Table of content:
- What Is The Print() Function In Python?
- How Does The print() Function Work In Python?
- How To Print Single & Multi-line Strings In Python?
- How To Print Built-in Data Types In Python?
- Print() Function In Python For Values Stored In Variables
- Print() Function In Python With sep Parameter
- Print() Function In Python With end Parameter
- Print() Function In Python With flush Parameter
- Print() Function In Python With file Parameter
- How To Remove Newline From print() Function In Python?
- Use Cases Of The print() Function In Python
- Understanding Print Statement In Python 2 Vs. Python 3
- Conclusion
- Frequently Asked Questions
Print() Function In Python | Variations & Use Cases (+Code Examples)
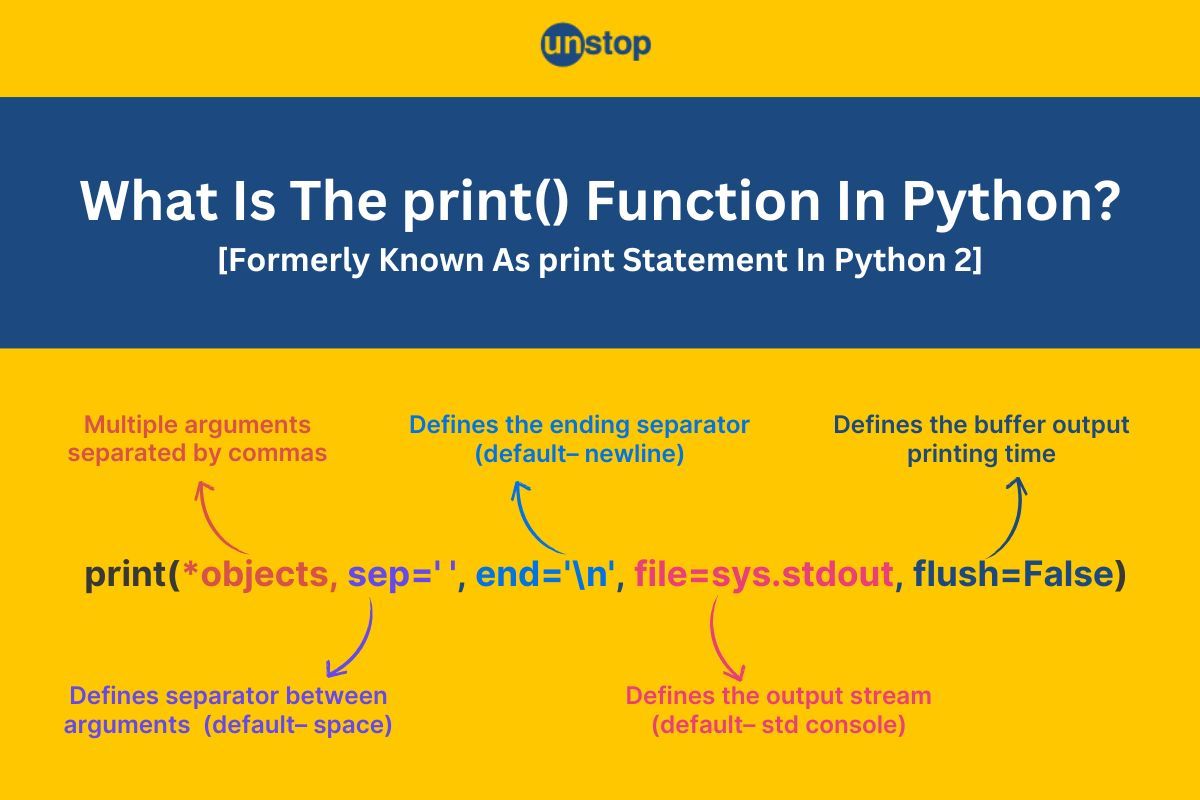
The print statement in Python is your go-to tool for displaying information on the screen. Whether you're debugging, showcasing results, or simply saying "Hello, World!", the print() function is an essential starting point for any Python developer.
In this article, we will discuss the print statement in Python language in detail, as well as its use cases, variations, and more, with the help of code examples.
What Is The Print() Function In Python?
The print() function/ statement in Python is a built-in function used to output information to the console/ standard output device. It helps programmers display data, debug code, or present results during program execution.
Whether it's a simple message, a variable's value, or a combination of multiple data types, the print function is the most common way to communicate with users or track what your program is doing. Its syntax varies from simple string literals to advanced parameters like sep, end, flush, etc.
Syntax Of The print() Function In Python
Before diving into the nuts and bolts of the print function, let’s take a quick look at its syntax.
print(*objects, sep=' ', end='\n', file=sys.stdout, flush=False)
Let's break down the different components of this syntax.
Parameters Of print Statement In Python
The print() function in Python comes with several optional parameters that let you customize its behavior. These include:
- The objects parameter refers to the multiple arguments, separated by commas that the function accepts. Each argument is converted to a string and printed.
- The separator/ sep parameter helps define a string to be inserted between arguments. By default, it is a blank space (' ').
- The End Character/ end parameter specifies what is appended at the end of the output. The default is a newline character ('\n').
- The file parameter is used to redirect the output to a file or stream instead of the default sys.stdout.
- The flush parameter takes boolean (True or False) values. When set to True, this boolean parameter forces the output to be written immediately to the file or stream, which is useful for buffering scenarios.
The parameters end, sep, flush and file are all optional as they have default values and can also be referred to as keyword arguments. We will discuss these parameters/ keyword arguments and the variations they bring to the print statement in Python programming in later sections.
Return Type Of print Statement In Python
The print() function doesn’t return any value. Instead, it returns None because its sole purpose is to display output to the console or a specified stream. Let's look at the lines of code below for a better understanding.
Code Example:
I1VzaW5nIHByaW50IHN0YXRlbWVudCB0byBvdXRwdXQgYSBzdHJpbmcgbGl0ZXJhbCBhbmQgYXNzaWduaW5nIGl0IHRvIHJlc3VsdCB2YXJpYWJsZQpyZXN1bHQgPSBwcmludCgiSGVsbG8sIFVuc3RvcHBhYmxlISIpCgojUHJpbnRpbmcgdGhlIHZhbHVlIHN0b3JlZCBpbiB0aGUgcmVzdWx0IHZhcmlhYmxlCnByaW50KHJlc3VsdCk=
Output:
Hello, Unstoppable!
None
Explanation:
This initial code example is the simplest form of the print statement in Python. Here,
- We first use the print statement to display the string– "Hello, Unstoppable!", it outputs the string to the console.
- Simultaneously, we assign the return value of the print statement to the variable result.
- When we print the value stored in this variable, it outputs None, indicating that this is the value returned by print().
How Does The print() Function Work In Python?
The print() function in Python bridges the gap between your program and the console, enabling you to display text or other data.
- Internally, print() takes the objects you pass to it, converts them to their string representation using the str() function, and sends the resulting text to the standard output (usually the console).
- When multiple arguments are provided, they are separated by the sep parameter, which defaults to a single space.
- The output automatically ends with the character defined by the end parameter, which is a newline (\n) by default.
- This newline character ensures that subsequent calls to print() begin on a new line, keeping your output neatly formatted.
Example Of print() Function In Python
Let’s see how the built-in function print() works in action. The simple Python program example below showcases various aspects of the function, including its default newline behavior and the ability to modify the separator between strings.
Code Example:
I1VzaW5nIHByaW50IHRvIGRpc3BsYXkgYSBzaW1wbGUgbWVzc2FnZQpwcmludCgiSGVsbG8sIFdvcmxkISIpCgojUHJpbnRpbmcgbXVsdGlwbGUgYXJndW1lbnRzIHNlcGFyYXRlZCBieSBkZWZhdWx0IHNwYWNlCnByaW50KCJVbnN0b3AiLCAiaXMiLCAiYXdlc29tZSEiKQoKI1ByaW50aW5nIHdpdGggYSBjdXN0b20gc2VwYXJhdG9yCnByaW50KCIyMDI0IiwgIjExIiwgIjIwIiwgc2VwPSItIikKCiNQcmludGluZyBvbiB0aGUgc2FtZSBsaW5lIHVzaW5nIGEgY3VzdG9tIGVuZCBwYXJhbWV0ZXIKcHJpbnQoIlB5dGhvbiByb2NrcyEiLCBlbmQ9IiAiKQpwcmludCgiUmlnaHQ/Iik=
Output:
Hello, World!
Unstop is awesome!
2024-11-20
Python rocks! Right?
Explanation:
In the simple Python code example,
- We first use the print() function for a single output string– "Hello, World!" enclosed in double quotes. As mentioned before, the function, by default, ends with a newline character (\n), moving the cursor to the next line.
- In the second statement, we pass three strings– "Unstop", "is", and "awesome!" –separated by commas. The statement prints these string objects with the default separator, a blank space.
- Then, in the third statement, we again pass three numbers in string format and specify a custom separator (a hyphen "-") using the sep parameter. This replaces the default single space between arguments, resulting in a formatted date.
- Next, we pass a string literal and use the end parameter set to a blank space in the print statement. This replaces the default newline character in the function and allows the next print statement to display the result in the same line.
How To Print Single & Multi-line Strings In Python?
Printing strings is one of the most basic and frequent tasks when working with Python. You can also combine multiple strings or format them for more complex use cases.
- The print() function in Python makes it incredibly easy to display string literals/ data.
- As with defining strings, we can use the single quotes (') or double quotes (") enclosure methods when printing strings.
- Alternatively, we also print multi-line strings using triple quotes (''' or """).
The Python program example below illustrates how to print different string formats using the print() function.
Code Example:
I1ByaW50aW5nIGEgc2luZ2xlLWxpbmUgc3RyaW5nIGxpdGVyYWwgdXNpbmcgc2luZ2xlIHF1b3RlcwpwcmludCgnSGVsbG8sIFVuc3RvcCEnKQoKI1ByaW50aW5nIGEgc2luZ2xlLWxpbmUgc3RyaW5nIHVzaW5nIGRvdWJsZSBxdW90ZXMKcHJpbnQoIkhlbGxvLCBVbnN0b3BwYWJsZSEiKQoKI1ByaW50aW5nIGEgbXVsdGktbGluZSBzdHJpbmcgdXNpbmcgdHJpcGxlIGRvdWJsZSBxdW90ZXMKbXVsdGlfbGluZV9zdHJpbmcgPSAiIiJUaGlzIGlzIGEgbXVsdGktbGluZSBzdHJpbmcuCkl0IHNwYW5zIG11bHRpcGxlIGxpbmVzLAphbmQgaXMgZW5jbG9zZWQgaW4gdHJpcGxlIHF1b3Rlcy4iIiIKcHJpbnQobXVsdGlfbGluZV9zdHJpbmcpCgojRGlyZWN0bHkgcHJpbnRpbmcgbXVsdGktbGluZSB0ZXh0IHVzaW5nIHRyaXBsZSBzaW5nbGUgcXVvdGVzCnByaW50KCcnJyBQeXRob24gbWFrZXMgaGFuZGxpbmcgbXVsdGktbGluZQpzdHJpbmdzIHN0cmFpZ2h0Zm9yd2FyZCEgJycnKQ==
Output:
Hello, Unstop!
Hello, Unstoppable!
This is a multi-line string.
It spans multiple lines,
and is enclosed in triple quotes.
Python makes handling multi-line
strings straightforward!
Explanation:
In the Python code example,
- In the first two print() function calls, we print single-line string literals using the single quotes(') and double quotes (") methods. Both methods are interchangeable in Python.
- In the next print() function call, we use the triple double quotes (""") method to define a multi-line string, allowing the text to span multiple lines.
- In the last print() function call, we print a multi-line text directly using triple single quotes ('''), which works similarly to triple double quotes.
This flexibility ensures you can choose the style that suits your code, whether for single-line messages or structured multi-line text.
Check out this amazing course to become the best version of the Python programmer you can be.
How To Print Built-in Data Types In Python?
The print() function can handle more than just strings. You can use it to output integers, floats, booleans, lists, dictionaries, and more. The example Python program below illustrates how to use the print statement with a variety of built-in data types.
Code Example:
I1ByaW50aW5nIGRpZmZlcmVudCBidWlsdC1pbiBkYXRhIHR5cGVzCnByaW50KDQyKSAjIEludGVnZXIKcHJpbnQoMy4xNCkgIyBGbG9hdApwcmludChUcnVlKSAjIEJvb2xlYW4KcHJpbnQoWzEsIDIsIDNdKSAjIExpc3QKcHJpbnQoeyJuYW1lIjogIlZhbmkiLCAiYWdlIjogMjV9KSAjIERpY3Rpb25hcnk=
Output:
42
3.14
True
[1, 2, 3]
{'name': 'Vani', 'age': 25}
Explanation:
In the example Python code,
- We use three print statements to display the built-in types– float, integer and boolean.
- Note that the print() function in Python automatically converts non-string data types like floats, booleans, and integer to string representation.
- We then use the print statement to display lists and dictionary type in their literal forms, complete with square brackets ([]) for Python lists and curly braces ({}) for dictionaries.
This example illustrates the versatility of the print statement in Python and its use for a wide range of data visualization tasks.
Print() Function In Python For Values Stored In Variables
The print() function is often used to display the contents stored in variables. This is especially helpful for debugging or tracking the flow of your program. You can print single variables, combine multiple variables, or even format them for readability.
Code Example:
I1N0b3JpbmcgdmFsdWVzIGluIHZhcmlhYmxlcwp4ID0gMTAKeSA9IDIwCnJlc3VsdCA9IHggKyB5CgojUHJpbnRpbmcgdmFyaWFibGVzIGluZGl2aWR1YWxseQpwcmludCgieDoiLCB4KQpwcmludCgieToiLCB5KQoKI1ByaW50aW5nIGNvbWJpbmVkIHZhcmlhYmxlcyB3aXRoIGEgbWVzc2FnZQpwcmludCgiVGhlIHN1bSBvZiB4IGFuZCB5IGlzOiIsIHJlc3VsdCk=
Output:
x: 10
y: 20
The sum of x and y is: 30
Explanation:
In the Python example code,
- We first create three variables: x with the value 10, y with the value 20 and the result with the sum of x and y.
- Then, we use two print statements to display the values of x and y variables.
- Next, we use another print statement combining a string message with the value of result, which holds the sum of x and y.
This approach provides clear and readable outputs, making it easy to understand what the variables represent and how their values change.
Print() Function In Python With sep Parameter
By default, the print() function separates multiple arguments with a single space. This is also the default value for the sep parameter, meaning it controls the behavior of the separator in print statements in Python.
- You can, hence, use this parameter to specify a custom separator for the arguments being printed.
- For example, you can replace the default blank space with a dash, a comma, or even a combination of characters.
In short, you can easily modify the separator to suit your needs with the sep keyword argument.
Code Example:
I0RlZmF1bHQgc2VwYXJhdG9yIChzcGFjZSkKcHJpbnQoIlB5dGhvbiIsICJpcyIsICJmdW4iKQoKI1VzaW5nIGEgY3VzdG9tIHNlcGFyYXRvcjogZGFzaApwcmludCgiUHl0aG9uIiwgImlzIiwgImZ1biIsIHNlcD0iLSIpCgojVXNpbmcgYSBjdXN0b20gc2VwYXJhdG9yOiBzbGFzaApwcmludCgiMjAyNCIsICIxMSIsICIyMCIsIHNlcD0iLyIpCgojVXNpbmcgYSBjb21iaW5hdGlvbiBvZiBjaGFyYWN0ZXJzIGFzIGEgc2VwYXJhdG9yCnByaW50KCJIZWxsbyIsICJXb3JsZCIsIHNlcD0iIDwtLT4gIik=
Output:
Python is fun
Python-is-fun
2024/11/20
Hello <--> World
Explanation:
In the sample Python program,
- We first print three strings using a single print statement. As seen in the output here, the strings are separated by single spaces by default.
- Next, we print the same set of string literals but also specify a custom separator– hyphen (–) sep argument.
- Then, we use a slash (/) as a separator to format a date, where we pass three numbers in string format to the print statement.
- And lastly, we use a more complex separator (<-->), showcasing the flexibility of the parameter and the print statement in Python.
Print() Function In Python With end Parameter
By default, every print statement in Python ends with a newline (\n). This means the output of the next print call begins on a new line.
- Hence, you can use the end parameter to customize this behavior by specifying what should be appended at the end of the printed output.
- That is, you can use it to continue output on the same line or add custom characters.
Code Example:
I0RlZmF1bHQgYmVoYXZpb3IgKG5ld2xpbmUpCnByaW50KCJIZWxsbyIpCnByaW50KCJXb3JsZCIpCgojVXNpbmcgYSBzcGFjZSBhcyB0aGUgZW5kIGNoYXJhY3RlcgpwcmludCgiSGVsbG8iLCBlbmQ9IiAiKQpwcmludCgiV29ybGQiKQoKI1VzaW5nIHRocmVlIGRvdHMgYXMgdGhlIGVuZCBjaGFyYWN0ZXIKcHJpbnQoIkxvYWRpbmciLCBlbmQ9Ii4uLiIpCnByaW50KCJDb21wbGV0ZSIpCgojQ29tYmluaW5nIGRpZmZlcmVudCBlbmQgY2hhcmFjdGVycwpwcmludCgiUHl0aG9uIiwgZW5kPSIsICIpCnByaW50KCJpcyIsIGVuZD0iIC0gIikKcHJpbnQoImF3ZXNvbWUhIik=
Output:
Hello
World
Hello World
Loading...Complete
Python, is - awesome!
Explanation:
In the sample Python code,
- We use two print statements to display the contents of strings– "Hello" and "World".
- As is evident in the output, the content of the second string is displayed in a newline after the first one. This shows the default endline (newline character) behavior of the print statement in Python since we did not use the end parameter.
- Then, we use another pair of print statements to display two more strings. This time, we use the end parameter and replace the default with three dots in the first statement. As a result, both the strings are displayed in the same line, separated by the dots.
- In the next three print statements, we combine multiple characters with the use of the end parameter to format the output dynamically.
Print() Function In Python With flush Parameter
By default, the output of the print() function is buffered, meaning it may not be displayed immediately. The flush argument/ parameter forces the output to be written to the console or stream immediately when set to True. This is particularly useful in scenarios like real-time logging or progress updates.
Code Example:
I0ltcG9ydGluZyB0aGUgdGltZSBtb2R1bGUKaW1wb3J0IHRpbWUKCiNQcmludGluZyB3aXRob3V0IGZsdXNoIChkZWZhdWx0IGJ1ZmZlcmluZyBiZWhhdmlvcikKZm9yIGkgaW4gcmFuZ2UoNSk6CiAgcHJpbnQoaSwgZW5kPSIgIiwgZmx1c2g9RmFsc2UpCiAgdGltZS5zbGVlcCgxKSAjIFNpbXVsYXRlIGRlbGF5CnByaW50KCkgIyBOZXdsaW5lIGZvciBjbGFyaXR5CgojUHJpbnRpbmcgd2l0aCBmbHVzaCAoaW1tZWRpYXRlIG91dHB1dCkKZm9yIGkgaW4gcmFuZ2UoNSk6CiAgcHJpbnQoaSwgZW5kPSIgIiwgZmx1c2g9VHJ1ZSkKICB0aW1lLnNsZWVwKDEpICMgU2ltdWxhdGUgZGVsYXk=
Output:
0 1 2 3 4
0 1 2 3 4 # Flushed (Each number appears immediately)
Explanation:
In the Python program sample,
- We use a for loop and a print statement to display five numbers (0-4) with the flush parameter set to its default value (False).
- Python's standard output is line-buffered by default, i.e., it is stored in a buffer and displayed all at once when the buffer is flushed (typically at the end of the loop or when a newline character is encountered).
- As a result, even though time.sleep(1) introduces a 1-second delay between iterations and all numbers (0–4) are displayed at once when the loop completes.
- Then, we use a second for loop to print the same numbers, with the flush argument set to True.
- This forces the output buffer to flush immediately after each print() call, ensuring each number appears on the console right away, even with the 1-second delay between iterations.
- This behavior is particularly useful for real-time logging, progress indicators, or streaming output scenarios.
This example highlights how flush can be used to gain precise control over the timing of output in Python programs.
Print() Function In Python With file Parameter
The Python print statement outputs data to the console (standard output) by default. However, there are scenarios where you may want to redirect the output to a file instead of the screen. For this, you must use the file parameter of the print() function. It accepts a file-like object.
In other words, the print() function in Python, by default, sends its output to sys.stdout. But you can alter this by passing a file object to the file parameter to direct the output to a file instead. To use this Python feature,
- First, open a file in writing mode ('w' or 'a'). If the file doesn't exist, Python will create it.
- For binary files or more complex file handling, ensure that the file is opened in the appropriate mode ('wb' for binary, for example).
- Once the file is open, you can use the file parameter to specify where you want to direct the output.
When working with file-like objects, the print() function will write the output to the file, just as it would display it on the console. Let's look at an example where we use a dummy file to show the use of this parameter.
Code Example:
I09wZW4gYSBkdW1teSBmaWxlIGluIHdyaXRlIG1vZGUKd2l0aCBvcGVuKCdvdXRwdXQudHh0JywgJ3cnKSBhcyBzb3VyY2VGaWxlOgogICMgVXNpbmcgcHJpbnQoKSB0byB3cml0ZSBkYXRhIHRvIHRoZSBmaWxlCiAgcHJpbnQoIlRoaXMgaXMgYSB0ZXN0IG1lc3NhZ2UuIiwgZmlsZT1zb3VyY2VGaWxlKQogIHByaW50KCJQeXRob24gaXMgd3JpdGluZyB0aGlzIHRvIGEgZmlsZS4iLCBmaWxlPXNvdXJjZUZpbGUpCgojT3BlbmluZyB0aGUgZHVtbXkgZmlsZSBpbiByZWFkaW5nIG1vZGUgdG8gc2hvdyBpdHMgY29udGVudAp3aXRoIG9wZW4oJ291dHB1dC50eHQnLCAncicpIGFzIHNvdXJjZUZpbGU6CiAgY29udGVudCA9IHNvdXJjZUZpbGUucmVhZCgpCiAgcHJpbnQoIkNvbnRlbnRzIG9mIG91dHB1dC50eHQ6IikKICBwcmludChjb250ZW50KQ==
Output In Console:
Contents of output.txt:
This is a test message.
Python is writing this to a file.
Explanation:
In the Python code sample,
- We first open a dummy file (output.txt) in writing mode ('w') using the open() function. This creates the file if it doesn’t already exist.
- Within the with block, we use two print statements to write messages to the file by specifying the file-like object (sourceFile) as the file parameter.
- The file parameter redirects the standard output (console) to the specified file.
- After writing to the file, we reopen it in the reading mode ('r') to verify its contents.
- We use the read() method to read the contents of the file and display it on the console using print inside the with block.
Notes:
- Automatic File Handling: The with statement ensures proper opening and closing of the file, even if an error occurs during the file operation.
- Default Behavior: If the file doesn’t exist, Python creates it automatically. If it already exists, opening it in 'w' mode overwrites its contents.
- Use with Binary Files: By switching the mode string to 'wb', you can write binary data to a file, adapting this method for diverse use cases.
- Application: The file parameter to write to files is useful for logging, saving outputs for future reference, generating reports, or persisting data.
- By using file objects in writing mode, you can redirect output effectively, bypassing the default console printing.
Level up your coding skills with the 100-Day Coding Sprint at Unstop and claim the bragging rights, now!
How To Remove Newline From print() Function In Python?
As we've mentioned before, the Python print() function, by default, adds a newline character (\n) after each output. This results in the output of the next print statement appearing on a new line.
- In certain situations, you might want to suppress this default behavior and print on the same line.
- To remove the newline, you can set the end parameter to specify how to end the line.
- For example, you can replace the newline with an empty string (end='') or use any other string (such as a space, comma, or dot) to separate printed outputs without starting a new line.
For more, read: How To Print Without Newline In Python? (Mulitple Ways + Examples)
Use Cases Of The print() Function In Python
Let’s explore some use cases of the print statement in Python, showcasing its flexibility to handle different data types, special characters, and even file output. While we’ve already discussed parameters like sep, end, and flush, this section will highlight additional features that elevate the print() function’s utility.
1. Printing Unicode And Special Characters
Python allows printing special characters like Unicode symbols, emojis, and even characters from non-English alphabets. To do this, you simply include the character or Unicode escape sequence directly in the string.
Code Example:
I0V4YW1wbGUgb2YgcHJpbnRpbmcgVW5pY29kZSBhbmQgc3BlY2lhbCBjaGFyYWN0ZXJzCnByaW50KCJIZWxsbywgd29ybGQhIPCfmIoiKQpwcmludCgiVW5pY29kZSBjaGFyYWN0ZXIgZm9yIFBpOiBcdTAzQTAiKQ==
Output:
Hello, world! 😊
Unicode character for Pi: Π
In this case, the print() function handles the Unicode characters seamlessly. Unicode characters can be specified using the escape sequence (e.g., \u03A0 for Pi). These characters make it easy to work with internationalization and special symbols.
2. Printing Custom Strings With Format Strings
If you want to print custom strings with dynamic values, Python’s string formatting options—like f-strings, .format(), or the % operator—work seamlessly with the print statement. This allows you to integrate variables directly into strings.
Code Example:
bmFtZSA9ICJTaGl2YW5pIgphZ2UgPSAzMAoKI1VzaW5nIGYtc3RyaW5ncyBmb3IgZm9ybWF0dGluZwpwcmludChmIkhlbGxvLCBteSBuYW1lIGlzIHtuYW1lfSBhbmQgSSBhbSB7YWdlfSB5ZWFycyBvbGQuIik=
Output:
Hello, my name is Shivani and I am 30 years old.
Here, f-strings let you embed variables directly within strings. The result is a custom string that can change dynamically depending on the values of the variables, making it ideal for displaying dynamic information.
3. Printing A Formatted Numeric String
Sometimes, you need to format numbers, like controlling the number of decimal places, padding, or alignment. You can do that by using string formatting within the print statement in Python.
Code Example:
I1ByaW50aW5nIGEgbnVtYmVyIHdpdGggc3BlY2lmaWMgZm9ybWF0dGluZwp2YWx1ZSA9IDMuMTQxNTkKCiNQcmludCAyIGRlY2ltYWwgcGxhY2VzCnByaW50KGYiVmFsdWUgb2YgUGk6IHt2YWx1ZTouMmZ9Iik=
Output:
Value of Pi: 3.14
Here, we use a formatted string approach inside the print statement to display text with numbers. We also use the format specifier (.2f) to ensure that the floating-point number is displayed with two decimal places. This comes in handy when you need to format numerical data cleanly for reports, logs, or user-facing interfaces.
4. Printing With File-like Objects
As we discussed earlier, print() can be used to direct output to a file or file-like object (like sys.stdout). When working with file output, you can specify the file parameter in print() to control where the output goes.
Code Example:
I0V4YW1wbGUgb2YgcmVkaXJlY3Rpbmcgb3V0cHV0IHRvIGEgZmlsZQp3aXRoIG9wZW4oJ291dHB1dC50eHQnLCAndycpIGFzIGZpbGU6CiAgcHJpbnQoIlRoaXMgaXMgd3JpdHRlbiB0byB0aGUgZmlsZS4iLCBmaWxlPWZpbGUp
Output:
This is written to the file.
By passing a file object (like file) to the print() function, you redirect the output from the console to the file. This is a great way to log information or generate reports programmatically.
5. Printing Concatenated Strings
String concatenation is the process of combining multiple strings into a single one. The print() function can seamlessly handle string concatenation using the plus/ concatenation operator (+) or by separating the strings with commas (,).
Code Example:
I1VzaW5nIHRoZSArIG9wZXJhdG9yIGZvciBjb25jYXRlbmF0aW9uCmZpcnN0X25hbWUgPSAiQXJ5YSIKbGFzdF9uYW1lID0gIk1hbGxpayIKcHJpbnQoIkhlbGxvLCAiICsgZmlyc3RfbmFtZSArICIgIiArIGxhc3RfbmFtZSArICIhIikKCiNVc2luZyBjb21tYXMgZm9yIGNvbmNhdGVuYXRpb24gKGF2b2lkaW5nIGV4cGxpY2l0ICspCmdyZWV0aW5nID0gIldlbGNvbWUiCnByaW50KGdyZWV0aW5nLCBmaXJzdF9uYW1lLCBsYXN0X25hbWUgKyAiISIpCgojQ29tYmluaW5nIHZhcmlhYmxlcyBhbmQgbGl0ZXJhbHMgaW4gb25lIGxpbmUKYWdlID0gMjUKcHJpbnQoZmlyc3RfbmFtZSArICIgaXMgIiArIHN0cihhZ2UpICsgIiB5ZWFycyBvbGQuIik=
Output:
Hello, Arya Mallik!
Welcome Arya Mallik!
Arya is 25 years old.
Here,
- We first define two variables, first_name with the value "Arya" and last_name with the value "Mallik".
- Then, we use the addition operator (+) to concatenate them with other strings and explicit spaces/ punctuation formatting inside a print statement.
- Next, we define another string variable greeting with the value "Welcome".
- After that, we pass all three variables to a print statement, separated by commas and concatenate an exclamation mark at the end. Here, print() automatically inserts default spaces between arguments.
- Lastly, we define an age variable with value 25, and use a print statement to demonstrate the concatenation of strings with numeric values by converting the number into a string using the str() function. This is necessary because the concatenation operator (+) only works between strings.
Understanding Print Statement In Python 2 Vs. Python 3
The print functionality in Python underwent a significant change with Python 3. In Python 2, print was a statement, while in Python 3, it became a function. This change was made to bring consistency and improve extensibility within the language.
Print Is A Function In Python 3
In Python 3, print is a built-in function, which means it requires parentheses around its arguments. This design allows for more advanced features like parameterized outputs and improved readability.
Code Example:
I1ByaW50aW5nIHdpdGggUHl0aG9uIDMKcHJpbnQoIkhlbGxvLCBQeXRob24gMyEiKQpwcmludCgiTnVtYmVyczoiLCAxLCAyLCAzLCBzZXA9IiAtICIp
Output:
Hello, Python 3!
Numbers: 1 - 2 - 3
Note that the parentheses are mandatory because print is a function. Additional features like sep and end parameters provide flexibility, such as customizing separators or avoiding newlines.
Print Was A Statement In Python 2
In Python 2, print was a statement, so no parentheses were required (though they could still be used as part of grouping). This approach limited the flexibility of the print keyword and made it harder to extend functionality.
Code Example:
I1ByaW50aW5nIHdpdGggUHl0aG9uIDIKcHJpbnQgIkhlbGxvLCBQeXRob24gMiEiCnByaW50ICJTdW06IiwgMSwgMiwgMw==
Output:
Hello, Python 2!
Sum: 1 2 3
Note that here, the parentheses were optional unless explicitly needed for grouping expressions and features like sep and end were unavailable, so custom behaviors required additional workarounds. Also, redirecting output (e.g., to files) also lacked the simplicity introduced in Python 3.
The shift from a statement in Python 2 to a function in Python 3 not only modernized the language but also provided users with more control over output formatting.
Looking for guidance? Find the perfect mentor from select experienced coding & software experts here.
Conclusion
The print statement in Python is a versatile tool that goes beyond basic data display. From simple outputs to managing complex tasks like formatting, file redirection, and special parameters, the print() function embodies Python's philosophy of simplicity and power.
Its flexible syntax, powered by positional and keyword arguments (such as sep, end, flush, and file), offers precise control over output behavior for a wide range of scenarios. Understanding the print statement in Python will equip you to handle intricate projects with ease, enabling you to write clear, effective, and user-friendly programs.
Frequently Asked Questions
Q1: What is the default behavior of the print statement in Python?
The print statement in Python prints the provided arguments to the console, separated by a space (sep=' ') and ends with a newline character (end='\n') by default. It also writes to the standard output stream unless redirected using the file parameter.
Q2: Can I use print() to write output to a file?
Yes, the print() function supports the file parameter, which allows you to redirect the output to a file-like object instead of the console. For example:
with open('output.txt', 'w') as file:
print("Hello, File!", file=file)
This will write "Hello, File!" to output.txt.
Q3: How do I print without adding a newline?
You can use the end parameter to modify the default behavior. For example:
print("Hello", end=" ")
print("World!")
This outputs: Hello World! (without a newline between the two prints).
Q4: What does the flush parameter do in print()?
The flush parameter controls whether the output is immediately flushed to the console or file. By default, it is set to False, meaning output may be buffered. Setting it to True forces immediate output. This is particularly useful when working with real-time applications or logging.
Q5: Can I format strings using print()?
Yes, you can format strings using Python's f-strings, the format() method, or concatenation. For example:
name = "Shivani"
print(f"Hello, {name}!") # Using f-string
print("Hello, {}!".format(name)) # Using format()
The output for both print statements will be: Hello, Shivani!
Q6: Is print() available in Python 2?
Yes, but with a key difference. In Python 2, print is a statement, not a function. For instance:
print "Hello, World!" # Python 2 syntax
In Python 3, print() is a function and requires parentheses:
print("Hello, World!") # Python 3 syntax
Q10: How does print() differ from logging in Python?
While print() is primarily used for debugging and displaying output, the logging module is designed to manage application logs. Unlike print(), logging provides different levels of severity (e.g., DEBUG, INFO, WARNING), output formatting, and the ability to write logs to files or other destinations.
Using the print statement in Python must now be a cakewalk for you. Here are a few more Python topics you will enjoy:
- Python input() Function (+Input Casting & Handling With Examples)
- Python String.Replace() And 8 Other Ways Explained (+Examples)
- How To Convert Python List To String? 8 Ways Explained (+Examples)
- Relational Operators In Python | All 6 Types With Code Examples
- Difference Between List And Tuple In Python Explained (+Examples)
- Python Logical Operators, Short-Circuiting & More (With Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment