Table of content:
- Understanding The Continue Statement In Python
- How Does Continue Statement Work In Python?
- Python Continue Statement With For Loops
- Python Continue Statement With While Loops
- Python Continue Statement With Nested Loops
- Python Continue With If-Else Statement
- Difference Between Pass and Continue Statement In Python
- Practical Applications Of Continue Statement In Python
- Conclusion
- Frequently Asked Questions
Continue Statement In Python | Working, Applications & Examples
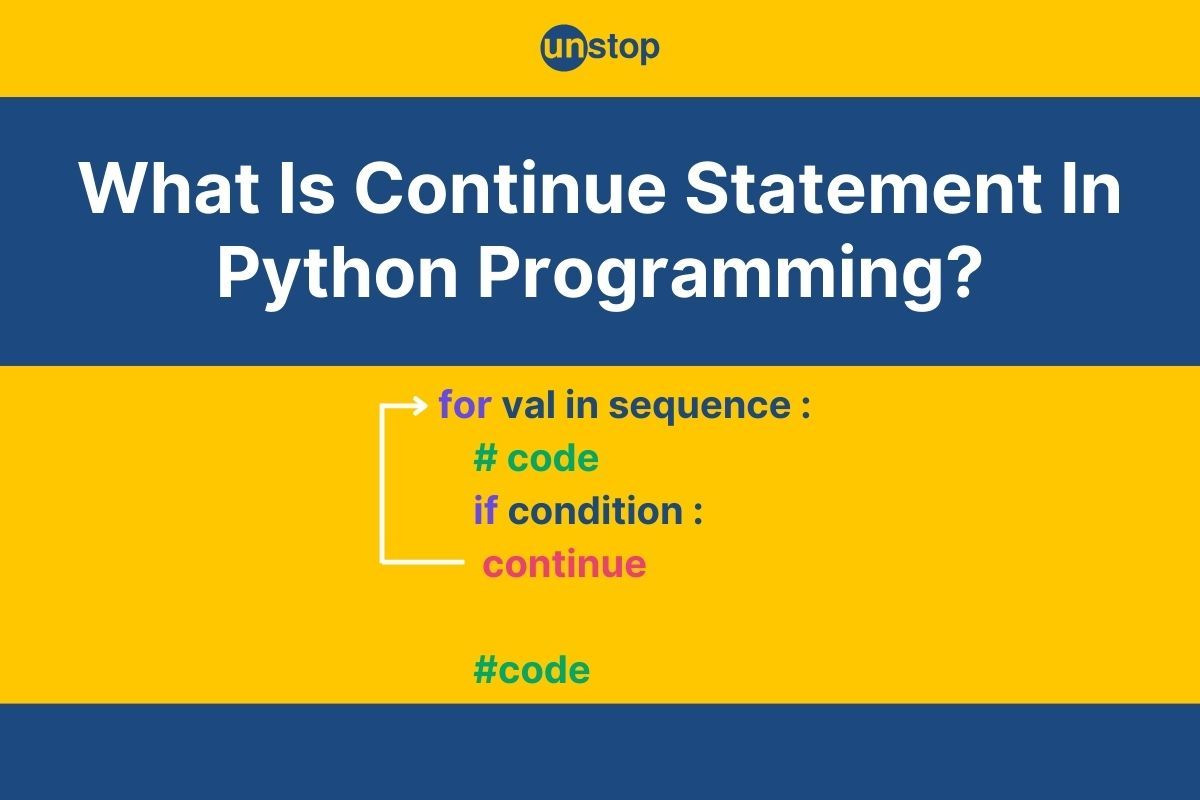
The continue statement in Python is used to alter the flow of execution in loops, allowing us to skip the remaining code inside the current iteration and proceed to the next iteration of the loop. This can be particularly useful when we want to bypass certain conditions or situations without completely breaking out of the loop.
In this article, we will explore how the continue statement works, its syntax, and practical examples to illustrate its use in real-world programming scenarios. By the end, you'll have a clear understanding of when and how to effectively use continue in your Python projects.
Understanding The Continue Statement In Python
The continue statement in Python programming is used inside loops to skip the current iteration and proceed directly to the next iteration of the loop. When the continue statement is encountered, the remaining code in that iteration is ignored, and the loop moves on to the next cycle, checking the loop condition again. This can be particularly helpful when you want to bypass specific conditions without terminating the loop completely.
The continue statement is most commonly used in for and while loops to improve control flow and avoid unnecessary computations.
Syntax Of Continue Statement In Python
continue
This is the basic form of the continue statement. It's used without any conditions, but it can be part of a more complex condition within the loop.
How Does Continue Statement Work In Python?
The continue statement in Python works by altering the flow of control within a loop, causing it to skip the remaining code in the current iteration and move directly to the next iteration. Here's how it works step by step:
- Start Loop Iteration: The loop begins executing from the top, checking the loop condition (whether it's a for or while loop). If the condition is True, the loop continues its execution.
- Evaluate the Condition for continue: Inside the loop, the program encounters a condition that may trigger the continue statement. This is typically wrapped in an if condition, but it can be used without any conditions as well.
- Execute continue: When Python reaches the continue statement inside the loop, it immediately skips all the remaining code in the current iteration (after the continue statement) and jumps to the next iteration of the loop.
- Move to the Next Iteration: After skipping the remaining code, the loop condition is re-evaluated (in the case of a while loop) or moves to the next item (in the case of a for loop). The loop will continue running until the loop condition becomes False.
Pseudocode For Continue Statement In Python
Given below is a simple pseudo code to demonstrate how the continue statement works within a loop:
Rk9SIGVhY2ggaXRlbSBpbiB0aGUgbGlzdDoKICAgIElGIGl0ZW0gaXMgZXF1YWwgdG8gYSBjZXJ0YWluIHZhbHVlOgogICAgICAgIENPTlRJTlVFIHRvIG5leHQgaXRlcmF0aW9uICAjIFNraXAgdGhlIGN1cnJlbnQgaXRlcmF0aW9uCiAgICBQUklOVCB0aGUgaXRlbSAgIyBFeGVjdXRlIHRoaXMgb25seSBpZiBjb250aW51ZSB3YXMgbm90IHRyaWdnZXJlZApFTkQgRk9SCg==
Python Continue Statement With For Loops
The continue statement is used to skip over the current iteration of the loop and move on to the next one. In a for loop, this helps when we want to ignore certain values or conditions while iterating over a sequence.
- The loop iterates over a sequence of elements.
- When a specific condition is met, the continue statement is executed, skipping the remaining code in the current iteration and moving to the next item in the sequence.
Code Example:
IyBVc2luZyBjb250aW51ZSBpbiBhIGZvciBsb29wCmZvciBpIGluIHJhbmdlKDEsIDYpOgogICAgaWYgaSA9PSAzOgogICAgICAgIGNvbnRpbnVlICAjIFNraXAgd2hlbiBpIGVxdWFscyAzCiAgICBwcmludChpKQo=
Output:
1
2
4
5
Explanation:
In the above code example-
- We begin by using a for loop that iterates through numbers in the range from 1 to 5, inclusive.
- Inside the loop, we check if the value of i equals 3.
- When i is 3, the continue statement is executed. This causes the program to skip the current iteration and move on to the next value of i without executing the print(i) statement for that iteration.
- For all other values of i, the print(i) statement is executed, printing the value of i to the console.
- The output of this code will be the numbers 1, 2, 4, and 5, with 3 being skipped due to the continue statement.
Explore this amazing course and master all the key concepts of Python programming effortlessly!
Python Continue Statement With While Loops
The continue statement in a while loop allows you to skip the rest of the current iteration and jump to the next iteration by re-evaluating the loop condition.
- The loop continues to execute as long as the condition is True.
- When the continue statement is encountered, the rest of the code inside the loop is skipped, and the loop condition is checked again.
Code Example:
IyBVc2luZyBjb250aW51ZSBpbiBhIHdoaWxlIGxvb3AKaSA9IDAKCndoaWxlIGkgPCA1OgogICAgaSArPSAxCiAgICBpZiBpID09IDM6CiAgICAgICAgY29udGludWUgICMgU2tpcCB3aGVuIGkgZXF1YWxzIDMKICAgIHByaW50KGkpCg==
Output:
1
2
4
5
Explanation:
In the above code example-
- We start by initializing the variable i to 0.
- Next, we use a while loop that runs as long as i is less than 5.
- Inside the loop, we increment i by 1 at the beginning of each iteration.
- We then check if i equals 3. When i is 3, the continue statement is triggered, causing the loop to skip the rest of the code inside the loop for that iteration and move to the next cycle.
- For all other values of i, the print(i) statement is executed, printing the current value of i.
- The output of this code will be the numbers 1, 2, 4, and 5, with 3 being skipped due to the continue statement.
Python Continue Statement With Nested Loops
In nested loops, the continue statement affects only the innermost loop. It skips the remaining code in that loop and continues with the next iteration of that loop. To skip outer loops, you would need additional control structures, but for inner loops, continue works similarly to how it works in a single loop.
- The continue statement is used in the inner loop to skip the current iteration and proceed to the next iteration of the inner loop.
- It doesn't affect the outer loop directly.
Code Example:
IyBVc2luZyBjb250aW51ZSB3aXRoIG5lc3RlZCBsb29wcwpmb3IgaSBpbiByYW5nZSgxLCA0KToKICAgIGZvciBqIGluIHJhbmdlKDEsIDQpOgogICAgICAgIGlmIGogPT0gMjoKICAgICAgICAgICAgY29udGludWUgICMgU2tpcCB3aGVuIGogZXF1YWxzIDIKICAgICAgICBwcmludChmImkgPSB7aX0sIGogPSB7an0iKQo=
Output:
i = 1, j = 1
i = 1, j = 3
i = 2, j = 1
i = 2, j = 3
i = 3, j = 1
i = 3, j = 3
Explanation:
In the above code example-
- We start by using an outer for loop that iterates through the values 1, 2, and 3 for the variable i.
- Inside this outer loop, there is a nested for loop that iterates through the values 1, 2, and 3 for the variable j.
- We then check if j equals 2. When j is 2, the continue statement is executed, causing the program to skip the remaining code inside the inner loop and move to the next iteration of j.
- For all other values of j, the print() statement is executed, printing the current values of i and j to the console.
- As a result, for each value of i, the output will show the pairs (i, j) for j values of 1 and 3, but not for j = 2.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Python Continue With If-Else Statement
The continue in Python can be used in conjunction with an if-else statement to control the flow of the loop based on specific conditions. The continue statement allows you to skip the remaining part of the current iteration when a certain condition is met, while the else part can handle other conditions that need to be executed.
- In a loop, the if condition checks for specific criteria.
- If the condition is met, the continue statement is executed, skipping the rest of the loop's body for that iteration.
- If the condition isn't met, the else block (or other code after the if block) is executed, ensuring the loop continues processing normally.
Code Example:
IyBVc2luZyBjb250aW51ZSB3aXRoIGlmLWVsc2Ugc3RhdGVtZW50IGluIGEgbG9vcApmb3IgaSBpbiByYW5nZSgxLCA2KToKICAgIGlmIGkgPT0gMzoKICAgICAgICBjb250aW51ZSAgIyBTa2lwIGl0ZXJhdGlvbiB3aGVuIGkgaXMgMwogICAgZWxzZToKICAgICAgICBwcmludChmImkgaXMgbm90IDMsIGkgPSB7aX0iKQo=
Output:
i is not 3, i = 1
i is not 3, i = 2
i is not 3, i = 4
i is not 3, i = 5
Explanation:
In the above code example-
- We start with a for loop that iterates through the values 1 to 5 for the variable i.
- Inside the loop, we check if i equals 3.
- When i is 3, the continue statement is triggered, causing the loop to skip the remaining code inside the loop and move to the next iteration without printing anything for that iteration.
- If i is not 3, the else block is executed, and it prints the message i is not 3, with the current value of i.
- The output will show the message for all values of i except 3.
Difference Between Pass and Continue Statement In Python
The pass statement in Python is a placeholder that does nothing when executed. Here is a comprehensive table comparing the pass and continue statements in Python:
Aspect |
The pass statement |
The continue statement |
---|---|---|
Purpose |
The pass statement is a no-op; it does nothing and allows the code to continue executing. |
The continue statement skips the rest of the current loop iteration and proceeds to the next iteration. |
Effect on Loop |
No effect on the loop execution; it just acts as a placeholder. |
Skips the current iteration of the loop and continues with the next iteration. |
Usage |
Used when a statement is syntactically required but no action is needed (e.g., empty function, class, loop). |
Used when you want to skip over a specific condition within a loop but still keep the loop running. |
Type of Statement |
A null statement; used as a placeholder. |
A control flow statement; alters the loop's flow. |
Can It Be Used in Functions or Classes? |
Yes, often used in defining empty functions or classes. |
No, is used only inside loops and cannot be used in functions or classes directly. |
Control Flow |
Does not alter the flow of execution. |
Alters the control flow by skipping to the next iteration of the loop. |
Example Use Case |
When defining a function or class without implementing it yet. |
When you want to skip processing certain items in a loop (e.g., skip even numbers in a loop). |
Loop Execution |
The loop continues to execute as normal. |
The loop jumps to the next iteration, skipping the remaining code inside the loop for the current iteration. |
Also Read: Break And Continue Statement In Python | Working, Uses & Examples
Practical Applications Of Continue Statement In Python
The continue statement in Python is useful for skipping over certain iterations in loops based on specific conditions. Here are some practical applications where the continue statement is commonly used:
-
Skipping Even Numbers in a Loop: When we want to process only odd numbers or skip even numbers in a sequence, the continue statement can help by skipping over the even numbers during iteration.
# Skipping even numbers in a range
for i in range(1, 11):
if i % 2 == 0:
continue # Skip even numbers
print(i)
-
Skipping Invalid User Inputs: In programs that process user inputs, you might want to skip invalid or unwanted inputs and only process valid data. The continue statement helps avoid unnecessary steps for invalid inputs.
# Skipping invalid user inputs
for i in range(5):
user_input = input("Enter a number greater than 10: ")
if not user_input.isdigit() or int(user_input) <= 10:
print("Invalid input. Try again.")
continue # Skip the rest of the loop for invalid input
print(f"Valid input: {user_input}")
-
Skipping Empty Strings in Data Processing: When processing data (like in a list or file), you might want to skip empty strings, lines, or other null values to avoid processing invalid or incomplete data.
# Skipping empty strings in a list
data = ["apple", "", "banana", " ", "cherry", " ", "date"]
for item in data:
if item.strip() == "": # Skip empty or whitespace strings
continue
print(f"Processing: {item}")
-
Skipping Over Specific Items in Complex Loops: In a loop with multiple conditions, you may want to skip specific items under certain circumstances. For example, when processing a list of numbers, you can skip specific values based on certain criteria.
# Skipping specific items in a list
numbers = [1, 3, 7, 9, 10, 12]
for num in numbers:
if num == 7 or num == 9: # Skip 7 and 9
continue
print(f"Processing number: {num}")
-
Skipping Unwanted Characters in String Processing: In string manipulation tasks, you may need to skip over certain characters (e.g., whitespace, punctuation, or specific letters) to perform actions on the remaining characters.
# Skipping unwanted characters in a string
sentence = "Hello, World! Python is awesome."
for char in sentence:
if char in ",.!":
continue # Skip punctuation marks
print(char, end="")
-
Handling Errors in File Processing: When working with files, you might want to skip lines that don’t meet certain criteria (e.g., empty lines, lines with errors) while continuing to process the rest of the file.
# Skipping empty lines in a file processing task
lines = ["First line", "", "Second line", " ", "Third line"]
for line in lines:
if line.strip() == "":
continue # Skip empty or whitespace-only lines
print(f"Processing: {line}")
-
Skipping Items Based on Multiple Conditions: In cases where there are multiple conditions, the continue statement can be used to skip iterations that don’t meet any of the conditions. For example, when processing a list of mixed data types, you can skip non-numeric types.
# Skipping non-numeric types in a list
data = [1, "apple", 3.14, None, 42, "banana"]
for item in data:
if not isinstance(item, (int, float)): # Skip non-numeric items
continue
print(f"Processing numeric item: {item}")
Thus, by using the continue statement in Python, you can write cleaner and more efficient code in situations where specific conditions should be bypassed without exiting the entire loop.
Searching for someone to answer your programming-related queries? Find the perfect mentor here.
Conclusion
The continue statement in Python is a handy tool that lets us skip certain parts of a loop and jump straight to the next round of repeating the loop. By using continue, we can avoid executing specific steps in a loop when we don’t need them—like skipping over unwanted numbers or skipping actions when a condition isn’t met.
Learning how to use continue helps make our code shorter and easier to read. Whether in a for loop or while loop, continue lets us control exactly how each loop behaves, making our Python programs more efficient and flexible.
Frequently Asked Questions
Q. What is the purpose of the continue statement in Python?
The continue statement is used in loops to skip the rest of the code inside the current iteration and move to the next iteration of the loop. It is often used when certain conditions are met, and you don't want to execute the remaining part of the loop for that particular iteration.
Q. Can I use the continue statement outside of loops?
No, the continue statement is specifically designed for use inside loops (for and while). It will result in a syntax error if used outside a loop, such as in functions or conditionals.
Q. How does the continue statement affect the flow of a loop?
When the continue statement is encountered in a loop, it skips the current iteration and immediately moves to the next iteration. This allows you to avoid executing certain parts of the loop when specific conditions are met.
Q. Can I use continue with multiple conditions?
Yes, you can use the continue statement with multiple conditions in Python. The continue statement can be placed within any conditional expression (like an if or elif statement) inside the loop, allowing you to skip the current iteration when any one (or combination) of those conditions is met.
Code Example:
IyBTa2lwcGluZyBldmVuIG51bWJlcnMgYW5kIG51bWJlcnMgZGl2aXNpYmxlIGJ5IDMKZm9yIGkgaW4gcmFuZ2UoMSwgMTEpOgogICAgaWYgaSAlIDIgPT0gMCBvciBpICUgMyA9PSAwOiAgIyBTa2lwIGlmIHRoZSBudW1iZXIgaXMgZXZlbiBvciBkaXZpc2libGUgYnkgMwogICAgICAgIGNvbnRpbnVlCiAgICBwcmludChpKQo=
Output:
1
5
7
Explanation:
In this example:
- The loop iterates over the range from 1 to 10.
- If a number is either even (i % 2 == 0) or divisible by 3 (i % 3 == 0), the continue statement is triggered.
- This skips the current iteration for such numbers and prints only those that do not meet the conditions (1, 5, and 7).
Q. How is the continue statement different from the break statement?
While both continue and break alter the flow of a loop, they work differently:
- The continue statement skips the current iteration and moves to the next iteration of the loop.
- The break statement completely exits the loop, terminating it entirely.
Q. Can I use continue with a for loop and a while loop?
Yes, the continue statement can be used in both for and while loops. In a for loop, it skips to the next item in the iteration, while in a while loop, it moves back to the loop condition and checks whether the loop should continue.
With this, we conclude our discussion on the Python continue statement. Here are a few other topics that you might be interested in reading:
- Hello, World! Program In Python | 7 Easy Methods (With Examples)
- Calculator Program In Python - Explained With Code Examples
- Swap Two Variables In Python- Different Ways | Codes + Explanation
- Python Logical Operators, Short-Circuiting & More (With Examples)
- Random Number Generator Python Program (16 Ways + Code Examples)
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment