Table of content:
- NTT DATA Interview Questions
- NTT DATA Interview Questions: HR Round
Want To Work At NTT Data? Revise These NTT Data Interview Questions
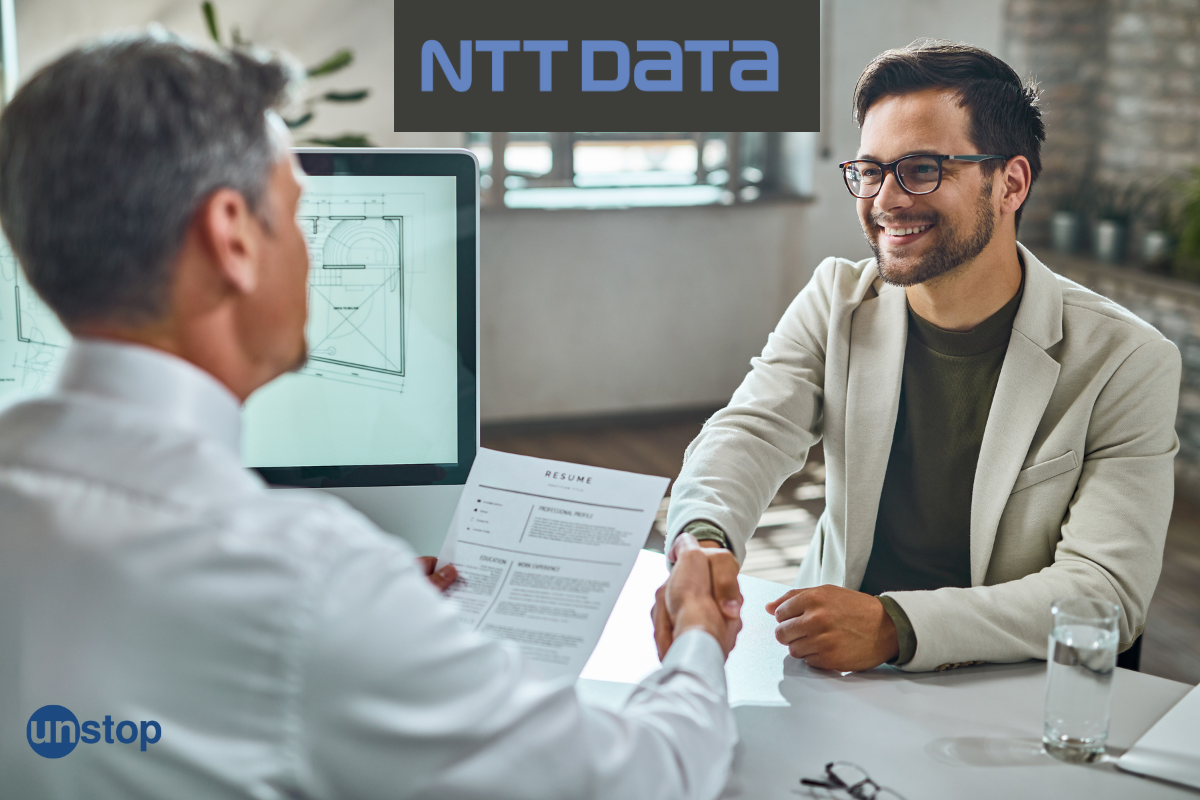
NTT Data is a Japanese multinational information technology (IT) services company headquartered in Tokyo, Japan. Through consulting, industry solutions, business process services, IT modernization, and managed services, it is a reputable global innovator of IT and business growth that aids a range of clients in transforming agile projects.
The company has a consultative approach and deep industry expertise that delivers practical and scalable IT solutions to accelerate digital journeys. NTT DATA is a partially-owned subsidiary of Nippon Telegraph and Telephone (NTT), which is Japan's state-owned telecommunications company.
It offers a lot of opportunities in the IT field and is a dynamic and forward-thinking company. If you are interested in NTT DATA company, you must go through this blog which covers all the important technical interview questions.
NTT DATA Interview Questions
Q1. How to sort an array in Java with duplicate values?
We can sort an array with duplicate values with the help of the counting sort algorithm. The counting sort algorithm will create a new array to store counts of elements in the input array. It will sort the input array by iterating through the input array and incrementing the count of each element in the count array. Finally, it will iterate through the count array to output the sorted array.
Sample code of counting sort array:
Let’s sort an array of integers: [4, 2, 3, 4, 1, 2, 4, 3]
import java.util.Arrays;
public class CountingSortExample {
public static void countingSort(int[] array) {
// Step 1: Determine the range
int min = Arrays.stream(array).min().getAsInt();
int max = Arrays.stream(array).max().getAsInt();
int range = max - min + 1;
// Step 2: Create a count array
int[] count = new int[range];
// Step 3: Count the occurrences
for (int i = 0; i < array.length; i++) {
count[array[i] - min]++;
}
// Step 4: Calculate cumulative counts
for (int i = 1; i < range; i++) {
count[i] += count[i - 1];
}
// Step 5: Create the sorted array
int[] sortedArray = new int[array.length];
for (int i = array.length - 1; i >= 0; i--) {
sortedArray[count[array[i] - min] - 1] = array[i];
count[array[i] - min]--;
}
// Step 6: Output the sorted array
System.arraycopy(sortedArray, 0, array, 0, array.length);
}
public static void main(String[] args) {
int[] array = {4, 2, 3, 4, 1, 2, 4, 3};
System.out.println("Original array: " + Arrays.toString(array));
countingSort(array);
System.out.println("Sorted array: " + Arrays.toString(array));
}
}
Sample answer:
Original array: [4, 2, 3, 4, 1, 2, 4, 3]
Sorted array: [1, 2, 2, 3, 3, 4, 4, 4]
Q2. Find the smallest array sorting window that will sort the entire array in JAVA.
We can use the sliding window approach to find the smallest array sorting window. The sliding window approach is a computational technique that is used to solve problems that require checking the answer of some ranges inside a given array or list.
By consolidating two nested loops into a single loop, the sliding window technique's primary goal is to reduce the algorithm's temporal complexity. When we need to locate a continuous subarray or substring of a specific length that meets the criteria, we can use this method to travel over an array or list in a single loop.
A window is moved over an array in the sliding window strategy. Each window's result is computed, and the highest or lowest result observed is noted.
Sample Code
import java.util.Arrays;
public class SmallestSortingWindow {
public static int findSmallestSortingWindow(int[] arr) {
int n = arr.length;
// Find the first element out of sorting order from the beginning
int start = 0;
while (start < n - 1 && arr[start] <= arr[start + 1]) {
start++;
}
if (start == n - 1) {
// The array is already sorted
return 0;
}
// Find the first element out of sorting order from the end
int end = n - 1;
while (end > 0 && arr[end] >= arr[end - 1]) {
end--;
}
// Find the minimum and maximum elements within the unsorted window
int min = Integer.MAX_VALUE;
int max = Integer.MIN_VALUE;
for (int i = start; i <= end; i++) {
min = Math.min(min, arr[i]);
max = Math.max(max, arr[i]);
}
// Extend the window to include any elements that are greater than the minimum
while (start > 0 && arr[start - 1] > min) {
start--;
}
// Extend the window to include any elements that are smaller than the maximum
while (end < n - 1 && arr[end + 1] < max) {
end++;
}
// Calculate the size of the sorting window
return end - start + 1;
}
public static void main(String[] args) {
int[] arr = {3, 7, 5, 6, 9};
System.out.println("Original array: " + Arrays.toString(arr));
int windowSize = findSmallestSortingWindow(arr);
System.out.println("Smallest sorting window size: " + windowSize);
}
}
Sample Answer:
Original array: [3, 7, 5, 6, 9]
Smallest sorting window size: 3
Q3. In JAVA, which approach can be used to find the largest or smallest number from a set?
We can use the sorting and iteration approach to find the largest or smallest number from a set.
Sorting: We can sort the collection of numbers using a sorting algorithm like quicksort or merge sort and then access the first and last items in the sorted set to obtain the smallest and greatest numbers, respectively.
Iteration: The largest and lowest numbers can be tracked by using a loop to run over the set of numbers. This method entails comparing each number in the set to the largest and smallest variables, updating them as necessary, and initializing the largest and smallest variables to the smallest and largest feasible integer values, respectively.
Q4. Give an example of when you used binary search in real life.
A sorted list or array can be searched using the binary search method to locate a particular item. The search interval is divided in half repeatedly until the object is located or the search interval is empty.
An example of binary search in daily life is:
Finding a book in a library: Books are frequently sorted in alphabetical order or according to some numerical code. We can utilize binary search to locate the book we're seeking rather than linearly going through every book in the library.
Q5. How will you find the length of the longest path in the matrix so that the elements in the path are in their increasing order?
The length of the longest path in a matrix with ascending-ordered path components can be calculated using a dynamic programming technique. The 2D matrix dp, which can be maintained and has the same size as the input matrix, represents the length of the longest growing path that ends at the element (i, j) in the input matrix. Then, by doing a depth-first search (DFS) on the matrix, the dp array can be updated.
Example:
public class LongestIncreasingPath {
private static final int[][] DIRECTIONS = {{-1, 0}, {1, 0}, {0, -1}, {0, 1}};
public static int longestIncreasingPath(int[][] matrix) {
if (matrix == null || matrix.length == 0 || matrix[0].length == 0) {
return 0;
}
int rows = matrix.length;
int columns = matrix[0].length;
int[][] dp = new int[rows][columns];
int maxLength = 0;
for (int i = 0; i < rows; i++) {
for (int j = 0; j < columns; j++) {
maxLength = Math.max(maxLength, dfs(matrix, i, j, dp));
}
}
return maxLength;
}
private static int dfs(int[][] matrix, int i, int j, int[][] dp) {
if (dp[i][j] != 0) {
return dp[i][j];
}
int maxLength = 1;
for (int[] direction : DIRECTIONS) {
int newRow = i + direction[0];
int newColumn = j + direction[1];
if (newRow >= 0 && newRow < matrix.length && newColumn >= 0 && newColumn < matrix[0].length
&& matrix[newRow][newColumn] > matrix[i][j]) {
maxLength = Math.max(maxLength, 1 + dfs(matrix, newRow, newColumn, dp));
}
}
dp[i][j] = maxLength;
return maxLength;
}
public static void main(String[] args) {
int[][] matrix = {
{9, 9, 4},
{6, 6, 8},
{2, 1, 1}
};
int longestPathLength = longestIncreasingPath(matrix);
System.out.println("Length of the longest increasing path: " + longestPathLength);
}
}
OUTPUT:
Length of the longest increasing path: 4
Q6. Describe Quicksort.
A sorting algorithm called QuickSort uses the divide-and-conquer strategy. It operates by choosing a pivot element to split the input array into two sub-arrays.
The steps involved in quicksort are:
First, we will choose a pivot component from the array.
Then we will create two sub-arrays out of the array, one with elements fewer than the pivot and the other with elements greater.
Then to sort the full array, we will repeat the previous two steps for each sub-array.
Example:
import java.util.Arrays;
public class QuickSort {
public static void quickSort(int[] arr) {
if (arr == null || arr.length == 0) {
return;
}
quickSort(arr, 0, arr.length - 1);
}
private static void quickSort(int[] arr, int low, int high) {
if (low < high) {
// Partition the array into two parts
int pivotIndex = partition(arr, low, high);
// Recursively sort the two partitions
quickSort(arr, low, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, high);
}
}
private static int partition(int[] arr, int low, int high) {
// Choose the rightmost element as the pivot
int pivot = arr[high];
// Index of the smaller element
int i = low - 1;
for (int j = low; j < high; j++) {
// If the current element is smaller than or equal to the pivot
if (arr[j] <= pivot) {
i++;
// Swap arr[i] and arr[j]
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
// Swap arr[i+1] and arr[high] (or the pivot)
int temp = arr[i + 1];
arr[i + 1] = arr[high];
arr[high] = temp;
// Return the index of the pivot
return i + 1;
}
public static void main(String[] args) {
int[] arr = {7, 2, 1, 6, 8, 5, 3, 4};
System.out.println("Original array: " + Arrays.toString(arr));
quickSort(arr);
System.out.println("Sorted array: " + Arrays.toString(arr));
}
}
OUTPUT:
Original array: [7, 2, 1, 6, 8, 5, 3, 4]
Sorted array: [1, 2, 3, 4, 5, 6, 7, 8]
Q7. Tell me the use of ALTER DATABASE command?
The primary purpose of the ALTER DATABASE command is to modify the structure of a database.
We can alter, add, or drop columns and constraints, reassign and rebuild partitions, and disable and enable constraints and triggers. We can also alter the constraints, columns, or other features of an existing database by using the ALTER DATABASE command.
Example:
ALTER DATABASE NewDatabase
MODIFY FILE (NAME = NewDatabase_Data, FILENAME = 'C:\NewDatabase\NewDatabase_Data.mdf');
Q8. Tell me the SQL command that will return the year, month, day, species_id and weight in mg.
We can use the following SQL command:
SELECT YEAR(Date) AS Year, MONTH(Date) AS Month, DAY(Date) AS Day, SpeciesID, Weight * 1000 AS Weight_in_mg
FROM YourTableName;
Q9. Explain Javascript.
Javascript is a web technology programming language. It has first-class functions and is a lightweight, interpreted language. JavaScript enables us to create dynamically updating content, control multimedia, animate images, etc. It is a multi-paradigm that supports event-driven, functional, and imperative programming styles.
Q10. Tell me the names of Javascript concepts of the framework.
Some JAVASCRIPT concepts of the framework are:
- React
- AngularJS
- Vue.js
- Ember.js
- Node.js
- Svelte.js
- Gatsby.js
- Meteor.js
- Next.js
- Jest
- Backbone.js
Q11. Explain the design pattern.
Design patterns are used by software developers to address frequent issues when creating a system or application. They are solutions to typical issues that software developers run across when creating new software, discovered over time through trial and error by many software engineers. Experienced developers of object-oriented software employ design patterns as their best practices.
Design patterns are typically categorized by their intent and divided into three groups: creational patterns, structural patterns, and behavioral patterns.
Q12. Explain MVC.
According to MVC, Model, View, and Controller are the three primary logical components that make up an application. MVC is the most widely used industrial standard web development framework for building scalable and flexible projects.
- Model: All of the user's data-related logic is represented by the Model logic component.
- View: The view component implements an application’s whole UI functionality. It creates an interface for the user.
- Controller: The controller logic functions as a mediator between the views and the model.
Q13. Do you know the name of the methods to join two tables in SQL?
We can join two tables in SQL by using INNER JOIN, LEFT JOIN, RIGHT JOIN, FULL JOIN, and CROSS JOIN.
Q14. Explain Oracle data integrator.
Oracle Data Integrator (ODI) is a comprehensive data integration platform that provides users with a new declarative design approach to define data transformation as well as integration processes. It is an extract, load, and transform (ELT) tool produced by Oracle, which provides a graphical platform for creating, managing, and maintaining data integration procedures in business intelligence systems.
Q15. Tell me some advantages of using DBMS.
Some advantages of DBMS are:
- Better decision-making: DBMS provides with Better and more consistent data which means that high-quality, usable information can help users in making decisions with the exact data they need.
- Simplicity: DBMS provides a simple way of managing data.
- Recovery and backup: DBMS provides a way to recover and backup data in case of any system failure or data loss.
- Improved data integration: Due to the database management system, we have access to synchronized, well-managed data that is simple to use. Additionally, it provides an integrated view of how a specific organization operates and maintains track of one area of the business that affects another one.
Q16. Explain Relational Data Base Management System (RDBMS).
Relational Database Management System is the extended form of RDBMS. In RDBMS, Data is organized in tables made up of rows and columns in this form of the database management system. Each table in the database represents a particular database object, such as users, products, orders, and so ON. Data security, accuracy, integrity, and consistency are all maintained by the RDBMS's functions.
Q17. What is std?
'std' is a namespace in C++ that holds the standard library's built-in classes and defined functions.
A namespace is a technique to put similar components like variables, functions, and classes under one common name.
Q18. Tell me the full form of STL.
In C++, STL stands for Standard Template Library. The STL includes containers, iterators, algorithms, and function objects.
Q19. Tell me the points of dissimilarities between C++ and JAVA.
Some differences between C++ and JAVA are:
- Memory management: C++ supports manual object management with the help of keywords- new and delete. Whereas Java has built-in automatic garbage collection.
- Platform dependence: Java is platform-independent, whereas C++ is platform-dependent and requires compilation on every platform.
- Object management: Java has an integrated automatic garbage collection feature, whereas C++ provides manual object management using the new and delete keywords.
Q20. What do you mean by object cloning?
object cloning is a process to create an exact copy of an object, and it can be done in Java using the clone() method of the Object class. The class whose object clone we want to create must implement the Cloneable interface
Q21. Can you tell me the names of some data-structure operations?
Some data structure operations are:
- Traversing: It means systematically going from one element in the data structure to the next.
- Insertion: it refers to including a component in a data structure.
- Deletion: It is the removal of a component from a data structure.
- Searching: Finding a certain element in the data structure through search
- Sorting: It involves putting the components in a particular order.
- Accessing: obtaining a data structure element.
Q22. Explain the local variables and global variables in Python.
- Local Variables: These are those variables that are declared inside a function. Note that one can access these variables only from within that function.
- Global Variables: The term global variables is used to refer to those that are declared outside a function. Everyone has access to global variables, both inside and outside of functions.
Example:
global_variable = "I am a global variable"
def example_function():
local_variable = "I am a local variable"
print("Inside the function:")
print("Local variable:", local_variable)
print("Global variable:", global_variable)
# Calling the function
example_function()
print("\nOutside the function:")
# Attempting to access the local variable outside the function will result in an error
# print("Local variable:", local_variable)
# Accessing the global variable outside the function works fine
print("Global variable:", global_variable)
OUTPUT:
Inside the function:
Local variable: I am a local variable
Global variable: I am a global variable
Outside the function:
Global variable: I am a global variable
Q23. Tell me the names of some data types in Python.
Some data types in Python are:
- Numeric types: int, float, complex
- String type: str
- Sequence types: list, tuple, range
- Mapping type: dict
- Set types: set, frozenset
- Boolean type: bool
- Binary types: bytes, bytearray, memoryview
Q24. Do you know to create classes in Python?
We can create classes in Python by using the class keyword.
Example:
class MyClass:
x = 5
def my_method(self):
print("Hello, World!")
my_object = MyClass()
my_object.my_method()
Q25. What is merge sort?
Merge sort separates an array into smaller subarrays, sorts each subarray, and then combines the merged subarrays to get the final sorted array.
Example:
def merge_sort(arr):
if len(arr) > 1:
mid = len(arr) // 2
left_half = arr[:mid]
right_half = arr[mid:]
merge_sort(left_half)
merge_sort(right_half)
i = j = k = 0
while i < len(left_half) and j < len(right_half):
if left_half[i] < right_half[j]:
arr[k] = left_half[i]
i += 1
else:
arr[k] = right_half[j]
j += 1
k += 1
while i < len(left_half):
arr[k] = left_half[i]
i += 1
k += 1
while j < len(right_half):
arr[k] = right_half[j]
j += 1
k += 1
arr = [64, 34, 25, 12, 22, 11, 90]
merge_sort(arr)
print(arr)
OUTPUT
[11, 12, 22, 25, 34, 64, 90]
Q26. Describe denormalization.
Denormalization is a database optimization approach that enhances the readability of a database by adding redundant copies of data or by grouping data. It is a strategy used on a previously-normalized database to increase performance. Denormalization involves adding back specific instances of redundant data after the data structure has been normalized.
Q27. Describe DLL.
A doubly linked list (DLL) is a type of linear data structure that combines the next pointer and data from a singly linked list with an additional pointer, usually referred to as the previous pointer.
DLLs have various applications, like:
- They are used in navigation systems where front and back navigation is required
- Used in Implementing backward and forward navigation in web browsers
- Used in Implementing undo-redo features
- Helps in Constructing the most recently used and least recently used cache
- It is used in Constructing different data structures such as hash tables, stacks, etc.
Q28. Explain Real-Time Operating Systems (RTOS).
An operating system known as an RTOS was created For real-time computer applications, which can manage data and events under severe time limits. An RTOS is different from a time-sharing operating system.
Real-time operating systems are employed in situations when a lot of events, most of which are external to the computer system, need to be acknowledged and handled quickly or by a specified deadline. Examples of such applications include real-time simulations, telephone switching devices, industrial control, etc.
Q29. Describe Banker's algorithm.
The Banker's algorithm is a resource allocation and deadlock avoidance algorithm that simulates allocating the predetermined maximum possible amounts of all resources, then performs an "s-state" check to look for potential activities before deciding whether allocating should be permitted to continue.
Banker's algorithm is used in computer systems to avoid deadlock so that resources can be safely allocated to each process.
Q30. Name some Artificial Intelligence (AI) development platforms.
Artificial Intelligence development platforms are:
- Google Cloud AI Platform
- Microsoft Azure AI
- Google Dialogflow
- Viso Suite
- TensorFlow
- PyTorch
- Scikit-learn
- Google Cloud Learning Machine
Q31. What is Data-Driven Test?
A data-driven test involves writing test scripts that read test data or output values from data files rather than repeatedly using the same hard-coded values. In this manner, testers may assess how well the application manages multiple inputs.
Q32. Describe method overloading.
In the object-oriented programming method, the overloading feature enables a class to have numerous methods with the same name but distinct parameters.
In method overloading, the objects behave differently depending on the context in which they are used.
NTT DATA Interview Questions: HR Round
The last round in the NTT Data interview process is the HR round. Within the recruitment process, technical rounds test an individual's technical skills and knowledge and may include questions on programming language, operating systems, etc. On the other hand, the HR round includes behavioral interview questions that test an individual's communication skills, attitude towards work, an array of personalities, etc., to see how prospective employees fit into the company culture.
Here is a list of sample questions that individuals may encounter in this round:
Q1: How do you maintain a work-life balance?
When it comes to work-life balance, compartmentalization helps. I make a conscious effort not to take work pressure home and find time for activities that interest me outside of work. At the same time, I'm aware of NTT Data's human-centric approach to work culture and flexible work style. Naturally, this provides the reassurance and confidence that work-life balance would not be a distant dream at NTT Data Corporation.
Q2: How do you handle tight deadlines?
When it comes to such questions, the interviewer is testing your problem-solving skills. A good answer is one where you give actionable responses explaining your approach. If you have any specific example of working under a tight deadline, you can include that in the answer. Here is a sample:
With tight deadlines, I adopt a divide-and-conquer approach i.e., I divide the work into smaller, manageable tasks and conquer the most urgent tasks first. Depending on the work requirements, I may even divide the tasks on the basis of difficulty level. This ensures that even in the face of a tight deadline, the quality of work is not compromised. Dividing the work into manageable tasks also acts as a form of positive reinforcement where every completed task motivates you to move on to the next - instead of being overwhelmed by the deadline.
Q3: What drives you towards success?
Such subjective questions are common in the HR round. Here the interviewer wants to understand your motivation to see how the company can best serve it. The aim is also to judge your ability to answer questions not rooted in statistics or data.
As a software engineer, the idea of creating something new that not only has an impact on clients but also improves my knowledge is how I define success.
Q4: What are some leadership roles or skills that a manager must have?
With this question, the interviewer wants to see the management style you best respond to. Be sure to mention qualities that you think are compatible with your working style.
According to me, active listening skills, a positive attitude, and the ability to delegate without micromanaging are essential leadership skills for any manager. It's also important that a manager is a team player who works with the team to prepare prospective leaders.
Q5: How will you be able to handle it if technology is upgraded at NTT?
Though the question focuses on technology changes, your answer displays how you adapt to changes in the company and whether you can maintain efficiency in the face of changes.
I'm aware that the company philosophy at NTT Data revolves around innovation and I'm well prepared to adapt to technology upgrades for professional growth. I shall embrace any technology upgrade by understanding how it differs from existing technology, learning any niche skills it may require, and mastering the desirable skills through continuous practice.
Q6: Tell us about the last project you worked on in your spare time.
With this question, an interviewer is testing your domain knowledge and trying to understand the value you bring to the company. When mentioning a project, be sure to mention results that were data-driven and can factually support the achievements you're listing.
I was involved in an application development key project that incorporates both features of a food delivery app and a social networking app. It helps in increasing food sales. And it helps clients discover new dishes to try while also availing great deals. It was a blank project where I used to customize data according to my preference. The implementation of the project helped me to incorporate several features in my workplace projects as well.
Q7: What's your stance on working night shifts?
There can be times when the role you're applying for requires relocation, night shifts, etc. In such cases, answer honestly - there's no point in cracking an interview but landing a shift that you'll not be able to work. Also, be sure to mention any help or support you may need in working an irregular shift.
I am comfortable working night shifts in front-end and back-end processes. Though actual timings may differ from company to company, I'm well aware that working in shifts is a prerequisite in the software industry.
Q8: Tell me about yourself in brief.
This is an excellent opportunity to highlight your strengths in a creative manner while also listing your achievements that may not have made it to the resume. Essentially, this question allows the interviewer to see a side of you that may not be apparent in the resumes or through technical interviews.
I am a budding DIY enthusiast, and while there were a few half-finished projects that lined my house at one time, I'm proud to say most are now complete. Because I don't give up on a project just because it's new or challenging, and I understand the importance of sticking to a budget. Additionally, if I wasn't a software engineer, I'd be an event manager because I spent my college days organizing multiple fests, interacting with various key teams, and effectively pushing myself out of my comfort zone to develop as a person and a leader.
Q9: What is the toughest decision you ever made?
Making tough decisions is part of any job or business environment and your answer here shows how you handle difficult situations at work. It's also an excellent way for interviewers to judge your decision-making skills. Your answer can include any formative experience from your prior employment opportunities or your college days.
During my internship, I was leading a small team that consisted of interns handling a software development project. During the course of the project, the Project Manager asked me to eliminate people whose computing skills were not up-to-mark or who were unproductive and not a right fit for the project. That was a tough decision to make.
We hope the questions discussed above will help to make your interview experience a smooth one. All the best! Keep practicing and #BeUnstoppable.
More reads:
I am a biotechnologist-turned-content writer and try to add an element of science in my writings wherever possible. Apart from writing, I like to cook, read and travel.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Blogs you need to hog!
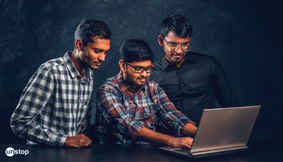
This Is My First Hackathon, How Should I Prepare? (Tips & Hackathon Questions Inside)
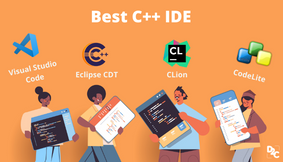
10 Best C++ IDEs That Developers Mention The Most!
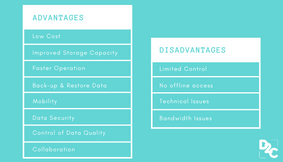
Advantages and Disadvantages of Cloud Computing that you should know!
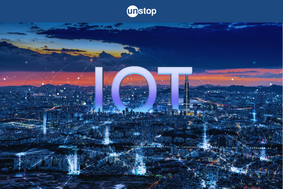
Comments
Add comment