- What Is A Python Module?
- How To Effectively Use Python Modules (Ways To Import)?
- Built-In Python Modules List
- Example Of Python Modules Usage
- Other Python Modules List
- Exploring Python Modules With The dir() Function
- User-Defined Python Modules
- Best Practices For Using Python Modules
- Conclusion
- Frequently Asked Questions
Python Modules | Definition, Usage, Lists & More (+Code Examples)
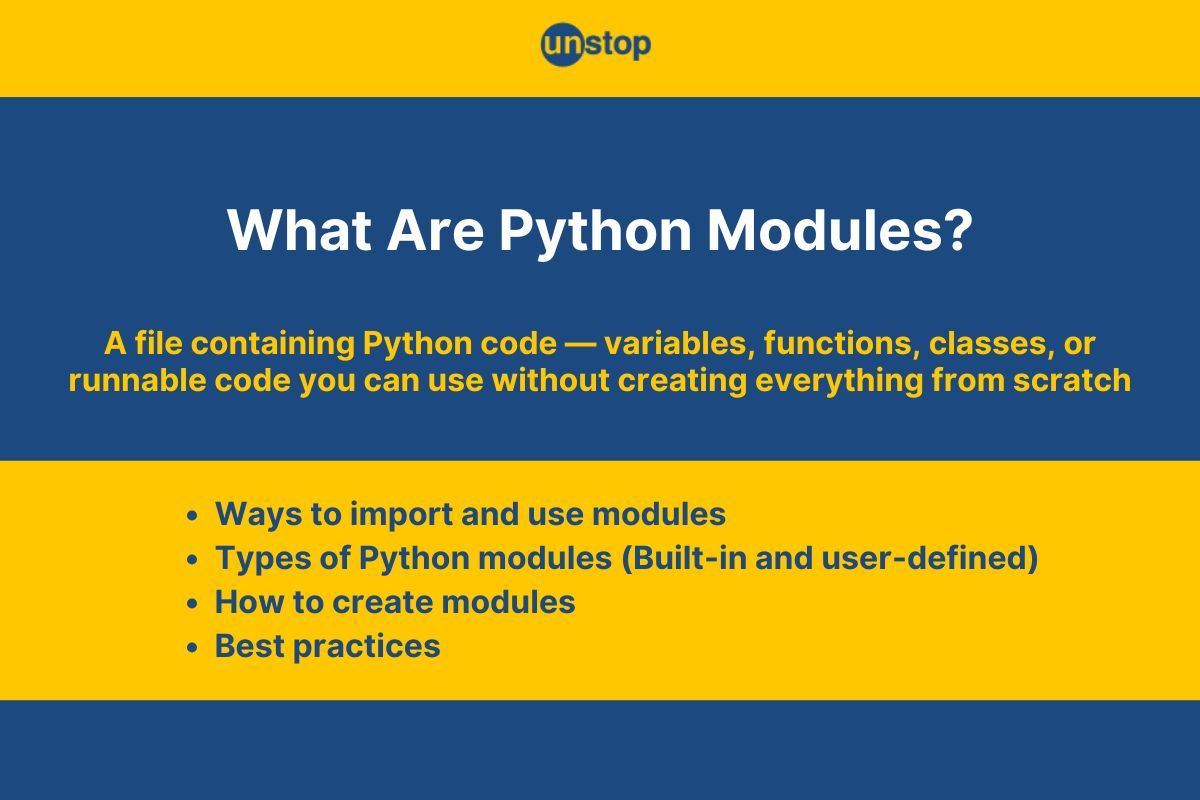
Python, the Swiss Army knife of programming languages, owes much of its versatility to modules. These building blocks allow developers to write clean, organized, and reusable code while tapping into Python's vast ecosystem of built-in functionalities.
In this article, we will discuss everything you need to know about Python modules, from the essentials to their effective use. We have also provided curated Python module lists of built-in and lesser-known modules categoriesed as per functionality.
What Is A Python Module?
At its core, a module is simply a file containing Python code — variables, functions, classes, or runnable code. Think of a module as a toolkit: it contains tools (code) you can use without creating everything from scratch.
How Are Python Modules Different From Libraries?
While the terms "module" and "library" are often used interchangeably, they are technically different:
- Module: A single Python file (e.g., math.py) that can be imported and used in your scripts.
- Python Library: A collection of multiple modules bundled together, like a toolbox with several compartments (e.g., NumPy, which has multiple modules for array handling, mathematical operations, and more).
Why Use Python Modules?
Modules promote reusability and organization, allowing developers to break a large program into smaller, manageable files. Here's why Python modules are indispensable:
- Code Reusability: Modules let you reuse functionality across multiple programs.
- Separation of Concerns: By splitting functionality into separate files, modules make debugging and maintenance easier.
- Built-In Power: Python's standard library is a treasure trove of built-in modules, saving you the effort of coding common functionalities from scratch.
Whether you're importing a module to handle date operations (datetime) or creating your own to structure a project, modules are fundamental to writing efficient Python code.
How To Effectively Use Python Modules (Ways To Import)?
Using Python modules effectively can greatly simplify your code and boost productivity. Here’s a guide to ensure you're making the most out of them:
Importing Python Modules
The simplest way to use a module is by using the import statement to make the respective module and its components accessible in your Python code.
Code Example:
#Importing the math module
import math
#Using sqrt() from math
print(math.sqrt(16)) # Output: 4.0
When you use import, you gain access to all the functionalities of the module. For example, here, we want to use the srt() Python function from the math module. But note that you need to reference them using the module name as a prefix (e.g., math.sqrt).
Importing Specific Functions/Variables From Python Modules
If you only need certain parts of a module, you can use the from...import statement in Python programming. This makes your code cleaner and avoids loading unnecessary elements.
Code Example:
#Importing specific components from the math module
from math import sqrt, pi
#Using sqrt() function and pi variable
print(sqrt(25)) # Output: 5.0
print(pi) # Output: 3.141592653589793
In the example, we import only the sqrt() function and the Python variable pi, from the math module. This approach is ideal for optimizing performance and improving readability, especially when working with large modules.
Importing All Names From Python Modules
There is another way to import everything from a schedule, and that is by using the from…import* statement. It also helps you gain access to all the components of the module in your code.
Code Example:
from math import *
print(factorial(5)) # Output: 120
While this is convenient, it’s generally discouraged as it can lead to namespace collisions — where functions or variables from different modules have the same name.
Using Aliases For Python Modules
There are quite a few Python modules that have long name,s and when you have to reference these modules in function calls, it might become a bit tedious. This is why, for longer module names, you can use as to create an alias. Or you can just customize the names if you want.
Code Example:
#Importing matplotlib⦠with an alias name plt
import matplotlib.pyplot as plt
#Using the plit() method from the module, using its alias
plt.plot([1, 2, 3], [4, 5, 6])
plt.show()
This way of importing and using Python modules improves code readability and reduces typing effort, especially for frequently used modules.
Understanding Python Module Search Paths
When you import a module, Python looks for it in specific locations, which are defined in the sys.path list. This includes:
- The current directory.
- Standard library directories.
- Directories listed in the PYTHONPATH environment variable.
To check the search paths in your environment:
import sys
print(sys.path)
Selecting The Right Python Module For Your Needs
When choosing a module, consider the following:
- Purpose: What functionality do you need? For example, use os for file operations and random for generating random values.
- Documentation: Always check the official documentation to ensure the module suits your requirements.
- Community Support: For third-party modules, look for active development and support.
By mastering these techniques and strategies, you can efficiently incorporate modules into your projects and write cleaner, more modular code.
Check out this amazing course to become the best version of the Python programmer you can be.
Built-In Python Modules List
Python’s Standard Library is a goldmine of built-in modules that provide solutions for everyday programming tasks. Below is a curated list of some of the most commonly used modules (along with a description and examples of built-in functions), categorized by their functionality.
