Table of content:
- What Is Python? An Introduction
- What Is The History Of Python?
- Key Features Of The Python Programming Language
- Who Uses Python?
- Basic Characteristics Of Python Programming Syntax
- Why Should You Learn Python?
- Applications Of Python Language
- Advantages And Disadvantages Of Python
- Some Useful Python Tips & Tricks For Efficient Programming
- Python 2 Vs. Python 3: Which Should You Learn?
- Python Libraries
- Conclusion
- Frequently Asked Questions
- It's Python Basics Quiz Time!
Table of content:
- Python At A Glance
- Key Features of Python Programming
- Applications of Python
- Bonus: Interesting features of different programming languages
- Summing up...
- FAQs regarding Python
- Take A Quiz To Rehash Python's Features!
Table of content:
- What Is Python IDLE?
- What Is Python Shell & Its Uses?
- Primary Features Of Python IDLE
- How To Use Python IDLE Shell? Setting Up Your Python Environment
- How To Work With Files In Python IDLE?
- How To Execute A File In Python IDLE?
- Improving Workflow In Python IDLE Software
- Debugging In Python IDLE
- Customizing Python IDLE
- Code Examples
- Conclusion
- Frequently Asked Questions (FAQs)
- How Well Do You Know IDLE? Take A Quiz!
Table of content:
- What Is A Variable In Python?
- Creating And Declaring Python Variables
- Rules For Naming Python Variables
- How To Print Python Variables?
- How To Delete A Python Variable?
- Various Methods Of Variables Assignment In Python
- Python Variable Types
- Python Variable Scope
- Concatenating Python Variables
- Object Identity & Object References Of Python Variables
- Reserved Words/ Keywords & Python Variable Names
- Conclusion
- Frequently Asked Questions
- Rehash Python Variables Basics With A Quiz!
Table of content:
- What Is A String In Python?
- Creating String In Python
- How To Create Multiline Python Strings?
- Reassigning Python Strings
- Accessing Characters Of Python Strings
- How To Update Or Delete A Python String?
- Reversing A Python String
- Formatting Python Strings
- Concatenation & Comparison Of Python Strings
- Python String Operators
- Python String Functions
- Escape Sequences In Python Strings
- Conclusion
- Frequently Asked Questions
- Rehash Python Strings Basics With A Quiz!
Table of content:
- What Is Python Namespace?
- Lifetime Of Python Namespace
- Types Of Python Namespace
- The Built-In Namespace In Python
- The Global Namespace In Python
- The Local Namespace In Python
- The Enclosing Namespace In Python
- Variable Scope & Namespace In Python
- Python Namespace Dictionaries
- Changing Variables Out Of Their Scope & Python Namespace
- Best Practices Of Python Namespace
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Namespaces!
Table of content:
- What Are Logical Operators In Python?
- The AND Python Logical Operator
- The OR Python Logical Operator
- The NOT Python Logical Operator
- Short-Circuiting Evaluation Of Python Logical Operators
- Precedence of Logical Operators In Python
- How Does Python Calculate Truth Value?
- Final Note On How AND & OR Python Logical Operators Work
- Conclusion
- Frequently Asked Questions
- Python Logical Operators Quiz– Test Your Knowledge!
Table of content:
- What Are Bitwise Operators In Python?
- List Of Python Bitwise Operators
- AND Python Bitwise Operator
- OR Python Bitwise Operator
- NOT Python Bitwise Operator
- XOR Python Bitwise Operator
- Right Shift Python Bitwise Operator
- Left Shift Python Bitwise Operator
- Python Bitwise Operations And Negative Integers
- The Binary Number System
- Application of Python Bitwise Operators
- Python Bitwise Operator Overloading
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Bitwise Operators!
Table of content:
- What Is The Print() Function In Python?
- How Does The print() Function Work In Python?
- How To Print Single & Multi-line Strings In Python?
- How To Print Built-in Data Types In Python?
- Print() Function In Python For Values Stored In Variables
- Print() Function In Python With sep Parameter
- Print() Function In Python With end Parameter
- Print() Function In Python With flush Parameter
- Print() Function In Python With file Parameter
- How To Remove Newline From print() Function In Python?
- Use Cases Of The print() Function In Python
- Understanding Print Statement In Python 2 Vs. Python 3
- Conclusion
- Frequently Asked Questions
- Know The print() Function In Python? Take A Quiz!
Table of content:
- Working Of Normal Print() Function
- The New Line Character In Python
- How To Print Without Newline In Python | Using The End Parameter
- How To Print Without Newline In Python 2.x? | Using Comma Operator
- How To Print Without Newline In Python 3.x?
- How To Print Without Newline In Python With Module Sys
- The Star Pattern(*) | How To Print Without Newline & Space In Python
- How To Print A List Without Newline In Python?
- How To Remove New Lines In Python?
- Conclusion
- Frequently Asked Questions
- Think You Can Print Without a Newline in Python? Prove It!
Table of content:
- What Is A Python For Loop?
- How Does Python For Loop Work?
- When & Why To Use Python For Loops?
- Python For Loop Examples
- What Is Rrange() Function In Python?
- Nested For Loops In Python
- Python For Loop With Continue & Break Statements
- Python For Loop With Pass Statement
- Else Statement In Python For Loop
- Conclusion
- Frequently Asked Questions
- Think You Know Python's For Loop? Prove It!
Table of content:
- What Is Python While Loop?
- How Does The Python While Loop Work?
- How To Use Python While Loops For Iterations?
- Control Statements In Python While Loop With Examples
- Python While Loop With Python List
- Infinite Python While Loop in Python
- Python While Loop Multiple Conditions
- Nested Python While Loops
- Conclusion
- Frequently Asked Questions
- Mastered Python While Loop? Let’s Find Out!
Table of content:
- What Are Conditional If-Else Statements In Python?
- Types Of If-Else Statements In Python
- If Statement In Python
- If-Else Statement In Python
- Nested If-Else Statement In Python
- Elif Statement In Python
- Ladder If-Elif-Else Statement In Python
- Short Hand If-Statement In Python
- Short Hand If-Else Statement In Python
- Operators & If-Esle Statement In Python
- Other Statements With If-Else In Python
- Conclusion
- Frequently Asked Questions
- Quick If-Else Statement Quiz– Let’s Go!
Table of content:
- What Is Control Structure In Python?
- Types Of Control Structures In Python
- Sequential Control Structures In Python
- Decision-Making Control Structures In Python
- Repetition Control Structures In Python
- Benefits Of Using Control Structures In Python
- Conclusion
- Frequently Asked Questions
- Control Structures in Python – Are You the Master? Take A Quiz!
Table of content:
- What Are Python Libraries?
- How Do Python Libraries Work?
- Standard Python Libraries (With List)
- Important Python Libraries For Data Science
- Important Python Libraries For Machine & Deep Learning
- Other Important Python Libraries You Must Know
- Working With Third-Party Python Libraries
- Troubleshooting Common Issues For Python Libraries
- Python Libraries In Larger Projects
- Importance Of Python Libraries
- Conclusion
- Frequently Asked Questions
- Quick Quiz On Python Libraries – Let’s Go!
Table of content:
- What Are Python Functions?
- How To Create/ Define Functions In Python?
- How To Call A Python Function?
- Types Of Python Functions Based On Parameters & Return Statement
- Rules & Best Practices For Naming Python Functions
- Basic Types of Python Functions
- The Return Statement In Python Functions
- Types Of Arguments In Python Functions
- Docstring In Python Functions
- Passing Parameters In Python Functions
- Python Function Variables | Scope & Lifetime
- Advantages Of Using Python Functions
- Recursive Python Function
- Anonymous/ Lambda Function In Python
- Nested Functions In Python
- Conclusion
- Frequently Asked Questions
- Python Functions – Test Your Knowledge With A Quiz!
Table of content:
- What Are Python Built-In Functions?
- Mathematical Python Built-In Functions
- Python Built-In Functions For Strings
- Input/ Output Built-In Functions In Python
- List & Tuple Python Built-In Functions
- File Handling Python Built-In Functions
- Python Built-In Functions For Dictionary
- Type Conversion Python Built-In Functions
- Basic Python Built-In Functions
- List Of Python Built-In Functions (Alphabetical)
- Conclusion
- Frequently Asked Questions
- Think You Know Python Built-in Functions? Prove It!
Table of content:
- What Is A round() Function In Python?
- How Does Python round() Function Work?
- Python round() Function If The Second Parameter Is Missing
- Python round() Function If The Second Parameter Is Present
- Python round() Function With Negative Integers
- Python round() Function With Math Library
- Python round() Function With Numpy Module
- Round Up And Round Down Numbers In Python
- Truncation Vs Rounding In Python
- Practical Applications Of Python round() Function
- Conclusion
- Frequently Asked Questions
- Revisit Python’s round() Function – Take The Quiz!
Table of content:
- What Is Python pow() Function?
- Python pow() Function Example
- Python pow() Function With Modulus (Three Parameters)
- Python pow() Function With Complex Numbers
- Python pow() Function With Floating-Point Arguments And Modulus
- Python pow() Function Implementation Cases
- Difference Between Inbuilt-pow() And math.pow() Function
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python’s pow() Function!
Table of content:
- Python max() Function With Objects
- Examples Of Python max() Function With Objects
- Python max() Function With Iterable
- Examples Of Python max() Function With Iterables
- Potential Errors With The Python max() Function
- Python max() Function Vs. Python min() Functions
- Conclusion
- Frequently Asked Questions
- Think You Know Python max() Function? Take A Quiz!
Table of content:
- What Are Strings In Python?
- What Are Python String Methods?
- List Of Python String Methods For Manipulating Case
- List Of Python String Methods For Searching & Finding
- List Of Python String Methods For Modifying & Transforming
- List Of Python String Methods For Checking Conditions
- List Of Python String Methods For Encoding & Decoding
- List Of Python String Methods For Stripping & Trimming
- List Of Python String Methods For Formatting
- Miscellaneous Python String Methods
- List Of Other Python String Operations
- Conclusion
- Frequently Asked Questions
- Mastered Python String Methods? Take A Quiz!
Table of content:
- What Is Python String?
- The Need For Python String Replacement
- The Python String replace() Method
- Multiple Replacements With Python String.replace() Method
- Replace A Character In String Using For Loop In Python
- Python String Replacement Using Slicing Method
- Replace A Character At a Given Position In Python String
- Replace Multiple Substrings With The Same String In Python
- Python String Replacement Using Regex Pattern
- Python String Replacement Using List Comprehension & Join() Method
- Python String Replacement Using Callback With re.sub() Method
- Python String Replacement With re.subn() Method
- Conclusion
- Frequently Asked Questions
- Know How To Replace Python Strings? Prove It!
Table of content:
- What Is String Slicing In Python?
- How Indexing & String Slicing Works In Python
- Extracting All Characters Using String Slicing In Python
- Extracting Characters Before & After Specific Position Using String Slicing In Python
- Extracting Characters Between Two Intervals Using String Slicing In Python
- Extracting Characters At Specific Intervals (Step) Using String Slicing In Python
- Negative Indexing & String Slicing In Python
- Handling Out-of-Bounds Indices In String Slicing In Python
- The slice() Method For String Slicing In Python
- Common Pitfalls Of String Slicing In Python
- Real-World Applications Of String Slicing
- Conclusion
- Frequently Asked Questions
- Quick Python String Slicing Quiz– Let’s Go!
Table of content:
- Introduction To Python List
- How To Create A Python List?
- How To Access Elements Of Python List?
- Accessing Multiple Elements From A Python List (Slicing)
- Access List Elements From Nested Python Lists
- How To Change Elements In Python Lists?
- How To Add Elements To Python Lists?
- Delete/ Remove Elements From Python Lists
- How To Create Copies Of Python Lists?
- Repeating Python Lists
- Ways To Iterate Over Python Lists
- How To Reverse A Python List?
- How To Sort Items Of Python Lists?
- Built-in Functions For Operations On Python Lists
- Conclusion
- Frequently Asked Questions
- Revisit Python Lists Basics With A Quick Quiz!
Table of content:
- What Is List Comprehension In Python?
- Incorporating Conditional Statements With List Comprehension In Python
- List Comprehension In Python With range()
- Filtering Lists Effectively With List Comprehension In Python
- Nested Loops With List Comprehension In Python
- Flattening Nested Lists With List Comprehension In Python
- Handling Exceptions In List Comprehension In Python
- Common Use Cases For List Comprehensions
- Advantages & Disadvantages Of List Comprehension In Python
- Best Practices For Using List Comprehension In Python
- Performance Considerations For List Comprehension In Python
- For Loops & List Comprehension In Python: A Comparison
- Difference Between Generator Expression & List Comprehension In Python
- Conclusion
- Frequently Asked Questions
- Rehash Python List Comprehension Basics With A Quiz!
Table of content:
- What Is A List In Python?
- How To Find Length Of List In Python?
- For Loop To Get Python List Length (Naive Approach)
- The len() Function To Get Length Of List In Python
- The length_hint() Function To Find Length Of List In Python
- The sum() Function To Find The Length Of List In Python
- The enumerate() Function To Find Python List Length
- The Counter Class From collections To Find Python List Length
- The List Comprehension To Find Python List Length
- Find The Length Of List In Python Using Recursion
- Comparison Between Ways To Find Python List Length
- Conclusion
- Frequently Asked Questions
- Know How To Get Python List Length? Prove it!
Table of content:
- List of Methods To Reverse A Python List
- Python Reverse List Using reverse() Method
- Python Reverse List Using the Slice Operator ([::-1])
- Python Reverse List By Swapping Elements
- Python Reverse List Using The reversed() Function
- Python Reverse List Using A for Loop
- Python Reverse List Using While Loop
- Python Reverse List Using List Comprehension
- Python Reverse List Using List Indexing
- Python Reverse List Using The range() Function
- Python Reverse List Using NumPy
- Comparison Of Ways To Reverse A Python List
- Conclusion
- Frequently Asked Questions
- Time To Test Your Python List Reversal Skills!
Table of content:
- What Is Indexing In Python?
- The Python List index() Function
- How To Use Python List index() To Find Index Of A List Element
- The Python List index() Method With Single Parameter (Start)
- The Python List index() Method With Start & Stop Parameters
- What Happens When We Use Python List index() For An Element That Doesn't Exist
- Python List index() With Nested Lists
- Fixing IndexError Using The Python List index() Method
- Python List index() With Enumerate()
- Real-world Examples Of Python List index() Method
- Difference Between find() And index() Method In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python List Indexing? Take A Quiz!
Table of content:
- How To Remove Elements From List In Python?
- The remove() Method To Remove Element From Python List
- The pop() Method To Remove Element From List In Python
- The del Keyword To Remove Element From List In Python
- The clear() Method To Remove Elements From Python List
- List Comprehensions To Conditionally Remove Element From List In Python
- Key Considerations For Removing Elements From Python Lists
- Why We Need to Remove Elements From Python List
- Performance Comparison Of Methods To Remove Element From List In Python
- Conclusion
- Frequently Asked Questions
- Quiz– Prove You Know How To Remove Item From Python Lists!
Table of content:
- How To Remove Duplicates From A List In Python?
- The set() Function To Remove Duplicates From Python List
- Remove Duplicates From Python List Using For Loop
- Using List Comprehension Remove Duplicates From Python List
- Remove Duplicates From Python List Using enumerate() With List Comprehension
- Dictionary & fromkeys() Method To Remove Duplicates From Python List
- Remove Duplicates From Python List Using in, not in Operators
- Remove Duplicates From Python List Using collections.OrderedDict.fromkeys()
- Remove Duplicates From Python List Using Counter with freq.dist() Method
- The del Keyword Remove Duplicates From Python List
- Remove Duplicates From Python List Using DataFrame
- Remove Duplicates From Python List Using pd.unique and np.unipue
- Remove Duplicates From Python List Using reduce() function
- Comparative Analysis Of Ways To Remove Duplicates From Python List
- Conclusion
- Frequently Asked Questions
- Think You Know How to Remove Duplicates? Take A Quiz!
Table of content:
- What Is Python List & How To Access Elements?
- What Is IndexError: List Index Out Of Range & Its Causes In Python?
- Understanding Indexing Behavior In Python Lists
- How to Prevent/ Fix IndexError: List Index Out Of Range In Python
- Handling IndexError Gracefully Using Try-Except
- Debugging Tips For IndexError: List Index Out Of Range Python
- Conclusion
- Frequently Asked Questions
- Avoiding ‘List Index Out of Range’ Errors? Take A Quiz!
Table of content:
- What Is the Python sort() List Method?
- Sorting In Ascending Order Using The Python sort() List Method
- How To Sort Items In Descending Order Using Python sort() List Method
- Custom Sorting Using The Key Parameter Of Python sort() List Method
- Examples Of Python sort() List Method
- What Is The sorted() List Method In Python
- Differences Between sorted() And sort() List Methods In Python
- When To Use sorted() & When To Use sort() List Method In Python
- Conclusion
- Frequently Asked Questions
- Take A Quick Python's sort() Quiz!
Table of content:
- What Is A List In Python?
- What Is A String In Python?
- Why Convert Python List To String?
- How To Convert List To String In Python?
- The join() Method To Convert Python List To String
- Convert Python List To String Through Iteration
- Convert Python List To String With List Comprehension
- The map() Function To Convert Python List To String
- Convert Python List to String Using format() Function
- Convert Python List To String Using Recursion
- Enumeration Function To Convert Python List To String
- Convert Python List To String Using Operator Module
- Python Program To Convert String To List
- Conclusion
- Frequently Asked Questions
- Convert Lists To Strings Like A Pro! Take A Quiz
Table of content:
- What Is Inheritance In Python?
- Python Inheritance Syntax
- Parent Class In Python Inheritance
- Child Class In Python Inheritance
- The __init__() Method In Python Inheritance
- The super() Function In Python Inheritance
- Method Overriding In Python Inheritance
- Types Of Inheritance In Python
- Special Functions In Python Inheritance
- Advantages & Disadvantages Of Inheritance In Python
- Common Use Cases For Inheritance In Python
- Best Practices for Implementing Inheritance in Python
- Avoiding Common Pitfalls in Python Inheritance
- Conclusion
- Frequently Asked Questions
- 💡 Python Inheritance Quiz – Are You Ready?
Table of content:
- What Is The Python List append() Method?
- Adding Elements To A Python List Using append()
- Populate A Python List Using append()
- Adding Different Data Types To Python List Using append()
- Adding A List To Python List Using append()
- Nested Lists With Python List append() Method
- Practical Use Cases Of Python List append() Method
- How append() Method Affects List Performance
- Avoiding Common Mistakes When Using Python List append()
- Comparing extend() With append() Python List Method
- Conclusion
- Frequently Asked Questions
- 🧠 Think You Know Python List append()? Take A Quiz!
Table of content:
- What Is A Linked List In Python?
- Types Of Linked Lists In Python
- How To Create A Linked List In Python
- How To Traverse A Linked List In Python & Retrieve Elements
- Inserting Elements In A Linked List In Python
- Deleting Elements From A Linked List In Python
- Update A Node Of Linked List In Python
- Reversing A Linked List In Python
- Calculating Length Of A Linked List In Python
- Comparing Arrays And Linked Lists In Python
- Advantages & Disadvantages Of Linked List In Python
- When To Use Linked Lists Over Other Data Structures
- Practical Applications Of Linked Lists In Python
- Conclusion
- Frequently Asked Questions
- 🔗 Linked List Logic: Can You Ace This Quiz?
Table of content:
- What Is Extend In Python?
- Extend In Python With List
- Extend In Python With String
- Extend In Python With Tuple
- Extend In Python With Set
- Extend In Python With Dictionary
- Other Methods To Extend A List In Python
- Difference Between append() and extend() In Python
- Conclusion
- Frequently Asked Questions
- Think You Know extend() In Python? Prove It!
Table of content:
- What Is Recursion In Python?
- Key Components Of Recursive Functions In Python
- Implementing Recursion In Python
- Recursion Vs. Iteration In Python
- Tail Recursion In Python
- Infinite Recursion In Python
- Advantages Of Recursion In Python
- Disadvantages Of Recursion In Python
- Best Practices For Using Recursion In Python
- Conclusion
- Frequently Asked Questions
- Recursive Thinking In Python: Test Your Skills!
Table of content:
- What Is Type Conversion In Python?
- Types Of Type Conversion In Python
- Implicit Type Conversion In Python
- Explicit Type Conversion In Python
- Functions Used For Explicit Data Type Conversion In Python
- Important Type Conversion Tips In Python
- Benefits Of Type Conversion In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Type Conversion? Take A Quiz!
Table of content:
- What Is Scope In Python?
- Local Scope In Python
- Global Scope In Python
- Nonlocal (Enclosing) Scope In Python
- Built-In Scope In Python
- The LEGB Rule For Python Scope
- Python Scope And Variable Lifetime
- Best Practices For Managing Python Scope
- Conclusion
- Frequently Asked Questions
- Think You Know Python Scope? Test Yourself!
Table of content:
- Understanding The Continue Statement In Python
- How Does Continue Statement Work In Python?
- Python Continue Statement With For Loops
- Python Continue Statement With While Loops
- Python Continue Statement With Nested Loops
- Python Continue With If-Else Statement
- Difference Between Pass and Continue Statement In Python
- Practical Applications Of Continue Statement In Python
- Conclusion
- Frequently Asked Questions
- Python 'continue' Statement Quiz: Can You Ace It?
Table of content:
- What Are Control Statements In Python?
- Types Of Control Statements In Python
- Conditional Control Statements In Python
- Loop Control Statements In Python
- Control Flow Altering Statements In Python
- Exception Handling Control Statements In Python
- Conclusion
- Frequently Asked Questions
- Mastering Control Statements In Python – Take the Quiz!
Table of content:
- Difference Between Mutable And Immutable Data Types in Python
- What Is Mutable Data Type In Python?
- Types Of Mutable Data Types In Python
- What Are Immutable Data Types In Python?
- Types Of Immutable Data Types In Python
- Key Similarities Between Mutable And Immutable Data Types In Python
- When To Use Mutable Vs Immutable In Python?
- Conclusion
- Frequently Asked Questions
- Quiz Time: Mutable vs. Immutable In Python!
Table of content:
- What Is A List?
- What Is A Tuple?
- Difference Between List And Tuple In Python (Comparison Table)
- Syntax Difference Between List And Tuple In Python
- Mutability Difference Between List And Tuple In Python
- Other Difference Between List And Tuple In Python
- List Vs. Tuple In Python | Methods
- When To Use Tuples Over Lists?
- Key Similarities Between Tuples And Lists In Python
- Conclusion
- Frequently Asked Questions
- 🧐 Lists vs. Tuples Quiz: Test Your Python Knowledge!
Table of content:
- Introduction to Python
- Downloading & Installing Python, IDLE, Tkinter, NumPy & PyGame
- Creating A New Python Project
- How To Write Python Hello World Program In Python?
- Way To Write The Hello, World! Program In Python
- The Hello, World! Program In Python Using Class
- The Hello, World! Program In Python Using Function
- Print Hello World 5 Times Using A For Loop
- Conclusion
- Frequently Asked Questions
- 👋 Python's 'Hello, World!'—How Well Do You Know It?
Table of content:
- Algorithm Of Python Program To Add To Numbers
- Standard Program To Add Two Numbers In Python
- Python Program To Add Two Numbers With User-defined Input
- The add() Method In Python Program To Add Two Numbers
- Python Program To Add Two Numbers Using Lambda
- Python Program To Add Two Numbers Using Function
- Python Program To Add Two Numbers Using Recursion
- Python Program To Add Two Numbers Using Class
- How To Add Multiple Numbers In Python?
- Add Multiple Numbers In Python With User Input
- Time Complexities Of Python Programs To Add Two Numbers
- Conclusion
- Frequently Asked Questions
- 💡 Quiz Time: Python Addition Basics!
Table of content:
- Swapping in Python
- Swapping Two Variables Using A Temporary Variable
- Swapping Two Variables Using The Comma Operator In Python
- Swapping Two Variables Using The Arithmetic Operators (+,-)
- Swapping Two Variables Using The Arithmetic Operators (*,/)
- Swapping Two Variables Using The XOR(^) Operator
- Swapping Two Variables Using Bitwise Addition and Subtraction
- Swap Variables In A List
- Conclusion
- Frequently Asked Questions (FAQs)
- Quiz To Test Your Variable Swapping Knowledge
Table of content:
- What Is A Quadratic Equation? How To Solve It?
- How To Write A Python Program To Solve Quadratic Equations?
- Python Program To Solve Quadratic Equations Directly Using The Formula
- Python Program To Solve Quadratic Equations Using The Complex Math Module
- Python Program To Solve Quadratic Equations Using Functions
- Python Program To Solve Quadratic Equations & Find Number Of Solutions
- Python Program To Plot Quadratic Functions
- Conclusion
- Frequently Asked Questions
- Quadratic Equations In Python Quiz: Test Your Knowledge!
Table of content:
- What Is Decimal Number System?
- What Is Binary Number System?
- What Is Octal Number System?
- What Is Hexadecimal Number System?
- Python Program to Convert Decimal to Binary, Octal, And Hexadecimal Using Built-In Function
- Python Program To Convert Decimal To Binary Using Recursion
- Python Program To Convert Decimal To Octal Using Recursion
- Python Program To Convert Decimal To Hexadecimal Using Recursion
- Python Program To Convert Decimal To Binary Using While Loop
- Python Program To Convert Decimal To Octal Using While Loop
- Python Program To Convert Decimal To Hexadecimal Using While Loop
- Convert Decimal To Binary, Octal, And Hexadecimal Using String Formatting
- Python Program To Convert Binary, Octal, And Hexadecimal String To A Number
- Complexity Comparison Of Python Programs To Convert Decimal To Binary, Octal, And Hexadecimal
- Conclusion
- Frequently Asked Questions
- 💡 Decimal To Binary, Octal & Hex: Quiz Time!
Table of content:
- What Is A Square Root?
- Python Program To Find The Square Root Of A Number
- The pow() Function In Python Program To Find The Square Root Of Given Number
- Python Program To Find Square Root Using The sqrt() Function
- The cmath Module & Python Program To Find The Square Root Of A Number
- Python Program To Find Square Root Using The Exponent Operator (**)
- Python Program To Find Square Root With A User-Defined Function
- Python Program To Find Square Root Using A Class
- Python Program To Find Square Root Using Binary Search
- Python Program To Find Square Root Using NumPy Module
- Conclusion
- Frequently Asked Questions
- 🤓 Think You Know Square Roots In Python? Take A Quiz!
Table of content:
- Understanding the Logic Behind the Conversion of Kilometers to Miles
- Steps To Write Python Program To Convert Kilometers To Miles
- Python Program To Convert Kilometer To Miles Without Function
- Python Program To Convert Kilometer To Miles Using Function
- Python Program to Convert Kilometer To Miles Using Class
- Tips For Writing Python Program To Convert Kilometer To Miles
- Conclusion
- Frequently Asked Questions
- 🧐 Mastered Kilometer To Mile Conversion? Prove It!
Table of content:
- Why Build A Calculator Program In Python?
- Prerequisites To Writing A Calculator Program In Python
- Approach For Writing A Calculator Program In Python
- Simple Calculator Program In Python
- Calculator Program In Python Using Functions
- Creating GUI Calculator Program In Python Using Tkinter
- Conclusion
- Frequently Asked Questions
- 🧮 Calculator Program In Python Quiz!
Table of content:
- The Calendar Module In Python
- Prerequisites For Writing A Calendar Program In Python
- How To Write And Print A Calendar Program In Python
- Calendar Program In Python To Display A Month
- Calendar Program In Python To Display A Year
- Conclusion
- Frequently Asked Questions
- Calendar Program In Python – Quiz Time!
Table of content:
- What Is The Fibonacci Series?
- Pseudocode Code For Fibonacci Series Program In Python
- Generating Fibonacci Series In Python Using Naive Approach (While Loop)
- Fibonacci Series Program In Python Using The Direct Formula
- How To Generate Fibonacci Series In Python Using Recursion?
- Generating Fibonacci Series In Python With Dynamic Programming
- Fibonacci Series Program In Python Using For Loop
- Generating Fibonacci Series In Python Using If-Else Statement
- Generating Fibonacci Series In Python Using Arrays
- Generating Fibonacci Series In Python Using Cache
- Generating Fibonacci Series In Python Using Backtracking
- Fibonacci Series In Python Using Power Of Matix
- Complexity Analysis For Fibonacci Series Programs In Python
- Applications Of Fibonacci Series In Python & Programming
- Conclusion
- Frequently Asked Questions
- 🤔 Think You Know Fibonacci Series? Take A Quiz!
Table of content:
- Different Ways To Write Random Number Generator Python Programs
- Random Module To Write Random Number Generator Python Programs
- The Numpy Module To Write Random Number Generator Python Programs
- The Secrets Module To Write Random Number Generator Python Programs
- Understanding Randomness and Pseudo-Randomness In Python
- Common Issues and Solutions in Random Number Generation
- Applications of Random Number Generator Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python's Random Module? Prove It!
Table of content:
- What Is A Factorial?
- Algorithm Of Program To Find Factorial Of A Number In Python
- Pseudocode For Factorial Program in Python
- Factorial Program In Python Using For Loop
- Factorial Program In Python Using Recursion
- Factorial Program In Python Using While Loop
- Factorial Program In Python Using If-Else Statement
- The math Module | Factorial Program In Python Using Built-In Factorial() Function
- Python Program to Find Factorial of a Number Using Ternary Operator(One Line Solution)
- Python Program For Factorial Using Prime Factorization Method
- NumPy Module | Factorial Program In Python Using numpy.prod() Function
- Complexity Analysis Of Factorial Programs In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Factorials In Python? Take A Quiz!
Table of content:
- What Is Palindrome In Python?
- Check Palindrome In Python Using While Loop (Iterative Approach)
- Check Palindrome In Python Using For Loop And Character Matching
- Check Palindrome In Python Using The Reverse And Compare Method (Python Slicing)
- Check Palindrome In Python Using The In-built reversed() And join() Methods
- Check Palindrome In Python Using Recursion Method
- Check Palindrome In Python Using Flag
- Check Palindrome In Python Using One Extra Variable
- Check Palindrome In Python By Building Reverse, One Character At A Time
- Complexity Analysis For Palindrome Programs In Python
- Real-World Applications Of Palindrome In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Palindromes? Take A Quiz!
Table of content:
- Best Python Books For Beginners
- Best Python Books For Intermediate Level
- Best Python Books For Experts
- Best Python Books To Learn Algorithms
- Audiobooks of Python
- Best Books To Learn Python And Code Like A Pro
- To Learn Python Libraries
- Books To Provide Extra Edge In Python
- Python Project Ideas - Reference
- Quiz To Rehash Your Knowledge Of Python Books!
Python Bitwise Operators | Positive & Negative Numbers (+Examples)
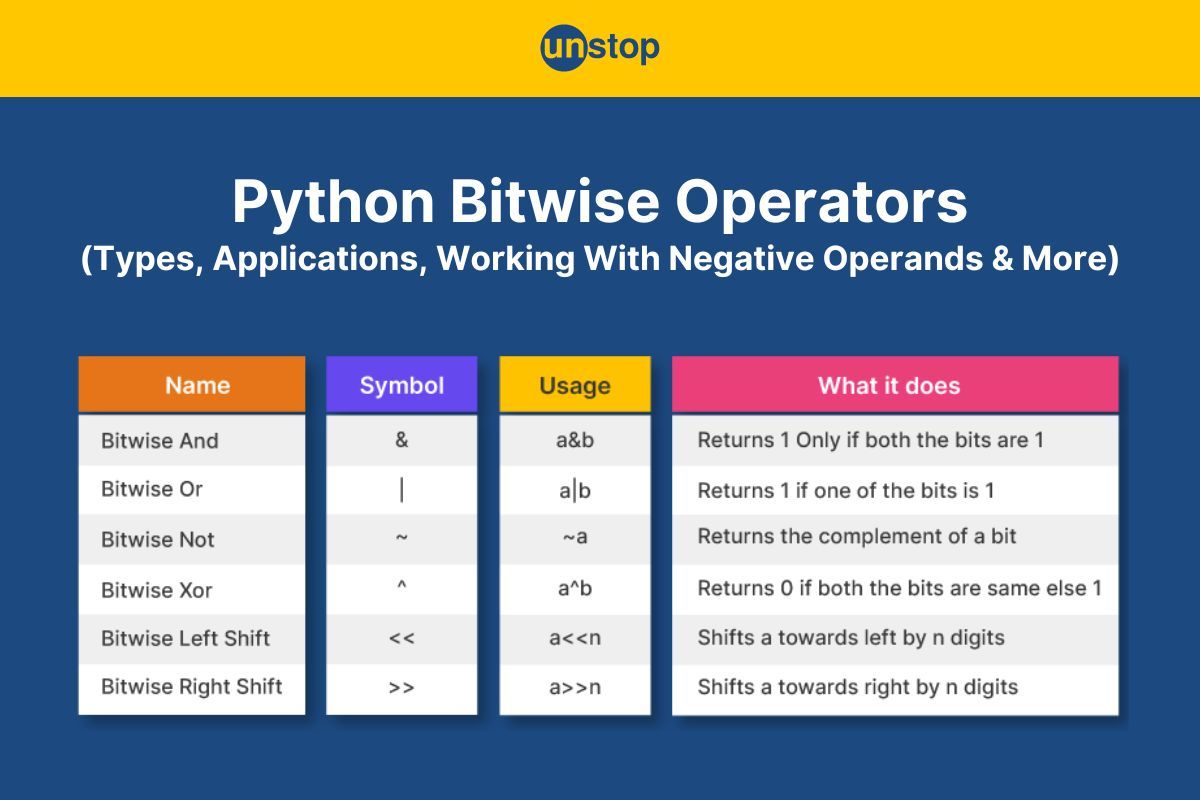
Operators in programming languages allow you to manipulate data in various ways, from performing mathematical calculations to modifying values stored in variables. We have many different types of operators, such as arithmetic, comparison, logical, and bitwise operators in Python programming. Each type serves its purpose, making them indispensable for data manipulation and computation.
In this article, we’ll focus on Python bitwise operators, which operate at the binary level on the data. While these might seem difficult to grasp at first glance, they are incredibly powerful and efficient, especially in low-level system programming, network protocols, cryptography, and optimization tasks.
What Are Bitwise Operators In Python?
Bitwise operators in Python are used to perform operations on the binary representations of integers. These operators treat each integer as a sequence of bits (0s and 1s) and manipulate them directly. This allows for precise and low-level control over data, and in certain cases, bitwise operations are more efficient than arithmetic operations.
Python Bitwise operators fall into two categories:
- Logical Bitwise Operators: These are used to perform logical operations/ calculations on integers, i.e., between right operand bits and left operand bits. The Bitwise logical operators include- AND, OR, NOT, and XOR.
- Bitwise Shift Operators: Also referred to as bit shift operators, these are used to move all the operand's bits left or right a certain amount of times. These are referred to as bitwise shift operations carried on by bitwise shift operators- the left shift operator and the right shift operator, respectively.
When using Python bitwise operators, the operands are converted into binary representations of integers, and operations are performed on each bit or matching pair of bits. After that, the result in binary format is converted into the original integer format/ decimal integer literals.
When To Use Python Bitwise Operators?
Bitwise operators are handy in scenarios that require direct manipulation of data at the bit level. Some common use cases of Python bitwise operators include:
- Flags and Bit Manipulation: Use individual bits as flags to represent different states or options in programs.
- Optimizations: Bitwise operations can outperform standard arithmetic operations in certain cases, especially with large datasets.
- Data Compression: Algorithms that reduce data size often rely on bitwise manipulation.
- Cryptography: Encryption algorithms commonly employ bitwise operations to secure data.
- Low-Level System Programming: Hardware interactions often require operations at the bit level for efficiency and precision.
List Of Python Bitwise Operators
The following table outlines the six bitwise operators in Python, along with their symbols, syntax, and descriptions. In the sections that follow, we’ll look at each operator in more detail.
Name |
Operator |
Syntax |
Description |
Bitwise AND |
& |
A & B |
Compares each bit of two integers and returns 1 only if both bits are 1. If either bit is 0, the result is 0. |
Bitwise OR |
| |
A | B |
If any of the operand bit is 1 then the result is 1 else the result is 0. |
Bitwise NOT |
~ |
~A |
This is an unary bitwise operator that flips individual bits of integer/ operand. |
Bitwise XOR Operator |
^ |
A ^ B |
If the bits for both the left operand and right operand are either 0 or 1 then the result is 0; else, the result is 1. |
Right Shift Operator |
>> |
A>>n |
This bit shift operator shifts the value of the left operand to the right by the number of bits given by the right operand. |
Left Shift Operator |
<< |
A<<n |
The bit shift operator shifts the value of the left operand to the left by the number of bits given by the right operand. |
AND Python Bitwise Operator
The bitwise AND operator (&) in Python compares each bit position of two numbers/ integers and returns a result where each corresponding bit is set to 1 only if both bits in that position are 1. So, this binary operator works on the binary representations of integers, comparing pairs of bits at the same position.
Syntax Of AND Bitwise Operator In Python:
Result = operand1 & operand 2
Here,
- operand 1 and operand 2 represent the left operand and the right operand being compared, respectively.
- The ampersand symbol (&) represents the AND operator, which conducts logical conjunction on the operands.
- The result variable stores the output of the Bitwise AND operation.
Let's look at a truth table for the bitwise AND operation:
Operand 1 |
Operand 2 |
Operand 1 & Operand 2 |
0 |
0 |
0 |
0 |
1 |
0 |
1 |
0 |
0 |
1 |
1 |
1 |
Example: Consider two nonnegative integers, i.e., a = 12 and b = 25.
The binary representation of these numbers is as follows:
a = 12 (Binary: 1100)
b = 25 (Binary: 11001)
Performing Bitwise AND operation:
01100
& 11001
--------------
01000 (Result: 8 in decimal)
The simple Python program example below illustrates the code implementation of how the Python bitwise AND operator works.
Code Example:
#Declaring and initializing two variables
data1=6
data2=10
#Using the Bitwise AND operator to compare the variables
final=data1 & data2
#Printing the output of the AND operation
print(final)
Output:
2
Explanation:
In the simple Python code example,
- We first declare and initialize two integer variables, data1 and data2, with the values 6 and 10, respectively.
- Next, we apply the Bitwise logical operator AND (&) to perform the AND bitwise calculation on the binary representations of data1 and data2.
- The binary representation of data1 (6) is 0110, and the binary representation of data2 (10) is 1010.
- The outcome of the bitwise AND operation on these bits is 0010 in binary, which is equivalent to the decimal number 2.
- We store the outcome of the bitwise AND operation in the final variable and print it to the console using the print() statement.
An Explanation Of The Mechanism:
0110 (Binary number 6)
& 1010 (Binary number 10)
-------------------
0010 (Result after AND operation, i.e., 2)
OR Python Bitwise Operator
The bitwise OR operator in Python, represented by the pipe symbol (|), performs a bitwise inclusive OR operation between the binary representations of two integers (right and left operands). The binary bitwise operator compares each bit position of both operands and sets the corresponding result bit to 1 if either of the bits is 1; otherwise, it sets the result bit to 0.
This operator is commonly used in low-level programming tasks, such as setting specific bits within binary data or dealing with flags.
OR Python Bitwise Operator Syntax:
Result = operand1 | operand 2
Here,
- Terms operand1 and operand2 are the left operand and right operand, respectively.
- The pipe symbol (|) represents the OR operator, which performs the bitwise OR operation.
- The outcome of the bitwise OR operation is stored in the variable Result.
Truth Table:
Below is the truth table for the Python Bitwise OR operator, indicating when the operation results in 1 or 0 bit value.
Operand 1 |
Operand 2 |
Operand 1 | Operand 2 |
0 |
0 |
0 |
0 |
1 |
1 |
1 |
0 |
1 |
1 |
1 |
1 |
Example: Let’s take two integers, a = 12 and b = 25, and perform a bitwise OR operation on them.
a = 12 → 01100
b = 25 → 11001
Performing bitwise OR:
01100
| 11001
-------------
11101 (Result: 29 in decimal)
The basic Python program example below gives the code implementation of applying the Python Bitwise OR operation.
Code Example:
#Creating two variables/ operands
data1=6
data2=10
#Applying the bitwise OR operator
final=data1 | data2
print(final)
Output:
14
Explanation:
In the basic Python code example-
- We declare two integer variables data1 and data2 with values 6 and 10, respectively.
- The bitwise OR operator (|) is applied to their binary representations:
- data1 = 0110 (binary of 6)
- data2 = 1010 (binary of 10)
- The result is 1110 in binary, which is equivalent to the decimal number 14.
- We store the result of the bitwise OR operation in the variable final and print it ot the console.
An Explanation Of The Mechanism:
0110 (Binary number 6)
| 1010 (Binary number 10)
-------------------
1110 (Result after OR operation, i.e.,14)
Also read- Python IDLE | The Ultimate Beginner's Guide With Images & Codes!
NOT Python Bitwise Operator
The bitwise NOT operator (~) in Python performs a bitwise inversion (also called one's complement) on the binary representation of a single operand. This means that this unary Bitwise operator flips each bit of the operand: 0 becomes 1, and 1 becomes 0.
The result is the bitwise complement of the original number. In Python, this operator works in conjunction with the two's complement representation for signed integers, effectively yielding the negative of the number.
NOT Python Bitwise Operator Syntax:
result = ~ operand
Here,
- The operand is the integer on which the bitwise NOT operation is performed.
- The tilde symbol (~) represents the unary bitwise operator, NOT in Python.
- The result variable stores the output of Bitwise NOT operation.
Example: Let’s take an integer a = 12.
The binary representation of the numbers is:
a = 12 → 01100
Performing bitwise NOT:
~01100
------------
-01101 (Result: -13 in decimal)
Now, look at the Python program example that gives the code implementation of the same.
Example code:
#Creating the single operand
data1=6
#Applying the NOT operation
final= ~ data1
print(final)
Output:
-7
Explanation:
In the Python code example,
- We first declare and initialize an integer variable named data1 with the value 6.
- Then, we apply the Bitwise NOT operator (~) on the binary representation of data1, which flips each bit:
- 0110 becomes 1001 (bitwise inversion).
- In Python, the result is represented as a signed integer using two's complement, i.e., ~1001 = -7.
- We store the result in the variable final and print it to the console.
An Explanation Of The Working Mechanism:
(sign bit)0 110 (Binary number 6)
~
-------------------
(sign bit)1 001 (Intermediate result after one's complement operation)
Two’s complement of 1 =~(000)=111 (-7) —Final Result.
Here,
- One's Complement Operation: The one's complement operation (~) flips each bit; 0s become 1s, and 1s become 0s.
- Intermediate Result (One’s Complement Representation): The one's complement operation on the binary number (sign bit)0 110 results in the binary representation (sign bit)1001.
- Final Result (Two's Complement Representation): To obtain the 8-bit two's complement representation of a negative number, you add 1 to the one's complement result. Adding 1 to (sign bit)1 001 results in (sign bit)1 010.
XOR Python Bitwise Operator
The bitwise XOR operator (^) in Python performs a bitwise exclusive OR operation on the binary representations of two operands. It compares each pair of corresponding bits, and the result at each bit position is 1 if the bits are different; otherwise, it is 0.
This bitwise operation results in a new binary number where each bit is the result of the XOR operation between the corresponding bits of the original operands. The XOR operator is commonly used in fields like cryptography, error detection, and toggling specific bits.
XOR Python Bitwise Operator Syntax:
result = operand1 ^ operand 2
Here,
- The variables operand1 and operand2 are the two integers on which the XOR operation is performed.
- Caret symbol (^) represents the bitwise XOR operator in Python.
- The result variable stores the output of the Bitwise XOR operation.
Truth Table:
Operand 1 |
Operand 2 |
Operand 1 ^ Operand 2 |
0 |
0 |
0 |
0 |
1 |
1 |
1 |
0 |
1 |
1 |
1 |
0 |
Example: Consider two integers, say, a = 12 and b = 25.
Their binary representation is:
a = 12 → 1100 (binary)
b = 25 → 11001 (binary)
Performing bitwise XOR:
01100
^ 11001
------------
10101 (Result: 21 in decimal)
Below is an example Python program that shows the code implementation of the Bitwise XOR operator in Python.
Code Example:
#Creating the Python variables
data1=6
data2=10
#Applying Bitwise XOR operation of the two operands
final=data1 ^ data2
print(final)
Output:
12
Explanation:
In the example Python code above,
- We first declare and initialize two integer variables, data1 with the value 6 (binary: 0110) and data2 with 10 (binary: 1010).
- Then, we apply the XOR operation (^), which compares the corresponding bits of data1 and data2.
- It results in 1100 (binary), which is equivalent to the decimal number 12.
- We store this in the variable final and print it.
The Working Mechanism Of Bitwise XOR:
0110 (Binary digits for number 6)
^ 1010 (Binary digits for number 10)
-------------------
1100 (Result after XOR operation)
Right Shift Python Bitwise Operator
In Python, the right shift operator (>>) performs a bitwise right shift operation on the binary representation of a single operand. It shifts the bits of a number to the right by a specified number of positions, functioning similarly to the floor division operator. Bits that are shifted beyond the rightmost position are discarded, and zero bits are introduced on the left.
The right bitwise shift effectively divides the operand by 2 to the power of n, where n is the number of positions shifted. This operator is useful for optimizing calculations involving powers of two and is commonly used in scenarios such as integer division by powers of two or extracting specific bits from an integer.
Bitwise Operator Syntax:
Result = operand1 >> n
Here,
- The operand1 refers to the integer whose bits are to be shifted, and the number pf position is given by number n.
- The double greater-than symbol (>>) represents the right bitwise shift operator in Python.
- The result variable stores the output of the Bitwise right shift operation.
For Example: Consider two integers a = 12 and b = 3.
The binary representation is:
a = 12 → 1100 (binary)
b = 3 → 11 (binary)
Performing Bitwise Right Shift:
1100
>> 1
----------
0110 (Result: 6 in decimal)
This shows how the bits of the binary representation of the number are shifted to the right by 3. Below is a Python example program showcasing its implementation.
Code Example:
#Creating two variables
data1=6
n=1
#Employing the Bitwise right shift operator
final=data1>>n
print(final)
Output:
3
Explanation:
In the Python example code above-
- We initialize data1 variable with the value 6 (binary: 0110) and another integer variable n to 1.
- Then, we apply the right shift operator (>>) to shift the bits of data1 to the right by n positions.
- After shifting the binary representation of data1 (0110) by 1 position, we get 0011, which is equivalent to 3 in decimal.
- We store the outcome in the variable final and print it.
The Working Mechanism Of Right Bitwise Shift Operator:
0110 (Binary number 6)
>> 1
-------------------
0011 (Result after right shift, i.e., 3)
Here,
- Right Shifting: The right shift operation (>>1) involves moving each bit of the binary number to the right by one position.
- Result: Shifting 0110 (binary for 6) to the right by one position results in 0011, which represents 3 in decimal. The right shift operation effectively divides the number by 2 (rounded towards zero).
Left Shift Python Bitwise Operator
In Python, the left shift operator (<<) performs a bitwise left shift operation on the binary representation of a single operand. This operator shifts the bits to the left by a specified number of positions. Bits that are shifted beyond the leftmost position are discarded, and zero bits are introduced on the right.
The left shift operation effectively multiplies the operand by 2 to the power of n, where n is the number of positions shifted. This operator is commonly used for efficient multiplication by powers of two and for various bit manipulation tasks.
Syntax:
result = operand1 << n
Here,
- The operand1 is the integer whose bits are to be shifted and n is the number of positions to shift.
- The double less-than symbol (<<) represents the left shift operator in Python.
- The result variable stores the output of the bitwise left shift operation.
For example, consider two integers, i.e., a = 12 and b = 3, whose binary representation is as follows:
a = 12 → 1100 (binary)
b = 3 → 11 (binary)
Performing Bitwise Left Shift:
1100
<< 3
-----------
110000 (Result: 96 in decimal)
Look at the sample Python program below for a better understanding.
Code Example:
#Declaring and initializing Python variables
data1=6
n=1
#Applying Python left shift bitwise operations on integers
final=data1<< n
print(final)
Output:
12
Explanation:
In the sample Python code above-
- We first declare and initialize an integer variable data1 with the value 6 (binary: 0110), and another integer variable n with the value 1.
- Next, we apply the Python bitwise left shift operator (<<) to to shift the bits of data1 to the left by n positions.
- The binary representation of data1 is 0110 and after performing a left shift by 1 position results in 1100, which is equivalent to 12 in decimal.
- We store the result in the variable final and print the same.
The Working Mechanism:
0110 (Binary number 6)
<< 1
-------------------
1100 (Result after left shift, i.e.,12)
Here,
- Left Shifting: The left shift operation (<<1) involves moving each bit to the left by one position. In this case, the entire binary form representation of 6 is shifted to the left by one position.
- Result: Shifting 0110 (binary for 6) to the left by one position effectively multiplies the number by 2. Thus, the left shift of 0110 yields 1100, representing 12 in decimal.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Python Bitwise Operations And Negative Integers
In Python, integers are typically represented using a fixed number of bits (32 or 64 bits). When performing bitwise operations on negative integers, it’s crucial to understand their representation in binary, which uses a method called two's complement. This method represents negative numbers by inverting all bits of the corresponding positive number and adding 1.
Here's a brief overview of two's complement representation:
- Positive Integers: They are represented as usual in binary. For example, the decimal number 5 is represented as 101 in binary.
- Negative Integers (Two's Complement): They are represented using the two's complement. Here,
- We invert all the bits of the positive binary representation/ counterpart and add 1.
- For example, say we want to represent -5. Its binary representation is 101, and the corresponding inverted bits are 010. By adding 1, we get 011 (resulting in the two's complement representation of -5).
AND Python Bitwise Operator
The AND bitwise operator in Python works the same way for negative integers as it does for positive/ nonnegative integers. Even though negative integers in Python are represented using two's complement, the AND operator compares each bit, and the result is 1 if both bits are 1; otherwise, it is 0.
Code Example:
#Creating two variables with negative values
data1= -3
data2= -2
#AND-ing the two negative value variables
final = data1 & data2
print(final)
Output:
-4
Explanation:
In the Python program sample above-
- We first declare and initialize two integer variables, data1 and data2, with the negative values -3 and -2, respectively.
- Next, we apply the Bitwise AND operator (&) to perform a bitwise AND operation between the binary representations of data1 and data2.
- Two's complement of -3: 1101
- Two's complement of -2: 1110
- Performing 1101 & 1110 gives 1100, which is -4 in two's complement.
- We store the result in the variable final and print it.
The Working Mechanism:
-3 Two’s complement of -3 in binary = one’s complement of 2=~(010)=101
-2 Two’s complement of -2 in binary = one’s complement of 1=~(001)=110
(Sign bit)1 101 (-3 binary)
& (Sign bit)1 110 (-2 binary)
—-----------—-----------------
(Sign bit)1 100 (-4 binary)
Final output = -4
OR Python Bitwise Operator
The OR bitwise operator in Python works consistently for both negative and positive/ nonnegative integers. The crucial aspect is understanding the two's complement bit sequences representation of negative integers. The operator compares each bit and sets the result bit to 1 if at least one corresponding bit is 1.
Code Example:
#Initializing variables with negative values
data1= -3
data2= -2
final = data1 | data2
print(final)
Output:
-1
Explanation:
In the Python program above,
- We first declare and initialize two integer variables, data1 and data2, with the values -3 and -2, respectively.
- Next, we apply the Bitwise OR operator (|) to the binary representations of data1 and data2.
- The two's complement representation of -3 is obtained by taking the bitwise NOT (one's complement) of 2 and adding 1, i.e., ~(010) + 1 = 101.
- The two's complement representation of -2 is obtained by taking the bitwise NOT (one's complement) of 1 and adding 1: ~(001) + 1 = 110.
- Performing 1101 | 1110 results in 1111, which is -1 in two's complement. We store it in variable final ad print it as shown in the output.
The Working Mechanism:
-3 Two’s complement of -3 in binary = one’s complement of 2=~(010)=101
-2 Two’s complement of -2 in binary = one’s complement of 1=~(001)=110
(Sign bit)1 101 (-3 binary)
| (Sign bit)1 110 (-2 binary)
—---------------------------
(Sign bit)1 111 (-1 binary) Intermediate result.
Final output = -1
NOT Python Bitwise Operator
The NOT bitwise operator in Python inverts all bits, including the sign bit, for both negative and positive integers.
Code Example:
#Creating a variable with negative integer value
data1=-3
#Applying the NOT operator to the negative value
final=~data1
print(final)
Output:
2
Explanation:
In the example Python code above-
- We first declare and initialize an integer variable data1 with the value -3.
- Next, we apply the Bitwise NOT operator (~) to the binary representation of data1.
- The two's complement representation of -3 is obtained by taking the bitwise NOT (one's complement) of 2 and adding 1, i.e., ~(010) + 1 = 101.
- The Bitwise NOT operation is performed on each bit of the two's complement representation, i.e., ~101. And the result of this operation is 010 in binary, which is equivalent to the decimal number 2.
- The outcome of this NOT operation is stored in the final variable.
The Working Mechanism:
-3 Two’s complement of -3 in binary = one’s complement of 2=~(010)=101
signbit(1) 101 (-3 binary)
~
—--—--------------
signbit(0) 010 (2 binary)
Final result=2
XOR Python Bitwise Operator
Similar to the OR operator, the XOR bitwise operator behaves consistently for negative and positive integers. The sign bit is handled similarly to other bits during this operation. The XOR operator (^) sets a bit to 1 if it is set in either operand, but not both.
Code Example:
#Assigning variables negative numeric values
data1= -3
data2= -2
#Applying XOR operation
final = data1 ^ data2
print(final)
Output:
3
Explanation:
In the Python program above-
- We first declare and initialize two integer variables, data1 and data2, with the values -3 and -2, respectively.
- Next, we apply the Bitwise XOR operator (^) between the binary representations of data1 and data2.
- The two's complement representation of -3 is obtained by taking the bitwise NOT (one's complement) of 2 and adding 1, i.e., ~(010) + 1 = 101.
- The two's complement representation of -2 is obtained by taking the bitwise NOT (one's complement) of 1 and adding 1, i.e., ~(001) + 1 = 110.
- The Bitwise XOR operation is performed on original bit patterns, i.e., 101 ^ 110. The result is 011 in binary, which is equivalent to the decimal number 3.
- The result of this XOR operation is stored in the final variable which we print.
The Working Mechanism:
Two’s complement of -3 in binary: 1…1011…101
Two’s complement of -2 in binary: 1…1101…110
1...101 (Two’s complement of -3)
^ 1...110 (Two’s complement of -2)
-------------------
0...011 (Result after XOR operation)
Final output = 3
Python Bitwise Right Shift Operator
Again, the right shift operator works consistently for both negative and positive integers. In two's complement representation, it shifts the bits of a number to the right by a specified number of positions. Depending on the sign bit, zero bits or sign bits are filled in from the left.
Code Example:
#Creating two variables with positive and negative numeric values
data1= -3
n= 1
#Applying the right shift operation on variables created above
final = data1>>n
print(final)
Output:
-2
Explanation:
In the Python program above,
- We first declare and initialize an integer variable, data1, with the value -3 and another integer variable, n, with the value 1.
- Next, we apply the right shift operator (>>) to the binary representation of data1.
- Next, the two's complement representation of -3 is obtained by taking the bitwise NOT (one's complement) of 2 and adding 1, i.e., ~(010) + 1 = 101.
- The Bitwise right shift operation is performed on each bit of the two's complement representation: 101 >> 1.
- The result is 110 in binary, which is equivalent to the decimal value -2.
- The result of this right shift operation is stored in the variable final.
The Working Mechanism:
Two’s complement of -3 in binary, i.e., 1 101 (Sign bit followed by binary representation of 3)
Two’s complement of 2 in binary, i.e., (010)=101 (010)=101 (One's complement of binary representation of 2)
1...101 (Two’s complement of -3)
>> 1
-------------------
1...110 (Result after right shift)
Python Bitwise Left Shift Operator
The left shift operator also works consistently for both negative and positive integers. In two's complement representation, it shifts the bits of a number to the left by a specified number of positions. Zero bits are filled in from the right, irrespective of the sign bit.
Code Example:
#Creating variables
data1= -3
n= 1
#Applying left shift operator
final = data1<< n
print(final)
Output:
-6
Explanation:
In the sample Python program above-
- We first declare and initialize an integer variable data1 with the value -3, and another integer variable n with the value 1.
- Next, we apply the left shift operator (<<) to the binary representation of data1.
- The two's complement representation of -3 is obtained by taking the bitwise NOT (one's complement) of 2 and adding 1: ~(010) + 1 = 101.
- The Bitwise left shift operation is performed on each bit of the two's complement representation: 101 << 1.
- The result is 010 in binary, which is equivalent to the decimal form number -6.
- The result of this left shift operation is stored in the variable final.
The Working Mechanism:
-3 Two’s complement of -3 in binary = Two’s complement of 2=~(010)=101
1...101 (Two’s complement of -3)
<< 1
-------------------
1...010 (Result after left shift)
Also read- Flask vs Django: Understand Which Python Framework You Should Choose
The Binary Number System
The binary number system is a base-2 numeral system that uses only two digits, 0 and 1, to represent numbers. It serves as the foundational numeral system in computers and digital systems. In contrast, the decimal system (base-10) employs ten digits (0 to 9), relying on powers of 2 for binary representation.
Key Concepts
- Bit: The fundamental unit in the binary system, a bit (short for binary digit) can hold a value of either 0 or 1.
- Conversion to Decimal: To convert a binary number to decimal, you sum up the products of each bit with its corresponding power of 2. For example, the binary number 1011 is equivalent to 1⋅23+0⋅22+1⋅21+1⋅201⋅23+0⋅22+1⋅21+1⋅20, which equals 11 in decimal.
Integers are represented and interpreted in a binary number system as follows:
Signed integers use a bit to indicate the sign of the number, typically using the two's complement representation.
- In an 8-bit signed integer, the most significant bit (MSB) is the sign bit, and the remaining seven fixed-length bit patterns represent the magnitude.
- This allows signed integers to represent both positive and negative values within the specified bit range.
- For instance, in an 8-bit signed integer, extreme values range from -128 to 127(127 is the maximum integer range value).
Unsigned integers are non-negative integers represented solely by their magnitude in binary, without a dedicated sign bit. For example, in an 8-bit unsigned data type, distinct values range from 0 to 255. The absence of a sign bit allows these unsigned integer types to represent only non-negative immutable values.
The binary number system plays a crucial role, especially when working with Python bitwise operators. Python provides several bitwise operators, as discussed below, that enable the manipulation and comparison of binary numbers at the bit level with alternative integer types.
What Is Byte Order?
Byte order, or endianness, refers to the sequence in which bytes (8-bit data units) are stored in computer memory. The two common types are:
- Little-Endian: The least significant byte (LSB) is stored at the lowest memory address, with subsequent bytes stored in increasing order of significance. For examples: x86 and x86-64 architectures.
- Big-Endian: The most significant byte (MSB) is stored at the lowest memory address, with subsequent bytes stored in decreasing order of significance. For examples: PowerPC, SPARC, and Motorola 68k architectures.
Representation Example: For a 32-bit signed integer 0x123456780x123456780x12345678, the byte representation would differ based on byte order:
- Little-Endian: 78 56 34 12
- Big-Endian: 12 34 56 78
How Byte Order Affects Python Bitwise Operators?
In Python bitwise operations, the impact of byte order is particularly relevant when dealing with multi-byte fixed-point data types, such as distinct integer types represented by multiple bytes. Here’s how various bitwise operations are influenced by byte order:
- Bitwise AND (&), OR (|), XOR (^): During these operations, the corresponding bits of each byte are compared based on the system's byte order. The outcome may differ depending on whether the system is little-endian or big-endian.
- Bitwise NOT (~): This operation inverts each bit of a binary number. Its result is influenced by byte order when working with multi-byte data.
- Bitwise Shift Operators (<< and >>): These bitwise shift operators shift bits within a binary representation. The result can vary based on byte order, especially with multi-byte integers.
Application of Python Bitwise Operators
Python bitwise operators find applications in various scenarios, offering efficient solutions to specific challenges. Here are some common applications of Python bitwise operators:
- Flag and Permission Management: Bitwise compound operators are often used to manage flags and permissions compactly. Each bit in an integer can represent a specific permission or feature. By combining these bits using bitwise OR, AND, and XOR operations, developers can efficiently represent and manage complex permission systems.
- Data Compression and Optimization: In memory-constrained scenarios, Python bitwise operations can pack multiple boolean values into a single integer. This is especially useful when handling numerous flags or settings, which would otherwise consume significant memory.
- Bit Manipulation for Algorithms: Python bitwise operators are commonly employed in algorithmic challenges and optimizations. They enable efficient solutions for tasks like counting set bits, checking for powers of two, or finding the single non-repeating element in an array.
- Bitwise Filtering and Masking: Bitwise AND and OR operations are useful for filtering or masking specific bits within an integer. This approach is great for extracting or setting particular information within a fixed-length bit sequence.
- Low-Level System Programming: Python bitwise operations are used in system programming to facilitate low-level interactions with hardware, such as setting or clearing specific bit string ready in registers, and perform other low-level tasks. This is common in embedded systems programming or device driver development.
- Network Programming: Python bitwise operators are used in network programming to manipulate IP addresses, subnet masks, and perform bitwise operations on flags within network protocols.
- Encryption: Using bitwise XOR, data can be secured by converting ciphertext to plaintext and vice versa, enhancing the security of the information.
- Image Processing: Use of Python bitwise operations facilictates pixel manipulation in images. Techniques such as image masking and steganography can be implemented using these operations.
Also read- 20+ Best Python Projects Ideas
Python Bitwise Operator Overloading
In Python programming language, you can customize the behavior of bitwise operators for instances of your custom classes by overloading special or magic methods. Python bitwise operator overloading involves defining special methods such as __and__, __or__, __xor__, and __invert__ in your class. This allows instances of your class to participate in bitwise operations, just like built-in numeric types.
Here is a compact syntax for Python bitwise operator overloading:
class YourClass:
def __init__(self, value):
self.value = value
def __and__(self, other):
return YourClass(self.value & other.value)
def __or__(self, other):
return YourClass(self.value | other.value)
def __xor__(self, other):
return YourClass(self.value ^ other.value)
def __invert__(self):
return YourClass(~self.value)
def __repr__(self):
return f'YourClass({self.value})'
Here's a brief explanation of the provided syntax:
- The class keyword marks the beginning/ definition of the class called YourClass.
- The def keyword is used to create or define a function.
- The __int__ function/ method is the initializer method that sets the initial value for instances of the class.
- Next, __and__() refers to the Bitwise AND method that overloads the Bitwise AND (&) operator. It performs a bitwise AND operation between corresponding bits of two instances (self and other) and returns a new instance with the result.
- The method __or__() refers to the Bitwise OR function, which overloads the Bitwise OR (|) operator.
- Then, the __xor__() function overloads the bitwise XOR (^) operator.
- The __invert__() method overloads the bitwise NOT (~) operator. It performs a bitwise NOT operation on the instance (self) and returns a new instance with the result.
- Lastly, the __repr__() method provides an individual string format character representation of the instance for easy printing. It returns a string in the format YourClass(value).
Now, let's take a look at a code example that shows the implementation of these Python bitwise operator overloading methods.
Code Example:
class BitwiseExample:
def __init__(self, value):
self.value = value
def __and__(self, other):
return BitwiseExample(self.value & other.value)
def __or__(self, other):
return BitwiseExample(self.value | other.value)
def __xor__(self, other):
return BitwiseExample(self.value ^ other.value)
def __invert__(self):
return BitwiseExample(~self.value)
def __repr__(self):
return f'BitwiseExample({self.value})â
# Example usage
a = BitwiseExample(5)
b = BitwiseExample(3)
result_and = a & b # Bitwise AND
result_or = a | b # Bitwise OR
result_xor = a ^ b # Bitwise XOR
result_not = ~a # Bitwise NOT
print(result_and) # Output: BitwiseExample(1)
print(result_or) # Output: BitwiseExample(7)
print(result_xor) # Output: BitwiseExample(6)
print(result_not) # Output: BitwiseExample(-6)
Output:
BitwiseExample(1)
BitwiseExample(7)
BitwiseExample(6)
BitwiseExample(-6)
Explanation:
In the example Python program above-
- We first define a class named BitwiseExample to demonstrate overloading bitwise operators.
- Inside the class, we define an initializer method (__init__) that takes a value parameter and sets it as an attribute (self.value) for instances of the class.
- Next, we define the __and__() method to overload Python's bitwise AND (&) operator. It takes another instance (other) as a parameter, performs a bitwise AND operation between the individual bit value attribute of the current instance and the other instance, and returns a new instance of BitwiseExample with the result.
- Similarly, we define the __or__() method, which overloads Python's bitwise OR (|) operator, the __xor__() method, which overloads the bitwise XOR (^) operator, and the __invert__() method, which overloads the bitwise NOT (~) operator.
- These three methods follow a common pattern of performing the corresponding bitwise operation and returning a new instance.
- Lastly, we define the __repr__() method, which is implemented to provide a string representation of instances. It returns an individual string character in the format BitwiseExample(value).
- We then create example instances a and b with actual values 5 and 3, respectively.
- Next, we implement the bitwise methods. The Bitwise operations are demonstrated as follows:
- result_and = a & b performs bitwise AND.
- result_or = a | b performs bitwise OR.
- result_xor = a ^ b performs bitwise XOR.
- result_not = ~a performs bitwise NOT.
- Finally, we use a set of print() statements on the output of these operations, showcasing the results of the customized bitwise operations on instances a and b.
The output reflects the modified numeric values based on the bitwise operations, demonstrating how instances of the BitwiseExample class can participate in bitwise operations similar to integers.
Conclusion
Python bitwise operators provide a versatile and powerful toolkit for manipulating individual bits within integers. While they may not be the everyday choice for most developers, their role becomes crucial in scenarios requiring low-level bit manipulation. A solid understanding of bitwise AND, OR, XOR, NOT, and the left and right shift operations is essential for various tasks, from managing flags and permissions to optimizing data storage and implementing efficient algorithms.
The practical applications of Python bitwise operators are vast. They allow for compact representation of flags and permissions, optimize data storage for boolean values, and tackle algorithmic challenges with finesse. Whether it’s counting set bits in an integer or handling permissions with minimal memory consumption, bitwise operations prove their worth across multiple programming domains. As you venture into more advanced topics or engage in system programming, network protocols, or algorithmic optimizations, mastering Python bitwise operators will undoubtedly enhance your coding toolkit.
Frequently Asked Questions
Q1. Why do we use the bitwise operator?
In Python, bitwise operators are used for low-level data manipulation of individual bits within unsigned integers. These kinds of operators provide a means to perform operations at the binary level, enabling more efficient and compact representation of certain data structures and solving specific programming challenges.
The Python bitwise operators- AND, OR, XOR, and bitwise shift operators are commonly employed in scenarios such as flag and permission management, data compression, algorithmic optimizations, and low-level system programming.
Q2. Can we say whether a number is even or odd using the Bitwise AND operation?
Yes, we can determine whether a number is even or odd using the bitwise AND operation. In the binary digit representation of integers, the least significant bit (LSB) indicates whether the number is even or odd.
- For even numbers, the LSB is always 0.
- For odd numbers, the LSB is always 1.
By performing a bitwise AND operation with 1, we can extract the LSB to determine if a number is even or odd. If the result is 0, the number is even; if the result is 1, the number is odd.
Example Code:
n1 = 4
n2 = 7
print(f"{n1} is {'even' if n1 & 1 == 0 else 'odd'}.")
print(f"{n2} is {'even' if n2 & 1 == 0 else 'odd'}.")
Output:
4 is even.
7 is odd.
Q3. What is the difference between bitwise OR and XOR?
Bitwise OR (|): The Python bitwise operator OR sets a bit to 1 if at least one of the corresponding bits in the operands is 1. In other words, it combines the set bits from both operands.
For example: 5 | 3 # Binary: 0101 | 0011 → Result: 0111 (Decimal format: 7)
Bitwise XOR (^): The Python bitwise operator XOR sets a bit to 1 if exactly one of the corresponding bits in the operands is 1. It results in a 1 only if the bits are different. It results in a 1 only if the bits are different.
For example: 5 ^ 3 # Binary: 0101 ^ 0011 → Result: 0110 (Decimal format: 6)
Below is a comparison of the Bitwise OR and Bitwise XOR Truth table:
A |
B |
A | B |
A ^ B |
1 |
1 |
1 |
0 |
1 |
0 |
1 |
1 |
0 |
1 |
1 |
1 |
0 |
0 |
0 |
0 |
Q4. How to use bitwise NOT operator in Python?
We can use Python's bitwise NOT operator by using the ~ symbol before the operand. Note that it is a unary operator, which requires only a single operand to operate. It flips the bits: if a bit is 1, it becomes 0, and vice versa. It also changes the sign bit of the fixed-precision integers.
Example:
n=4
print(~n) #The output will be -5
Q5. What are bitwise vs logical operators?
Python bitwise operators perform manipulation on the bit level. They operate on every bit of the operand with its corresponding bit of the other operand. The plain integer values are first converted to binary internal representation, and the operation is performed on them at the bit level. Once the operation is done, the resulting binary bits are converted back to plain integers format/ decimal notation.
On the other hand, logical operators evaluate the logical conjunction between two boolean values or boolean expressions and yield a boolean result. The result of logical operators is always Boolean; it may be True or False.
The table below highlights the key differences between logical and Python bitwise operators.
Aspect |
Bitwise Operators |
Logical Operators |
Purpose |
Operate on individual bits of integers. |
Operate on Boolean values or expression. |
Operands |
Operands are integers/ decimal integer literals. |
Operands are Boolean values or boolean expressions. |
Use Cases |
Low-level bit manipulation, flags, etc. |
Boolean expressions are used in boolean logic and conditional statements. |
Evaluation Short Circuit |
No short-circuiting. All bits are evaluated. |
Short-circuiting consistent behavior. Stops on first False (for and) or first True (for or). |
Result Type |
Integer result. |
Boolean result. |
Q6. What is the result of the sign bit of negative and positive operands when performed bitwise AND operation?
In a 32-bit signed integer representation, the leftmost bit is often designated as the sign bit. For a 32-bit signed integer in two's complement form, the sign bit is the most significant bit (MSB). The sign bit is 0 for positive numbers and 1 for negative numbers.
When performing a bitwise AND operation between a positive and a negative operand, the result of the sign operation will always be 0. This is because the sign bit of the positive operand is 0, and the bitwise AND operation with the sign bit of the negative operand will always yield 0.
Here's a source code implementation of the same:
positive = 5 # Binary: 00000101
negative = -3 # Binary: 11111101 (Two's complement)
result = positive & negative
print(bin(result)) # Output: 0b1
Output:
0b101
Explanation:
In this example, the AND operation between the positive operand (5) and the negative operand (-3) results in the binary 0001, where the sign bit is 0. The sign bit of the negative operand does not affect the result in this context, as the AND operation with 0 always yields 0.
Q7. Is the expression 9>>-1 a valid Bitwise operation?
Yes, the entire expression 9 >> -1 is a valid bitwise operation in Python. The right shift (>>) operator shifts the bits of a number to the right by a specified number of positions. In this case, the number 9 is being right-shifted by -1.
When you right-shift a number by a negative value in Python, it is treated as if you are left-shifting the number by the absolute value of that negative number. Therefore, 9 >> -1 is equivalent to 9 << 1.
Q8. What is operator overloading in Python?
Operator overloading in Python refers to the ability to define multiple behaviors for a single operator, depending on the types of its operands. This allows objects of user-defined classes to utilize standard Python operators, providing a natural and intuitive syntax. In other words, you can give special meaning to operators for instances of your classes, allowing for more elegant mathematical operations with user-defined types.
Q9. How is the built-in Modulo operator used with Python's bitwise operators?
In Python, the built-in modulo operator, represented by the percent sign (%), is used to find the remainder of the division of two numbers. While the modulo operator and bitwise operators serve different purposes, they can be combined in specific situations.
For example, using the bitwise AND operator (&) with the modulo operation can check if a number is even or odd.
Code Example:
n = 10
is_even = n & 1 == 0
print(f"{n} is {'even' if is_even else 'odd'}.")
Output:
10 is even.
In this example, the bitwise AND operation with 1 is used to check the least significant bit (LSB) of the binary representation of the number. If the LSB is 1, the number is odd; if it's 0, the number is even. This works because the binary representation of odd numbers always ends with 1, and even numbers end with 0.
Q10. How do bitwise operations handle floating-point values in Python?
Bitwise operations in Python are designed to work with integers. When applied to floating-point values, the floating-point numbers are first converted to their binary representation, which can lead to unexpected results if not properly handled. To perform bitwise operations on floating-point numbers, one would typically need to convert them to fixed-precision integers first, process them, and then convert them back to a floating-point representation if needed.
Test Your Knowledge Of Python Bitwise Operators!
After mastering the concept of Python bitwise operators, you must also be well-versed in the following topics:
- 12 Ways To Compare Strings In Python Explained (With Examples)
- Relational Operators In Python | 6 Types, Usage, Examples & More!
- Python String.Replace() And 8 Other Ways Explained (+Examples)
- Python For Loop | Syntax & Application (With Multiple Examples)
- Python Namespace & Variable Scope Explained (With Code Examples)
- Difference Between List And Tuple In Python Explained (+Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Ujjwal Sharma 2 weeks ago
Divyansh Shrivastava 3 weeks ago
Esuru Pooja.C 4 weeks ago
Anjali Nimesh 1 month ago