Table of content:
- Types of Memory Allocation
- Static Memory Allocation
- Dynamic Memory Allocation
- Similarity between Static and Dynamic Memory Allocation
- Difference between Static and Dynamic Allocation
- Conclusion
- FAQs
Differences Between Static And Dynamic Memory Allocation (Comparison Chart)
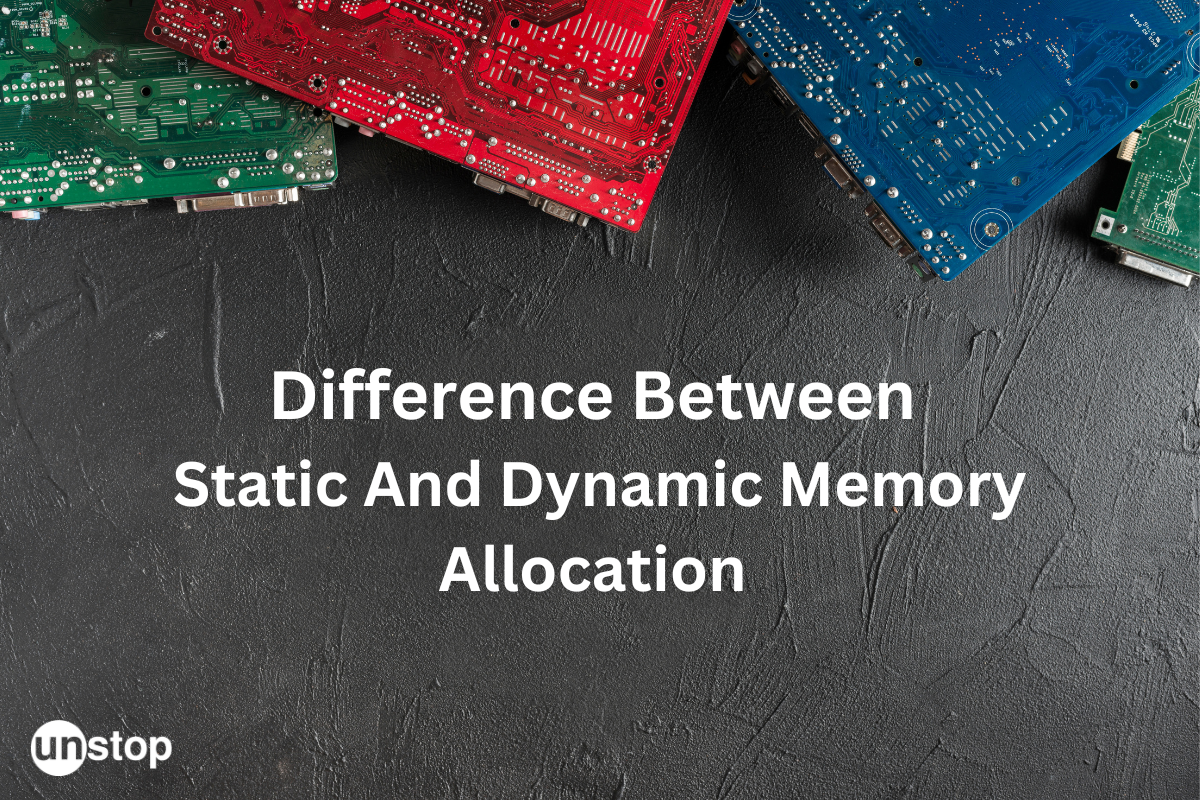
The effective management of the storage of data for programs is made possible by the fundamental idea of memory allocation in computer programming. For this, two well-known techniques for allocating memory are static and dynamic memory allocation.
Memory allocation refers to assigning a particular space in the memory of a computer for a program or code. This may include assigning a partial or complete memory space. A virtual or physical memory address space is assigned to a program consisting of data and instructions.
In this article, we will explore the differences between static and dynamic memory allocation, along with other details.
Types of Memory Allocation
There are two types of memory allocations, which are given as follows:
1. Static memory Allocation (Stack memory)
2. Dynamic memory Allocation (Heap memory)
The key difference between static and dynamic memory allocation is that static memory allocation allows fixed memory size after allocation, while dynamic memory allocation allows changes in the memory size after allocation.
Static Memory Allocation
Static Memory Allocation, also known as Compile-time Memory Allocation, is used for the allocation of memory during the compilation process of data in a fixed size. The compiler allocates memory for variables present in the program. It allows for efficient memory access.
The size of data required for the program execution is mentioned beforehand, before the initiation of the execution. If it is unknown, the Static content size is guessed by the system. Small size leads to inappropriate execution, and large size leads to wastage.
Here, the allocation process is not carried out during execution but is carried out before initiation of the process. This type is useful for arrays where the static keyword is used in the C programming language to assign a particular value to a variable.
Properties:
- The compiler allocates declared static variables, which can be accessed using the 'address of' operator assigned to a pointer variable.
- Static variables are permanently allocated.
- Memory allocation is carried out during compilation time.
- Stack Memory is used here.
- Static memory allocation scheme is fast but less efficient.
- Memory cannot be reused after the initial allocation.
- Memory cannot be resized after the initial allocation.
- The allocation process is simple.
- It is used in an array.
Advantages:
- Static Memory Allocation allocates memory to a variable, like a fixed array, which is declared 'ahead of time'.
- It is easy to use.
- Variables are permanently allocated and hence, cannot be changed which leads to improved performance.
- The execution speed time is efficiently controlled and has faster memory allocation.
Disadvantages:
- This method leads to memory wastage.
- If memory is not required, it cannot be freed. It remains unchanged permanently and cannot use unused memory.
Dynamic Memory Allocation
Dynamic Memory Allocation, also known as Run-time Memory Allocation, is used for the allocation of memory during the process of execution (Runtime) of data. It allows allocation to entities when the program is running for the first time.
It allocates exact space and size for the Dynamic content, and hence, allocation is done during the runtime, which decreases the wastage of space.
It creates overhead of every operation over the system repeatedly during the execution of the program, which makes the entire process slow. It provides flexibility in memory allocation by carrying out operations on different parts of the program, which also helps to reduce wastage.
Dynamic memory allocation process under the stdlib.h header file uses some functions to assign a value to a dynamic variable. There are 4 functions that are used here:
1. malloc function
The function malloc() is used to allocate a single block of the memory that has been requested. The initial value in this command is garbage since memory is not initialized during execution. It gives a NULL output when the memory is not enough. It allocated memory in bytes.
Syntax: ptr=(data_type*)malloc(byte_size)
2. calloc function
This command is used to assign multiple blocks of the requested memory, where the memory is initialized as all bytes to zero. It also gives NULL output in case the memory is not enough.
Syntax: ptr=(data_type*)calloc(number, byte_size)
3. realloc function
Realloc function is used to reassign the memory that has already been assigned and occupied by the malloc() or the calloc() function. This function argument is used to change the size of the memory allocated because the assigned memory space is not enough.
Syntax: ptr=realloc(ptr, new_size)
4. free()
free() command empties the memory that has been assigned and occupied by the malloc() or calloc() function of the program. If this function is not used then the program will keep on consuming memory.
Syntax: free(ptr)
Properties:
- Value is assigned to a pointer variable, which is returned by different functions.
- Memory is allocated during execution.
- Memory can be allocated at any time and released at any time.
- Heap memory is used here.
- Dynamic memory allocation is slow but more efficient.
- Implementation of the program is complicated.
- Memory can be resized dynamically or reused.
- It is used in linked lists.
Advantages:
- It allows the allocation of memory at runtime.
- The memory of data structure size can be increased or decreased based on the requirements of the dynamic memory allocation.
- Memory wastage doesn't occur because it allocates exact memory space to the program to enhance the performance of the system, which leads to flexible memory usage.
Disadvantages:
- It requires more execution process time due to execution during runtime.
- Once the program is executed, it needs to be freed, or bugs are formed which are difficult to find.
Similarity between Static and Dynamic Memory Allocation
The similarities between both types of allocation memory are as follows:
1. They are both memory allocation mechanisms.
2. They both can be implemented manually with the help of a programmer.
Difference between Static and Dynamic Allocation
The major differences between static and dynamic memory allocation are:
Characteristic | Dynamic Memory Allocation | Static Memory Allocation |
Time of allocation | It allows the allocation of memory at execution time (Run-time). | It allows allocation of memory at compile time (Compile-time). |
Area of memory used for allocation | It uses heap memory for management and storage. | It uses the stack memory for management and storage. |
Memory Size and Memory Reusability | Dynamic memory is not fixed and can be reused and changed for different applications. | Static memory is fixed and hence cannot be reused for different applications. |
Nature | Memory can be allocated or changed at any point in time. | Allocation of memory is permanent and cannot be changed. |
Application | This is used in linked lists. | This is used in an array. |
Command in C programming language | For this type of allocation, there are various commands used like realloc(), malloc(), free(), and calloc(), all of them under the stdlib.h header file. | Static memory allocation can be carried out by stat() command. |
Ability to increase memory | It allows an increase in memory size during program execution. | It does not allow an increase in memory during the execution of the program. |
The time period for allocation | Memory can be allocated at any point of time during the program. | Memory is allocated from the beginning to the end of the program. |
Time of program execution. | Execution is slower. | Execution is faster and saves running time |
The efficiency of the allocation scheme | A dynamic memory allocation scheme is more efficient than a Static Memory Allocation scheme. | Static Memory Allocation Scheme is less efficient. |
Implementation of program | This process is complicated or complex for dynamic allocation. | This process is simple for static allocation. |
Functions for C programming language |
The following functions specify it: a. malloc() function Syntax: malloc (number *sizeof(int)); b. calloc() function Syntax: calloc (number, sizeof(int)); c. realloc() function Syntax: realloc (pointer, number * sizeof(int)); d. free() function Syntax: free (pointer); |
The static keyword specifies it static int m=3; Here, 'm' variable is of integer value data type and is equal to 3. |
Conclusion
This article is aimed at providing the users an insight into the following concepts:
- Memory allocation is a process of assigning a particular space in the memory to a computer program or code such that the data of the user can remain stored in the system.
- There are two mechanisms that can be used to allocate memory; these include Static Memory Allocation and Dynamic Memory Allocation.
- Static Memory Allocation, which refers to the memory allocation technique, is used for the allocation of memory during the compilation process of data in a fixed size, such that it cannot be changed, reused, or resized.
- Dynamic Memory Allocation, also known as Run-time Memory Allocation, is used for the allocation of memory during the process of execution (Runtime) of data, where it can be resized, reused as well as changed.
- They are similar in terms of their mechanisms and the fact that they both can be operated manually.
- The key difference between static and dynamic memory allocation is that static memory allocation allows fixed memory size after allocation, while dynamic memory allocation allows changes in the memory size after allocation.
FAQs
1. What is memory allocation in C?
In C, the process of allocating memory to variables and data structures used in a program is referred to as memory allocation. There are two methods for allocating memory: static and dynamic. Static memory allocation is done before program execution by the compiler and is used for declared variables, and the size of the memory is fixed and cannot be changed during program execution. As opposed to this, dynamic memory allocation takes place while a program is running and is managed and serviced using pointers that refer to the freshly created memory space in a region known as the heap.
2. How does static memory allocation lead to wastage of memory?
Static memory allocation can lead to wastage of memory due to the following reasons:
Static memory allocation, which assumes that the program will always use a particular amount of memory, allots a set amount of memory space to a process at build time. The drawback is that if the program doesn't truly require that much memory, this might result in memory waste.
If the estimated size of memory required by the program is larger than the actual size needed, it will lead to memory wastage.
3. Is memory reuse possible in dynamic memory allocation?
Yes, memory reuse is possible in dynamic memory allocation. Dynamic memory allocation allows for flexibility in allocating memory size and can be reallocated if needed. The heap memory is unorganized and can be treated as a resource that can be used and released as needed. The user can allocate more memory when required and release the memory when it is no longer needed. Additionally, dynamic memory allocation allows for memory reusability, which means that once a variable is assigned memory, it can be used for other purposes as well.
4. What are memory allocation tools?
Memory allocation tools are software programs that help developers manage memory allocation in their programs. These tools can help detect memory leaks, optimize memory usage, and prevent memory-related issues. Here are some examples of memory allocation tools:
- GCeasy
Eclipse MAT
Valgrind
5. Which keyword is used to deallocate memory in C++?
The keyword used to deallocate memory in C++ is the delete keyword. It is used to release the memory that was dynamically allocated using the new operator. When the delete keyword is used, the memory is returned to the operating system, making it available for other uses.
6. What is a memory buffer?
In the field of computer science, a memory buffer is a section of memory that is used to store data while it is being transferred from one location to another. It serves as a storage hub between two things with dissimilar properties. Here are some crucial ideas about memory buffers:
- Normally, data is stored in a memory buffer either just prior to being delivered to an output device or as it is being retrieved from an input device.
- It can be implemented in software as a virtual data buffer that refers to a location in physical memory or in hardware as a fixed memory location.
7. What are some similarities between static and dynamic memory allocation?
Some similarities between static and dynamic memory allocation are:
- Both static and dynamic memory allocation are memory allocation mechanisms used in computer programming.
- They both can be implemented manually with the help of a programmer.
- They both allocate memory for variables and data structures used in a program.
Hope you found the article helpful. For more such content, stay tuned to Unstop!
You might also be interested in reading:
- What Is The Difference Between Procedural And Object-Oriented Programming?
- What Is The Difference Between HTML and CSS?
- Difference Between DBMS And RDBMS: Why RDBMS Is An Advanced Version Of DBMS?
- What Is The Difference Between Data And Information? Explained
- Computer Is Just A Box Of Metal Without These Programmers
I am a biotechnologist-turned-content writer and try to add an element of science in my writings wherever possible. Apart from writing, I like to cook, read and travel.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Blogs you need to hog!
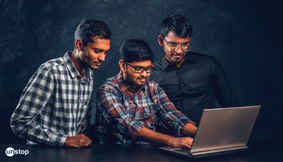
This Is My First Hackathon, How Should I Prepare? (Tips & Hackathon Questions Inside)
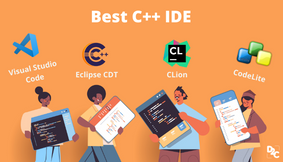
10 Best C++ IDEs That Developers Mention The Most!
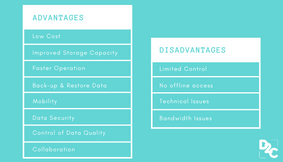
Advantages and Disadvantages of Cloud Computing that you should know!
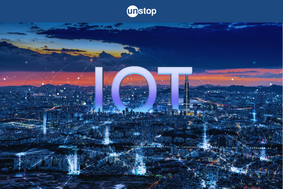
Comments
Add comment