Game 21 Challenge - Winning Strategy Explained!
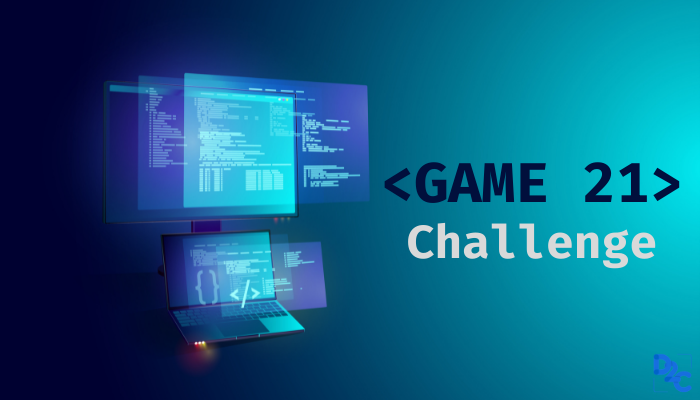
Apparently, competitive programming is a fad, and why wouldn't it be? Almost all IT companies select suitable candidates for software engineering positions by conducting placement rounds based on competitive programming only. It develops your analytical and logical abilities and is a good exercise for your brain cells.
Such programming contests typically include problems based on mathematical and logical reasoning and require one to write the source code to solve those problems. Categories covered are usually algorithmic game theory, data structures, computational geometry, and strings, to mention a few.
Whatever the problem might be, constructing an efficient algorithm by dry running your code several times to know the loopholes and then implementing it in a preferred programming language is obviously the most significant and foremost step.
What is Game Number 21?
Alternatively known as Game 21, Twenty plus One, and Bagram, it’s a game generally played between two players (though you can include more) which advances by checking up 1 to 21, with the player who calls "21" is disposed of.
Consider two players A and B. They are given a bucket list of numbers 1,2 and 3 to play the game. Whatever number the first player, say A chooses from the given list, player B’s choice of number gets added to it. In the next round, A’s chosen number gets added to the previous addendum with B. The game proceeds in the same manner until the addendum with any player becomes 21, and that player is said to have lost the game.
What are the Rules and Constraints to be kept in mind?
- A player can decide whether he wishes to take the initial plunge. Before proceeding with game 21, the list of numbers a player can choose from is displayed on the screen.
- On the off chance that consecutive numbers are not given in input, that player loses and can't proceed with the game.
- Neither of the players can exceed or pass the total count of 21. For example, if the addendum with a player at a particular stage is 19, he cannot choose 3 as it will make the total count reach 22, which isn’t allowed. Hence, that player can choose either 1 or 2 to continue playing the game.
- The game ends whenever a player calls 21, making the other player win henceforth.
Confused? Let’s look at a detailed approach.
Suppose player A decides to go first and chooses number 2 from the list [1,2,3]. After this, B decides to go with the number 1, so the final number with B becomes (2+1) =3.
Now, in the next round, say A chooses number 2, so the addendum with A becomes the previous addendum with B plus A’s choice, i.e. (3+2) = 5. Then, suppose B goes with the number 3, so the total count with B now adds up to (5+3) = 8, and so on.
The below-mentioned table shows the entire scenario till the end:
Player A (Makes the first turn) |
Player B |
2 |
(2 + B’s Choice, say 1) = 2+1 = 3 |
(3+ A’s Choice, say 2) = 3+2 = 5 |
(5 + B’s Choice, say 3) = 5+3 = 8 |
(8 + A’s Choice, say 1) = 8+1 = 9 |
(9 + B’s Choice, say 3) = 9+3 = 12 |
(12 + A’s Choice, say 2) = 12+2 = 14 |
(14 + B’s Choice, say 2) = 14+2 = 16 |
(16 + A’s choice, say 3) = 16 + 3 = 19 |
Now at this stage, B can only choose from the list [1,2] as adding 3 to 19 exceeds the total count of 21. ( 19 + B’s Choice, say 2) = 19 + 2 = 20 |
Since adding any other number to 20 other than 1 will make it greater than 21, Hence player A has got no choice but to go with 1 and become 21. Therefore A LOSES the game |
Player B emerges as the WINNER! |
How was this amazing strategy devised?
Reverse Engineering is the answer to a majority of winning stage strategies devised for algorithmic games similar to Game 21 in the arena of competitive programming.
To put it simply, we start from the end step, that is to win the game player B's ultimate strategy should be to get to a total of 20 (as the total count cannot exceed 21, player A would lose the game by choosing the only option number 1).
To reach 21, player B should have an addendum of 16 at the just previous round because if it chooses any other number, player A can add a suitable number and become 20, and thus A will get onto the winning stage and win the game.
If B chooses 16, no matter what number player A chooses, B can make it 20. How?
- 16 + A’s choice (say, 1) = 17, then, 17 + B’s choice, i.e., 3 = 20
- 16 + A’s choice (say, 2) = 18, then, 18 + B’s choice, i.e., 2 = 20
- 16 + A’s choice (say, 3) = 19, then, 19 + B’s choice, i.e., 1 = 20
In a similar fashion, the total number with player B just before 16 should be 12, and just before 12 should be 8, and in the first round, should be 4.
In this way, maintaining multiples of four at every stage will continue to keep B on the winning stage thus making it win the game at the end.
Player A (Makes the first turn) |
Player B |
1 / 2 / 3 |
4 |
5 / 6 / 7 |
8 |
9 / 10 / 11 |
12 |
13 / 14 / 15 |
16 |
17 / 18 / 19 |
20 |
21 (LOSES THE GAME) |
WINNER! WINNER! |
- From the above table, we can clearly infer that if you are on a winning stage, then your opponent can only be on a non-winning stage.
- Also, regardless of the non-winning state condition your opponent creates, you can always reach a winning state. Isn't Game number 21 really mind-blowing!
The following rough draft of the code to play Game 21 (written in Python Programming Language) shows the function you can write to check for multiples of four.
# Python code to play twenty plus one game
# Returns 4’s nearest multiples
def foursMultiple(n):
if n >= 4:
nearest_multiple = n + (4 - (n % 4))
else:
nearest_multiple = 4
return nearest_multiple
The best part about the approach on which twenty-plus one game is based is that you can include certain level-ups and other variations like, including only odd or even numbers, playing with binary numbers, or increasing the bucket list and the sum total of the game.
Try it out and experiment for yourself by dry-running the different cases!
For more amazing concepts and latest stories, check out the following:
- Aligarh-based boy bags 100% scholarship at Stanford University
- IIT Delhi Industry Day Coverage: Unveiling glass properties with Python for Glass Genomics (PyGGi)
- Failing Coding Interviews? Get inside tips on how to crack interviews in top companies
- How do competitions provide real-time solutions? Know from Aritro Datta, D2C Competitive Leaders 2021
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment