Table of content:
- What Is Python While Loop?
- How Does The Python While Loop Work?
- How To Use Python While Loops For Iterations?
- Control Statements In Python While Loop With Examples
- Python While Loop With Python List
- Infinite Python While Loop in Python
- Python While Loop Multiple Conditions
- Nested Python While Loops
- Conclusion
- Frequently Asked Questions
Python While Loop | Types With Control Statements (Code Examples)
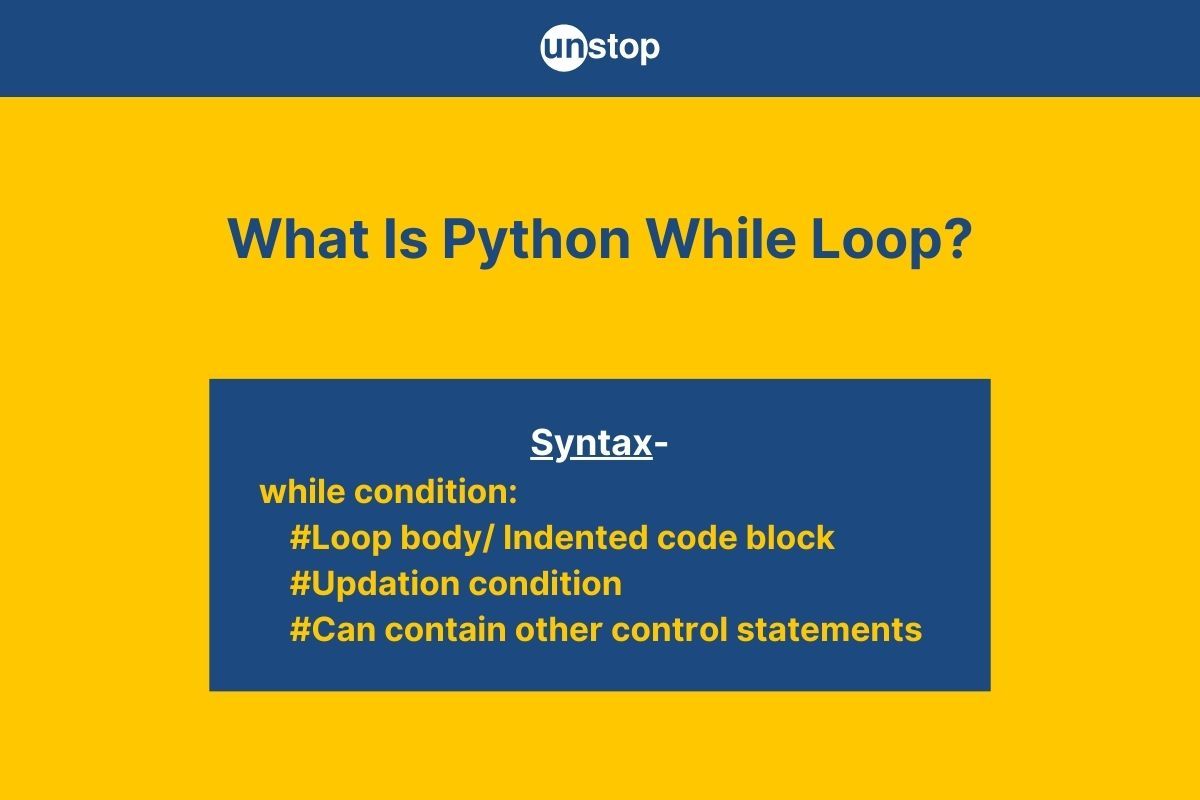
In programming, loops are fundamental constructs that allow code to be executed repeatedly based on a condition. Python, a versatile and widely used programming language, provides various loop types that we can use to execute an iterative approach for manipulations, modifications, calculations, etc. In this article, we will explore the Python while loop and learn how to use it with the right syntax and best practices.
What Is Python While Loop?
A while loop in Python programming language is a control flow statement/ programming construct that allows code to be executed repeatedly based on a given Boolean condition. The loop continues to execute as long as the condition remains True. This type of loop is especially useful when the number of current loop iterations is not predetermined and depends on dynamic conditions evaluated during runtime.
Syntax For Python While Loop:
while condition:
# code block to be executed
Here,
- The while keyword marks the beginning of the loop header, and the condition is the expression (boolean test expression) evaluated at the beginning of every iteration.
- If the boolean expression evaluates to True, the loop body (the indented code block) is executed. If the outcome of the boolean test expression is False, the loop terminates.
- The code block (in code comments) refers to the statement block/ statements that make up the loop body and will be executed as long as the loop conditional statement evaluates to true. Note that they must be indented to indicate that it is part of the loop.
The number of statements inside the code block (as mentioned in the statement syntax above) can sometimes help determine the type of loop. For example, a Python while loop with a single statement is often referred to as a single statement while loop. In such cases, we can include the line of code right after the colon, and there is no need for indentation.
Single Statement While Loop Example
I0luaXRpYWxpemluZyB0aGUgY291bnQvIGxvb3AgY29udHJvbCB2YXJpYWJsZQpjb3VudCA9IDAKCiNEZWZpbmluZyB0aGUgc2luZ2xlIGxpbmUgUHl0aG9uIHdoaWxlIGxvb3AKd2hpbGUgY291bnQgPCA1OiBwcmludCgiQ291bnQgaXM6IiwgY291bnQpOyBjb3VudCArPSAx
Output:
Count is: 0
Count is: 1
Count is: 2
Count is: 3
Count is: 4
Explanation:
In the simple Python code example-
- We declare a variable called count and initialize it with the value 0.
- Next, we create a while loop with the condition count<5. This means that the loop will continue iterations until the count variable is less than 5.
- Inside the loop statement, we have a print() function which displays the value of the count variable.
- After printing the value in one iteration, the value of count is increased by 1, i.e., count +=1.
- The flow then passes back to the beginning of the loop, where the condition is implemented, and the program checks if the count is still less than 5.
- If the condition is true, the loop repeats the steps of printing and incrementing.
- Once the count variable equals 5, the condition count < 5 becomes false. At this point, the loop stops running, and the program exits the loop.
How Does The Python While Loop Work?
A Python while loop works by repeatedly executing a block of code as long as the specified condition remains True. The while loop flowchart of Python given above, illustrates the program flow. Here’s a detailed explanation of how a while loop operates, broken down into key steps:
- Initialization: Initialize all relevant variables (like the loop counter variable) that are used in the loop or the loop condition.
- Condition Evaluation: The loop checks the loop condition. If the condition evaluates to False, the loop terminates, and the program continues with the next statement after the loop.
- Execution of Code Block: If the loop conditional expression is evaluated to be true, then the code block inside the while loop is executed. This block of statements contains the set of instructions that need to be repeated as long as the condition is True.
- Update: The loop code block will contain an updating expression, which will increment variable counter before the next iteration. This update is crucial to eventually making the condition False and thereby terminating the loop. For example, if we do not update the loop variable, the condition will always remain true, leading to indefinite iterations.
- Repeat: After executing the code block and updating the necessary variables, the loop goes back to step 2 (condition evaluation). This process repeats until the loop conditional expression (condition) is evaluated as false.
- Termination: When the condition evaluates to False, the loop stops executing, and the control moves to the next statement immediately following the loop.
When Should You Use Python While Loop?
A while loop is useful in scenarios where you must repeatedly execute a block of code (looping block) as long as a specified condition remains true. Here are some common situations where using a Python while loop is appropriate:
- Unknown Number of Iterations: When you don't know in advance how many times the loop needs to run. In this case, you can define the condition such that the Python while loop runs till the condition remains true. Example: Reading user input until a valid input is received.
- Waiting for a Condition to Change: You can use Python while loop when your loop needs to wait for an external condition or event to change before it can stop. Example: Polling a sensor until a specific value is detected.
- Sentinel-Controlled Loops: Use Python while loop when using a sentinel value to signify the end of a loop. The loop continues to process data until the sentinel value is encountered. Example: Reading data from a file until an end-of-file marker is reached.
- Infinite Loops: When you need an infinite loop that runs until explicitly terminated by a break statement. This is often used in event-driven programs or servers. Example: A server that continuously listens for client connections.
- Resource Management: You can use the Python while loop when managing resources such as network connections or file handles, and you need to keep the loop running until the resource is no longer needed. Example: Continuously processing messages from a message queue.
How To Use Python While Loops For Iterations?
Loops are, by definition, used for iterations. But it is beneficial to use Python while loop when you need more control over the loop than what a for loop provides. They can be especially useful if you need to dynamically change the loop's condition or the loop variable within the loop body. Here's how you can iterate over a range of numbers using a while loop and use the current iteration in the loop body.
Python While Loop To Iterate Over A Range
In this method, we initialize a loop variable to the starting value and define a condition that determines when the loop should terminate. The loop body contains the code to be executed in each iteration. After executing the loop body, we update the loop variable to ensure progress towards the termination condition and avoid infinite loops. Below is a simple Python program example that illustrates this concept.
Code Example:
IyBJbml0aWFsaXphdGlvbjogc3RhcnRpbmcgZGVmYXVsdCB2YWx1ZSBvZiB0aGUgcmFuZ2UKc3RhcnQgPSAwIAojIERlZmluZSB0aGUgZW5kIG9mIHRoZSByYW5nZQplbmQgPSA0IAoKIyBDb25kaXRpb246IGxvb3AgY29udGludWVzIGFzIGxvbmcgYXMgc3RhcnQgaXMgbGVzcyB0aGFuIGVuZAp3aGlsZSBzdGFydCA8IGVuZDogCiAgcHJpbnQoIkN1cnJlbnQgaXRlcmF0aW9uOiIsIHN0YXJ0KSAjIFVzZSB0aGUgY3VycmVudCBpdGVyYXRpb24gaW4gdGhlIGxvb3AgYm9keQogIHN0YXJ0ICs9IDEgIyBVcGRhdGU6IGluY3JlbWVudCB0aGUgbG9vcCB2YXJpYWJsZQ==
Output:
Current iteration: 0
Current iteration: 1
Current iteration: 2
Current iteration: 3
Explanation:
In the example Python code-
- We initialize two variables, start and end, with the values 0 and 4, respectively.
- The start variable establishes the starting point of the range. and end variables sets the endpoint.
- Then, we create a while loop with the condition start<end. This ensures that the loop continues as long as the value of the start variable is less than the value of the end variable.
- Inside the loop, the print() statement displays the current value of the start variable.
- It then increments the value of the start variable by 1 before moving on to the next iteration.
- This process repeats until the start variable is greater than the end variable, at which point the Python while loop terminates.
Simple Python While Loop To Iteration Using The Counter Variable
This refers to the simple method of using the current iteration value of the counter variable to iterate over the loop body. As usual, you must initialize a loop variable and define a termination condition. Inside the loop body, use the current iteration value to perform operations and update the loop variable as needed to ensure progress towards the termination condition.
Code Example:
IyBJbml0aWFsaXphdGlvbgpjb3VudCA9IDEKCiMgTG9vcCB1bnRpbCBjb3VudCByZWFjaGVzIDQKd2hpbGUgY291bnQgPD0gNDoKICBwcmludCgiQ3VycmVudCBudW1iZXI6IiwgY291bnQpICMgUHJpbnQgY3VycmVudCBpdGVyYXRpb24gdmFsdWUKICBjb3VudCArPSAxICMgSW5jcmVtZW50IGNvdW50IGZvciB0aGUgbmV4dCBpdGVyYXRpb24=
Output:
Current number: 1
Current number: 2
Current number: 3
Current number: 4
Explanation:
In the example Python program-
- We first initialize a variable count with the value 1. This will act as the loop counter variable.
- Then, we create a Python while loop with the condition count<=4. This ensures that the loop continues until the count value is less than or equal to 4.
- Inside the loop, the print() statement displays the current value of the count variable with a string message.
- After printing the current value, we increment the count variable by 1 (i.e., count += 1), ensuring the loop moves on to the next iteration.
- This process repeats until the value of count reaches 4, at which point the condition count <= 4 becomes false, and the loop terminates.
Control Statements In Python While Loop With Examples
Control statements in Python help manage the flow of the loop and modify to the natural successive iteration ability. These include continue, break, pass, and the use of else with while loops. Additionally, we can use sentinel-controlled loops and loops with Boolean values.
We use the if-statement inside the loops to define the condition, which then leads the program to encounter these additional control statements. The flowchart above illustrates how the control flows in a Python while loop with an if-statement.
Python While Loop With Continue Statement
The continue statement is used to skip the current iteration of the loop and proceed directly to the next iteration. This is useful when you want to avoid executing certain parts of the loop for some specific conditions or in specific cases. We generally use the continue statement inside an if-statement to induce the specific cases.
For instance, if you are iterating through a range of numbers and want to skip processing when a particular number is encountered, you can use the continue statement inside the Python while loop. Below is a Python program example that illustrates the use of continue inside a while loop.
Code Example:
I0luaXRpYWxpemluZyB0aGUgbG9vcCBjb3VudGVyIHZhcmlhYmUKY291bnQgPSAwCgojQ3JlYXRpbmcgYSBQeXRob24gd2hpbGUgbG9vcCB0byBpdGVyYXRlIHRpbGwgY291bnRlciB2YXJpYWJsZSBpcyBsZXNzIHRoYW4gNQp3aGlsZSBjb3VudCA8IDU6CiAgY291bnQgKz0gMQogIGlmIGNvdW50ID09IDM6CiAgICBjb250aW51ZSAjIFNraXAgdGhlIHJlc3Qgb2YgdGhlIGxvb3Agd2hlbiBjb3VudCBpcyAzCiAgcHJpbnQoIkN1cnJlbnQgY291bnQ6IiwgY291bnQp
Output:
Current count: 1
Current count: 2
Current count: 4
Current count: 5
Explanation:
In the Python code example-
- We initialize a variable count with the value 1 and then create a while loop.
- The loop condition is count<5, stipulating that the loop will run as the count value is less than 5.
- Inside the loop, we increment the value of the count variable by 1 at the beginning of each iteration (using the compound assignment operator).
- Then, we have an if-statement, which checks if the value of the count variable is equal to 3.
- If the condition is true, then the if-block is executed, and we encounter the continue statement. As a result, the program skips the rest of the loop body, and the control passes to the next iteration.
- If the condition count==3 is false, then the if-block is ignored, and we move to the next line of the loop.
- Next, we have a print() statement which displays the current value of the count variable.
- The loop continues iterations till the value of the count variable reaches 5. At this point, the loop terminates.
Python While Loop With Break Statement
The break statement is used to terminate the loop immediately when encountered, regardless of the condition that controls the loop. This is useful when you need to exit the loop prematurely based on a specific condition, such as finding a particular value in a list or when an error occurs. The sample Python program below shows how you can use the break statement with a Python while loop.
Code Example:
I0luaXRpYWxpemUgdGhlIGNvdW50IHZhcmlhYmxlIApjb3VudCA9IDAKCiNEZWZpbmUgdGhlIHdoaWxlIGxvb3AKd2hpbGUgY291bnQgPCA1OgogIGNvdW50ICs9IDEKICBpZiBjb3VudCA9PSAzOgogICAgYnJlYWsgIyBFeGl0IHRoZSBsb29wIHdoZW4gY291bnQgaXMgMwogIHByaW50KCJDdXJyZW50IGNvdW50OiIsIGNvdW50KQ==
Output:
Current count: 1
Current count: 2
Explanation:
In the sample Python code-
- We initialize a variable count with the value 0.
- Then, we begin a while loop with the condition count<5. This means that the loop will run as long as the value of count is less than 5.
- Inside the loop, we increment count by 1 at the beginning of each iteration.
- Next, we have an if-statement, which checks if the value of count is equal to 3.
- If the condition is false, the if-block is ignored, and we move to the print() statement in the next line of the loop.
- If the condition is true, the if-block is executed. We encounter the break statement, which causes the program to exit the loop immediately. This means the loop will terminate, and no further iterations will occur.
- The loop has a print() statement, which displays the current value of the count variable with a strong message.
- In this example, the loop continues until count reaches 3, at which point it exits due to the break statement.
Python While Loop With Pass Statement
The pass statement is a null operation; it is used as a placeholder where the code will eventually go. This is useful during development when you want to outline the structure of your code without implementing the logic yet. It ensures that, in such cases, the code runs without syntax errors. The Python program sample below shows the working of the pass statement inside a Python while loop.
Code Example:
I0luaXRpYWxpemluZyB0aGUgbG9vcCBjb250ZXIgdmFyaWFibGUKY291bnQgPSAwCgojRGVmaW5pbmcgdGhlIGxvb3AgdG8gaXRlcmF0ZSB0aWxsIGNvdW50IGlzIGxlc3MgdGhhbiA1CndoaWxlIGNvdW50IDwgNToKICBjb3VudCArPSAxCiAgaWYgY291bnQgPT0gMzoKICAgIHBhc3MgIyBEb2VzIG5vdGhpbmcgd2hlbiBjb3VudCBpcyAzCiAgcHJpbnQoIkN1cnJlbnQgY291bnQ6IiwgY291bnQp
Output:
Current count: 1
Current count: 2
Current count: 3
Current count: 4
Current count: 5
Explanation:
In the Python code sample-
- We declare and initialize a variable count with the value 0 and then start a while loop with the condition count<5.
- This means that the Python while loop will run as long as the value of count is less than 5.
- Inside the loop, we increment count by 1 at the beginning of each iteration.
- Then, the if-statement inside the loop checks if the value of count is equal to 3, i.e., count==3.
- If the condition is true, the pass statement inside the if-block is executed. The pass statement does nothing and allows the program to continue to the next line. This means no special action is taken when the count is 3.
- After the if statement, we print the current value of count.
- The loop continues until count reaches 5.
Python While Loop With Else Statement/ Clause
The else clause in a while loop is executed after the loop condition becomes false or after the loop has completed execution. However, the individual else statement/ clause can be used only if the loop is not terminated by a break statement inside the loop body. This is useful for running a single block of code once after the loop completes all its iterations.
Code Example:
I0luaXRpYWxpemluZyB0aGUgbG9vcCBjb250cm9sIHZhcmlhYmxlCmNvdW50ID0gMAoKI0NyZWF0aW5nIHRoZSBQeXRob24gd2hpbGUgbG9wCndoaWxlIGNvdW50IDwgMzoKICBjb3VudCArPSAxCiAgcHJpbnQoIkN1cnJlbnQgY291bnQ6IiwgY291bnQpCmVsc2U6CiAgcHJpbnQoIkxvb3AgY29tcGxldGVkIHdpdGhvdXQgYSBicmVhayIp
Output:
Current count: 1
Current count: 2
Current count: 3
Loop completed without a break
Explanation:
In the Python code-
- We initialize a variable called count with 0 and begin a while loop with the condition count<3.
- This means the loop will run till the value of count variable is less than 3.
- Inside the loop, we first increment the value of count by 1, and then a print() statement displays that value to the console.
- This process repeats until the value of count reaches 3, after which the loop terminates.
- After the loop finishes (if it completes normally without encountering a break statement), the else clause/ statement is executed.
- The else block prints the string message- 'Loop completed without a break', to the console.
Python While Loop With Sentinel Controlled Statement
A sentinel-controlled loop is when we define a loop such that it terminates as soon as the specified variable reaches a certain value/Sentinal value. Think of it like this: you want to terminate an iterative loop for a certain value. Then, you can set the loop condition such that the loop continues to execute until a specific sentinel value is encountered. This is useful when the number of iterations is not known beforehand, and the loop should terminate based on input or a particular condition.
Code Example:
IyBJbml0aWFsaXppbmcgdGhlIFNlbnRpbmVsIHZhbHVlIGFuZCB0aGUgbG9vcCBjb3VudGVyIHZhcmlhYmxlCnNlbnRpbmVsID0gLTEKbnVtYmVyID0gMAoKI0RlZmluaW5nIHRoZSBQeXRob24gd2hpbGUgbG9vcAp3aGlsZSBudW1iZXIgIT0gc2VudGluZWw6CiAgbnVtYmVyID0gaW50KGlucHV0KCJFbnRlciBhIG51bWJlciAoLTEgdG8gZW5kKTogIikpCiAgaWYgbnVtYmVyICE9IHNlbnRpbmVsOgogICAgcHJpbnQoIllvdSBlbnRlcmVkOiIsIG51bWJlcik=
Output:
Enter a number (-1 to end): 3
You entered: 3
Enter a number (-1 to end): 2
You entered: 2
Enter a number (-1 to end): -1
Explanation:
In the Python example-
- We initialize two variables, sentinel and number, with the values -1 and 0, respectively.
- We then enter a while loop that continues as long as number is not equal to the sentinel value.
- Inside the loop, we prompt the user to enter a number, converting the input to an integer.
- We check if the entered number is not the sentinel value.
- If the number is not the sentinel, we print the entered number.
- The loop terminates when the user enters the sentinel value (-1).
Python While Loop With Boolean Values
A Python while loop can use boolean values to set the loop condition and decide when to execute or terminate the loop body. Such loops are referred to as loops with boolean values. They are in cases when you need to run a loop based on complex conditions or multiple criteria that are consolidated into a Boolean variable. Look at the example below for a better understanding of the concept.
Code Example:
IyBJbml0aWFsaXppbmcgdGhlIEJvb2xlYW4gdmFyaWFibGUgYW5kIHRoZSBjb3VudGVyIHZhcmlhYmxlCnJ1bm5pbmcgPSBUcnVlCmNvdW50ID0gMAoKI0RlZmluaW5nIHRoZSBQeXRob24gd2hpbGUgbG9vcAp3aGlsZSBydW5uaW5nOgogIGNvdW50ICs9IDEKICBwcmludCgiQ3VycmVudCBjb3VudDoiLCBjb3VudCkKICBpZiBjb3VudCA9PSA0OgogICAgcnVubmluZyA9IEZhbHNlICMgU2V0IHRoZSBCb29sZWFuIHZhcmlhYmxlIHRvIEZhbHNlIHRvIHRlcm1pbmF0ZSB0aGUgbG9vcA==
Output:
Current count: 1
Current count: 2
Current count: 3
Current count: 4
Explanation:
In the example code-
- We begin by setting a Boolean variable running to True. This variable controls whether the loop continues to run.
- Next, we initialize the variable count to 0 and start a while loop that runs as long as running is True.
- Inside the loop, we increment count by 1, and then a print() statement displays the current value of count along with the message "Current count:".
- Then, we use an if-statement to check if the value of count is equal to 5, i.e., count ==5.
- If the condition is false, the loop keeps running as usual, and the if-block is ignored.
- If the condition is true, the if-block sets the value of the variable running to False.
- This will cause the while loop to terminate after the current iteration because the loop condition running will no longer be True.
- In this example, the loop continues to run until count reaches 4, at which point running is set to False, and the loop stops.
Python While Loop With Python List
A list in Python is an ordered but mutable data structure that can contain different types of elements. We can use Python while loop to iterate over the elements of a list while performing specific tasks or running statement checks.
We use the index variable/ position to refer to the number or the position of the item in the list. There are countless scenarios where you might need to iterate over such data structures.
Syntax:
index = 0 # Initialize the index variable
while index < len(my_list):
# Access my_list[index] and perform operations
index += 1 # Update the index variable
Here,
- The index variable refers to the position of the item in the list, and index+=1 is the updation expression, which increments the index to move to the nest element in the list.
- The len() function, here len(my_list) provides the length of the list whose name is given by my_list. It helps determine the limit at which the while loop should stop.
Look at the example below to see how to use Python while loops with lists.
Code Example:
IyBEZWZpbmUgYSBsaXN0Cm15X2xpc3QgPSBbMTAsIDIwLCAzMCwgNDAsIDUwXQoKIyBJbml0aWFsaXplIHRoZSBpbmRleCB2YXJpYWJsZQppbmRleCA9IDAKCiMgTG9vcCB0aHJvdWdoIHRoZSBsaXN0IGFuZCBwcmludCBlYWNoIGVsZW1lbnQKd2hpbGUgaW5kZXggPCBsZW4obXlfbGlzdCk6CiAgcHJpbnQoIkVsZW1lbnQgYXQgaW5kZXgiLCBpbmRleCwgIjoiLCBteV9saXN0W2luZGV4XSkKICAjIEluY3JlbWVudGluZyBpbmRleCB2YWx1ZSB0byBtb3ZlIHRvIHRoZSBuZXh0IGVsZW1lbnQKICBpbmRleCArPSAx
Output:
Element at index 0 : 10
Element at index 1 : 20
Element at index 2 : 30
Element at index 3 : 40
Element at index 4 : 50
Explanation:
In the example-
- We start by defining a list of integers named my_list containing integers [10, 20, 30, 40, 50].
- Then, we initialize a variable index to 0, which will be used to iterate through the elements of the list.
- Next, we use a while loop to iterate through the list as long as the index is less than the length of my_list, obtained using len(my_list).
- Inside the loop, the print() statement prints the current element of my_list at the index index using string formatting.
- After printing the element, we increment the value of index by 1, moving to the next index in the list.
- The loop continues until the index becomes equal to the length of my_list, at which point the condition index < len(my_list) becomes false, and the loop terminates.
Infinite Python While Loop in Python
An infinite loop is a loop that runs for infinite time because its condition is always true. Infinite/ indefinite loops are useful in situations where you need a loop to continue running until an external condition is met or an event occurs that explicitly breaks the loop.
Syntax:
while True:
# Code block to be executed infinitely
Here,
- The loop condition is given by True, which ensures that the loop runs indefinitely.
- The loop body is signified by the code comment mentioning the code block, which is the indented block.
Code Example:
I0RlZmluaW5nIGEgUHl0aG9uIHdoaWxlIGxvb3Agd2l0aCB0aGUgY29uZGl0aW9uIHNldCB0byBUcnVlCndoaWxlIFRydWU6CiAgI1Byb21wdGluZyB1c2VyIHRvIHByb3ZpZGUgaW5wdXQKICB1c2VyX2lucHV0ID0gaW5wdXQoIkVudGVyICdleGl0JyB0byBzdG9wIHRoZSBsb29wOiAiKQogICNDaGVja2luZyB1c2VyIGlucHV0CiAgaWYgdXNlcl9pbnB1dC5sb3dlcigpID09ICdleGl0JzoKICAgIHByaW50KCJFeGl0aW5nIHRoZSBsb29wLiIpCiAgICBicmVhayAjQnJlYWsgc3RhdGVtZW50CmVsc2U6CiAgcHJpbnQoZiJZb3UgZW50ZXJlZDoge3VzZXJfaW5wdXR9Iik=
Output:
Enter 'exit' to stop the loop: hello
You entered: hello
Enter 'exit' to stop the loop: exit
Exiting the loop.
Explanation:
In the piece of code above-
- We start a while loop with the condition set to True. This means the loop will continue indefinitely until it is explicitly stopped.
- Inside the loop, we use the input() function to prompt the user to enter the word 'exit' if they want the loop to terminate, with the message- "Enter 'exit' to stop the loop: ".
- The input provided by the user is stored in the variable user_input.
- Next, we have an if-else statement which checks if the user's input is actually the string/ character array- 'exit'. We convert the user input to lowercase using the lower() function so that there are no discrepancies in the case.
- If the condition is true, i.e. the user inputs exit, we print the string message- "Exiting the loop.". Then, the break statement inside the if-block causes the program to immediately exit the loop.
- If the condition is false, then the print() statement in the else-block displays the value entered by the user using f-string formatting. Then, keep prompting the user to input another value.
- This loop will go on indefinitely until the user enters the term- exit.
Python While Loop Multiple Conditions
A Python while loop can evaluate multiple conditions to determine whether it should continue executing. We can use any of the logical operators (AND, OR, and NOT) to connect multiple conditions/ boolean test expressions. These expressions can be referred to as logical expressions.
In this case, the body of the Python while loop will be executed only if the combined logical expression is evaluated to be true.
Syntax For Python While Loop With Multiple Conditions:
while condition1 and condition2 and ... :
# Code block to be executed
Here,
- The expressions condition1, condition2, and so on are the boolean expressions that are connected using the logical AND operator (and). These together make the loop condition.
- Since we have used the AND (and) operator, all conditions must be True for the loop to continue. If any condition is False, the loop terminates.
Code Example:
IyBJbml0aWFsaXphdGlvbgpjb3VudCA9IDAKbWF4X2NvdW50ID0gNAp0aHJlc2hvbGQgPSA4Cgp3aGlsZSBjb3VudCA8IG1heF9jb3VudCBhbmQgdGhyZXNob2xkID4gMDoKICBwcmludChmIkNvdW50IGlzIHtjb3VudH0sIFRocmVzaG9sZCBpcyB7dGhyZXNob2xkfSIpCiAgY291bnQgKz0gMSAjIFVwZGF0ZSB0aGUgY291bnQKICB0aHJlc2hvbGQgLT0gMiAjIERlY3JlYXNlIHRoZSB0aHJlc2hvbGQ=
Output:
Count is 0, Threshold is 8
Count is 1, Threshold is 6
Count is 2, Threshold is 4
Count is 3, Threshold is 2
Explanation:
In the above code,
- We begin by initializing three variables: count, max_count, and threshold with values 0, 4, and 8, respectively.
- Then, we initiate a while loop with the condition, i.e., count<max_count and threshold>0.
- This is a single condition comprising two boolean expressions connected using the and operator.
- The loop condition ensures that the Python while loop runs as long as the count value is less than the max_count value and the threshold value is greater than 0.
- Inside the loop, the print() statement displays the current values of count and threshold in a string format.
- After that, the updation conditions (count +=1 and threshold -=2) increment the value of count by 1 and decrement the value of threshold by 2.
- The loop then checks the loop condition again and repeats the process if both conditions are still met.
- The loop continues until count reaches max_count or threshold becomes 0 or less. Once the lop condition becomes false, the loop terminates.
Nested Python While Loops
A nested while loop is when we have one Python while loop inside another Python while loop. The concept of nested loop allows for more complex iterations where one loop runs inside another. Note that the inner loop runs to completion for every iteration of the out loop. This allows us to handle more complex manipulations or iterative tasks with ease.
Syntax For Nested Python While Loop:
while outer_condition:
# Outer loop code block
while inner_condition:
# Inner loop code block
Here,
- The while keyword marks the beginning of the two loop programming structures.
- The outer_condition refers to the boolean expression/ test condition for the outer Python while loop, and the inner_condition refers to the boolean expression for the inner Python while loop.
- The outer and inner loop code blocks refer to the program code that makes the outer and inner loop bodies, respectively. These codes will be executed only if the respective loop conditions are true.
Code Example:
IyBJbml0aWFsaXphdGlvbgpvdXRlciA9IDEKCiMgT3V0ZXIgUHl0aG9uIHdoaWxlIGxvb3AgYW5kIGNvbmRpdGlvbgp3aGlsZSBvdXRlciA8PSAzOgogIGlubmVyID0gMQogICMgSW5uZXIgUHl0aG9uIHdoaWxlIGxvb3AgYW5kIGNvbmRpdGlvbgogIHdoaWxlIGlubmVyIDw9IDM6CiAgICBwcmludChmIntvdXRlcn0gKiB7aW5uZXJ9ID0ge291dGVyICogaW5uZXJ9IikKICAgIGlubmVyICs9IDEgIyBVcGRhdGUgdGhlIGlubmVyIGxvb3AgdmFyaWFibGUKICBvdXRlciArPSAxICMgVXBkYXRlIHRoZSBvdXRlciBsb29wIHZhcmlhYmxl
Output:
1 * 1 = 1
1 * 2 = 2
1 * 3 = 3
2 * 1 = 2
2 * 2 = 4
2 * 3 = 6
3 * 1 = 3
3 * 2 = 6
3 * 3 = 9
Explanation:
In the above code,
- We begin by initializing a variable called outer with the value 1. This will be the counter variable for the outer loop.
- Then, we create a while loop with the condition that it continues to run as long as the outer variable is less than or equal to 3, i.e., outer<=3.
- Inside this outer loop, we initialize another variable called inner with the value 1.
- Next, we create another while loop inside the initial loop, say the inner while loop that runs as long as the inner variable is less than or equal to 3, i.e., inner<=3.
- Within the inner loop, we have a print() statement that calculates and prints the product of the inner and outer variables using a formatted string (f-string).
- After that, the loop variable inner is incremented by 1, and a new iteration of the inner loop begins.
- The inner loop repeats this process until the condition becomes false, i.e., inner exceeds 3.
- Once the inner loop finishes, the flow returns to the outer loop and the value of the variable outer is incremented by 1.
- The outer loop then repeats the entire process with the new value of for outer variable.
- This continues until the outer variable exceeds 3, at which point the outer loop terminates.
Conclusion
The Python while loop is a fundamental program control structure that provides powerful and flexible ways to perform repetitive tasks. Its ability to continue executing as long as a specified condition remains true makes it invaluable for scenarios where the number of iterations is not predetermined. Understanding the various control statements that can be used within a Python while loop, such as continue, break, and pass and using the else clause enhances the ability to manage and manipulate loop flow effectively.
Additionally, sentinel-controlled loops and Boolean-controlled loops offer further customization, enabling developers to build robust and efficient looping mechanisms tailored to their specific needs. Mastering the nuances of Python while loops can equip you with essential tools to handle complex iterative processes and logic, ultimately contributing to developing more dynamic and responsive Python applications.
Frequently Asked Questions
Q: What is the difference between a while loop and a for loop in Python?
A Python while loop continues to execute as long as a specified condition is true, making it ideal for situations where the number of iterations is not known beforehand. In contrast, a for loop iterates over a sequence (like a list, tuple, or range) and is typically used when the number of iterations is predetermined or when you need to iterate through each element in a collection.
The table below highlights the differences between Python for loop and the Python while loop.
Feature | While Loop | For Loop |
---|---|---|
Purpose | Executes as long as a specified condition is true | Iterates over a sequence (list, tuple, string, etc.) |
Use Case | Used when the number of iterations is not known | Used when the number of iteration counts is known or finite |
Condition | Evaluated before each iteration | Implicitly determined by the sequence or iterable |
Initialization | Requires manual initialization of loop variable | The loop variable is automatically initialized by the sequence |
Termination | The loop condition/ body must manually ensure the condition becomes false | Terminates after the sequence is exhausted |
Update | Loop variable must be updated manually | The loop variable is updated automatically |
Infinite Loop Risk | Higher risk if the condition is not properly managed | Lower risk as the iterable eventually exhausts |
Common Syntax | while condition: \n # loop body \n update | for element in sequence: \n # loop body |
Control Statements | Supports break, continue, else, pass | Supports break, continue, else, pass |
Example | count = 0 \n while count < 5: \n print(count) \n count += 1 | for i in range(5): \n print(i) |
Q: What is the purpose of the else clause in a while loop?
The else clause in a Python while loop executes after the loop condition becomes false, but only if the loop is not terminated by a break statement. It is useful for executing code that should run after the loop completes all iterations naturally, allowing for additional actions once the loop has finished.
Q: Can I use multiple control statements like continue and break within the same while loop?
Yes, you can use multiple loop control statements like continue and break within the same while loop. The continue statement skips the rest of the current iteration and moves to the next iteration, while the break statement exits the loop entirely. Combining these can provide fine-grained control over the entire loop's execution flow.
Q: How do sentinel-controlled loops work in Python?
Sentinel-controlled loops continue to execute until a specific sentinel value is encountered. This approach is useful when the exact number of iterations is not known in advance. The loop keeps running until the input or condition matches the sentinel value, which signals the loop to terminate. This is commonly used for processing input where the end conditional expression is indicated by a special value.
Q: How can I prevent an infinite loop when using a while loop?
To prevent an infinite loop bug, ensure that the condition for the Python while loop will eventually become false. This usually involves updating the loop variable within the loop body for every new iteration. Common mistakes that lead to infinite Python while loops include forgetting to update the loop variable or having a condition that can never be false.
Do read the following for more:
- Python Bitwise Operators | Positive & Negative Numbers (+Examples)
- Python String.Replace() And 8 Other Ways Explained (+Examples)
- Python IDLE | The Ultimate Beginner's Guide With Images & Codes
- How To Convert Python List To String? 8 Ways Explained (+Examples)
- How To Reverse A String In Python? 10 Easy Ways With Examples
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment