Ternary (Conditional) Operator In C Explained With Code Examples
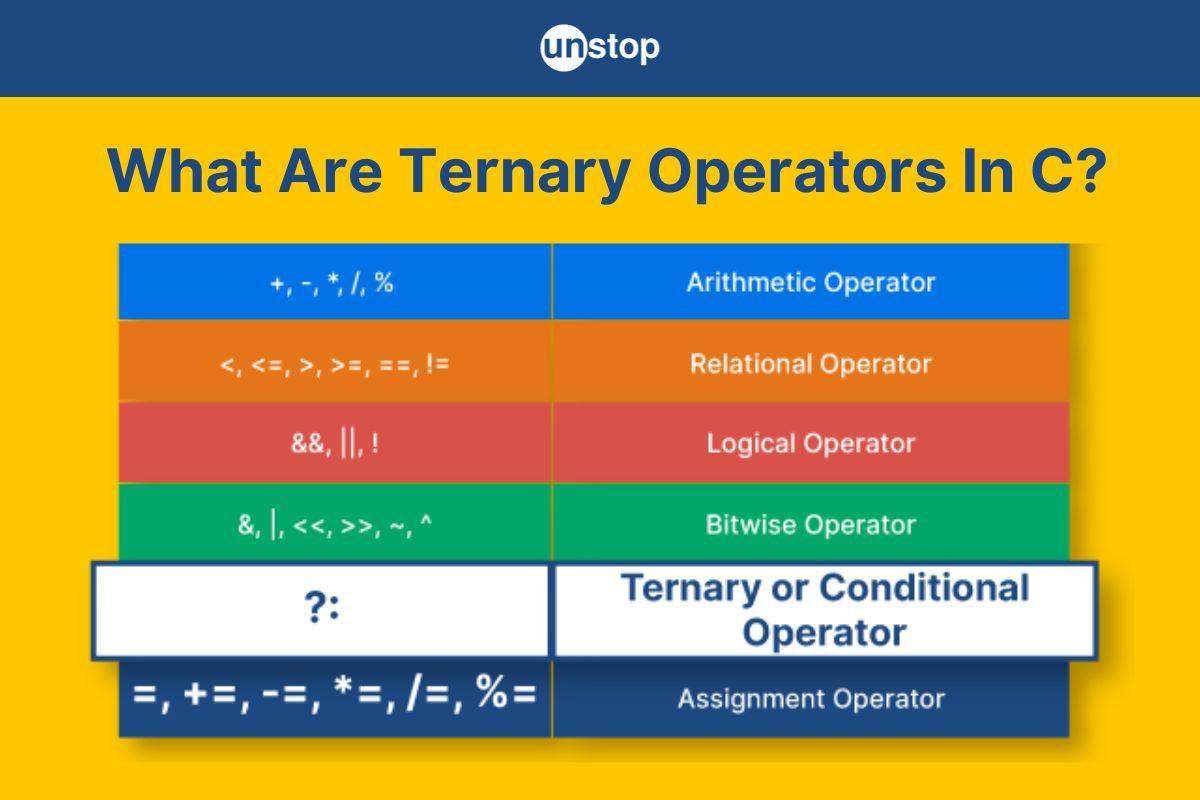
The ternary operator in C language, also known as the conditional operator, is denoted by the question mark and colon symbols, i.e., (? :). It is a powerful operator that provides a shorthand way of expressing conditional assignment statements, allowing developers to write compact code for simple decision-making scenarios. In other words, the conditional operator in C is a concise alternative to traditional if-else statements, offering a streamlined approach for making decisions within a single line of code.
In this article, we will unravel the syntax, applications, and best practices surrounding the ternary operator in C programming. From its fundamental structure to its versatile applications and nuanced best practices, we'll delve into the intricacies of this operator, empowering developers to wield it effectively while maintaining code readability.
What Is Conditional/ Ternary Operator In C?
Conditional operators in C are an alternative to the popular decision-making statements, i.e., the if/ if-else statements. To begin with, the if-else statements are used to perform operations based on a specific condition being met. That is, if a condition holds true, a pre-specified conditional operation is run, and if the condition holds false, then the alternative operation is run.
The syntax for the if-statements generally runs in multiple lines, which might become tedious to write over and over again. This is where conditional operators come into play. They shorten the code by a lot and still fulfill the same purpose.
Syntax Of Conditional/ Ternary Operator In C
Expression1? expression2: expression3;
Here,
- Expression1: It is the boolean expression that can be true or false.
- Expression2: If Expression1 is true, then this expression will be executed.
- Expression3: If Expression1 is false, then this expression will be executed
Arguments Of Ternary/ Conditional Operator In C
As shown in the syntax above, a ternary operator in C consists of three comparison arguments (just like if-else and loops). They are-
- First, a condition, followed by a Question Mark (?)
- Second, an expression to execute if the condition is True, followed by a Colon (:)
- Third, an expression to execute if the condition is False.
Working Of Ternary/ Conditional Operator In C
Here is a step-by-step explanation of how the conditional/ ternary operator in C works:
- The result of conditional expression (expression1 in syntax) is evaluated first, as it undergoes implicit boolean conversion.
- The rest of the flow of the C program is dependent on whether this is true or false.
- For example, we have two variables, a and b, with values 30 and 20, respectively.
- The base conditional expression is for the ternary operator to check whether a or b is greater and implicitly convert the result to bool.
- If the condition is true, then the first expression (the expression after the question mark) will be executed. In the example above, we have a>b (i.e., 30>20), so the result of the conditional expression is TRUE. Hence, this expression will be executed.
- If the condition is false, then the second expression (the expression after the colon) will be executed. Had the result for the condition from step 1 been False, then this expression would have been executed.
Note- As is evident from the steps listed above, the process is just like the if-else statements, but the actual code is much shorter.
Flowchart Of Conditional/ Ternary Operator In C
Ternary Operator In C Example
Here is a very simple example of the conditional operator in C, where we are trying to find a maximum of two numbers.
Program Code:
Output:
Enter any two numbers
45 33
45 is the larger from the given numbers.
Explanation:
In this sample C program-
- We begin by including the stdio.h header file since it consists of the essential input/ output operations.
- Next, we initiate the main() function, which is the entry point of execution of the program.
- Inside the main(), we declare three variables of integer data type, i.e., a, b, and max.
- Then, using the printf() function, we display a message to the console that prompts the user to input two integers. We also use the newline escape sequence to move the cursor to the next line.
- The program reads the user-input numbers with the scanf() function, and these are stored in the variables a and b. Here, we use the %d format specifier inside the formatted string as a placeholder for the integer values.
- Next, we initialize the third variable, max, by using the ternary operator to determine which of a and b is greater, as mentioned in code comments. Here-
- The initial condition is to check if a is greater than b. If the result of this condition is true, then the value of a will be assigned to the variable max.
- If the condition is false, then the value of variable b will be assigned to max.
- Once the conditional operation is done, we use the printf() function twice to display the value of max, along with a phrase. Note that since we don't use the newline (\n) in these statements, the output gets printed in a single line.
- Finally, the program terminates execution with a return 0 statement.
Ternary Operator In C: A Shorter Version Of If-Else Conditional Statements
As we discussed above, the conditional (ternary) operator is an alternative to the if-else statement as it serves as a shorter version, wherein we just have to write a single line of code instead of 4-5 lines of if-else blocks, and this makes our code faster and concise. To better understand how it shortens the code, let’s look at the difference between the syntax of both methods.
If- Else Conditional Statements
if (condition) {
// code block executed if the condition is true
}else {
// code block executed if the condition is false
}
Ternary Operator
(condition) ? value_if_true : value_if_false;
Comparing the syntax gives a clear idea of how the ternary operator reduces the code and serves as a shorter alternative to if-else statements. This concise structure is particularly useful when a simple decision needs to be made in a single line of code, enhancing code readability and reducing verbosity.
For example, instead of writing multiple lines with if-else statements, a programmer can use the ternary operator to achieve the same result more succinctly. This can lead to cleaner and more compact code, especially in situations where the decision logic is straightforward.
Some Important Remarks On Ternary Operator In C
The ternary/ conditional operator in C is a powerful tool for concise conditional expressions, but there are some important remarks and considerations to keep in mind when using it.
- Conciseness vs. Readability: While the ternary operator can make code concise, it's essential to balance conciseness with readability. Overuse or nesting of ternary operators, especially in complex expressions, can lead to code that is difficult to understand.
- Avoiding Side Effects: Be cautious when using the conditional operator with expressions that have side effects. Both the true and false expressions are always evaluated, which might lead to unintended consequences if the expressions modify variables or have other side effects.
- Use for Simple Conditions: The ternary operator in C programs is most suitable for simple conditions and expressions. For more complex conditions or multiple statements, using if-else statements is generally more readable and maintainable.
- Parentheses for Clarity: When using the conditional operator within more complex expressions, consider using parentheses to ensure clarity and to explicitly define the order of evaluation.
- Assignment and Return Value: The ternary operator can be used for inline assignment. It provides a concise way to return values based on conditions, making it useful in function return statements.
- Not a Replacement for if-else: The ternary operator is not meant to replace if-else statements entirely. Both have their places in the code, and the choice between them depends on the specific requirements and context.
- Avoiding Nested Ternary Operators: Excessive nesting of ternary/ conditional operators in C code can reduce its readability. If conditions become too complex, it's often better to use if-else statements, as they offer improved clarity.
- Type Consistency: Ensure that the true and false expressions in the ternary operator have consistent types to avoid unexpected type conversions or errors.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Nested Conditional Operator In C
When we use one or more conditional (ternary) operators within a ternary operator, it becomes the nested form and is hence referred to as a nested conditional operator. This helps to evaluate multi-level conditions in a single line of code.
Let us look at its syntax for better understanding:
(condition1) ? (statement3) : (condition2) ? (statement1) : (statement2);
Here,
- Condition 1 and 2 are the base conditions for the ternary operator.
- Statements 1, 2, and 3 refer to the actions to be performed/ code statements to be executed, depending on if the conditions are true or false.
- First, the ternary operator involving condition 2 is evaluated, and whatever statement1 or statement2 returns serves as the expression that would be executed if condition1 returns False or 0.
But how do we execute them? What pattern do we follow when dealing with nested ternary operators? For this, we should understand the associativity of the conditional operator in C, which we have discussed in a later section.
Nested Conditional Operator In C Example:
Output :
The number 7 is Odd number.
Explanation:
The example C program above determines the characteristics of a given number (num) and prints the result. Here-
- We begin by including the essential header file and initiate the main() function.
- Then, we declare and initialize an integer variable, num, with the value 7.
- Next, we declare a pointer variable result and use the nested ternary operator to assign a value to it. In that-
- There are two base conditions, i.e., the outer condition num>0, which determines if the number is positive or not.
- If the outer condition is true, then the inner condition/ternary operator is evaluated. But if the condition is false, then the result variable is initialized with the phrase- Negative number.
- Now, if the outer condition is true, we move to the inner condition num % 2 == 0, which determines if the number is even or odd.
- If the number is, in fact, even, then we assign the phrase- Even number to the result variable. If the number is odd, then the program assigns the phrase- Odd number to the result.
- After the evaluation of the nested ternary operator, we use the pintf() function to display the value of the result to the console.
- Inside the print statement, we use the %d and %s specifiers as placeholders of values for num and result.
- Finally, the program completes execution successfully.
Note- This example shows how nested conditional operators simplify and make the code cleaner. However, one should be careful when using nested operators since it might make the code complicated and confusing if the base conditions are complex.
Associativity Of the Conditional Operator In C
The conditional operator (? :) in C is right-associative. This means that if there are multiple conditional operators in a single expression, the rightmost one is evaluated first.
For example:
int a = 5, b = 10, c = 15;
int result = (a > b) ? (a > c) ? a : c : (b > c) ? b : c;
In this expression, the right-associativity of the conditional operator means that the rightmost operator, i.e., (b > c) ? b : c is evaluated first. This is followed by the next rightmost expression, i.e., (a > c) ? a : c, and finally the leftmost (a > b) ? and so on
Note- It's important to note that the associativity of the conditional operator affects the order of evaluation but doesn't affect the grouping of the operands. Parentheses can be used to explicitly specify the grouping and ensure the desired order of evaluation.
Also read- Compilation In C | A Step-By-Step Explanation & More (+Examples)
Assigning Ternary Operator In C To A Variable
We can assign the result obtained from a ternary operator to any variable of appropriate type. This helps us to compare, compute, execute, and store results in a single line of code. Below is an example that shows how you can achieve this.
Code Snippet:
Output:
Moderate
Explanation:
This C program categorizes a temperature value (temperature) into different categories (i.e., Hot, Moderate, or Cold) and uses the ternary operator to evaluate and print the result.
- We begin by declaring an integer variable temperature and assign the value 25 to it inside the main() function.
- Next, we declare a pointer variable called status, which will store the category in which the given temperature falls.
- We use the nested ternary operator to determine the category and assign the value to status, as follows-
- The outer condition checks if the temperature is greater than 30. If it is true, then the string value- Hot is assigned to status.
- If not, then we move to the second condition, which checks if the temperature is greater than 20.
- The status variable will be assigned the string value- Moderate if the inner condition is true. If not, then it will assign the string value Cold.
- After the evaluation is done, we use the printf() statement with a formatted string to display the value of the status variable.
Examples Of How To Use Ternary Operator In C Program
Ip until now, we have seen how the conditional (ternary) operator in C can be used in place of if-else statements and execute such conditional statements in a single line of code. We also looked at the syntax, flowcharts, and a few examples to understand the concept better.
Now, it is time to look for more examples where ternary operators can prove helpful.
Example 1:
Output:
17 is a prime number.
Explanation:
In this program, we check whether a given number (number) is a prime number.
- First, we initialize an integer variable number with the value 17.
- We then declare another variable called isPrime and use the ternary operator to determine its value. In that-
- The outer condition (number <= 1) checks if the number is less than or equal to 1. If true, isPrime is set to 0 (not prime).
- If the outer condition is false, the program moves to check the inner condition (number <= 3).
- If the inner condition is true, isPrime is set to 1 (prime).
- If both previous conditions are false, the program checks the third condition ((number % 2 == 0) || (number % 3 == 0)). If true, isPrime is set to 0 (not prime). Here, we use the logical OR operator, which produces true only if one of the operands is true.
- In case all previous conditions are false, isPrime is set to 1 (prime).
- After the ternary evaluation is done, we use the printf() statement to display the output.
Example 2:
In this example of a C program, we check if a given year is a leap year or not.
Code:
Output:
2024 is a leap year.
Explanation :
In this example-
- We declare and initialize the year integer variable with the value 2024 inside the main() function.
- Then, we declare another variable called isLeapYear and use the ternary operator to assign a value to the variable. In that-
- The outer condition uses the logical OR operator to compare two conditions.
- Of these, the left-hand operant (i.e., year % 4 == 0 && year % 100 != 0) checks if the year is divisible by 4 and not by 100. And the right-hand operant checks if the year is divisible by 400.
- If the condition is true, isLeapYear is set to 1 (indicating a leap year); otherwise, it's set to 0 (not a leap year).
- After that, we start an if-else statement to check the value of the isLeapYear variable. If isLeapYear is true, then the conditional code inside the if block is executed. It uses the printf() statement to output- the year is a leap year.
- If the condition is not true, then the block inside the else block is executed, and the printf() function outputs- the year is not a leap year.
Difference Between If-else Statement & Conditional Operator In C
The if-else statement and the conditional(ternary) operator have the same functionality, but their syntax and lines of code are different, which makes everything from complexity to readability different.
That is, ternary operators are more compact, concise, and clear and generally used for simple conditions. Whereas if-else statements provide more flexibility and readability when we are dealing with multiple code execution paths. The table below lists the primary difference between these two.
Feature | Conditional Operator (?;) | if-else Statement |
---|---|---|
Syntax | condition? expression_if_true : expression_if_false; | if (condition) { // Code to execute if true } else { // Code to execute if false } |
Use Cases | Concise conditional assignments in expressions. | Complex conditional branching and multiple statements. |
Return Value | Returns a value based on the evaluation of the condition. | Does not have a direct return value. |
Readability | Concise and compact syntax, suitable for simple conditions. | More verbose but potentially clearer for complex conditions. |
Side Effects | May be prone to issues with side effects due to always evaluating both expressions. | Better control over side effects as only the relevant block is executed. |
Example Of Conditional Operator In C:
Output:
The maximum value is: 20
Explanation:
- In the C program for ternary operators, we declare and initialize two integer variables x and y, with the values 10 and 20, respectively.
- We then declare a third variable, max, and use the ternary operator to assign it the max of the values of x and y.
- So, the condition checks if x is greater than y. If this is true, then max is assigned the value of x. Otherwise, it's assigned the value of y.
- Lastly, we use printf() to display the output before the program finishes execution.
Example Of If-Else Statement In C
Output:
15 is odd.
Explanation:
In the example-
- We initialize the integer variable number with the value 15.
- Then, we create an if-else statement to check if the number is even or odd.
- If the number is divisible by 2, that means it is even. Then, the code inside the if-block is executed, which uses printf() to output that number is even.
- If the condition is false, then the else block is executed, and the program prints that the number is odd.
- Finally, the program closes with a return 0 statement indicating no errors.
This clearly shows how concise ternary operators are, and it highlights the readability of if-else statements. Both the ways of comparing and executing have their own benefits and can be implemented according to the purpose.
Benefits of Ternary Operator In C
- Conciseness: The ternary operator allows for a more compact representation of simple conditional statements, making the code concise and readable. It also makes for efficient code in comparison to conditional statements.
- Expressiveness: It can enhance code readability by succinctly expressing conditional logic in a single line, reducing the need for multiline if-else expressions.
- Inline Assignment: The conditional operator in C facilitates the inline assignment of values based on conditions, eliminating the need for additional temporary variables.
Conclusion
The ternary operator in C is a valuable tool for simplifying conditional expressions and enhancing code readability. By providing a concise syntax for expressing simple conditions, it enables developers to write more compact and expressive code. However, it is crucial to use the conditional operator in C judiciously, keeping in mind readability and avoiding unnecessary complexity. With a clear understanding of its syntax and applications, developers can leverage the ternary operator effectively in their C programs.
Also read- 100+ Top C Interview Questions With Answers (2023)
Frequently Asked Questions
Q. What are the different types of operators in C?
In the C programming language, operators are symbols that represent computations and manipulations of variables and values. C operators can be broadly classified into the following categories:
- Arithmetic Operators (+, -, *, /, %)
- Relational Operators (==, !=, <, >, <=, >=)
- Logical Operators (&&, ||, !)
- Bitwise Operators (&, |, ^, ~, <<, >>)
- Assignment Operators (=, +=, -=, *=, /=, %=)
- Increment and Decrement Operators (++, --)
- Conditional (Ternary) Operator (? :)
- Miscellaneous Operators: sizeof, ,, ->, &, *
These operators serve various purposes, including arithmetic calculations, comparisons, logical expressions, bitwise manipulations, assignment shortcuts, and more.
Q. What is the meaning of ternary?
Ternary basically means something that is three in number. In the case of ternary operators in C, this refers to the three expressions that we use to analyze a condition and help in decision-making.
- The syntax for the ternary operator, also known as the conditional operator, is- (condition ? expression_if_true : expression_if_false) in C.
- It takes three operands. i.e., a condition to be evaluated, an expression to be executed if the condition is true, and another expression to be executed if the condition is false.
- The result of the ternary operation is determined based on the conditional evaluation.
Q. Can we use ternary operators for multiple conditions?
Yes, you can use nested ternary operators to handle multiple conditions in a concise manner. However, it's important to note that excessive nesting of ternary operators can lead to code that is difficult to read and understand. It's generally recommended to use nested ternary operators only for simple, straightforward conditions to maintain code readability.
Here's an example of the nested conditional operator that is also known as a nested ternary operator in C-
Code:
Output:
The maximum value is: 20
Explanation:
In this example, the nested ternary operator is used to find the maximum of two numbers (x and y).
- If x is greater than y, the x is chosen as the maximum and assigned to the max variable.
- If not, it checks whether y is greater than 0. If true, then y is chosen as the maximum.
- Otherwise, 0 is chosen as the maximum and assigned to the variable.
Q. What are the 3 conditions of the ternary operator in C?
The ternary operator in C has three components or conditions, and they are-
- Condition: The first part of the ternary operator is the condition, which is a ternary expression that evaluates to either true or false. It is followed by a question mark ?.
- Expression if True: The second part is the expression that is evaluated if the intial condition statement evaluated to be true comparison. It comes after the question mark sign (?) and is separated from the second expression by a colon (:).
- Expression if False: The third part is the expression that is evaluated if the initial condition evaluates to be a false comparison. It comes after the colon (:) sign in the ternary operator expression.
The general syntax of the ternary operator in C is as follows:
condition ? expression_if_true : expression_if_false;
Q. Can we use continue or break in ternary operators?
No, the continue and break statements cannot be used directly within a ternary operator in C. The ternary/ conditional operator is designed for evaluating simple expressions based on a condition and providing a concise syntax for conditional assignments. It does not support the inclusion of statements, and continue and break are control flow statements rather than expressions.
Q. What is the difference between unary, binary, and ternary operators in C?
There are three primary types of operators in C, that are classified based on the number of operands they take. These are- unary, binary, and ternary operators.
Unary Operators |
Binary Operators |
Ternary Operators |
Unary Operators require only one operand to perform any action. |
Binary Operators require two operands to perform any action. |
Ternary Operators require three operands to perform any action. |
Examples: sizeof(), increment (++), decrement (--) etc. |
Examples: Arithmetic, logical, bitwise operators, comma operator, etc. |
Example: Ternary conditional operators in C (?:) |
You might also be interested in reading the following:
- Difference Between C And C++ Explained With Code Example
- Identifiers In C | Rules For Definition, Types, And More!
- 5 Types Of Literals In C & More Explained With Examples!
- Tokens In C | A Complete Guide To 7 Token Types (With Examples)
- Keywords In C Language | Properties, Use, & Detailed Explanation!
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment