Check Palindrome In Python | 8 Methods With Detailed Code Examples
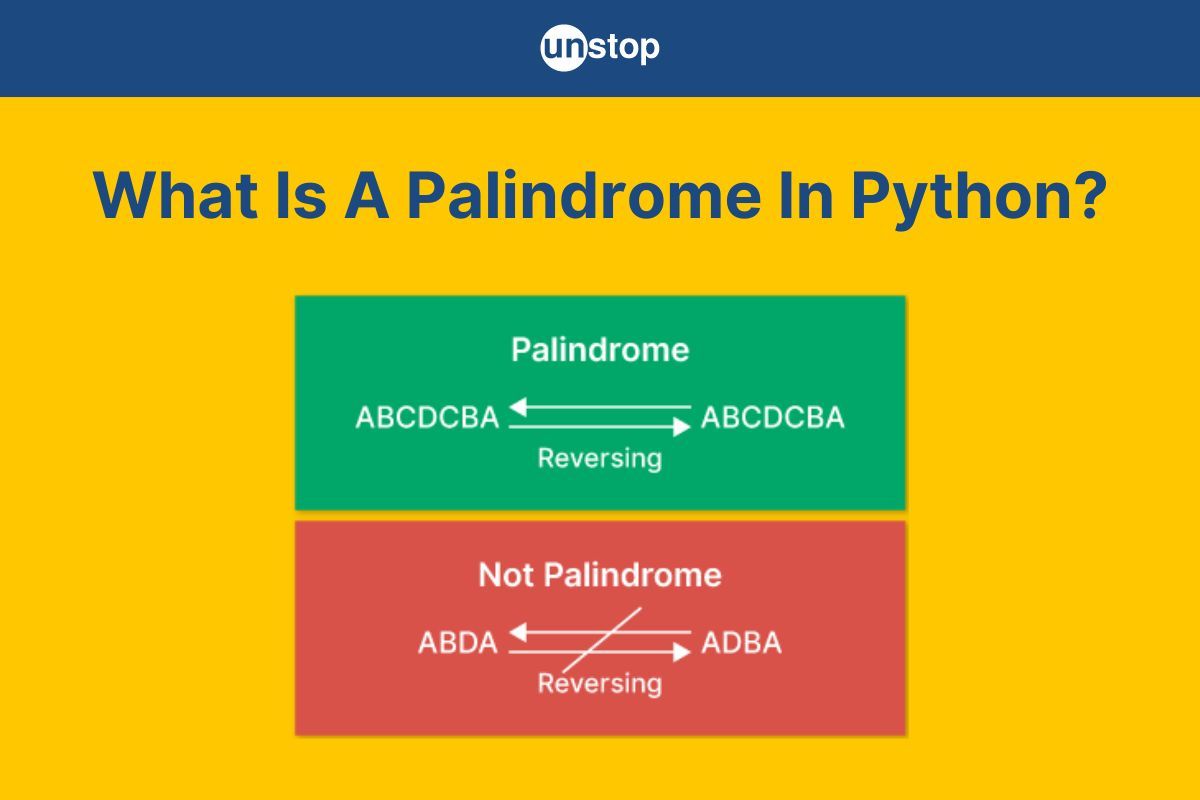
A palindrome is a text (word/ phrase) or a number that remains the same whether you read from the front or the back. This means that the reverse of a palindrome is the same as the original. For example, the word Mom and the number 1991 are both Palindromes. This concept is widely used in genetic analysis, computational theory, music, writing, etc.
In this article, we will discuss the various ways to write a program to check for a palindrome in Python language, with detailed examples. We will also highlight the real-world applications of palindromes.
What Is Palindrome In Python?
As mentioned, a palindrome is a number or text whose reverse is the same as the original content. A palindrome in Python usually refers to strings, whether a number (which must be represented in a string format) or any text. This is because palindrome checking inherently involves comparing sequences of characters (or digits) in this language.
In other words, when checking for a palindrome in Python programs, we typically compare the original string with its reversed version. If both are identical, the string is a palindrome. Python language offers various techniques to achieve this, including slicing, loops, and recursion.
For example, let's consider the word "madam":
- Original string: "madam"
- Reversed string: "madam" (when reversed, it remains the same)
Since both the original and reversed strings are identical, "madam" is a palindrome.
Algorithm To Find Palindrome In Python
Let's look at a generalized approach for finding palindrome in Python:
- Input: Accept a string or number to check if it's a palindrome.
- Preprocess: (Optional) Convert the input to lowercase or remove non-alphanumeric characters if required, especially when working with sentences or phrases.
- Reverse: Reverse the input.
- Compare: Compare the original string input with the reversed version.
- Result: If both are the same, the input is a palindrome; otherwise, it is not.
When working with palindromes in Python, there are multiple ways to approach the problem, each offering unique advantages regarding readability, performance, and simplicity. In the sections ahead, we will explore various methods to check if a given string or number is a palindrome.
Check Palindrome In Python Using While Loop (Iterative Approach)
In this method, we use a while loop to iterate over a string from beginning to end and determine if it is a palindrome in Python program. We iteratively compare the corresponding characters at both ends. If they match, the string is a palindrome; otherwise, it is not.
Working Mechanism:
- Initialize two variables, start and end, to the beginning and end of the string, respectively.
- Compare the characters at both positions using a while loop.
- If the character mismatch, exit the iterative loop and return that the string is not a palindrome.
- If all characters match, the string is a palindrome.
Code Example:
Output:
'madam' is a palindrome.
Explanation:
In the above code example-
-
We start by defining a function is_palindrome() that takes a string "word" as input.
-
Inside, we initialize two variables: start to 0 and end to the last index of the string (i.e. length of the word minus 1).
-
Then, we enter a while loop that continues as long as start is less than end. This loop will iterate through the word from both ends, comparing characters.
-
Inside the loop, using the not equal to relational operator(!=), we check if the character at index start is not equal to the character at index end-
-
If the characters don’t match, we return False since the word is not a palindrome.
-
If they do match, we move the start index forward and the end index backward to continue checking the next pair of characters.
-
Once we finish the loop without mismatches, we return True, confirming that the word is a palindrome.
-
-
Finally, we test the function with the word "madam" using an if-else statement and print the result depending on whether it is a palindrome or not.
Time Complexity: O(n)
Space Complexity: O(1)
Check Palindrome In Python Using For Loop And Character Matching
In this method, we check if a string is a palindrome by using a for loop to compare characters from the beginning and the end of the string iteratively. If the characters match in every iteration, we determine that the string is a palindrome; otherwise, it is not.
Working Mechanism:
- Take the input string.
- Loop through the string from the start to the midpoint.
- Compare the characters at the current index and the corresponding character from the end.
- If any characters don’t match, return that the string is not a palindrome.
- If all pairs match, the string is a palindrome.
Code Example:
Output:
'level' is a palindrome.
Explanation:
In the above code example-
-
We start by defining a function is_palindrome() that accepts a string "word" as its parameter.
-
Inside, we use a for loop to iterate over the first half of the string, up to the midpoint i.e. len(word) // 2.
-
In each iteration, we compare the character at the current index (word[i]) with the character at the mirrored position from the end i.e.(word[-(i + 1)])-
-
If the characters do not match, we return False, indicating that the word is not a palindrome.
-
If we complete the loop without finding any mismatches, we return True, confirming that the word is a palindrome.
-
-
Finally, we test the function with the word "level" using an if-else statement and print the result, indicating whether it is a palindrome or not.
Time Complexity: O(n)
Space Complexity: O(1)
Check Palindrome In Python Using The Reverse And Compare Method (Python Slicing)
In this native approach, we check if a string is a palindrome by reversing it and comparing it with the original string. If both the original and reversed strings are identical, then the input is a palindrome.
Here, we use the slice operator to check for the palindrome in Python program. The operator is represented by a double colon sign (::), which allows us to extract a specific portion of a string or list.
Working Mechanism:
- Take the input string.
- Reverse the string using Python's slicing technique.
- Compare the original string with the reversed string.
- If they are the same, the string is a palindrome; otherwise, it is not.
Code Example:
Output:
'racecar' is a palindrome.
Explanation:
In the above code example-
-
We start by defining a function is_palindrome() that takes a string "word" as input.
-
Inside the function, we create a new variable reversed_word, which stores the reversed version of the input string using Python slicing (word[::-1]).
-
Here, -1 is the step parameter that ensures the slice operator starts from the end of the string and moves one step back each time.
-
We then compare the original string word with reversed_word using an equality relational operator(==):
-
If both strings are equal, we return True, indicating that the word is a palindrome.
-
If they are not equal, we return False, indicating that the word is not a palindrome.
-
-
Finally, we test the function with the word "racecar" using an if-else statement and print the result, showing whether it is a palindrome or not.
Time Complexity: O(n)
Space Complexity: O(n)
Check Palindrome In Python Using The In-built reversed() And join() Methods
In this method, we write a program to check for a palindrome in Python by first using the language's predefined function reversed(). This function returns an iterator that accesses the string elements in reverse order. Then, we use the join() function to reassemble the string and compare it to the original. If they are identical, the string is a palindrome.
Working Mechanism:
- Convert the input string into a list of characters.
- Reverse the list using Python's built-in reversed() function.
- Use join() method to combine the reversed list into a string.
- Compare the original string with the reversed string.
- If they are the same, it is a palindrome string; otherwise, it is not.
Code Example:
Output:
'deified' is a palindrome.
Explanation:
In the above code example-
-
We start by defining the is_palindrome() function, which takes a string "word" as input.
-
Inside, we use Python's built-in function reversed() to create a reversed iterator over the word's characters.
-
We then use the join() function to join these reversed characters into a new string, effectively reversing the word.
-
Now we will compare the original string word with the newly created reversed_word using an equality relational operator(==):
-
If both strings are the same, we return True, indicating that the word is a palindrome.
-
If the strings do not match, we return False, meaning the word is not a palindrome.
-
-
Finally, we test the function with the word "deified" using an if-else statement and print whether it is a palindrome or not.
Time Complexity: O(n)
Space Complexity: O(n)
Check Palindrome In Python Using Recursion Method
Recursion is a technique where a function calls itself to solve smaller instances of the same problem. In this approach, we use recursion to check whether a string is a palindrome. For a palindrome check, we compare the first and last characters of the string. If they are equal, we move inward and check the next set of characters, repeating the process until we either find a mismatch or reach the centre of the string.
Working Mechanism:
- Check if the first character of the string is the same as the last one.
- If the characters don't match, the string is not a palindrome, so return False.
- If the characters are equal, recursively call the function again with the substring obtained by removing the first and last characters.
- The recursion continues until the string becomes empty or has only one character left. In either case, the string is a palindrome, so return True (base case).
Code Example:
Output:
'madam' is a palindrome.
Explanation:
In the above code example-
- We start by defining the is_palindrome() function, which takes a string "word" as input.
- Inside the function, we first check the base case, i.e., if the string is either empty or has only one character, we return True since such strings are always palindromes.
- If the string is longer, we compare the first character (word[0]) with the last character (word[-1]). Here-
- If the characters match, we recursively call the is_palindrome() function on the substring between them (excluding the first and last characters). This effectively narrows down the string from both ends. The process repeats recursively until the base case is met.
- If the respective characters don't match (in any iteration), the function returns False, indicating that the string is not a palindrome.
- Finally, we test the function with the word "madam" using an if-else statement and print whether it is a palindrome based on the function's result.
Time Complexity: O(n)
Space Complexity: O(n)
Check Palindrome In Python Using Flag
In this method, we use a boolean variable flag with a for loop to compare characters and determine if a string is a palindrome. The flag helps track whether the string satisfies the condition for palindrome.
We initially set a flag to True, assuming the string is a palindrome. Then, we iterate over the string, comparing characters from the beginning and corresponding end. At the first instance of a mismatch, we set the flag to False and terminate the loop, indicating that the string is not a palindrome.
Working Mechanism:
- Initialize a flag variable as True.
- Loop over the first half of the string.
- Compare characters from the start and end of the string.
- If any pair of characters doesn’t match, set the flag to False and break out of the loop.
- After the loop, check the value of the flag. If it is True, the string is a palindrome; otherwise, it is not.
Code Example:
Output:
'radar' is a palindrome.
Explanation:
In the above code-
- We start by defining the is_palindrome function(), which takes a string "word" as input.
- Inside, we initialize a variable flag to True, assuming the word is a palindrome at the start.
- We then use a for loop to iterate through the first half of the string (i.e., range len(word)//2) since we only need to check half of the characters.
- In each iteration, we use an if-statement to compare the character at the current index (word[i]) with the corresponding character from the end of the string (word[-(i + 1)]).
- If any pair of characters doesn't match, we set flag to False to indicate that the word is not a palindrome.
- We then encounter the break statement inside the if-block and break out of the loop to stop further comparisons.
- After the loop completes, we return the value of flag, which will be True if all characters matched, or False if there is a mismatch.
- Finally, in the test section, we check the word "radar" using an if-else statement and print whether it is a palindrome or not, based on the result of the function.
Time Complexity: O(n)
Space Complexity: O(1)
Check Palindrome In Python Using One Extra Variable
In this method, we check if a string is a palindrome using an additional variable to store the reversed version of the original string. We manually construct the reversed string by iterating through the original string, appending each character to the new variable in reverse order, and then comparing the two.
Working Mechanism:
- Initialize an empty variable to hold the reversed string.
- Iterate through the original string from the end to the beginning.
- In each iteration, append the current character to the new variable.
- After constructing the reversed string, compare it with the original string.
- If they match, return True, indicating the string is a palindrome; otherwise, return False.
Code Example:
Output:
Palindrome
Explanation:
In the above code example-
- We start by defining the checkPalindrome() function, which takes a string "str" as input.
- Inside, we initialize an empty variable reversed_str to store the reversed version of the input string.
- We then use a for loop to iterate through the original string from the end to the beginning (reverse order).
- The range(len(str) - 1, -1, -1) part specifies the range of indices to iterate over.
- Here, len(str)-1 marks the starting index, which is the index of the last character.
- Following this, -1 and -1 mark the ending index (the index of the first character) and the step (
- which indicates that we should iterate backwards), respectively.
- In every iteration,, we append the character to the reversed_str using the compound addition assignment operator (+=). This effectively reverses the order of the characters.
- After the loop reverses the string, we use an if-statement to compare the original string str with the reversed string reversed_str. If they are equal, it means the string is a palindrome, so we return True. Otherwise, we return False.
- In the main function, we assign the string "level" to the variable s and then call the checkPalindrome() function with s as the input.
- If the function returns True, we print "Palindrome". Otherwise, we print "Not Palindrome".
Time Complexity: O(n)
Space Complexity: O(n)
Check Palindrome In Python By Building Reverse, One Character At A Time
This method is similar to the extra variable method above. But here, we focus on constructing the reversed string incrementally, one character at a time, by prepending each character to the reverse string. Instead of appending characters to the end of the reverse string, in this method, we will insert each character at the beginning of the reversed string to build it in reverse order.
Working Mechanism:
- Initialize an empty string to store the reversed version of the input.
- Iterate through each character of the original string.
- For each character, prepend it (i.e., add it to the front) to the reversed string.
- After constructing the reversed string, compare it with the original string.
- If both are identical, the string is a palindrome; otherwise, it is not.
Code Example:
Output:
Palindrome
Explanation:
In the above code example-
- We start by defining the checkPalindrome() function, which takes a string "str" as input.
- Inside the function, we initialize an empty string reversed_str to store the reversed version of the input.
- We then iterate over each character in the original string using a for loop.
- Now, we prepend each character to reversed_str using the arithmetic operator(+). This effectively reverses the order of the characters step by step.
- After iterating over all characters, we compare the original string str with the reversed string reversed_str.
- If the original string matches the reversed string, we return True, indicating the string is a palindrome; otherwise, we return False.
- Next, in the main function, we assign the string "radar" to the variable s. We then call the checkPalindrome() function with s as the input.
- If the function returns True, we print "Palindrome". Otherwise, we print "Not Palindrome".
Time Complexity: O(n)
Space Complexity: O(n)
Complexity Analysis For Palindrome Programs In Python
The table below compares the time and space complexities of all the implementation techniques discussed above to check for a palindrome in Python programs:
Methods For Checking Palindrome In Python |
Time Complexity |
Space Complexity |
Using While Loop (Iterative Approach) | O(n) | O(1) |
Using For Loop And Character Matching | O(n) | O(1) |
Using The Reverse And Compare Method (Python Slicing) | O(n) | O(n) |
Using The Reverse And Join Method | O(n) | O(n) |
Using Recursion | O(n) | O(n) |
Using Flag | O(n) | O(1) |
Using One Extra Variable | O(n) | O(n) |
Building Reverse One Character At A Time | O(n) | O(n) |
Real-World Applications Of Palindrome In Python
Palindromes may seem like a fun programming skill challenge, but they also have various practical applications in the real world. Here are some key areas where palindrome concepts are applied:
- Data Validation: Palindromes are often used in data validation processes to ensure consistency, data security and correctness.
- DNA and Genomics: Palindromic sequences help form important DNA structures like hairpins in genetic research.
- Cryptography: Symmetrical palindromic patterns are used in some encryption and decryption algorithms.
- Text Processing: Palindrome detection aids in Natural language processing (NLP) tasks like pattern recognition and text searching.
- Error Detection: Palindromes help identify and correct errors in transmission via symmetrical patterns.
- Automata Theory: In computer science, palindromes are often studied in finite automata to recognize symmetrical input in formal languages.
- Network Routing: Certain routing algorithms use palindromes for reliable bidirectional data transmission.
Conclusion
Palindrome in Python is a basic programming concept that offers a simple yet powerful way to explore string manipulation and control flow logic. Whether using string slicing, loops, or built-in functions, Python provides multiple methods to efficiently verify if a word or number is a palindrome. Understanding these approaches to check for a palindrome in Python programs will improve your comprehension of core programming concepts such as string handling, iteration, and recursion and help you solve other problems.
Frequently Asked Questions
Q. How can I check if a string is a palindrome in Python?
You can check if a string is a palindrome by comparing it to its reverse. In Python, this can be done using string slicing:
def is_palindrome(s):
return s == s[::-1]
Q. Are spaces and punctuation considered in palindrome checks?
It depends on the specific requirements. If you want to ignore spaces and punctuation, you can preprocess the string by removing these characters and converting it to a uniform case (such as lowercase):
import re
def is_palindrome(s):
cleaned = re.sub(r'[^A-Za-z0-9]', '', s).lower()
return cleaned == cleaned[::-1]
Q. What is a palindrome?
A palindrome is a sequence of characters—whether a word, phrase, or number—that remains the same when read both forward and backwards. Examples include "radar," "level," and "12321". In programming, palindromes are commonly used as a coding exercise to practice string manipulation and condition checking, helping developers understand how to work with sequences and improve algorithm efficiency.
Q. Can numbers be palindromes in Python?
Yes, numbers can also be palindromes. For example, the number 121 is a palindrome because reversing its digits gives the same number.
To check if a number is a palindrome in Python, one common method is to convert the number into a string and then compare it to its reverse. Here's a simple way to check if a number is a palindrome:
def is_palindrome_number(n):
num_str = str(n)
return num_str == num_str[::-1]
Q. How can I find all palindromic substrings in a given string?
To find all palindromic substrings, you can use a nested loop to check each possible substring within the given string. Here, the outer loop iterates through each character, treating it as the starting point of a potential substring, while the inner loop extends that substring to include subsequent characters.
For each substring formed, you can then check if it reads the same forwards and backwards (i.e. if it is a palindrome). This can be efficiently done by comparing the substring to its reverse. For Example-
def find_palindromic_substrings(s):
palindromes = []
for i in range(len(s)):
for j in range(i, len(s)):
substring = s[i:j+1]
if substring == substring[::-1]:
palindromes.append(substring)
return palindromes
You might also be interested in reading the following:
- Hello, World! Program In Python | 7 Easy Methods (With Examples)
- Calculator Program In Python - Explained With Code Examples
- Swap Two Variables In Python- Different Ways | Codes + Explanation
- Python Logical Operators, Short-Circuiting & More (With Examples)
- Random Number Generator Python Program (16 Ways + Code Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment