How To Print Without Newline In Python? (Mulitple Ways + Examples)
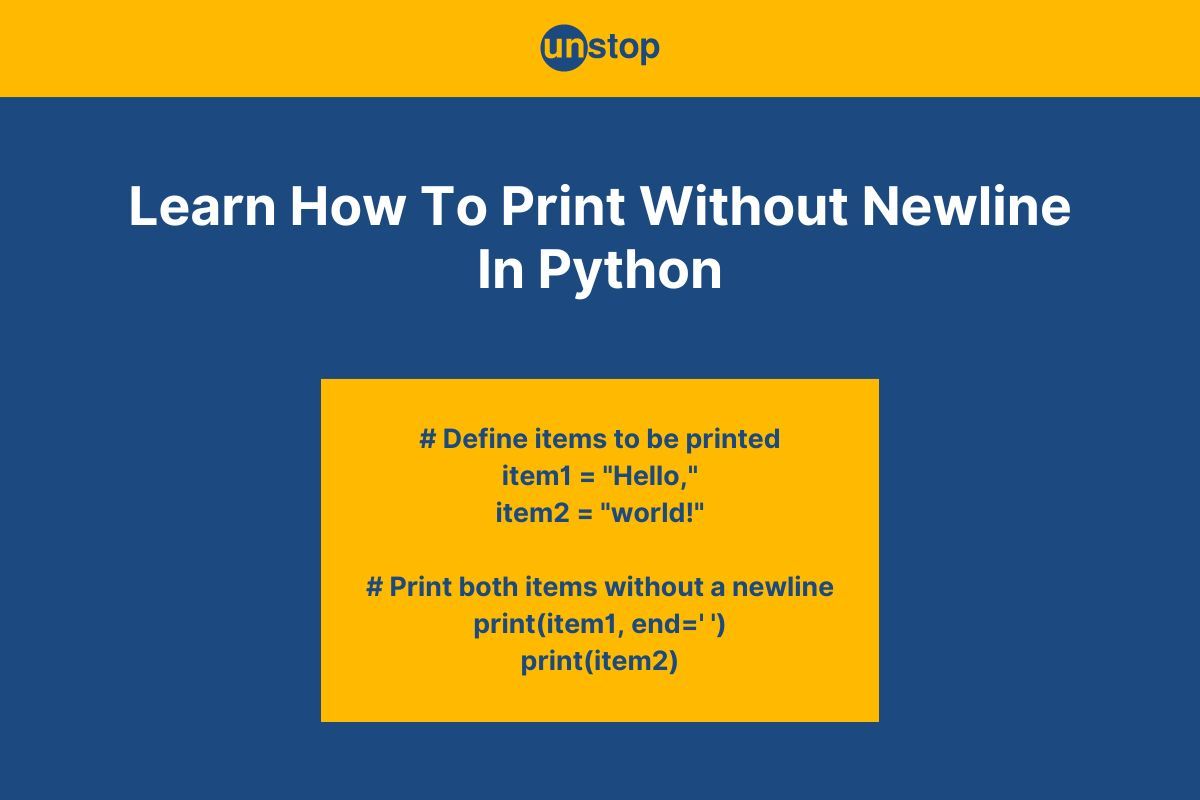
Table of content:
- Working Of Normal Print() Function
- The New Line Character In Python
- How To Print Without Newline In Python | Using The End Parameter
- How To Print Without Newline In Python 2.x? | Using Comma Operator
- How To Print Without Newline In Python 3.x?
- How To Print Without Newline In Python With Module Sys
- The Star Pattern(*) | How To Print Without Newline & Space In Python
- How To Print A List Without Newline In Python?
- How To Remove New Lines In Python?
- Conclusion
- Frequently Asked Questions
Printing of output or other elements is a common task performed by developers to display information to the console or terminal. The print() function is a built-in function in Python language used to print the specified message or object to any standard output device. This function, by default, adds a newline character at the end of each printed statement, which moves the cursor to the next line. But if that were not the case, would you know how to print without newline in Python programs?
There might be situations where you might want to print without this newline, such as when you want to display output on the same line or when formatting output for a specific purpose. The newline is a character or sequence of characters, represented by (/n), that indicates the end of a line in a string or a text file. In this article, we'll explore various techniques to print without a newline character in Python, covering different Python versions and their respective methods.
Working Of Normal Print() Function
To understand how to print without newline in Python, we have to first understand the functioning of the print function. The print() function in Python programming language takes any string or object as input and prints it to a screen or any other standard output device. If the object taken as input is not a string, then it converts the object to a string and displays it on screen. The steps explained below showcase the detailed functioning of the built-in function print():
- Function Call: The first step is the call to the print() function, i.e., the process via which the print function is invoked in the Python code when we need to print any message or value.
- Pass the arguments: We can pass zero or even multiple arguments to the print() function as per the requirements. The positional arguments can contain variables as well as data types like integers, floats, strings, etc.
- Conversion to a string: If the arguments inside the print() function are not already strings, then those arguments are converted into strings by applying the str() function. The str() function performs type conversion on any datatype and converts it to a string.
- String concatenation: If the print() function contains multiple arguments, then the string representations of those arguments are concatenated, and space characters are included between them by default.
- Output: The resultant string representation of the arguments is printed on the default print output device or screen.
Syntax Of Print() Function:
print(object, sep=separator, end=end, file=file, flush=flush)
Here,
- object: It is one or more values to be printed.
- sep: It separates objects to be printed. Its default value is ‘ ‘.
- end: It specifies what to print at the end of the line. Its default value is ‘\n’.
- file: It specifies where to print the output. Its default value is sys.stdout.
- flush: It specifies if the output is true or false. Its default value is false.
Code Example:
Output:
The value of x is 67
Explanation:
In the basic Python program-
- We first create a variable x and assign the integer value 67 to it.
- After that, we invoke/ call the print() function with two arguments, where one is the string message- 'The value of x is', and the other is the variable x.
- As a result the print function converts argument x to a string using the str() function.
- After conversion, the converted string version of x is concatenated with the first string argument.
- At last, the concatenated string is displayed on the screen.
Time complexity: The time complexity is O(1).
Space complexity: The space complexity of the print() function is O(1).
The New Line Character In Python
The newline character, often represented as \n, is a special control character used in text processing to indicate the end of a line. When encountered in a string, the newline specifier instructs the output device (such as a terminal, console, or text file) to move the cursor to the beginning of the next line. This allows for the organization and formatting of text into separate lines, improving readability and structure.
Syntax:
\n
Here,
- \: It is a backslash, which is called an escape sequence. It is used to add any special character to a string that has some functionality.
- n: It stands for a new line.
Code Example:
Output:
Hello,
world!
Explanation:
In this Python example-
- We create a string variable called message, and assign the text "Hello," followed by a newline character (\n), and then "world!" to it.
- Next, we use the print() function display the value of message variable to the console.
- When this string is printed using the print() function, the newline character \n instructs the program to move to the next line before printing "world!", resulting in the output being displayed on two separate lines.
- The print() function directly outputs the content of the message string, respecting the newline character present within it.
- As a result, "Hello," is printed on the first line, and "world!" is printed on the second line due to the newline character.
How To Print Without Newline In Python | Using The End Parameter
Printing without newline in Python allows you to display multiple items or pieces of information on the same line, without automatically moving to the next line after each print statement. This can be useful when you want to concatenate multiple outputs or maintain a specific formatting style.
- To print without a newline by default in Python, you can use the end parameter of the print() function.
- The print function, by default, adds a newline character at the end of each print statement. However, by specifying an alternative value for the end parameter, you can control what is printed after the content of the print() statement.
Syntax:
print("Message", end=' ')
Here,
- print(): It is a function that prints values and messages.
- end: It is a parameter in the print() function that defines the end of the line.
Let us understand how to print without newline in Python with the help of a simple Python program example below.
Code Example:
Output:
Hello, world!
This is another line.
Explanation:
In the Python code example-
- We define two string variables, i.e., item1 with the value "Hello," and item2 with the value "world!".
- Next, we use the print() function to display the first string value. By default, the print function adds a newline character at the end, moving to the next line after printing.
- This is why we have specified end=' ' as an argument, which overrides the default behavior and sets the end character to a space instead of a newline.
- As a result, when item1 is printed, it ends with a space instead of a newline. Then, the next print() statement displays item2 on the same line as item1 with a space in between. As shown in the output, we have a single line with the string message- "Hello, world!"
- After that, we use another print() function without any modifications, demonstrating that subsequent print() statements will start on a new line unless specified otherwise.
- Hence, the last print function displays the string contained in a new line. This example specifies how to print without newline in Python and provides a comparison for this with the usual case.
Time Complexity: The time complexity of a Python print without newline is O(1), as it does not depend on the length of the string being printed.
Space Complexity: The space complexity of a Python print without newline is O(1) because no additional space is used by the print() function to print a given value.
How To Print Without Newline In Python 2.x? | Using Comma Operator
In Python 2.x, a comma is placed after the print statement to print without a new line. When we add a comma to the end of a print statement, it signifies that the next print statement will begin on the same line. It also automatically puts a space between the printed values or messages for clarity.
Syntax To Print Without Newline In Python 2.x:
print "message to be printed",
Here,
- print: It represents the print statement.
- "message to be printed": The message or content to be printed without a newline.
- The comma (,) at the end of the print statement suppresses the automatic newline.
For a better understanding of how to print without newline in Python 2.x, look at the sample Python program given below.
Code Example:
Output:
Hi there! Good Evening.
How to print without newline in Python?
Explanation:
In the Python code sample-
- We demonstrate how to print without automatically appending a newline character at the end of each print statement in Python 2.x.
- We begin by utilizing a print statement to output the string "Hi there!" followed by a comma (,) at the end.
- In Python 2.x, when a comma is used at the end of a print statement, it prevents the addition of a newline character. Therefore, after the string message is printed to the console, the cursor does not move to the next line.
- So, the string message in the next print() function, i.e., Good Evening, is printed on the same line as the previous print statement, separated by a space.
- Lastly, we use a print statement to output the string "How to print without newline in Python?". As this is a separate print statement, it appears on a new line, adhering to the default behaviour of print statements to move to the next line after execution.
Time Complexity: The time complexity of a Python print without newline 2.x version is O(1).
Space Complexity: The space complexity of the single print statement is also O(1).
How To Print Without Newline In Python 3.x?
Printing without newline in Python 3.x uses the end parameter method that we have already discussed above in detail. That is, an additional argument is provided in the print() function in Python 3.x, which helps to remove the new line placed at the end of the message to be printed by default. This parameter is called the ‘end’ parameter.
The default value of the end parameter is ‘\n’. In order to print without new line in Python 3.x, the empty string (space character) is passed as a value to the end parameter. It ensures that the next message is printed on the same line. The example given below demonstrates how to print without newline in Python 3.x.
Code Example:
Output:
Hello world. Welcome to the Python at Unstop!
How To Print Without Newline In Python With Module Sys
The Python sys module is a built-in module that provides access to various variables and functions used to manipulate the Python runtime environment. It operates on the interpreter through the variables and functions that interact with the interpreter.
- In order to execute a Python print without newline using the sys module, first we have to import the sys module into our Python code using the import keyword.
- After that, we use the stdout.write() function of the sys module to print the desired string without the newline.
- The stdout.write() function only works with strings. It gives an error if we try to print a number or list.
Syntax:
import sys
# Using stdout.write() from sys module
sys.stdout.write(“Message to be printed”)
Here,
- import: It is a keyword to import the desired library or module.
- sys: A built-in module in Python that helps to operate on the interpreter.
- stdout.write(): A function of the sys module used to print strings without a newline.
To understand how to print without newline in Python using the sys module, let us look at the example Python program below.
Code Example:
Output:
Hello world! What a lovely day!
Explanation:
In this example Python code-
- We import the sys module using the import keyword in order to inherit its variables and functions in the code.
- After that, we use the stdout.write() function of the sys module to print the string value- “Hello World! ”. Note that there is a space character at the end of the string.
- Since the stdout.write() function does not have a newline default like the print() function, the cursor remains in the same line after printing the message.
- We then call the stdout.write() function again to print another string value- “What a lovely day!”
- As shown in the output, both strings are printed one after the other without any newline between them.
Time Complexity: The time complexity of the sys.stdout.write() function in Python print without newline using the sys module remains O(1) like the ordinary print() function because it does not depend on the input size.
Space Complexity: The space complexity of the sys.stdout.write() function in Python print without newline using the sys module is also O(1) because it is a constant-time operation that does not depend on the size of the string to be printed.
The Star Pattern(*) | How To Print Without Newline & Space In Python
The star pattern is commonly used in various programming exercises and applications for visual representation or formatting purposes. To print the star pattern (*) without newline and space in Python, we can use nested loops to control the number of stars printed on each line and the number of lines in the pattern. We use the print() function with the end parameter set to an empty string ('') to prevent it from adding a newline at the end of each line.
Syntax:
for i in range(rows):
for j in range(columns):
print('*', end='')
print(end='')
Here,
- rows: The number of rows in the pattern.
- columns: The number of stars (*) to be printed in each row.
Code Example:
Output:
*************************
Explanation:
In the sample Python code-
- We define the number of rows and columns in the pattern, both set to 5 in this example.
- Then, we use nested for loops to iterate over each row and column in the pattern.
- The inner for loop prints a star (*) character for each column in the current row, without adding spaces between them.
- The print() function inside the inner loop has its end parameter set to an empty string (' ') to prevent it from adding a new line at the end of each line.
- After printing all the stars in a row, the outer for loop prints a new line using another print() statement with no arguments, effectively moving to the next line.
Time Complexity: The time complexity of this code is O(n^2), where n is the total number of stars printed in the pattern.
Space Complexity: The space complexity is also O(n^2), as the code only temporarily stores the stars to be printed in memory.
How To Print A List Without Newline In Python?
The list in Python is a datatype used for the collection of items. When we want to print the items inside the list, we use a loop and iterate through the list, printing each item one by one. To print a list without a newline, we should follow these steps:
- First of all, initialize a list with some items.
- Then, execute a for loop to iterate through the list with the help of an iterator object.
- Inside the for loop, implement the print() function with the iterator as its argument to print the items inside the list one by one.
- Pass an empty string as an argument to the end parameter of the print() function to ensure that the cursor doesn’t move to the next line after printing the current list item.
Syntax:
mylist = [item1, item2, item3]
for iterator_obj in mylist:
print(iterator_obj, end= " ")
Here,
- The term mylist refers to the name of the list we are working with.
- The for keyword marks the beginning of the for loop, with iterator_obj referring to the elements/ objects of the list we are iterating over.
- The print() function is used to output values and end=" " is an argument in the function that denotes the end of the string.
Code Example:
Output:
Compete Upskill Learn Practice Prep
Explanation:
In the Python program example-
- We create a list called actions to represent things to do for career development & growth and initialize it with the elements- Compete, Upskill, Learn, Practice, and Prep.
- Then, we execute a for loop to iterate through the list with the help of the iterator object i.
- Inside the for loop, the print() function is executed with i as an argument to print the current item in the list.
- The end parameter in the list is initialized as an empty string with a single space so that all the items are printed in the same line with a single space between every two items.
- Note that had the end parameter not been used, the elements of the list would have been printed in separate lines.
- The empty print() statement at the last line prints a newline at the end.
- In this way, the output is obtained as a list of all fruits in the same line.
Time Complexity: The time complexity of this code is O(n).
Space Complexity: The space complexity of this code is O(n).
How To Remove New Lines In Python?
We can use the strip() function in order to remove new lines from a string in Python. The strip() function eliminates all the extra spaces and new line characters at the beginning and end of the string. However, it does not remove any space or newline character present between the string itself. Here is how to print without newline in Python using the strip() function:
- The first step is to initialize a string with some variable values having newline characters or extra spaces at its starting or ending.
- Then, call the strip() function on the desired string, eliminating all the new line characters and extra spaces from the beginning and end of the string.
- The output of the strip() function is stored in another variable.
- Then, the new string that is stored in the variable is printed.
Syntax:
string.strip()
Here, the term string is the name of the string variable containing the message with a newline at its beginning or end. The strip() function is used to eliminate the extra spaces and new lines from the string’s beginning and end.
Code Example:
Output:
Original string:
'\n Hello, world! \n'
Stripped string:
'Hello, world!'
Explanation:
In the Python example code-
- We begin by initializing a string s with a value that has newline characters or extra spaces at its beginning and end.
- Next, we apply the strip() function to remove the leading and trailing whitespace characters, including newlines, from the string.
- The result of the strip() operation is stored in the variable stripped_s.
- To demonstrate the changes made, we print the original string using repr(s) to display special characters such as newline (\n).
- We then print the stripped string using repr(stripped_s) to show the string after whitespace removal.
Time Complexity: The time complexity of the strip() function is O(n).
Space Complexity: The space complexity of the strip() function is O(n).
Conclusion
By default, the print() function in Python includes a newline character whenever it displays a value. In this article, we have discussed how to print without using newline in Python code. This can be achieved using various advanced techniques, depending on the Python version you are using and your specific requirements.
Whether you're working with Python 2.x or 3.x, understanding how to print without newline in Python empowers you to format output efficiently and effectively. The various ways to print without a newline in Python include using the end argument, custom functions, or compatibility features; you can control the formatting of your printed output to suit your needs. Remember to choose the method that best fits your context and coding style, ensuring clear and readable output in your Python scripts and applications.
Frequently Asked Questions
Q. How do you print output in Python?
We generally use the print() function to display the output in Python. The print() function takes the value or message as an argument and prints it on the output screen. It converts the given argument (here integer) to a string and displays it on the output console.
Syntax:
print(message)
Code Example:
Output:
Hello world 5
Explanation:
- In this program, we first initialized a variable x with an integer value 5.
- Then, we executed the print() function by passing the string “Hello World” and variable x as arguments.
- The print() function converts x into a string, and the other argument is already a string.
- In the end, the output is printed as “Hello world 5”, where Hello world is the string, and 5 is the value of x.
Q. What is \r in Python?
The \r escape sequence in Python stands for carriage return. It helps to move the cursor to the beginning of the line without moving it to a new line. Whenever \r is encountered in the print() function, the cursor goes back to the start of the line and prints the next character from there.
Code Example:
#Print string with carriage return
print("Hello everyone. It's a good \rday")
Output:
daylo everyone. It’s a good
Explanation:
- In this print() function, we pass a string containing \r escape sequence as an argument.
- The string is printed from the beginning.
- When the escape sequence \r is encountered, it shifts the cursor to the beginning of the line.
- The rest of the string is then printed by erasing the previously printed characters.
- As ‘day’ contains 3 letters, the first 3 letters of the previously printed string are erased and ‘day’ is printed at that place.
- As a result, the output is printed as ‘daylo everyone. It’s a good’.
Q. What is \n used for in Python?
The expression (\n) in Python stands for newline character. It is a type of escape sequence character that moves the cursor to the next line. Whenever \n is encountered in code, the cursor is moved to the new line.
Code Example:
#Print a string containing newline character
print("It is a \nsunny day!")
Output:
It is a
sunny day!
Explanation:
- In the print() function, we pass a string with \n in between the characters as an argument.
- When the print() function is executed, the set of characters before \n is printed.
- After encountering \n in the string, the cursor moves to the next line, and the remaining characters are printed on that line.
- Therefore, ‘sunny day!’ is printed in the next line.
Q. How do you remove a new line character from a list in Python?
Removing a new line character from a list in Python is similar to printing without a new line in Python. The steps are as follows:
- Iterate over the list using the list comprehension.
- Then, use the strip() method to remove the leading and trailing newline characters from each string in the list.
- Print the updated list without newline characters.
Code Example:
Output:
['lion\n', '\nbear\r\n', '\nelephant', 'fox\r\n']
['lion', 'bear', 'elephant', 'fox']
Explanation:
- In this program, we initialized a list called animals with four strings as list items.
- All the items in the list contain newline characters.
- Then, the list is printed as it is in its original form.
- After that, iterate over the list using list comprehension and use the strip() method inside it to remove the heading and trailing newline characters from each item as it iterates over it.
- Print the updated list without the new line characters.
Q. How do you print a single line in Python?
If you know how to print without newline in Python, then you'd find that it is similar to printing a single line. In order to implement this, we should pass an empty string as the argument to the end parameter of the print() function. When the print() function is used to print a single line, it prints the line and moves the cursor to the next line by default. To avoid the cursor moving to the next line, the end parameter of the print() function is given an empty string as its value to ensure that the cursor stays in the same line.
Code Example:
Output:
How to print without newline in Python.
Explanation:
- In this program, the print() function is executed with the “How to print without newline in Python.” string as input.
- The end parameter of the print() function is assigned an empty string in order to stop the cursor from moving to the next line.
- Therefore, “How to print without newline in Python.” is printed and the cursor remains in the same line.
Q. How do I skip a line in Python using \n?
To skip a line in Python using the newline escape sequence (\n), we can pass double \n as an argument to the end parameter of the print() function. In this method, a line is skipped while printing in Python. The double \n ensures that the cursor moves to the next line two times, which results in skipping one line.
Code Snippet:
Output:
Hello Everyone!
How to print without newline in Python.
Explanation:
- In this program, a print() function is called by passing a string as input and setting the end parameter to double \n.
- Then, this print() function prints the string “Hello Everyone!” and moves the cursor to the next line two times due to the end parameter value.
- After that, another print() function is executed, which takes the string “How to print without newline in Python.” as input and prints it on the output screen.
Q. What does the strip() function do in Python?
The strip() function in Python removes any heading or trailing whitespaces or newline character from a string. It can also remove any other heading or trailing character from the string if specified. It is useful for updating the string.
Syntax:
string.strip(character)
Here,
- string: The string from which whitespaces are to be removed.
- strip(): Function to remove characters or whitespaces.
- character: Specific characters to be removed from the beginning and ending of a string.
Code Example:
Output:
Hii there!
Hii there!
Explanation:
- In this program, a variable s is assigned a string with heading and trailing newline characters and spaces.
- Then, the original string is printed as output with all the new line characters.
- After that, the strip() method is called upon s which removes all the heading and trailing new line characters and stores the modified string in variable s_new.
- Then, the s_new string is printed as output using the lines print command. The example shows how to print without a newline in Python, using the strip() function.
You must also read through the following:
- How To Reverse A String In Python? 10 Easy Ways With Examples!
- Python IDLE | The Ultimate Beginner's Guide With Images & Codes!
- How To Convert Python List To String? 8 Ways Explained (+Examples)
- Python Logical Operators, Short-Circuiting & More (With Examples)
- Python Bitwise Operators | Positive & Negative Numbers (+Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment