Table of content:
Python List Functions: A Guide To Essential Operations (+Examples)
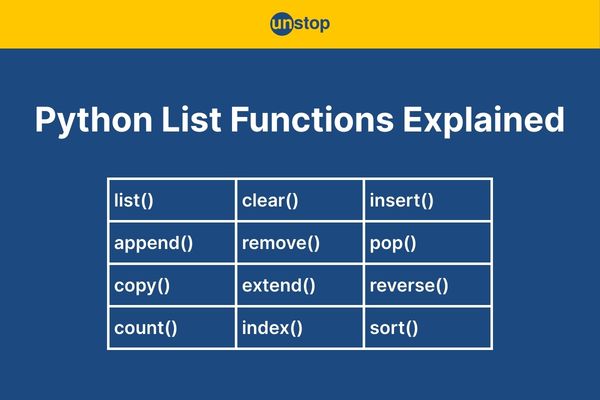
Python lists are like the Swiss Army knives of programming–versatile, flexible, and packed with built-in functions that make data handling a breeze. Whether you're adding, removing, or sorting elements, Python provides an array of list functions to simplify your workflow.
But with so many functions available, where do you start? In this guide, we break down the most commonly used Python list functions, explaining what they do and how to use them effectively.
What Is A Python List?
A list in Python is an ordered, mutable collection that can store multiple data types in a single variable. Unlike arrays in some other languages, Python lists can hold integers, strings, floats, and even other lists.
Syntax of a List in Python:
my_list = [1, "hello", 3.14, [5, 6, 7]]
Lists support indexing, slicing, and various operations that allow efficient data manipulation.
To learn more about Python lists, including their properties and usage, check out our in-depth guide: Python List | Everything You Need To Know (With Detailed Examples).
What Are Python List Functions? (Table)
Python provides several built-in functions to manipulate lists efficiently. These functions help you add, remove, find, and modify elements without writing extra code.
The table below summarizes the most commonly used Python list functions:
Function |
Syntax |
Description |
list() |
list(iterable) |
Creates a new list from an iterable (string, tuple, etc.). |
append() |
list.append(element) |
Adds an element to the end of the list. |
copy() |
list.copy() |
Returns a shallow copy of the list. |
count() |
list.count(value) |
Returns the number of occurrences of a value in the list. |
clear() |
list.clear() |
Removes all elements from the list. |
remove() |
list.remove(value) |
Removes the first occurrence of a value in the list. |
extend() |
list.extend(iterable) |
Extends the list by adding elements from another iterable. |
index() |
list.index(value) |
Returns the index of the first occurrence of a value. |
insert() |
list.insert(index, value) |
Inserts an element at a specified position. |
pop() |
list.pop(index) |
Removes and returns an element at a given index (default: last element). |
reverse() |
list.reverse() |
Reverses the order of elements in the list. |
sort() |
list.sort() |
Sorts the list in ascending order by default. |
We will explore these commonly used Python list functions in greater detail, with examples, in the following sections.
Quick Knowledge Check!
QUIZZ SNIPPET IS HERE
Check out this amazing course to become the best version of Python programmer you can be.
The list() Function In Python
The list() function is used to create a new list from an iterable, such as a string, tuple, or set. If no argument is provided, it returns an empty list.
Syntax:
list(iterable)
Here, the iterable (optional) is any iterable object (string, tuple, dictionary, etc.) that we want to convert to a list. The function creates an empty list when called without an argument.
Code Example:
Code Explanation:
In the simple Python program example:
- We first call list() without an argument, which creates an empty list.
- Then, the call list("Unstop") converts a string variable (“Unstop”) into a list of characters.
- Next, we call the function to convert a tuple to a list, i.e., list((1, 2, 3)).
- After that, we call the list() function to convert a set into a list, i.e., list({4, 5, 6}). Note that the order of elements in the list returned may vary since sets are unordered.
- Finally, we use the print() function in Python to display all the outcomes to the console.
Since list() is often used to convert strings to lists, you can check out our guide– String to List Conversion in Python for deeper insights.
The append() Python List Function
The append() function is used to add an element to the end of a list. Unlike extend(), which merges another iterable, append() treats the input as a single element.
Syntax:
list.append(element)
Here, the element refers to the item to be added to the list given by the name list before the dot operator.
Code Example:
For a detailed guide, including use cases and performance insights, check out our full article: Python List append() | Syntax & Working Explained (+Code Examples).
The copy() Python List Function
The copy() function creates a shallow copy of a list, meaning it duplicates the original list but does not copy nested objects deeply.
Syntax:
list.copy()
The function is called on the list using the dot operator, and it returns a new list with the same elements as the original.
Code Example:
For a deeper dive into list copy methods and shallow vs. deep copying, check out our full guide: Python List Copy Methods | Shallow & Deep Copies (+Code Examples).
The count() Python List Function
The count() function in Python helps you determine how many times a specific element appears in a list. This is particularly useful when analyzing data, tracking occurrences, or validating inputs. Instead of writing a loop to count occurrences manually, count() provides a simple and efficient way to get the result.
Syntax:
list.count(value)
Here, the value parameter is the element whose occurrences you want to count.
Code Example:
In this example, "apple" appears twice in the list, so count("apple") returns 2.
To learn more about how count() works and its applications, check out our detailed guide: Python count() Function | Explained In Detail With Code Examples.
The clear() And remove() Python List Functions
Managing elements in a list often involves either removing a specific item or wiping the list clean. Python provides two distinct functions for this:
- clear(): Removes all elements, leaving an empty list.
- remove(): Deletes the first occurrence of a specified value.
Both functions modify the list in place, but they serve different purposes–clear() erases everything, while remove() targets a single element.
Syntax:
list.clear() # Removes all elements from the list
list.remove(value) # Removes the first occurrence of 'value'
Code Example:
Notice that remove("apple") deletes only the first occurrence, not all instances.
For detailed information on these functions and other ways to remove list elements, read our complete guide: Remove Item From Python List | 5 Ways & Comparison (+Code Examples).
The extend() Python List Function
The extend() function allows you to merge another iterable (like a list, tuple, or set) into an existing list. Unlike append(), which treats the argument as a single element, extend() adds each item from the iterable individually. This function is especially useful when you need to combine lists without creating nested structures.
Syntax:
list.extend(iterable)
Here, the iterable parameter refers to any iterable (list, tuple, set, etc.) whose elements will be added to the list.
Code Example:
Here, extend() merges list2 into list1 without creating nested lists, unlike append().
For an in-depth breakdown of extend(), check out our detailed guide: Python extend() Function | Syntax, Techniques & More (+Examples).
The index() Python List Function
The index() function helps locate the position of the first occurrence of a specified element in a list. If the element isn’t found, Python raises a ValueError.
This function is useful when you need to determine where a specific value appears in a list, whether for searching, sorting, or modifying elements dynamically.
Syntax:
list.index(value, start, end)
Here, the parameters:
- value– The element to search for.
- start (optional)– The index to begin the search (default: 0).
- end (optional)– The index to stop the search (default: end of the list).
Code Example:
Here, the first "apple" is found at index 0, but when searching from index 1, the next occurrence is at index 3.
For more advanced examples and error handling tips, check out our full guide: Python List index() Method | Use Cases Explained (+Code Examples).
The insert() Python List Function
The insert() function allows you to add an element at a specific position in a list. Unlike append(), which only adds to the end, insert() lets you place an element exactly where you need it. This function is particularly useful when order matters, such as inserting new data in a sorted list or maintaining a sequence.
Syntax:
list.insert(index, element)
Here, the parameters:
- index– The position where the new element should be inserted.
- element– The value to insert into the list.
Code Example:
Here, element 3 is inserted at index 2, shifting the remaining elements to the right.
For more examples and edge cases, check out our full guide: Python List insert() Method Explained With Detailed Code Examples.
The pop() Python List Function
The pop() function removes and returns an element from a list. By default, it removes the last element, but you can specify an index to remove an element from a particular position.
This function is useful when you need to extract and use an element while modifying the original list.
Syntax:
list.pop(index)
Here, the parameter index (optional) refers to the position of the element to remove. Defaults to -1 (last element).
Code Example:
For more examples and best practices, check out our detailed guide: Python pop() Function | Syntax, Uses & Alternative With Examples.
The reverse() Python List Function
The reverse() function in Python reverses the order of elements in a list in place, meaning it modifies the original list instead of creating a new one. This is useful when you need to flip a list for easier processing, such as reversing sorting orders or working with stack-like operations.
Syntax:
list.reverse()
Code Example:
To know about this Python list function and other ways of reversal, read: Python Reverse List | 10 Ways & Complexity Analysis (+Examples)
Quick Knowledge Check!
QUIZZ SNIPPET IS HERE
The sort() Python List Function
The sort() function in Python arranges the elements of a list in ascending order by default. It modifies the list in place, meaning it doesn’t return a new list but directly sorts the existing one. You can also customize the sorting order using parameters like reverse and key.
Syntax:
list.sort(key=None, reverse=False)
Here,
- key (optional)– A function that defines the sorting criteria (e.g., len for sorting by length).
- reverse (optional)– If True, sorts in descending order. Default is False (ascending).
Code Example:
For advanced sorting techniques and custom key functions, check out our detailed guide: Python List sort() | All Use Cases Explained (+Code Examples).
Quick Knowledge Check!
QUIZZ SNIPPET IS HERE
Looking for guidance? Find the perfect mentor from select experienced coding & software development experts here.
The len() Python List Function
The len() function returns the number of elements in a list. Unlike functions like append() or pop(), which modify the list, len() is a non-modifying function that simply provides information about the list’s size.
This function is widely used for looping through lists, validating inputs, and performing conditional checks based on the list’s length.
Syntax:
len(list)
The function returns an integer representing the number of elements in the list.
Code Example:
The len() list function in Python is highly optimized, making it the fastest way to get a list’s length.
For more ways to calculate list length and handle edge cases, check out our detailed guide: Find Length Of List In Python | 8 Ways (+Examples) & Analysis.
Conclusion
Python provides a variety of list functions that make it easy to manipulate, search, and modify lists efficiently. Whether you need to add elements (append(), insert(), extend()), remove them (pop(), remove(), clear()), find their positions (index(), count()), or reorder them (sort(), reverse()), these functions offer simple and effective solutions.
By understanding how the Python list functions work and when to use them, you can write cleaner and more efficient Python code. If you’re looking for hands-on practice, check out the coding challenges and exercises on Unstop!
Frequently Asked Questions
Q1. What is the difference between append() and extend() in Python?
- append() adds a single element to the end of a list, treating it as a whole.
- extend() takes an iterable (like another list) and adds its elements individually to the existing list.
Code Example:
a = [1, 2, 3]
a.append([4, 5])
print(a) # Output: [1, 2, 3, [4, 5]]b = [1, 2, 3]
b.extend([4, 5])
print(b) # Output: [1, 2, 3, 4, 5]
2. How can I remove duplicates from a Python list?
The easiest way is to convert the list into a set, which removes duplicates automatically:
numbers = [1, 2, 2, 3, 4, 4, 5]
unique_numbers = list(set(numbers))
print(unique_numbers) # Output: [1, 2, 3, 4, 5]
However, this method does not preserve order. If order matters, use a loop or dict.fromkeys().
3. How to find the maximum and minimum elements in a Python list?
You can use the built-in Python functions max() and min() as shown in the snippet example below:
numbers = [10, 5, 8, 20]
print(max(numbers)) # Output: 20
print(min(numbers)) # Output: 5
For more details, check out our guides:
4. What is list comprehension in Python?
List comprehension is a concise way to create lists using a single line of code.
Code Example:
squares = [x**2 for x in range(5)]
print(squares) # Output: [0, 1, 4, 9, 16]
It replaces loops and map() functions for simple list transformations. Learn more here: List Comprehension in Python.
5. How can I sort a list of tuples based on a specific element?
Use the key parameter in sort(), specifying which tuple index to sort by.
Example (Sorting by second element):
students = [("Alice", 85), ("Bob", 92), ("Charlie", 78)]
students.sort(key=lambda x: x[1])
print(students)
# Output: [('Charlie', 78), ('Alice', 85), ('Bob', 92)]
6. Can I reverse a list without modifying the original list?
Yes! Instead of using reverse(), which modifies the list in place, use slicing, which keeps the original list unchanged. Below is an example that illustrates the same:
original = [1, 2, 3, 4]
reversed_list = original[::-1]
print(reversed_list) # Output: [4, 3, 2, 1]
This compiles our discussion on the Python list functions. Here are a few more topics you must explore:
- How To Convert Python List To String? 8 Ways Explained (+Examples)
- Difference Between List And Tuple In Python Explained (+Examples)
- Python Linked Lists | A Comprehensive Guide (With Code Examples)
- Fix Indexerror (List Index Out Of Range) In Python +Code Examples
- 40+ Python String Methods Explained | List, Syntax & Code Examples