- What Are Relational Operators In Python?
- Type Of Relational Operators In Python
- Equal-To Relational Operator In Python
- Greater Than Relational Operator In Python
- Less-Than Relational Operator In Python
- Not Equal-To Relational Operator In Python
- Greater-Than Or Equal-To Relational Operator In Python
- Less-Than Or Equal-To Relational Operator In Python
- Why And When To Use Relational Operators?
- Precedence & Associativity Of Relational Operators In Python
- Advantages & Disadvantages Of Relational Operators In Python
- Real-World Applications Of Relational Operators In Python
- Conclusion
- Frequently Asked Questions
Relational Operators In Python | All 6 Types With Code Examples
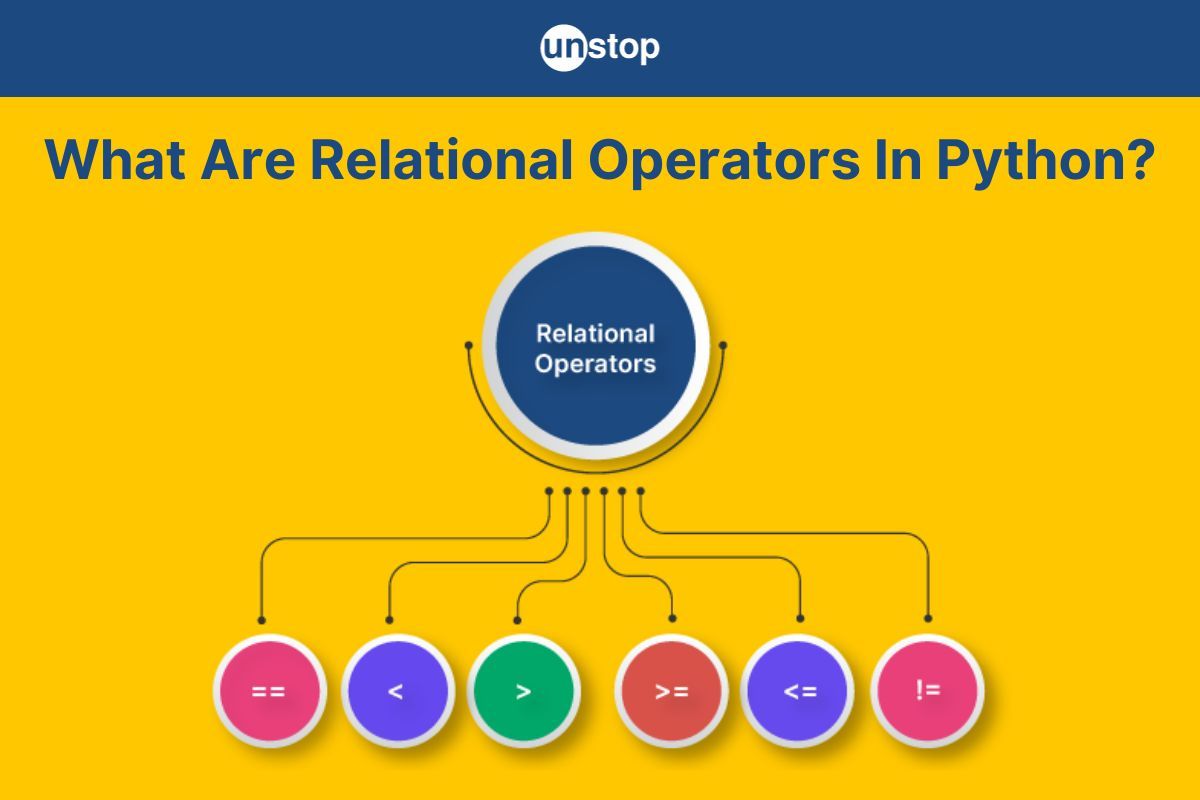
Operators are special symbols used to perform arithmetic, logical, or other operations on the given variables/ values/ data. You can also conduct comparison operations to analyze relationships between values/ operands, like checking if a number is equal to or lesser than the other, etc. This is where the relational operators in Python language come into play.
In this article, we will focus specifically on relational operators in Python, their syntax, relevant examples, and more.
What Are Relational Operators In Python?
Relational operators in Python programming are generally used to compare the operands on either side, draw inferences, or check conditions. They are, hence, also known as comparison operators.
- They serve the basic purpose of conducting relational comparisons between operands, thus facilitating the decision-making process in a program.
- The basic mechanism behind the working of comparison operators is to check the relation between two operands by comparing their values and return a Boolean value.
- If the comparison of the operand values produces a result that meets the logical condition (pre-defined), the statement returns True, and that block is executed.
- If the result of the comparison of values does not meet the set logical condition, then the statement returns False, and the respective block is executed.
- After the task assigned to be done in the case of True or False is executed, the program moves to the next line of code.
- Other uses of relational operators include controlling the program flow, filtering data, etc. These operators can be used with numerical values, strings, and objects.
Type Of Relational Operators In Python
There are six different symbols relational operators in Python that are used to compare the left operand to the right operand and return the result of comparison. They are described below in the table below.
Operator |
Name |
Description |
Syntax |
---|---|---|---|
> |
Greater than |
Returns True if the value of the left variable is greater than that of the right variable; otherwise, False. |
x > y |
< |
Less than |
Returns True if the value of the left variable is smaller than that of the right variable; otherwise, False. |
x < y |
>= |
Greater than or equal to |
Returns True if the value of the left variable is greater than or equal to that of the right variable; otherwise, it is False. |
x >= y |
<= |
Less than or equal to |
Returns True if the value of the left variable is less than or equal to that of the right variable; otherwise, False. |
x <= y |
== |
Equal to |
Returns True if the value of the left variable is equal to that of the right variable; otherwise, False. |
x == y |
!= |
Not equal to |
Returns True if the value of the left variable is not equal to that of the right variable; otherwise, False. |
x !=y |
The table shows six basic relational operators in Python programming language, each serving a specific purpose in making comparisons. Some of the rules and guidelines to keep in mind while using relational operators in a Python program are:
- Precedence of Comparison Operators: These operators have a pre-defined order that determines the sequence of their evaluation in a program. For instance, a<b<=c is evaluated as a<b and then b<=c.
- Type Compatibility: These operators can only be used to compare variables of the same data type. For instance, we cannot compare an integer number to a string type.
- Truth value: A syntax using relational operators always returns a Boolean value as output, which can be either True or False.
- Short circuit Evaluation: An expression may not be evaluated completely if the result is already known. For instance, x<y & x>z is evaluated only if x<y is True.
Equal-To Relational Operator In Python
This operator is also called the relational equality operator and is represented by the symbol (==). The equality operator compares two values and returns True if they are equal and False otherwise. It can be used in code readability and code implementation within conditional statements such as if-else, while loop, and for loop.
The time complexity of the equal-to operator is constant, i.e., O(1) for the simple data types such as integers, floats, and booleans, and O(n) for the complex data types such as strings and objects. This is because the operator can directly compare the values for the simple data types, while it may need to compare each data type element for the complex ones.
Below is a simple Python program example illustrating this relational operator.
Code Example:
#Declaring and initializing a variable
x = 13
# Check if x is even
if x % 2 == 0:
print("x is even")
else:
print("x is not even")
Output:
x is not even
Explanation:
In the example Python program-
- We begin by declaring and initializing a variable x with the value 13.
- Then, we use an if-else statement to check if the remainder of x divided by 2 is equal to zero.
- Here, we use the modulus arithmetic operator (%) to calculate the remainder and the equal to (==) relational operator to compare the two values.
- If the remainder equals 0, the if-block is executed, printing the string message-"x is even" to the console. Otherwise, the else-block prints "x is not even."
- In this case, since 13 % 2 equals 1 (as 13 is an odd number), the else block is executed, and the program outputs "x is not even" to the console.
Also read- Python IDLE | The Ultimate Beginner's Guide With Images & Codes
Greater Than Relational Operator In Python
As the name suggests, this relational operator is represented by the greater than symbol (>). It compares two values and returns True if the value of the left operand is greater than the right operand; False otherwise.
The time complexity of this relational operator in Python is constant, i.e., O(1) for simple data types such as integers, doubles, and characters, and O(n) for complex data types such as lists of strings. This is because the operator can directly compare the values for the simple data types while it may need to iterate over each data type element for the complex ones.
Look at the Python program example given below to understand how this operator is implemented.
Code Example:
#Creating variables for comparison
x = 13
y = 9
# Check if x is greater than y
if x > y:
print("x is greater than y")
Output:
x is greater than y.
Explanation:
In the Python program sample-
- We declare and initialize two integer variables, x and y, with the values 13 and 9, respectively.
- Next, we use an if statement to check if the value of x is greater than that of y using the greater than relational operator (>).
- If the condition is true (i.e., x is greater than y), the print() statement inside the if-block is executed by printing the statement "x is greater than y."
- If the condition is false, the control moves to the next line of code.
- In this case, since x is assigned the value 13 and y is assigned the value 9, the condition x > y is true. So, the if-block executes the print statement, displaying "x is greater than y" to the console.
Less-Than Relational Operator In Python
The less than operator is denoted by the mathematical less than symbol (<). It compares two given values and returns True if the value of the left operand is smaller than the right operand and False otherwise.
The time complexity of the less than relational operator in Python (<) is constant, i.e. O(1). This implies that the time taken for this comparison is independent of the size of the input.
Code Example:
#Creating two variables
x = 27
y = 39
# Check if x is smaller than y
if x < y:
print("x is smaller than y")
Output:
x is smaller than y.
Explanation:
In the sample Python program-
- We declare and initialize two integer variables, x and y, with the values 27 and 39, respectively.
- Next, we use an if statement to check if the value of x is smaller than the value of y using the less than relational operator (<).
- If the condition is true, the if-block is executed which contains an print() statement to display the message- "x is smaller than y."
- In this specific case, since x is assigned the value 27 and y is assigned the value 39, the condition x<y is true, and the program executes the print statement, displaying "x is smaller than y" to the console.
Not Equal-To Relational Operator In Python
The not equal to operator, also known as the inequality operator, is denoted by the (!=) symbol. This relational operator in Python performs comparison between two given values and returns False if both the right and left operands are equal and True otherwise.
The time complexity of the inequality operator (!=) is constant, i.e., O(1). This means that the time taken for the comparison is independent of the size of the input provided.
Code Example:
#Initializing two variables
x = 23
y = 23
# Check if x is not equal to y
if x != y:
print("x is not equal to y")
else:
print("x is equal to y")
Output:
x is equal to y
Explanation:
In the example Python code-
- We declare and initialize two integer variables, x and y, with the values 23 for both.
- Next, we use an if-else statement to check if the value of 'x' is not equal to the value of 'y' using the not equal to relational operator (!=).
- If the condition is true (i.e., x is not equal to y), the if-block is executed the enclosed print() statement outputting the message- "x is not equal to y."
- If the condition is false, (i.e., x is equal to y), the else-block is executed printing the string message- "x is equal to y."
- In this specific case, since both x and y are assigned the value 23, the condition x != y is false, and the program executes the else block, printing "x is equal to y" to the console.
Greater-Than Or Equal-To Relational Operator In Python
The greater-than or equal-to operator is a compound comparison operator denoted by the (>=) symbol. It compares the left operand with the right one and returns True if the value on the left is greater than that on the right and False otherwise.
The time complexity of this relational operator in Python is constant, i.e., O(1). This means that the time taken for the comparison is independent of the input size.
Code Example:
#Initializing variables to be used as operands
a = 24
b = 13
# Check if a is greater than or equal to b
if a >= b:
print("a is greater than or equal to b")
Output:
a is greater than or equal to b.
Explanation:
In the Python code example-
- We declare and initialize two integer variables, a and b, with the values 24 and 13, respectively.
- Next, we use the if statement to compare the values of a and b using the greater-than-or-equal-to operator (>=).
- The condition a >= b checks whether the value of a is greater than or equal to the value of b. If the condition is true, the if-block is executed printing the respective message to the console.
- If the condition is false, the code block inside the if statement is skipped.
- In this case, since the condition is true, the print() statement displays the message- "a is greater than or equal to b" to the console.
Less-Than Or Equal-To Relational Operator In Python
The less than or equal to operator is denoted by the compound symbol (<=). It compare two values and return True if the left one is smaller than the right; otherwise, it returns False.
The time complexity of this relational operator in Python is constant, i.e., O(1). This means that the time taken for the comparison is independent of the size of the input.
Code Example:
# Assign values to variables
a = 32
b = 64
# Check if a is less than or equal to b
if a <= b:
# If the condition is true, execute the following block of code
print("a is less than or equal to b")
Output:
a is less than or equal to b.
Explanation:
In the sample Python code-
- We first initialize two integer variables, a and b, with the values 32 and 64, respectively.
- Then, we use the less-than-or-equal-to relational operator (<=) inside an if statement to compare the values of a and b. The condition (a <= b) checks whether the value of a is less than or equal to the value of b.
- If the condition is true, the if-block gets executed, and a descriptive string is printed to the console.
- If the condition is false, the code block under the if statement is skipped.
- Since in this example the condition is true, the if-block prints the message- :a is less than or equal to b" to the console.
Why And When To Use Relational Operators?
Relational operators simplify various tasks in Python programs, making them more efficient and easier to read. These are used to check the relation between two values, like, whether they are equal or not, if one is greater or lesser than the other, etc. The general purposes they serve in programming are elaborated below.
Decision Making: This is the most significant use of relational operators in Python to make decisions in a program by using the if-else conditional statements. For example, the if-block is executed if the condition is met, otherwise the else-block is executed.
Code Example:
#Using an if-else statement with a relational operator for decision-making
if 2 <= 3:
print("Yes")
else:
print("No")
Output:
Yes
Explanation:
In the Python code sample-
- We use an if-else statement to compare values 2 and 3 using the less than or equal to (<=) relational operator.
- If the condition 2 <= 3 evaluates to true, the if-block is executed printing "Yes" to the console.
- If the condition is false, the program executes the else block of code, thus printing "No."
- In this case, since 2 is less than or equal to 3, the condition (2 <= 3) is true.
- As a result, the program executes the code under the if statement, and "yes" is printed to the console.
Control The Flow Of The program: We can use relational operators inside Python loops to iterate over a sequence of values and perform operations as needed. In the example below we will see how to use relational operators in Python with a for loop to print values.
Code Example:
#Using a for loop to print a range of values
for i in range(4):
print(i, end= " ")
Output:
0 1 2 3
Explanation:
In the Python code snippet-
- We use a for loop to iterate over a range of values from 0 to 4 (exclusive). We use the range() function with argument 4 to signify the end of the range.
- In each iteration of the loop, the variable i takes on the successive values from the specified range.
- The print() statement within the loop then outputs the current value of i to the console.
- Note that since the print() function by default shifts the cursor to the next line, we use the end parameter with white space to print the output without a newline (in a single line).
- So, for each iteration of the loop, the program prints the values 0, 1, 2, 3, 4, and 5 to the console.
- The range function generates a sequence of numbers, and the loop iterates through each one, printing it one by one.
Data Filtering: Relational operators in Python are also used to filter data, for example, by creating a new list from the given list. This new list contains only the values that satisfy the conditions specified in the syntax.
Code Example:
#Creating an initial list
nums = [1, 2, 3, 4, 5, 6, 7]
#Using relational operators to filter data
odds = [number for number in nums if number % 2 != 0]
#Printing filtered data
print(odds)
Output:
[1, 3, 5, 7]
Explanation:
In the Python code-
- We first declare and initialize a list named nums with the values [1, 2, 3, 4, 5, 6, 7].
- Then, we create another Python list called odds using list comprehension to filter and add values to it.
- The list comprehension iterates through each element in the nums list and includes only those elements for which the condition (number % 2 != 0) is true.
- This condition uses not equal to relational operator to check if the element is not evenly divisible by 2, meaning it is an odd number.
- After the list comprehension, the odds list contains only the odd numbers from the original nums list.
- Finally, we use a print() statement to output the contents of the odds list to the console, displaying [1, 3, 5, 7].
Precedence & Associativity Of Relational Operators In Python
Precedence and associativity are crucial concepts in programming when working with operators. They determine the order in which operators are evaluated in an expression. Understanding these concepts is essential for writing correct and efficient code, especially when dealing with complex expressions.
-
Precedence: This indicates the relative priority of the operators. Operators with higher precedence are evaluated before operators with lower precedence. When operators have the same precedence level, their associativity determines the order of evaluation.
-
Associativity: This specifies the direction in which operators of the same precedence level are evaluated. For relational operators in Python, the associativity is left-to-right, meaning expressions are evaluated from left to right.
Here’s a table summarizing the precedence and associativity of the six relational operators in Python:
Operator | Description | Example | Precedence | Associativity |
---|---|---|---|---|
< | Less than | a<b | Equal | Left-to-Right |
<= | Less than or equal to | a<=b | Equal | Left-to-Right |
> | Greater than | a>b | Equal | Left-to-Right |
>= | Greater than or equal to | a>=b | Equal | Left-to-Right |
== | Equal to | a==b | Equal | Left-to-Right |
!= | Not equal to | a!=b | Equal | Left-to-Right |
For example, let's consider a situation where we are chaining comparison operators in a single condition.
result = 3 < 7 <= 12
print(result) # Output: True
In the expression 3 < 7 <= 12, Python evaluates 3 < 7 first, and if it's True, it then evaluates 7 <= 12. Since both conditions are True, the final result is True.
This behaviour highlights the concept of operator chaining in Python. When chaining comparison operators, you can combine multiple relational operators in a single expression. Here, each comparison is evaluated from left to right. The overall expression returns True only if all individual comparisons are True.
Overriding Precedence With Parentheses
While the default precedence and associativity determine the evaluation order, you can override this order using parentheses. Parentheses have the highest precedence in Python, so any expression inside parentheses is evaluated first.
result = (3 < 7) and (12 > 10)
print(result) # Output: True
In this example, using parentheses ensures that comparisons are evaluated separately before applying the logical AND operator.
Understanding precedence, associativity, and operator chaining is crucial when combining multiple operators in an expression. It ensures the correct evaluation of complex expressions and helps avoid logical errors in your code. You can write more precise and efficient Python programs by mastering these concepts.
Advantages & Disadvantages Of Relational Operators In Python
Advantages of Relational Operators in Python:
- Simplicity: Relational operators provide a simple and concise way to express conditions or relationships between values in Python programs.
- Readability: Code that uses relational operators is often more readable and easier to understand than code with complex nested conditions.
- Ease of Use: Relational operators in Python are straightforward, making it easier for developers, especially those new to programming, to write conditionals.
- Expressiveness: Relational operators allow you to express relationships between values naturally and intuitively, contributing to the expressiveness of the language.
- Compatibility: Relational operators work with a wide range of data types, providing flexibility and compatibility across different variable types.
Disadvantages of Relational Operators in Python:
- Limited Expressiveness: While relational operators are powerful, they might be insufficient for expressing complex conditions that involve multiple variables or intricate logic.
- Not Suitable for All Scenarios: In some cases, more advanced conditionals, such as those requiring complex mathematical or string manipulations, may be better addressed using other constructs like functions or custom comparison logic.
- Potential for Chained Conditions: Chaining multiple relational operators in Python programs may lead to confusion or mistakes if not handled carefully. For example, the order of evaluation is important, and unexpected results may occur if parentheses are not used appropriately.
- May Not Cover All Scenarios: Relational operators are primarily designed for basic comparisons, and they may not cover all scenarios where more sophisticated conditions are needed.
- Equality Issues with Floating-Point Numbers: When comparing floating-point numbers, precision issues may arise, and direct equality checks using relational operators may not always yield the expected results. It's recommended to use tolerance thresholds for such comparisons.
Real-World Applications Of Relational Operators In Python
Relational operators find extensive use in a variety of scenarios:
- User Authentication: In user authentication systems that follow the core concept of truthiness, relational operators are used to check whether the entered password matches the stored password for a given user.
- Sorting Algorithms: Custom comparison functions in sorting algorithms often leverage relational comparative operators to define the order of elements.
- Input Validation: Relational operators are crucial in validating user inputs to ensure they meet certain requirements.
- Boolean Expressions: In constructing complex boolean expressions for intricate decision-making in programs.
- Stock Market Analysis: In financial applications, relational operators can be used to analyze stock prices for internet users and make decisions based on market trends.
- Game Development: In game development, relational operators are employed to manage various aspects, such as player scores, achievements, and level progression.
Conclusion
Relational operators in Python are a part of a core concept in programming, i.e., operators. They are fundamental for making logical comparisons between values. Whether used in conditional statements or for data analysis, these operators provide a concise and readable way to express relationships between variables.
Understanding how to use and combine relational operators in Python programming is essential for writing efficient code. As you explore more complex programming tasks and a collection of code snippets, a solid grasp of these operators will undoubtedly enhance your ability to create robust and functional programs.
Frequently Asked Questions
Q1. What is an expression in Python?
The combination/series of a list of operators and operands form expressions in Python. They are used to perform a variety of tasks, including calculation, comparison, and flow control of a program.
For example,
- (5*2) is an expression.
- (“hello”+“world”) is an expression.
In the context of relational operators, expressions that consist of these operators are referred to as relational expressions/ conditional expressions. Similarly, an expression consisting of logical operators can be referred to as a logical expression.
Q2. What is an operand function?
An operand function in Python is a function that takes an operand of any data type as input and returns a value. These functions can be used to determine the type of operand at runtime.
Code Example:
def operand_func(operand):
if isinstance(operand, int):
return "integer"
elif isinstance(operand, str):
return "string"
else:
return "unknown"
a = 3
b = "unstop"
c = "4.17"
print(operand_func(a))
print(operand_func(b))
print(operand_func(c))
Output:
integer
string
unknown
Explanation:
The operand_func function takes an argument 'operand' and checks its type using the isinstance function. If 'operand' is an integer, it returns "integer"; if it's a string, it returns "string"; otherwise, it returns "unknown".
The code then declares variables 'a', 'b', and 'c' with different types and prints the results of calling operand_func on each variable, indicating their respective types: "integer" for 'a' (since it's an integer), "string" for 'b' (as it's a string), and "unknown" for 'c' (since it's not an integer or string).
Q3. What are the two types of operands?
The two basic types of operands in Python are Literal and Variable.
- Immediate operands are the literal values stored directly in an instruction. For example, the instruction MOV AX, 3 has an immediate operand of 5.
- Memory Operands are the addresses of memory locations that contain the values to be operated on. For example, the instruction MOV AX, [BX] has a memory operand of the address stored in the BX.
Q4. How many operands does a relational operator take?
Relational operators in Python take two operands as input, often referred to as the left and right operands. These are then compared according to the relational operator used in the syntax. For example, in the single expression 7 >= 3, 7 and 3 are considered as the left and right operands, respectively, thus returning True as the output.
Q5. What are the operators with one operand?
In Python, the operators with one operand are known as the unary operators. They perform operations on a single operand and return a single value.
For example,
- -5 uses the negative operator (-) to perform the negation of the given integer value.
- !True uses the logical negation operator (!) to invert the boolean value and return False as the result.
Q6. Can relational operators be used within an if condition?
Yes, the relational operators can be used within the if-else conditions or while and for looping statements to serve the purpose of decision-making and flow control of the program. Moreover, ‘if’ statements are the most common syntax used by relational operators in a program.
Code Example:
a = 18
b = 18
if a == b:
print("a and b are equal.")
Output:
a and b are equal.
Q7. Explain the concept of combining multiple conditions in Python using relational operators.
In Python, you can combine multiple conditions using relational operators and logical operators to make a more expressive code. The keywords for expressing a combination of conditions are:
- and: Used to create a compound condition where both conditions must be true.
- or: Used to create a complex condition where at least one of the conditions must be true.
- not: Used to negate a condition.
These combinations of conditions are often referred to as combined conditions or compound conditions.
Q8. What are the different type of operators in Python?
There are seven primary types of operators used in Python programming, including:
- Arithmetic Operators: These are used to perform various mathematical operations on the input numbers, such as addition (+), multiplication (*), subtraction (-), etc.
- Assignment Operators: They are used to assign a specified value to the given variable, such as the assignment equality operator.
- Logical Operators: These operators are used to combine Boolean values to return another Boolean value as the output. Included in logical operators are AND (and), OR (or), and NOT (not).
- Bitwise Operators: These perform bit-level operations on the given numbers using the AND (&), OR (|), etc.
- Identity Operators: They compare two input entities to check if they are the same object with the same memory location or not. They include the is and is not operators.
- Membership Operators: These operators (including in and not in) check if a specified value exists in a given sequence or not.
And lastly, we have the relational operators in Python, which we will discuss in depth above.
Do check out the following:
- Python Program To Add Two Numbers | 8 Techniques With Code Examples
- Python Namespace & Variable Scope Explained (With Code Examples)
- Python String.Replace() And 8 Other Ways Explained (+Examples)
- Python Program To Convert Kilometers To Miles (+Detailed Examples)
- Difference Between List And Tuple In Python Explained (+Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Ujjwal Sharma 2 weeks ago
Vishwajeet Singh 1 month ago
niyati m singh 1 month ago
Pardha Venkata Sai Patnam 1 month ago
Shivam Kumar Jha 1 month ago