1. Operating System & System Utilities Python Modules List
Name |
Purpose |
Example Functions |
os |
Interact with the operating system. |
os.getcwd(), os.rename() |
sys |
Access system-specific parameters. |
sys.exit(), sys.argv |
shutil |
Perform high-level file operations. |
shutil.copy(), shutil.rmtree() |
platform |
Retrieve system information. |
platform.system(), platform.release() |
2. Data Handling/ Serialization/ Parsing Python Modules List
Name |
Purpose |
Example Functions |
json |
Parse and create JSON data. |
json.dumps(), json.loads() |
csv |
Work with CSV files. |
csv.reader(), csv.writer() |
sqlite3 |
Interface with SQLite databases. |
sqlite3.connect(), sqlite3.execute() |
pickle |
Serialize and deserialize Python objects. |
pickle.dump(), pickle.load() |
3. Mathematics Operations & Numbers Python Modules List
Name |
Purpose |
Example Functions |
math |
Perform mathematical operations. |
math.sqrt(), math.pi |
random |
Generate random numbers. |
random.randint(), random.choice() |
decimal |
Perform high-precision calculations. |
decimal.Decimal(), decimal.getcontext() |
statistics |
Perform statistical calculations. |
statistics.mean(), statistics.median() |
cmath |
Handle complex numbers. |
cmath.phase(), cmath.sqrt() |
4. String Manipulation/ Text Processing Python Modules List
Name |
Purpose |
Example Functions |
re |
Perform regular expression matching. |
re.match(), re.findall() |
string |
Access string constants and utilities. |
string.ascii_letters, string.digits |
5. Date & Time Handling Python Modules List
Name |
Purpose |
Example Functions |
datetime |
Work with dates and times. |
datetime.datetime.now(), datetime.timedelta |
time |
Access time-related functions. |
time.sleep(), time.time() |
6. Advanced Data Structures Python Modules List
Name |
Purpose |
Example Functions |
collections |
Work with advanced data structures. |
collections.Counter(), collections.defaultdict() |
itertools |
Create efficient iterators. |
itertools.chain(), itertools.combinations() |
Note: The modules listed above represent only a fraction of Python’s built-in capabilities. Explore the full Standard Library on the official website to discover more modules tailored to your needs.
Example Of Python Modules Usage
In the simple Python program example below, we have illustrated how to use the os Python module and its components to fetch the current working directory
Code Example:
#Importing the module
import os
# Get the current working directory
cwd = os.getcwd()
print(f"Current Working Directory: {cwd}")
Output:
Current Working Directory: /Users/username/projects
Code Explanation:
In the simple Python code example:
- We import the os module to access system-level operations.
- We then use os.getcwd() which fetches the directory path from where the script is executed.
This section introduces some of the most powerful tools in Python’s Standard Library, making it easier to tackle diverse programming challenges without relying on external libraries.
Looking for guidance? Find the perfect mentor from select experienced coding & software development experts here.
Other Python Modules List
Beyond Python's built-in modules, there’s a whole universe of third-party modules that extend the language’s functionality. These modules aren't bundled with Python but can be easily installed using package managers like pip. Here’s a list of some lesser-known but highly useful modules you might want to consider:
Name |
Purpose |
Example Functions |
requests |
Simplifies HTTP requests. |
requests.get(), requests.post() |
beautifulsoup4 |
Web scraping and parsing HTML/XML. |
BeautifulSoup(), find_all() |
pandas |
Data manipulation and analysis. |
pandas.DataFrame(), pandas.read_csv() |
numpy |
Efficient numerical computations. |
numpy.array(), numpy.dot() |
matplotlib |
Data visualization. |
matplotlib.pyplot.plot(), matplotlib.pyplot.show() |
flask |
Web framework for building applications. |
flask.Flask(), flask.render_template() |
sqlalchemy |
Database toolkit and ORM. |
sqlalchemy.create_engine(), sqlalchemy.sessionmaker() |
pytest |
Testing framework. |
pytest.mark.parametrize(), pytest.fixture() |
Below is a Python program example that illustrates how to use the requests Python module to fetch data from an API.
Code Example:
#Importing the Python module
import requests
# Make a GET request to a URL
response = requests.get("https://jsonplaceholder.typicode.com/posts/1")
# Print the response content
print(response.json())
Output:
{
"userId": 1,
"id": 1,
"title": "sunt aut facere repellat provident occaecati excepturi optio reprehenderit",
"body": "quia et suscipit\nsuscipit..."
}
Code Explanation:
In the Python code example:
- The requests module is used to send an HTTP GET request to a URL.
- The function call response.json() parses the JSON response into a Python dictionary.
Installing Third-Party Python Modules
To install any of these modules, you can use the pip package manager. For example, to install requests, you would run the following command in your terminal:
pip install requests
Exploring Python Modules With The dir() Function
The dir() method is a built-in Python function that provides a convenient way to inspect the attributes and methods of a module or object. It’s especially useful when you’re exploring an unfamiliar module and want to see what functionality it offers.
How the dir() Function Works
The syntax for the dir() function is straightforward:
dir([object])
Here,
- If no argument is passed, dir() returns the names in the current local scope.
- If an object (like a module) is passed as an argument, dir() returns a list containing all the attributes and methods available in that object.
Using dir() With Python Modules
The example Python program below illustrates how you can use dir() to explore the math module.
Code Example:
#Importing a Python module
import math
# List all attributes and methods in the math module
print(dir(math))
Output:
['__doc__', '__loader__', '__name__', '__package__', '__spec__', 'acos', 'acosh', 'asin', 'asinh', ...]
This output includes all the methods and constants available in the math module, like acos, asin, and pi.
Filtering Attributes: Sometimes, you might want to explore specific features of a module. You can still use the dir() function to focus on the useful attributes or methods of Python modules and ignore the special ones (like __doc__ or __name__). For this, you must use list comprehension with a filter to get the needed output. The example Python code below illustrates how this can be done.
Code Example:
#Importing the Python module
import os
# Filter out special methods
attributes = [attr for attr in dir(os) if not attr.startswith('__')]
print(attributes)
Output:
['chdir', 'getcwd', 'listdir', 'mkdir', ...]
Code Explanation:
- We begin by importing the os Python module using the import statement.
- Then, we use the dir() function inside list comprehension with the if-conditional to filter out attributes that start with the character ‘___’.
- This helps filter out the special attributes, and the remaining are stored in the object attribute.
- We then use the print() function to display them to the console.
Why Use dir() With Python Modules?
- Exploration: Quickly see what a module offers without diving into documentation.
- Learning: Understand a module’s functionality while experimenting interactively.
- Debugging: Identify the available attributes or methods in a user-defined module.
User-Defined Python Modules
In addition to built-in and third-party modules, Python allows you to create your own modules. This means you can organize your code into separate files, making it more modular and easier to maintain. User-defined modules allow you to reuse code across different projects and improve code readability.
How To Create User-Defined Module In Python?
To create a module, simply write a Python file with functions, variables, and classes that you want to reuse. The file should have a .py extension. For example, let's create a module named math_utils.py.
To create a Python module of your own, you simply need to create a Python file (with a .py extension) that contains your functions, classes, or variables. This file can be created in the same directory as your main Python script or in a separate directory, which can be treated as a package (if needed).
Where To Place The Python Module
Same Directory: If you place the module in the same directory as your main script (e.g., main.py), you can simply import it using the module's filename (without the .py extension). File structure example:
project/
├── main.py # Main script where you will use the module
├── math_utils.py # User-defined module with functions (e.g., add, subtract)
Different Directory (Package): If you have a more complex project, you might want to create a directory to store multiple modules. If the modules are in a subdirectory, you should add an __init__.py file to turn it into a Python package. Example structure for using a package:
project/
├── main.py
└── utils/
├── __init__.py # Marks this directory as a package
└── math_utils.py
Now, in main.py, you can import your math_utils.py from the utils directory using the import statement, like: from utils.math_utils import add, subtract.
Here, math_utils.py is the user-defined module, and it contains the code for mathematical operations. You can create this module anywhere within your project directory as long as you ensure the module is accessible from your main script.
Example (math_utils.py): Defining The Module
# math_utils.py
def add(a, b):
return a + b
def subtract(a, b):
return a - b
In this example, math_utils.py contains two functions: add() and subtract(). You can place this file alongside your main script or in a subfolder if you want to organize your project better.
How To Use A User-Defined Python Module
To use a user-defined module, use the import statement. Assuming math_utils.py is in the same directory as your main Python script, you can import and use it as follows:
Example (main.py):
import math_utils
result_add = math_utils.add(5, 3)
result_sub = math_utils.subtract(10, 4)
print(f"Addition Result: {result_add}")
print(f"Subtraction Result: {result_sub}")
Output:
Addition Result: 8
Subtraction Result: 6
Renaming A User-Defined Module
You can also rename a module during import using the as keyword. This can be useful for shorter or more convenient names. For example, you can import math_utils.py as mu:
import math_utils as mu
result_add = mu.add(5, 3)
result_sub = mu.subtract(10, 4)
print(f"Addition Result: {result_add}")
print(f"Subtraction Result: {result_sub}")
Best Practices For Using Python Modules
To maximize the efficiency and maintainability of your Python code, following best practices for working with modules is essential. Here are some crucial guidelines:
- Import Only What You Need: Instead of importing the entire module, import only the specific components (functions, classes, etc.) you need. This makes your code more efficient and easier to read.
- Use Aliases for Clarity and Brevity: If the module name is long or commonly used, consider using an alias. This reduces code verbosity and can improve readability.
- Organize Modules Into Packages: For larger projects, organize your modules into subdirectories or packages. This helps maintain a clean structure, especially when dealing with multiple modules that serve different purposes.
- Avoid Circular Imports: Circular imports occur when two modules depend on each other. These can cause errors and should be avoided. Consider restructuring the module dependencies to break circular references.
- Use Virtual Environments: Manage project-specific dependencies with virtual environments. This ensures that each project has its own set of dependencies, which avoids conflicts with other Python projects or system-wide installations.
- Document Your Code: Use docstrings to document your functions, classes, and modules. This makes your code more understandable to others (or your future self) and is especially useful in collaborative environments.
- Prefer Built-in Modules First: Before installing third-party modules, check if the functionality is already available in Python’s Standard Library. Using built-in modules can simplify your project by avoiding unnecessary external dependencies.
- Maintain Version Control for Dependencies: Use requirements.txt (or similar tools) to track the versions of external libraries. This ensures that all contributors to the project use the same versions of dependencies, preventing compatibility issues.
Conclusion
Python modules are essential for organizing and reusing code in a scalable way. By understanding how to create, use, and import modules effectively, you can greatly improve the readability and maintainability of your Python projects. Whether you're utilizing built-in modules, incorporating third-party libraries, or crafting your own custom modules, following best practices will ensure your code remains clean, efficient, and easy to manage.
Remember, modular programming not only helps in organizing your code but also boosts collaboration, testing, and long-term maintenance. As you continue to explore Python’s rich ecosystem of modules, you'll find that knowing how and when to leverage them is key to building high-quality applications.
Ready to upskill your Python game? Dive deeper into actual problem statements and elevate your coding game with hands-on practice!
Frequently Asked Questions
Q1. What is the difference between a Python module and a Python package?
A module is a single file containing Python code, such as functions, classes, and variables. A package, on the other hand, is a collection of related modules bundled together in a directory. A package can contain subdirectories and an __init__.py file, which signifies that the directory is a Python package.
Q2. How do I know which modules to use for my project?
The module you choose should depend on the task you need to accomplish. For common tasks like file handling, the os or shutil modules may suffice. For data manipulation, pandas might be the go-to choice. Before using third-party libraries, check if the functionality you need is available in Python's Standard Library.
Q3. Can I import a user-defined module from another directory?
Yes, you can import user-defined modules from other directories by structuring your project as a package. Ensure that the directory containing the module has an __init__.py file, and then you can import the module using the appropriate path.
Q4. How can I make my custom modules reusable across different projects?
You can make your custom modules reusable by organizing them into packages and keeping them well-documented. For broader reuse, you can even upload them to Python's package index (PyPI), where they can be installed and shared across projects.
Q5. What is the best way to handle dependencies when using third-party modules?
Using a virtual environment to manage dependencies is the best practice. This ensures that the libraries and their versions are isolated to your project, preventing conflicts with other Python projects or system-wide packages. You can also track dependencies using a requirements.txt file.
By now, you must be familiar with the contents of our Python modules list and know how to use them to enhance your projects. You might also like:
- 40+ Python String Methods Explained | List, Syntax & Code Examples
- Python Namespace & Variable Scope Explained (With Code Examples)
- Python input() Function (+Input Casting & Handling With Examples)
- Python Program To Convert Kilometers To Miles (+Detailed Examples)
- Python Program To Solve Quadratic Equations (With Code Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment