Table of content:
- What Is Python? An Introduction
- What Is The History Of Python?
- Key Features Of The Python Programming Language
- Who Uses Python?
- Basic Characteristics Of Python Programming Syntax
- Why Should You Learn Python?
- Applications Of Python Language
- Advantages And Disadvantages Of Python
- Some Useful Python Tips & Tricks For Efficient Programming
- Python 2 Vs. Python 3: Which Should You Learn?
- Python Libraries
- Conclusion
- Frequently Asked Questions
- It's Python Basics Quiz Time!
Table of content:
- Python At A Glance
- Key Features of Python Programming
- Applications of Python
- Bonus: Interesting features of different programming languages
- Summing up...
- FAQs regarding Python
- Take A Quiz To Rehash Python's Features!
Table of content:
- What Is Python IDLE?
- What Is Python Shell & Its Uses?
- Primary Features Of Python IDLE
- How To Use Python IDLE Shell? Setting Up Your Python Environment
- How To Work With Files In Python IDLE?
- How To Execute A File In Python IDLE?
- Improving Workflow In Python IDLE Software
- Debugging In Python IDLE
- Customizing Python IDLE
- Code Examples
- Conclusion
- Frequently Asked Questions (FAQs)
- How Well Do You Know IDLE? Take A Quiz!
Table of content:
- What Is A Variable In Python?
- Creating And Declaring Python Variables
- Rules For Naming Python Variables
- How To Print Python Variables?
- How To Delete A Python Variable?
- Various Methods Of Variables Assignment In Python
- Python Variable Types
- Python Variable Scope
- Concatenating Python Variables
- Object Identity & Object References Of Python Variables
- Reserved Words/ Keywords & Python Variable Names
- Conclusion
- Frequently Asked Questions
- Rehash Python Variables Basics With A Quiz!
Table of content:
- What Is A String In Python?
- Creating String In Python
- How To Create Multiline Python Strings?
- Reassigning Python Strings
- Accessing Characters Of Python Strings
- How To Update Or Delete A Python String?
- Reversing A Python String
- Formatting Python Strings
- Concatenation & Comparison Of Python Strings
- Python String Operators
- Python String Functions
- Escape Sequences In Python Strings
- Conclusion
- Frequently Asked Questions
- Rehash Python Strings Basics With A Quiz!
Table of content:
- What Is Python Namespace?
- Lifetime Of Python Namespace
- Types Of Python Namespace
- The Built-In Namespace In Python
- The Global Namespace In Python
- The Local Namespace In Python
- The Enclosing Namespace In Python
- Variable Scope & Namespace In Python
- Python Namespace Dictionaries
- Changing Variables Out Of Their Scope & Python Namespace
- Best Practices Of Python Namespace
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Namespaces!
Table of content:
- What Are Logical Operators In Python?
- The AND Python Logical Operator
- The OR Python Logical Operator
- The NOT Python Logical Operator
- Short-Circuiting Evaluation Of Python Logical Operators
- Precedence of Logical Operators In Python
- How Does Python Calculate Truth Value?
- Final Note On How AND & OR Python Logical Operators Work
- Conclusion
- Frequently Asked Questions
- Python Logical Operators Quiz– Test Your Knowledge!
Table of content:
- What Are Bitwise Operators In Python?
- List Of Python Bitwise Operators
- AND Python Bitwise Operator
- OR Python Bitwise Operator
- NOT Python Bitwise Operator
- XOR Python Bitwise Operator
- Right Shift Python Bitwise Operator
- Left Shift Python Bitwise Operator
- Python Bitwise Operations And Negative Integers
- The Binary Number System
- Application of Python Bitwise Operators
- Python Bitwise Operator Overloading
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Bitwise Operators!
Table of content:
- What Is The Print() Function In Python?
- How Does The print() Function Work In Python?
- How To Print Single & Multi-line Strings In Python?
- How To Print Built-in Data Types In Python?
- Print() Function In Python For Values Stored In Variables
- Print() Function In Python With sep Parameter
- Print() Function In Python With end Parameter
- Print() Function In Python With flush Parameter
- Print() Function In Python With file Parameter
- How To Remove Newline From print() Function In Python?
- Use Cases Of The print() Function In Python
- Understanding Print Statement In Python 2 Vs. Python 3
- Conclusion
- Frequently Asked Questions
- Know The print() Function In Python? Take A Quiz!
Table of content:
- Working Of Normal Print() Function
- The New Line Character In Python
- How To Print Without Newline In Python | Using The End Parameter
- How To Print Without Newline In Python 2.x? | Using Comma Operator
- How To Print Without Newline In Python 3.x?
- How To Print Without Newline In Python With Module Sys
- The Star Pattern(*) | How To Print Without Newline & Space In Python
- How To Print A List Without Newline In Python?
- How To Remove New Lines In Python?
- Conclusion
- Frequently Asked Questions
- Think You Can Print Without a Newline in Python? Prove It!
Table of content:
- What Is A Python For Loop?
- How Does Python For Loop Work?
- When & Why To Use Python For Loops?
- Python For Loop Examples
- What Is Rrange() Function In Python?
- Nested For Loops In Python
- Python For Loop With Continue & Break Statements
- Python For Loop With Pass Statement
- Else Statement In Python For Loop
- Conclusion
- Frequently Asked Questions
- Think You Know Python's For Loop? Prove It!
Table of content:
- What Is Python While Loop?
- How Does The Python While Loop Work?
- How To Use Python While Loops For Iterations?
- Control Statements In Python While Loop With Examples
- Python While Loop With Python List
- Infinite Python While Loop in Python
- Python While Loop Multiple Conditions
- Nested Python While Loops
- Conclusion
- Frequently Asked Questions
- Mastered Python While Loop? Let’s Find Out!
Table of content:
- What Are Conditional If-Else Statements In Python?
- Types Of If-Else Statements In Python
- If Statement In Python
- If-Else Statement In Python
- Nested If-Else Statement In Python
- Elif Statement In Python
- Ladder If-Elif-Else Statement In Python
- Short Hand If-Statement In Python
- Short Hand If-Else Statement In Python
- Operators & If-Esle Statement In Python
- Other Statements With If-Else In Python
- Conclusion
- Frequently Asked Questions
- Quick If-Else Statement Quiz– Let’s Go!
Table of content:
- What Is Control Structure In Python?
- Types Of Control Structures In Python
- Sequential Control Structures In Python
- Decision-Making Control Structures In Python
- Repetition Control Structures In Python
- Benefits Of Using Control Structures In Python
- Conclusion
- Frequently Asked Questions
- Control Structures in Python – Are You the Master? Take A Quiz!
Table of content:
- What Are Python Libraries?
- How Do Python Libraries Work?
- Standard Python Libraries (With List)
- Important Python Libraries For Data Science
- Important Python Libraries For Machine & Deep Learning
- Other Important Python Libraries You Must Know
- Working With Third-Party Python Libraries
- Troubleshooting Common Issues For Python Libraries
- Python Libraries In Larger Projects
- Importance Of Python Libraries
- Conclusion
- Frequently Asked Questions
- Quick Quiz On Python Libraries – Let’s Go!
Table of content:
- What Are Python Functions?
- How To Create/ Define Functions In Python?
- How To Call A Python Function?
- Types Of Python Functions Based On Parameters & Return Statement
- Rules & Best Practices For Naming Python Functions
- Basic Types of Python Functions
- The Return Statement In Python Functions
- Types Of Arguments In Python Functions
- Docstring In Python Functions
- Passing Parameters In Python Functions
- Python Function Variables | Scope & Lifetime
- Advantages Of Using Python Functions
- Recursive Python Function
- Anonymous/ Lambda Function In Python
- Nested Functions In Python
- Conclusion
- Frequently Asked Questions
- Python Functions – Test Your Knowledge With A Quiz!
Table of content:
- What Are Python Built-In Functions?
- Mathematical Python Built-In Functions
- Python Built-In Functions For Strings
- Input/ Output Built-In Functions In Python
- List & Tuple Python Built-In Functions
- File Handling Python Built-In Functions
- Python Built-In Functions For Dictionary
- Type Conversion Python Built-In Functions
- Basic Python Built-In Functions
- List Of Python Built-In Functions (Alphabetical)
- Conclusion
- Frequently Asked Questions
- Think You Know Python Built-in Functions? Prove It!
Table of content:
- What Is A round() Function In Python?
- How Does Python round() Function Work?
- Python round() Function If The Second Parameter Is Missing
- Python round() Function If The Second Parameter Is Present
- Python round() Function With Negative Integers
- Python round() Function With Math Library
- Python round() Function With Numpy Module
- Round Up And Round Down Numbers In Python
- Truncation Vs Rounding In Python
- Practical Applications Of Python round() Function
- Conclusion
- Frequently Asked Questions
- Revisit Python’s round() Function – Take The Quiz!
Table of content:
- What Is Python pow() Function?
- Python pow() Function Example
- Python pow() Function With Modulus (Three Parameters)
- Python pow() Function With Complex Numbers
- Python pow() Function With Floating-Point Arguments And Modulus
- Python pow() Function Implementation Cases
- Difference Between Inbuilt-pow() And math.pow() Function
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python’s pow() Function!
Table of content:
- Python max() Function With Objects
- Examples Of Python max() Function With Objects
- Python max() Function With Iterable
- Examples Of Python max() Function With Iterables
- Potential Errors With The Python max() Function
- Python max() Function Vs. Python min() Functions
- Conclusion
- Frequently Asked Questions
- Think You Know Python max() Function? Take A Quiz!
Table of content:
- What Are Strings In Python?
- What Are Python String Methods?
- List Of Python String Methods For Manipulating Case
- List Of Python String Methods For Searching & Finding
- List Of Python String Methods For Modifying & Transforming
- List Of Python String Methods For Checking Conditions
- List Of Python String Methods For Encoding & Decoding
- List Of Python String Methods For Stripping & Trimming
- List Of Python String Methods For Formatting
- Miscellaneous Python String Methods
- List Of Other Python String Operations
- Conclusion
- Frequently Asked Questions
- Mastered Python String Methods? Take A Quiz!
Table of content:
- What Is Python String?
- The Need For Python String Replacement
- The Python String replace() Method
- Multiple Replacements With Python String.replace() Method
- Replace A Character In String Using For Loop In Python
- Python String Replacement Using Slicing Method
- Replace A Character At a Given Position In Python String
- Replace Multiple Substrings With The Same String In Python
- Python String Replacement Using Regex Pattern
- Python String Replacement Using List Comprehension & Join() Method
- Python String Replacement Using Callback With re.sub() Method
- Python String Replacement With re.subn() Method
- Conclusion
- Frequently Asked Questions
- Know How To Replace Python Strings? Prove It!
Table of content:
- What Is String Slicing In Python?
- How Indexing & String Slicing Works In Python
- Extracting All Characters Using String Slicing In Python
- Extracting Characters Before & After Specific Position Using String Slicing In Python
- Extracting Characters Between Two Intervals Using String Slicing In Python
- Extracting Characters At Specific Intervals (Step) Using String Slicing In Python
- Negative Indexing & String Slicing In Python
- Handling Out-of-Bounds Indices In String Slicing In Python
- The slice() Method For String Slicing In Python
- Common Pitfalls Of String Slicing In Python
- Real-World Applications Of String Slicing
- Conclusion
- Frequently Asked Questions
- Quick Python String Slicing Quiz– Let’s Go!
Table of content:
- Introduction To Python List
- How To Create A Python List?
- How To Access Elements Of Python List?
- Accessing Multiple Elements From A Python List (Slicing)
- Access List Elements From Nested Python Lists
- How To Change Elements In Python Lists?
- How To Add Elements To Python Lists?
- Delete/ Remove Elements From Python Lists
- How To Create Copies Of Python Lists?
- Repeating Python Lists
- Ways To Iterate Over Python Lists
- How To Reverse A Python List?
- How To Sort Items Of Python Lists?
- Built-in Functions For Operations On Python Lists
- Conclusion
- Frequently Asked Questions
- Revisit Python Lists Basics With A Quick Quiz!
Table of content:
- What Is List Comprehension In Python?
- Incorporating Conditional Statements With List Comprehension In Python
- List Comprehension In Python With range()
- Filtering Lists Effectively With List Comprehension In Python
- Nested Loops With List Comprehension In Python
- Flattening Nested Lists With List Comprehension In Python
- Handling Exceptions In List Comprehension In Python
- Common Use Cases For List Comprehensions
- Advantages & Disadvantages Of List Comprehension In Python
- Best Practices For Using List Comprehension In Python
- Performance Considerations For List Comprehension In Python
- For Loops & List Comprehension In Python: A Comparison
- Difference Between Generator Expression & List Comprehension In Python
- Conclusion
- Frequently Asked Questions
- Rehash Python List Comprehension Basics With A Quiz!
Table of content:
- What Is A List In Python?
- How To Find Length Of List In Python?
- For Loop To Get Python List Length (Naive Approach)
- The len() Function To Get Length Of List In Python
- The length_hint() Function To Find Length Of List In Python
- The sum() Function To Find The Length Of List In Python
- The enumerate() Function To Find Python List Length
- The Counter Class From collections To Find Python List Length
- The List Comprehension To Find Python List Length
- Find The Length Of List In Python Using Recursion
- Comparison Between Ways To Find Python List Length
- Conclusion
- Frequently Asked Questions
- Know How To Get Python List Length? Prove it!
Table of content:
- List of Methods To Reverse A Python List
- Python Reverse List Using reverse() Method
- Python Reverse List Using the Slice Operator ([::-1])
- Python Reverse List By Swapping Elements
- Python Reverse List Using The reversed() Function
- Python Reverse List Using A for Loop
- Python Reverse List Using While Loop
- Python Reverse List Using List Comprehension
- Python Reverse List Using List Indexing
- Python Reverse List Using The range() Function
- Python Reverse List Using NumPy
- Comparison Of Ways To Reverse A Python List
- Conclusion
- Frequently Asked Questions
- Time To Test Your Python List Reversal Skills!
Table of content:
- What Is Indexing In Python?
- The Python List index() Function
- How To Use Python List index() To Find Index Of A List Element
- The Python List index() Method With Single Parameter (Start)
- The Python List index() Method With Start & Stop Parameters
- What Happens When We Use Python List index() For An Element That Doesn't Exist
- Python List index() With Nested Lists
- Fixing IndexError Using The Python List index() Method
- Python List index() With Enumerate()
- Real-world Examples Of Python List index() Method
- Difference Between find() And index() Method In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python List Indexing? Take A Quiz!
Table of content:
- How To Remove Elements From List In Python?
- The remove() Method To Remove Element From Python List
- The pop() Method To Remove Element From List In Python
- The del Keyword To Remove Element From List In Python
- The clear() Method To Remove Elements From Python List
- List Comprehensions To Conditionally Remove Element From List In Python
- Key Considerations For Removing Elements From Python Lists
- Why We Need to Remove Elements From Python List
- Performance Comparison Of Methods To Remove Element From List In Python
- Conclusion
- Frequently Asked Questions
- Quiz– Prove You Know How To Remove Item From Python Lists!
Table of content:
- How To Remove Duplicates From A List In Python?
- The set() Function To Remove Duplicates From Python List
- Remove Duplicates From Python List Using For Loop
- Using List Comprehension Remove Duplicates From Python List
- Remove Duplicates From Python List Using enumerate() With List Comprehension
- Dictionary & fromkeys() Method To Remove Duplicates From Python List
- Remove Duplicates From Python List Using in, not in Operators
- Remove Duplicates From Python List Using collections.OrderedDict.fromkeys()
- Remove Duplicates From Python List Using Counter with freq.dist() Method
- The del Keyword Remove Duplicates From Python List
- Remove Duplicates From Python List Using DataFrame
- Remove Duplicates From Python List Using pd.unique and np.unipue
- Remove Duplicates From Python List Using reduce() function
- Comparative Analysis Of Ways To Remove Duplicates From Python List
- Conclusion
- Frequently Asked Questions
- Think You Know How to Remove Duplicates? Take A Quiz!
Table of content:
- What Is Python List & How To Access Elements?
- What Is IndexError: List Index Out Of Range & Its Causes In Python?
- Understanding Indexing Behavior In Python Lists
- How to Prevent/ Fix IndexError: List Index Out Of Range In Python
- Handling IndexError Gracefully Using Try-Except
- Debugging Tips For IndexError: List Index Out Of Range Python
- Conclusion
- Frequently Asked Questions
- Avoiding ‘List Index Out of Range’ Errors? Take A Quiz!
Table of content:
- What Is the Python sort() List Method?
- Sorting In Ascending Order Using The Python sort() List Method
- How To Sort Items In Descending Order Using Python sort() List Method
- Custom Sorting Using The Key Parameter Of Python sort() List Method
- Examples Of Python sort() List Method
- What Is The sorted() List Method In Python
- Differences Between sorted() And sort() List Methods In Python
- When To Use sorted() & When To Use sort() List Method In Python
- Conclusion
- Frequently Asked Questions
- Take A Quick Python's sort() Quiz!
Table of content:
- What Is A List In Python?
- What Is A String In Python?
- Why Convert Python List To String?
- How To Convert List To String In Python?
- The join() Method To Convert Python List To String
- Convert Python List To String Through Iteration
- Convert Python List To String With List Comprehension
- The map() Function To Convert Python List To String
- Convert Python List to String Using format() Function
- Convert Python List To String Using Recursion
- Enumeration Function To Convert Python List To String
- Convert Python List To String Using Operator Module
- Python Program To Convert String To List
- Conclusion
- Frequently Asked Questions
- Convert Lists To Strings Like A Pro! Take A Quiz
Table of content:
- What Is Inheritance In Python?
- Python Inheritance Syntax
- Parent Class In Python Inheritance
- Child Class In Python Inheritance
- The __init__() Method In Python Inheritance
- The super() Function In Python Inheritance
- Method Overriding In Python Inheritance
- Types Of Inheritance In Python
- Special Functions In Python Inheritance
- Advantages & Disadvantages Of Inheritance In Python
- Common Use Cases For Inheritance In Python
- Best Practices for Implementing Inheritance in Python
- Avoiding Common Pitfalls in Python Inheritance
- Conclusion
- Frequently Asked Questions
- 💡 Python Inheritance Quiz – Are You Ready?
Table of content:
- What Is The Python List append() Method?
- Adding Elements To A Python List Using append()
- Populate A Python List Using append()
- Adding Different Data Types To Python List Using append()
- Adding A List To Python List Using append()
- Nested Lists With Python List append() Method
- Practical Use Cases Of Python List append() Method
- How append() Method Affects List Performance
- Avoiding Common Mistakes When Using Python List append()
- Comparing extend() With append() Python List Method
- Conclusion
- Frequently Asked Questions
- 🧠 Think You Know Python List append()? Take A Quiz!
Table of content:
- What Is A Linked List In Python?
- Types Of Linked Lists In Python
- How To Create A Linked List In Python
- How To Traverse A Linked List In Python & Retrieve Elements
- Inserting Elements In A Linked List In Python
- Deleting Elements From A Linked List In Python
- Update A Node Of Linked List In Python
- Reversing A Linked List In Python
- Calculating Length Of A Linked List In Python
- Comparing Arrays And Linked Lists In Python
- Advantages & Disadvantages Of Linked List In Python
- When To Use Linked Lists Over Other Data Structures
- Practical Applications Of Linked Lists In Python
- Conclusion
- Frequently Asked Questions
- 🔗 Linked List Logic: Can You Ace This Quiz?
Table of content:
- What Is Extend In Python?
- Extend In Python With List
- Extend In Python With String
- Extend In Python With Tuple
- Extend In Python With Set
- Extend In Python With Dictionary
- Other Methods To Extend A List In Python
- Difference Between append() and extend() In Python
- Conclusion
- Frequently Asked Questions
- Think You Know extend() In Python? Prove It!
Table of content:
- What Is Recursion In Python?
- Key Components Of Recursive Functions In Python
- Implementing Recursion In Python
- Recursion Vs. Iteration In Python
- Tail Recursion In Python
- Infinite Recursion In Python
- Advantages Of Recursion In Python
- Disadvantages Of Recursion In Python
- Best Practices For Using Recursion In Python
- Conclusion
- Frequently Asked Questions
- Recursive Thinking In Python: Test Your Skills!
Table of content:
- What Is Type Conversion In Python?
- Types Of Type Conversion In Python
- Implicit Type Conversion In Python
- Explicit Type Conversion In Python
- Functions Used For Explicit Data Type Conversion In Python
- Important Type Conversion Tips In Python
- Benefits Of Type Conversion In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Type Conversion? Take A Quiz!
Table of content:
- What Is Scope In Python?
- Local Scope In Python
- Global Scope In Python
- Nonlocal (Enclosing) Scope In Python
- Built-In Scope In Python
- The LEGB Rule For Python Scope
- Python Scope And Variable Lifetime
- Best Practices For Managing Python Scope
- Conclusion
- Frequently Asked Questions
- Think You Know Python Scope? Test Yourself!
Table of content:
- Understanding The Continue Statement In Python
- How Does Continue Statement Work In Python?
- Python Continue Statement With For Loops
- Python Continue Statement With While Loops
- Python Continue Statement With Nested Loops
- Python Continue With If-Else Statement
- Difference Between Pass and Continue Statement In Python
- Practical Applications Of Continue Statement In Python
- Conclusion
- Frequently Asked Questions
- Python 'continue' Statement Quiz: Can You Ace It?
Table of content:
- What Are Control Statements In Python?
- Types Of Control Statements In Python
- Conditional Control Statements In Python
- Loop Control Statements In Python
- Control Flow Altering Statements In Python
- Exception Handling Control Statements In Python
- Conclusion
- Frequently Asked Questions
- Mastering Control Statements In Python – Take the Quiz!
Table of content:
- Difference Between Mutable And Immutable Data Types in Python
- What Is Mutable Data Type In Python?
- Types Of Mutable Data Types In Python
- What Are Immutable Data Types In Python?
- Types Of Immutable Data Types In Python
- Key Similarities Between Mutable And Immutable Data Types In Python
- When To Use Mutable Vs Immutable In Python?
- Conclusion
- Frequently Asked Questions
- Quiz Time: Mutable vs. Immutable In Python!
Table of content:
- What Is A List?
- What Is A Tuple?
- Difference Between List And Tuple In Python (Comparison Table)
- Syntax Difference Between List And Tuple In Python
- Mutability Difference Between List And Tuple In Python
- Other Difference Between List And Tuple In Python
- List Vs. Tuple In Python | Methods
- When To Use Tuples Over Lists?
- Key Similarities Between Tuples And Lists In Python
- Conclusion
- Frequently Asked Questions
- 🧐 Lists vs. Tuples Quiz: Test Your Python Knowledge!
Table of content:
- Introduction to Python
- Downloading & Installing Python, IDLE, Tkinter, NumPy & PyGame
- Creating A New Python Project
- How To Write Python Hello World Program In Python?
- Way To Write The Hello, World! Program In Python
- The Hello, World! Program In Python Using Class
- The Hello, World! Program In Python Using Function
- Print Hello World 5 Times Using A For Loop
- Conclusion
- Frequently Asked Questions
- 👋 Python's 'Hello, World!'—How Well Do You Know It?
Table of content:
- Algorithm Of Python Program To Add To Numbers
- Standard Program To Add Two Numbers In Python
- Python Program To Add Two Numbers With User-defined Input
- The add() Method In Python Program To Add Two Numbers
- Python Program To Add Two Numbers Using Lambda
- Python Program To Add Two Numbers Using Function
- Python Program To Add Two Numbers Using Recursion
- Python Program To Add Two Numbers Using Class
- How To Add Multiple Numbers In Python?
- Add Multiple Numbers In Python With User Input
- Time Complexities Of Python Programs To Add Two Numbers
- Conclusion
- Frequently Asked Questions
- 💡 Quiz Time: Python Addition Basics!
Table of content:
- Swapping in Python
- Swapping Two Variables Using A Temporary Variable
- Swapping Two Variables Using The Comma Operator In Python
- Swapping Two Variables Using The Arithmetic Operators (+,-)
- Swapping Two Variables Using The Arithmetic Operators (*,/)
- Swapping Two Variables Using The XOR(^) Operator
- Swapping Two Variables Using Bitwise Addition and Subtraction
- Swap Variables In A List
- Conclusion
- Frequently Asked Questions (FAQs)
- Quiz To Test Your Variable Swapping Knowledge
Table of content:
- What Is A Quadratic Equation? How To Solve It?
- How To Write A Python Program To Solve Quadratic Equations?
- Python Program To Solve Quadratic Equations Directly Using The Formula
- Python Program To Solve Quadratic Equations Using The Complex Math Module
- Python Program To Solve Quadratic Equations Using Functions
- Python Program To Solve Quadratic Equations & Find Number Of Solutions
- Python Program To Plot Quadratic Functions
- Conclusion
- Frequently Asked Questions
- Quadratic Equations In Python Quiz: Test Your Knowledge!
Table of content:
- What Is Decimal Number System?
- What Is Binary Number System?
- What Is Octal Number System?
- What Is Hexadecimal Number System?
- Python Program to Convert Decimal to Binary, Octal, And Hexadecimal Using Built-In Function
- Python Program To Convert Decimal To Binary Using Recursion
- Python Program To Convert Decimal To Octal Using Recursion
- Python Program To Convert Decimal To Hexadecimal Using Recursion
- Python Program To Convert Decimal To Binary Using While Loop
- Python Program To Convert Decimal To Octal Using While Loop
- Python Program To Convert Decimal To Hexadecimal Using While Loop
- Convert Decimal To Binary, Octal, And Hexadecimal Using String Formatting
- Python Program To Convert Binary, Octal, And Hexadecimal String To A Number
- Complexity Comparison Of Python Programs To Convert Decimal To Binary, Octal, And Hexadecimal
- Conclusion
- Frequently Asked Questions
- 💡 Decimal To Binary, Octal & Hex: Quiz Time!
Table of content:
- What Is A Square Root?
- Python Program To Find The Square Root Of A Number
- The pow() Function In Python Program To Find The Square Root Of Given Number
- Python Program To Find Square Root Using The sqrt() Function
- The cmath Module & Python Program To Find The Square Root Of A Number
- Python Program To Find Square Root Using The Exponent Operator (**)
- Python Program To Find Square Root With A User-Defined Function
- Python Program To Find Square Root Using A Class
- Python Program To Find Square Root Using Binary Search
- Python Program To Find Square Root Using NumPy Module
- Conclusion
- Frequently Asked Questions
- 🤓 Think You Know Square Roots In Python? Take A Quiz!
Table of content:
- Understanding the Logic Behind the Conversion of Kilometers to Miles
- Steps To Write Python Program To Convert Kilometers To Miles
- Python Program To Convert Kilometer To Miles Without Function
- Python Program To Convert Kilometer To Miles Using Function
- Python Program to Convert Kilometer To Miles Using Class
- Tips For Writing Python Program To Convert Kilometer To Miles
- Conclusion
- Frequently Asked Questions
- 🧐 Mastered Kilometer To Mile Conversion? Prove It!
Table of content:
- Why Build A Calculator Program In Python?
- Prerequisites To Writing A Calculator Program In Python
- Approach For Writing A Calculator Program In Python
- Simple Calculator Program In Python
- Calculator Program In Python Using Functions
- Creating GUI Calculator Program In Python Using Tkinter
- Conclusion
- Frequently Asked Questions
- 🧮 Calculator Program In Python Quiz!
Table of content:
- The Calendar Module In Python
- Prerequisites For Writing A Calendar Program In Python
- How To Write And Print A Calendar Program In Python
- Calendar Program In Python To Display A Month
- Calendar Program In Python To Display A Year
- Conclusion
- Frequently Asked Questions
- Calendar Program In Python – Quiz Time!
Table of content:
- What Is The Fibonacci Series?
- Pseudocode Code For Fibonacci Series Program In Python
- Generating Fibonacci Series In Python Using Naive Approach (While Loop)
- Fibonacci Series Program In Python Using The Direct Formula
- How To Generate Fibonacci Series In Python Using Recursion?
- Generating Fibonacci Series In Python With Dynamic Programming
- Fibonacci Series Program In Python Using For Loop
- Generating Fibonacci Series In Python Using If-Else Statement
- Generating Fibonacci Series In Python Using Arrays
- Generating Fibonacci Series In Python Using Cache
- Generating Fibonacci Series In Python Using Backtracking
- Fibonacci Series In Python Using Power Of Matix
- Complexity Analysis For Fibonacci Series Programs In Python
- Applications Of Fibonacci Series In Python & Programming
- Conclusion
- Frequently Asked Questions
- 🤔 Think You Know Fibonacci Series? Take A Quiz!
Table of content:
- Different Ways To Write Random Number Generator Python Programs
- Random Module To Write Random Number Generator Python Programs
- The Numpy Module To Write Random Number Generator Python Programs
- The Secrets Module To Write Random Number Generator Python Programs
- Understanding Randomness and Pseudo-Randomness In Python
- Common Issues and Solutions in Random Number Generation
- Applications of Random Number Generator Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python's Random Module? Prove It!
Table of content:
- What Is A Factorial?
- Algorithm Of Program To Find Factorial Of A Number In Python
- Pseudocode For Factorial Program in Python
- Factorial Program In Python Using For Loop
- Factorial Program In Python Using Recursion
- Factorial Program In Python Using While Loop
- Factorial Program In Python Using If-Else Statement
- The math Module | Factorial Program In Python Using Built-In Factorial() Function
- Python Program to Find Factorial of a Number Using Ternary Operator(One Line Solution)
- Python Program For Factorial Using Prime Factorization Method
- NumPy Module | Factorial Program In Python Using numpy.prod() Function
- Complexity Analysis Of Factorial Programs In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Factorials In Python? Take A Quiz!
Table of content:
- What Is Palindrome In Python?
- Check Palindrome In Python Using While Loop (Iterative Approach)
- Check Palindrome In Python Using For Loop And Character Matching
- Check Palindrome In Python Using The Reverse And Compare Method (Python Slicing)
- Check Palindrome In Python Using The In-built reversed() And join() Methods
- Check Palindrome In Python Using Recursion Method
- Check Palindrome In Python Using Flag
- Check Palindrome In Python Using One Extra Variable
- Check Palindrome In Python By Building Reverse, One Character At A Time
- Complexity Analysis For Palindrome Programs In Python
- Real-World Applications Of Palindrome In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Palindromes? Take A Quiz!
Table of content:
- Best Python Books For Beginners
- Best Python Books For Intermediate Level
- Best Python Books For Experts
- Best Python Books To Learn Algorithms
- Audiobooks of Python
- Best Books To Learn Python And Code Like A Pro
- To Learn Python Libraries
- Books To Provide Extra Edge In Python
- Python Project Ideas - Reference
- Quiz To Rehash Your Knowledge Of Python Books!
Python List sort() | All Use Cases Explained (+Code Examples)
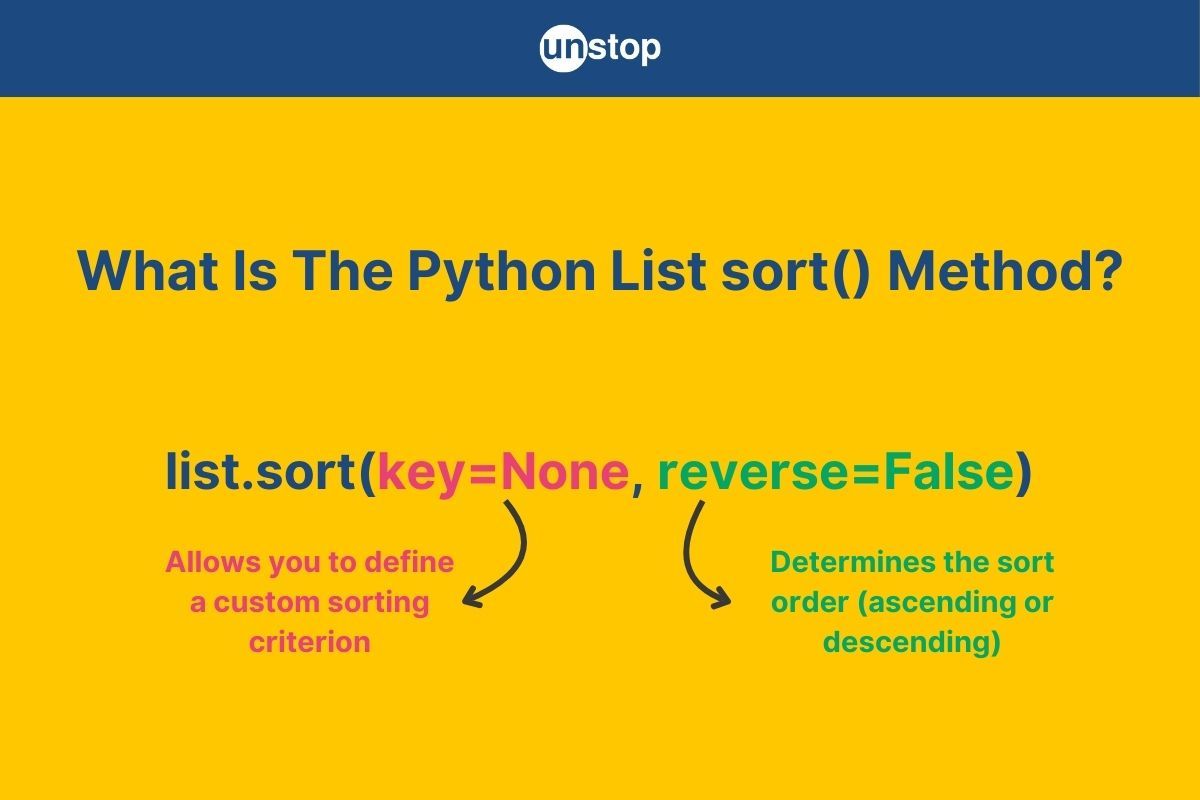
In Python, lists are ordered, mutable collections that store data of any type. Sorting is a crucial operation when you need to organize your data in a specific order, such as ascending or descending. The sort() method is a powerful tool for arranging elements of a list directly and modifying the list in place. Whether you're working with numbers, strings, or more complex structures, sort() makes it easy to organize data.
In this article, we’ll explore the Python sort() list method, its syntax, usage, and how it compares to the sorted() function, giving you the tools to sort lists efficiently.
What Is the Python sort() List Method?
The sort() method is a built-in Python function specifically designed for sorting lists in place. This means that it arranges the elements of a list in a defined order (either ascending or descending), modifying the original list rather than creating a new one.
The sort() method in Python programming can sort lists containing numbers, strings, or any objects that have a defined comparison order. It’s a handy tool when you need to maintain the order of items in a list but organize them according to a specific criterion.
Here’s a quick breakdown of what makes sort() useful:
- In-place sorting: It sorts the list without creating a new one. The list is directly modified.
- Sorting options: You can sort in ascending or descending order.
- Custom sorting: It allows you to define custom sorting criteria using the key parameter, which can be especially useful for more complex data.
In the following sections, we'll break down how to use the sort() method, explore its syntax and parameters, and show how you can use it for different kinds of sorting tasks.
Syntax of Python sort() List Method
list.sort(key=None, reverse=False)
Python sort() List Method Parameters
The sort() method accepts two optional parameters:
key: The key parameter allows you to define a custom sorting criterion by passing a function.
- This function is applied to each element before sorting.
- If you don’t specify a key, Python will use the elements themselves for sorting.
- Example: If you have a list of strings and want to sort based on their lengths, you can use the key parameter with len.
reverse: The reverse parameter is a boolean that determines the sort order.
- By default, it’s set to False, meaning the list is sorted in ascending order.
- Setting it to True sorts the list in descending order.
These parameters provide flexibility to sort lists based on custom rules or in the opposite order, making the sort() function in Python more adaptable to your needs.
Python sort() List Method Return Value
The sort() method modifies the original list and does not return a new list. In fact, it returns None, which is an important distinction compared to the sorted() function, which returns a new sorted list.
This in-place sorting is more memory efficient since it doesn’t create a duplicate list. Instead, the existing list is rearranged according to the specified sorting order.
Also read: Python List | Everything You Need To Know (With Detailed Examples)
Sorting In Ascending Order Using The Python sort() List Method
By default, the Python sort() list method sorts a list in ascending order, meaning the smallest values (for numbers) or the lexicographically first items (for strings) come first. This is the most common way to sort a list when you want the elements arranged from the lowest to the highest.
How it works: When you call the sort() method without any parameters, it will automatically sort the list in ascending order. The simple Python program example below illustrates the same.
Code Example:
#Creating a list
numbers = [4, 2, 9, 1, 5]
#Calling the sort() function
numbers.sort()
print(numbers)
Output:
[1, 2, 4, 5, 9]
Code Explanation:
In the simple Python code example:
- We begin by creating a list, numbers, with five integer values.
- Then, we call the sort() method on the numbers list. It rearranges the list elements in ascending order, with the smallest number 1 at the front and the largest number 9 at the end.
- The function directly modifies the list, which we then display using the print() function.
How To Sort Items In Descending Order Using Python sort() List Method
To sort a list in descending order (from highest to lowest), you can use the reverse=True parameter in the sort() method. This tells Python to sort the list in reverse order, meaning larger values come first.
How it works: By adding the reverse=True parameter, you change the sorting order from ascending to descending. The basic Python program example below illustrates the same.
Code Example:
numbers = [4, 2, 9, 1, 5]
#Calling sort() method with reverse parameter set to True
numbers.sort(reverse=True)
print(numbers)
Output:
[9, 5, 4, 2, 1]
Code Example:
In the basic Python code example:
- We create a list, numbers and then call sort() function on it.
- Here, we set the reverse parameter to True, and as a result, the method sorts the list in descending order. The largest number, 9, comes first, followed by 5, 4, and so on.
- Then, we print it to the console to show descended sorting.
Check out this amazing course to become the best version of the Python programmer you can be.
Custom Sorting Using The Key Parameter Of Python sort() List Method
One of the powerful features of the sort() method is the ability to specify a custom/ user-defined sorting criterion through the key parameter. This allows you to sort your list based on any function or rule you define rather than just the default order.
How it works: The key parameter accepts a function (or other callable) that takes a single argument and returns a value to be used for sorting purposes.
- This function is applied to each element in the iterable before the sorting operation.
- By default, if no key is provided, Python will use the element itself for comparison (like how numbers are sorted numerically or strings lexicographically).
This functionality is particularly useful in more complex scenarios like sorting lists of objects by specific attributes or sorting strings in a custom order. In this section, we will explore the various ways we can customise Python’s sort() list method using the key parameter.
Sorting Strings By Length Using The Python sort() List Method
Let’s say we want to sort a list of words based on their length rather than their lexicographical order. In this case, you can pass the built-in len() function to the key parameter, which ensures that list elements are sort based on their length. The Python program example below illustrates the same.
Code Example:
words = ['apple', 'banana', 'cherry', 'date']
#Passing the len() function as key to sort on basis of element length
words.sort(key=len)
print(words)
Output:
['date', 'apple', 'cherry', 'banana']
Code Explanation:
In this example:
- We first define a list– words, containing four string elements: ['apple', 'banana', 'cherry', 'date'].
- Then, we call the sort() function on it, passing the len() function as the key argument.
- This len() function calculates the length of each string/ word, which is used to sort the list.
- The result is a list sorted from the shortest word ('date') to the longest word ('banana').
Sorting Strings Case-Insensitively Using The Python sort() List Method
When sorting a list of strings in Python, the default behavior is case-sensitive, meaning uppercase letters are ordered before lowercase ones. However, in many situations, you may want to perform a case-insensitive sort, where 'apple' and 'Apple' are treated as equal.
- To achieve this, you can use the str.casefold() method, which is specifically designed to handle case-insensitive comparisons of strings, etc.
- It converts all characters to lowercase in a way that is more comprehensive than str.lower(), making it ideal for sorting strings regardless of their original casing.
Look at the Python code example below, which illustrates how this is done.
Code Example:
words = ['apple', 'Banana', 'cherry', 'Date']
#Using str.casefold to ensure case-insensitive sorting
words.sort(key=str.casefold)
print(words)
Output:
['apple', 'Banana', 'cherry', 'Date']
Code Explanation:
In the example,
- We create a list that contains strings with mixed case: ['apple', 'Banana', 'cherry', 'Date'].
- Then, we call the sort() function on the list, passing the str.casefold() method as the key function.
- This method converts all string characters to lowercase before sorting, ensuring a case-insensitive order.
- The result is that strings are sorted without considering the case, with 'apple' coming first because it’s lexicographically the smallest when the case is ignored.
Sorting Tuples By Specific Element With The Python sort() List Method
When working with a list of tuples, sorting by specific elements inside the tuple can be extremely useful. For example, if you have a list of student records where each tuple contains a name, grade, and age, you might want to sort the list by age instead of name.
- One way to get this done with the Python sort() list method is to pass a lambda function as the key.
- The lambda function is ideal in this scenario. It allows you to define a function on the fly that extracts a specific element from each tuple to be used as the sorting key.
- By passing the lambda function to the key parameter, you can control which element of the tuple is used to determine the order.
This approach works particularly well when you need to sort complex data structures like tuples or lists of dictionaries, providing you with great flexibility in how you sort them. The example Python program below illustrates how this can be done.
Code Example:
students = [('Aarti', 'A', 15), ('Raj', 'B', 12), ('Simran', 'B', 10)]
#Sorting the list on the basis of the age
students.sort(key=lambda student: student[2])
print(students)
Output:
[('Simran', 'B', 10), ('Raj', 'B', 12), ('Aarti', 'A', 15)]
Code Explanation:
In the example:
- We begin by defining a list of tuples called students, where each tuple contains a name, grade, and age.
- Then, we call the sort() function on this list and define a lambda function to pass as the key argument.
- The lambda function extracts the age (third element) from each tuple, and the list is sorted by age.
- This sorts the list in reference to the order of tuples by age in ascending order, starting from 10 years old (Dave) to 15 years old (John).
Sorting Using Multiple Criteria In The Python sort() List Method
Sorting by multiple criteria involves sorting a list based on more than one key parameter. This is useful in cases where you want a primary sorting criterion and a secondary criterion to break ties in the order.
- To do this, the key function can return a tuple, where each element in the tuple represents a separate sorting criterion.
- Python will first sort the list based on the first element of the tuple, and if there are ties, it will use the second element to break the tie.
- This process can continue with more elements if necessary.
This method allows you to perform complex multi-level sorting, making it an essential tool for handling sophisticated data structures and ensuring that your sorting behavior matches your specific needs. The example Python code below illustrates the same.
Code Example:
items = [('apple', 2), ('banana', 1), ('cherry', 2), ('date', 1)]
#Sorting the list with multiple criteria
items.sort(key=lambda item: (item[1], item[0]))
print(items)
Output:
[('banana', 1), ('date', 1), ('apple', 2), ('cherry', 2)]
Code Explanation:
- We first define a list containing tuples where the first element is a fruit and the second element is a number.
- Then, we call the sort function, passing a lambda function as key argument.
- This function returns a tuple with two elements: the second element of each tuple (the number) and the first element (the fruit name).
- The list is first sorted by the number, and in case of a tie (same number), it is sorted by the fruit name.
- The result is that items are sorted first by their number, and then alphabetically by the fruit name when the numbers are the same.
Custom Key Functions In The Python sort() List Method
While Python libraries provide many built-in functions for sorting, you can also define your own custom functions to control how the elements in your list are compared.
- A custom key function allows you to implement more specific or complex sorting logic that isn’t covered by the default behavior.
- The custom function should take an element from the list as input and return a value that will be used for comparison.
- For example, you could create a custom function that sorts a list of tuples based on a specific element, or even by performing some transformation or calculation on each element before sorting.
Custom key functions provide maximum flexibility, allowing you to tailor the sorting behavior exactly to your needs, whether you’re sorting by complex criteria or applying specific rules to each element.
Code Example:
#Defining the custom key function
def custom_key(item):
return item[1] # Sort by the second element
#Creating a list with tuples
items = [('apple', 2), ('banana', 1), ('cherry', 3)]
#Sorting the list using the custom key
items.sort(key=custom_key)
print(items)
Output:
[('banana', 1), ('apple', 2), ('cherry', 3)]
Code Explanation:
In this example:
- We define a custom function custom_key(), which returns the second element of each tuple in the list.
- When calling sort(), we pass custom_key as the key function.
- The list is sorted based on the second element of each tuple, resulting in the list being sorted from the smallest to the largest second element.
Level up your coding skills with the 100-Day Coding Sprint at Unstop and get the bragging rights now!
Examples Of Python sort() List Method
Let’s go through some practical examples to demonstrate how the sort() method works with different types of data.
Sorting List Numbers Using Python sort() List Method
Sorting a list of numbers is one of the most common use cases for the sort() method. By default, the list will be sorted in ascending order.
Example:
numbers = [8, 1, 3, 9, 5, 2]
numbers.sort() # Sort in ascending order
print(numbers) # Output: [1, 2, 3, 5, 8, 9]
Here, the sort() method rearranges the numbers in ascending order, from the smallest (1) to the largest (9).
Sorting Complex Data Structures Using Python sort() List Method
The sort() method is not limited to simple lists of numbers or strings. It can be used to sort more complex data structures, such as lists of tuples or dictionaries.
Example: Sorting a List of Tuples
Let’s say we have a list of tuples, and we want to sort them based on the second element of each tuple.
Code Example:
items = [('apple', 2), ('banana', 1), ('cherry', 3)]
#Sorting the list of tuples based on second element
items.sort(key=lambda x: x[1])
#Printing the sorted list
print(items)
Output:
[('banana', 1), ('apple', 2), ('cherry', 3)]
Here, we use a lambda function to sort the list of tuples based on the second element. The list is sorted in ascending order by the numbers in each tuple.
Example: Sorting a List of Dictionaries
You can also sort a list of dictionaries based on a specific key in each dictionary.
Code Example:
#Creating a list of dictionaries
students = [{'name': 'Ajay', 'age': 25}, {'name': 'Bijay', 'age': 22}, {'name': 'Digvijay', 'age': 24}]
#Sorting the list by age as key
students.sort(key=lambda x: x['age'])
print(students)
Output:
[{'name': 'Bijay', 'age': 22}, {'name': 'Digvijay', 'age': 24}, {'name': 'Ajay', 'age': 25}]
In this example, the list of dictionaries is sorted based on the 'age' value in each dictionary, from the youngest to the oldest.
What Is The sorted() List Method In Python
While the sort() method is used to sort a list in place, sorted() is a built-in function that returns a new list containing the sorted elements. The original list remains unchanged, making it more suitable when you need to retain the original data and work with a sorted version of it.
The sorted() function can be used on any iterable (like lists, tuples, dictionaries, etc.), not just lists, and it also accepts the same parameters as sort() for customizing the sort order.
Syntax Of sorted() Function
sorted(iterable, key=None, reverse=False)
Parameters:
- iterable: The collection (list, tuple, dictionary, etc.) to be sorted. Unlike sort(), which can only be used with lists, sorted() works with any iterable.
- key (optional): A function that serves as a sorting criterion. By default, the elements themselves are sorted.
- reverse (optional): A boolean that determines whether the sorting should be in descending order (True) or ascending order (False, default).
Return Value: The function returns a new list containing the sorted elements of the given iterable without altering the original iterable.
Looking for guidance? Find the perfect mentor from select experienced coding & software development experts here.
Differences Between sorted() And sort() List Methods In Python
Both sort() and sorted() are used for sorting, but they have important differences that can influence how and when you should use them. Here’s a comparison table to highlight the key differences:
Feature |
sort() |
sorted() |
Return Value |
Modifies the list in place; returns None. |
Returns a new sorted list; the original remains unchanged. |
Usage |
Can only be used with lists. |
Can be used with any iterable (lists, tuples, strings, etc.). |
Modification |
Changes the original list. |
Does not modify the original iterable. |
Time Complexity |
O(n log n) |
O(n log n) |
Memory Usage |
Modifies the list in place, no additional space needed. |
Creates a new sorted list, consuming more memory. |
When To Use sorted() & When To Use sort() List Method In Python
Choosing between sort() and sorted() depends on whether you want to modify the original list or if you need a new sorted list. Here's a breakdown of when to use each:
1. When to Use sort():
- Modify the original list: If you don't need to retain the original order of the list, use sort(). It sorts the list in place and does not return a new list.
- When working with a list: The Python sort() list method is exclusive to lists, so it’s used when you specifically need to work with a list.
2. When to Use sorted():
- Preserve the original list: If you need to keep the original list unchanged and get a new sorted list, use sorted(). This function returns a new list while leaving the original one intact.
- For other iterables: The sorted() method works with any iterable, including tuples and strings, not just lists. It’s more versatile than sort() in that regard.
Example Of Using The sorted() Method
In the example below, we use the sorted() method to sort a list of numbers. Let’s see how this works.
Code Example:
numbers = [8, 3, 1, 5, 9]
# Using sorted() to create a new sorted list
sorted_numbers = sorted(numbers)
print("Original list:", numbers)
print("Sorted with sorted():", sorted_numbers)
Output:
[8, 3, 1, 5, 9]
[1, 3, 5, 8, 9]
Here, the sorted() method returns a new sorted list without modifying the original numbers list. The original list remains unchanged, and a new sorted list is returned.
Conclusion
The Python sort() list method is an efficient way to sort a list in place. Its syntax is pretty straightforward and simple to use: list.sort(key=None, reverse=False).
- It allows you to sort lists in ascending or descending order and provides flexibility through the key parameter for custom sorting.
- The sort() method modifies the original list, making it a great choice when you don't need to preserve the original order.
- Its time complexity is O(n log n), making it efficient for most use cases.
- While sorted() offers a similar function, sort() remains the go-to option when working directly with lists and when memory efficiency is a concern.
With the Python sort() list method, you can easily handle sorting tasks in your Python programs, whether you're working with numbers, strings, or more complex data structures.
Frequently Asked Questions
Q1. How do you sort a list in Python?
In Python, you can sort a list using the sort() method, which sorts the list in place. For example:
numbers = [5, 2, 9, 1, 5, 6]
numbers.sort()
print(numbers) # Output: [1, 2, 5, 5, 6, 9]
Alternatively, you can use the sorted() function, which returns a new sorted list. For example:
numbers = [5, 2, 9, 1, 5, 6]
sorted_numbers = sorted(numbers)
print(sorted_numbers) # Output: [1, 2, 5, 5, 6, 9]
Q2. Is sort() or sorted() better?
The choice between sort() and sorted() depends on your needs:
- sort(): Modifies the original list in place and returns None. It's more memory-efficient when you don't need to preserve the original list.
- sorted(): Returns a new sorted list and leaves the original list unchanged. It's useful when you need to keep the original list intact.
Q3. What does sort() mean in Python?
The sort() method in Python is a built-in function that sorts the elements of a list in ascending order by default. It modifies the list in place and does not return a new list.
Q4. What is list.sort() in Python?
The notation, list.sort() is the syntax for the sort() method available to list objects in Python. Here, list refer to the name of the list you want to sort, the sort() is the built-in Python function and the dot operator allows us to call the function on the specified list. The function sorts the elements of the list in place, meaning the original list is modified. It accepts two optional parameters: key and reverse.
Q5. Can sort() be used with other data types?
The sort() method is specifically designed for lists. If you need to sort other iterable types, such as tuples or dictionaries, you can use the sorted() function, which works with any iterable and returns a new sorted list.
Q6. What happens if the list contains different data types?
If a list contains elements of different data types, attempting to sort it using sort() or sorted() will raise a TypeError unless a custom sorting function is provided that can handle the comparisons.
Q7. How can I sort a list of tuples by the second element?
You can use the key parameter with a lambda function to specify the sorting criterion. For example:
my_list = [(1, 'one'), (3, 'three'), (2, 'two')]
my_list.sort(key=lambda x: x[1])
print(my_list) # Output: [(1, 'one'), (3, 'three'), (2, 'two')]
In this example, the Python sort() list method, sorts the input list based on the second element of each tuple.
Take A Quick Python's sort() Quiz!
Do check out the following for more amazing reads:
- Python List index() Method | Use Cases Explained (+Code Examples)
- Python String Concatenation In 10 Easy Ways Explained (+Examples)
- Nested List In Python | Initialize, Manipulate & Flatten (+Examples)
- Remove Item From Python List | 5 Ways & Comparison (+Code Examples)
- Remove Duplicates From Python List | 12 Ways With Code Examples
- Python input() Function (+Input Casting & Handling With Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Divyansh Shrivastava 3 weeks ago
Pranjul Srivastav 3 weeks ago
niyati m singh 1 month ago
Vishwajeet Singh 1 month ago
Pardha Venkata Sai Patnam 1 month ago
A Vishnuvardhan 1 month ago