Strings In C | Declare, Initialize & String Functions (+Examples)
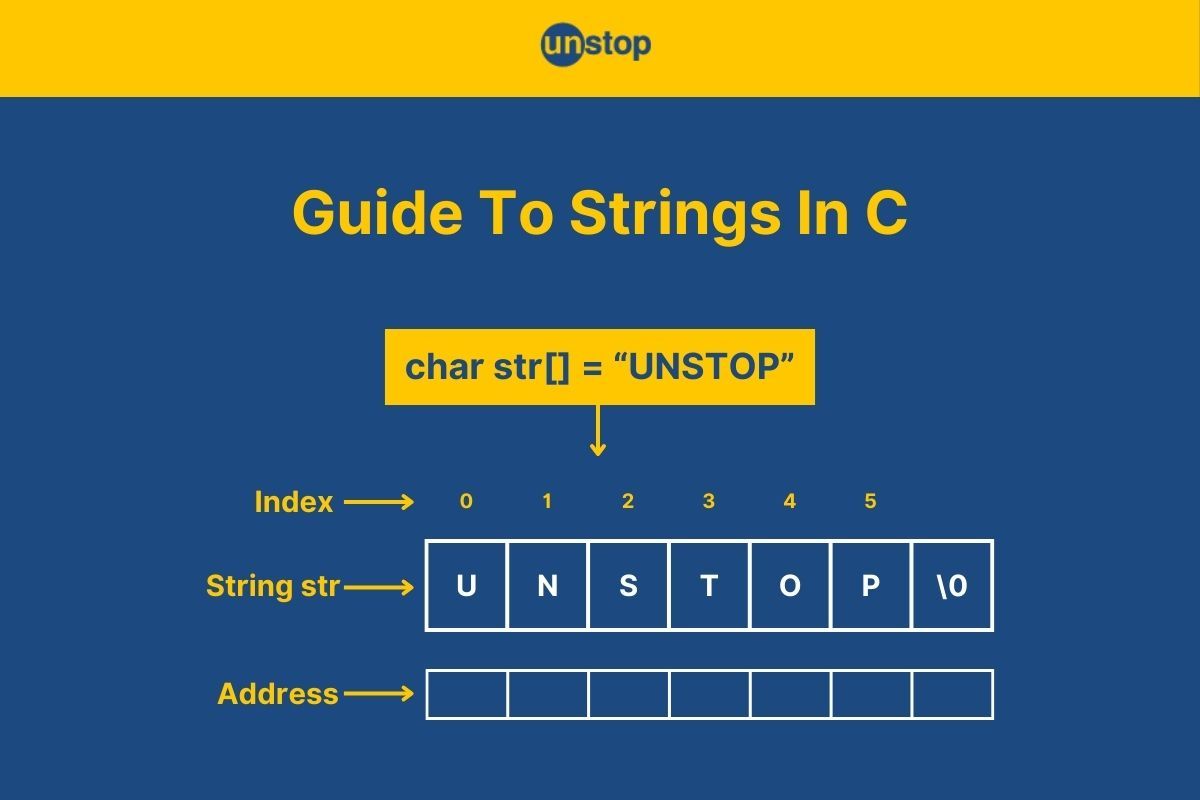
Table of content:
- What Are Strings In C?
- Declaration Of Strings In C Language
- 4 Ways For Initialization Of Strings In C
- Assigning Values To Strings In C
- How To Take String Input From Users
- The Most Used Functions In C Strings
- Conclusion
- Frequently Asked Questions
Are you a C programmer looking to level up your coding skills? If so, understanding how to use strings in C programming is essential. Whether you're new or an experienced developer, strings provide the perfect opportunity to enhance and refine your programming knowledge. In this blog, we will explore everything there is to know about using strings in C language, including data types and why they are important for manipulating text-based information within programs. Let's get started!
What Are Strings In C?
A string is a sequence of characters belonging to a specific character set. For example, "hello world" is a string containing five characters: "h," "e", "l", "l", and "o". In C, a string is represented using a character array that ends with the null character(\0). To create a string in C, you must declare an array of characters.
Syntax:
char str[size];
Example:
Output:
Enter your name: unstop
Your name is unstop
Explanation:
In the code-
- we create an empty character array of size 20 and assign it to the variable 'str' with char str[20].
- Next when the user enters their name, which is then stored in the "str" variable.
- This value is then printed on the screen using printf().
- The return 0 statement at the end indicates that this program has run successfully.
Declaration Of Strings In C Language
We use the character data type to declare a string in C, and the syntax is the same as mentioned above. The process involves declaring an array of characters with a size that reflects how long you want your string to be. Let's take another look at the syntax and see what it means.
The syntax for declaring strings in C:
Char str_name[size];
Here,
- str_name is the string variable's name
- The size is the maximum number of characters the string can hold, excluding the null character.
Now let's look at an implementation example for the same:
Output:
Enter a message (up to 30 characters): Hello unstop
The message you entered is: Hello
Explanation:
In the program above we-
- First, create a string variable 'message' with a size of 30 characters.
- Next, we use the printf() function to enter the user message.
- We then use the scanf() function to read up to 30 characters of input from the user and store it in the message variable.
- Lastly, we again use the printf() to print the user message stored in the variable string message.
4 Ways For Initialization Of Strings In C
There are 4 ways in which we can initialize string in C language. These are by-
1. Assigning a string literal with size
2. Assigning a string literal without size
3. Assigning character by character with size
4. Assigning character by character without size
We will discuss each of these methods in greater detail and with the help of examples ahead.
Initializing Strings In C By Assigning String Literal With Size
This is the most common way to initialize strings in C, as it allows for the direct assignment of array size and value at once. The syntax used for this method is given below.
Syntax:
char string name[Size] = “String_Literal”;
Here.
- The string name is the name of the string variable.
- The size is the maximum number of characters in the string (basically, it is the space that will be allocated to the array).
- The “string_Literal” is the string that you are going to assign.
Code Example:
Output:
Name: Unstop
Explanation:
In the example-
- We initialized the character Array named ‘Name’ by assigning it with ‘Programming'.
- This will occupy 6 characters + 1 Null Character ('/0'), which indicates the ending of the string.
- If you don't provide the space for a Null character, then the compiler will automatically add a Null character at the end.
- Also, if the size we provide is lower than the actual character count, then the result will be up to the defined size only.
Code example:
Output:
Name: Unsto
Code Explanation:
- We initialized the character Array named ‘Name’ by assigning it with ‘Programming'.
- This will occupy 5 characters, so what happens is the compiler reads only up to 5 characters which is Unsto.
Initialization By Assigning A String Literal Without Size
In C, a string variable may be initialized by assigning a literal string to it without specifying the array's size. The compiler automatically allocates the null terminator and sufficient memory.
Syntax:
char stringName[] = "string literal"
Code Example:
Output:
Hello unstop!
Explanation:
In this case, the compiler automatically sets the variable to have enough memory to store the entire string. Since we used 11 characters (including '0') in our declared text "Hello unstop!" the compiler already knows that you are defining an array of 12 bytes when you write your code.
Initialization Of String In C By Assigning Character By Character With Size
We can initialize a string variable in C by assigning it characters individually and specifying the maximum size of the string as an array size. The syntax and an example for this are given ahead.
Syntax:
char array_name[static size] = {'C', 'H',' A', 'R', '\0'}
Code Example:
Output:
char
Explanation:
Each element must be initialized individually along with a '\0' (null-terminator) as the last element, which specifies that object ends here only. The initializer provided in braces must provide exactly enough items for initialization.
Otherwise, a compilation error occurs, i.e., the number of elements given should not exceed the array dimension specified while declaring the variable. This is because the compiler will throw an error if we try to initialize more than what it can hold. However, assigning a count of less than the array's declared length is allowed even though the compiler throws a warning saying unused.
Initialization By Assigning Character By Character Without Size
Finally, we can also initialize a string in C by assigning it characters individually without specifying the size of the array. The compiler will automatically allocate enough memory to store the string, including the null terminator.
Syntax:
char str[ ] = {'s', 't', 'r', 'i', 'n', 'g',}
Code Example:
Output:
Hello
Explanation:
- Here we initialize the char array str without specifying its length/size, as size specification is not compulsory, The compiler will automatically determine its size.
- So here, we assigned each letter (character) into individual blocks created inside that character array called "str".
- When we call the str array, the compiler reads them one by one and stops when the str array ends.
Assigning Values To Strings In C
As we have mentioned before, strings in C are denoted by enclosing characters in double quotes. A string is a sequence of one or more characters that can include letters, numbers, and special symbols such as punctuation marks. Assigning values to strings can be done by either using the assignment operator '=' (equals sign) or the strcpy() function to store data in the memory allocated for this purpose.
Using the '=' operator is straightforward since it requires only two operands, i.e., an initializer consisting of any valid character array and a value that could be specified as another character array or just any character enclosed within single quote signs (e.g., 'A').
For example,
char str[] = "Example String";
This line of code implies the creation of an 8-byte long string with a null-terminator at its end to assign it a value of "Example String".
The same result can also be achieved by giving a size parameter, like-
char str[50] = {'E', 'x', 'a', 'm', etc.…};
Here, individual elements are mentioned instead of written out all at once. However, unnecessary space must still be reserved even if less than the actual length of the resulting string is given here otherwise, segment fault errors may arise during compilation time due to accessing invalid memory locations outside the bounds.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
How To Take String Input From Users
Reading a string in C requires using a character array to store the input and some library functions for manipulating strings. We’ll discuss how to read strings into variables using the scanf() function in this section.
The syntax of the scanf() function is as follows:
scanf("%s", variableName);
Here,
- The %s means “read characters until you reach white space or end-of-line”
- Variable Name represents any valid character array name where user input has to be stored.
- Since it reads only one word (i.e., tokens) at once, we can concatenate multiple calls.
Code Example:
Output:
Enter a message: Unstop
Unstop
Explanation:
In this example, the program reads the user's string character, stores it in the 'str', and prints the same string output. Here the scanf() function is used to read the input user-given data, and the printf() function prints the user-given data that is stored in the string.
How To Read A Line Of Text In C?
Reading a line of text in C as a string can be accomplished using the fgets() function. This function is part of the stdio library and takes three parameters –
- An array for storing the string,
- An integer indicating the maximum length to read from its input stream, and
- A FILE pointer is pointing to where it should start reading from.
The return value is either Null or a pointer that points at buff, which stores the string. To use this function effectively, you must first open a file handle with fopen(), then pass this file handle into fgets().
Code Example:
Output:
Unstop
String is:
Unstop
Explanation:
This program defines a character array of a maximum size of 50, which stores a string entered by the user. The fgets() function takes input from stdin and stores it in the str variable until MAX characters are taken in or until the newline character \n is encountered. Then, using puts(), the str value is printed onto stdout for displaying onscreen.
Passing Strings In C To Functions
In C, passing strings to functions is done using the char pointer parameter. This parameter type allows a C program to pass strings as arguments during function calls. Doing this in code can be written out like normal parameters with the asterisk (*) before them.
For example:
int my_function(int arg1, char *arg2){...}.
Here, the first argument (arg1) will handle integers, while the second one (arg2) takes a string character-by-character and stores it in memory when passed at runtime.
To read such a string from within a function, you would use something similar to "char* buff = arg2", where buff stands for a buffer which, in effect, holds all characters of your inputted string until you finish reading one or more characters from it or reach its end ("\0").
The Most Used Functions In C Strings
String functions are a type of function that can be used to manipulate and work with strings. These allow you to do many different things, such as combining two separate strings into one string, searching for a given character within a string, returning information about the length of a string, changing cases in a text (e.g., upper/lower), extracting part(s) from an existing string and so on.
Some of the most commonly used string functions in C are-
- strlen(): used to find the length of a string in C language
- strcpy(): used to copy one string into another
- strcat(): used for concatenating two strings by appending one at the end of other
- strcmp(): used for comparing two strings character-by-character and returns 0 if both strings are the same; otherwise, it will return either a negative (if first < second) or positive value(if first > second).
- memset(): fills all elements with a particular value in an array
- strstr(): finds the position where the given substring is within a larger string; this function works similarly to the "find" command on the Linux terminal.
- strchr(): used to search for characters within a string
- strdup(): creates a duplicate copy of the given string
- memmove(): used to move one block of memory from one location to another without corrupting its contents
- sprintf(): writes formatted data into a buffer or file; it is commonly used for converting numerical values into strings in C language
Example Of String Functions In C
Output:
Lengths before concatenation: 5 6
Concatenated string: HelloWorld!
Length after concatenation: 11
Not Equal!
Explanation:
This program uses four string functions strlen(), strcpy(), and strcat(), to demonstrate the concept of concatenation of strings and strcmp() for comparison.
- First, we declared two different strings with values ‘hello’ and ‘world!’ respectively.
- We then use the strlen() function to find the length of both variables or strings passed as an argument.
- Next, we proceeded towards concatenating them, which was done by using the strcat() function while also printing out their value after being combined into a single one inside 1st variable (str1).
- After that, again same built-in function strlen() was called to check out the new size/ length produced due to combining two separate inputs earlier.
- Finally, we finish our program by comparing these with each other using the comparison function strcmp().
- If they match, it will return 0, indicating equality.
- Otherwise, some non-zero value will return if the strings are not equal lexicographically.
This concludes how string functions such as strlen, strcpy, strcat, and strcmp worked together to carry on a series of operations on two different strings in C.
Example 2- The memset(), strchr(), strdup(), and strstr() functions
Output:
Source String: Welcome to stringsunstop
After Memset(): $$$$$$$$$$ stringsunstop
character e not found
Copied String Using strdup(): $$$$$$$$$$ stringsunstop
Substring "unstop" identified in source: unstop
Explanation:
This program uses four different string functions from the string.h library which are memset(), strchr(), strdup() and strstr().
- The source string is initialized as "Welcome to stringsunstop."
- Then, the first ten characters of it are changed using the memset() function, and the result, i.e., "$$$$$$$$$$ stringsunstop" is printed on the output screen.
- After this, pointer ptr1 is declared for finding the index position of 'e' appearing in that source string returns by stachr(). It will return the 19th index (zero-based).
- The strdup() function returns a copy of the source, while ptr3 stores the returned value after executing the strdup() operation over the source character array src[].
- The last step includes the declaration of pointer 4, i.e., Ptr4, which stores the substring "unstop" found in the source string returned by StrStr().
- The final output with all changes made as an effect of all the operations is then displayed on the output screen.
Conclusion
In conclusion, understanding how to use strings in C programming is a valuable skill for any programmer. With suitable data types and library functions, programmers can efficiently store and manipulate text-based information within their programs.
As a programmer, becoming proficient in strings can help open up more opportunities within the field. With increased expertise, you can create more sophisticated programs and develop skills that could lead to career advancement. Strings are an essential component of the C programming language, and being aware of their basics is key for any aspiring developer.
Frequently Asked Questions
Q. How to initialize variable C?
Initializing variables involves utilizing the keyword 'var', succeeded by the type and name of said variable. An example of this concept is-
var myVar = 5;
If a value is not immediately assigned, the variable can still be initialized with its respective type declared, for instance-
int myVar;
Alternatively, one can use the 'new' keyword to instate a default value into an uninitialized variable like-
int myVar = new int();
This particular method sets the value automatically to 0.
Q. Can you initialize an empty string in C?
In the programming language C, it is possible to declare and initialize an empty string by creating a new variable that is a character array with no specified length, then giving it no value except for two quotes side-by-side, symbolizing blank space.
Syntax:
char myString[0] = " "
Q. How to declare a string array in C?
In C, the string array can be declared by using the following syntax-
char array_name[size][size_of_each_string];
Say, for example, we want to declare a string array mystr, with 20 strings of 30 characters each. The syntax then becomes-
char mystr[20][30];
So, here, we specified a maximum size of 30, and an array comprising 20 strings is declared using the identifier 'mystr'.
Another example can be as follows-
char mystr[][30] = {“str1”, “str2”, “str3”};
Here, an automatic system has been put in place to determine the size of the first array. This system factors in all associated strings included and initializes them accordingly. Furthermore, as for designing limitations alongside usage to ensure no confusions occur later, the length for individual strings inside our second module has stayed within 30.
Q. How to store a string in C?
In C programming language, strings that are declared as character arrays behave much like other arrays. Specifically, they are allocated storage space according to their variable type. For example, strings stored as auto variables exist on the stack, while those stored as global or static variables occupy space within the data segment.
Q. What is a string in programming?
A string in programming is a sequence of characters (letters, numbers, symbols) treated as text. Strings are primarily used to store and manipulate textual data.
Q. Can we declare two strings in C in the same program? How?
Yes, we can declare two strings in a single program. The syntax to declare two strings in C is as follows:
char str1[size];
char str2[size];Example:
Char str1[30];
Char str2[40];
Here the two strings are declared as two arrays, “str1” and “str2” of sizes 30 and 40. We can use these variables to store and manipulate strings in the c program.
Q. How are strings represented in C?
In C, strings are represented as arrays of characters. A string is typically declared as an array of char, for example-
char str[100];
The array may contain a sequence of characters, including spaces and punctuation, followed by a null character (‘\0’) to signify the end of the string.
Example:
char string[100] = "unstop";
The string "unstop" is stored as an array of characters in the variable string. The array includes the characters 'u', 'n', 's', 't', 'o', 'p', and a null character ('\0') that signifies the end of the string.
We are sure that by now, you know exactly what strings in C mean and how to declare, initialize, and carry on a series of operations on them. You might also be interested in reading the following:
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment