Table of content:
- What Is Python? An Introduction
- What Is The History Of Python?
- Key Features Of The Python Programming Language
- Who Uses Python?
- Basic Characteristics Of Python Programming Syntax
- Why Should You Learn Python?
- Applications Of Python Language
- Advantages And Disadvantages Of Python
- Some Useful Python Tips & Tricks For Efficient Programming
- Python 2 Vs. Python 3: Which Should You Learn?
- Python Libraries
- Conclusion
- Frequently Asked Questions
- It's Python Basics Quiz Time!
Table of content:
- Python At A Glance
- Key Features of Python Programming
- Applications of Python
- Bonus: Interesting features of different programming languages
- Summing up...
- FAQs regarding Python
- Take A Quiz To Rehash Python's Features!
Table of content:
- What Is Python IDLE?
- What Is Python Shell & Its Uses?
- Primary Features Of Python IDLE
- How To Use Python IDLE Shell? Setting Up Your Python Environment
- How To Work With Files In Python IDLE?
- How To Execute A File In Python IDLE?
- Improving Workflow In Python IDLE Software
- Debugging In Python IDLE
- Customizing Python IDLE
- Code Examples
- Conclusion
- Frequently Asked Questions (FAQs)
- How Well Do You Know IDLE? Take A Quiz!
Table of content:
- What Is A Variable In Python?
- Creating And Declaring Python Variables
- Rules For Naming Python Variables
- How To Print Python Variables?
- How To Delete A Python Variable?
- Various Methods Of Variables Assignment In Python
- Python Variable Types
- Python Variable Scope
- Concatenating Python Variables
- Object Identity & Object References Of Python Variables
- Reserved Words/ Keywords & Python Variable Names
- Conclusion
- Frequently Asked Questions
- Rehash Python Variables Basics With A Quiz!
Table of content:
- What Is A String In Python?
- Creating String In Python
- How To Create Multiline Python Strings?
- Reassigning Python Strings
- Accessing Characters Of Python Strings
- How To Update Or Delete A Python String?
- Reversing A Python String
- Formatting Python Strings
- Concatenation & Comparison Of Python Strings
- Python String Operators
- Python String Functions
- Escape Sequences In Python Strings
- Conclusion
- Frequently Asked Questions
- Rehash Python Strings Basics With A Quiz!
Table of content:
- What Is Python Namespace?
- Lifetime Of Python Namespace
- Types Of Python Namespace
- The Built-In Namespace In Python
- The Global Namespace In Python
- The Local Namespace In Python
- The Enclosing Namespace In Python
- Variable Scope & Namespace In Python
- Python Namespace Dictionaries
- Changing Variables Out Of Their Scope & Python Namespace
- Best Practices Of Python Namespace
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Namespaces!
Table of content:
- What Are Logical Operators In Python?
- The AND Python Logical Operator
- The OR Python Logical Operator
- The NOT Python Logical Operator
- Short-Circuiting Evaluation Of Python Logical Operators
- Precedence of Logical Operators In Python
- How Does Python Calculate Truth Value?
- Final Note On How AND & OR Python Logical Operators Work
- Conclusion
- Frequently Asked Questions
- Python Logical Operators Quiz– Test Your Knowledge!
Table of content:
- What Are Bitwise Operators In Python?
- List Of Python Bitwise Operators
- AND Python Bitwise Operator
- OR Python Bitwise Operator
- NOT Python Bitwise Operator
- XOR Python Bitwise Operator
- Right Shift Python Bitwise Operator
- Left Shift Python Bitwise Operator
- Python Bitwise Operations And Negative Integers
- The Binary Number System
- Application of Python Bitwise Operators
- Python Bitwise Operator Overloading
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Bitwise Operators!
Table of content:
- What Is The Print() Function In Python?
- How Does The print() Function Work In Python?
- How To Print Single & Multi-line Strings In Python?
- How To Print Built-in Data Types In Python?
- Print() Function In Python For Values Stored In Variables
- Print() Function In Python With sep Parameter
- Print() Function In Python With end Parameter
- Print() Function In Python With flush Parameter
- Print() Function In Python With file Parameter
- How To Remove Newline From print() Function In Python?
- Use Cases Of The print() Function In Python
- Understanding Print Statement In Python 2 Vs. Python 3
- Conclusion
- Frequently Asked Questions
- Know The print() Function In Python? Take A Quiz!
Table of content:
- Working Of Normal Print() Function
- The New Line Character In Python
- How To Print Without Newline In Python | Using The End Parameter
- How To Print Without Newline In Python 2.x? | Using Comma Operator
- How To Print Without Newline In Python 3.x?
- How To Print Without Newline In Python With Module Sys
- The Star Pattern(*) | How To Print Without Newline & Space In Python
- How To Print A List Without Newline In Python?
- How To Remove New Lines In Python?
- Conclusion
- Frequently Asked Questions
- Think You Can Print Without a Newline in Python? Prove It!
Table of content:
- What Is A Python For Loop?
- How Does Python For Loop Work?
- When & Why To Use Python For Loops?
- Python For Loop Examples
- What Is Rrange() Function In Python?
- Nested For Loops In Python
- Python For Loop With Continue & Break Statements
- Python For Loop With Pass Statement
- Else Statement In Python For Loop
- Conclusion
- Frequently Asked Questions
- Think You Know Python's For Loop? Prove It!
Table of content:
- What Is Python While Loop?
- How Does The Python While Loop Work?
- How To Use Python While Loops For Iterations?
- Control Statements In Python While Loop With Examples
- Python While Loop With Python List
- Infinite Python While Loop in Python
- Python While Loop Multiple Conditions
- Nested Python While Loops
- Conclusion
- Frequently Asked Questions
- Mastered Python While Loop? Let’s Find Out!
Table of content:
- What Are Conditional If-Else Statements In Python?
- Types Of If-Else Statements In Python
- If Statement In Python
- If-Else Statement In Python
- Nested If-Else Statement In Python
- Elif Statement In Python
- Ladder If-Elif-Else Statement In Python
- Short Hand If-Statement In Python
- Short Hand If-Else Statement In Python
- Operators & If-Esle Statement In Python
- Other Statements With If-Else In Python
- Conclusion
- Frequently Asked Questions
- Quick If-Else Statement Quiz– Let’s Go!
Table of content:
- What Is Control Structure In Python?
- Types Of Control Structures In Python
- Sequential Control Structures In Python
- Decision-Making Control Structures In Python
- Repetition Control Structures In Python
- Benefits Of Using Control Structures In Python
- Conclusion
- Frequently Asked Questions
- Control Structures in Python – Are You the Master? Take A Quiz!
Table of content:
- What Are Python Libraries?
- How Do Python Libraries Work?
- Standard Python Libraries (With List)
- Important Python Libraries For Data Science
- Important Python Libraries For Machine & Deep Learning
- Other Important Python Libraries You Must Know
- Working With Third-Party Python Libraries
- Troubleshooting Common Issues For Python Libraries
- Python Libraries In Larger Projects
- Importance Of Python Libraries
- Conclusion
- Frequently Asked Questions
- Quick Quiz On Python Libraries – Let’s Go!
Table of content:
- What Are Python Functions?
- How To Create/ Define Functions In Python?
- How To Call A Python Function?
- Types Of Python Functions Based On Parameters & Return Statement
- Rules & Best Practices For Naming Python Functions
- Basic Types of Python Functions
- The Return Statement In Python Functions
- Types Of Arguments In Python Functions
- Docstring In Python Functions
- Passing Parameters In Python Functions
- Python Function Variables | Scope & Lifetime
- Advantages Of Using Python Functions
- Recursive Python Function
- Anonymous/ Lambda Function In Python
- Nested Functions In Python
- Conclusion
- Frequently Asked Questions
- Python Functions – Test Your Knowledge With A Quiz!
Table of content:
- What Are Python Built-In Functions?
- Mathematical Python Built-In Functions
- Python Built-In Functions For Strings
- Input/ Output Built-In Functions In Python
- List & Tuple Python Built-In Functions
- File Handling Python Built-In Functions
- Python Built-In Functions For Dictionary
- Type Conversion Python Built-In Functions
- Basic Python Built-In Functions
- List Of Python Built-In Functions (Alphabetical)
- Conclusion
- Frequently Asked Questions
- Think You Know Python Built-in Functions? Prove It!
Table of content:
- What Is A round() Function In Python?
- How Does Python round() Function Work?
- Python round() Function If The Second Parameter Is Missing
- Python round() Function If The Second Parameter Is Present
- Python round() Function With Negative Integers
- Python round() Function With Math Library
- Python round() Function With Numpy Module
- Round Up And Round Down Numbers In Python
- Truncation Vs Rounding In Python
- Practical Applications Of Python round() Function
- Conclusion
- Frequently Asked Questions
- Revisit Python’s round() Function – Take The Quiz!
Table of content:
- What Is Python pow() Function?
- Python pow() Function Example
- Python pow() Function With Modulus (Three Parameters)
- Python pow() Function With Complex Numbers
- Python pow() Function With Floating-Point Arguments And Modulus
- Python pow() Function Implementation Cases
- Difference Between Inbuilt-pow() And math.pow() Function
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python’s pow() Function!
Table of content:
- Python max() Function With Objects
- Examples Of Python max() Function With Objects
- Python max() Function With Iterable
- Examples Of Python max() Function With Iterables
- Potential Errors With The Python max() Function
- Python max() Function Vs. Python min() Functions
- Conclusion
- Frequently Asked Questions
- Think You Know Python max() Function? Take A Quiz!
Table of content:
- What Are Strings In Python?
- What Are Python String Methods?
- List Of Python String Methods For Manipulating Case
- List Of Python String Methods For Searching & Finding
- List Of Python String Methods For Modifying & Transforming
- List Of Python String Methods For Checking Conditions
- List Of Python String Methods For Encoding & Decoding
- List Of Python String Methods For Stripping & Trimming
- List Of Python String Methods For Formatting
- Miscellaneous Python String Methods
- List Of Other Python String Operations
- Conclusion
- Frequently Asked Questions
- Mastered Python String Methods? Take A Quiz!
Table of content:
- What Is Python String?
- The Need For Python String Replacement
- The Python String replace() Method
- Multiple Replacements With Python String.replace() Method
- Replace A Character In String Using For Loop In Python
- Python String Replacement Using Slicing Method
- Replace A Character At a Given Position In Python String
- Replace Multiple Substrings With The Same String In Python
- Python String Replacement Using Regex Pattern
- Python String Replacement Using List Comprehension & Join() Method
- Python String Replacement Using Callback With re.sub() Method
- Python String Replacement With re.subn() Method
- Conclusion
- Frequently Asked Questions
- Know How To Replace Python Strings? Prove It!
Table of content:
- What Is String Slicing In Python?
- How Indexing & String Slicing Works In Python
- Extracting All Characters Using String Slicing In Python
- Extracting Characters Before & After Specific Position Using String Slicing In Python
- Extracting Characters Between Two Intervals Using String Slicing In Python
- Extracting Characters At Specific Intervals (Step) Using String Slicing In Python
- Negative Indexing & String Slicing In Python
- Handling Out-of-Bounds Indices In String Slicing In Python
- The slice() Method For String Slicing In Python
- Common Pitfalls Of String Slicing In Python
- Real-World Applications Of String Slicing
- Conclusion
- Frequently Asked Questions
- Quick Python String Slicing Quiz– Let’s Go!
Table of content:
- Introduction To Python List
- How To Create A Python List?
- How To Access Elements Of Python List?
- Accessing Multiple Elements From A Python List (Slicing)
- Access List Elements From Nested Python Lists
- How To Change Elements In Python Lists?
- How To Add Elements To Python Lists?
- Delete/ Remove Elements From Python Lists
- How To Create Copies Of Python Lists?
- Repeating Python Lists
- Ways To Iterate Over Python Lists
- How To Reverse A Python List?
- How To Sort Items Of Python Lists?
- Built-in Functions For Operations On Python Lists
- Conclusion
- Frequently Asked Questions
- Revisit Python Lists Basics With A Quick Quiz!
Table of content:
- What Is List Comprehension In Python?
- Incorporating Conditional Statements With List Comprehension In Python
- List Comprehension In Python With range()
- Filtering Lists Effectively With List Comprehension In Python
- Nested Loops With List Comprehension In Python
- Flattening Nested Lists With List Comprehension In Python
- Handling Exceptions In List Comprehension In Python
- Common Use Cases For List Comprehensions
- Advantages & Disadvantages Of List Comprehension In Python
- Best Practices For Using List Comprehension In Python
- Performance Considerations For List Comprehension In Python
- For Loops & List Comprehension In Python: A Comparison
- Difference Between Generator Expression & List Comprehension In Python
- Conclusion
- Frequently Asked Questions
- Rehash Python List Comprehension Basics With A Quiz!
Table of content:
- What Is A List In Python?
- How To Find Length Of List In Python?
- For Loop To Get Python List Length (Naive Approach)
- The len() Function To Get Length Of List In Python
- The length_hint() Function To Find Length Of List In Python
- The sum() Function To Find The Length Of List In Python
- The enumerate() Function To Find Python List Length
- The Counter Class From collections To Find Python List Length
- The List Comprehension To Find Python List Length
- Find The Length Of List In Python Using Recursion
- Comparison Between Ways To Find Python List Length
- Conclusion
- Frequently Asked Questions
- Know How To Get Python List Length? Prove it!
Table of content:
- List of Methods To Reverse A Python List
- Python Reverse List Using reverse() Method
- Python Reverse List Using the Slice Operator ([::-1])
- Python Reverse List By Swapping Elements
- Python Reverse List Using The reversed() Function
- Python Reverse List Using A for Loop
- Python Reverse List Using While Loop
- Python Reverse List Using List Comprehension
- Python Reverse List Using List Indexing
- Python Reverse List Using The range() Function
- Python Reverse List Using NumPy
- Comparison Of Ways To Reverse A Python List
- Conclusion
- Frequently Asked Questions
- Time To Test Your Python List Reversal Skills!
Table of content:
- What Is Indexing In Python?
- The Python List index() Function
- How To Use Python List index() To Find Index Of A List Element
- The Python List index() Method With Single Parameter (Start)
- The Python List index() Method With Start & Stop Parameters
- What Happens When We Use Python List index() For An Element That Doesn't Exist
- Python List index() With Nested Lists
- Fixing IndexError Using The Python List index() Method
- Python List index() With Enumerate()
- Real-world Examples Of Python List index() Method
- Difference Between find() And index() Method In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python List Indexing? Take A Quiz!
Table of content:
- How To Remove Elements From List In Python?
- The remove() Method To Remove Element From Python List
- The pop() Method To Remove Element From List In Python
- The del Keyword To Remove Element From List In Python
- The clear() Method To Remove Elements From Python List
- List Comprehensions To Conditionally Remove Element From List In Python
- Key Considerations For Removing Elements From Python Lists
- Why We Need to Remove Elements From Python List
- Performance Comparison Of Methods To Remove Element From List In Python
- Conclusion
- Frequently Asked Questions
- Quiz– Prove You Know How To Remove Item From Python Lists!
Table of content:
- How To Remove Duplicates From A List In Python?
- The set() Function To Remove Duplicates From Python List
- Remove Duplicates From Python List Using For Loop
- Using List Comprehension Remove Duplicates From Python List
- Remove Duplicates From Python List Using enumerate() With List Comprehension
- Dictionary & fromkeys() Method To Remove Duplicates From Python List
- Remove Duplicates From Python List Using in, not in Operators
- Remove Duplicates From Python List Using collections.OrderedDict.fromkeys()
- Remove Duplicates From Python List Using Counter with freq.dist() Method
- The del Keyword Remove Duplicates From Python List
- Remove Duplicates From Python List Using DataFrame
- Remove Duplicates From Python List Using pd.unique and np.unipue
- Remove Duplicates From Python List Using reduce() function
- Comparative Analysis Of Ways To Remove Duplicates From Python List
- Conclusion
- Frequently Asked Questions
- Think You Know How to Remove Duplicates? Take A Quiz!
Table of content:
- What Is Python List & How To Access Elements?
- What Is IndexError: List Index Out Of Range & Its Causes In Python?
- Understanding Indexing Behavior In Python Lists
- How to Prevent/ Fix IndexError: List Index Out Of Range In Python
- Handling IndexError Gracefully Using Try-Except
- Debugging Tips For IndexError: List Index Out Of Range Python
- Conclusion
- Frequently Asked Questions
- Avoiding ‘List Index Out of Range’ Errors? Take A Quiz!
Table of content:
- What Is the Python sort() List Method?
- Sorting In Ascending Order Using The Python sort() List Method
- How To Sort Items In Descending Order Using Python sort() List Method
- Custom Sorting Using The Key Parameter Of Python sort() List Method
- Examples Of Python sort() List Method
- What Is The sorted() List Method In Python
- Differences Between sorted() And sort() List Methods In Python
- When To Use sorted() & When To Use sort() List Method In Python
- Conclusion
- Frequently Asked Questions
- Take A Quick Python's sort() Quiz!
Table of content:
- What Is A List In Python?
- What Is A String In Python?
- Why Convert Python List To String?
- How To Convert List To String In Python?
- The join() Method To Convert Python List To String
- Convert Python List To String Through Iteration
- Convert Python List To String With List Comprehension
- The map() Function To Convert Python List To String
- Convert Python List to String Using format() Function
- Convert Python List To String Using Recursion
- Enumeration Function To Convert Python List To String
- Convert Python List To String Using Operator Module
- Python Program To Convert String To List
- Conclusion
- Frequently Asked Questions
- Convert Lists To Strings Like A Pro! Take A Quiz
Table of content:
- What Is Inheritance In Python?
- Python Inheritance Syntax
- Parent Class In Python Inheritance
- Child Class In Python Inheritance
- The __init__() Method In Python Inheritance
- The super() Function In Python Inheritance
- Method Overriding In Python Inheritance
- Types Of Inheritance In Python
- Special Functions In Python Inheritance
- Advantages & Disadvantages Of Inheritance In Python
- Common Use Cases For Inheritance In Python
- Best Practices for Implementing Inheritance in Python
- Avoiding Common Pitfalls in Python Inheritance
- Conclusion
- Frequently Asked Questions
- 💡 Python Inheritance Quiz – Are You Ready?
Table of content:
- What Is The Python List append() Method?
- Adding Elements To A Python List Using append()
- Populate A Python List Using append()
- Adding Different Data Types To Python List Using append()
- Adding A List To Python List Using append()
- Nested Lists With Python List append() Method
- Practical Use Cases Of Python List append() Method
- How append() Method Affects List Performance
- Avoiding Common Mistakes When Using Python List append()
- Comparing extend() With append() Python List Method
- Conclusion
- Frequently Asked Questions
- 🧠 Think You Know Python List append()? Take A Quiz!
Table of content:
- What Is A Linked List In Python?
- Types Of Linked Lists In Python
- How To Create A Linked List In Python
- How To Traverse A Linked List In Python & Retrieve Elements
- Inserting Elements In A Linked List In Python
- Deleting Elements From A Linked List In Python
- Update A Node Of Linked List In Python
- Reversing A Linked List In Python
- Calculating Length Of A Linked List In Python
- Comparing Arrays And Linked Lists In Python
- Advantages & Disadvantages Of Linked List In Python
- When To Use Linked Lists Over Other Data Structures
- Practical Applications Of Linked Lists In Python
- Conclusion
- Frequently Asked Questions
- 🔗 Linked List Logic: Can You Ace This Quiz?
Table of content:
- What Is Extend In Python?
- Extend In Python With List
- Extend In Python With String
- Extend In Python With Tuple
- Extend In Python With Set
- Extend In Python With Dictionary
- Other Methods To Extend A List In Python
- Difference Between append() and extend() In Python
- Conclusion
- Frequently Asked Questions
- Think You Know extend() In Python? Prove It!
Table of content:
- What Is Recursion In Python?
- Key Components Of Recursive Functions In Python
- Implementing Recursion In Python
- Recursion Vs. Iteration In Python
- Tail Recursion In Python
- Infinite Recursion In Python
- Advantages Of Recursion In Python
- Disadvantages Of Recursion In Python
- Best Practices For Using Recursion In Python
- Conclusion
- Frequently Asked Questions
- Recursive Thinking In Python: Test Your Skills!
Table of content:
- What Is Type Conversion In Python?
- Types Of Type Conversion In Python
- Implicit Type Conversion In Python
- Explicit Type Conversion In Python
- Functions Used For Explicit Data Type Conversion In Python
- Important Type Conversion Tips In Python
- Benefits Of Type Conversion In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Type Conversion? Take A Quiz!
Table of content:
- What Is Scope In Python?
- Local Scope In Python
- Global Scope In Python
- Nonlocal (Enclosing) Scope In Python
- Built-In Scope In Python
- The LEGB Rule For Python Scope
- Python Scope And Variable Lifetime
- Best Practices For Managing Python Scope
- Conclusion
- Frequently Asked Questions
- Think You Know Python Scope? Test Yourself!
Table of content:
- Understanding The Continue Statement In Python
- How Does Continue Statement Work In Python?
- Python Continue Statement With For Loops
- Python Continue Statement With While Loops
- Python Continue Statement With Nested Loops
- Python Continue With If-Else Statement
- Difference Between Pass and Continue Statement In Python
- Practical Applications Of Continue Statement In Python
- Conclusion
- Frequently Asked Questions
- Python 'continue' Statement Quiz: Can You Ace It?
Table of content:
- What Are Control Statements In Python?
- Types Of Control Statements In Python
- Conditional Control Statements In Python
- Loop Control Statements In Python
- Control Flow Altering Statements In Python
- Exception Handling Control Statements In Python
- Conclusion
- Frequently Asked Questions
- Mastering Control Statements In Python – Take the Quiz!
Table of content:
- Difference Between Mutable And Immutable Data Types in Python
- What Is Mutable Data Type In Python?
- Types Of Mutable Data Types In Python
- What Are Immutable Data Types In Python?
- Types Of Immutable Data Types In Python
- Key Similarities Between Mutable And Immutable Data Types In Python
- When To Use Mutable Vs Immutable In Python?
- Conclusion
- Frequently Asked Questions
- Quiz Time: Mutable vs. Immutable In Python!
Table of content:
- What Is A List?
- What Is A Tuple?
- Difference Between List And Tuple In Python (Comparison Table)
- Syntax Difference Between List And Tuple In Python
- Mutability Difference Between List And Tuple In Python
- Other Difference Between List And Tuple In Python
- List Vs. Tuple In Python | Methods
- When To Use Tuples Over Lists?
- Key Similarities Between Tuples And Lists In Python
- Conclusion
- Frequently Asked Questions
- 🧐 Lists vs. Tuples Quiz: Test Your Python Knowledge!
Table of content:
- Introduction to Python
- Downloading & Installing Python, IDLE, Tkinter, NumPy & PyGame
- Creating A New Python Project
- How To Write Python Hello World Program In Python?
- Way To Write The Hello, World! Program In Python
- The Hello, World! Program In Python Using Class
- The Hello, World! Program In Python Using Function
- Print Hello World 5 Times Using A For Loop
- Conclusion
- Frequently Asked Questions
- 👋 Python's 'Hello, World!'—How Well Do You Know It?
Table of content:
- Algorithm Of Python Program To Add To Numbers
- Standard Program To Add Two Numbers In Python
- Python Program To Add Two Numbers With User-defined Input
- The add() Method In Python Program To Add Two Numbers
- Python Program To Add Two Numbers Using Lambda
- Python Program To Add Two Numbers Using Function
- Python Program To Add Two Numbers Using Recursion
- Python Program To Add Two Numbers Using Class
- How To Add Multiple Numbers In Python?
- Add Multiple Numbers In Python With User Input
- Time Complexities Of Python Programs To Add Two Numbers
- Conclusion
- Frequently Asked Questions
- 💡 Quiz Time: Python Addition Basics!
Table of content:
- Swapping in Python
- Swapping Two Variables Using A Temporary Variable
- Swapping Two Variables Using The Comma Operator In Python
- Swapping Two Variables Using The Arithmetic Operators (+,-)
- Swapping Two Variables Using The Arithmetic Operators (*,/)
- Swapping Two Variables Using The XOR(^) Operator
- Swapping Two Variables Using Bitwise Addition and Subtraction
- Swap Variables In A List
- Conclusion
- Frequently Asked Questions (FAQs)
- Quiz To Test Your Variable Swapping Knowledge
Table of content:
- What Is A Quadratic Equation? How To Solve It?
- How To Write A Python Program To Solve Quadratic Equations?
- Python Program To Solve Quadratic Equations Directly Using The Formula
- Python Program To Solve Quadratic Equations Using The Complex Math Module
- Python Program To Solve Quadratic Equations Using Functions
- Python Program To Solve Quadratic Equations & Find Number Of Solutions
- Python Program To Plot Quadratic Functions
- Conclusion
- Frequently Asked Questions
- Quadratic Equations In Python Quiz: Test Your Knowledge!
Table of content:
- What Is Decimal Number System?
- What Is Binary Number System?
- What Is Octal Number System?
- What Is Hexadecimal Number System?
- Python Program to Convert Decimal to Binary, Octal, And Hexadecimal Using Built-In Function
- Python Program To Convert Decimal To Binary Using Recursion
- Python Program To Convert Decimal To Octal Using Recursion
- Python Program To Convert Decimal To Hexadecimal Using Recursion
- Python Program To Convert Decimal To Binary Using While Loop
- Python Program To Convert Decimal To Octal Using While Loop
- Python Program To Convert Decimal To Hexadecimal Using While Loop
- Convert Decimal To Binary, Octal, And Hexadecimal Using String Formatting
- Python Program To Convert Binary, Octal, And Hexadecimal String To A Number
- Complexity Comparison Of Python Programs To Convert Decimal To Binary, Octal, And Hexadecimal
- Conclusion
- Frequently Asked Questions
- 💡 Decimal To Binary, Octal & Hex: Quiz Time!
Table of content:
- What Is A Square Root?
- Python Program To Find The Square Root Of A Number
- The pow() Function In Python Program To Find The Square Root Of Given Number
- Python Program To Find Square Root Using The sqrt() Function
- The cmath Module & Python Program To Find The Square Root Of A Number
- Python Program To Find Square Root Using The Exponent Operator (**)
- Python Program To Find Square Root With A User-Defined Function
- Python Program To Find Square Root Using A Class
- Python Program To Find Square Root Using Binary Search
- Python Program To Find Square Root Using NumPy Module
- Conclusion
- Frequently Asked Questions
- 🤓 Think You Know Square Roots In Python? Take A Quiz!
Table of content:
- Understanding the Logic Behind the Conversion of Kilometers to Miles
- Steps To Write Python Program To Convert Kilometers To Miles
- Python Program To Convert Kilometer To Miles Without Function
- Python Program To Convert Kilometer To Miles Using Function
- Python Program to Convert Kilometer To Miles Using Class
- Tips For Writing Python Program To Convert Kilometer To Miles
- Conclusion
- Frequently Asked Questions
- 🧐 Mastered Kilometer To Mile Conversion? Prove It!
Table of content:
- Why Build A Calculator Program In Python?
- Prerequisites To Writing A Calculator Program In Python
- Approach For Writing A Calculator Program In Python
- Simple Calculator Program In Python
- Calculator Program In Python Using Functions
- Creating GUI Calculator Program In Python Using Tkinter
- Conclusion
- Frequently Asked Questions
- 🧮 Calculator Program In Python Quiz!
Table of content:
- The Calendar Module In Python
- Prerequisites For Writing A Calendar Program In Python
- How To Write And Print A Calendar Program In Python
- Calendar Program In Python To Display A Month
- Calendar Program In Python To Display A Year
- Conclusion
- Frequently Asked Questions
- Calendar Program In Python – Quiz Time!
Table of content:
- What Is The Fibonacci Series?
- Pseudocode Code For Fibonacci Series Program In Python
- Generating Fibonacci Series In Python Using Naive Approach (While Loop)
- Fibonacci Series Program In Python Using The Direct Formula
- How To Generate Fibonacci Series In Python Using Recursion?
- Generating Fibonacci Series In Python With Dynamic Programming
- Fibonacci Series Program In Python Using For Loop
- Generating Fibonacci Series In Python Using If-Else Statement
- Generating Fibonacci Series In Python Using Arrays
- Generating Fibonacci Series In Python Using Cache
- Generating Fibonacci Series In Python Using Backtracking
- Fibonacci Series In Python Using Power Of Matix
- Complexity Analysis For Fibonacci Series Programs In Python
- Applications Of Fibonacci Series In Python & Programming
- Conclusion
- Frequently Asked Questions
- 🤔 Think You Know Fibonacci Series? Take A Quiz!
Table of content:
- Different Ways To Write Random Number Generator Python Programs
- Random Module To Write Random Number Generator Python Programs
- The Numpy Module To Write Random Number Generator Python Programs
- The Secrets Module To Write Random Number Generator Python Programs
- Understanding Randomness and Pseudo-Randomness In Python
- Common Issues and Solutions in Random Number Generation
- Applications of Random Number Generator Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python's Random Module? Prove It!
Table of content:
- What Is A Factorial?
- Algorithm Of Program To Find Factorial Of A Number In Python
- Pseudocode For Factorial Program in Python
- Factorial Program In Python Using For Loop
- Factorial Program In Python Using Recursion
- Factorial Program In Python Using While Loop
- Factorial Program In Python Using If-Else Statement
- The math Module | Factorial Program In Python Using Built-In Factorial() Function
- Python Program to Find Factorial of a Number Using Ternary Operator(One Line Solution)
- Python Program For Factorial Using Prime Factorization Method
- NumPy Module | Factorial Program In Python Using numpy.prod() Function
- Complexity Analysis Of Factorial Programs In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Factorials In Python? Take A Quiz!
Table of content:
- What Is Palindrome In Python?
- Check Palindrome In Python Using While Loop (Iterative Approach)
- Check Palindrome In Python Using For Loop And Character Matching
- Check Palindrome In Python Using The Reverse And Compare Method (Python Slicing)
- Check Palindrome In Python Using The In-built reversed() And join() Methods
- Check Palindrome In Python Using Recursion Method
- Check Palindrome In Python Using Flag
- Check Palindrome In Python Using One Extra Variable
- Check Palindrome In Python By Building Reverse, One Character At A Time
- Complexity Analysis For Palindrome Programs In Python
- Real-World Applications Of Palindrome In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Palindromes? Take A Quiz!
Table of content:
- Best Python Books For Beginners
- Best Python Books For Intermediate Level
- Best Python Books For Experts
- Best Python Books To Learn Algorithms
- Audiobooks of Python
- Best Books To Learn Python And Code Like A Pro
- To Learn Python Libraries
- Books To Provide Extra Edge In Python
- Python Project Ideas - Reference
- Quiz To Rehash Your Knowledge Of Python Books!
40+ Python String Methods Explained | List, Syntax & Code Examples
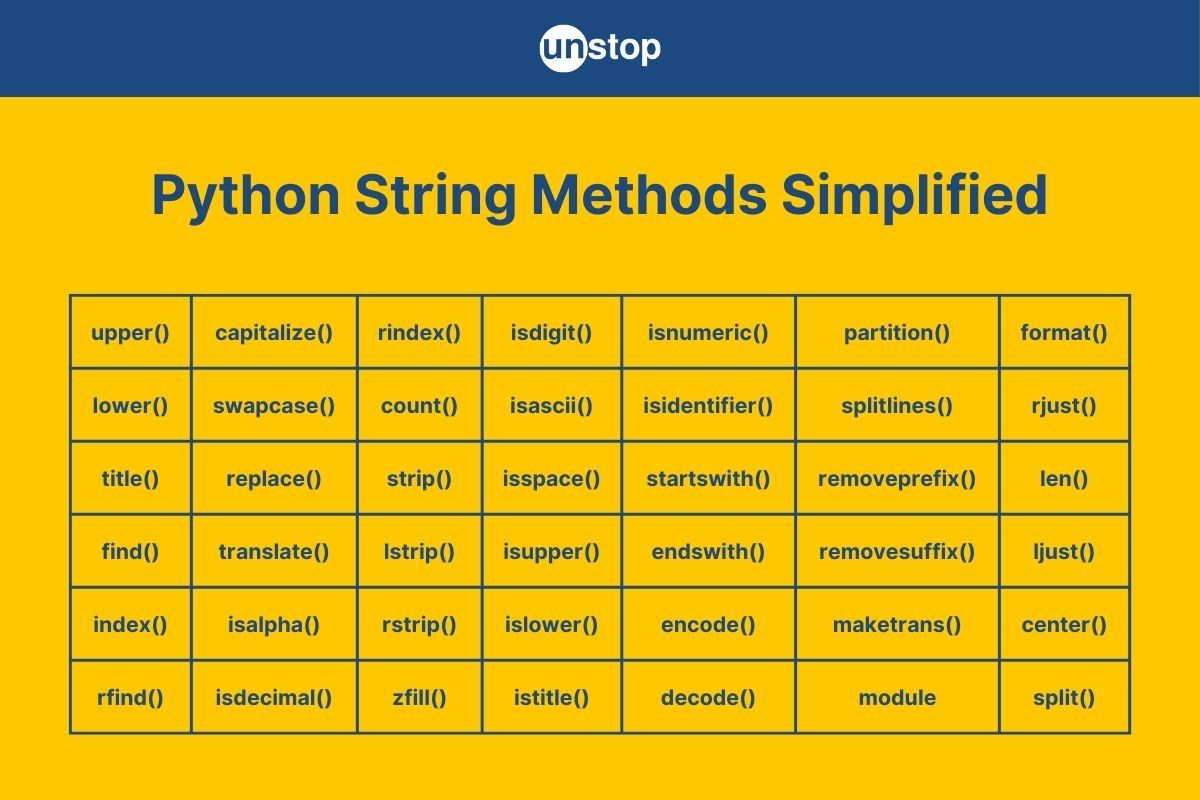
Strings are one of the most fundamental ways to handle text in Python. From storing names and email addresses to processing entire books, strings are everywhere in the programming world. But working with strings isn't just about storing or printing them. Python gives us a powerful toolkit called string methods that can transform, analyze, or polish strings to perfection.
In this article, we will discuss the Python string methods, what they are, and why we use them. We will also provide a list of the string methods, categorizing them by their use cases and showing how they can simplify real-world tasks.
What Are Strings In Python?
A string in Python is a sequence of characters enclosed in quotes. You can use either single quotes ('), double quotes ("), or triple quotes (''' or """) for defining strings. Strings are immutable, meaning their content can’t be changed after creation. However, Python provides several methods to manipulate them effectively without altering the original string.
For more information on strings, check out Python Strings | Create, Format, Reassign & More (+Examples)
What Are Python String Methods?
String methods refer to built-in functions in Python designed to work specifically with strings. Think of them as tools in a Swiss Army knife—each one is tailored to handle a specific operation, such as transforming text to uppercase, trimming unwanted spaces, or checking whether a string contains only numbers.
The Python string methods are important because they:
- Make string manipulation simple and intuitive.
- Save time and effort by eliminating the need to write complex code for common tasks.
- Help improve code readability, making it easier to understand and maintain.
Whether you're building a chatbot, processing data, or just formatting a title for your blog post, string methods are your go-to companions for clean and efficient code.
List Of Python String Methods For Manipulating Case
When working with text, you often need to standardize capitalization—for example, converting user input to lowercase or formatting titles. Python programming offers several methods to manipulate the case of strings, as listed in the table below.
Name |
Syntax |
Description |
upper() |
string.upper() |
Converts all characters in the string to uppercase. |
lower() |
string.lower() |
Converts all characters in the string to lowercase. |
capitalize() |
string.capitalize() |
Capitalizes the first character of the string. |
title() |
string.title() |
Converts the first character of each word to uppercase. |
swapcase() |
string.swapcase() |
Swaps the case of all characters in the string. |
Let’s look at a simple Python program example, which illustrates the use of two of the most common case manipulation methods.
Code Example:
#Creating a string
text = "python string methods"
#Using upper() to convert string to caps
print(text.upper()) # Output: PYTHON STRING METHODS
#Using title() to convert string to title case
print(text.title()) # Output: Python String Methods
Code Explanation:
In the simple Python code example:
- We first create a string named text, containing the value “python string method” (all small cases).
- Then, we call the method upper() to convert the entire string to uppercase (i.e., text.upper()), making it useful for standardizing text input like usernames.
- Similarly, we call the title() Python string method to apply the title case. It capitalizes the first letter of each word, often used for formatting headlines or titles.
- We use the print() function to call the methods and display the strings to the console.
List Of Python String Methods For Searching & Finding
There will be instances when you may need to locate or search for specific content within a string. Listed in the table below are the various methods that Python language provides to help you find substrings, indices, or patterns.
Name |
Syntax |
Description |
find() |
string.find(substring) |
Returns the index of the first occurrence of the substring, or -1 if not found. |
index() |
string.index(substring) |
Similar to find(), but raises a ValueError if the substring is not found. |
rfind() |
string.rfind(substring) |
Returns the index of the last occurrence of the substring, or -1 if not found. |
rindex() |
string.rindex(substring) |
Similar to rfind(), but raises a ValueError if the substring is not found. |
string.count(substring) |
Returns the number of non-overlapping occurrences of the substring. |
The basic Python program example below illustrates how to use two of the most common finding functions.
Code Example:
#Creating a string variable
text = "Unstop helps you learn, practice, and compete"
#Using find() to find the index position of the substring
print(text.find("learn")) # Output: 12
#Using count() to get the frequency of a character
print(text.count("e")) # Output: 7
Code Explanation:
In the basic Python code example:
- We create a string variable named text, containing the value “Unstop helps you learn, practice, and compete"
- Then, we call the find() Python string method on text, passing the string “learn” as an argument. The function searches for the substring and returns the starting index of its first occurrence.
- Similarly, we call the count() Python string method on text, passing the character “e” as an argument. It counts and returns the number of times the letter "e" appears in the string.
Check out this amazing course to become the best version of the Python programmer you can be.
List Of Python String Methods For Modifying & Transforming
Python provides methods for removing unwanted characters, replacing text, and more, as well as cleaning up or modifying strings. We have listed the Python string methods used for this purpose in the table below.
Name |
Syntax |
Description |
strip() |
string.strip([chars]) |
Removes leading and trailing characters (default is whitespace). |
lstrip() |
string.lstrip([chars]) |
Removes leading characters (default is whitespace). |
rstrip() |
string.rstrip([chars]) |
Removes trailing characters (default is whitespace). |
string.replace(old, new[, count]) |
Replaces all occurrences of a substring with another substring. |
|
zfill() |
string.zfill(width) |
Pads the string on the left with zeros to make it a specific length. |
translate() |
string.translate(map) |
Replaces characters in the string based on a translation map (used with str.maketrans). |
The Python program example below illustrates the use of the zfill() and the strip() Python string methods.
Code Example:
#Creating a string with whitespaces
text = " Python "
#Using strip() to remove whitespaces
cleaned = text.strip()
#Using zfill() to pad left space
padded = cleaned.zfill(12)
print(cleaned) # Output: "Python"
print(padded) # Output: "0000Python"
Code Explanation:
In the Python code example:
- We have a string object containing a string with whitespaces both on the left and the right sides.
- Then, we use the strip() Python string operation to remove the extra spaces around "Python", leaving the core text.
- Similarly, we use the zfill() Python string method, passing 12 as an argument. It pads the string with zeros on the left to ensure it has a total width of 12 characters.
List Of Python String Methods For Checking Conditions
These methods are used to check specific properties of a string, such as whether it's numeric, alphabetic, uppercase, etc. They are essential for validating or filtering data based on certain criteria.
Name |
Syntax |
Description |
isalpha() |
string.isalpha() |
Returns True if all characters in the string are alphabetic; otherwise, False. |
isdigit() |
string.isdigit() |
Returns True if all characters in the string are digits; otherwise, False. |
isdecimal() |
string.isdecimal() |
Returns True if all characters in the string are decimal characters; otherwise, False. |
isascii() |
string.isascii() |
Returns True if all characters in the string are ASCII characters; otherwise, False. |
isspace() |
string.isspace() |
Returns True if all characters in the string are whitespace characters; otherwise, False. |
isupper() |
string.isupper() |
Returns True if all characters in the string are uppercase; otherwise, False. |
islower() |
string.islower() |
Returns True if all characters in the string are lowercase; otherwise, False. |
istitle() |
string.istitle() |
Returns True if the string is in title case; otherwise, False. |
isnumeric() |
string.isnumeric() |
Returns True if all characters in the string are numeric; otherwise, False. |
isidentifier() |
string.isidentifier() |
Returns True if the string is a valid Python identifier; otherwise, False. |
startswith() |
string.startswith(prefix) |
Returns True if the string starts with the specified prefix; otherwise, False. |
endswith() |
string.endswith(suffix) |
Returns True if the string ends with the specified suffix; otherwise, False. |
We have illustrated the use of three of these functions in the example Python program below.
Code Example:
#String with number and title case
text = "Python3"
#Using Python string methods to check for uppercase, space and alphanumerics
print(text.isalnum()) # Output: True
print(text.isupper()) # Output: False
print(text.isspace()) # Output: False
Code Explanation:
In the example Python code:
- We begin with an object text, containing string value “Python3”.
- Then, we use the isalnum() to check if the string contains only letters and numbers.
- Similarly, the Python string function isupper() checks if the string has all uppercase letters.
- Finally, the Python string function isspace() checks if the string is made up entirely of whitespace characters.
- We call all the Python string methods inside the print() function in Python so as to directly print the outcome to the console.
List Of Python String Methods For Encoding & Decoding
When working with different character sets or preparing strings for transmission over networks, encoding and decoding become crucial. Python provides methods to handle these operations effectively.
Name |
Syntax |
Description |
encode() |
string.encode([encoding], [errors]) |
Encodes the string using the specified encoding (default is UTF-8). |
decode() |
string.decode([encoding], [errors]) |
Decodes the byte string back to a regular string using the specified encoding. |
Look at the sample Python program below, which illustrates how these Python string functions work.
Code Example:
#Creating a string
text = "Python is fun!"
#Using encode() and decode()
encoded_text = text.encode() # Default is UTF-8
decoded_text = encoded_text.decode()
print(encoded_text) # Output: b'Python is fun!'
print(decoded_text) # Output: Python is fun!
Code Explanation:
In the sample Python code:
- We use the encode() string built-in function in Python to convert the string "Python is fun!" into a byte string. The default UTF-8 encoding is essential for file I/O and web communication.
- Then, we use the Python string function decode(), which converts the encoded byte string back to a regular string, allowing us to work with the original text again.
Looking for guidance? Find the perfect mentor from select experienced coding & software development experts here.
List Of Python String Methods For Stripping & Trimming
When dealing with strings, it’s common to encounter extra whitespace or unwanted characters that need to be removed. There is a suite of string built-in functions in Python libraries designed specifically for this task.
Name |
Syntax |
Description |
strip() |
string.strip([chars]) |
Removes leading and trailing characters (default is whitespace). |
lstrip() |
string.lstrip([chars]) |
Removes leading characters (default is whitespace). |
rstrip() |
string.rstrip([chars]) |
Removes trailing characters (default is whitespace). |
We have illustrated how to use these string methods in the Python program sample below.
Code Example:
#String with whitespace
text = " Hello, World! "
print(text.strip()) # Output: "Hello, World!"
print(text.lstrip()) # Output: "Hello, World! Â "
print(text.rstrip()) # Output: " Â Hello, World!"
Code Explanation:
In the Python code sample:
- We create a variable text and assign a string value with whitespaces.
- The, we call the strip() Python string method to remove both leading and trailing spaces from the string, cleaning up unnecessary padding.
- Similarly, the lstrip() and rstrip() Python string functions allow us to remove spaces only from the left or right side, respectively.
- The outcome of the first two operations is the “Hello, World!” string without any spaces.
List Of Python String Methods For Formatting
Formatting strings is a common task, especially when you need to present data in a specific structure or pattern. Python provides several methods to make string formatting flexible and efficient.
Name |
Syntax |
Description |
format() |
string.format(*args, **kwargs) |
Inserts the arguments into placeholders defined in the string. |
f"string {variable}" |
A more concise way to embed expressions inside string literals (available from Python 3.6). |
|
rjust() |
string.rjust(width, [fillchar]) |
Right-justifies the string, padding it with spaces (or a specified character) to make it a specified width. |
ljust() |
string.ljust(width, [fillchar]) |
Left-justifies the string, padding it with spaces (or a specified character) to make it a specified width. |
center() |
string.center(width, [fillchar]) |
Centers the string, padding it with spaces (or a specified character) to make it a specified width. |
Code Example:
name = "Shivani"
#Using the string.format() method
greeting = "Hello, {}!".format(name)
#Using the f-stirng method
formatted_greeting = f"Hello, {name}!"
print(greeting) # Output: Hello, Shivani!
print(formatted_greeting) # Output: Hello, Shivani!
Code Explanation:
- We begin with a string object containing the value– “Shivani”.
- Then, we use the format() Python string function that allows us to place variables within the string using curly braces {} and is more flexible for inserting multiple variables.
- Next, we use the f-string method in Python, which is a shorthand for string formatting. Introduced in Python 3.6, making the code cleaner and easier to read.
- The outcome of both the Python string methods is the same.
Miscellaneous Python String Methods
This section will include Python string methods that don’t fall directly under other categories but are still valuable for various string manipulations.
Name |
Syntax |
Description |
partition() |
string.partition(separator) |
Splits the string into a 3-part tuple at the first occurrence of the separator. |
splitlines() |
string.splitlines([keepends]) |
Splits the string at line breaks and returns a list of lines. If keepends is True, line breaks are included. |
removeprefix() |
string.removeprefix(prefix) |
Removes the specified prefix from the string (available from Python 3.9+). |
removesuffix() |
string.removesuffix(suffix) |
Removes the specified suffix from the string (available from Python 3.9+). |
maketrans() |
string.maketrans(x, y) |
Creates a mapping table for translation (used in translate() method). |
List Of Other Python String Operations
This section covers common string operations that don't necessarily qualify as methods but are widely used in Python for manipulating and working with strings.
Operation |
Syntax |
Description |
Length |
len(string) |
Is used to find the length of a string, i.e., returns the number of characters in the string. |
string[start:end:step] |
Extracts a portion of the string based on the specified start, end, and step indices. |
|
Replacing |
string.replace(old, new, count) |
Replaces occurrences of the substring old with new in the string. Optionally, a count argument can limit the number of replacements. |
Finding Substring |
string.find(substring) |
Returns the index of the first occurrence of the substring in the string. Returns -1 if the substring is not found. |
Formatting |
string.format(*args, **kwargs) |
Allows you to format strings by inserting values at specified positions. Also supports named placeholders and formatted representations. |
F-strings |
f"string {variable}" |
A shorthand for string interpolation, introduced in Python 3.6, which allows embedding expressions directly within string literals. |
string1 + string2 |
Combines two or more strings into one. |
|
Splitting |
string.split(separator) |
Splits the string into a list of substrings based on the specified separator. |
Trimming |
string.strip(), string.lstrip(), string.rstrip() |
Removes leading/trailing spaces or specified characters from the string. |
Code Example:
text = " Hello, World! "
# Slicing
print(text[1:5]) # Output: "Hell"
# Replacing
print(text.replace("World", "Universe")) # Output: " Hello, Universe! "
# Finding
print(text.find("World")) # Output: 7
Code Explanation:
- Slicing lets you extract a part of the string using a range of indices.
- The replace() Python string method modifies the string by replacing one substring with another.
- The find() function searches for a substring and returns its index, or -1 if not found.
Conclusion
In this article, we’ve explored a wide variety of Python string methods and operations that are essential for manipulating and working with strings. From checking conditions like whether a string is numeric or uppercase, to formatting and slicing strings, Python provides a rich set of tools for every string-related task.
Whether you're a beginner or an experienced developer, understanding these methods and operations will significantly enhance your ability to work with text and string data in Python. With the comprehensive list of string methods we've covered, you'll be well-equipped to handle string manipulation for various applications—from data processing to text analysis.
Ready to upskill your Python game? Dive deeper into actual problem statements and elevate your coding game with hands-on practice!
Frequently Asked Questions
Q1. What is the difference between find() and index() in Python?
Both find() and index() functions are used to search for a substring in a string. However, find() returns -1 if the substring is not found, while index() raises a ValueError in the same case.
Q2. How can I concatenate strings in Python?
You can concatenate strings using the + operator or by using the join() method for more efficient concatenation, especially when joining a list of strings.
Q3. How do I check if a string is empty in Python?
You can check if a string is empty using the len() function to find the length and the equality relational operator to check if it is zero, i.e., len(string) == 0. Alternatively, you can simply if not string: to test its truth value.
Q4. What is the use of strip() method in Python?
The strip() method is used to remove any leading and trailing spaces or specified characters from a string.
Q5. How do I format strings in Python?
String formatting in Python can be done using methods like format(), f-strings (available from Python 3.6), or older % formatting. Each method has its use case, but f-strings are generally preferred for their simplicity and readability.
Mastered Python String Methods? Take A Quiz!
Keep experimenting with these Python string methods, and you'll find that string handling is both powerful and versatile. You might also enjoy reading:
- 12 Ways To Compare Strings In Python Explained (With Examples)
- How To Reverse A String In Python? 10 Easy Ways With Examples
- How To Convert Python List To String? 8 Ways Explained (+Examples)
- Convert Int To String In Python | Learn 6 Methods With Examples
- If-Else Statement In Python | All Conditional Statements + Examples
- List Comprehension In Python | A Complete Guide With Example Codes
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Divyansh Shrivastava 3 weeks ago
Pranjul Srivastav 1 month ago
Vishwajeet Singh 1 month ago
SIRIVERU MAHESWARI 1 month ago
niyati m singh 1 month ago
Pardha Venkata Sai Patnam 1 month ago