enter an integer number to get its corresponding factorial value. The scanf() function is used along with &n operator to store the entered integer's value into variable "n". Then we check if "n" contains negative values or not using if statement conditions before proceeding further, as factorials of negative numbers do not exist. If "n" holds any negative values, then an error message will be printed via the print () function; otherwise looping control structure (for loop) will start executing from i=1 until the condition (i<=n) turns false. Every time loop gets executed multiplication operation between the previous result and the current iteration's 'i' value gets stored back into the same variables named 'factorial'. Finally, after the completion of all iterations, the final result containing the factorial is displayed on the output screen through print statement inside the last else block.
28. What distinguishes linear data structures from non-linear data structures?
There are several differences between linear and non-linear data structures. Here's how the two data structures are distinguished from each other:
Category | Linear Data Structures | Non-Linear Data Structures |
Storage |
A linear data structure is a data structure that stores items in sequential order. |
A non-linear data structure does not follow any particular order; rather, it has links between arbitrary pairs of objects inside it. |
Movement |
It refers to an arrangement of objects, such as nodes, where the elements are accessible only by moving from one element to another in a single direction, not both forward and backward. |
In non-linear data structures, we can move randomly throughout the tree without following the sequence. |
Time Complexity |
The time complexity for insertion or deletion is always O(n). |
The time complexity for insertion and deleting may vary depending on what algorithm we use, but usually much less than On (O(log n)). |
29. Write a program to find the largest and smallest number in an unsorted integer array.
Using Java, this program will demonstrate how to find the largest and smallest number in an unsorted integer array:
This code finds the largest and smallest number in an unsorted integer array. The program creates an integer variable called "smallest" to store the value of the first element in the array, which is assumed to be the smallest initially. It also creates another integer variable called "largest" that stores the same initial value. Then it iterates through all array elements with a for loop, comparing each number one by one against the largest and smallest variables; if it's larger than any of those two values, they are replaced with new bigger or smaller numbers correspondingly. Finally, after looping through all elements, printout statements displaying what was found on screen: largest and/or smallest numbers depending on circumstances.
30. Write a program to check if two strings are a rotation of each other.
This program checks if two strings are rotations of each other. A string is said to be a rotation of another when it consists of the same letters and in the same order but at a different index position within the string's length. The function compares lengths before determining whether or not they are rotational, ensuring an empty string check is applied for both inputs. The below code is in C:
This code checks if two strings are a rotation of each other by concatenating the first string with itself and then checking to see whether the second string is present as a substring. If so, it returns true; otherwise false. It also has safeguards against undefined behaviour when either of the two strings has zero length or unequal lengths.
Summing Up
As discussed, studying popular coding questions is important for interviews. The above popular coding questions and advanced questions will brush up your knowledge and basic concepts from this topic. This will help the student to understand data structures as well as algorithmic-questions in a simplified way. These conceptual interview questions, trial questions and algorithm-based questions will help students ace the job interview and will bring the candidate closer to his/her dream job.
You may also like to read:
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Blogs you need to hog!
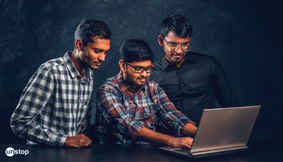
This Is My First Hackathon, How Should I Prepare? (Tips & Hackathon Questions Inside)
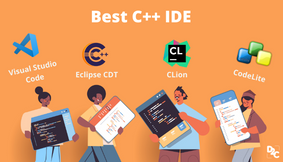
10 Best C++ IDEs That Developers Mention The Most!
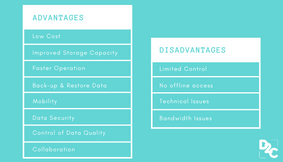
Advantages and Disadvantages of Cloud Computing that you should know!
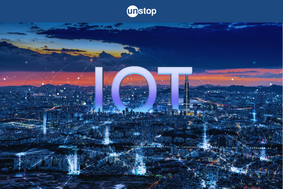
Comments
Add comment