Difference Between List And Tuple In Python Explained (+Examples)
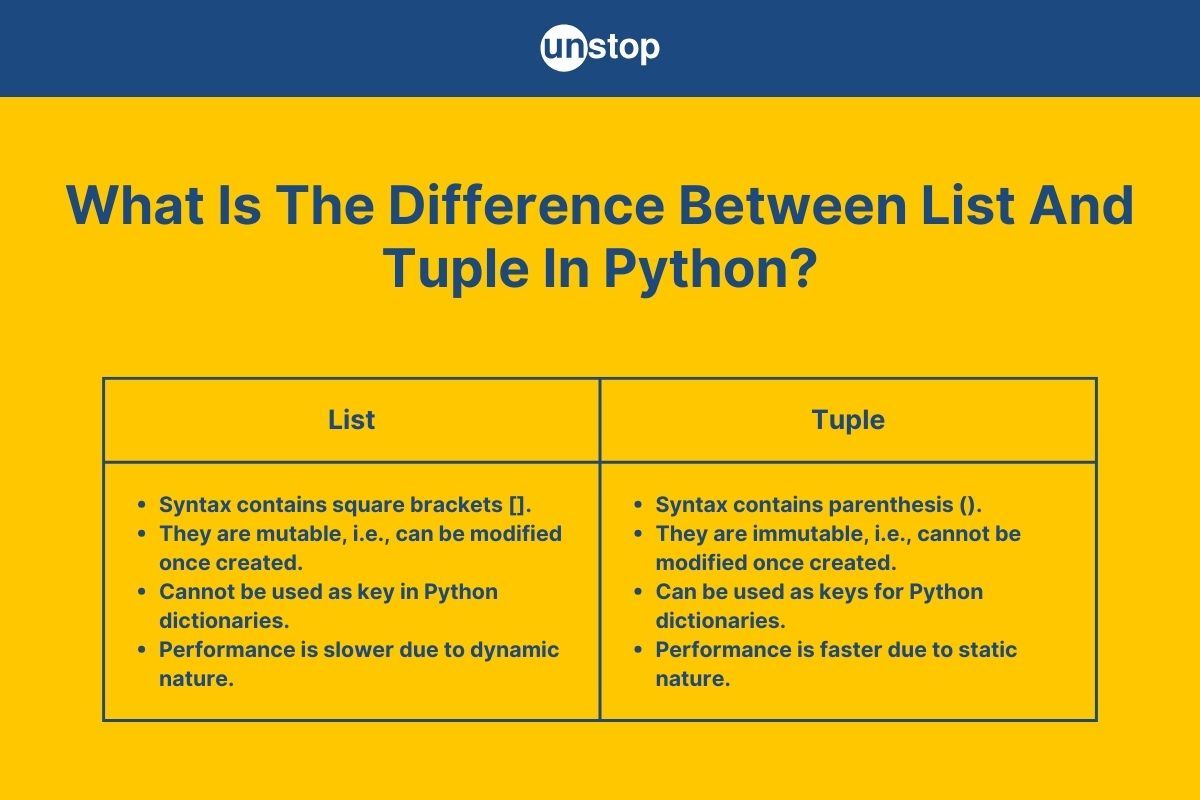
Python, a widely used programming language, offers various data structures to handle collections of items. Among these, lists and tuples are two fundamental structures that every Python programmer must understand. While they might appear similar at first glance (especially since they are both heterogeneous data types), they do have distinct characteristics and uses.
In this article, we will explore the key difference between list and tuple in Python programming and discuss the fundamentals of both data structures.
What Is A List?
A list in Python is a versatile, ordered collection of items that can hold elements of different data types. Lists are commonly used to store collections of related data, allowing for easy access, modification, and iteration over the elements. They are especially useful for handling dynamic data where the number of items can change, such as user inputs, data streams, or any sequence of items needing processing.
For example:
fruits = ["apple", "banana", "cherry"]
print(fruits[0]) # Output: apple
In this example, the fruits list stores three string elements representing different types of fruit. We can access the first list element using its index with the indexing operator [], as shown in the second line of the example.
What Is A Tuple?
A tuple in Python is an ordered collection of items that is immutable, meaning its elements cannot be changed after the tuple is created. Tuples are used to store collections of heterogeneous data and are particularly useful for representing fixed sequence data types that should not be modified. They are often used for grouping related pieces of data, such as coordinates, database records, or any set of values that belong together.
For example:
coordinates = (10.0, 20.0)
print(coordinates[0]) # Output: 10.0
In this example, the tuple named coordinates contains two float elements representing a point in a 2D space. We can access the first tuple object (10.0) using its index value, i.e., 0.
Difference Between List And Tuple In Python (Comparison Table)
The following table highlights the major differences between a list and a tuple in Python:
Feature | List | Tuple |
---|---|---|
Mutability | Mutable, i.e., elements can be changed, added, or removed. | Immutable, i.e., elements cannot be changed after creation. |
Syntax | Defined using square brackets []. | Defined using parentheses (). |
Performance | Slower due to dynamic nature and overhead. | Faster due to static nature and optimization. |
Use Cases | Suitable for collections of items that may change over time, such as user inputs or list objects. | Ideal for fixed collections of items, like coordinates, configuration settings, or constants. |
Methods | Extensive methods for modification, such as append(), remove(), pop(), sort(). | Limited methods, mostly for counting and indexing, such as count(), index(). |
Dictionary Keys | The list data type cannot be used as dictionary keys due to mutability. | The tuple type can be used as dictionary keys due to immutability. |
Iteration | Generally slower to iterate due to dynamic size and mutability. | Generally faster to iterate due to fixed size and immutability. |
Memory Consumption | Consumes more memory because of dynamic nature. | Consumes less memory because of static nature. |
Copying | Creating a copy results in a new identical list (shallow copy by default). | Creating a copy results in a reference to the same data (no new data allocation). |
Immutability Benefits | No benefits from immutability; changes can introduce bugs. | Benefits from immutability include safe sharing between threads and preventing accidental changes. |
Element Assignment | Elements can be reassigned and modified. | Elements cannot be reassigned or modified. |
Size Flexibility | It can grow and shrink dynamically. | It has a fixed size after creation and cannot grow or shrink. |
Element Access | Supports random access to elements by indexing. | Supports random access with indexing. |
Heterogeneous | It can store elements of different data types. | It can store elements of different data types. |
Literal Example | Example: [1,2,3] | Example: (1,2,3) |
Syntax Difference Between List And Tuple In Python
Lists and tuples are fundamental data structures used to store collections of items. Despite their similar purposes, the syntax for defining lists and tuples is distinct, reflecting their different characteristics and uses. Understanding these syntax differences is crucial for correctly utilizing lists and tuples in your programs.
List Syntax:
my_list = [item1, item2, item3, ...]
It is defined using square brackets [ ] and can hold a series of items, including different data types. The list syntax allows for dynamic resizing, meaning elements can be added, removed, or modified after the list is created. For example-
my_list = [1, "hello", 3.14]
This line of code leads to the creation of a list with an integer, a string, and a float as list elements.
Tuple Syntax:
my_tuple = (item1, item2, item3, ...)
It is defined using parentheses () and stores a series of items, but they are immutable. Once a tuple is created, its elements cannot be changed, added, or removed. This immutability provides certain performance benefits and ensures the integrity of the data. For example-
my_tuple = (1, "hello", 3.14)
This line of code leads to creating a tuple with an integer, a string, and a float as tuple elements.
Now let's look at a Python program example to further highlight the difference between list and tuple in Python.
Code Example:
Output:
Original list: [10, 20, 30, 40]
Modified list: [10, 25, 30, 40]
Original tuple: (100, 200, 300, 400)
Unexpected Error: 'tuple' object does not support item assignment
Explanation:
In the Python code example-
- We start by defining a list named my_list with the elements [10, 20, 30, 40].
- Then, we use the print() function with a formatted string to print the original list with the message "Original list:" followed by the list contents.
- Next, we use the indexing number to modify the second element of my_list by setting my_list[1] to 25.
- After that, we print the modified list with the message "Modified list:" followed by the updated list contents.
- We then define a tuple named my_tuple with the elements (100, 200, 300, 400).
- Next, we use the print() function to print the original tuple with the message "Original tuple:" followed by the tuple contents.
- We then attempt to modify the second element of my_tuple by setting my_tuple[1] to 250.
- Since tuples are immutable, this tuple operation raises a TypeError.
- We catch the TypeError exception in a try block and print the error message.
Mutability Difference Between List And Tuple In Python
Mutability refers to the ability of an object to be changed after it has been created. In Python, this concept distinguishes lists from tuples.
Lists: Lists are mutable, meaning their elements can be altered, added, or removed after the list is created. This makes lists suitable for dynamic data collections where modifications are frequently required. For example:
my_list = [1, 2, 3]
my_list[1] = 20 # List after modification: [1, 20, 3]
my_list.append(4) # List after appending: [1, 20, 3, 4]
my_list.remove(20) # List after removal: [1, 3, 4]
In this simple Python example snippet-
- We first create and initialize a list my_list with elements [1, 2, 3].
- The ability to modify lists is demonstrated by changing the element at index 1 to 20, appending 4 to the list, and then removing 20.
- These operations illustrate the mutability of lists, allowing for dynamic changes to the collection of elements throughout the program's execution.
Tuples: Tuples are immutable, meaning once a tuple is created, its elements cannot be changed, added, or removed. This immutability makes tuples ideal for fixed collections of items where data integrity and performance are important. For example:
my_tuple = (1, 2, 3)
# Attempting to change an element raises a TypeError
try:
my_tuple[1] = 20
except TypeError as e:
print("Error:", e) # Output: Error: 'tuple' object does not support item assignment
In this short Python code sample-
- We create and initialize a tuple my_tuple with elements (1, 2, 3).
- Tuples are immutable, meaning their elements cannot be changed once the tuple is created.
- Attempting to modify an element, as shown by my_tuple[1] = 20, raises a TypeError because tuples do not support item assignment after creation.
Other Difference Between List And Tuple In Python
Beyond mutability, lists and tuples differ in several other key aspects. In this section, we will explore all such differences between tuple and list in Python in detail.
List vs Tuple In Python | Operations
A key difference between list and tuple in Python arises from the common operations we can perform on these data structures.
Lists: Lists support a variety of operations that allow modification, such as append(), remove(), insert(), pop(), clear(), extend(), sort(), and reverse(). For example:
my_list = [1, 2, 3]
my_list.append(4) # Adds 4 to the end: [1, 2, 3, 4]
my_list.remove(2) # Removes the first occurrence of 2: [1, 3, 4]
my_list.insert(1, 'a') # Inserts 'a' at index 1: [1, 'a', 3, 4]
my_list.sort() # Sorts the list: [1, 3, 4]
Tuples: Due to the immutability of Tuples, they support limited operations primarily focused on accessing data. These include count() and index(). For example:
my_tuple = (1, 2, 3, 2)
my_tuple.count(2) # Returns the count of 2: 2
my_tuple.index(3) # Returns the index of the first occurrence of 3: 2
List vs. Tuple In Python | Functions Support
- Lists provide a wide range of built-in functions for manipulation and data management, making them versatile for tasks where data may change frequently.
- In contrast, tuples have limited built-in methods due to their immutability, focusing on accessing elements and querying data.
List vs. Tuple In Python | Size
The sizes of tuples and lists differ in that lists have variable sizes, whereas tuples are of a fixed size. This means-
- Lists can grow or shrink dynamically, depending on operations like appending or removing elements, which dynamically reallocates memory.
- Tuples have a fixed size once created, resulting in constant consumption of memory throughout their lifetime.
List Vs. Tuple In Python | Type of Elements
Another key difference between list and tuple in Python is the type of elements we can store in them.
Lists can store elements of different data types and are designed for heterogeneous collections. This flexibility allows list elements to store integers, floats, strings, and even other lists as elements. For example:
my_list = [1, 'hello', 3.14, [1, 2, 3]]
Tuples can also store elements of different data types and support heterogeneous collections.
my_tuple = (1, 'hello', 3.14, (4, 5))
List Vs. Tuple In Python | Efficiency
Memory efficiency is important in selecting the appropriate data structure based on the specific requirements and constraints of our Python application. The difference between list and tuple in Python when it comes to efficiency is as follows:
- Lists are less memory-efficient compared to tuples due to their dynamic nature, which may involve frequent reallocation of memory chunks.
- Tuples are more memory-efficient because they are immutable, allowing Python to optimize memory usage without reallocating memory.
List Vs. Tuple In Python | Memory Allocation
-
Lists are mutable and dynamically resizable. Python initially allocates single memory block and reallocates as needed when elements of list are added or removed, which can lead to occasional overhead.
-
Tuples are immutable and fixed in size. They allocate specific chunks of memory upon creation based on their elements, avoiding the need for dynamic resizing and making them more memory-efficient since they need lesser memory capacity as compared to tuples.
List Vs. Tuple In Python | Methods
In Python, lists and tuples are both used for storing collections of items, but they differ significantly in their mutability and the methods they support. In this section, we will see the difference between list and tuple in Python when it comes to the methods we can use to manipulate the data stored in them.
Python Lists: Mutable And Extensive Methods
Lists are mutable, meaning their elements can be modified after creation. This mutability allows lists to support a wider range of methods that facilitate dynamic data manipulation. Here are some key methods commonly used with lists:
Method | Description | Example | Output |
append(obj) | Appends an element to the end of the list | [1, 2, 3].append(4) | [1, 2, 3, 4] |
len(list) | Returns the number of elements in list or items in the list |
n = [10, 11, 12, 13] len(n) |
4 |
extend(seq) | Adds all items of one list to another list. | [1, 2, 3].extend([4, 5, 6]) | [1, 2, 3, 4, 5, 6] |
count(obj) | Returns the number of occurrences of a given element in the list | [2, 3, 2, 4, 2].count(2) | 3 |
remove(obj) | Removes specified elements from the list | [1, 2, 3, 4].remove(2) | [1, 3, 4] |
index(obj) | Returns the index of the first occurrence of the specified item; otherwise throws an exception |
[1, 2, 3, 4].index(2) [1, 2, 3].index(4) |
1 Exception |
pop() | Removes the last element from the list. | [1, 2, 3, 4].pop() | 4 |
insert(index, obj) | Insert the element obj into the list at the given index. | [1, 2, 3].insert(1, 4) | [1, 4, 2, 3] |
Python Tuples: Immutable And Limited Methods
Tuples are immutable, meaning once they are created, their elements cannot be modified. Consequently, tuples support a more limited set of methods compared to lists, primarily focused on accessing elements rather than modifying them. Here are some common methods available for tuples:
Method | Description | Example | Output |
cmp(tuple1, tuple2) | Returns 0 if elements in both the tuples are the same; otherwise compares the elements based on their ASCII values |
cmp((123, 'xyz'), (123, 'xyz')) cmp((123, 'xyz'), (123, 'xyz', 456)) |
0 -1 |
len(tuple) | Returns the length of the tuple. | len((1, 2, 3)) | 3 |
max(tuple) | Returns the maximum element in the tuple. | max((1, 3, 26, 4)) | 26 |
min(tuple) | Returns the minimum element in the tuple. | min((1, 3, 26, 4)) | 1 |
tuple(seq) | Converts a list or a sequence into a tuple | tuple([1, 2, 3]) |
(1, 2, 3) |
sorted() | The built-in sorted() function is used to sort elements based on the default natural sorting order. | t1 = (6, 5, 1, 4) t = sorted(t1) |
[1, 4, 5, 6] |
index(obj) | Returns the index of the first occurrence of the specified item; otherwise, it throws an exception |
(1, 2, 3, 4).index(2) (1, 2, 3).index(4) |
1 Exception |
When To Use Tuples Over Lists?
Choosing between tuples and lists in Python depends on the specific requirements of your program, considering factors such as mutability, performance, and intended use. Here are several scenarios where the tuple data types are typically preferred over lists, along with examples illustrating their use:
1. Immutable Data: It is beneficial to use the tuple data type when using data that should not change throughout the program's execution.
Example: Storing constants or configuration settings.
# Example of configuration settings stored in a tuple
database_config = ('localhost', 3306, 'username', 'password')
Tuples provide data integrity because they are immutable. Once initialized, their values cannot be modified accidentally, ensuring critical configuration data remains unchanged during program execution.
2. Performance Optimization: Using tuple type over lists is better when working with large datasets or performance-critical code where immutability and efficient memory usage are beneficial.
Example: Returning multiple values from a Type function.
# Function returning multiple values as a tuple
def get_user_info(user_id):
# Fetch user data from database
user_data = ... # Assume fetched user data
return user_data['name'], user_data['email'], user_data['age']
Tuples are more memory-efficient than lists because Python does not need additional memory allocation to support dynamic resizing or modification operations. This efficiency can be crucial in scenarios where performance optimization is a priority.
3. Sequence Packing and Unpacking: When you need to pack multiple values together into a single entity or unpack them into individual variables, you may prefer using tuple type over lists.
Example: Iterating over multiple lists simultaneously.
# Iterating over multiple lists simultaneously using zip and tuple unpacking
names = ['Alice', 'Bob', 'Charlie']
ages = [30, 25, 35]for name, age in zip(names, ages):
print(f'{name} is {age} years old.')
Tuples facilitate packing and unpacking of data using Python's tuple unpacking feature, making it straightforward to work with multiple values simultaneously without explicitly indexing each element.
4. Dictionary Keys: When using tuples as keys in dictionaries is better to use tuple type over lists in Python.
Example: Creating a dictionary where tuple keys represent coordinates.
# Dictionary using tuples as keys (coordinates to values)
coordinates_data = {
(10, 20): 'Location A',
(30, 40): 'Location B',
(50, 60): 'Location C'
}# Accessing data using tuple keys
print(coordinates_data[(10, 20)]) # Output: Location A
Tuples are hashable and can be used as keys in dictionaries because of their immutability. This makes tuples suitable for scenarios where you need to map unique combinations of values to corresponding data.
5. Ensuring Data Integrity: It is better to use a tuple data type when you want to ensure that data remains unchanged and consistent throughout its lifecycle.
Example: Representing records in a database where fields should not be modified.
# Example of database records represented as tuples
user_record = (1001, 'Alice', 'alice@example.com')
By using tuples to represent data that should not change, you enforce data integrity and reduce the risk of accidental modifications, ensuring that critical data remains consistent and reliable.
Key Similarities Between Tuples And Lists In Python
Despite the difference between list and tuple in Python, in terms of mutability and intended use cases, the two data structures share several key similarities, including:
- Sequential Data Storage: Both tuples and lists are sequential data structures that store collections of items in a specific order. Elements within both tuples and lists can be accessed using indexing.
- Heterogeneous Collection: Both tuples and lists can store elements of different data types (e.g., integers, strings, floats) within the same collection.
- Iterable: Both tuples and lists are iterable, meaning you can iterate over their collection of elements using loops or other iterative constructs in Python (e.g., for loops, list comprehensions).
- Slicing: Both tuples and lists support slicing operations, allowing you to extract subsets of elements based on start, stop, and step indices.
- Membership Testing: Both tuples and lists allow you to check if an element exists within the collection using the in and not in operators.
- Length Calculation: You can determine the number of elements in both tuples and lists using the built-in len() function.
- Indexing: Elements in both tuples and lists are accessed via indexing. Positive indices start from 0 (first element), while negative indices count backwards from the last element.
Conclusion
Both lists and tuples are fundamental data structures in Python, each serving unique purposes based on their characteristics. By understanding the difference between list and tuple in Python you can choose the right data structure for your specific needs, ensuring efficient and effective code design. Lists excel in scenarios requiring dynamic data manipulation, offering flexibility with operations like appending, removing, and sorting elements from lists. On the other hand, tuples provide immutability and fixed-size storage, ensuring data integrity and optimal blocks of memory usage.
Also, Python lists are best suited for situations where data needs to be modified frequently, the order of the elements matters. In contrast, tuples, are best suited for situations where the data needs to be accessed frequently but not modified.
Frequently Asked Questions
Q. Why would we use a tuple instead of a list?
Tuples are preferred when you need an immutable collection of tuple elements that should not change during program execution, such as storing configuration settings or database records. They also offer performance benefits due to their fixed size and memory efficiency.
Q. When should we use a list over a tuple?
Lists are suitable when you need a collection that can be modified dynamically, such as adding or removing elements, sorting, or performing list operations that change the data structure over time. They are ideal for scenarios requiring flexibility and frequent data updates.
Q. What are the performance differences between lists and tuples in Python?
Tuples generally offer better performance compared to lists in terms of memory usage and iteration speed because of their immutability and fixed size. Lists, being mutable, may require more/ extra memory blocks and incur additional overhead for dynamic resizing and mathematical operations.
Q. How do I decide between using a list or a tuple for my data?
The decision depends on whether you need mutable or immutable object data. Choose lists for scenarios where you need to modify or manipulate data frequently. Opt for tuples when data integrity and performance optimization are priorities, and the data should remain unchanged once defined.
Q. Can tuples contain elements of different data types, like lists?
Yes, tuples can contain elements of different data types, just like lists. Both tuples and lists can hold heterogeneous collections, meaning they can store a mix of different types of elements, such as integers, strings, floats, and even other lists or tuples.
Example:
my_tuple = (1, 'apple', 3.14, [5, 6, 7], (8, 9))
my_list = [1, 'banana', 2.71, [10, 11], (12, 13)]
In this sample Python code:
- my_tuple contains an integer (1), a string ('apple'), a float (3.14), a list ([5, 6, 7]), and another tuple ((8, 9)).
- my_list contains an integer (1), a string ('banana'), a float (2.71), a list ([10, 11]), and another tuple ((12, 13)).
By now, you must know the key difference between list and tuple in Python. Here are a few other topics you must explore:
- Python Program To Find The Square Root (8 Methods + Code Examples)
- Find Area Of Triangle In Python In 8 Ways (Explained With Examples)
- Python Program To Convert Decimal To Binary, Octal And Hexadecimal
- Python Program To Find LCM Of Two Numbers | 5 Methods With Examples
- Convert Int To String In Python | Learn 6 Methods With Examples
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment