- What Is Python’s split() String Function?
- How Does Python's split() String Method Work?
- Using Python's split() String Method With & Without maxsplit
- Parsing A String Using split() Function In Python
- Examples Of Using Python's split() String Method (10 Use Cases)
- Conclusion
- Frequently Asked Questions
Python split() String | Syntax, Working, & Use Cases (+Examples)
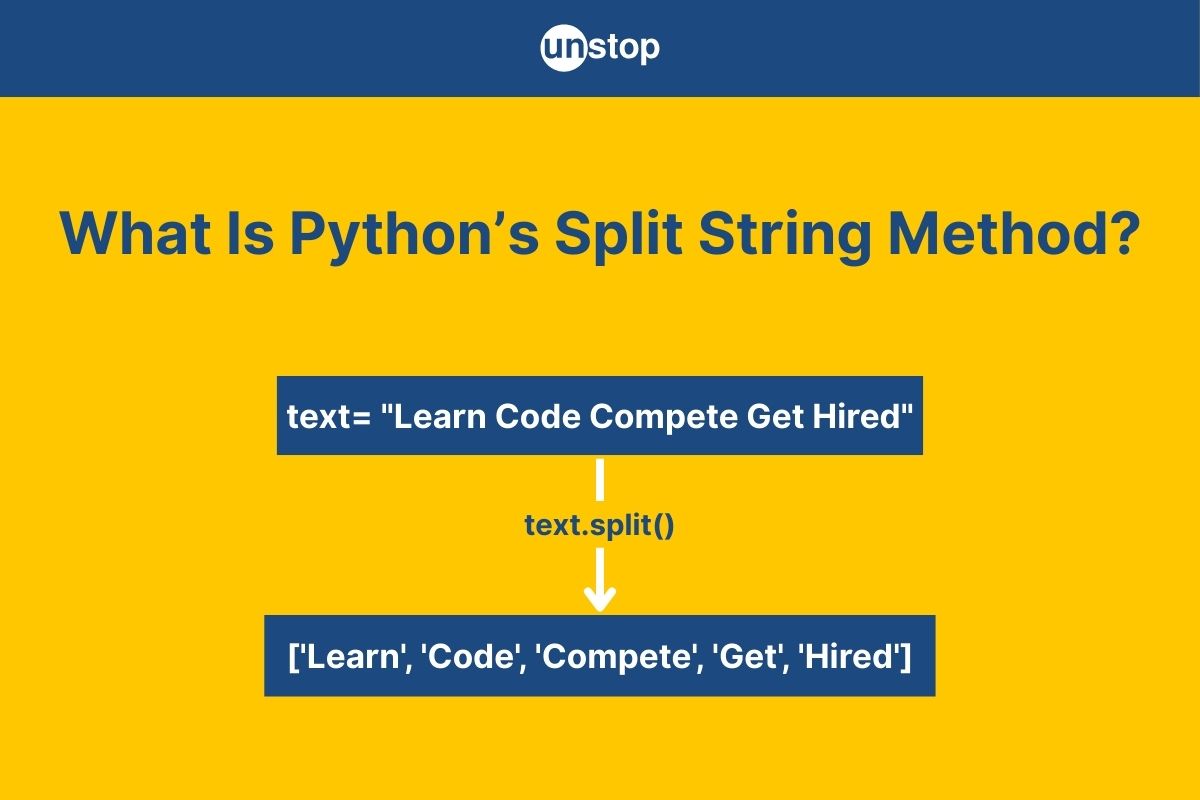
Ever tried breaking a sentence into words manually? Imagine having to extract names from a comma-separated list or splitting a paragraph into individual lines. Sounds tedious, right?
That’s where Python’s split() string method comes in handy. Whether you need to break text into words, parse data, or process files, split() simplifies the task by slicing strings into manageable chunks. In this article, we’ll explore how split() works, its parameters, and real-world use cases where this method shines.
What Is Python’s split() String Function?
The split() method is a built-in function in Python used to break a string into multiple parts based on a specified delimiter. By default, it splits on whitespace, but you can provide any character or sequence as a separator.
Syntax:
string.split(separator, maxsplit)
Parameters Of Python’s split() String Method
- separator (optional)– The delimiter that determines where the string is split. If not provided, whitespace is used.
- maxsplit (optional)– Defines the maximum number of splits. If omitted or set to -1, all possible splits occur.
Return Value Of Python’s split() String Method
The method returns a list containing the split parts of the original string value/variable.
Quick Knowledge Check!
Check out this amazing course to become the best version of Python programmer you can be.
How Does Python's split() String Method Work?
At its core, Python’s split() method scans the string for the specified separator, chops the string at those points, and returns the resulting pieces as a list. Here’s how it works step by step:
- Identify the separator (if provided). If omitted, whitespace is used as the default.
- Scan the string from left to right, finding occurrences of the separator.
- Split the string at each separator occurrence, creating segments.
- Limit the number of splits if maxsplit is specified. If maxsplit = 2, only the first two occurrences will be used for splitting.
- Return the list of split segments.
Code Example (Basic Usage):
#Creating a string variable
text = "Python is fun to learn"
#Using split() on the string variable
words = text.split()
print(words)
Output:
['Python', 'is', 'fun', 'to', 'learn']
Code Explanation:
In the example, we first create a variable text and assing a string value to it.
- Then, we call the function split() on the string using the dot operator and assing the outcome to the words variable.
- The function detects whitespace as the default separator.
- It splits "Python is fun to learn" at each space.
- The result is a list containing individual words, which we display to the console using the print() function.
Using Python's split() String Method With & Without maxsplit
The maxsplit parameter is one of the optional parameters for the split() method in Python programming. It controls how many times the string should be split.
- Without maxsplit (default behavior): The string is split at every occurrence of the separator.
- With maxsplit: Only the specified number of splits are performed, and the remaining string is returned as the last list element.
Example 1: Splitting Without maxsplit
#Creating a string
text = "Learn Code Compete Get Hired"
#Using split() without maxsplit parameter
words = text.split()
print(words)
Output:
['Learn', 'Code', 'Compete', 'Get', 'Hired']
Code Explanation:
In the simple Python program example, the string is split at every space because maxsplit is not set. All words are returned as separate elements in the list.
Example 2: Splitting With maxsplit
#Creating the same string variable
text = "Learn Code Compete Get Hired"
#Using split() with maxsplit set to 2
limited_words = text.split(" ", 2)
print(limited_words)
Output:
['Learn', 'Code', 'Compete Get Hired']
Code Explanation:
Here, the string is split at only the first two spaces (since maxsplit=2). The first two words are separated, while the rest of the string remains as one element in the list. This is useful when handling structured data where only a certain number of splits are needed.
Quick Knowledge Check!
Ready to upskill your Python game? Dive deeper into actual problem statements and elevate your coding game with hands-on practice!
Parsing A String Using split() Function In Python
Parsing refers to breaking a string into meaningful parts for further processing. The split() function helps extract structured data from text, such as names from a CSV line, timestamps from logs, or details from user input.
Process of Parsing a String with split():
- Identify the delimiter used in the data (comma, space, tab, etc.).
- Apply split() to break the string at the delimiter positions.
- Store the result as a list for easy access and manipulation.
Example: Extracting Data from a CSV Line
csv_data = "John,25,Software Engineer"
parsed_data = csv_data.split(",")
print(parsed_data)
Output:
['John', '25', 'Software Engineer']
Code Explanation:
We create a cvs data string containing values separated by commas.
- Then we call the split() function, passing a comma as a delimiter. This breaks it into three parts:
- "John" (Name)
- "25" (Age)
- "Software Engineer" (Job Title)
- The result is a list where each element represents a meaningful chunk of data.
- This approach is commonly used for processing files, database records, and user inputs.
Examples Of Using Python's split() String Method (10 Use Cases)
The split() method is highly flexible and can be applied in various scenarios. Below, we'll explore different ways to use it, each with an example.
1. Splitting String Based On Occurrence Of A Character
When working with structured text data, strings often contain consistent character separators such as commas, colons, or dashes. The split() method lets us extract meaningful parts by breaking the string wherever a specific character appears. This is particularly useful when working with timestamps, dates, or formatted data entries.
Code Example:
#Creating a string containing timestamp
time_string = "12:30:45"
#Using split() with colon delimiter
time_parts = time_string.split(":")
print(time_parts)
Output:
['12', '30', '45']
Code Explanation:
Here, the split(":") function looks for every occurrence of the colon character (:) in the string and splits the text at those positions.
- As a result, "12:30:45" becomes three separate components: hours, minutes, and seconds.
- This technique is commonly used in clock-based calculations and timestamp parsing.
2. Using Python's split() Method To Split A File Into A List
When reading a file as a string, we often need to convert the content into a structured format, such as a list of words or sentences. Using split(), we can process file contents efficiently, making it easier to search, filter, or manipulate data.
Code Example:
file_content = "Python makes coding fun"
#Using split to break the file into a list of strings
words = file_content.split()
print(words)
Output:
['Python', 'makes', 'coding', 'fun']
Code Explanation:
Here, the split() function automatically splits the text at spaces and stores each word in a list. This approach is useful for tokenizing text, such as processing log files, extracting keywords, or building word frequency models.
3. Using Python's split() Method With Newline Character To Split A String
In real-world applications, text data is often stored across multiple lines, whether in paragraphs, logs, or structured reports. The newline character (\n) represents a line break, and split("\n") allows us to process each line as an independent unit.
Code Example:
#Creating a string containing newline characters
multi_line_text = "Line 1\nLine 2\nLine 3"
#Splitting a multi-line string
lines = multi_line_text.split("\n")
print(lines)
Output:
['Line 1', 'Line 2', 'Line 3']
Code Explanation:
Here, the split() function detects every \n (newline character) and splits the text accordingly.
- Each line of text is stored as an individual string in the resulting list.
- This method is commonly used for processing logs, reading CSV files, or analyzing structured reports.
4. Using split() With Multiple Delimiters
The standard split() method only allows one delimiter at a time, but in many cases, data contains multiple separators. To handle this, we use the re.split() function from Python’s re (regular expressions) module. This allows us to split text using multiple delimiters simultaneously.
Code Example:
import re
#Creating a string with multiple special characters
text = "apple,banana;cherry orange"
#Splitting using commas and semicolons
fruits = re.split(",|;| ", text)
print(fruits)
Output:
['apple', 'banana', 'cherry', 'orange']
Code Explanation:
We begin the example by importing the Python module re to use regular expression functions. Then, we define a string name text and use the re.split() method to split it.
- Here, we define ",|;| " as a regular expression, meaning it splits the string at commas, semicolons, or spaces.
- Unlike split(), which can only handle one delimiter at a time, re.split() provides greater flexibility.
- This is useful when working with messy data, such as user inputs or unstructured text.
Quick Knowledge Check!
Looking for guidance? Find the perfect mentor from select experienced coding & software development experts here.
5. Using Python's split() Method To Convert String To List (Or Char Array)
Sometimes, we need to break a word down into its individual characters—for example, in text processing, password validation, or working with letter-based algorithms. The split() method doesn’t directly split a string into characters, but we can achieve this using list().
Code Example:
#Creating a string variable containing one word
word = "Python"
#Using list() function to split the string into a list()
char_list = list(word)
print(char_list)
Output:
['P', 'y', 't', 'h', 'o', 'n']
Code Explanation:
Unlike split(), which requires a delimiter, list() directly extracts each character.
- Each letter of "Python" is stored as an individual element in the list.
- This approach is helpful in string manipulations, cryptography, and text analysis.
Also read: How To Convert String To List In Python? 9 Ways With Code Examples
6. Using Python's split() Method To Split String Into List (Array Of Strings)
A common use case for split() is converting a sentence into a list of words, making it easier to analyze or manipulate the text. This is particularly useful in natural language processing (NLP) and search algorithms.
Code Example:
#Creating a string
sentence = "Learn Practice Compete"
#Using split() to convert string to list
word_list = sentence.split()
print(word_list)
Output:
['Learn', 'Practice', 'Compete']
Code Explanation:
The function splits the sentence into separate words, making it easier to access individual components. This technique is widely used in text tokenization, chatbots, and search engines.
7. Using Python's split() String Method Without Any Parameters
When split() is called without arguments, it behaves differently compared to when a separator is explicitly provided. It removes extra whitespace and splits only where there’s actual content, making it ideal for cleaning messy text.
Code Example:
#Creating a string variable containing multiple whitespaces
text = " Python is awesome "
#Splitting it into list of words
words = text.split()
print(words)
Output:
['Python', 'is', 'awesome']
Code Explanation:
Unlike split(" "), which preserves extra spaces, calling split() without parameters automatically removes them. This is useful when handling user-generated text, ensuring cleaner data input and processing.
8. Using Python’s split() Method With Tab As Separator For Splitting A String
In data processing, especially when dealing with TSV (Tab-Separated Values) files or structured logs, fields are often separated by tab characters (\t). Python's split() method can be employed to parse such strings effectively.
Code Example:
data = "Name\tAge\tOccupation"
#Using split() with tab as delimiter
fields = data.split("\t")
print(fields)
Output:
['Name', 'Age', 'Occupation']
Code Explanation:
We first create a variable with a string value containing fields separated by tab characters.
- By specifying "\t" as the separator, split() divides the string at each tab, resulting in a list of field names.
- This approach is particularly useful when parsing TSV files or data where tab separation is standard.
9. Using Python’s split() Method With Comma As Separator For Splitting A String
Commas are commonly used as delimiters in CSV (Comma-Separated Values) files. Utilizing split(",") allows for the extraction of individual elements from such strings.
Code Example:
csv_line = "apple,banana,cherry,dragonfruit"
fruits = csv_line.split(",")
print(fruits)
Output:
['apple', 'banana', 'cherry', 'dragonfruit']
Code Explanation:
The string lists fruit names separated by commas. Using split(","), the string is divided at each comma, producing a list of fruit names. This method is essential for processing CSV data where items are delineated by commas.
10. Splitting A String Using A Substring Separator
Beyond single characters, split() can utilize entire substrings as delimiters. This is beneficial when dealing with text where sections are separated by specific words or phrases.
Code Example:
#Creating a string containing double hyphens
text = "2025-03-31--New Delhi--Python Workshop"
#Splitting using double hyphens as a substring
details = text.split("--")
print(details)
Output:
['2025-03-31', 'New Delhi', 'Python Workshop']
Code Explanation:
The string contains event details separated by the substring "--". By passing "--" as the separator to split(), the string is divided at each occurrence of this substring. This technique is useful for parsing strings where segments are separated by multi-character delimiters.
Conclusion
Python’s split() string method is a versatile and powerful tool for string manipulation, enabling developers to parse and process text data efficiently.
- By dividing strings into lists based on specified delimiters, split() facilitates tasks such as data cleaning, parsing structured files like CSVs or TSVs, and tokenizing text for natural language processing.
- Its flexibility, including handling multiple delimiters and controlling the number of splits with the maxsplit parameter, makes it indispensable for both simple and complex string operations.
- Understanding and effectively utilizing the split() method enhances your ability to manage and manipulate textual data in Python, contributing to more efficient and readable code.
Whether you're processing user input, analyzing logs, or preparing data for machine learning models, mastering split() can help you write better Python programs.
Also read: 40+ Python String Methods Explained | List, Syntax & Code Examples
Frequently Asked Questions
Q1. What is the primary function of the split() method in Python?
The split() method divides a string into a list of substrings based on a specified delimiter. By default, it uses whitespace (spaces, tabs, newlines) as the delimiter, effectively breaking the string into individual words. This is particularly useful for parsing and analyzing text data.
Q2. How does the split() method handle string splitting by default?
When no delimiter is specified, split() treats any consecutive whitespace characters as a single separator and removes them from the resulting substrings. This means that multiple spaces between words do not result in empty strings in the output list. For example:
text = "Python is awesome"
words = text.split()
print(words)
# Output: ['Python', 'is', 'awesome']
In this case, the multiple spaces between words are ignored, and the string is split into individual words.
Q3. Can I limit the number of splits performed by the split() method?
Yes, the split() method includes an optional maxsplit parameter that controls the maximum number of splits to perform. After reaching this limit, the remaining part of the string is returned as the final element in the list. For example:
text = "apple,banana,cherry,dragonfruit"
fruits = text.split(",", maxsplit=2)
print(fruits)
# Output: ['apple', 'banana', 'cherry,dragonfruit']
Here, the string is split at the first two commas, resulting in three elements in the list.
Q4. How can I split a string using multiple delimiters?
The standard split() method does not support splitting a string using multiple delimiters directly. However, you can achieve this using the re.split() function from Python library's re (regular expressions) module. For example, to split a string using both commas and semicolons as delimiters:
import re
text = "apple,banana;cherry orange"
fruits = re.split(r'[;, ]', text)
print(fruits)
# Output: ['apple', 'banana', 'cherry', 'orange']
In this example, the regular expression [;, ] matches any comma, semicolon, or space, effectively splitting the string at each of these characters.
Q5. How does split() behave when the delimiter is not found in the string?
If the specified delimiter is not present in the string, the split() method returns a list containing the original string as its only element. For example:
text = "hello world"
result = text.split(",")
print(result)
# Output: ['hello world']
Here, there is no comma in the string “hello world”. As the split() function does not find the comma delimiter, the entire string is returned as a single-element list.
Q6. Can split() be used to parse strings in formats like CSV or TSV?
Yes, split() can be used to parse simple CSV (Comma-Separated Values) or TSV (Tab-Separated Values) strings by specifying the appropriate delimiter (, for CSV, \t for TSV). However, for more complex CSV files that include quoted fields, newline characters within fields, or other special cases, it's recommended to use Python's built-in csv module, which is specifically designed to handle these complexities.
Q7. Is the split() method case-sensitive when specifying delimiters?
Yes, the split() method is case-sensitive when it comes to the delimiter. For instance, specifying a lowercase letter as the delimiter will not split the string at uppercase letters, even if they are the same letter.
Q8. How does split() handle leading and trailing whitespace in a string?
When using split() without specifying a delimiter, leading and trailing whitespace are automatically removed before splitting the string. This ensures that the resulting list does not contain empty strings due to extra spaces at the beginning or end of the input string.
This compiles our discussion on the Python split() string method. Also, check the following out:
- f-string In Python | Syntax & Usage Explained (+Code Examples)
- Python List index() Method | Use Cases Explained (+Code Examples)
- String Slicing In Python | Syntax, Usage & More (+Code Examples)
- Python Reverse List | 10 Ways & Complexity Analysis (+Examples)
- Python List Functions: A Guide To Essential Operations (+Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment