Table of content:
- What Is Python? An Introduction
- What Is The History Of Python?
- Key Features Of The Python Programming Language
- Who Uses Python?
- Basic Characteristics Of Python Programming Syntax
- Why Should You Learn Python?
- Applications Of Python Language
- Advantages And Disadvantages Of Python
- Some Useful Python Tips & Tricks For Efficient Programming
- Python 2 Vs. Python 3: Which Should You Learn?
- Python Libraries
- Conclusion
- Frequently Asked Questions
- It's Python Basics Quiz Time!
Table of content:
- Python At A Glance
- Key Features of Python Programming
- Applications of Python
- Bonus: Interesting features of different programming languages
- Summing up...
- FAQs regarding Python
- Take A Quiz To Rehash Python's Features!
Table of content:
- What Is Python IDLE?
- What Is Python Shell & Its Uses?
- Primary Features Of Python IDLE
- How To Use Python IDLE Shell? Setting Up Your Python Environment
- How To Work With Files In Python IDLE?
- How To Execute A File In Python IDLE?
- Improving Workflow In Python IDLE Software
- Debugging In Python IDLE
- Customizing Python IDLE
- Code Examples
- Conclusion
- Frequently Asked Questions (FAQs)
- How Well Do You Know IDLE? Take A Quiz!
Table of content:
- What Is A Variable In Python?
- Creating And Declaring Python Variables
- Rules For Naming Python Variables
- How To Print Python Variables?
- How To Delete A Python Variable?
- Various Methods Of Variables Assignment In Python
- Python Variable Types
- Python Variable Scope
- Concatenating Python Variables
- Object Identity & Object References Of Python Variables
- Reserved Words/ Keywords & Python Variable Names
- Conclusion
- Frequently Asked Questions
- Rehash Python Variables Basics With A Quiz!
Table of content:
- What Is A String In Python?
- Creating String In Python
- How To Create Multiline Python Strings?
- Reassigning Python Strings
- Accessing Characters Of Python Strings
- How To Update Or Delete A Python String?
- Reversing A Python String
- Formatting Python Strings
- Concatenation & Comparison Of Python Strings
- Python String Operators
- Python String Functions
- Escape Sequences In Python Strings
- Conclusion
- Frequently Asked Questions
- Rehash Python Strings Basics With A Quiz!
Table of content:
- What Is Python Namespace?
- Lifetime Of Python Namespace
- Types Of Python Namespace
- The Built-In Namespace In Python
- The Global Namespace In Python
- The Local Namespace In Python
- The Enclosing Namespace In Python
- Variable Scope & Namespace In Python
- Python Namespace Dictionaries
- Changing Variables Out Of Their Scope & Python Namespace
- Best Practices Of Python Namespace
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Namespaces!
Table of content:
- What Are Logical Operators In Python?
- The AND Python Logical Operator
- The OR Python Logical Operator
- The NOT Python Logical Operator
- Short-Circuiting Evaluation Of Python Logical Operators
- Precedence of Logical Operators In Python
- How Does Python Calculate Truth Value?
- Final Note On How AND & OR Python Logical Operators Work
- Conclusion
- Frequently Asked Questions
- Python Logical Operators Quiz– Test Your Knowledge!
Table of content:
- What Are Bitwise Operators In Python?
- List Of Python Bitwise Operators
- AND Python Bitwise Operator
- OR Python Bitwise Operator
- NOT Python Bitwise Operator
- XOR Python Bitwise Operator
- Right Shift Python Bitwise Operator
- Left Shift Python Bitwise Operator
- Python Bitwise Operations And Negative Integers
- The Binary Number System
- Application of Python Bitwise Operators
- Python Bitwise Operator Overloading
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Bitwise Operators!
Table of content:
- What Is The Print() Function In Python?
- How Does The print() Function Work In Python?
- How To Print Single & Multi-line Strings In Python?
- How To Print Built-in Data Types In Python?
- Print() Function In Python For Values Stored In Variables
- Print() Function In Python With sep Parameter
- Print() Function In Python With end Parameter
- Print() Function In Python With flush Parameter
- Print() Function In Python With file Parameter
- How To Remove Newline From print() Function In Python?
- Use Cases Of The print() Function In Python
- Understanding Print Statement In Python 2 Vs. Python 3
- Conclusion
- Frequently Asked Questions
- Know The print() Function In Python? Take A Quiz!
Table of content:
- Working Of Normal Print() Function
- The New Line Character In Python
- How To Print Without Newline In Python | Using The End Parameter
- How To Print Without Newline In Python 2.x? | Using Comma Operator
- How To Print Without Newline In Python 3.x?
- How To Print Without Newline In Python With Module Sys
- The Star Pattern(*) | How To Print Without Newline & Space In Python
- How To Print A List Without Newline In Python?
- How To Remove New Lines In Python?
- Conclusion
- Frequently Asked Questions
- Think You Can Print Without a Newline in Python? Prove It!
Table of content:
- What Is A Python For Loop?
- How Does Python For Loop Work?
- When & Why To Use Python For Loops?
- Python For Loop Examples
- What Is Rrange() Function In Python?
- Nested For Loops In Python
- Python For Loop With Continue & Break Statements
- Python For Loop With Pass Statement
- Else Statement In Python For Loop
- Conclusion
- Frequently Asked Questions
- Think You Know Python's For Loop? Prove It!
Table of content:
- What Is Python While Loop?
- How Does The Python While Loop Work?
- How To Use Python While Loops For Iterations?
- Control Statements In Python While Loop With Examples
- Python While Loop With Python List
- Infinite Python While Loop in Python
- Python While Loop Multiple Conditions
- Nested Python While Loops
- Conclusion
- Frequently Asked Questions
- Mastered Python While Loop? Let’s Find Out!
Table of content:
- What Are Conditional If-Else Statements In Python?
- Types Of If-Else Statements In Python
- If Statement In Python
- If-Else Statement In Python
- Nested If-Else Statement In Python
- Elif Statement In Python
- Ladder If-Elif-Else Statement In Python
- Short Hand If-Statement In Python
- Short Hand If-Else Statement In Python
- Operators & If-Esle Statement In Python
- Other Statements With If-Else In Python
- Conclusion
- Frequently Asked Questions
- Quick If-Else Statement Quiz– Let’s Go!
Table of content:
- What Is Control Structure In Python?
- Types Of Control Structures In Python
- Sequential Control Structures In Python
- Decision-Making Control Structures In Python
- Repetition Control Structures In Python
- Benefits Of Using Control Structures In Python
- Conclusion
- Frequently Asked Questions
- Control Structures in Python – Are You the Master? Take A Quiz!
Table of content:
- What Are Python Libraries?
- How Do Python Libraries Work?
- Standard Python Libraries (With List)
- Important Python Libraries For Data Science
- Important Python Libraries For Machine & Deep Learning
- Other Important Python Libraries You Must Know
- Working With Third-Party Python Libraries
- Troubleshooting Common Issues For Python Libraries
- Python Libraries In Larger Projects
- Importance Of Python Libraries
- Conclusion
- Frequently Asked Questions
- Quick Quiz On Python Libraries – Let’s Go!
Table of content:
- What Are Python Functions?
- How To Create/ Define Functions In Python?
- How To Call A Python Function?
- Types Of Python Functions Based On Parameters & Return Statement
- Rules & Best Practices For Naming Python Functions
- Basic Types of Python Functions
- The Return Statement In Python Functions
- Types Of Arguments In Python Functions
- Docstring In Python Functions
- Passing Parameters In Python Functions
- Python Function Variables | Scope & Lifetime
- Advantages Of Using Python Functions
- Recursive Python Function
- Anonymous/ Lambda Function In Python
- Nested Functions In Python
- Conclusion
- Frequently Asked Questions
- Python Functions – Test Your Knowledge With A Quiz!
Table of content:
- What Are Python Built-In Functions?
- Mathematical Python Built-In Functions
- Python Built-In Functions For Strings
- Input/ Output Built-In Functions In Python
- List & Tuple Python Built-In Functions
- File Handling Python Built-In Functions
- Python Built-In Functions For Dictionary
- Type Conversion Python Built-In Functions
- Basic Python Built-In Functions
- List Of Python Built-In Functions (Alphabetical)
- Conclusion
- Frequently Asked Questions
- Think You Know Python Built-in Functions? Prove It!
Table of content:
- What Is A round() Function In Python?
- How Does Python round() Function Work?
- Python round() Function If The Second Parameter Is Missing
- Python round() Function If The Second Parameter Is Present
- Python round() Function With Negative Integers
- Python round() Function With Math Library
- Python round() Function With Numpy Module
- Round Up And Round Down Numbers In Python
- Truncation Vs Rounding In Python
- Practical Applications Of Python round() Function
- Conclusion
- Frequently Asked Questions
- Revisit Python’s round() Function – Take The Quiz!
Table of content:
- What Is Python pow() Function?
- Python pow() Function Example
- Python pow() Function With Modulus (Three Parameters)
- Python pow() Function With Complex Numbers
- Python pow() Function With Floating-Point Arguments And Modulus
- Python pow() Function Implementation Cases
- Difference Between Inbuilt-pow() And math.pow() Function
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python’s pow() Function!
Table of content:
- Python max() Function With Objects
- Examples Of Python max() Function With Objects
- Python max() Function With Iterable
- Examples Of Python max() Function With Iterables
- Potential Errors With The Python max() Function
- Python max() Function Vs. Python min() Functions
- Conclusion
- Frequently Asked Questions
- Think You Know Python max() Function? Take A Quiz!
Table of content:
- What Are Strings In Python?
- What Are Python String Methods?
- List Of Python String Methods For Manipulating Case
- List Of Python String Methods For Searching & Finding
- List Of Python String Methods For Modifying & Transforming
- List Of Python String Methods For Checking Conditions
- List Of Python String Methods For Encoding & Decoding
- List Of Python String Methods For Stripping & Trimming
- List Of Python String Methods For Formatting
- Miscellaneous Python String Methods
- List Of Other Python String Operations
- Conclusion
- Frequently Asked Questions
- Mastered Python String Methods? Take A Quiz!
Table of content:
- What Is Python String?
- The Need For Python String Replacement
- The Python String replace() Method
- Multiple Replacements With Python String.replace() Method
- Replace A Character In String Using For Loop In Python
- Python String Replacement Using Slicing Method
- Replace A Character At a Given Position In Python String
- Replace Multiple Substrings With The Same String In Python
- Python String Replacement Using Regex Pattern
- Python String Replacement Using List Comprehension & Join() Method
- Python String Replacement Using Callback With re.sub() Method
- Python String Replacement With re.subn() Method
- Conclusion
- Frequently Asked Questions
- Know How To Replace Python Strings? Prove It!
Table of content:
- What Is String Slicing In Python?
- How Indexing & String Slicing Works In Python
- Extracting All Characters Using String Slicing In Python
- Extracting Characters Before & After Specific Position Using String Slicing In Python
- Extracting Characters Between Two Intervals Using String Slicing In Python
- Extracting Characters At Specific Intervals (Step) Using String Slicing In Python
- Negative Indexing & String Slicing In Python
- Handling Out-of-Bounds Indices In String Slicing In Python
- The slice() Method For String Slicing In Python
- Common Pitfalls Of String Slicing In Python
- Real-World Applications Of String Slicing
- Conclusion
- Frequently Asked Questions
- Quick Python String Slicing Quiz– Let’s Go!
Table of content:
- Introduction To Python List
- How To Create A Python List?
- How To Access Elements Of Python List?
- Accessing Multiple Elements From A Python List (Slicing)
- Access List Elements From Nested Python Lists
- How To Change Elements In Python Lists?
- How To Add Elements To Python Lists?
- Delete/ Remove Elements From Python Lists
- How To Create Copies Of Python Lists?
- Repeating Python Lists
- Ways To Iterate Over Python Lists
- How To Reverse A Python List?
- How To Sort Items Of Python Lists?
- Built-in Functions For Operations On Python Lists
- Conclusion
- Frequently Asked Questions
- Revisit Python Lists Basics With A Quick Quiz!
Table of content:
- What Is List Comprehension In Python?
- Incorporating Conditional Statements With List Comprehension In Python
- List Comprehension In Python With range()
- Filtering Lists Effectively With List Comprehension In Python
- Nested Loops With List Comprehension In Python
- Flattening Nested Lists With List Comprehension In Python
- Handling Exceptions In List Comprehension In Python
- Common Use Cases For List Comprehensions
- Advantages & Disadvantages Of List Comprehension In Python
- Best Practices For Using List Comprehension In Python
- Performance Considerations For List Comprehension In Python
- For Loops & List Comprehension In Python: A Comparison
- Difference Between Generator Expression & List Comprehension In Python
- Conclusion
- Frequently Asked Questions
- Rehash Python List Comprehension Basics With A Quiz!
Table of content:
- What Is A List In Python?
- How To Find Length Of List In Python?
- For Loop To Get Python List Length (Naive Approach)
- The len() Function To Get Length Of List In Python
- The length_hint() Function To Find Length Of List In Python
- The sum() Function To Find The Length Of List In Python
- The enumerate() Function To Find Python List Length
- The Counter Class From collections To Find Python List Length
- The List Comprehension To Find Python List Length
- Find The Length Of List In Python Using Recursion
- Comparison Between Ways To Find Python List Length
- Conclusion
- Frequently Asked Questions
- Know How To Get Python List Length? Prove it!
Table of content:
- List of Methods To Reverse A Python List
- Python Reverse List Using reverse() Method
- Python Reverse List Using the Slice Operator ([::-1])
- Python Reverse List By Swapping Elements
- Python Reverse List Using The reversed() Function
- Python Reverse List Using A for Loop
- Python Reverse List Using While Loop
- Python Reverse List Using List Comprehension
- Python Reverse List Using List Indexing
- Python Reverse List Using The range() Function
- Python Reverse List Using NumPy
- Comparison Of Ways To Reverse A Python List
- Conclusion
- Frequently Asked Questions
- Time To Test Your Python List Reversal Skills!
Table of content:
- What Is Indexing In Python?
- The Python List index() Function
- How To Use Python List index() To Find Index Of A List Element
- The Python List index() Method With Single Parameter (Start)
- The Python List index() Method With Start & Stop Parameters
- What Happens When We Use Python List index() For An Element That Doesn't Exist
- Python List index() With Nested Lists
- Fixing IndexError Using The Python List index() Method
- Python List index() With Enumerate()
- Real-world Examples Of Python List index() Method
- Difference Between find() And index() Method In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python List Indexing? Take A Quiz!
Table of content:
- How To Remove Elements From List In Python?
- The remove() Method To Remove Element From Python List
- The pop() Method To Remove Element From List In Python
- The del Keyword To Remove Element From List In Python
- The clear() Method To Remove Elements From Python List
- List Comprehensions To Conditionally Remove Element From List In Python
- Key Considerations For Removing Elements From Python Lists
- Why We Need to Remove Elements From Python List
- Performance Comparison Of Methods To Remove Element From List In Python
- Conclusion
- Frequently Asked Questions
- Quiz– Prove You Know How To Remove Item From Python Lists!
Table of content:
- How To Remove Duplicates From A List In Python?
- The set() Function To Remove Duplicates From Python List
- Remove Duplicates From Python List Using For Loop
- Using List Comprehension Remove Duplicates From Python List
- Remove Duplicates From Python List Using enumerate() With List Comprehension
- Dictionary & fromkeys() Method To Remove Duplicates From Python List
- Remove Duplicates From Python List Using in, not in Operators
- Remove Duplicates From Python List Using collections.OrderedDict.fromkeys()
- Remove Duplicates From Python List Using Counter with freq.dist() Method
- The del Keyword Remove Duplicates From Python List
- Remove Duplicates From Python List Using DataFrame
- Remove Duplicates From Python List Using pd.unique and np.unipue
- Remove Duplicates From Python List Using reduce() function
- Comparative Analysis Of Ways To Remove Duplicates From Python List
- Conclusion
- Frequently Asked Questions
- Think You Know How to Remove Duplicates? Take A Quiz!
Table of content:
- What Is Python List & How To Access Elements?
- What Is IndexError: List Index Out Of Range & Its Causes In Python?
- Understanding Indexing Behavior In Python Lists
- How to Prevent/ Fix IndexError: List Index Out Of Range In Python
- Handling IndexError Gracefully Using Try-Except
- Debugging Tips For IndexError: List Index Out Of Range Python
- Conclusion
- Frequently Asked Questions
- Avoiding ‘List Index Out of Range’ Errors? Take A Quiz!
Table of content:
- What Is the Python sort() List Method?
- Sorting In Ascending Order Using The Python sort() List Method
- How To Sort Items In Descending Order Using Python sort() List Method
- Custom Sorting Using The Key Parameter Of Python sort() List Method
- Examples Of Python sort() List Method
- What Is The sorted() List Method In Python
- Differences Between sorted() And sort() List Methods In Python
- When To Use sorted() & When To Use sort() List Method In Python
- Conclusion
- Frequently Asked Questions
- Take A Quick Python's sort() Quiz!
Table of content:
- What Is A List In Python?
- What Is A String In Python?
- Why Convert Python List To String?
- How To Convert List To String In Python?
- The join() Method To Convert Python List To String
- Convert Python List To String Through Iteration
- Convert Python List To String With List Comprehension
- The map() Function To Convert Python List To String
- Convert Python List to String Using format() Function
- Convert Python List To String Using Recursion
- Enumeration Function To Convert Python List To String
- Convert Python List To String Using Operator Module
- Python Program To Convert String To List
- Conclusion
- Frequently Asked Questions
- Convert Lists To Strings Like A Pro! Take A Quiz
Table of content:
- What Is Inheritance In Python?
- Python Inheritance Syntax
- Parent Class In Python Inheritance
- Child Class In Python Inheritance
- The __init__() Method In Python Inheritance
- The super() Function In Python Inheritance
- Method Overriding In Python Inheritance
- Types Of Inheritance In Python
- Special Functions In Python Inheritance
- Advantages & Disadvantages Of Inheritance In Python
- Common Use Cases For Inheritance In Python
- Best Practices for Implementing Inheritance in Python
- Avoiding Common Pitfalls in Python Inheritance
- Conclusion
- Frequently Asked Questions
- 💡 Python Inheritance Quiz – Are You Ready?
Table of content:
- What Is The Python List append() Method?
- Adding Elements To A Python List Using append()
- Populate A Python List Using append()
- Adding Different Data Types To Python List Using append()
- Adding A List To Python List Using append()
- Nested Lists With Python List append() Method
- Practical Use Cases Of Python List append() Method
- How append() Method Affects List Performance
- Avoiding Common Mistakes When Using Python List append()
- Comparing extend() With append() Python List Method
- Conclusion
- Frequently Asked Questions
- 🧠 Think You Know Python List append()? Take A Quiz!
Table of content:
- What Is A Linked List In Python?
- Types Of Linked Lists In Python
- How To Create A Linked List In Python
- How To Traverse A Linked List In Python & Retrieve Elements
- Inserting Elements In A Linked List In Python
- Deleting Elements From A Linked List In Python
- Update A Node Of Linked List In Python
- Reversing A Linked List In Python
- Calculating Length Of A Linked List In Python
- Comparing Arrays And Linked Lists In Python
- Advantages & Disadvantages Of Linked List In Python
- When To Use Linked Lists Over Other Data Structures
- Practical Applications Of Linked Lists In Python
- Conclusion
- Frequently Asked Questions
- 🔗 Linked List Logic: Can You Ace This Quiz?
Table of content:
- What Is Extend In Python?
- Extend In Python With List
- Extend In Python With String
- Extend In Python With Tuple
- Extend In Python With Set
- Extend In Python With Dictionary
- Other Methods To Extend A List In Python
- Difference Between append() and extend() In Python
- Conclusion
- Frequently Asked Questions
- Think You Know extend() In Python? Prove It!
Table of content:
- What Is Recursion In Python?
- Key Components Of Recursive Functions In Python
- Implementing Recursion In Python
- Recursion Vs. Iteration In Python
- Tail Recursion In Python
- Infinite Recursion In Python
- Advantages Of Recursion In Python
- Disadvantages Of Recursion In Python
- Best Practices For Using Recursion In Python
- Conclusion
- Frequently Asked Questions
- Recursive Thinking In Python: Test Your Skills!
Table of content:
- What Is Type Conversion In Python?
- Types Of Type Conversion In Python
- Implicit Type Conversion In Python
- Explicit Type Conversion In Python
- Functions Used For Explicit Data Type Conversion In Python
- Important Type Conversion Tips In Python
- Benefits Of Type Conversion In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Type Conversion? Take A Quiz!
Table of content:
- What Is Scope In Python?
- Local Scope In Python
- Global Scope In Python
- Nonlocal (Enclosing) Scope In Python
- Built-In Scope In Python
- The LEGB Rule For Python Scope
- Python Scope And Variable Lifetime
- Best Practices For Managing Python Scope
- Conclusion
- Frequently Asked Questions
- Think You Know Python Scope? Test Yourself!
Table of content:
- Understanding The Continue Statement In Python
- How Does Continue Statement Work In Python?
- Python Continue Statement With For Loops
- Python Continue Statement With While Loops
- Python Continue Statement With Nested Loops
- Python Continue With If-Else Statement
- Difference Between Pass and Continue Statement In Python
- Practical Applications Of Continue Statement In Python
- Conclusion
- Frequently Asked Questions
- Python 'continue' Statement Quiz: Can You Ace It?
Table of content:
- What Are Control Statements In Python?
- Types Of Control Statements In Python
- Conditional Control Statements In Python
- Loop Control Statements In Python
- Control Flow Altering Statements In Python
- Exception Handling Control Statements In Python
- Conclusion
- Frequently Asked Questions
- Mastering Control Statements In Python – Take the Quiz!
Table of content:
- Difference Between Mutable And Immutable Data Types in Python
- What Is Mutable Data Type In Python?
- Types Of Mutable Data Types In Python
- What Are Immutable Data Types In Python?
- Types Of Immutable Data Types In Python
- Key Similarities Between Mutable And Immutable Data Types In Python
- When To Use Mutable Vs Immutable In Python?
- Conclusion
- Frequently Asked Questions
- Quiz Time: Mutable vs. Immutable In Python!
Table of content:
- What Is A List?
- What Is A Tuple?
- Difference Between List And Tuple In Python (Comparison Table)
- Syntax Difference Between List And Tuple In Python
- Mutability Difference Between List And Tuple In Python
- Other Difference Between List And Tuple In Python
- List Vs. Tuple In Python | Methods
- When To Use Tuples Over Lists?
- Key Similarities Between Tuples And Lists In Python
- Conclusion
- Frequently Asked Questions
- 🧐 Lists vs. Tuples Quiz: Test Your Python Knowledge!
Table of content:
- Introduction to Python
- Downloading & Installing Python, IDLE, Tkinter, NumPy & PyGame
- Creating A New Python Project
- How To Write Python Hello World Program In Python?
- Way To Write The Hello, World! Program In Python
- The Hello, World! Program In Python Using Class
- The Hello, World! Program In Python Using Function
- Print Hello World 5 Times Using A For Loop
- Conclusion
- Frequently Asked Questions
- 👋 Python's 'Hello, World!'—How Well Do You Know It?
Table of content:
- Algorithm Of Python Program To Add To Numbers
- Standard Program To Add Two Numbers In Python
- Python Program To Add Two Numbers With User-defined Input
- The add() Method In Python Program To Add Two Numbers
- Python Program To Add Two Numbers Using Lambda
- Python Program To Add Two Numbers Using Function
- Python Program To Add Two Numbers Using Recursion
- Python Program To Add Two Numbers Using Class
- How To Add Multiple Numbers In Python?
- Add Multiple Numbers In Python With User Input
- Time Complexities Of Python Programs To Add Two Numbers
- Conclusion
- Frequently Asked Questions
- 💡 Quiz Time: Python Addition Basics!
Table of content:
- Swapping in Python
- Swapping Two Variables Using A Temporary Variable
- Swapping Two Variables Using The Comma Operator In Python
- Swapping Two Variables Using The Arithmetic Operators (+,-)
- Swapping Two Variables Using The Arithmetic Operators (*,/)
- Swapping Two Variables Using The XOR(^) Operator
- Swapping Two Variables Using Bitwise Addition and Subtraction
- Swap Variables In A List
- Conclusion
- Frequently Asked Questions (FAQs)
- Quiz To Test Your Variable Swapping Knowledge
Table of content:
- What Is A Quadratic Equation? How To Solve It?
- How To Write A Python Program To Solve Quadratic Equations?
- Python Program To Solve Quadratic Equations Directly Using The Formula
- Python Program To Solve Quadratic Equations Using The Complex Math Module
- Python Program To Solve Quadratic Equations Using Functions
- Python Program To Solve Quadratic Equations & Find Number Of Solutions
- Python Program To Plot Quadratic Functions
- Conclusion
- Frequently Asked Questions
- Quadratic Equations In Python Quiz: Test Your Knowledge!
Table of content:
- What Is Decimal Number System?
- What Is Binary Number System?
- What Is Octal Number System?
- What Is Hexadecimal Number System?
- Python Program to Convert Decimal to Binary, Octal, And Hexadecimal Using Built-In Function
- Python Program To Convert Decimal To Binary Using Recursion
- Python Program To Convert Decimal To Octal Using Recursion
- Python Program To Convert Decimal To Hexadecimal Using Recursion
- Python Program To Convert Decimal To Binary Using While Loop
- Python Program To Convert Decimal To Octal Using While Loop
- Python Program To Convert Decimal To Hexadecimal Using While Loop
- Convert Decimal To Binary, Octal, And Hexadecimal Using String Formatting
- Python Program To Convert Binary, Octal, And Hexadecimal String To A Number
- Complexity Comparison Of Python Programs To Convert Decimal To Binary, Octal, And Hexadecimal
- Conclusion
- Frequently Asked Questions
- 💡 Decimal To Binary, Octal & Hex: Quiz Time!
Table of content:
- What Is A Square Root?
- Python Program To Find The Square Root Of A Number
- The pow() Function In Python Program To Find The Square Root Of Given Number
- Python Program To Find Square Root Using The sqrt() Function
- The cmath Module & Python Program To Find The Square Root Of A Number
- Python Program To Find Square Root Using The Exponent Operator (**)
- Python Program To Find Square Root With A User-Defined Function
- Python Program To Find Square Root Using A Class
- Python Program To Find Square Root Using Binary Search
- Python Program To Find Square Root Using NumPy Module
- Conclusion
- Frequently Asked Questions
- 🤓 Think You Know Square Roots In Python? Take A Quiz!
Table of content:
- Understanding the Logic Behind the Conversion of Kilometers to Miles
- Steps To Write Python Program To Convert Kilometers To Miles
- Python Program To Convert Kilometer To Miles Without Function
- Python Program To Convert Kilometer To Miles Using Function
- Python Program to Convert Kilometer To Miles Using Class
- Tips For Writing Python Program To Convert Kilometer To Miles
- Conclusion
- Frequently Asked Questions
- 🧐 Mastered Kilometer To Mile Conversion? Prove It!
Table of content:
- Why Build A Calculator Program In Python?
- Prerequisites To Writing A Calculator Program In Python
- Approach For Writing A Calculator Program In Python
- Simple Calculator Program In Python
- Calculator Program In Python Using Functions
- Creating GUI Calculator Program In Python Using Tkinter
- Conclusion
- Frequently Asked Questions
- 🧮 Calculator Program In Python Quiz!
Table of content:
- The Calendar Module In Python
- Prerequisites For Writing A Calendar Program In Python
- How To Write And Print A Calendar Program In Python
- Calendar Program In Python To Display A Month
- Calendar Program In Python To Display A Year
- Conclusion
- Frequently Asked Questions
- Calendar Program In Python – Quiz Time!
Table of content:
- What Is The Fibonacci Series?
- Pseudocode Code For Fibonacci Series Program In Python
- Generating Fibonacci Series In Python Using Naive Approach (While Loop)
- Fibonacci Series Program In Python Using The Direct Formula
- How To Generate Fibonacci Series In Python Using Recursion?
- Generating Fibonacci Series In Python With Dynamic Programming
- Fibonacci Series Program In Python Using For Loop
- Generating Fibonacci Series In Python Using If-Else Statement
- Generating Fibonacci Series In Python Using Arrays
- Generating Fibonacci Series In Python Using Cache
- Generating Fibonacci Series In Python Using Backtracking
- Fibonacci Series In Python Using Power Of Matix
- Complexity Analysis For Fibonacci Series Programs In Python
- Applications Of Fibonacci Series In Python & Programming
- Conclusion
- Frequently Asked Questions
- 🤔 Think You Know Fibonacci Series? Take A Quiz!
Table of content:
- Different Ways To Write Random Number Generator Python Programs
- Random Module To Write Random Number Generator Python Programs
- The Numpy Module To Write Random Number Generator Python Programs
- The Secrets Module To Write Random Number Generator Python Programs
- Understanding Randomness and Pseudo-Randomness In Python
- Common Issues and Solutions in Random Number Generation
- Applications of Random Number Generator Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python's Random Module? Prove It!
Table of content:
- What Is A Factorial?
- Algorithm Of Program To Find Factorial Of A Number In Python
- Pseudocode For Factorial Program in Python
- Factorial Program In Python Using For Loop
- Factorial Program In Python Using Recursion
- Factorial Program In Python Using While Loop
- Factorial Program In Python Using If-Else Statement
- The math Module | Factorial Program In Python Using Built-In Factorial() Function
- Python Program to Find Factorial of a Number Using Ternary Operator(One Line Solution)
- Python Program For Factorial Using Prime Factorization Method
- NumPy Module | Factorial Program In Python Using numpy.prod() Function
- Complexity Analysis Of Factorial Programs In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Factorials In Python? Take A Quiz!
Table of content:
- What Is Palindrome In Python?
- Check Palindrome In Python Using While Loop (Iterative Approach)
- Check Palindrome In Python Using For Loop And Character Matching
- Check Palindrome In Python Using The Reverse And Compare Method (Python Slicing)
- Check Palindrome In Python Using The In-built reversed() And join() Methods
- Check Palindrome In Python Using Recursion Method
- Check Palindrome In Python Using Flag
- Check Palindrome In Python Using One Extra Variable
- Check Palindrome In Python By Building Reverse, One Character At A Time
- Complexity Analysis For Palindrome Programs In Python
- Real-World Applications Of Palindrome In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Palindromes? Take A Quiz!
Table of content:
- Best Python Books For Beginners
- Best Python Books For Intermediate Level
- Best Python Books For Experts
- Best Python Books To Learn Algorithms
- Audiobooks of Python
- Best Books To Learn Python And Code Like A Pro
- To Learn Python Libraries
- Books To Provide Extra Edge In Python
- Python Project Ideas - Reference
- Quiz To Rehash Your Knowledge Of Python Books!
Python Program To Find The Square Root (8 Methods + Code Examples)
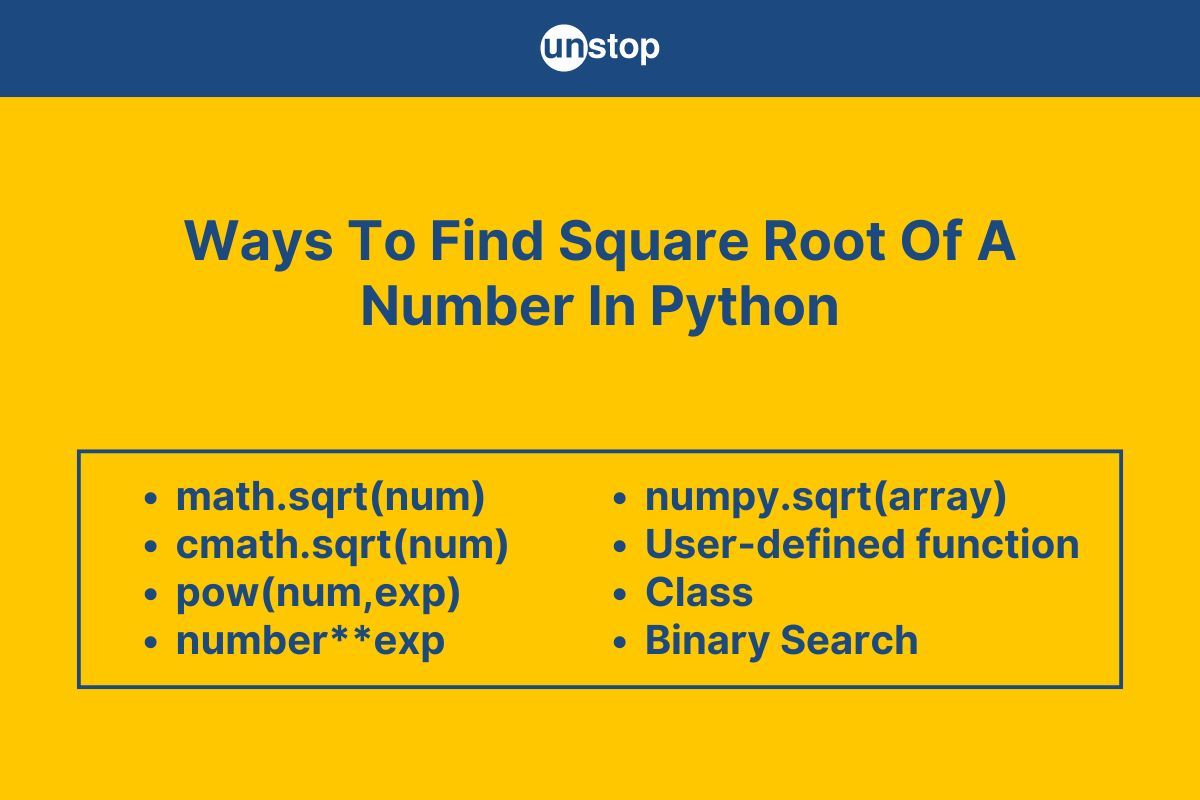
Calculating the square root of a number is a fundamental operation that you must be familiar with in mathematics. The square root of a number is defined as a value or number whose square produces the initial number. This mathematical concept (like many others) translates into programming as well. In this article, we will discuss how to write a Python program to find the square root of a number using various techniques.
Python language, with its simplicity and versatility, offers various methods to compute square roots efficiently. There are many different approaches to finding the square root in Python, including built-in functions, mathematical libraries, and iterative methods.
What Is A Square Root?
A square root is a mathematical operation that, when applied to a non-negative real number, returns a value that, when multiplied by itself, equals the original number. In simpler terms, it is a number that, when multiplied by itself, yields the original number.
Suppose x is the square root of y, then it is represented as x=√y, or we can express the same equation as x2 = y. Here, (√) is the radical symbol used to represent the root of numbers. For example, the square root of 9 is 3 because 3 multiplied by itself (3 * 3) equals 9.
Square roots are fundamental in various mathematical and scientific contexts, like calculating distances, solving equations, finding areas and volumes, and many other real-world applications.
Python Program To Find The Square Root Of A Number
You can write a Python program to find the square root of a number using a variety of methods. For this, you must be familiar with writing Python code, defining variables, creating functions, and various operators to write efficient code to find square roots using Python. Before diving into writing a Python program to find the square root, it's essential to have a basic understanding of the following concepts:
- Variables and Data Types: Familiarity with variables and different data types in Python, such as integers (int), floating-point numbers (float), and mathematical operations.
- Operators & Operations: Understanding various operators in Python, including arithmetic operators, relational operators, logical operators, etc.
- Control Flow: Knowledge of control flow structures like conditional statements (if-else) and loops (for, while) to control the flow of the program.
- Functions: Understanding how to define and call functions in Python to encapsulate reusable pieces of code.
General Outline Of Python Program To Find The Square Root Of A Number
Here's a general outline of a Python program to find the square root of a number:
- Prompt the user to enter a number for which they want to find the square root.
- Convert the input from string format to a numerical data type (integer or float) for computation.
- Check if the number is valid for the method. For example, there are some methods where you can calculate the square root of a negative number, but for others you must check the input number.
- Perform the square root calculation using one of the available methods like the built-in sqrt() function from the math module, Newton's method, or binary search.
- Display the result of the square root calculation to the user.
The pow() Function In Python Program To Find The Square Root Of Given Number
We can use the pow() function from the math library to write a Python program to find the square root of a number. It is one of the simplest and most efficient methods and can be used with both integers and float numbers. However, it always returns a float value, irrespective of the input data type.
- The function pow takes two parameters, namely the input number and the exponent.
- The exponent is used to specify the power to which the given number is to be raised.
- This returns the required value of the given number raised to the power of exponent.
- Hence, we use the power of 0.5 for the purpose of calculating the square root.
Syntax:
pow(number, exponent)
Here,
- The name of the function is pow() and the parentheses contain number referring to the input number whose square root we are finding.
- The term exponent refers to the power to which the given number is to be raised. Since we are writing a Python program to find the square root of a number, this power will be 0.5.
The basic Python program example below illustrates how to use this function.
Code Example:
#Calling the pow() function with a number whose root we want to find and the power
a = pow(49, 0.5)
#Printing the value returned by the pow() function
print(a)
Output:
7.0
Explanation:
In the basic Python code example-
- We use the pow() function to calculate the exponentiation of 49 raised to the power of 0.5, which effectively computes the square root of 49.
- The outcome of the calculation is assigned to the variable a. Note that the first argument of pow() is the base (49), and the second argument is the exponent (0.5).
- Then, we use the print() function to display the value stored in variable a, which is the square root of 49, i.e., 7.
Time Complexity: O(1)
Python Program To Find Square Root Using The sqrt() Function
The sqrt() function is a component of the math module in Python and is considered one of the most accurate methods to calculate the square root of a number. It takes a number as input and returns its square root using the algorithm of binary search.
- Similar to the pow() function, the sqrt() function also belongs to the math module.
- But unlike pow(), this function does not require two arguments.
- It only inputs a number and directly returns its square root.
Given below is the syntax for the function followed by two examples. In one, we directly call the function with a given number and in the next, we take the user-generated number and check for validity.
Syntax:
math.sqrt(number)
Here,
- sqrt() is the predefined method to find the square root function of the math module.
- The number refers to the input number whose square root we are finding.
Look at the simple Python program example below to see how to use this function to write a program to find the square root of a given number.
Code Example:
#Importing the math module
import math
#Using the sqrt() function to find the square root of a given number
a = math.sqrt(16)
#Printing the square root
print(a)
Output:
4.0
Explanation:
In the simple Python code example-
- We import the math module to use the sqrt() function, which calculates the square root of a number.
- Then, we use the math.sqrt() function to calculate the square root of 16 and the outcome is stored in variable a.
- Next, we use the print() function to display the value of the square root of 16 in a, i.e., 4.
Time Complexity: O(1)
In the simple example above, we use a given number and sqrt() function. Now, let's look at a Python program sample that shows how to use the sqrt() function from the math module to calculate the square root of a user input number.
Note that here we will check the validity of the number (as mentioned in the outline section above) since sqrt() can be used only on positive numbers.
Code Example:
#Importing the math module
import math
def find_square_root():
# Prompting user to provide an input value
number_str = input("Enter a number to find its square root: ")
# Converting the input into a suitbale type
try:
number = float(number_str)
except ValueError:
print("Invalid input. Please enter a valid number.")
return
# Using an if-else statement for root calculation
if number >= 0:
square_root = math.sqrt(number)
print("Square root of", number, "is:", square_root)
else:
print("Cannot find square root of a negative number.")
# Printing the output
find_square_root()
Output:
Enter a number to find its square root: 78
Square root of 78.0 is: 8.831760866327848
Explanation:
In the Python code sample-
- We import the math module to use the sqrt() function, which calculates the square root of a number.
- Then, we define the function find_square_root() to find the square root of a number.
- Inside the function:
- We prompt the user to enter a number to find its square root using the input() function.
- We convert the input string variable to a floating-point number using the float() function.
- If the input cannot be converted to a float, a ValueError exception is caught by the try-except block, and an error message is printed.
- If the input number is non-negative, i.e., num>=0, we calculate its square root using the math.sqrt() method and print the result.
- If the input number is negative, we print an error message indicating that the square root of a negative number cannot be found.
- In the main part, we call the find_square_root() function to execute the square root calculation.
- As shown in the output console, the input provided is 78, and the function invoked prints the square root.
The cmath Module & Python Program To Find The Square Root Of A Number
Similar to the math module, the cmath module (complex math) is a math library function module that can also be used to find the square root of a number. However, unlike the math module, this module operates on complex numbers, i.e., its sqrt() function is used to calculate the square root of a complex number.
Even if we input a purely real number, this module interprets it as a complex number with zero imaginary parts. It further processes the input according to the given syntax and returns a complex value as the output. For example, if we input (3+4j) as the argument of the sqrt() function, it returns (2+1j) as the output.
Syntax:
import cmath
cmath.sqrt(number)
Here,
- The sqrt function is the in-built function of the cmath module, which can be used on complex objects/ numbers.
- The number refers to the input complex number whose square root we are finding.
The sample Python program below illustrates the use of the sqrt() function from cmath to find the square root of a number.
Code Example:
#Importing the complex math (cmath) module
import cmath
#Using the sqrt() function from the cmath module
a = cmath.sqrt(4 + 7j)
#Printing the square root
print(a)
Output:
(2.455835677350843+1.4251767869809258j)
Explanation:
In the sample Python code-
- We import the cmath module to work with complex numbers.
- Then, we use the cmath.sqrt() function to calculate the square root of a complex number. In this case, the complex number is 4 + 7j.
- The result of this calculation is assigned to the variable a, which we then print to the console using the print() function.
- The output will be the square root of the complex number 4 + 7j, which is a complex number itself.
Time Complexity: O(log(n))
Python Program To Find Square Root Using The Exponent Operator (**)
The exponent operator, also known as the power operator (**) raises the given number to the specified power. For example, 5 ** 2 equals 25 because 5 raised to the power of 2 is 25. We can use this operator to write a Python program to find the square root of a number.
To find the square root of a number using the ** operator, you need to raise the number to the power of 0.5. However, there are some points we need to keep in mind while using this method:
- The given number must be positive. If we input a negative number with the exponential operator, it will return a complex number as the output.
- The exponential operator returns an accurate approximation of the square root, whose accuracy depends on the number. For example, it will return the accurate square root of 16 but not for 17.
Syntax:
(number)**(exponent)
Here,
- The double asterisk (**) is the exponent operator.
- The number refers to the input number whose square root we are finding.
- And exponent refers to the power to which the given number is to be raised.
The example Python program given below illustrates the use of the exponent operator to find the root of a given number.
Code Example:
#Using the exponent operator to raise number 16 to the power 0.5
a = 16 ** 0.5
#PrintingÂ
print(a)
Output:
4.0
Explanation:
In the example Python code-
- We declare a variable a and assign it the outcome of the exponential operation.
- For this, we use the exponentiation operator ** to calculate the square root of 16, which is equivalent to raising 16 to the power of 0.5.
- Next, we use the print() function to display the root of 16, i.e., 4.
Time Complexity: O(n^2)
Python Program To Find Square Root With A User-Defined Function
The process of creating a user-defined function to find the square root of a number in Python does not use a specific algorithm. It simply refers to defining a function in the program that uses another in-built function method to calculate the root and return the output value.
Let us simplify this concept with the help of an example that defines a user-defined function named find_square_root to calculate the square root of a given number using the Babylonian method (also known as Heron's method). This method is an iterative algorithm that gradually refines the approximation of the square root until it converges to the correct value.
Below is a Python program example illustrating how to create a user-defined function with this iterative approach.
Code Example:
def find_square_root(number, precision=0.0001):
if number < 0:
return "Square root of a negative number is undefined"
guess = number / 2 # Initial guess, could be any reasonable value
while abs(guess * guess - number) > precision:
guess = (guess + number / guess) / 2
return guess
# Example usage
number = 25
result = find_square_root(number)
print("Square root of", number, "is approximately:", result)
Output:
Square root of 25 is approximately: 5.000000000016778
Explanation:
In the Python code example-
- We define the function find_square_root() to approximate the square root of a given number using Newton's method.
- The function takes two parameters: number (the number for which we want to find the square root) and precision (the precision level for the approximation, with a default value of 0.0001).
- Inside the function, we use an if-statement to check if the input number is negative, i.e., number<0.
- If the condition is true, the function returns a message indicating that the square root of a negative number is undefined. If the condition is false, the flow moves to the next line in the function definition.
- Next, we initialize a variable guess with the value (number / 2), as an initial guess for the square root. This initial guess could be any reasonable value.
- Then, we enter a while loop that continues until the absolute difference between the square of the guess variable and the number variable is less than or equal to the specified precision, i.e., (guess*guess - number) > precision.
- We use the abs() function to calculate the absolute value in the previous step.
- Inside the loop, we update the guess variable using Newton's method formula, i.e., guess = (guess + number/guess) / 2.
- Once the loop terminates, the function returns the final value of guess, which is an approximation of the square root of the input number.
- In the main part of the program, we declare a variable number and initialize it with the value 25.
- Then, we call the find_square_root() function with number as the argument, which returns an approximation of the square root of number. The outcome is assigned to the result variable.
- Lastly, we use the print() function with a formatted string message to print the value of the approximation of the square root of the number.
Time Complexity: O(log n)
Python Program To Find Square Root Using A Class
A class in Python may be defined as a template for creating objects that have similar properties and behaviors. It is a way of organizing the code so that it can be reused in the program further.
We can write a Python program to find the square root of a number using the concept of classes. Within this, a constructor is used to input a number and calculate its square root using the method defined inside the class.
Code Example:
#Defining a class with an object and method to calculate the square root
class SqRt:
#The class constructor
def __init__(self, num):
self.num = num
#Function to calculate the square root
def calc_sqrt(self):
sqrt = 0
#using a for loop
for i in range(1, self.num + 1):
if i * i <= self.num:
sqrt = i
else:
break
return sqrt
#Initializing a number to create an object of the class
#Then calling the class method to calculate the sqr root
num = 25
sqrt_obj = SqRt(num)
print(sqrt_obj.calc_sqrt())
Output:
5
Explanation:
In the Python code-
- We define a class SqRt to represent an object that calculates the square root of a given number.
- The class has an __init__() method, which acts as a constructor. It initializes the object with the given num.
- Inside the constructor, we assign the value of num passed as an argument to the instance variable self.num.
- The class also has a method calc_sqrt(), which calculates the square root of the number stored in self.num.
- Inside this method, we initialize a variable sqrt to 0 to store the calculated square root.
- Then, we use a for loop to iterate through numbers from 1 to self.num. In the loop, we have an if-else statement.
- The if condition checks if the square of the current number (i * i) is less than or equal to self.num, i.e., i * i <= self.num.
- If the condition is true, the if-block updates the value of the sqrt variable to the current number i.
- If the condition is false, i.e., the square of the current number exceeds self.num, then the else-block is executed, and the break statement makes the flow break out of the loop.
- In the main part of the program, we first initialize a variable num with the value 25.
- Then, we create an instance sqrt_obj of the SqRt class with the num variable.
- Next, we call the calc_sqrt() method on sqrt_obj object to calculate the square root of the number stored in num.
- Lastly, we use the print() function to display the value of the square root.
Time Complexity: O(√n)
Python Program To Find Square Root Using Binary Search
The binary search method is also known as the half-interval search or logarithmic search. It is used to find the position of the target value, i.e., the square root of the given number, within a sorted range. This is done by comparing this target value to that of the middle element of the range. Below is an example Python program to find the square root of a number using this approach.
Code Example:
#Defining the function
def square_root(num):
low = 0
high = num
#Using a while loop
while low <= high:
mid = (low + high) // 2
if mid * mid == num:
return mid
elif mid * mid < num:
low = mid + 1
else:
high = mid - 1
return mid
print(square_root(9))
Output:
3
Explanation:
In the code-
- We first define a function square_root(num) to calculate the square root of a given number (here, parameter num) using binary search.
- Inside the function, we initialize two variables, low and high, with the values 0 and num, respectively. These will act as the lower and upper bounds for the binary search.
- Then, we employ a while loop that iterates until the loop condition low<=high, i.e., low is less than or equal to high, is true.
- In the loop, we calculate the midpoint of the range from the average of low and high and assign this to the variable mid.
- Next, we use an if-elif-else statement to calculate and return the square root. Here-
- The if-condition checks if the square of mid is equal to num, i.e., mid*mid==num. If this is true, then mid is returned as the square root. If not, then we will move to the next line.
- The elif-condition checks whether the square of mid is less than num, i.e., mid*mid<num. If so, the value of low is updated to mid + 1 to search in the higher half.
- If this is also false, then it means that the square of mid is greater than num. So, we move to the else-block and update the value of high to mid-1 to search in the lower half.
- If the loop exits without finding the exact square root, the last value of mid is returned as an approximation.
- In the main part, we call the square_root() function with an argument of 9 inside a print() function.
- The function calculates the square root of 9 using binary search and prints the result to the console.
Time Complexity: O(log n)
Python Program To Find Square Root Using NumPy Module
The NumPy module in Python is a collection of mathematical functions for the array objects. Similar to the math and cmath modules, this module also contains the sqrt() function to find the square root of a number in Python. The numpy.sqrt() function is used explicitly to find the square root of the elements in an array.
Syntax:
numpy.sqrt(arr)
Here,
- The sqrt() is the inbuilt function of the numpy module.
- The arr refers to an array of objects that is a parameter for the sqrt() function. The function will find the square root of each number in the array.
Code Example:
#Import numpy module
import numpy
#Creating a numpy array using the array() function from this module
arr = numpy.array([4, 9, 16, 25])
#Printing the array of square roots of the previous array
print(numpy.sqrt(arr))
Output:
[2. 3. 4. 5.]
Explanation:
In the above code-
- We first import the numpy module to utilize its mathematical functions.
- Then, we create a NumPy array arr containing the numbers [4, 9, 16, 25], using the array() function.
- Next, we use the numpy.sqrt() function to calculate the square root of each element in the array arr.
- The numpy.sqrt() function returns a new array containing the square roots of the elements in the input array, which is printed to the console by the print() function.
Time Complexity: O(n)
Conclusion
We can write a Python program to find the square root of a number using various techniques, functions, or methods. These include the in-built square root function from the various modules, i.e., math, cmath, and NumPy. We can also implement iterative algorithms like Heron's method or binary search. All in all, the Python programming language provides flexibility and efficiency in square root calculations. Understanding these methods equips programmers with the tools to handle square root computations effectively in their projects.
Frequently Asked Questions
Q. How to find an exponent in Python?
There are many built-in methods in Python to find an exponent. These include the pow() function and the exponent (**) operator, where a given number is raised to a specific value.
Syntax for Exponent (**) Operator:
(number) ** (exponent)
Here, the double asterisk (**) is the operator, the number refers to the input number, and the exponent refers to the power to which the given number is to be raised.
Code Example:
#Using exponent (**) operator to calculate the cube of a number
expon = 2 ** (3)
#Printing the cube
print(expon)
Output:
8
Explanation:
- We begin by initializing a variable named expon.
- This variable defines the operation of raising the given number to the power of a specified exponent. Here, we input 3 as the exponent.
- We then instruct the program to output the value of the given variable using the print() function.
- Hence, we obtain 8 as the square of the input number 2.
Q. How do you square a number in Python?
Squaring a number is similar to using the methods of exponent in Python. We can use the pow() function or the exponent (**) operator to raise the given number to the power 2 and obtain the squared value. For this, we input the second argument of the pow() function as 2 to obtain the answer. The functions pow(3,2) and 3**2 return the value 9, i.e., the square of the number 3.
Code Example:
#Using pow() function where first parameter is the number to be raised, and second is the power
sqr = pow(5,2)
#Printing the sqaure of given number
print(sqr)
Output:
4
Explanation:
- We begin by initializing a variable sqr. and assign it the value using the pow() function.
- In the pow() function, we have the first input as 5, which is the number that we want to sqaure.
- And the second argument is 2, since we want to calculate the square (power 2) of the first number.
Q. How do you find a cube root in Python?
We can use the same methods of exponentiation to find the cube root of a given number by only changing the value of the exponent, i.e., to which the given number is to be raised. For the purpose of obtaining the cube root, we raise the number to the power of 1⁄3, i.e., pow(27,⅓) and 27**(⅓) return the value 3 as the cube root of the number 27.
Code Example:
#Calculating cube root by raising the number to the power (1/3)
cube_root = 27 ** (1/3)
#Printing the cube root of 27, stored in variable cube_root
print(cube_root)
Output:
3.0
Explanation:
- We begin by initializing a variable cube_root and assigning it the value of number 27, raised to the power (1/3) using the exponent (**) operator.
- Then, we use the print() function to display the value for the same.
Q. How do you square root an array in Python?
The most suitable method of calculating the square root of an array in Python is to use the sqrt() function under the NumPy module. The numpy.sqrt() function takes an array as the input and returns the array of the square roots of each element.
Syntax:
numpy.sqrt(array)
Here, the function sqrt() is predefined in the NumPy module, and the array is the input array argument.
Code Example:
#Importing the NumPy module to use the function predefined in it
import numpy
#Using Numpy's array() function to create an array of integers
array = numpy.array([1, 4, 9, 16, 25])
#Calling the sqrt() function from NunmPy inside print()
#This calculates the square root of each element in the array and prints the new array
print(numpy.sqrt(array))
Output:
[1. 2. 3. 4. 5.]
Explanation:
- We begin our program by importing the numpy module into our program.
- Next, we define a variable named array into the module with the values 1, 4, 9, 16, 25 in it.
- We further instruct the program to return the square root using the numpy.sqrt() function on the array.
- Hence, we obtain the array of square roots as [1. 2. 3. 4. 5.] using the print() function.
Q. What is the fastest way to find square roots in Python?
The fastest method of finding square roots in Python usually depends on the requirements. However, in general, the sqrt() function of the math module is considered the best choice as it is fast and available for all versions of Python. This function is considered the absolute fastest in the NumPy module of Python as well.
Syntax:
math.sqrt(number)
Here, the function sqrt() is defined in the math module, and the parameter number is the values whose root will be calculated.
Code Example:
#Importing the math module
import math
#Calling the math.sqrt() function with given number 16, and assigning outcome to variable a
a = math.sqrt(16)
#Using print() to display the outcome
print(a)
Output:
4.0
Explanation:
- We begin our code by importing the math module to use the sqrt() function.
- Next, we define the variable a, which stores the math.sqrt() function with 16 as the input argument.
- Then, we print the value of an (i.e., the square root) using the print() function.
🤓 Think You Know Square Roots In Python? Take A Quiz!
You must now know how to write a Python program to find the square root of a number. Here are a few more topics you will enjoy exploring:
- 12 Ways To Compare Strings In Python Explained (With Examples)
- Python Bitwise Operators | Positive & Negative Numbers (+Examples)
- Python String.Replace() And 8 Other Ways Explained (+Examples)
- How To Reverse A String In Python? 10 Easy Ways With Examples
- Difference Between List And Tuple In Python With Examples
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Vishwajeet Singh 1 month ago
niyati m singh 1 month ago
Shradha Manure 1 month ago
Pardha Venkata Sai Patnam 1 month ago
PUJALA JYOTHIRMAYE 1 month ago
Deepak Kaura 1 month ago