Python Reverse List | 10 Ways & Complexity Analysis (+Examples)
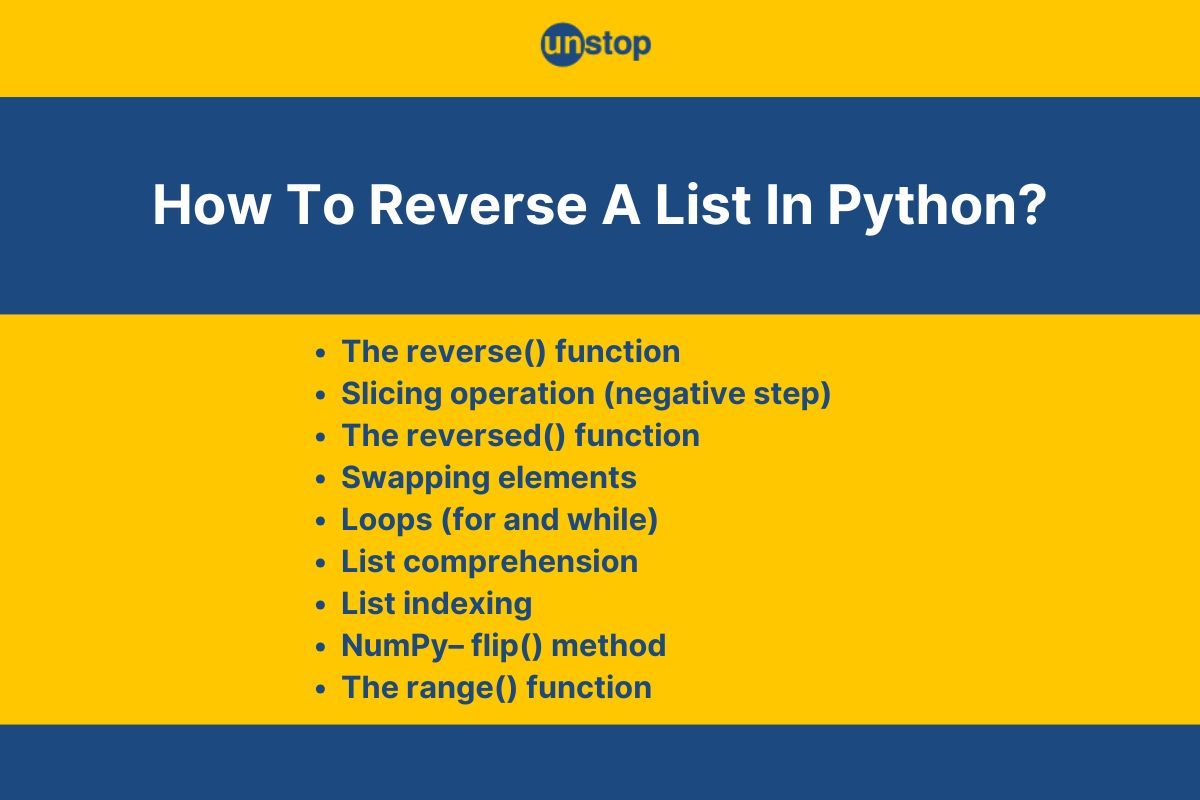
In Python, a list is one of the most versatile and commonly used data structures. You can think of a list as a container that holds an ordered collection of items, much like a shopping list, where the items are listed in a specific order. Lists in Python can hold different types of data—strings, numbers, and even other lists.
One operation that’s particularly useful when working with lists is reversing them. Python reverse list is simply the act of rearranging the elements in reverse order. This operation is essential in many algorithms and can help in situations where the order of elements needs to be flipped for processing. In this article, we will discuss the various ways to reverse a list in Python with the help of code examples.
List of Methods To Reverse A Python List
There are several ways to reverse a list in Python programming, each with its own use case and performance benefits. Let’s take a look at the different methods we’ll be covering:
- The reverse() method: A built-in function that modifies the list in place, changing its order directly. It’s one of the most straightforward methods to reverse a list.
- Slice Operator/Slicing ([::-1]): This method allows us to slice a Python list in a specific way. By using the slicing technique [::-1], we can split the list and then combine it in reverse order, giving us a reversed copy of the original list.
- Swapping Elements: A manual method where we reverse the list by swapping elements at both ends. This is a more hands-on approach and requires a bit of logic, but it helps understand how reversing works at the element level.
- The reversed() function: This built-in method returns an iterator that yields the elements of the list in reverse order. Unlike the Python function reverse(), reversed() does not modify the original list but creates an iterator, which can be used to iterate over the list in reverse.
- For Loop: A basic loop that runs for a fixed number of times. In this method, we implement a simple logic to reverse the list manually by appending elements in reverse order.
- While Loop: Similar to the for loop, but with a different structure. This loop continues until a condition is met, and we use it to reverse a list by manually iterating through it in reverse order.
- List Comprehension: A one-liner using Python’s powerful list comprehension feature. List comprehensions allow you to create a new list by iterating over an existing one, and in this case, we’ll use it to reverse the list in a single line. (We’ll explain this feature in detail in the next section!)
- List Indexing: Reversing the list by directly manipulating its indices. Here, we’ll use negative indexing to access the elements in reverse order.
- The range() function: This function can be used to generate a sequence of indices to access elements in reverse order. It’s a more advanced method, often used when you want to iterate over a list in reverse using a custom range.
- NumPy: If you're working with large datasets or numerical data, NumPy offers a way to reverse a list efficiently. NumPy arrays are designed to handle data more efficiently than standard Python lists, especially when dealing with large amounts of data.
In the following sections, we’ll discuss how to reverse a list in Python using each of these methods in detail, with the syntax and code examples.
To know more about lists, read: Python List | Everything You Need To Know (With Detailed Examples)
Python Reverse List Using reverse() Method
The reverse() method is one of the simplest ways to reverse a list in Python. It's a built-in method that modifies the original list in place, meaning it directly changes the order of the elements without creating a new list.
How it works:
- When you call the reverse() method on a list, it reverses the elements of that list.
- Unlike some other methods, it doesn’t return a new list.
- Instead, it updates the original list. If you print the list after using reverse(), you’ll see the reversed order of elements.
Syntax:
list.reverse()
Here, the list refers to the name of the list you want to reverse, and the dot operator is used to call the method. This method doesn’t take any arguments and returns None because it modifies the list in place.
Code Example:
Output:
[5, 4, 3, 2, 1]
Explanation:
In the basic Python program example,
- We first create a list called my_list containing 5 integer values.
- Then, we call the reverse() method on it, i.e., my_list.reverse(). It reverses the list, and the elements change in order to [5, 4, 3, 2, 1].
- Next, we use the print() function to display the reversed list to the console.
- Notice that the reverse() method doesn’t return a new list; it simply modifies the original list.
Why Use reverse() Method?
- In-place modification: The reverse() method doesn’t create a new list, which can save memory, especially when working with large datasets.
- Efficiency: Since it directly modifies the list without needing to create another one, it can be more efficient in terms of both time and space.
- Simplicity: It's one of the easiest methods to use when you want to reverse a list quickly and without any frills.
However, if you need to keep the original list intact and return a reversed version, you may want to use other methods, like slicing or the reversed() function, which don’t alter the original list.
Python Reverse List Using the Slice Operator ([::-1])
The slice operator in Python is a powerful tool, allowing you to access subparts of a list (or any iterable) in a variety of ways. One of the most useful applications of slicing is reversing a list. By using a specific slice notation [::-1], you can create a new list where the elements are arranged in reverse order.
How it works:
- The syntax [::-1] is a slice operation that means "take the whole list, but step backwards."
- The syntax for slice operator is– list[start:stop:step]. Here, start is the index where the slice begins, stop is where the slice ends (not inclusive) and step determines the stride between elements.
When we use [::-1], it means that we:
- Start from the beginning of the list (start is omitted, so it defaults to 0).
- Go all the way to the end of the list (stop is omitted).
- Use a step of -1, which means we iterate backwards through the list.
Syntax:
reversed_list = my_list[::-1]
Here, my_list refers to the original list you want to reverse, and reversed_list refers to the new list created with the elements of my_list in reverse order.
Code Example:
Output:
[5, 4, 3, 2, 1]
Explanation:
In the simple Python code example,
- We create a list called my_list containing [1, 2, 3, 4, 5]. This is the original list.
- Then, we use the slice operator with a negative step, i.e., my_list[::-1]. This creates a new list reversed_list where the elements are in reverse order: [5, 4, 3, 2, 1].
- Note that this method does not modify the original list. Instead, it returns a new list with the reversed order.
Why Use The Slice Operator?
- Concise and elegant: The slice operator is a compact, one-liner method to reverse a list.
- Non-destructive: Unlike reverse(), this method does not modify the original list. If you need to keep the original list intact, slicing is a great choice.
- Flexibility: You can use slicing in a variety of contexts beyond just reversing lists (like extracting sublists, skipping elements, etc.).
Performance Considerations
- While slicing is a convenient and fast method, it does create a new list. If you're working with large datasets, this might not be the most memory-efficient method compared to in-place reversal methods like reverse().
- It’s a great option when you don’t need to modify the original list and want a quick, one-liner solution.
Python Reverse List By Swapping Elements
Reversing a list by swapping elements is a manual approach, where you reverse the list by iterating through it and swapping the elements at both ends. It’s like physically flipping the list, one pair of items at a time, until the list is completely reversed.
How it works:
In this method, you loop through the list and swap the elements at the beginning and the end.
- You start from the first element (index 0) and the last element (index -1).
- Swap the two elements.
- Move inward, swapping the next pair of elements, and so on, until the center of the list is reached.
The process continues until you’ve swapped all the necessary elements. This method ensures that the list is reversed in place without creating a new list, so it's memory efficient.
Syntax:
There’s no specific syntax for this method because it involves manual swapping. However, here's the general structure of the logic:
for i in range(len(my_list) // 2):
my_list[i], my_list[len(my_list) - i - 1] = my_list[len(my_list) - i - 1], my_list[i]
Note that here, we use the range() and len() functions to get the length of the list and then iterate across that range.
Code Example:
Output:
[5, 4, 3, 2, 1]
Explanation:
In the simple Python program example,
- We initialize a list named my_list with values [1, 2, 3, 4, 5].
- Then, we use a for loop to iterate through the list, swapping the first element with the last, the second element with the second-to-last, and so on.
- The loop runs until we’ve swapped all the necessary pairs. In this case, the middle element (3) doesn’t need to be swapped because it's already in its correct position.
- Lastly, we print the reversed list to the console.
Why Use Swapping?
- In-place modification: This method does not require additional memory to create a new list. It modifies the original list directly.
- Efficient for large lists: Since the operation is done in place, it’s memory-efficient, especially when dealing with large datasets.
- Good for understanding: This approach helps you understand the underlying process of list reversal and gives you control over how the elements are manipulated.
Check out this amazing course and learn to code in the Python programming language with ease.
Python Reverse List Using The reversed() Function
The reversed() function is another built-in tool in Python that allows you to reverse a list. However, unlike the reverse() method, reversed() doesn’t modify the original list. Instead, it returns an iterator that yields the elements of the list in reverse order. This gives you more flexibility if you want to work with the reversed elements without changing the original list.
How it works:
- The reversed() function creates an iterator, meaning it doesn't generate a new list in memory.
- It just returns an object that will allow you to iterate over the list in reverse order.
- This can be useful when you need to reverse the list temporarily or iterate over the list backward without storing the entire reversed list in memory.
Syntax:
reversed_list = reversed(my_list)
Here, the my_list is the original list you want to reverse and reversed_list is an iterator that allows you to access the elements in reverse order. If you need the result as a list, you can convert the iterator to a list using list(reversed(my_list)).
Code Example:
Output:
[5, 4, 3, 2, 1]
Explanation:
In the Python program example,
- We create a list named my_list containing integer values: [1, 2, 3, 4, 5].
- Then, we call the reversed() function, passing the list as an argument. It returns an iterator that yields elements in reverse order.
- We then convert the iterator to a list using the list() function, i.e., list(reversed(my_list)), and store the outcome in a new list reversed_list.
- Finally, we print the new list [5, 4, 3, 2, 1] to the console.
- Note that here, the original list my_list remains unchanged, so this method doesn't modify it in place.
Why Use reversed()?
- Non-destructive: Like slicing, reversed() doesn't alter the original list. It returns an iterator, which allows for flexible manipulation of the reversed data.
- Efficient for iteration: Since reversed() returns an iterator instead of a new list, it's more memory-efficient for large datasets when you only need to iterate over the elements in reverse order without needing the entire reversed list at once.
Why Not To Use reversed()?
- Requires conversion for list: If you need to get a list (instead of just iterating over the reversed elements), you must convert the iterator to a list explicitly using list(reversed(my_list)). This extra step could be seen as a drawback compared to methods like reverse() or slicing that directly returns a list.
- Not in-place: If you need to reverse the list in place (without creating a new one), this method won’t work since it only creates an iterator and doesn’t modify the original list.
Python Reverse List Using A for Loop
A for loop offers a manual but straightforward way to reverse a list by iterating through it and appending its elements to a new list in reverse order. This method doesn't modify the original list and creates a new reversed list.
How it works:
- Start iterating over the original list from the last element to the first.
- Append each element to a new list. (We generally use the append() function for this)
- Once the iteration is complete, the new list contains all elements of the original list in reverse order.
There is no predefined structure/ syntax for this purpose besides the syntax of the for loop. The point to note is that when defining the range for iterations on the list, we can use either the slice/ index notation or the range function. Look at the basic Python code example below, which illustrates this approach for list reversal.
Code Example:
Output:
[5, 4, 3, 2, 1]
Explanation:
In the Python code example,
- We create the original list, my_list, containing [1, 2, 3, 4, 5].
- Then, we use the slice operation to create a reverse iterator for the loop, i.e., my_list[::-1].
- We use this iterator as the range for the for loop, which then iterates through the list in reverse order.
- In every iteration, the loop uses the append() method to append the respective element to a new list called reversed_list.
- After the iterations are done, the reversed_list contains the elements of the original one in reversed order, which we print to the console.
Why Not To Use For Loop Method?
- Extra space required: This method creates a new list to store the reversed elements, which increases memory usage compared to in-place methods like reverse() or swapping.
- Less elegant: Compared to built-in methods, a manual loop might feel verbose and less Pythonic.
- Slower for large datasets: Iterating and appending elements can be slower than using optimized built-in methods for reversing.
Python Reverse List Using While Loop
The while loop provides another manual way to reverse a list by iterating over it in reverse order. This approach is similar to using a for loop, but the while loop gives you more flexibility in controlling the iteration process.
How it works:
- Initialize a counter variable to the index of the last element in the list.
- Use a while loop to iterate from the end of the list to the beginning.
- Append each element to a new list during each iteration.
- Decrease the counter after processing each element until it reaches zero.
Code Example:
Output:
[5, 4, 3, 2, 1]
Explanation:
In the example Python program,
- We initialize the loop counter variable i to len(my_list) - 1, pointing to the last index of the list.
- Then, we define a while loop that runs as long as i is greater than or equal to 0.
- During each iteration, we use the index value, i.e., my_list[i] (the current element) and append it to the reversed_list.
- After processing an element, the counter i is decremented to move to the previous index.
- Once the loop ends, reversed_list contains the elements of my_list in reverse order.
Why Not To Use While Loop For Python Reverse List?
- Verbose implementation: The while loop requires manual control of the index and iteration logic, which makes it less concise compared to other methods.
- Extra memory usage: Similar to the for loop, this approach creates a new list, which increases memory consumption.
- Less Pythonic: Python provides built-in methods like reverse() and slicing that are more intuitive and readable for reversing lists.
Python Reverse List Using List Comprehension
List comprehension is one of Python’s most powerful and expressive features, allowing you to construct a new list from an existing one in a concise, single-line format. You can use list comprehension to reverse a list by iterating through it in reverse order.
How it works: The idea is to iterate through the original list using slicing ([::-1]) and construct a new list in reverse order using list comprehension.
Syntax:
reversed_list = [item for item in original_list[::-1]]
Code Example:
Output:
[50, 40, 30, 20, 10]
Explanation:
In the example Python code,
- We use the slicing operation (i.e., my_list[::-1]) to generate a reverse iterator of my_list.
- The list comprehension [item for item in my_list[::-1]] iterates through this reversed iterator, collecting each element into a new list, reversed_list.
- The resulting reversed_list contains the elements of my_list in reverse order.
Why Not To Use List Comprehension For Python List Reversal?
- Redundant for Simple Reversal: List comprehension is more verbose than directly using slicing ([::-1]) to reverse a list. Both approaches create a new list, but slicing is simpler and more intuitive.
- Less Efficient: Since list comprehension doesn't add any unique performance advantage for this specific task, it’s often better to use slicing or built-in methods.
List comprehension shines when you need to apply transformations or filters during the reversal process. For simple reversals, however, slicing is usually the better choice.
Level up your coding skills with the 100-Day Coding Sprint at Unstop and claim the bragging rights now!
Python Reverse List Using List Indexing
List indexing allows you to access elements directly using their indices. By leveraging this feature, we can create a new reversed list by iterating through the indices of the original list in reverse order.
How it works:
- Iterate through the indices of the original list in reverse order using Python’s range() function.
- Access elements at these indices and append them to a new list.
Code Example:
Output:
[35, 28, 21, 14, 7]
Explanation:
In the sample Python program,
- We first use the len() function to get the length of the list and the range() function to generate a sequence of indices starting from the last index (len(my_list) - 1) down to the first index (0), stepping backward (-1).
- Then, we use list indexing (i.e., my_list[i]) to access elements at these indices and add them to the new list.
- The result is a new list, reversed_list, with elements in reverse order.
Why Not To Use List Indexing For Python List Reversal?
- Verbose Implementation: Like loops, using indexing for reversal is more verbose compared to slicing ([::-1]) or built-in methods like reverse().
- Extra Memory Usage: This approach creates a new list, which can be less memory-efficient compared to in-place reversal.
- Less Pythonic: Python offers more concise and readable ways to achieve the same result without explicitly handling indices.
List indexing is an interesting method for understanding the concept of reversing elements based on their positions. However, for everyday coding tasks, slicing and built-in methods remain more practical.
Python Reverse List Using The range() Function
The range() function is a versatile tool for generating sequences of numbers. You can use it to iterate over indices in reverse order and construct a new reversed list.
How it works:
- Uses the range() function to generate indices in reverse order, starting from the last index and moving to the first.
- Accesses elements at these indices from the original list and appends them to a new list.
Code Example:
Output:
[9, 7, 5, 3, 1]
Explanation:
In the sample Python code,
- We create an original list, my_list, containing five integer values.
- Then, we use the range() function to generate the indices in reverse order, i.e., range(len(my_list) - 1, -1, -1):
- Starts at len(my_list) - 1 (last index).
- Stops at -1 (just before the first index).
- Moves in steps of -1 (backward).
- Next, we access each element at the generated index and add it to the new list using list comprehension and my_list[i].
- The result is reversed_list, containing the elements of my_list in reverse order, which we then print to the console.
Why Not To Use range() For Python List Reversal?
- Verbosity: While using range() offers flexibility, the syntax is less concise than alternatives like slicing ([::-1]).
- Extra Memory: Similar to other methods that create a new list, this approach isn’t memory-efficient for large datasets.
- Readability: The range() function’s syntax for reverse iteration can be confusing, especially for beginners.
The range() function is a handy tool for understanding reverse iteration, but for reversing lists specifically, slicing or built-in methods remain the go-to choices.
Python Reverse List Using NumPy
NumPy, a popular Python library for numerical computations, includes a flip() method explicitly designed for reversing the order of elements along a specified axis. This makes it an ideal and distinct choice for reversing lists (or arrays) in a NumPy-centric workflow.
How it works:
- Converts the Python list into a NumPy array using numpy.array().
- Applies the numpy.flip() method to reverse the array.
- Converts the reversed array back into a Python list using the tolist() method.
Code Example:
Output:
[9, 7, 5, 3, 1]
Explanation:
In the Python program sample,
- We create a list my_list and use the array() method from NumPy to convert the Python list into a NumPy array (i.e., np.array(my_list)), enabling access to NumPy-specific operations.
- Then, we use the flip() method from NumPy to reverse the elements of the array, i.e., np.flip(numpy_array).
- Next, we use the tolist() method to convert the NumPy array back to a Python list, i.e., reversed_array.tolist().
- Finally, we print the new reversed_list list on the console.
Why Use NumPy’s flip() Method?
- Explicit Intent: The flip() method explicitly reverses the array, making the code more readable and intent clear.
- Handles Multidimensional Arrays: If working with multi-dimensional data, flip() allows reversing along specific axes.
Why Not To Use NumPy’s flip()?
- Unnecessary for Small Lists: For simple list reversal, importing and using NumPy can be overkill.
- Dependency Overhead: Projects not already using NumPy may not want to add this dependency just to reverse lists.
Comparison Of Ways To Reverse A Python List
When it comes to reversing a list in Python, different methods provide various performance trade-offs. To help you choose the best approach, let's compare the methods we discussed and analyze their complexities.
Method |
Time Complexity |
Space Complexity |
Notes |
reverse() Method |
O(n) |
O(1) |
In-place operation, meaning the list is modified directly. It's simple and efficient, especially for large lists. |
Slice Operator ([::-1]) |
O(n) |
O(n) |
Creates a new reversed list. While it’s concise, it uses extra space to store the reversed list, making it less space-efficient for large lists. |
Swapping Present & Last Numbers |
O(n) |
O(1) |
In-place method, but a little more manual than reverse(). Still highly efficient with minimal space usage. |
reversed() Built-in Function |
O(n) |
O(n) |
Creates an iterator, so you get a reversed view of the list. It does not directly modify the list, but it is useful for iteration. |
for Loop |
O(n) |
O(n) |
Involves creating a new list and manually reversing the elements. Good for understanding the process but not optimal for performance. |
while Loop |
O(n) |
O(n) |
Like the for loop method, which creates a new list. The main difference is how you loop through the original list. |
List Comprehension |
O(n) |
O(n) |
A compact way to reverse the list but create a new list, so it’s not the most space-efficient method. |
List Indexing |
O(n) |
O(n) |
Uses index-based swapping to reverse the list. Like for and while loops, it creates a new list and is less efficient than in-place methods. |
range() Function |
O(n) |
O(n) |
Works similarly to list comprehension but uses the range for iteration. It can be more flexible but not the most space-efficient. |
NumPy Method |
O(n) |
O(n) |
Leverages the power of NumPy arrays, which can be faster for large data but adds external dependencies and overhead. |
Let’s discuss what this table indicates in reference to the worst and best methods for Python list reversal.
Best Methods:
- reverse() Method: This is the most efficient in terms of time and space if you need to modify the list in place. It's the go-to choice for simple list reversal tasks.
- Swapping Present & Last Numbers: A simple and effective in-place solution for reversing a list. Although it requires manual handling, it’s quite efficient for space.
Less Efficient Methods:
- Slice Operator ([::-1]) and reversed() Function: While both of these methods are clean and Pythonic, they create a new list, resulting in higher space complexity. They are suitable for scenarios where you need a new reversed list but may not be the best choice for large data due to space constraints.
- Using for and while Loops: These methods are more verbose and also require extra space to store the new reversed list, making them less ideal for large datasets. They are, however, excellent for learning and understanding the concept of list reversal.
Worst Method:
Using NumPy: Although NumPy is a powerful library, it is typically overkill for basic tasks like list reversal. It introduces external dependencies and adds overhead for a relatively simple operation.
Looking for guidance? Find the perfect mentor from select experienced coding & software development experts here.
Conclusion
Reversing a list is a common task in programming, often needed when you want to manipulate data in reverse order, such as when processing items from last to first or when transforming sequences for specific algorithms.
There are multiple ways to get the Python reverse list, each suited for different needs. From the in-place reverse() method to the slicing technique ([::-1]) and the reversed() function, each approach balances simplicity, efficiency, and space requirements differently. Other methods are using loops, list comprehension, and even NumPy for large datasets.
The choice of method depends on the task at hand—whether you're optimizing for speed, memory, or simplicity. With these techniques, you can easily reverse a list in Python, selecting the most efficient approach based on your specific requirements.
Frequently Asked Questions
Q1. What is the difference between reverse() and reversed()?
The reverse() method modifies the original list in place and doesn’t return anything, meaning it changes the list directly. On the other hand, reversed() returns an iterator that can be used to iterate through the list in reverse order but doesn’t modify the original list. If you need a new reversed list, reversed() is more useful.
Q2. Can I use reverse() on a tuple or string in Python?
No, the reverse() method only works on lists and not tuples or strings. This is because tuple and strings are immutable in Python, so you can’t modify them in place. However, you can reverse them using slicing ([::-1]), or by converting them into a list first.
Q3. Does slicing ([::-1]) create a copy of the list?
Yes, slicing to reverse a list creates a new list, which is a reversed copy of the original one. This method does not modify the original list.
Q4. What is the time complexity of reversing a list in Python?
For most methods like reverse(), slicing ([::-1]), or reversed(), the time complexity is O(n), where n is the number of elements in the list. This means the operations take linear time in relation to the size of the list.
Q5. Is it better to use NumPy for list reversal?
While NumPy is great for large-scale numerical data manipulation, it’s generally unnecessary for simple tasks like reversing a list. For standard Python lists, using methods like reverse() or slicing is more efficient and doesn’t require external dependencies.
Q6. What is the best method for reversing a list in Python?
The best method depends on your specific use case. If you need an in-place reversal, reverse() is the most efficient. If you need a new list, slicing ([::-1]) and reversed() are simple options. For learning purposes, using loops (e.g., for or while) is useful, but they may not be the most efficient in terms of space and performance.
Q7. Can I reverse a list in-place without using reverse()?
Yes, you can reverse a list manually by swapping the elements. By iterating through half of the list and swapping the first and last elements, the second and second-to-last, and so on, you can reverse the list in-place.
Q8. Why does the reverse() method not return anything?
The reverse() method modifies the original list directly and does not return a new list. The list is reversed in-place, and the original list is updated. This method to get the Python reverse list avoids unnecessary memory usage.
Do check out the following Python topics:
- Python Program To Convert Celsius To Fahrenheit (+ Code Examples)
- Python Program To Convert Kilometers To Miles (+Detailed Examples)
- Python Program To Convert Decimal To Binary, Octal And Hexadecimal
- If-Else Statement In Python | All Conditional Statements + Examples
- Python Namespace & Variable Scope Explained (With Code Examples)
- Random Number Generator Python Program (16 Ways + Code Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment