12 Ways To Compare Strings In Python Explained (With Examples)
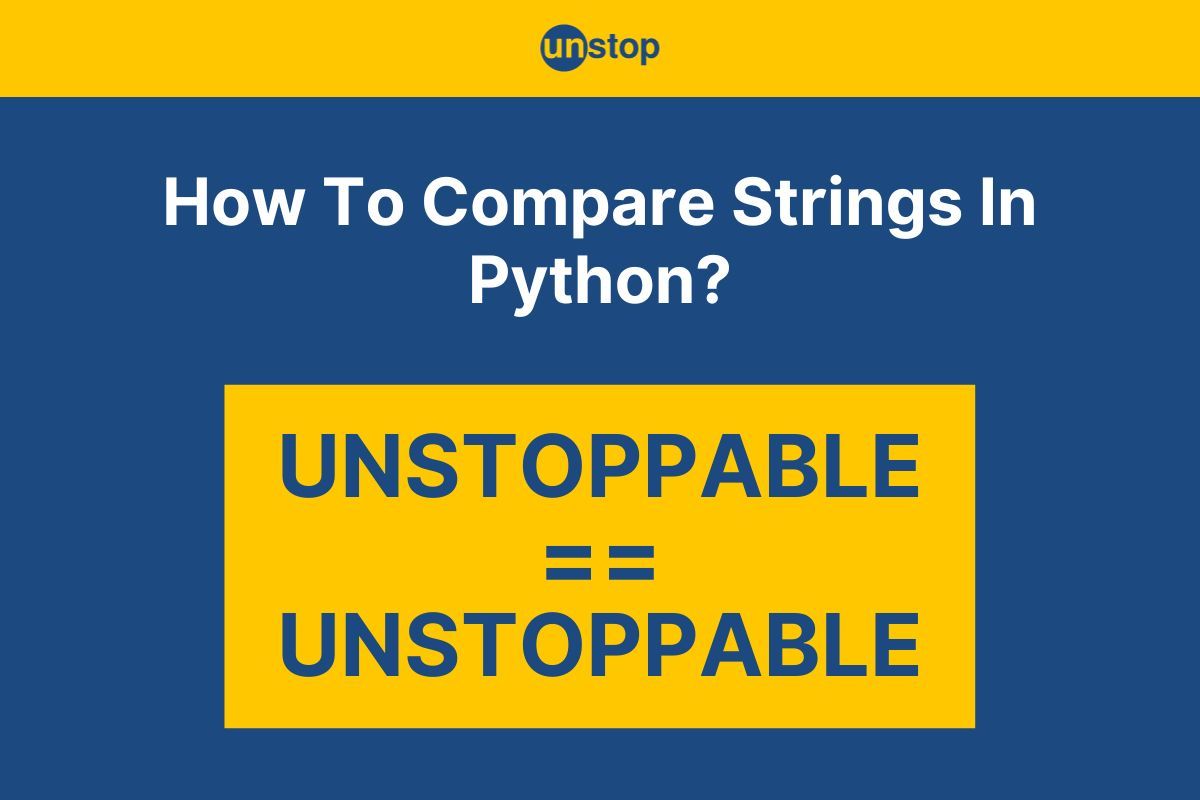
Table of content:
- Why Compare Strings In Python?
- How To Compare Two String In Python Using Relational Operators?
- How To Compare Strings In Python Using Equality Operator(==)?
- Compare Strings In Python Using Inequality Operator (!=)
- The is() Function To Compare Strings In Python
- The is not() Function To Compare Strings In Python
- The equals to (_eq_) Method For To Compare Strings in Python
- The re.match() Method To Compare Strings In Python
- Case Insensitive Python String Comparison With upper() And lower() Methods
- How to Compare String In Python With casefold() Method?
- How To Compare Two Strings In Python & Ignore Whitespace?
- Compare String In Python Using Collections.Counter() Method
- Compare Strings In Python Using Regular Expressions (RegEx)
- Using The SequenceMatcher (difflib)
- Compare Strings In Python With User-Defined Function
- Conclusion
- Frequently Asked Questions
A string is a collection of characters(letters, numbers, and symbols) that is used to represent text-based information in programming. Manipulations or operations on strings help us deal with and use textual data in programs. One such useful manipulation that is often performed is the comparison of strings. There are many met to compare strings in Python language.
When we compare strings in Python programs, the equality of strings and their relative order (according to the lexical or alphabetical order) are taken into account. In this article, we'll explore different built-in string comparison methods in Python and explore various string matching algorithms. We will also look at multiple examples of Python string comparison throughout the discussion.
Why Compare Strings In Python?
There are several reasons why we might need to compare strings, as they serve various purposes in programming. This is especially true in scenarios where you need to determine the equality of strings and their order, look for a target substring/ string, conduct string/ substring matching, etc. Listed here are some of the reasons why one may conduct string comparison in Python-
- Sorting Process: When you need to sort a list of strings alphabetically or based on a specific order.
- Searching and Filtering: To search for specific strings within larger string text bodies or to filter out specific strings from a dataset.
- Validation and Authentication: Checking if a user-provided password matches the stored one or validating input against predefined patterns.
- Conditional Logic: Making decisions based on the content of strings, such as controlling program flow or enabling specific functionality.
- Data Deduplication: Identifying and eliminating duplicate entries in a collection of strings.
Now that we know about the need for string comparison, let's look at the two primary classifications of the Python string comparison methods based on the case of letters in the string.
Case-Sensitive String Comparison
While doing a case-sensitive string comparison, uppercase and lowercase characters are distinguished from one another. The case of each character matters; that is, Apple and apple are considered to be separate strings. This type of string comparison in Python is especially helpful when you want exact letter-case differentiation, such as in usernames or identifiers, etc.
The following are the ways to perform case-sensitive comparisons in Python:
- The equality operator (==), for example- string1 == string2
- The str.casefold() method, for example- string1.casefold() == string2.casefold()
Code Example:
Output:
The strings are not equal.
Explanation:
In this sample Python program-
- We first declare and initialize two variables of string data type, i.e., string1 and string2, with the word Hello, but with different capitalization.
- Next, we start an if-else statement, which compares the two strings using the equality operator.
- If the condition holds true, then the code in if-block is executed. Here, we use the print() statement to display the message- The strings are equal.
- If the condition is false, then the code in the else block is executed, and the message in the respective print() is printed to the console.
- In this case, since the strings have different capitalization, the else block is executed.
Case-Insensitive String Comparisons
When using a case-insensitive string comparison, uppercase and lowercase characters are not taken into consideration. This means that if we compare the string/ words- Hello and hello, they will be considered as equal. Such a comparison is useful when you want to verify content equivalency without considering letter cases, such as in search functionalities or user input validation where variances in the case are prevalent.
Here are a few methods for performing the case-insensitive comparison in Python-
- The str.lower() method: With this method, you can lower string1 and string2 using the following formula: string1.lower() == string2.lower()
- The sr.upper() method, for example- string1.upper() == string2.upper()
Code Example:
Output:
The strings are equal (case-insensitive).
Explanation:
In the example Python code above-
- We declare two string variables, string1 and string2, and initialize them with the same word/ string (Hello) but with different cases for all letters.
- Next, we create an if-else statement to compare the strings and display the result to the window using the print() function. Inside the if-else statement-
- The if-condition uses the lower() method to convert both strings to lowercase for case-insensitive comparison.
- It then uses the equality operator to see if they are equal. If the condition returns true, then the print() statement in the if block is executed.
- if the condition returns false, then the print() statement inside the else-block is executed.
- In this case, since the strings have the same content and the same case after modification, the if block is executed.
How To Compare Two String In Python Using Relational Operators?
Relational operators are used to analyze the relation between two operands and draw inferences about their relationship. If the condition for comparison is true and, depending on the outcome of the comparison, they return a Boolean value (True or False). Here is a table showing popular Python relational operators:
Operator |
Description of Operator |
Example |
== |
Equal to |
5 == 5 (True) |
!= |
Not equal to |
10 != 7 (True) |
< |
Less than |
3 < 5 (True) |
<= |
Less than or equal to |
8 <= 8 (True) |
> |
Greater than |
12 > 9 (True) |
>= |
Greater than or equal to |
6 >= 6 (True) |
Now that we know about the different relational operators let's take a look at an example showcasing how to use them to compare strings in Python.
Output:
Equal: False
Not Equal: True
Less Than: True
Greater Than: False
Less Than or Equal: True
Greater Than or Equal: False
Explanation:
- We begin the Python program above by declaring and initializing two integer variables, x and y, with the values of 10 and 15, respectively.
- Then, as mentioned in the code comment, we use the relational operators to compare the two strings. Here-
- First, we use the equality operator (==) to compare if x is equal to y. The result of this comparison (either True or False) is stored in the variable equal_result. Here, the values of x and y are not equal, so the result is False.
- The is not equal operator (!=) is then used to compare if x is not equal to y, and the result is stored in the variable not_equal_result. Since the values of x and y are not equal, the result is True.
- Then, we use the less than (<) and greater than (>) operators to compare if x is less than y and if x is greater than y, respectively. The results of these comparisons are stored in the variables less_than_result (True) and greater_than_result (False), respectively.
- Next, we use the less than equal to (<=) operator to compare if x is less than or equal to y. The result is stored in the variable less_equal_result. Since the value of x is less than the value of y, the result is True.
- After that, the greater than equal to (>=) relational operator is employed to compare if x is greater than or equal to y. The result is stored in the variable greater_equal_result, which is False since x is not greater than y.
- After all the comparisons are done, we use a series of print() statements to print the results of all the comparisons.
- In the print statements, we use formatted strings specifying the operator name and add the respective variable with the result.
Time Complexity:
Relational operators have a constant execution time, denoted as O(1), making them consistently efficient regardless of data size.
How To Compare Strings In Python Using Equality Operator(==)?
In Python, the == string comparison operator is used to check for equality between two strings. It determines, character by character, whether the contents of two strings are precisely the same. String comparison using == is case-sensitive. Uppercase and lowercase letters are treated as distinct characters, affecting the comparison outcome. Because of its case sensitivity, this procedure treats capital and lowercase letters differently.
Syntax:
string1 == string2 .
Here,
- String1 and string2 refer to the names of strings that are being compared.
- The symbol (==) refers to the equality operator, which will compare the string character by character.
Note- If every character in both strings is present in the same order, it returns True; otherwise, False.
Code Example:
Output:
Result 1: False
Result 2: True
Explanation:
- We begin by declaring three string variables, i.e., text1, text2, and text3, with the values Hello, hello, and Hello, respectively.
- Next, we declare a fourth variable, result1, and assign it the value of comparison between text1 and text2.
- Here, we use the equality operator (==) to check if the strings are equal.
- If the two strings are equal, then the operator returns True, and if not, then it returns False.
- In this case, the two strings have the same content, but one is in the title case, and the other not, the result is False.
- We once again use the equality operator to compare text1 and text3 and assign the output of the comparison to the variable result2. Since here, both the strings are exactly the same, the result is True.
- After the comparisons are done, we use two print() statements to display the results to the console.
Time Complexity: O(min(len(string1), len(string2)))- The comparison process stops as soon as a mismatch is found or when one of the strings ends. Therefore, the time taken is proportional to the length of the shorter string.
Space Complexity: O(1)- No additional memory is required other than the input strings and a few variables.
Compare Strings In Python Using Inequality Operator (!=)
The inequality operator (!=) is used in Python to compare two strings for inequality. This string comparison method analyzes whether the contents of two strings are not the same, character by character. This operation is also case-sensitive, meaning it considers uppercase and lowercase letters as distinct characters.
Syntax:
string1 != string2
Here,
- String1 and string2 are the names of the string variables being compared.
- The symbol (!=) refers to the operator that performs a character-wise comparison between string1 and string2.
Note- The operator returns True if both strings have different characters or different lengths and False if they have the same characters in the same order.
Code Example:
Output:
Result 1: True
Result 2: True
Explanation:
In this Python code-
- We initialize three string variables, text1, text2, and text3, with the values Hello, hello, and World, respectively, using the double quotes method.
- Next, we declare another variable, result1, and use the inequality operator (!=) to compare text1 and text2.
- The output of this comparison is stored in result1. Since the two strings differ in case, the result of this string comparison is True.
- Then, we use the inequality operator again to compare text1 and text3. The result of this comparison is stored in the variable result2, which is True since both the strings are entirely different.
- Lastly, we call the print() function twice to display the result of the comparison we conducted in the code.
Time Complexity: O(min(len(string1), len(string2))): In this case, if any one of the strings ends or if a mismatch is found, then the comparison procedure stops. As a result, the time required is proportional to the length of the shorter string.
Space Complexity: O(1): In this case, only the string input and a few variables needed to be stored in memory.
The is() Function To Compare Strings In Python
We can use the is() function in Python to check the identity of two strings and conduct a comparison thereof. It determines whether two string variables point to the same memory object.
- However, note that the correct way to use the is() function for string comparison is not by using it as a function call but by using the is operator directly.
- The is operator in Python is case-sensitive when used for string comparison.
- It checks whether two strings reference the same memory location, and it doesn't consider the content of the strings.
Syntax:
string1 is string2
Here,
- The string1 and string2 are the names of the string variables.
- The is keyword refers to the is() function being used to compare the strings in Python code.
Note- The function determines whether string1 and string2 relate to the same memory address. If they match, it gives back True, denoting that the strings are identical. It returns False if they each refer to a distinct memory location.
Code Example:
Output:
Comparison 1: True
Comparison 2: False
Explanation:
- In this example, we initialize two string variables, string1, and string2, with the same value, i.e., Python, and then a third string variable, string3, with the value Java.
- Next, we use the is() function to compare string1 to string2 first, and the result of this comparison is stored in the comparison1 variable.
- The function checks if both the strings are the same object. And since both the strings are identical, the result is True.
- We once again use the is() function to compare string1 and string3, which checks if string 1 is the same object as string3. Since it is not, the result is False, which is stored in the variable comparison2.
- Finally, we use the print() function twice to display the results of the two comparisons.
Time Complexity is O(1): The is() method examines whether two references point to the same memory address, which may be done in constant time.
Space Complexity O(1): Minimal memory usage beyond the input strings and a few variables.
Also read- Python IDLE | The Ultimate Beginner's Guide With Images & Codes!
The is not() Function To Compare Strings In Python
In Python, the is not() method is used for string comparison to determine whether two strings do not reference the same memory location, indicating their non-identity in memory. This function is also case-sensitive and is the inverse of the is() function.
Syntax:
string1 is not string2
Here,
- The names string1 and string2 refer to the two strings being compared.
- The keyword is not, refers to the is not() function, which checks if both string1 and string2 do not refer to the same memory location.
- If they don't, the function returns True, denoting that the strings are different in memory. It returns False if they are located in the same memory location.
Code Example:
Output:
Comparison 1: True
Comparison 2: False
Explanation:
- We begin the example by declaring three string variables, i.e., name1, name2, and name3.
- Of these, name1 and name3 are initialized with the same value, i.e., Shivani. And name2 is initialized with the value Shreeya.
- Next, we use the is not() function to compare name1 to name2 (as mentioned in the code comments). It checks if the objects are not in the same memory location.
- If the location is the same, then the function returns False, and if they are not in the same location, the function returns True. Since the two strings are not the same, the result is True, which is stored in the variable comparison1.
- We once again use the is not() function, to compare strings name1 and name3. Since the two objects are the same, the function returns False, which is stored in the variable comparison2.
- Lastly, we use the print() function twice to display the results of the comparisons to the output console.
Time Complexity: O(1): The is not() method, which is a constant time fundamental operation, tests directly to see if two references point to separate memory regions.
Space Complexity: O(1): Use of memory beyond the input strings is minimal.
The equals to (_eq_) Method For To Compare Strings in Python
We can compare strings in Python by using the equals to() method, which is represented by the shotform _eq_. This function determines whether the characters in the two strings are equal or not, character by character, and it is case-sensitive.
It compares the strings exactly by considering the case of characters. If the characters in the compared strings have different cases, then the comparison will result in False.
Syntax:
string1.__eq__(string2)
Here,
- The names of the string variables are given by string1 and string2.
- The _eq_ represents the equals to() method, which compares each character in the strings individually.
Let's consider an example Python program to know more about the string comparison using __eq__method.
Code Example:
Output:
The messages are not the same.
Explanation:
- We initialize two string variables, message1, and message2, with the values Hello, world! and Hello, Python!, respectively.
- Next, we use the equals to() method to check if message1 is equal to message2 and store the result in variable result1.
- The function returns True if the characters in both strings are equal and returns False if they are not.
- Then, we initiate an if-else statement, which checks if the two strings are equal. If they are equal, then the if code block is executed. It uses the print() function to display the message- The messages are the same.
- If the messages are not equal, then the else code block is executed, and the respective message is printed to the console.
- Since here, the two messages are not equal, the else-block is executed in the end.
Time Complexity: O(n): As the __eq__ method compares each character in each string individually, the time complexity is linearly proportional to the length of the strings.
Space Complexity: O(1): The input strings and a few variables take up the least amount of new memory.
The re.match() Method To Compare Strings In Python
The re.match() is a Python function for matching a regular expression pattern at the beginning of a string. If a pattern is found at the beginning of the string, it returns a match object; otherwise, it returns None.
To determine if a regular expression pattern matches at the beginning of a string, the re.match() function in Python is used. While this is usually used to match patterns, it may also be used in a basic string comparison. The re.match() function is case-sensitive.
Syntax:
re.match(pattern, string)
Here,
- The string keyword refers to the string being analyzed to find a pattern fit.
- The parameter pattern indicates the pattern we are looking for in the string.
- The re.match() function provides a string that can be used for comparison and the pattern of an ordinary expression.
Now, let’s have an example program that showcases how to use the re.match() method to compare strings in Python.
Code Example:
Output:
The strings match.
Explanation:
At the beginning of the example, we have to import the built-in Re module. This is the Python regular expression module, which contains the re.match() function.
- After importing the module, we declare and initialize two variables, text1, and text2, with the values Hello, world! and Hello, respectively.
- We then use the re.match() method to compare the text2 string (which acts as the pattern parameter) to the start of the text1 string. The result of this comparison is stored in the match_result variable.
- Next, we initiate an if-else statement to check if the value of match_result is not None, indicating that a match was found.
- If the condition is True, the code in the if block will be executed, and the print() function, which displays the statement- The string matches, to the console.
- If the condition is False, the code in the else block will be executed, and the respective message will be printed.
Time Complexity: O(n): A function called re.match() compares the periodic expression pattern to the beginning of a string, resulting in linear time complexity that is proportional to its length and type complexity.
Space complexity: O(1): minimal use of additional memory beyond the input strings and a few variables.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Case Insensitive Python String Comparison With upper() And lower() Methods
As discussed before, we can conduct case-insensitive string comparisons in Python. In order to do that, you must first convert strings to a common case (usually uppercase or lowercase) before using the equality operator (==) to compare them.
The first way to carry out a case-insensitive comparison is by using the upper() method.
- This method, when applied to the strings, converts all characters of both strings to uppercase.
- After that, we can use the equality operator or any other method to compare the strings.
The other method to conduct a case-insensitive comparison is to use the lower() method on the Python strings you want to compare.
- This method is used to convert all characters of a string on which it is applied to lowercase.
- Once again, after converting the string you can for case-insensitive equality.
Applying these methods ensures that the Python string comparison is not affected by the letter casing, i.e., whether all the letters in strings are in uppercase or lowercase. This makes sure that the comparison does not take into account the differences in the letter casing, thereby making it easier to compare strings without worrying about their original capitalization.
Syntax:
string1.upper() == string2.upper()
string1.lower() == string2.lower()
Here,
- The names of the strings being compared are string1 and string2.
- The .upper() and .lower() indicate the methods we are applying to the strings.
- Last, the symbol == is the equality operator.
Given ahead is an example Python program that showcases case insensitive comparison with the upper() method.
Code Example:
Output:
The strings are case-insensitively equal.
Explanation:
- To begin with, we define two variables, string1 and string2, and initialize them with the values Hello and HeLLo, respectively.
- Then, we use the upper() method to convert both strings to uppercase before comparing them with the equality operator.
- The outcome of this comparison is stored in the result variable.
- Next, we apply an if-else conditional statement, which checks if the result of the equality operation is True or not.
- If the result is true, then the if block is executed, where we use a print() function to display the phrase- The strings are case-insensitively equal.
- If the result is false, then the else block is executed, and the phrase printed to the console is- The strings are not case-insensitively equal.
- In this case, the result is true, and hence the if-block is executed.
Time Complexity: O(n) As the upper() and lower() methods iterate over each character in the string, the time complexity is linearly inversely correlated to the length of the strings.
Space Complexity: O(1) - Only the input strings and a few variables require any additional memory.
How to Compare String In Python With casefold() Method?
In Python, the casefold() method is used for case-insensitive string comparison. It is similar to the lower () method, which converts the string to a single case for comparison. However, this method not only converts the string to lowercase but also performs additional transformations to handle special characters. This makes it more aggressive in terms of case-insensitivity compared to the lower() method.
Syntax:
string1.casefold() == string2.casefold()
Here,
- The .casefold() indicates we are calling the casefold() method on the string mentioned before.
- The names of the strings are given by string1 and string2.
Now, let's have a look at an example program where we use the casefold() method to compare strings in Python.
Code Example:
Output:
String 1: Python Programming
String 2: Javascript
The strings are not case-insensitively equal.
Explanation:
- We define two variables, string1 and string2, and initialize them with the values Python Programming and JavaScript, respectively.
- Next, we apply the casefold() method to the strings to transform them into a case-insensitive form. Then, we apply the equality operator to compare the transformed strings for equality.
- The outcome of this comparison on case insensitive form is stored in the result variable.
- After that, we use two print() statements to display the values of the string variable to the console.
- Then, we employ an if-else condition to check whether the value of the result is True or not.
- If the value is true (indicating that the strings are equal), then the if-block is executed.
- If the value of the result is False (indicating that the strings are not equal regardless of case), then the else block is executed.
- Since here, the strings are unequal, and the value of the result variable is False, the else block is executed, and the respective phrase is printed.
Time Complexity: O(n): The casefold() method iterates through each character of the strings, resulting in a linear time complexity proportional to the length of the strings.
Space Complexity: O(1): The memory usage is constant since the memory required for the transformation and comparison does not scale with the input size.
The casefold() and the lower() methods perform similar operations on Python strings. In the table below, we have given a description of casefold() vs. lower() methods.
Method |
Description |
casefold() |
It is used for case-insensitive comparison. The casefold() is more aggressive. It performs additional transformations that handle special characters and are based on Unicode values |
lower() |
It is also used for case-insensitive comparison. You can convert both strings to lowercase using the lower() method and then compare them for case-insensitive equality. Still, it may not handle all special characters and languages as comprehensively as casefold(). |
How To Compare Two Strings In Python & Ignore Whitespace?
Ignoring the whitespace when comparing two strings can be crucial to ensure accurate matching. This can be achieved by removing all whitespace characters from both strings before performing the comparison. We can use the Python string replace() method to replace the white spaces with no space.
By eliminating whitespace, the comparison focuses solely on the content of the strings, making it useful for scenarios like comparing user inputs or handling formatted text.
Syntax:
string1.replace(" ", "") == string2.replace(" ", "")
Here,
- The names string1 and string2 represent the strings we are comparing.
- The .replace() refers to the usual replace() method, and the parameters include space and no space.
- Lastly, we have the equality operator (==), which checks for equality between the strings.
Code Example:
Output:
String 1: The quick brown fox
String 2: The quick brown fox
String 1 without space: Thequickbrownfox
String 2 without space: Thequickbrownfox
The strings are equal after ignoring whitespace.
Explanation:
In the Python code example, we-
- Define two variables, string1 and string2, that are initialized with the same phrase- The quick brown fox, but with different amounts of whitespace.
- Then, we define two more variables, string1_no_space and string2_no_space, which are initialized as follows-
- The variables store the values of string1 and string2, but with no whitespace, respectively.
- For this, we apply the Python string.replace() method on the initial strings to remove all whitespace characters.
- Next, we compare the new versions of the strings with the equality operator. The outcome of this operation is stored in the result variable.
- After that, we call the print() function four times to display the values of the original strings (i.e., string1 and string2) and the values of the modified versions (string1_no_space and string2_no_space) to the console.
- We then use an if-else statement to check the value of the result variable.
- If the value is True (indicating that the modified strings are equal after ignoring whitespace), the if code block is executed.
- If the value is False (indicating that the modified strings are not equal after ignoring whitespace), the else code block is executed.
- Since here, the strings after whitespace removal are the same, the if-code block is executed. The print() function displays the phrase contained in the console.
Time Complexity: O(n): The Python string.replace() method iterates through each character of the strings to remove whitespace, resulting in a linear time complexity proportional to the length of the strings.
Space Complexity: O(n): The replace() method creates new strings without whitespace, requiring additional memory proportional to the length of the input strings.
Compare String In Python Using Collections.Counter() Method
In Python, the collections.Counter() class is a powerful tool for comparing strings, which allows you to consider the frequency of characters in each string. By creating counters for both strings, you can check if their character frequencies match, indicating equality. This approach is particularly useful for scenarios where the order of characters doesn't matter, such as anagrams or when comparing two sets of characters.
Syntax:
from collections import Counter
counter1 = Counter(string1)
counter2 = Counter(string2)
result = counter1 == counter2
Here,
- The term collections is the name of the module that contains the needed function, and the term Counter is the name of the library.
- The term counter() is the function that counts the frequency of all the characters in a string.
- The terms string1, string2, and the symbol (==) refer to the names of the strings and the equality operator, respectively.
Let's take a look at an example showcasing the implementation of this function to compare strings in Python.
Code Example:
Output:
String 1: programming
String 2: mmogginparr
Counter 1: Counter({'r': 2, 'g': 2, 'm': 2, 'p': 1, 'o': 1, 'a': 1, 'i': 1, 'n': 1})
Counter 2: Counter({'m': 2, 'g': 2, 'r': 2, 'o': 1, 'i': 1, 'n': 1, 'p': 1, 'a': 1})
The strings have matching character frequencies.
Explanation:
- Firstly, we import the Counter class from the collections module.
- We then define two strings, string1 (programming) and string2 (mmogginparr), with the same characters rearranged in different sequences.
- Next, we create counters for both strings, i.e., counter1 for string1 and counter2 for string1, using the Counter() constructor. Here, the counters will count the occurrences of each character.
- Then, we use the equality operator to compare the two counters.
- If the character frequencies match, the result will be True; otherwise, it will be False.
- The outcome of the comparison is stored in the result variable.
- After that, we use the print() function to print the original strings (i.e., string1 and string2) and the values of the counters to show the character frequencies of both strings.
- We employ an if-else statement to check the value of the result variable. If the value is True (indicating that the character frequencies match), then the if-code block is executed.
- If it is False (indicating that the character frequencies do not match), then the else-code block is executed.
- Here, the result is True, the print() statement in the if-block is executed, and the respective phrase is printed to the console.
Time Complexity: O(n + m): Creating the counters for both strings requires iterating through each character once, resulting in a linear time complexity proportional to the total length of the strings (n for string1 and m for string2).
Space Complexity: O(n + m): The space complexity is determined by the sizes of the counters, which store character frequency information for both strings.
Compare Strings In Python Using Regular Expressions (RegEx)
Regular expressions (RegEx) in Python provide a versatile way to compare strings with customizable patterns. By utilizing RegEx's pattern matching capabilities, you can create patterns that match both strings.
Then, the re.match() function checks if a string matches the pattern at the beginning. This approach is useful for cases where you need to perform more complex string comparisons based on specific patterns.
Syntax:
import re
match_result = re.match(pattern, string)
Explanation:
- The Python re module contains the functions and regex pattern we want to use.
- The re.match() function is used to compare a string against a given pattern.
- Terms pattern, string, and match_result refer to the pattern we are looking for, the string which we are checking for a match, and the variable in which we store the result, respectively.
If the string matches the pattern at the beginning, the match_result will contain the match; otherwise, it will be None. Let's consider an example program to learn more about string Python string comparison using Regular Expressions (RegEx).
Code Example:
Output:
String 1: programming is fun!
String 2: program
Pattern: ^program
The strings match based on the RegEx pattern.
Explanation:
- Firstly, import the re locale module to work with regular expressions and then initialize two string variables, string1 (programming is fun!) and string2 (program).
- We then create a RegEx pattern by combining the string2 with the start-of-line anchor (^). This pattern ensures that the comparison is based on matching the beginning of string1 with string2.
- Next, we use the re.match() function to check if the RegEx pattern (pattern) matches the beginning of string1. The outcome of this function is stored in the match_result variable.
- Then, we use the print() function to display the values of the original strings (i.e., string1 and string2) and then the RegEx pattern that is used for comparison.
- After that, we employ the if-else conditional statement to check the value of the match_result variable.
- If the value is not None (indicating that the RegEx pattern matches the beginning of string1), then the if-code block is executed.
- If the value is None (indicating that the RegEx pattern does not match the beginning of string1), then the else-code block is executed.
- Here, the beginning of both strings is the same, the if-block is executed, and the phrase contained in the respective print statement is displayed.
Time Complexity: O(n)- The re.match() function traverses the characters of the pattern and the string to check for a match, resulting in a linear time complexity proportional to the length of the strings.
Space Complexity: O(1)- The memory usage is constant since the memory required does not scale with the input size.
Using The SequenceMatcher (difflib)
The SequenceMatcher class from the Python difflib module offers a versatile approach to compare strings in Python and highlight their similarities and differences. It computes a similarity ratio between two strings, indicating their similarity as a floating-point value between 0 and 1. This method is useful for comparing strings with minor differences or when considering string similarity rather than strict equality.
Syntax:
from difflib import SequenceMatcher
sequence_matcher = SequenceMatcher(None, string1, string2)
similarity_ratio = sequence_matcher.ratio()
Here,
- The terms difflib and SequenceMatcher refer to the name of the module and the class, respectively, we must import.
- Names string1 and string2 refer to the two strings that we are comparing.
- The terms sequence_matcher and sequence_ratio refer to the object of the SequenceMatcher class and the ratio of the comparison, respectively.
Code Example:
Output:
String 1: programming is fun
String 2: programing is enjoyable
Similarity Ratio: 0.7317073170731707
The strings are not identical.
Explanation:
- Firstly, we import the SequenceMatcher class from the difflib module and then define two variables, i.e., string1 (programming is fun) and string2 (programming is enjoyable).
- We then create an object of the SequenceMatcher class named sequence_matcher by initializing it with the two strings to be compared.
- Next, we apply the ratio() method to compute the similarity ratio between the two strings. The outcome of this calculation is stored in the similarity_ratio variable.
- After that, we use the print() function three times to print the values of the initial strings and the similarity ratio between the strings.
- Then, we employ an if-else statement to check the values of the similarity_ratio variable.
- If the ratio is 1.0 (indicating identical strings), the code block following the if-segment is executed.
- If the similarity ratio is not 1.0 (indicating that the strings have differences), the code block under the else statement is executed.
- In this example, the ratio is not 1, so the print statement from the else statement prints the respective phrase to the console.
Time Complexity: O(n * m)- The SequenceMatcher algorithm compares all elements of both strings, resulting in a time complexity proportional to the product of the lengths of the strings (n for string1 and m for string2).
Space Complexity: O(n * m)- The memory usage is determined by the storage of the comparison matrix, which has dimensions based on the lengths of the input strings.
Compare Strings In Python With User-Defined Function
In cases where you have specific criteria for comparing strings, you can define a custom function that implements the comparison logic. This approach provides flexibility to tailor the comparison according to your needs. You can design the function to consider case sensitivity, ignore whitespace, or apply any other desired rules for comparison.
Syntax:
def custom_compare(string1, string2):
# Custom comparison logic here
return result
result = custom_compare(string1, string2)
Here,
- Custom_compare refers to the name of the function you are defining.
- String1 and string2 refer to the names of the string variables we are comparing.
- The term return refers to the return value of the comparison result (True or False) based on your desired comparison logic.
Let's take a look at a code example to understand how to define and use a custom function to compare strings in Python.
Code Example:
Output:
String 1: Web Development
String 2: web development
The strings are customarily equal.
Explanation:
- We begin the example by defining a function called custom_compare() that takes two string inputs. Inside the function-
- As per the custom comparison logic, we use the Python string replace() method to remove all whitespaces from both variables.
- We also use the lower() method to convert them to lowercase, and the result is stored in the variables stripped_string1 and striped_string2.
- After that, we use the equality operator to compare the string and the function returns a boolean value from this comparison.
- Next, we define (and initialize) two variables, string1 (_Web Development_) and string2 (web development_).
- We then call the custom_compare() function on the two string variables and store the comparison outcome in the result variable.
- After that, we use the print() function to print the values of the strings.
- Then, we initiate an if-else condition to check the value of the result variable.
- If the result is True (i.e., the custom comparison logic evaluates to True), the code block following the if statement is executed.
- If the result is False (i.e., the custom comparison logic evaluates to False), the code block following the else statement is executed.
- Here, the result is True, so the print() statement in the if-block prints the respective phrase to the console.
Time Complexity: O(n): The custom comparison logic operates linearly on the lengths of the input strings, resulting in a time complexity proportional to the length of the strings.
Space Complexity: O(1): The memory usage is constant since no additional data structures are used.
Conclusion
In conclusion, we have explored how to compare strings in Python by using various techniques and special methods. These include various relational operators, is() method, is not() method, regex pattern, re.match(), and more. Choosing the right string comparison method depends on the specific requirements of your task.
Whether it's a simple equality in strings check, case-insensitive matching, whitespace-agnostic comparison, regular expression-based matching, or similarity assessment, Python provides versatile tools to handle various scenarios. Understanding these methods empowers you to make informed decisions when comparing strings in your Python projects.
Frequently Asked Questions
Q. What is the difference between == and equals() in comparing two strings in Python?
The equality operator (==) in Python is used to compare two string values and determine whether they are equal. It compares the string's actual contents, character by character. This means that it checks if the two strings are pointing to the same object in memory. If they are, then the operator will return True, and if not, then False.
The Python built-in function equals() checks for the equality of strings as well. However, it is more widely used in other computer languages, such as Java.
Aspect |
== operator( equality operator) |
equals() operator |
Comparison of content |
Used for comparing string contents. |
Used for comparing string contents. |
Memory location |
Compare memory location. |
Compare memory location. |
Syntax |
string1==string2 |
string1.equals(string2) |
Usage in Python |
Commonly used |
Not used (use equals() for string comparison) |
Usage in Java |
Not used (use == operator for string comparison) |
Commonly used |
For example, the following code will return True, even though the two strings have different values:
Output:
The strings are equal.
Explanation:
- Initially, we define the variables named s1 and s2, where s1 is initialized as Hello, world! and s2 with s1.
- Secondly, the if condition is implemented. In such a case, if s1 is equal to s2, then it prints the statement in the print() function.
This is because the == operator is comparing the references of the strings, not the values of the strings. The s1 and s2 variables both refer to the same object in memory, so the == operator returns True.
Q. How do you compare strings in Python boolean?
You can compare strings in Python by using comparison operators. When comparing strings in Python using these operators, the result is a Boolean value, which means it's either True or False. This result indicates whether the comparison condition is satisfied or not. Here's how you can do it:
Output:
False
Explanation:
- We initialize the variables string1 and string2 with the values apple and banana.
- Next, we use the equality operator to compare string1 and string2. And its outcome is stored in the result variable.
- If the strings are equal, the result will be True, and if they are not equal, the result will be False.
- Then, we display the value of the result variable using the print() function.
Q. What is string comparison in Python, and why is it important?
A string comparison in Python is a process of comparing values to determine whether the two values are equal or different. The ability to compare strings in Python is an essential component that allows developers to create powerful and efficient applications, whether validating user inputs, searching for specific words, or assuring data quality.
Q. How do you compare variables to strings in Python?
In Python, you can compare variables to strings by using the comparison operators, such as ==, !=, <, >, <=, and >=. By using these operators, you can compare variable values with string literals and other string variables.
Here's an example of how to compare variables to strings:
Output:
The variable is not equal to 'banana'.
The variable and another_variable have the same value.
Explanation:
- We begin by declaring a variable my_variable and assigning it the value apple.
- Next, we compare my_variable to a string literal banana using the == operator. Since my_variable is not equal to banana, the code inside the else block is executed.
- We declare another variable, another_variable, and assign it the same value as my_variable, which is apple.
- Then we compare my_variable to another_variable using the == operator. Since they have the same value, the code inside the if block is executed.
Q. How do I perform a case-insensitive string comparison in Python?
To perform a case-insensitive comparison, you can convert both strings to lowercase or uppercase letters using the lower() or upper() methods, respectively. After the conversion, you can use the equality operator (==) to compare the strings for equality.
This ensures that the comparison is not affected by differences in letter casing. For example, say you want to compare two strings while disregarding whether the letters are uppercase or lowercase. Then, use the upper() or lower() method to convert the strings to a common case before performing a comparison. This approach shall ensure that the comparison is sensitive.
Q. Are there any Python libraries that specialize in advanced string comparison and normalization?
Python offers specialized libraries for advanced string comparison methods and normalized strings. For example,
- The fuzzywuzzy library is well-suited for fuzzy string matching and similarity measurement, making it ideal for scenarios with approximate string matches.
- The difflib library, on the other hand, is designed for comparing sequences, including strings, and generating differences, which is useful for text comparison and visual diffs.
These libraries extend the language's string comparison capabilities beyond exact matches. These advanced abilities to compare strings in Python are valuable tools in applications like spell checkers, version control systems, and document comparison tools.
Here are a few other articles that will quench your thirst for knowledge:
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment