Table of content:
- What Is Python? An Introduction
- What Is The History Of Python?
- Key Features Of The Python Programming Language
- Who Uses Python?
- Basic Characteristics Of Python Programming Syntax
- Why Should You Learn Python?
- Applications Of Python Language
- Advantages And Disadvantages Of Python
- Some Useful Python Tips & Tricks For Efficient Programming
- Python 2 Vs. Python 3: Which Should You Learn?
- Python Libraries
- Conclusion
- Frequently Asked Questions
- It's Python Basics Quiz Time!
Table of content:
- Python At A Glance
- Key Features of Python Programming
- Applications of Python
- Bonus: Interesting features of different programming languages
- Summing up...
- FAQs regarding Python
- Take A Quiz To Rehash Python's Features!
Table of content:
- What Is Python IDLE?
- What Is Python Shell & Its Uses?
- Primary Features Of Python IDLE
- How To Use Python IDLE Shell? Setting Up Your Python Environment
- How To Work With Files In Python IDLE?
- How To Execute A File In Python IDLE?
- Improving Workflow In Python IDLE Software
- Debugging In Python IDLE
- Customizing Python IDLE
- Code Examples
- Conclusion
- Frequently Asked Questions (FAQs)
- How Well Do You Know IDLE? Take A Quiz!
Table of content:
- What Is A Variable In Python?
- Creating And Declaring Python Variables
- Rules For Naming Python Variables
- How To Print Python Variables?
- How To Delete A Python Variable?
- Various Methods Of Variables Assignment In Python
- Python Variable Types
- Python Variable Scope
- Concatenating Python Variables
- Object Identity & Object References Of Python Variables
- Reserved Words/ Keywords & Python Variable Names
- Conclusion
- Frequently Asked Questions
- Rehash Python Variables Basics With A Quiz!
Table of content:
- What Is A String In Python?
- Creating String In Python
- How To Create Multiline Python Strings?
- Reassigning Python Strings
- Accessing Characters Of Python Strings
- How To Update Or Delete A Python String?
- Reversing A Python String
- Formatting Python Strings
- Concatenation & Comparison Of Python Strings
- Python String Operators
- Python String Functions
- Escape Sequences In Python Strings
- Conclusion
- Frequently Asked Questions
- Rehash Python Strings Basics With A Quiz!
Table of content:
- What Is Python Namespace?
- Lifetime Of Python Namespace
- Types Of Python Namespace
- The Built-In Namespace In Python
- The Global Namespace In Python
- The Local Namespace In Python
- The Enclosing Namespace In Python
- Variable Scope & Namespace In Python
- Python Namespace Dictionaries
- Changing Variables Out Of Their Scope & Python Namespace
- Best Practices Of Python Namespace
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Namespaces!
Table of content:
- What Are Logical Operators In Python?
- The AND Python Logical Operator
- The OR Python Logical Operator
- The NOT Python Logical Operator
- Short-Circuiting Evaluation Of Python Logical Operators
- Precedence of Logical Operators In Python
- How Does Python Calculate Truth Value?
- Final Note On How AND & OR Python Logical Operators Work
- Conclusion
- Frequently Asked Questions
- Python Logical Operators Quiz– Test Your Knowledge!
Table of content:
- What Are Bitwise Operators In Python?
- List Of Python Bitwise Operators
- AND Python Bitwise Operator
- OR Python Bitwise Operator
- NOT Python Bitwise Operator
- XOR Python Bitwise Operator
- Right Shift Python Bitwise Operator
- Left Shift Python Bitwise Operator
- Python Bitwise Operations And Negative Integers
- The Binary Number System
- Application of Python Bitwise Operators
- Python Bitwise Operator Overloading
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Bitwise Operators!
Table of content:
- What Is The Print() Function In Python?
- How Does The print() Function Work In Python?
- How To Print Single & Multi-line Strings In Python?
- How To Print Built-in Data Types In Python?
- Print() Function In Python For Values Stored In Variables
- Print() Function In Python With sep Parameter
- Print() Function In Python With end Parameter
- Print() Function In Python With flush Parameter
- Print() Function In Python With file Parameter
- How To Remove Newline From print() Function In Python?
- Use Cases Of The print() Function In Python
- Understanding Print Statement In Python 2 Vs. Python 3
- Conclusion
- Frequently Asked Questions
- Know The print() Function In Python? Take A Quiz!
Table of content:
- Working Of Normal Print() Function
- The New Line Character In Python
- How To Print Without Newline In Python | Using The End Parameter
- How To Print Without Newline In Python 2.x? | Using Comma Operator
- How To Print Without Newline In Python 3.x?
- How To Print Without Newline In Python With Module Sys
- The Star Pattern(*) | How To Print Without Newline & Space In Python
- How To Print A List Without Newline In Python?
- How To Remove New Lines In Python?
- Conclusion
- Frequently Asked Questions
- Think You Can Print Without a Newline in Python? Prove It!
Table of content:
- What Is A Python For Loop?
- How Does Python For Loop Work?
- When & Why To Use Python For Loops?
- Python For Loop Examples
- What Is Rrange() Function In Python?
- Nested For Loops In Python
- Python For Loop With Continue & Break Statements
- Python For Loop With Pass Statement
- Else Statement In Python For Loop
- Conclusion
- Frequently Asked Questions
- Think You Know Python's For Loop? Prove It!
Table of content:
- What Is Python While Loop?
- How Does The Python While Loop Work?
- How To Use Python While Loops For Iterations?
- Control Statements In Python While Loop With Examples
- Python While Loop With Python List
- Infinite Python While Loop in Python
- Python While Loop Multiple Conditions
- Nested Python While Loops
- Conclusion
- Frequently Asked Questions
- Mastered Python While Loop? Let’s Find Out!
Table of content:
- What Are Conditional If-Else Statements In Python?
- Types Of If-Else Statements In Python
- If Statement In Python
- If-Else Statement In Python
- Nested If-Else Statement In Python
- Elif Statement In Python
- Ladder If-Elif-Else Statement In Python
- Short Hand If-Statement In Python
- Short Hand If-Else Statement In Python
- Operators & If-Esle Statement In Python
- Other Statements With If-Else In Python
- Conclusion
- Frequently Asked Questions
- Quick If-Else Statement Quiz– Let’s Go!
Table of content:
- What Is Control Structure In Python?
- Types Of Control Structures In Python
- Sequential Control Structures In Python
- Decision-Making Control Structures In Python
- Repetition Control Structures In Python
- Benefits Of Using Control Structures In Python
- Conclusion
- Frequently Asked Questions
- Control Structures in Python – Are You the Master? Take A Quiz!
Table of content:
- What Are Python Libraries?
- How Do Python Libraries Work?
- Standard Python Libraries (With List)
- Important Python Libraries For Data Science
- Important Python Libraries For Machine & Deep Learning
- Other Important Python Libraries You Must Know
- Working With Third-Party Python Libraries
- Troubleshooting Common Issues For Python Libraries
- Python Libraries In Larger Projects
- Importance Of Python Libraries
- Conclusion
- Frequently Asked Questions
- Quick Quiz On Python Libraries – Let’s Go!
Table of content:
- What Are Python Functions?
- How To Create/ Define Functions In Python?
- How To Call A Python Function?
- Types Of Python Functions Based On Parameters & Return Statement
- Rules & Best Practices For Naming Python Functions
- Basic Types of Python Functions
- The Return Statement In Python Functions
- Types Of Arguments In Python Functions
- Docstring In Python Functions
- Passing Parameters In Python Functions
- Python Function Variables | Scope & Lifetime
- Advantages Of Using Python Functions
- Recursive Python Function
- Anonymous/ Lambda Function In Python
- Nested Functions In Python
- Conclusion
- Frequently Asked Questions
- Python Functions – Test Your Knowledge With A Quiz!
Table of content:
- What Are Python Built-In Functions?
- Mathematical Python Built-In Functions
- Python Built-In Functions For Strings
- Input/ Output Built-In Functions In Python
- List & Tuple Python Built-In Functions
- File Handling Python Built-In Functions
- Python Built-In Functions For Dictionary
- Type Conversion Python Built-In Functions
- Basic Python Built-In Functions
- List Of Python Built-In Functions (Alphabetical)
- Conclusion
- Frequently Asked Questions
- Think You Know Python Built-in Functions? Prove It!
Table of content:
- What Is A round() Function In Python?
- How Does Python round() Function Work?
- Python round() Function If The Second Parameter Is Missing
- Python round() Function If The Second Parameter Is Present
- Python round() Function With Negative Integers
- Python round() Function With Math Library
- Python round() Function With Numpy Module
- Round Up And Round Down Numbers In Python
- Truncation Vs Rounding In Python
- Practical Applications Of Python round() Function
- Conclusion
- Frequently Asked Questions
- Revisit Python’s round() Function – Take The Quiz!
Table of content:
- What Is Python pow() Function?
- Python pow() Function Example
- Python pow() Function With Modulus (Three Parameters)
- Python pow() Function With Complex Numbers
- Python pow() Function With Floating-Point Arguments And Modulus
- Python pow() Function Implementation Cases
- Difference Between Inbuilt-pow() And math.pow() Function
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python’s pow() Function!
Table of content:
- Python max() Function With Objects
- Examples Of Python max() Function With Objects
- Python max() Function With Iterable
- Examples Of Python max() Function With Iterables
- Potential Errors With The Python max() Function
- Python max() Function Vs. Python min() Functions
- Conclusion
- Frequently Asked Questions
- Think You Know Python max() Function? Take A Quiz!
Table of content:
- What Are Strings In Python?
- What Are Python String Methods?
- List Of Python String Methods For Manipulating Case
- List Of Python String Methods For Searching & Finding
- List Of Python String Methods For Modifying & Transforming
- List Of Python String Methods For Checking Conditions
- List Of Python String Methods For Encoding & Decoding
- List Of Python String Methods For Stripping & Trimming
- List Of Python String Methods For Formatting
- Miscellaneous Python String Methods
- List Of Other Python String Operations
- Conclusion
- Frequently Asked Questions
- Mastered Python String Methods? Take A Quiz!
Table of content:
- What Is Python String?
- The Need For Python String Replacement
- The Python String replace() Method
- Multiple Replacements With Python String.replace() Method
- Replace A Character In String Using For Loop In Python
- Python String Replacement Using Slicing Method
- Replace A Character At a Given Position In Python String
- Replace Multiple Substrings With The Same String In Python
- Python String Replacement Using Regex Pattern
- Python String Replacement Using List Comprehension & Join() Method
- Python String Replacement Using Callback With re.sub() Method
- Python String Replacement With re.subn() Method
- Conclusion
- Frequently Asked Questions
- Know How To Replace Python Strings? Prove It!
Table of content:
- What Is String Slicing In Python?
- How Indexing & String Slicing Works In Python
- Extracting All Characters Using String Slicing In Python
- Extracting Characters Before & After Specific Position Using String Slicing In Python
- Extracting Characters Between Two Intervals Using String Slicing In Python
- Extracting Characters At Specific Intervals (Step) Using String Slicing In Python
- Negative Indexing & String Slicing In Python
- Handling Out-of-Bounds Indices In String Slicing In Python
- The slice() Method For String Slicing In Python
- Common Pitfalls Of String Slicing In Python
- Real-World Applications Of String Slicing
- Conclusion
- Frequently Asked Questions
- Quick Python String Slicing Quiz– Let’s Go!
Table of content:
- Introduction To Python List
- How To Create A Python List?
- How To Access Elements Of Python List?
- Accessing Multiple Elements From A Python List (Slicing)
- Access List Elements From Nested Python Lists
- How To Change Elements In Python Lists?
- How To Add Elements To Python Lists?
- Delete/ Remove Elements From Python Lists
- How To Create Copies Of Python Lists?
- Repeating Python Lists
- Ways To Iterate Over Python Lists
- How To Reverse A Python List?
- How To Sort Items Of Python Lists?
- Built-in Functions For Operations On Python Lists
- Conclusion
- Frequently Asked Questions
- Revisit Python Lists Basics With A Quick Quiz!
Table of content:
- What Is List Comprehension In Python?
- Incorporating Conditional Statements With List Comprehension In Python
- List Comprehension In Python With range()
- Filtering Lists Effectively With List Comprehension In Python
- Nested Loops With List Comprehension In Python
- Flattening Nested Lists With List Comprehension In Python
- Handling Exceptions In List Comprehension In Python
- Common Use Cases For List Comprehensions
- Advantages & Disadvantages Of List Comprehension In Python
- Best Practices For Using List Comprehension In Python
- Performance Considerations For List Comprehension In Python
- For Loops & List Comprehension In Python: A Comparison
- Difference Between Generator Expression & List Comprehension In Python
- Conclusion
- Frequently Asked Questions
- Rehash Python List Comprehension Basics With A Quiz!
Table of content:
- What Is A List In Python?
- How To Find Length Of List In Python?
- For Loop To Get Python List Length (Naive Approach)
- The len() Function To Get Length Of List In Python
- The length_hint() Function To Find Length Of List In Python
- The sum() Function To Find The Length Of List In Python
- The enumerate() Function To Find Python List Length
- The Counter Class From collections To Find Python List Length
- The List Comprehension To Find Python List Length
- Find The Length Of List In Python Using Recursion
- Comparison Between Ways To Find Python List Length
- Conclusion
- Frequently Asked Questions
- Know How To Get Python List Length? Prove it!
Table of content:
- List of Methods To Reverse A Python List
- Python Reverse List Using reverse() Method
- Python Reverse List Using the Slice Operator ([::-1])
- Python Reverse List By Swapping Elements
- Python Reverse List Using The reversed() Function
- Python Reverse List Using A for Loop
- Python Reverse List Using While Loop
- Python Reverse List Using List Comprehension
- Python Reverse List Using List Indexing
- Python Reverse List Using The range() Function
- Python Reverse List Using NumPy
- Comparison Of Ways To Reverse A Python List
- Conclusion
- Frequently Asked Questions
- Time To Test Your Python List Reversal Skills!
Table of content:
- What Is Indexing In Python?
- The Python List index() Function
- How To Use Python List index() To Find Index Of A List Element
- The Python List index() Method With Single Parameter (Start)
- The Python List index() Method With Start & Stop Parameters
- What Happens When We Use Python List index() For An Element That Doesn't Exist
- Python List index() With Nested Lists
- Fixing IndexError Using The Python List index() Method
- Python List index() With Enumerate()
- Real-world Examples Of Python List index() Method
- Difference Between find() And index() Method In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python List Indexing? Take A Quiz!
Table of content:
- How To Remove Elements From List In Python?
- The remove() Method To Remove Element From Python List
- The pop() Method To Remove Element From List In Python
- The del Keyword To Remove Element From List In Python
- The clear() Method To Remove Elements From Python List
- List Comprehensions To Conditionally Remove Element From List In Python
- Key Considerations For Removing Elements From Python Lists
- Why We Need to Remove Elements From Python List
- Performance Comparison Of Methods To Remove Element From List In Python
- Conclusion
- Frequently Asked Questions
- Quiz– Prove You Know How To Remove Item From Python Lists!
Table of content:
- How To Remove Duplicates From A List In Python?
- The set() Function To Remove Duplicates From Python List
- Remove Duplicates From Python List Using For Loop
- Using List Comprehension Remove Duplicates From Python List
- Remove Duplicates From Python List Using enumerate() With List Comprehension
- Dictionary & fromkeys() Method To Remove Duplicates From Python List
- Remove Duplicates From Python List Using in, not in Operators
- Remove Duplicates From Python List Using collections.OrderedDict.fromkeys()
- Remove Duplicates From Python List Using Counter with freq.dist() Method
- The del Keyword Remove Duplicates From Python List
- Remove Duplicates From Python List Using DataFrame
- Remove Duplicates From Python List Using pd.unique and np.unipue
- Remove Duplicates From Python List Using reduce() function
- Comparative Analysis Of Ways To Remove Duplicates From Python List
- Conclusion
- Frequently Asked Questions
- Think You Know How to Remove Duplicates? Take A Quiz!
Table of content:
- What Is Python List & How To Access Elements?
- What Is IndexError: List Index Out Of Range & Its Causes In Python?
- Understanding Indexing Behavior In Python Lists
- How to Prevent/ Fix IndexError: List Index Out Of Range In Python
- Handling IndexError Gracefully Using Try-Except
- Debugging Tips For IndexError: List Index Out Of Range Python
- Conclusion
- Frequently Asked Questions
- Avoiding ‘List Index Out of Range’ Errors? Take A Quiz!
Table of content:
- What Is the Python sort() List Method?
- Sorting In Ascending Order Using The Python sort() List Method
- How To Sort Items In Descending Order Using Python sort() List Method
- Custom Sorting Using The Key Parameter Of Python sort() List Method
- Examples Of Python sort() List Method
- What Is The sorted() List Method In Python
- Differences Between sorted() And sort() List Methods In Python
- When To Use sorted() & When To Use sort() List Method In Python
- Conclusion
- Frequently Asked Questions
- Take A Quick Python's sort() Quiz!
Table of content:
- What Is A List In Python?
- What Is A String In Python?
- Why Convert Python List To String?
- How To Convert List To String In Python?
- The join() Method To Convert Python List To String
- Convert Python List To String Through Iteration
- Convert Python List To String With List Comprehension
- The map() Function To Convert Python List To String
- Convert Python List to String Using format() Function
- Convert Python List To String Using Recursion
- Enumeration Function To Convert Python List To String
- Convert Python List To String Using Operator Module
- Python Program To Convert String To List
- Conclusion
- Frequently Asked Questions
- Convert Lists To Strings Like A Pro! Take A Quiz
Table of content:
- What Is Inheritance In Python?
- Python Inheritance Syntax
- Parent Class In Python Inheritance
- Child Class In Python Inheritance
- The __init__() Method In Python Inheritance
- The super() Function In Python Inheritance
- Method Overriding In Python Inheritance
- Types Of Inheritance In Python
- Special Functions In Python Inheritance
- Advantages & Disadvantages Of Inheritance In Python
- Common Use Cases For Inheritance In Python
- Best Practices for Implementing Inheritance in Python
- Avoiding Common Pitfalls in Python Inheritance
- Conclusion
- Frequently Asked Questions
- 💡 Python Inheritance Quiz – Are You Ready?
Table of content:
- What Is The Python List append() Method?
- Adding Elements To A Python List Using append()
- Populate A Python List Using append()
- Adding Different Data Types To Python List Using append()
- Adding A List To Python List Using append()
- Nested Lists With Python List append() Method
- Practical Use Cases Of Python List append() Method
- How append() Method Affects List Performance
- Avoiding Common Mistakes When Using Python List append()
- Comparing extend() With append() Python List Method
- Conclusion
- Frequently Asked Questions
- 🧠 Think You Know Python List append()? Take A Quiz!
Table of content:
- What Is A Linked List In Python?
- Types Of Linked Lists In Python
- How To Create A Linked List In Python
- How To Traverse A Linked List In Python & Retrieve Elements
- Inserting Elements In A Linked List In Python
- Deleting Elements From A Linked List In Python
- Update A Node Of Linked List In Python
- Reversing A Linked List In Python
- Calculating Length Of A Linked List In Python
- Comparing Arrays And Linked Lists In Python
- Advantages & Disadvantages Of Linked List In Python
- When To Use Linked Lists Over Other Data Structures
- Practical Applications Of Linked Lists In Python
- Conclusion
- Frequently Asked Questions
- 🔗 Linked List Logic: Can You Ace This Quiz?
Table of content:
- What Is Extend In Python?
- Extend In Python With List
- Extend In Python With String
- Extend In Python With Tuple
- Extend In Python With Set
- Extend In Python With Dictionary
- Other Methods To Extend A List In Python
- Difference Between append() and extend() In Python
- Conclusion
- Frequently Asked Questions
- Think You Know extend() In Python? Prove It!
Table of content:
- What Is Recursion In Python?
- Key Components Of Recursive Functions In Python
- Implementing Recursion In Python
- Recursion Vs. Iteration In Python
- Tail Recursion In Python
- Infinite Recursion In Python
- Advantages Of Recursion In Python
- Disadvantages Of Recursion In Python
- Best Practices For Using Recursion In Python
- Conclusion
- Frequently Asked Questions
- Recursive Thinking In Python: Test Your Skills!
Table of content:
- What Is Type Conversion In Python?
- Types Of Type Conversion In Python
- Implicit Type Conversion In Python
- Explicit Type Conversion In Python
- Functions Used For Explicit Data Type Conversion In Python
- Important Type Conversion Tips In Python
- Benefits Of Type Conversion In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Type Conversion? Take A Quiz!
Table of content:
- What Is Scope In Python?
- Local Scope In Python
- Global Scope In Python
- Nonlocal (Enclosing) Scope In Python
- Built-In Scope In Python
- The LEGB Rule For Python Scope
- Python Scope And Variable Lifetime
- Best Practices For Managing Python Scope
- Conclusion
- Frequently Asked Questions
- Think You Know Python Scope? Test Yourself!
Table of content:
- Understanding The Continue Statement In Python
- How Does Continue Statement Work In Python?
- Python Continue Statement With For Loops
- Python Continue Statement With While Loops
- Python Continue Statement With Nested Loops
- Python Continue With If-Else Statement
- Difference Between Pass and Continue Statement In Python
- Practical Applications Of Continue Statement In Python
- Conclusion
- Frequently Asked Questions
- Python 'continue' Statement Quiz: Can You Ace It?
Table of content:
- What Are Control Statements In Python?
- Types Of Control Statements In Python
- Conditional Control Statements In Python
- Loop Control Statements In Python
- Control Flow Altering Statements In Python
- Exception Handling Control Statements In Python
- Conclusion
- Frequently Asked Questions
- Mastering Control Statements In Python – Take the Quiz!
Table of content:
- Difference Between Mutable And Immutable Data Types in Python
- What Is Mutable Data Type In Python?
- Types Of Mutable Data Types In Python
- What Are Immutable Data Types In Python?
- Types Of Immutable Data Types In Python
- Key Similarities Between Mutable And Immutable Data Types In Python
- When To Use Mutable Vs Immutable In Python?
- Conclusion
- Frequently Asked Questions
- Quiz Time: Mutable vs. Immutable In Python!
Table of content:
- What Is A List?
- What Is A Tuple?
- Difference Between List And Tuple In Python (Comparison Table)
- Syntax Difference Between List And Tuple In Python
- Mutability Difference Between List And Tuple In Python
- Other Difference Between List And Tuple In Python
- List Vs. Tuple In Python | Methods
- When To Use Tuples Over Lists?
- Key Similarities Between Tuples And Lists In Python
- Conclusion
- Frequently Asked Questions
- 🧐 Lists vs. Tuples Quiz: Test Your Python Knowledge!
Table of content:
- Introduction to Python
- Downloading & Installing Python, IDLE, Tkinter, NumPy & PyGame
- Creating A New Python Project
- How To Write Python Hello World Program In Python?
- Way To Write The Hello, World! Program In Python
- The Hello, World! Program In Python Using Class
- The Hello, World! Program In Python Using Function
- Print Hello World 5 Times Using A For Loop
- Conclusion
- Frequently Asked Questions
- 👋 Python's 'Hello, World!'—How Well Do You Know It?
Table of content:
- Algorithm Of Python Program To Add To Numbers
- Standard Program To Add Two Numbers In Python
- Python Program To Add Two Numbers With User-defined Input
- The add() Method In Python Program To Add Two Numbers
- Python Program To Add Two Numbers Using Lambda
- Python Program To Add Two Numbers Using Function
- Python Program To Add Two Numbers Using Recursion
- Python Program To Add Two Numbers Using Class
- How To Add Multiple Numbers In Python?
- Add Multiple Numbers In Python With User Input
- Time Complexities Of Python Programs To Add Two Numbers
- Conclusion
- Frequently Asked Questions
- 💡 Quiz Time: Python Addition Basics!
Table of content:
- Swapping in Python
- Swapping Two Variables Using A Temporary Variable
- Swapping Two Variables Using The Comma Operator In Python
- Swapping Two Variables Using The Arithmetic Operators (+,-)
- Swapping Two Variables Using The Arithmetic Operators (*,/)
- Swapping Two Variables Using The XOR(^) Operator
- Swapping Two Variables Using Bitwise Addition and Subtraction
- Swap Variables In A List
- Conclusion
- Frequently Asked Questions (FAQs)
- Quiz To Test Your Variable Swapping Knowledge
Table of content:
- What Is A Quadratic Equation? How To Solve It?
- How To Write A Python Program To Solve Quadratic Equations?
- Python Program To Solve Quadratic Equations Directly Using The Formula
- Python Program To Solve Quadratic Equations Using The Complex Math Module
- Python Program To Solve Quadratic Equations Using Functions
- Python Program To Solve Quadratic Equations & Find Number Of Solutions
- Python Program To Plot Quadratic Functions
- Conclusion
- Frequently Asked Questions
- Quadratic Equations In Python Quiz: Test Your Knowledge!
Table of content:
- What Is Decimal Number System?
- What Is Binary Number System?
- What Is Octal Number System?
- What Is Hexadecimal Number System?
- Python Program to Convert Decimal to Binary, Octal, And Hexadecimal Using Built-In Function
- Python Program To Convert Decimal To Binary Using Recursion
- Python Program To Convert Decimal To Octal Using Recursion
- Python Program To Convert Decimal To Hexadecimal Using Recursion
- Python Program To Convert Decimal To Binary Using While Loop
- Python Program To Convert Decimal To Octal Using While Loop
- Python Program To Convert Decimal To Hexadecimal Using While Loop
- Convert Decimal To Binary, Octal, And Hexadecimal Using String Formatting
- Python Program To Convert Binary, Octal, And Hexadecimal String To A Number
- Complexity Comparison Of Python Programs To Convert Decimal To Binary, Octal, And Hexadecimal
- Conclusion
- Frequently Asked Questions
- 💡 Decimal To Binary, Octal & Hex: Quiz Time!
Table of content:
- What Is A Square Root?
- Python Program To Find The Square Root Of A Number
- The pow() Function In Python Program To Find The Square Root Of Given Number
- Python Program To Find Square Root Using The sqrt() Function
- The cmath Module & Python Program To Find The Square Root Of A Number
- Python Program To Find Square Root Using The Exponent Operator (**)
- Python Program To Find Square Root With A User-Defined Function
- Python Program To Find Square Root Using A Class
- Python Program To Find Square Root Using Binary Search
- Python Program To Find Square Root Using NumPy Module
- Conclusion
- Frequently Asked Questions
- 🤓 Think You Know Square Roots In Python? Take A Quiz!
Table of content:
- Understanding the Logic Behind the Conversion of Kilometers to Miles
- Steps To Write Python Program To Convert Kilometers To Miles
- Python Program To Convert Kilometer To Miles Without Function
- Python Program To Convert Kilometer To Miles Using Function
- Python Program to Convert Kilometer To Miles Using Class
- Tips For Writing Python Program To Convert Kilometer To Miles
- Conclusion
- Frequently Asked Questions
- 🧐 Mastered Kilometer To Mile Conversion? Prove It!
Table of content:
- Why Build A Calculator Program In Python?
- Prerequisites To Writing A Calculator Program In Python
- Approach For Writing A Calculator Program In Python
- Simple Calculator Program In Python
- Calculator Program In Python Using Functions
- Creating GUI Calculator Program In Python Using Tkinter
- Conclusion
- Frequently Asked Questions
- 🧮 Calculator Program In Python Quiz!
Table of content:
- The Calendar Module In Python
- Prerequisites For Writing A Calendar Program In Python
- How To Write And Print A Calendar Program In Python
- Calendar Program In Python To Display A Month
- Calendar Program In Python To Display A Year
- Conclusion
- Frequently Asked Questions
- Calendar Program In Python – Quiz Time!
Table of content:
- What Is The Fibonacci Series?
- Pseudocode Code For Fibonacci Series Program In Python
- Generating Fibonacci Series In Python Using Naive Approach (While Loop)
- Fibonacci Series Program In Python Using The Direct Formula
- How To Generate Fibonacci Series In Python Using Recursion?
- Generating Fibonacci Series In Python With Dynamic Programming
- Fibonacci Series Program In Python Using For Loop
- Generating Fibonacci Series In Python Using If-Else Statement
- Generating Fibonacci Series In Python Using Arrays
- Generating Fibonacci Series In Python Using Cache
- Generating Fibonacci Series In Python Using Backtracking
- Fibonacci Series In Python Using Power Of Matix
- Complexity Analysis For Fibonacci Series Programs In Python
- Applications Of Fibonacci Series In Python & Programming
- Conclusion
- Frequently Asked Questions
- 🤔 Think You Know Fibonacci Series? Take A Quiz!
Table of content:
- Different Ways To Write Random Number Generator Python Programs
- Random Module To Write Random Number Generator Python Programs
- The Numpy Module To Write Random Number Generator Python Programs
- The Secrets Module To Write Random Number Generator Python Programs
- Understanding Randomness and Pseudo-Randomness In Python
- Common Issues and Solutions in Random Number Generation
- Applications of Random Number Generator Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python's Random Module? Prove It!
Table of content:
- What Is A Factorial?
- Algorithm Of Program To Find Factorial Of A Number In Python
- Pseudocode For Factorial Program in Python
- Factorial Program In Python Using For Loop
- Factorial Program In Python Using Recursion
- Factorial Program In Python Using While Loop
- Factorial Program In Python Using If-Else Statement
- The math Module | Factorial Program In Python Using Built-In Factorial() Function
- Python Program to Find Factorial of a Number Using Ternary Operator(One Line Solution)
- Python Program For Factorial Using Prime Factorization Method
- NumPy Module | Factorial Program In Python Using numpy.prod() Function
- Complexity Analysis Of Factorial Programs In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Factorials In Python? Take A Quiz!
Table of content:
- What Is Palindrome In Python?
- Check Palindrome In Python Using While Loop (Iterative Approach)
- Check Palindrome In Python Using For Loop And Character Matching
- Check Palindrome In Python Using The Reverse And Compare Method (Python Slicing)
- Check Palindrome In Python Using The In-built reversed() And join() Methods
- Check Palindrome In Python Using Recursion Method
- Check Palindrome In Python Using Flag
- Check Palindrome In Python Using One Extra Variable
- Check Palindrome In Python By Building Reverse, One Character At A Time
- Complexity Analysis For Palindrome Programs In Python
- Real-World Applications Of Palindrome In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Palindromes? Take A Quiz!
Table of content:
- Best Python Books For Beginners
- Best Python Books For Intermediate Level
- Best Python Books For Experts
- Best Python Books To Learn Algorithms
- Audiobooks of Python
- Best Books To Learn Python And Code Like A Pro
- To Learn Python Libraries
- Books To Provide Extra Edge In Python
- Python Project Ideas - Reference
- Quiz To Rehash Your Knowledge Of Python Books!
Factorial Program In Python | 8 Ways & Complexity Analysis (+Codes)
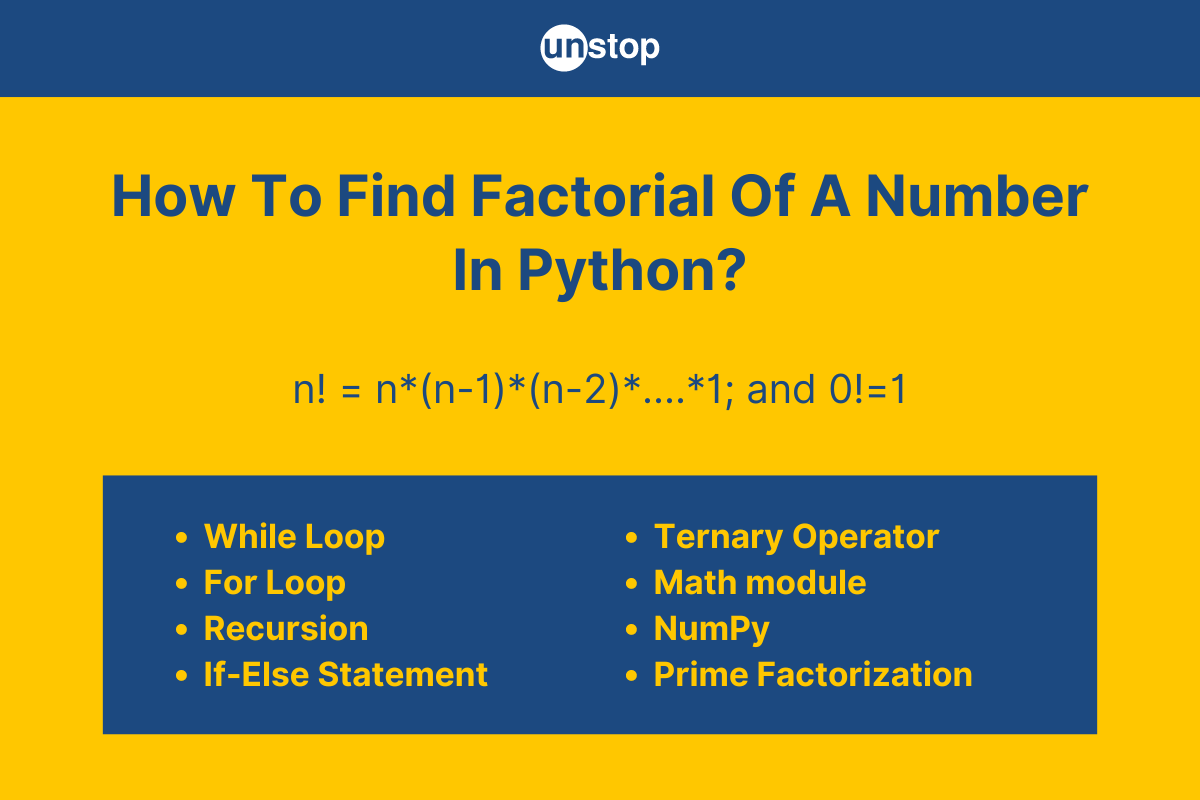
Factorial is a mathematical operation representing the product of all positive integers up to a given number. It is denoted by an exclamation mark (!). For example, the factorial of 5 (denoted as 5!) is calculated as 5 × 4 × 3 × 2 × 1 = 120. Factorials are widely used in permutations, combinations, and other mathematical computations.
There are many techniques you can use to write a factorial program in Python language. These include the iterative approach, recursion, and other optimized approaches using libraries like math and NumPy. In this article, we will explore various methods to calculate factorials in Python, starting with the basics.
What Is A Factorial?
The process of calculating the factorial of a number involves multiplying a sequence of descending positive integers from the respective number. That is, the factorial of a non-negative integer n is the product of all positive integers less than or equal to n.
n!=n×(n−1)×(n−2)×…×1
For n=0, by convention:
0!=1
Why 0!=1? The definition of 0!=1 is a convention that simplifies various mathematical expressions, particularly in combinatorics and series expansions. This definition ensures that formulas and equations work correctly even when n=0.
Algorithm Of Program To Find Factorial Of A Number In Python
The algorithm to find the factorial of a number can be implemented in various ways, but a common and straightforward method is to use an iterative approach. Below are the steps (as also illustrated in the flowchart) to find the factorial of a number in Python:
- Initialize the Result: Start by initialising a variable, say the result with the value 1. This will store the final factorial value.
- Iterate Through Numbers: Use a loop to iterate through all numbers from 1 to the given number n.
- Multiply and Update Result: In each iteration, multiply the current value of the result by the current number in the loop.
- Return the Result: After the loop completes, the variable result will hold the factorial of the number n.
Pseudocode For Factorial Program in Python
The pseudocode provides a high-level description of the algorithm without the syntax of a specific programming language. Below is the pseudocode for finding the factorial of a number using an iterative approach:
function factorial(n):
# Step 1: We begin by initializing the variable result with value 1.
#It will be the accumulator of products
result = 1
# Step 2: We create a for loop to iterate over the range 1 to n (inclusive)
for i from 1 to n:
# Step 3: Multiply the result by the current number and update its value
result = result * i
# Step 4: When loop completes iteration, the result variable contains the factorial, which it returns
return result
Factorial Program In Python Using For Loop
To find the factorial of a number using a for loop in Python, we start by initializing a result variable to 1. This variable will store the cumulative product of numbers from 1 to the given number n. We then use a for loop to iterate through each integer from 1 to n, multiplying the current value of the result by the loop counter in each iteration.
This iterative multiplication effectively computes the factorial by successively updating the result. Once the loop completes, the result variable contains the factorial of n. The Python program example below illustrates this approach to calculate and print the factorial.
Code Example:
def factorial_iterative(n):
result = 1
for i in range(1, n + 1):
result *= i
return result
# Example usage
number = 6
print(f"The factorial of {number} is {factorial_iterative(number)}")
Output:
The factorial of 6 is 720
Explanation:
In the Python code example-
- We define a function named factorial_iterative, which calculates the factorial of a given number n iteratively.
- We first initialize a variable result inside the function with the value 1. This variable will hold the final factorial value.
- Then, we use a for loop to iterate over the range from 1 to n inclusive. In each iteration, we multiply the result variable by the current value of i (the loop variable).
- After the loop completes iterations, the variable result contains the factorial of n, which the function returns.
- In the main part, we initialize a variable number with the value 6.
- Next, we call the factorial_iterative() function inside the print() function, passing the variable number as an argument.
- This function call and print() function displays the factorial of number with a descriptive message.
Time Complexity: O(n)
Space Complexity: O(1)
Factorial Program In Python Using Recursion
Recursion is a programming technique where a function calls itself to solve smaller instances of the same problem. To find the factorial of a number using recursion, we define a function that calls itself with a smaller argument until it reaches the base case, which is when the argument becomes 0.
In the recursive approach to find the factorial of a number, we define:
- Base Case: The factorial of 0 is defined as 1.
- Recursive Case: For any other number n, the factorial is calculated as n×(n−1)
Syntax for Recursive Function in Python:
def factorial_recursive(n):
# Base case
if n == 0:
return 1
# Recursive step
else:
return n * factorial_recursive(n - 1)
Here,
- The def keyword marks the beginning of the function with the name factorial_recursive and takes a parameter n.
- The if-else statement condition n==0 uses the relational operator to check if n is equal to 0. This is the base case.
- The return statements stipulate what the function will return given if the condition is met.
- The expression n*factorial_recursive(n-1) is the recursive step, which returns n multiplied by the result of calling itself with n - 1, reducing the problem size with each call until reaching the base case.
Let's look at a simple Python program example to understand how to use recursion for factorial calculations.
Code Example:
# Function to find factorial recursively
def factorial_recursive(n):
# Base case
if n == 0:
return 1
# Recursive step
else:
return n * factorial_recursive(n - 1)
# Example usage
number = 5
print(f"The factorial of {number} is {factorial_recursive(number)}")
Output:
The factorial of 5 is 120
Explanation:
In the simple Python code example-
- We define a function named factorial_recursive that takes n as a parameter and calculates its factorial recursively.
- In the function, we have an if-else statement that defines the base case and the recursive step.
- The if condition (base case) checks if n equals 0, i.e., n==0. If true, the function returns 1. This is because the factorial of 0 is defined as 1.
- If the condition is false, we proceed to the recursive step where the function returns the product of n and the factorial of n-1, achieved by calling factorial_recursive(n - 1).
- The function keeps calling itself with decreasing values of n until it reaches the base case.
- Once the base case is reached, the function returns values back up the call stack, each time multiplying n with the result of the factorial of n-1.
- In the main segment of the code, we initialize a variable n with the value 5 and then call the factorial_recursive function.
- The function calculates the factorial of the number 5, which we then print using the print() function.
Time Complexity: O(n)
Space Complexity: O(n)
Factorial Program In Python Using While Loop
To find the factorial of a number using a while loop in Python, we initialize a result variable to 1 to store the cumulative product of the numbers. We also initialize a counter variable to 1, which will be used to iterate through the numbers from 1 to the given number n.
Using a while loop, we repeatedly multiply the result by the counter variable and then increment the counter until it exceeds n. The example Python program below showcases this approach.
Code Example:
def factorial_while(n):
result = 1
i = 1
while i <= n:
result *= i
i += 1
return result
# Example usage
number = 5
print(f"The factorial of {number} is {factorial_while(number)}")
Output:
The factorial of 5 is 120
Explanation:
In the example Python code-
- We define a function named factorial_while that takes a parameter n and calculates its factorial using a while loop.
- Inside the function, we initialize variables result and i to value 1. The former will store the final factorial value and the latter will serve as a counter for the loop.
- Then, we use a while loop that continues as long as i is less than or equal to n.
- In every iteration, we multiply the result variable by the current value of i, and then increment i by 1 to move to the next number.
- When the loop completes its iterations, the result variable will contain the factorial of n, which the function returns.
- In the main part, we initialize a variable number with the value 5 and call the factorial_while() function, passing the number as an argument.
- This function calculates the factorial, which we print using the print() function and f-strings.
Time Complexity: O(n)
Space Complexity: O(1)
Factorial Program In Python Using If-Else Statement
The if-else statement helps execute one of the two code blocks depending upon whether the if-condition is met. Using an if-else statement to find the factorial of a number typically involves a recursive approach.
In this method, the function calls itself with a decremented value of the original number until it reaches the base case. The base case is when the input number is zero, and the factorial of zero is defined as one. Each recursive call multiplies the current number by the result of the factorial of the previous number. The Python program sample below illustrates this approach for a better understanding.
Code Example:
def factorial_recursive(n):
if n == 0:
return 1
else:
return n * factorial_recursive(n - 1)
# Example usage
number = 4
print(f"The factorial of {number} is {factorial_recursive(number)}")
Output:
The factorial of 4 is 24
Explanation:
In the Python code sample-
- We begin by defining a function named factorial_recursive , that takes a number n as parameter and calculates its factorial recursively.
- Inside the function, we use an if-else statement to check the base case. In case the if-condition n==0 is true, the function returns 1 because the factorial of 0 is defined as 1.
- If the condition is false, we proceed to the recursive step. Here, we return the product of n and the factorial of n-1, achieved by calling factorial_recursive(n - 1).
- The function keeps calling itself with decreasing values of n until it reaches the base case where n is 0.
- Once the base case is reached, the function returns values back up the call stack, each time multiplying n with the result of the factorial of n-1.
- For the example usage in the main part, we initialize a variable number with the value 4.
- Then, we call the factorial_recursive() function that calculates the factorial of the number 4.
- We print this result with a descriptive string message using the print() function.
Time Complexity: O(n)
Space Complexity: O(n)
The math Module | Factorial Program In Python Using Built-In Factorial() Function
The Math library in Python contains a built-in function for computing factorials called the factorial() function. Naturally, in this method we must import the math module and use the function math.factorial().
This is one of the most straightforward and efficient ways to calculate the factorial of a number, as it abstracts away the implementation details and is highly optimized. The sample Python program presents how this approach works.
Code Example:
import math
def factorial_builtin(n):
return math.factorial(n)
# Example usage
number = 5
print(f"The factorial of {number} is {factorial_builtin(number)}")
Output:
The factorial of 5 is 120
Explanation:
We begin the sample Python code by importing the math module, which provides access to mathematical functions. Then-
- We define a function named factorial_builtin that takes a number n as a parameter and calculates its factorial using the built-in math.factorial function.
- Inside the function, we simply return the result of calling the math.factorial() function passing the parameter n as an argument.
- The math.factorial function handles the calculation of the factorial internally.
- To showcase, in the main part, we initialize a variable number with value 5 and then call the factorial_builtin() function.
- We then print the factorial calculated to the output console using the print() function.
Time Complexity: O(n)
Space Complexity: O(1)
Python Program to Find Factorial of a Number Using Ternary Operator(One Line Solution)
In Python, a ternary operator provides a way to condense an if-else statement into a single line. This technique can be utilized to create a concise recursive function for calculating the factorial of a number. The ternary operator will handle the base case and the recursive step within one line of code. The syntax for this operator is given below, followed by a basic Python program example.
Syntax:
def factorial_ternary(n):
return 1 if n == 0 else n * factorial_ternary(n - 1)
Here,
- def factorial_ternary(n):: Defines a function named factorial_ternary with an input parameter n.
- return 1 if n == 0 else n * factorial_ternary(n - 1): Uses the ternary operator to return 1 if n is equal to 0; otherwise, returns n multiplied by the result of factorial_ternary(n - 1).
Code Example:
def factorial_ternary(n):
return 1 if n == 0 else n * factorial_ternary(n - 1)
# Example usage
number = 3
print(f"The factorial of {number} is {factorial_ternary(number)}")
Output:
The factorial of 3 is 6
Explanation:
In the basic Python code example-
- We define a function named factorial_ternary, which calculates the factorial of the parameter number n using a ternary operator and recursion.
- In the function, we use the ternary operator to check the base condition n==0. If this is true, then the ternary operator returns 1.
- If the condition is false, it returns n multiplied by the factorial of n-1, which is achieved by calling factorial_ternary(n - 1).
- This recursive approach continues until it reaches the base case where n is 0.
- Once the base case is reached, the function starts returning values back up the call stack, each time multiplying n with the result of the factorial of n-1.
- In the main part, we initialize a variable number with the value 3 and then call the factorial_ternary() function.
- The factorial is printed to the console using f-strings inside a print() function.
Time Complexity: O(n)
Space Complexity: O(n)
Python Program For Factorial Using Prime Factorization Method
The prime factorization method for finding the factorial of a number involves breaking down the number into its prime factors and then counting the occurrences of each prime factor. Since factorial involves multiplying consecutive numbers, the prime factors of the factorial can be derived from the prime numbers less than or equal to the given number.
We can write a factorial program in Python by counting the occurrences of each prime factor in the range [2, n].
Code Example:
def factorial_prime_factorization(n):
# Function to compute the prime factors of a number
def prime_factors(num):
factors = {}
divisor = 2
while divisor * divisor <= num:
if num % divisor == 0:
factors[divisor] = factors.get(divisor, 0) + 1
num //= divisor
else:
divisor += 1
if num > 1:
factors[num] = factors.get(num, 0) + 1
return factors
# Compute prime factors for each number in the range [2, n]
prime_factors_count = {}
for i in range(2, n + 1):
factors = prime_factors(i)
for factor, count in factors.items():
prime_factors_count[factor] = prime_factors_count.get(factor, 0) + count
# Calculate the factorial using prime factors
factorial = 1
for factor, count in prime_factors_count.items():
factorial *= factor ** count
return factorial
# Example usage
number = 5
print(f"The factorial of {number} is {factorial_prime_factorization(number)}")
Output:
The factorial of 5 is 120
Explanation:
In this sample factorial program in Python-
- We begin by defining a function named factorial_prime_factorization, which calculates the factorial of a given number n using prime factorization.
- Inside this function, there's another nested function named prime_factors that computes the prime factors of a given number.
- Inside, we initialize an empty dictionary named factors to store the prime factors and their respective counts.
- We then set a divisor to 2 and use a while loop that iterates until the square of the divisor is less than or equal to the input number.
- During each iteration, an if-else statement checks if the number is divisible by the divisor. If it is, we update the factors dictionary accordingly and reduce the number by dividing it by the divisor.
- If the number is not divisible by the current divisor, we increment the divisor by 1.
- After the loop, we have another if-statement, which checks if the remaining number is greater than 1. If it is, we update the factors dictionary accordingly.
- The prime_factors function returns the dictionary containing the prime factors and their counts.
- Moving back to the outer function, we create another empty dictionary prime_factors_count to store the prime factors and their respective counts for all numbers from 2 to n.
- Then, we use a set of nested for loops to iterate through the range from 2 to n, where the outer loop computes the prime factors for each number using the prime_factors() function and the inner loop updates prime_factors_count accordingly.
- Next, we initialize a variable factorial with the value 1 and then use a for loop to calculate the factorial using the prime factors stored in prime_factors_count.
- The loop iterates through the items of prime_factors_count, multiplying the factorial by each prime factor raised to its respective count (using the exponent operator).
- Finally, the function returns the calculated factorial stored inside the variable factorial.
- Lastly, we initialize a variable number with the value 5 and calculate and print its factorial using the factorial_prime_factorization() and print() functions.
Time Complexity: O(n sqrt{n})
Space Complexity: O(n log n)
NumPy Module | Factorial Program In Python Using numpy.prod() Function
The NumPy library in Python offers multiple efficient numerical operations on arrays, etc. We can leverage its capabilities to compute the factorial of a number using the numpy.prod() function. This method involves creating an array containing the integers from 1 to the given number and then using the numpy.prod() function to calculate their product.
The numpy.prod() function efficiently computes the product of array elements along a specified axis, providing a succinct and optimized solution for calculating factorials. Look at the simple Python example below, which illustrates this approach.
Code Example:
import numpy as np
def factorial_numpy(n):
# Create an array containing integers from 1 to n
numbers = np.arange(1, n + 1)
# Calculate the factorial using numpy.prod()
factorial = np.prod(numbers)
return factorial
# Example usage
number = 5
print(f"The factorial of {number} is {factorial_numpy(number)}")
Output:
The factorial of 5 is 120
Explanation:
In the Python example code, we first import the NumPy library as np, which provides support for mathematical operations on arrays and matrices.
- We then define a function named factorial_numpy(), which calculates the factorial of a given number n using NumPy.
- Inside the function, we create an array named numbers containing integers from 1 to n using the arrange() function, i.e., np.arange(1, n + 1).
- Next, we use the np.prod() function from NumPy to calculate the product of all elements in the numbers array, which effectively computes the factorial.
- The result is stored in a variable named factorial, which the function then returns.
- Lastly, we initialize a variable called number with the value 5 and calculate its factorial using the factorial_numpy() function.
Time Complexity: O(n)
Space Complexity: O(n)
Complexity Analysis Of Factorial Programs In Python
Here is a detailed complexity analysis of the different methods used to write a factorial program in Python programming language:
Method | Time Complexity | Space Complexity |
---|---|---|
math.factorial() | O(n) | O(1) |
While Loop | O(n) | O(1) |
For Loop | O(n) | O(1) |
Recursive Approach | O(n) | O(n) |
numpy.prod() | O(n) | O(n) |
Ternary Operator(One Line Solution) |
O(n) | O(n) |
If-else Statement | O(n) | O(n) |
Prime Factorization | O(n sqrt{n}) | O(n log n) |
Conclusion
Factorial of a number is the product of all preceding integers and the respective number itself. There are many ways to write a factorial program in Python, including iterative loops, recursive functions, and built-in functions like math.factorial() and numpy.prod(). Python provides flexibility to suit different needs. Factors such as input size, performance requirements, and code readability guide the selection of the most appropriate method. Despite the diversity of approaches, the factorial operation remains a foundational tool in mathematics and programming, supporting various algorithms and computations in Python applications.
Frequently Asked Questions
Q. What is a factorial, and why is it used?
A factorial of a non-negative integer n is the product of all positive integers less than or equal to n. It is denoted by n!. Factorials are commonly used in mathematics and computer science for various purposes, such as combinatorial problems, probability calculations, and algorithmic solutions.
Q. What are the limitations of using recursion to calculate factorials?
Recursive methods for calculating factorials can be elegant and concise, but they may have limitations, particularly for large inputs. Recursive functions rely on the call stack, and deep recursion may lead to stack overflow errors, especially with large input values. As a result, iterative or built-in function-based approaches may be preferred when writing a factorial program in Python language in terms of efficiency and scalability.
Q. In what scenarios should I use built-in functions like math.factorial() or libraries like NumPy for factorial calculations?
Inbuilt functions like math.factorial() and libraries like NumPy offer optimized implementations for factorial calculations, suitable for general-purpose numerical computing tasks. They are particularly useful when working with large numbers or performing computations on arrays or matrices containing factorial-related operations. These functions provide efficient, reliable, and well-tested solutions, making them suitable for a wide range of applications.
Q. How can I handle large factorial results in Python?
Python's integers are of arbitrary precision, meaning they can grow as large as the memory allows. However, when working with extremely large numbers, performance and memory usage can become concerns. You can use libraries like math module or NumPy, which are optimized for large-number computations. Additionally, you can consider using the gmpy2 library for specialised applications, which provides enhanced performance for large integer arithmetic.
Q. Are there any optimizations for computing factorials efficiently?
Yes, there are optimizations that can improve the efficiency of calculations in a factorial program in Python. For example, memoization in recursive functions can reduce redundant computations by caching previously calculated results. Additionally, leveraging mathematical properties of factorials, such as prime factorization, can lead to more efficient algorithms for computing factorials, especially for large inputs like when writing a factorial program in Python.
Think You Know Factorials In Python? Take A Quiz!
You might also be interested in reading the following:
- Python Logical Operators, Short-Circuiting & More (With Examples)
- Convert Int To String In Python | Learn 6 Methods With Examples
- Python Bitwise Operators | Positive & Negative Numbers (+Examples)
- Python String.Replace() And 8 Other Ways Explained (+Examples)
- How To Reverse A String In Python? 10 Easy Ways With Examples
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Ujjwal Sharma 2 weeks ago
Vishwajeet Singh 1 month ago
niyati m singh 1 month ago
Hari Charan Bonam 1 month ago
Shradha Manure 1 month ago
Pardha Venkata Sai Patnam 1 month ago