Table of content:
- What Is A Python For Loop?
- How Does Python For Loop Work?
- When & Why To Use Python For Loops?
- Python For Loop Examples
- What Is Rrange() Function In Python?
- Nested For Loops In Python
- Python For Loop With Continue & Break Statements
- Python For Loop With Pass Statement
- Else Statement In Python For Loop
- Conclusion
- Frequently Asked Questions
Python For Loop | Syntax & Application (With Multiple Examples)
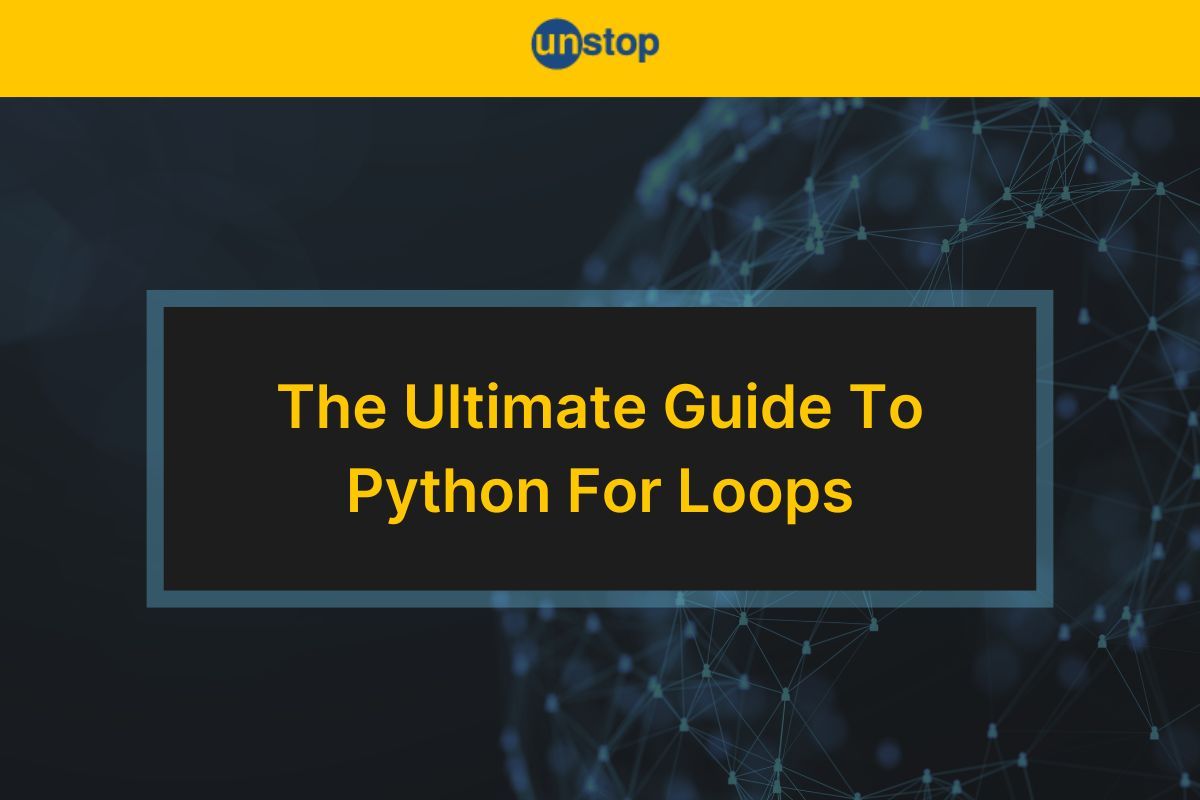
Python is a dynamic programming language renowned for its simplicity and versatility. It offers a plethora of tools and constructs to facilitate efficient programming. Among these, the for loop stands out as one of the fundamental programming constructs used for iteration of code. In this comprehensive guide, we'll delve into the intricacies of the Python for loop, its syntax, workflow, best practices, and potential pitfalls.
What Is A Python For Loop?
In the Python language, a for loop is a control flow statement used for iterating over a sequence of elements, i.e., carrying out sequential traversals with ease. It allows you to execute a block of code repeatedly for each item in an iterable object, such as lists, tuples, strings, dictionaries, or any other iterable data structure. The for loop simplifies the process of performing repetitive tasks by automating the iteration process.
Python For Loop Syntax
for item in iterable:
# Code block
Here,
- item: This is a variable that represents the current element being iterated over in each iteration of the loop.
- iterable: This is the sequence of elements over which the loop iterates. It can be any iterable object that contains multiple items.
Let's take a look at a basic Python example showcasing the functioning of the Python for loop, where we iterate through a string (sequence of characters) variable.
Code Example:
IyBEZWZpbmUgYSBzdHJpbmcKbXlfc3RyaW5nID0gIlVOU1RPUCIKCiMgSXRlcmF0ZSB0aHJvdWdoIGVhY2ggY2hhcmFjdGVyIGluIHRoZSBzdHJpbmcKZm9yIGNoYXIgaW4gbXlfc3RyaW5nOgogIHByaW50KGNoYXIp
Output:
U
N
S
T
O
P
Explanation:
In the simple Python program-
- We define a string variable named my_string and initialize it with the value- "UNSTOP" using the double quotes method.
- Then, we run a for loop that iterates through each character in the string my_string.
- During each iteration, the loop prints the current character to the console using the print() function.
- The loop terminates when all the characters in the string have been iterated over.
How Does Python For Loop Work?
As mentioned before, the Python for loop is a control flow statement used for iterating over a sequence of elements. Look at the loop flowchart below, followed by the explanation to understand its working mechanism.
Step 1- Initialization: The for loop initializes by iterating over a given iterable object, which could be a list, tuple, string, dictionary, or any other iterable data structure.
Step 2- Iteration: During each iteration, the loop variable takes on the current element's value in the iterable sequence.
Step 3- Execution of Body: Within the loop body, statements or code blocks are executed for each iteration. This allows us to perform operations, calculations, or manipulations on the current element.
Step 4- Control Flow: If other control flow statements like break and continue exist inside the loop body, they can alter the behaviour of the loop control statement as a whole. For example, break will lead the loop to terminate prematurely and continue can cause the program to skip the current iteration and proceed to the next one.
Step 5- Incrementing: After executing the loop body for each element in the iterable object, the loop variable automatically moves to the next element in the sequence, continuing until all elements have been processed.
Step 6- Completion: Once all elements in the iterable have been iterated over and the loop body executed for each, the loop terminates automatically.
Step 7- Exit: The program exits the loop after the last iteration or upon encountering a break statement. The program's execution continues with the statement immediately following the loop.
Below is another example of the Python for loop, where we iterate using the index value of the elements in a sequence.
Code Example:
IyBEZWZpbmUgYSBsaXN0Cm15X2xpc3QgPSBbJ0pvYnMnLCAnTGVhcm4nLCAnUHJhY3RpY2UnLCAnVXBza2lsbCddCgojIEl0ZXJhdGUgb3ZlciB0aGUgbGlzdCB1c2luZyBpbmRleApmb3IgaSBpbiByYW5nZShsZW4obXlfbGlzdCkpOgogIHByaW50KCJJbmRleDoiLCBpLCAiVmFsdWU6IiwgbXlfbGlzdFtpXSk=
Output:
Index: 0 Value: Jobs
Index: 1 Value: Learn
Index: 2 Value: Practice
Index: 3 Value: Upskill
Explanation:
In the sample Python program-
- We first define a list variable named my_list containing four elements, i.e., Jobs, Learn, Practice, and Upskill.
- Then, we execute a for loop that iterates over the indices of the list using the range() function and len() function.
- Here, the len() function provides the number of elements in the list and the range() function generates the indices from 0 to the length of the list minus one.
- In every iteration, the loop prints the index number of the respective element and the corresponding value in the list using the current index and print() function.
- The loop terminates after all the indices of the list have been iterated over.
Also read: Python IDLE | The Ultimate Beginner's Guide With Images & Codes!
When & Why To Use Python For Loops?
The for loop is a versatile construct suitable for many iteration tasks, from simple iterations over collections to complex nested loops and pattern generation. We already know how to use for loop in Python from the sections above. Here, we will discuss when and why to use Python for loops.
- Iterating Over Collections: The most common use of for loops is to iterate over collections like lists, tuples, dictionaries, etc., accessing each element individually.
- Sequence Generation: We should employ for loops with functions like range() when we want to generate sequences of numbers. This is because Python for loops are efficient in executing a block of code a specific number of times without the need to write the code again and again.
- Processing Data: When you need to process data, such as read lines from a file or parse data from external sources, using the Python for loop can facilitate the execution of operations on each piece of data.
- Multidimensional Data: We can make use of nested for loops in Python to iterate over multidimensional data structures or perform complex iterations. These loops facilitate tasks like matrix operations or pattern generation.
- Enhanced Readability: Python for loops enhances code readability by clearly indicating the intent to iterate over a sequence of elements, making the code easier to understand and maintain.
- Iteration with Indices: It is beneficial to use Python for loops with the range() function when you need to iterate over a sequence while also accessing the index of each element. This makes it possible to perform operations based on both the value and position of elements.
- Iterating Until a Condition is Met: In scenarios where you need to iterate until a certain condition is met, you can use a Python for loop in combination with conditional statements (if) to check the condition at each iteration and terminate the loop accordingly.
- Looping Through Enumerated Items: When iterating over a sequence and needing both the index and value of each item, you can use the enumerate() function within a for loop to retrieve both the index and value simultaneously, enhancing code clarity and efficiency.
- Iterating Over Iterators and Generators: The Python for loops are compatible with iterators and generators, allowing seamless iteration over potentially infinite sequences or lazy-loaded data without consuming excessive memory.
- Repeated Execution of Code Blocks: Use for loops when you need to execute a block of code repeatedly for each item in a sequence, eliminating the need for redundant code and promoting code reusability.
Python For Loop Examples
The Python for loop is the go-to tool for many programmers when they want to iterate over the elements of a sequence, access them and perform various operations on them. In this section, we will cover multiple scenarios where we can use these loops with the help of properly explained examples.
Iterating Over A List With Python For Loop
A list in Python is an ordered collection of elements, where each element can be of any data type. Using a for loop, we can iterate over each element in the list, performing operations or transformations as needed. This method is effective for processing sequential data stored in a list format.
Code Example:
IyBEZWZpbmUgYSBsaXN0ClVuc3RvcHBhYmxlID0gWydDb21wZXRpdGlvbnMnLCAnQ291cnNlcycsICdNZW50b3JzJ10KCiMgSXRlcmF0ZSBvdmVyIHRoZSBsaXN0CmZvciB1bnN0b3AgaW4gVW5zdG9wcGFibGU6CiAgcHJpbnQodW5zdG9wKQ==
Output:
Competitions
Courses
Mentors
Explanation:
In the example Python program-
- We first create a list variable called Unstoppable and initialize it with three elements: Competitions, Courses, and Mentors.
- Next, we initiate a for loop to iterate over each element of the list.
- For each iteration, the loop control variable unstop takes on the value of the current list item and prints it to the console using the print() function.
Iterating Over A Tuple Using Python For Loop
A tuple is an ordered collection of elements similar to a list, but tuples are immutable, meaning their elements cannot be modified after creation. By utilizing a for loop, we can iterate over each item in the tuple, accessing and processing individual elements. This approach is beneficial for handling immutable sequences of data.
Code Example:
IyBEZWZpbmUgYSB0dXBsZQpudW1iZXJzID0gKDEsIDIsIDMsIDQsIDUpCgojIEl0ZXJhdGUgb3ZlciB0aGUgdHVwbGUKZm9yIG51bSBpbiBudW1iZXJzOgogIHByaW50KG51bSk=
Output:
1
2
3
4
5
Explanation:
In the Python program example-
- We create a tuple called numbers containing five elements: 1, 2, 3, 4, and 5.
- Then, we use a for loop to iterate over each item in the numbers tuple.
- In every iteration, the loop variable num takes on the value of the current number.
- Inside the loop, we print each number using the print() function.
Iterating Over A Set Using Python For Loop
Sets in Python are unordered collections of unique elements, making them ideal for storing distinct values. We can iterate over each unique element in the set using the Python for loop, facilitating operations on unique values without duplication. This method is useful for handling sets of data where uniqueness is crucial.
Code Example:
IyBEZWZpbmUgYSBzZXQKY29sb3JzID0geydyZWQnLCAnZ3JlZW4nLCAnYmx1ZSd9CgojIEl0ZXJhdGUgb3ZlciB0aGUgc2V0CmZvciBjb2xvciBpbiBjb2xvcnM6CiAgcHJpbnQoY29sb3Ip
Output:
green
red
blue
Explanation:
In the example Python code-
- We have a set called colors, which contains three unique elements, i.e., 'red', 'green', and 'blue'.
- Next, we use a for loop to iterate over each item in the colors set.
- Since sets are unordered, the order of elements during iteration may vary.
- For each iteration, the loop variable color takes on the value of the current color.
- Inside the loop, we use the print() function to display each color/ element in the set.
Iterating Over A Dictionary Using Python For Loop
A dictionary in Python is an unordered collection of key-value pairs. Each key in a dictionary must be unique and immutable, and values can be of any data type. This technique is essential for processing dictionary data and performing tasks based on key-value relationships. Look at the example below to see how you can travese the dictionary using a for loop in Python.
Code Example:
IyBEZWZpbmUgYSBkaWN0aW9uYXJ5CnBlcnNvbiA9IHsnbmFtZSc6ICdBa2FzaCcsICdhZ2UnOiAzMCwgJ2NpdHknOiAnTmV3IERlbGhpJ30KCiMgSXRlcmF0ZSBvdmVyIHRoZSBkaWN0aW9uYXJ5CmZvciBrZXksIHZhbHVlIGluIHBlcnNvbi5pdGVtcygpOgogIHByaW50KGtleSwgIjoiLCB2YWx1ZSk=
Output:
name : Akash
age : 30
city : New Delhi
Explanation:
In the Python code example-
- We have a dictionary called person containing three key-value pairs, i.e., 'name': 'John', 'age': 30, and 'city': 'New York'.
- The for loop we create next iterates over each key-value pair in the person dictionary using the built-in function items(). This function returns a view object.
- For each iteration, the loop variables key and value take on the key and corresponding value of each pair, respectively.
- Inside the loop, we print each key-value pair in the format <key> : <value>.
Python For Loop & Zip() Function
The built-in Python method zip() combines corresponding elements from multiple iterables into a single iterable, say tuples. Utilizing a Python for loop with zip(), we can simultaneously iterate over paired data from different sequences, facilitating parallel processing of related information. This method is valuable for scenarios requiring coordinated handling of data from multiple sources.
Code Example:
IyBEZWZpbmUgbGlzdHMKbmFtZXMgPSBbJ0Fyc2hpJywgJ01pc2hrYScsICdTaGl2YW5pJ10KYWdlcyA9IFsyNSwgMzAsIDM0XQoKIyBJdGVyYXRlIG92ZXIgemlwcGVkIGxpc3RzCmZvciBuYW1lLCBhZ2UgaW4gemlwKG5hbWVzLCBhZ2VzKToKICBwcmludChuYW1lLCAiaXMiLCBhZ2UsICJ5ZWFycyBvbGQiKQ==
Output:
Arshi is 25 years old
Mishka is 30 years old
Shivani is 34 years old
Explanation:
In the sample Python code-
- We have two lists, names and ages, containing names and ages, respectively.
- The zip() function combines corresponding elements of both lists into tuples.
- Next, we use a for loop to iterate over each tuple, with variables name and age representing name and age pairs.
- Inside the loop, we print each name and age combination.
Python For Loop With A Step Size
The range() function generates a sequence of numbers with a specified step size. By incorporating this in the Python for loop, we can iterate over the generated sequence, skipping elements at defined intervals. This approach is helpful for situations where iterating with a fixed step size is necessary, such as processing data in specific increments.
Code Example:
IyBJdGVyYXRlIGZyb20gMCB0byAxMCB3aXRoIHN0ZXAgc2l6ZSAyCmZvciBpIGluIHJhbmdlKDAsIDEwLCAyKToKICBwcmludChpKQ==
Output:
0
2
4
6
8
Explanation:
In the Python code sample-
- We use the range() function to generate a sequence of numbers from 0 to 10 (exclusive) with a step size of 2.
- The for loop iterates over each number in the generated sequence.
- For each iteration, the loop variable i corresponds to the current number in the range.
- Inside the loop, we print each number.
What Is Rrange() Function In Python?
The range function in Python is used to generate a sequence of numbers within a specified range. It allows you to create sequences of integers with a starting value, ending value, and an optional step size/ step parameter. It's a versatile tool for generating integer sequences efficiently in Python.
Basic Syntax For Range Function In Python:
range(start, stop, step)
Here,
- The term start refers to the indices/ starting point of the sequence. This is optional and defaults to 0 if not provided explicitly.
- Similarly, step refers to the step size/ increment between each number in the sequence. If not provided, it defaults to 1.
- The stop term refers to the ending value of the sequence.
Usage Of Range() Function In Python For Loop
The range() function is commonly used in conjunction with for loops in Python programs to iterate over a sequence of numbers.
- The range() function takes one, two, or three arguments, i.e., the starting value (inclusive), the ending value (exclusive), and an optional step size.
- When used in a for loop, the range() function generates a sequence of numbers based on these arguments, and the loop iterates over each value in the sequence.
Code Example:
IyBVc2luZyByYW5nZSgpIGZ1bmN0aW9uIGluIGEgZm9yIGxvb3AKZm9yIGkgaW4gcmFuZ2UoMSwgNik6CiAgcHJpbnQoaSk=
Output:
1
2
3
4
5
Explanation:
In the Python code-
- We use the range() function to generate a sequence of integers starting from 1 and ending at 5.
- Here, the function takes start and stop parameters as 1 and 6 (exclusive) and skips the optional parameter step size.
- The function is part of a for loop which iterates over each number generated by range(1, 6).
- During each iteration, the loop variable i corresponds to the current value of the sequence/ range.
- Inside the loop, we print each value of i, resulting in numbers from 1 to 5 being printed sequentially.
Nested For Loops In Python
Nested for loops in Python refer to a situation where one for loop is placed inside another. Using such nested loops allows us to iterate over multiple sequences or perform complex iterations with each. They are extremely useful in multiple cases, such as for iterating over multidimensional data structures like matrices or lists of lists.
Syntax Of Nested For Loop In Python:
for outer_item in outer_iterable:
for inner_item in inner_iterable:
# Code block
Here,
- outer_item: Variable representing the current item in the outer iterable object.
- outer_iterable: The outer sequence over which the outer loop (parent loop) iterates.
- inner_item: Variable representing the current item in the inner iterable.
- inner_iterable: The inner sequence over which the inner loop (child loop) iterates.
- Code block: Block of code to be executed for each combination of outer and inner items.
Code Example:
IyBOZXN0ZWQgZm9yIGxvb3BzIGV4YW1wbGUKZm9yIGkgaW4gcmFuZ2UoMyk6CiAgZm9yIGogaW4gcmFuZ2UoMik6CiAgICBwcmludChmIih7aX0sIHtqfSkiKQ==
Output:
(0, 0)
(0, 1)
(1, 0)
(1, 1)
(2, 0)
(2, 1)
Explanation:
In the example code-
- We create a nested for loop, where the outer for loop iterates over a range of numbers from 0 to 2 using range(3). The control variable for this loop is i.
- Within each iteration of the outer loop, an inner for loop is executed. This inner loop iterates over a range of numbers from 0 to 1 using range(2) and control variable j.
- During each iteration of the inner loop, the current values of i and j are printed to the console using f-strings.
- This results in pairs of values (i, j) being printed, where i ranges from 0 to 2 and j ranges from 0 to 1, creating a nested loop structure.
- Finally, when all iterations of both loops are completed, the loop terminates.
Through this example, we demonstrate how nested for loops can be used to perform iterations over two-dimensional data structures or execute repetitive tasks in a nested fashion.
Python For Loop With Continue & Break Statements
In Python, continue and break are control flow statements that can be used within for loops to alter the flow of execution.
- Continue Statement: When encountered within a loop, continue causes the program to skip the remaining code in the loop body for the current iteration and proceed to the next iteration. It allows skipping certain iterations based on a condition without exiting the loop entirely.
- Break Statement: The break is used to prematurely exit the loop when a specific condition is met. When encountered, it terminates the loop immediately and continues execution with the code following the loop.
Syntax Of Python For Loop Containing Continue & Break:
for item in iterable:
if condition:
continue # Skip to the next iteration
if condition:
break # Exit the loop prematurely
# Code block
Here,
- item: Variable representing the current item in the iterable.
- iterable: The sequence over which the loop iterates.
- condition: A condition that determines whether to continue or break the loop.
- Code block: Block of code to be executed for each item in the iterable.
Code Example:
IyBGb3IgbG9vcCB3aXRoIGNvbnRpbnVlIGFuZCBicmVhayBzdGF0ZW1lbnRzCmZvciBpIGluIHJhbmdlKDEsIDYpOgogIGlmIGkgPT0gMzoKICAgIGNvbnRpbnVlICMgU2tpcCBpdGVyYXRpb24gd2hlbiBpIGVxdWFscyAzCiAgICBwcmludChpKQogIGlmIGkgPT0gNDoKICAgIGJyZWFrICMgRXhpdCBsb29wIHdoZW4gaSBlcXVhbHMgNA==
Output:
1
2
4
Explanation:
In the Python example code-
- We create a for loop that iterates over a range of numbers from 1 to 5 using range(1, 6).
- Inside the loop, we have an if-statement which checks if the value of the loop control variable i is equal to 3, using the relational equality operator.
- If the condition is true, the flow moves inside the if-statement, and the continue statement is encountered. This causes the program to skip the rest of the code and start the next iteration.
- If the condition is false, the print() function in the next line displays the corresponding value of loop variable i.
- Then, we have a second if-statement, which checks if the value of i == 4. If the condition is true, the program encounters the break statement and immediately terminates the for loop.
- As shown in the output, the continue statement ensures that when i equals 3, the loop continues to the next iteration without printing the value of i. The break statement ensures that the loop stops when i equals 4.
Through this example, we demonstrate how continue and break statements can be used to control the flow of execution within a for loop based on certain conditions. The continue statement makes the program skip iterations, while break enables early termination of the loop.
Python For Loop With Pass Statement
In Python, the pass statement is a placeholder that does nothing when executed. It is often used as a syntactic placeholder in situations where code is required syntactically but no action needs to be performed. When used within a Python for loop, the pass statement allows you to have an empty code block without causing any errors.
Syntax:
for item in iterable:
if condition:
pass # Does nothing, continue with the loop
# Code block
Here,
- item: Variable representing the current item in the iterable object.
- iterable: The sequence over which the loop iterates.
- condition: A condition that determines whether to execute the code block or pass without action.
- Code block: Code statements to be executed.
Code Example:
IyBGb3IgbG9vcCB3aXRoIHBhc3Mgc3RhdGVtZW50CmZvciBpIGluIHJhbmdlKDEsIDYpOgogIGlmIGkgPT0gMzoKICAgIHBhc3MgIyBEbyBub3RoaW5nIHdoZW4gaSBlcXVhbHMgMwogIGVsc2U6CiAgICBwcmludChpKQ==
Output:
1
2
4
5
Explanation:
In the code example-
- We create a for loop that iterates over a range of numbers from 1 to 5 using range(1, 6).
- The loop contains an if-esle statement where the if condition checks whether the value of variable i is 3, i.e., i==3.
- If the condition is true, the pass statement is encountered. The pass statement in Python is a null operation, meaning it does nothing. So, when i equals 3, nothing happens in that iteration and the else-block is ignored.
- For all other values of i, the if condition is false, and hence the else block is executed. The print() function in the else-block displays the value of i to the console.
- Finally, when the loop completes its iterations, it terminates. However, the pass statement ensures that the loop continues even when i equals 3, without executing any additional code in that case.
Else Statement In Python For Loop
In Python, the else statement, when used along with a Python for loop specifies the code of block to be executed when the loop completes all iterations. But the else-block is executed only if the for loop completes iterations without encountering a break statement. In other words, it allows execution of a block of code after the loop finishes its normal operation.
Syntax:
for item in iterable:
# Code block
else:
# Code to execute after the loop completes
Here,
- item: Variable representing the current item in the iterable.
- iterable: The sequence over which the loop iterates.
- Code block: Block of code to be executed for each item in the iterable.
- Code to execute after the loop completes: Additional code to be executed after the loop finishes all iterations.
Code Example:
IyBGb3IgbG9vcCB3aXRoIGVsc2Ugc3RhdGVtZW50CmZvciBpIGluIHJhbmdlKDEsIDYpOgogIHByaW50KGkpCmVsc2U6CiAgcHJpbnQoIkxvb3AgY29tcGxldGVkIHN1Y2Nlc3NmdWxseSIp
Output:
1
2
3
4
5
Loop completed successfully
Explanation:
In the code example-
- We execute a for loop that iterates over a range of numbers from 1 to 5 using range(1, 6).
- The loop contains a print() statement that displays the value of i, as per the given range/ iterable object.
- After all iterations are completed successfully, the else block is executed, thus printing the string message- "Loop completed successfully".
- The else statement here is associated with the for loop. It runs only if the loop completes all its iterations without any interruptions.
- Since the loop does not encounter any interruptions/ break statements.
Conclusion
Python for loop serves as a fundamental building block for iterating through data structures efficiently and elegantly. Its simple syntax and powerful functionality make it a cornerstone of Python programming, enabling developers to handle diverse types of data with ease. Whether you're traversing lists, strings, dictionaries, or nested structures, the Python for loop empowers you to process data effectively while maintaining code readability.
Furthermore, the for loop's flexibility extends beyond mere iteration. With flow control statements like break and continue, you can fine-tune the loop's behavior to suit specific requirements, enhancing its versatility and utility. Additionally, the ability to nest for loops allows us to handle multidimensional data structures seamlessly, opening up possibilities for complex data processing tasks. By mastering the for loop, you can unlock the ability to process and manipulate data with elegance and precision in Python.
Frequently Asked Questions
Q. What is the difference between a for loop and a while loop in Python?
Aspect | For Loop | While Loop |
---|---|---|
Syntax | for item in iterable: | while condition: |
Initialization | Initializes iteration variable explicitly. | Initializes iteration variable outside |
Condition | Iterates until the iterable is exhausted. | Iterates until the condition is false. |
Termination | Terminates automatically when done. | Requires explicit condition for termination. |
Control | Limited control using break and continue. | More control with condition-based iteration. |
Use Cases | Ideal for iterating over sequences. | Suitable for indefinite iteration. |
Q. Can I use a Python for loop to iterate over a range of numbers?
Yes, you can use a for loop to iterate over a range of values in Python. For this, you can use the range() function, which generates a normal sequence of numbers, which can then be iterated over using a for loop.
Here's how it works: When we use the range() function, we specify the start, stop, and, optionally, the step size of the sequence. The function returns an iterable object that generates numbers from the start value up to (but not including) the stop value, incrementing by the step size.
For example, in the below lines of code, range(5) generates the sequence of numbers [0, 1, 2, 3, 4]. This sequence can be iterated over using a for loop like so:
Code Example:
for i in range(5):
print(i)
Output:
0
1
2
3
4
Here, the loop iterates over each number in the sequence generated by range(5), assigning each number to the variable i in turn. The loop body then executes for each value of i, printing it to the console.
Q. Are nested for loops common in Python programming language?
Yes, nested for loops are commonly used types of loops, especially when dealing with multidimensional data structures such as matrices or nested lists. They allow you to iterate over each element of nested structures efficiently.
Q. Is it possible to prematurely terminate a Python for loop?
Yes, it is possible to prematurely terminate a for loop in Python using the break statement. The break keyword is a control flow statement that allows you to exit a loop prematurely based on a certain condition being met.
When the break statement is encountered within a Python for loop, the loop immediately terminates, and the program execution continues with the statement immediately following the loop. Below is an example demonstrating the use of the break statement in a for loop.
Code Example:
ZnJ1aXRzID0gWyJhcHBsZSIsICJiYW5hbmEiLCAiY2hlcnJ5IiwgImRhdGUiLCAiZWxkZXJiZXJyeSJdCgpmb3IgZnJ1aXQgaW4gZnJ1aXRzOgogIGlmIGZydWl0ID09ICJkYXRlIjoKICAgIGJyZWFrCiAgcHJpbnQoZnJ1aXQp
Output:
apple
banana
cherry
In this example, the loop iterates over each fruit in the fruits list. When the loop encounters the fruit element date, the condition fruit == "date" becomes true, and the break statement is executed. As a result, the loop terminates prematurely, and the program execution continues with the statement immediately following the loop.
Q. Can I skip the current iteration and continue with the next one in a Python for loop?
Yes, you can skip the current iteration and continue with the next one in a for loop using the continue statement. When the continue statement is encountered within a for loop, it immediately skips the remaining code within the loop for the current iteration and proceeds with the next iteration. This allows you to bypass specific iterations based on certain conditions without exiting the loop entirely. The continue statement is particularly useful when you want to skip certain elements or perform additional checks before executing the rest of the Python for loop body.
Here are a few Python topics you should explore:
- 12 Ways To Compare Strings In Python Explained (With Examples)
- Python Logical Operators, Short-Circuiting & More (With Examples)
- Python Bitwise Operators | Positive & Negative Numbers (+Examples)
- Python String.Replace() And 8 Other Ways Explained (+Examples)
- How To Reverse A String In Python? 10 Easy Ways With Examples!
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment