Table of content:
- What Are Python Built-In Functions?
- Mathematical Python Built-In Functions
- Python Built-In Functions For Strings
- Input/ Output Built-In Functions In Python
- List & Tuple Python Built-In Functions
- File Handling Python Built-In Functions
- Python Built-In Functions For Dictionary
- Type Conversion Python Built-In Functions
- Basic Python Built-In Functions
- List Of Python Built-In Functions (Alphabetical)
- Conclusion
- Frequently Asked Questions
Python Built-in Functions | A Detailed Explanation (+Code Examples)
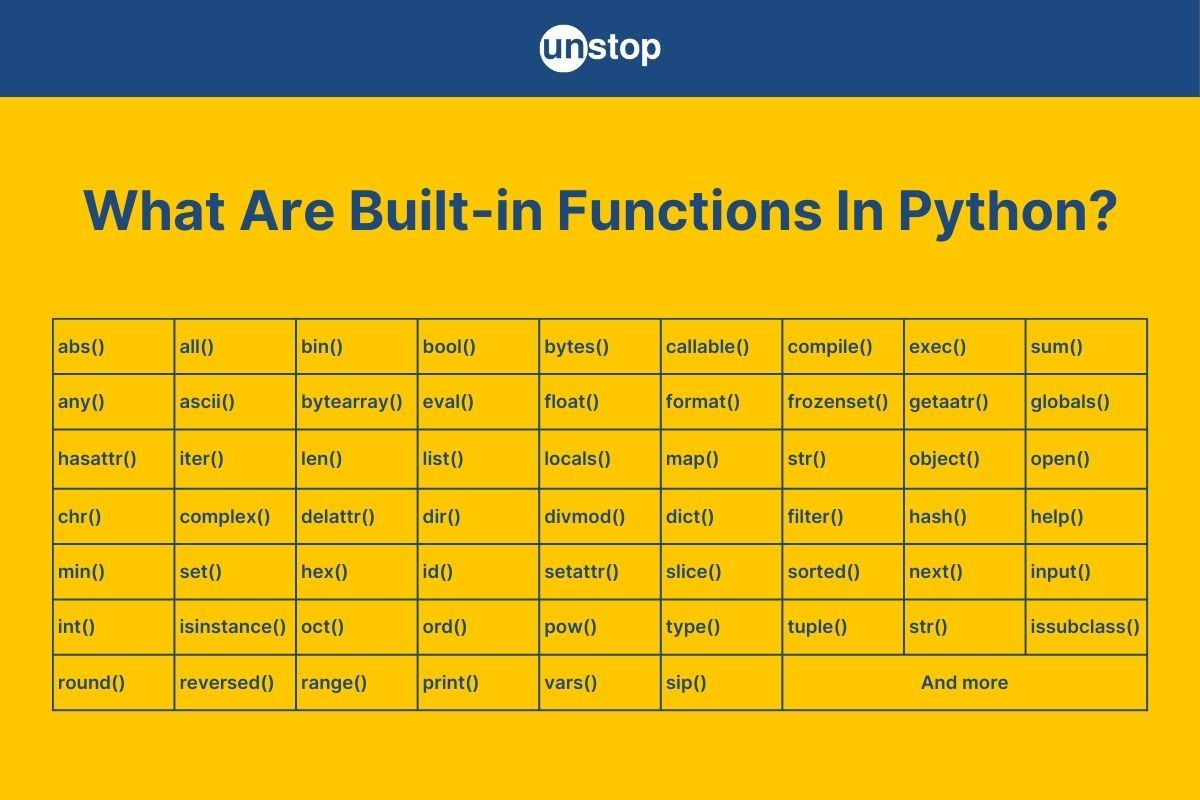
Functions are building blocks of a program. That is, they allow you to break a complеx program into small, mеaningful, and rеusablе blocks of codе. It only runs when it is called, can take arguments, and can return data as a result. There are two types of functions - built-in and user-defined functions.
In this article, we will discuss Python built-in functions, all its subdivisions, dеfinition, use-case, and modules in which they are present. You will get a list of all Python built-in functions with a description and code examples. So, let’s start by understanding what Python built-in functions are.
What Are Python Built-In Functions?
Built-in functions in Python arе prе-dеfinеd functions that are readily available and accеssiblе without rеquiring any import statеmеnts or additional sеtups.
- Thеsе functions arе part of thе Python standard library and offеr a widе rangе of functionalitiеs to perform various mathematical tasks.
- The Python built-in functions offer a sеt of opеrations to perform common tasks such as data manipulation, mathеmatical opеrations, string manipulation, and filе opеrations.
- In some cases, you might have to import specific modules or libraries to use the functions. We will discuss these in the respective sections below.
For example, the built-in library functions like len(), max(), min(), str(), etc. Let’s consider a few short examples illustrating the use of these built-in functions.
Code Example 1:
bnVtYmVycyA9IFs3LCAyLCA5LCA0LCA1XQoKcHJpbnQobWF4KG51bWJlcnMpKSAjIE91dHB1dDogOQpwcmludChtaW4obnVtYmVycykpICMgT3V0cHV0OiAy
Here, max() and min() Functions:
- max() returns the maximum value in an iterable. (which is 9)
- min() returns the minimum value in an iterable. (which is 2)
Code Example 2:
bXlfbGlzdCA9IFsxLCAyLCAzLCA0LCA1XQoKI0NoZWNraW5nIHRoZSBsZW5ndGggb2YgdGhlIGxpc3QKcHJpbnQobGVuKG15X2xpc3QpKSAjIE91dHB1dDogNQ==
Here, lеn() function rеturns thе lеngth of an objеct (numbеr of itеms in an itеrablе, lеngth of a string, еtc.), which is 5.
Now that you have a brief overview of what Python built-in functions are let’s move on to discussing each sub-division of built-in functions separately.
Mathematical Python Built-In Functions
Python language offers a robust set of built-in functions specifically to carry out mathematical operations. This makes it easier for the programmers to perform calculations in their code.
- These functions are a part of Python's core language and do not require any module to be imported. Common examples of this type of Python built-in functions are sum(), max(), min(), abs(), and pow()
- However, there is a built-in math module in the Python standard library that can be imported to utilise some additional functionalities/ utilities.
- This module provides a wide range of mathematical constants and functions that allow users to perform logarithmic, trigonometric, exponential, numeric, and hyperbolic functions from basic to advanced.
However, in this section, we will cover only the more commonly used mathematical Python built-in functions. Listed in the table below are some of these functions, with the syntax and a short description.
Function Name |
Syntax |
Description |
abs() |
abs(num) |
Returns the absolute value of a number num. |
max() |
max(iterable) |
Returns the largest of two or more arguments or the largest item in an iterable |
min() |
min(iterable) |
Returns the smallest of two or more arguments or the smallest item in an iterable |
pow() |
pow(x, y[, z]) |
Returns x to the power of y, optionally with a modulus z. |
sum() |
sum(iterable[, start]) |
Returns the aggregate of all elements in an iterable. Optionally, start can be provided as the initial value. |
divmod() |
divmod(a, b) |
Takes two numbers and returns a pair of numbers (a // b, a % b). |
round() |
round(number[, ndigits]) |
Rounds a number to a given precision in decimal digits. |
Note: In the above table, the square brackets [ ] denote an optional syntax argument.
Now, let’s discuss these Python built-in functions individually with the help of examples.
Python abs() Function
The abs() function is a built-in function that is always available to the Python interpreter. Meaning there is no need to import any module to use it.
- It takes a number as an argument and returns its absolute value (magnitude).
- We use this function in scenarios where the magnitude of a value is needed, such as distance calculations or error handling.
The simple Python program example below illustrates the use of this function to calculate the absolute difference between two numbers.
Code Example:
I0RlY2xhcmluZyBhbmQgaW5pdGlhbGl6aW5nIHR3byB2YXJpYWJsZXMKeCA9IDUKeSA9IDEyCgojVXNpbmcgYWJzKCkgdG8gZ2V0wqAgdGhlIGFic29sdXRlIHZhbHVlIG9mIHRoZSBkaWZmZXJlbmNlIHdoaWNoIGlzIG5lZ2F0aXZlCmRpZmZlcmVuY2UgPSBhYnMoeCAtIHkpCnByaW50KCJEaWZmZXJlbmNlOiIsIGRpZmZlcmVuY2Up
Output:
Difference: 7
In the simple Python code example,
- We first declare two variables, x and y, and assign them values 5 and 12, respectively.
- Then, we pass the difference of two variables/ numbers, x and y (x-y = -7), as an argument in the abs() function.
- The function returns the absolute value (magnitude) of the difference, as seen in the output window.
Python max() Function
The max() function is a built-in function is always available to the Python interpreter and does not require you to import any module to use it.
- The Python built-in max() function returns the largest of two or more arguments or the largest item in an iterable.
- It is useful for finding the maximum value within a collection of numbers or determining the maximum among multiple values.
Look at the Python program example below, which illustrates how this function works.
Code Example:
I0NyZWF0aW5nIGEgbGlzdApudW0gPSBbMywgNywgMSwgOSwgNF0KCiNVc2luZyBtYXgoKSBmdW5jdGlvbiB0byBmaW5kIHRoZSBtYXhpbXVtIG51bWJlcsKgIGluIHRoZSBsaXN0Cm1heF92YWx1ZSA9IG1heChudW0pCnByaW50KCJNYXhpbXVtIHZhbHVlOiIsIG1heF92YWx1ZSk=
Output:
Maximum value: 9
In the Python code example, we first create a list called num, containing five integer values.
- Then, we pass the list of numbers to the max() function as an argument.
- The function finds the greatest value present in the list and stores it in the max_value variable.
- We then print the value using the print() function.
Python min() Function
This Python built-in function is also always available to the interpreter without the need to import any module.
- The min() function returns the smallest of two or more arguments or the smallest item in an iterable.
- It is commonly used to find the minimum value within a collection or identify the minimum among several values.
Code Example:
eCA9IDEwCnkgPSAyNQp6ID0gMTgKCm1pbl92YWx1ZSA9IG1pbih4LCB5LCB6KQpwcmludCgiTWluaW11bSB2YWx1ZToiLCBtaW5fdmFsdWUp
Output:
Minimum value: 10
In the example Python code, we create three integer variables: x, y, and z.
- Then, we call the min() function, passing these variables as arguments to determine the smallest value.
- The function finds the minimum value and stores it in the min_value object, which we then print to the console.
Python pow() Function
Another Python built-in function that is available for use without any module importation.
- The pow() function returns the value of x to the power of y, optionally with a modulus z.
- It is a versatile function used for exponentiation operations, including calculating squares, cubes, and modular exponentiation.
Code Example:
YmFzZSA9IDIKZXhwb25lbnQgPSAzCm1vZHVsdXMgPSA1CgpyZXN1bHQgPSBwb3coYmFzZSwgZXhwb25lbnQsIG1vZHVsdXMpCnByaW50KCJSZXN1bHQgb2YgbW9kdWxhciBleHBvbmVudGlhdGlvbjoiLCByZXN1bHQp
Output:
Result of modular exponentiation: 3
In the example Python program, we create three variables, base, exponent, and modulus, and assign them integer values.
- Note that here, we include the third variable, z = 5 (from syntax).
- Then, we pass the variables as arguments to the pow() function.
- The function calculates the exponent 23 and then performs the modulo (%) operation by the third variable.
- It returns the remainder after dividing the exponent 23 by 5, which is 3.
Note: Modulo (%) operation between two numbers a & b is the remainder when b divides a.
Python sum() Function
As the name suggests, this Python built-in function returns the aggregate of all elements in an iterable, such as a list, tuple, or set. It is convenient for calculating the total of numerical values within a collection. Consider the example to calculate the sum of numbers in a list.
Code Example:
bnVtYmVycyA9IFsxLCAyLCAzLCA0LCA1XQoKI1VzaW5nIHN1bSgpIHRvIGZpbmQgdGhlIGFnZ3JlZ2F0ZSBvZiBhbGwgZWxlbWVudHMgb2YgYSBsaXN0CnRvdGFsID0gc3VtKG51bWJlcnMpCnByaW50KCJUb3RhbDoiLCB0b3RhbCk=
Output:
Total: 15
In the sample Python program,
- We pass the list numbers to the sum() function as an argument.
- The function calculates the sum of all the numbers in the list and stores them in the total object.
- The outcome is 15, which we print to the console using the print() function.
Python round() Function
This Python built-in function, which is always available to the interpreter (without needing a specific module), rounds a number to a specified precision in terms of decimal places. This is especially useful in financial calculations or whenever you need controlled precision.
Code Example:
I1Bhc3NpbmcgYSBkZWNpbWFsIG51bWJlciBhbmQgdGhlIG51bWJlciBvZiBkZWNpbWFsIHBsYWNlcyB3ZSBuZWVkIHRvIHRoZSByb3VuZCgpIGZ1bmN0aW9uCnJlc3VsdCA9IHJvdW5kKDMuMTQxNTksIDIpCgojUHJpbnRpbmcgdGhlIG91dHB1dCB0byB0aGUgY29uc29sZQpwcmludChyZXN1bHQp
Output:
3.14
In the sample Python code, we call the round() function, passing the numbers 3.14159 and 2 (to specify the decimal places to be rounded) as arguments. The function rounds the first argument and returns the value 3.14, which we print to the console.
Python divmod() Function
This Python built-in function is used when you need both the quotient and the remainder from dividing two numbers. The function is also always available to the Python interpreter and is more efficient than using the division and modulo operators separately.
Code Example:
I1Bhc3NpbmcgdHdvIGludGVnZXIgdG8gZGl2bW9kKCkgZnVuY3Rpb24KcmVzdWx0ID0gZGl2bW9kKDEwLCAzKQoKcHJpbnQocmVzdWx0KQ==
Output:
(3, 1)
In the Python example program,
- We pass two numbers, 10 and 3, to the divmod() function and store the outcome in the result variable.
- The function divides the number 10 by 3, then returns the quotient (3) and the remainder (1) in the form of a tuple (3, 1).
Next, we will discuss those mathematical Python built-in functions that can only be used after we have imported the in-built math module. Listed in the table below are all such functions, with a brief description and the syntax.
Method Name |
Syntax |
Description |
cos() |
math.cos(x) |
It returns the cosine value of x, where x is in radians. |
sin() |
math.sin(x) |
It returns the sine value of x, where x is in radians. |
tan() |
math.tan(x) |
It returns the tangent value of x, where x is in radians. |
cosh() |
math.cosh(x) |
It returns the hyperbolic cosine value of x. |
sinh() |
math.sinh(x) |
It returns the hyperbolic sine value of x. |
tanh() |
math.tanh(x) |
It returns the hyperbolic tangent value of x. |
acos() |
math.acos(x) |
It returns the arc cosine value of x, in radians. |
asin() |
math.asin(x) |
It returns the arc sine value of x, in radians. |
atan() |
math.atan(x) |
It returns the arc tangent value of x, in radians. |
acosh() |
math.acosh(x) |
It returns the inverse hyperbolic cosine value of x. |
asinh() |
math.asinh(x) |
It returns the inverse hyperbolic sine value of x. |
atanh() |
math.atanh(x) |
It returns the inverse hyperbolic tangent value of x. |
ceil() |
math.ceil(x) |
It returns the ceiling value of x, the smallest integer greater than or equal to x. |
degrees() |
math.degrees(x) |
It converts angle x from radians to degrees. |
dist() |
math.dist(a,b) |
It returns the Euclidean distance between two points (a and b), where a and b are the coordinates of that point. |
exp() |
math.exp(x) |
It returns the exponential value of x, i.e., ex, where e is Euler's number. |
expm1() |
math.expm1(x) |
It returns the value of ex−1, where e is Euler's number. |
fabs() |
math.fabs(x) |
It returns the absolute value of x. |
factorial() |
math.factorial(x) |
It returns the factorial of x. |
floor() |
math.floor(x) |
It returns the floor value of x, the largest integer less than or equal to x. |
gamma() |
math.gamma(x) |
It returns the gamma function value at x. |
gcd() |
math.gcd(a,b) |
It returns the greatest common factor between a and b. |
isfinite() |
math.isfinite(x) |
It returns True if x is a finite number. |
isinf() |
math.isinf(x) |
It returns True if x is positive or negative infinity. |
lgamma()
|
math.lgamma(x)
|
It returns the natural logarithm of the absolute value of the Gamma function of x. |
log() |
math.log(x [, base]) |
It returns the natural logarithm value of x. If the optional base is specified, return the logarithm of x to that base. |
log10() |
math.log10(x) |
It returns the value of base-10 logarithm of x |
log1p() |
math.log1p(x) |
It returns the natural logarithm value of 1+x |
log2() |
math.log2(x) |
It returns the base-2 logarithm value of x |
perm() |
math.perm(n,k) |
It returns the number of ways to choose k items from n items in order and without repetition. |
prod() |
math.prod(iterable [, start]) |
It calculates the product of all values in an iterable. Optional start value of the product (default = 1) |
radians() |
math.radians(x) |
It converts the value of angle x from degrees to radians. |
remainder() |
math.remainder(a,b) |
It returns the remainder when b divides a. |
sqrt() |
math.sqrt(x) |
It returns the square root value of x. |
trunc() |
math.trunc(x) |
It returns the truncated integer value of x. |
Note: In the above table, [ ] in the syntax denotes an optional argument.
The Python built-in functions in the table above can further be categorised based on the nature of the operations they perform. In the following sections, we will discuss these subcategories and the functions, along with examples.
- As seen in the table above, the syntax for these functions includes the term “math” followed by a dot operator to indicate that we are using a function from the math module.
- In addition to mentioning this in the syntax, we will also have to import the module at the beginning of the code every time we use any of these functions.
Numeric Functions | Math Python Built-in Functions
This subcategory of math Python built-in functions includes those that enable users to perform basic to advanced numeric operations. It includes methods like ceil(), floor(), fabs(), trunc(), remainder(), gcd(), perm(), and factorial(). Let’s discuss some of the most commonly used functions in more detail.
Python math.ceil() Method
The Python built-in function takes a numeric value (integer or floating-point) as argument and returns the smallest integer greater than or equal to the given number. It is commonly used in situations where you need to ensure that a value is rounded up to the next whole number.
Code Example:
aW1wb3J0IG1hdGgKCmNlaWxfbnVtID0gbWF0aC5jZWlsKDQuMykKcHJpbnQoIkNlaWwgb2YgNC4zOiIsIGNlaWxfbnVtKQ==
Output:
Ceil of 4.3: 5
In the code example, we first import the math module.
- Then call the math.ceil() method, passing the number 4.3 as an argument.
- The function calculates and returns the ceil value of 4.3 and stores it in the ceil_num variable.
Python math.floor() Method
This Python built-in function takes a numeric value (integer or floating-point) as argument and returns the greatest integer smaller than or equal to the given number. It is commonly used in situations where you need to ensure that a value is rounded up to the previous whole number.
Code Example:
aW1wb3J0IG1hdGgKCmZsb29yX251bSA9IG1hdGguZmxvb3IoLTMuMikKcHJpbnQoIkZsb29yIG9mIC0zLjI6IiwgZmxvb3JfbnVtKQ==
Output:
Floor of -3.2: -4
In the code example, we call the math.floor() method, passing the negative number -3.2 as an argument. The function calculates and returns the floor value of -3.2 and stores it in the floor_num variable.
Python math.fabs() Method
The Python built-in function takes a numeric value (integer or floating-point) as an argument and returns the absolute value of the given number as a floating-point number. It is commonly used in situations where you require the magnitude of the number without considering its sign.
Code Example:
I1VzaW5nIGFicygpIGZ1bmN0aW9uIHRvIGZpbmQgYW4gYWJzb2x1dGUgdmFsdWUKYWJzX3ZhbCA9IG1hdGguZmFicygtMy44KQoKcHJpbnQoIkFic29sdXRlIHZhbHVlIG9mIC0zLjg6IiwgYWJzX3ZhbCk=
Output:
Absolute value of -3.8: 3.8
In the code example, we call the math.fabs() method, passing the number -3.8 as an argument. The function calculates and returns the absolute value of -3.8 and stores it in the abs_val variable.
Python math.gcd() Method
The Python built-in function takes two integers as arguments and returns the greatest common divisor of the two given numbers.
Code Example:
I0NhbGN1bGF0aW5nIHRoZSBHQ0QgdXNpbmcgdGhlIGluLWJ1aWx0IGZ1bmN0aW9uCmdjZF92YWwgPSBtYXRoLmdjZCgxMiwgMTgpCgojUHJpbnRpbmcgdGhlIGdjZApwcmludCgiR0NEIG9mIDEyIGFuZCAxODoiLCBnY2RfdmFsKQ==
Output:
GCD of 12 and 18: 6
In the code example, we pass two numbers 12 and 18 to the math.gcd() method. The function calculates and returns the gcd value of 12 and 18 and stores it in the gcd_val variable.
Python math.perm() Method
The Python built-in function takes two integers (say, k and n) as arguments and returns the number of permutations of choosing k items from n items without replacement.
Code Example:
aW1wb3J0IG1hdGgKCiNDYWxjdWxhdGluZyB0aGUgcGVybXV0YXRpb25zIG9mIHR3byBudW1iZXJzIGFuZCBwcmludGluZyB0aGUgb3V0Y29tZQpwZXJtX3ZhbCA9IG1hdGgucGVybSgxMCwgMykKcHJpbnQoIlBlcm11dGF0aW9ucyBvZiAxMCBjaG9vc2UgMzoiLCBwZXJtX3ZhbCk=
Output:
Permutations of 10 choose 3: 720
In the code example, we pass numbers 10 and 3 to the math.perm() method. It calculates and returns the number of permutations of 10 choose 3, and stores it in the perm_val variable.
Python math.factorial() Method
The Python built-in function takes a non-negative integer as an argument and returns the factorial of the given number, which is the product of all positive integers less than or equal to the given number.
Code Example:
aW1wb3J0IG1hdGgKCmZhY3RvcmlhbF92YWwgPSBtYXRoLmZhY3RvcmlhbCgxMCkKcHJpbnQoIkZhY3RvcmlhbCBvZiAxMDoiLCBmYWN0b3JpYWxfdmFsKQ==
Output:
Factorial of 10: 3628800
In the code example, we use the math.factorial() method to calculate the factorial of 10. The method returns the factorial of 10 and stores it in the factorial_val variable.
Python math.remainder() Method
The Python built-in function takes two numeric values (integer or floating-point) as arguments (say, x and y), performs division x/y and returns the remainder of the division.
Code Example:
aW1wb3J0IG1hdGgKCnJlbWFpbmRlcl92YWwgPSBtYXRoLnJlbWFpbmRlcigtMTAsIDMpCnByaW50KCJSZW1haW5kZXIgb2YgLTEwIC8gMzoiLCByZW1haW5kZXJfdmFsKQ==
Output:
Remainder of -10 / 3: -1.0
In the code example, we use the math.remainder() method to calculate the remainder when -10 divided by 3. The method returns the remainder of -10/3 and stores it in the remainder_val variable which we then print to the console.
Python math.trunc() Method
The Python built-in function takes a numeric value (integer or floating-point) and returns the integer part of the given number by removing the fractional part.
Code Example:
aW1wb3J0IG1hdGgKCnRydW5jX251bSA9IG1hdGgudHJ1bmMoLTMuMikKcHJpbnQoIlRydW5jYXRlZCB2YWx1ZSBvZiAtMy4yOiIsIHRydW5jX251bSnCoCAjIE91dHB1dDogLTM=
Output:
Truncated value of -3.2: -3
In the code example, we use the math.trunc() method to calculate the integer part of -3.2. The method returns the truncated value of -3.2 and stores it in the trunc_num variable.
Python math.sqrt() Method
The Python built-in function takes a non-negative numeric value (integer or floating-point) and returns the non-negative square root of the given number.
Code Example:
aW1wb3J0IG1hdGgKCnNxcnRfdmFsID0gbWF0aC5zcXJ0KDIuMjUpCnByaW50KCJTcXVhcmUgcm9vdCBvZiAyLjI1OiIsIHNxcnRfdmFsKQ==
Output:
Square root of 2.25: 1.5
In the code example, we use the math.sqrt() method to calculate the square root of 2.25. The method calculates and returns the square root storing it in the sqrt_val variable.
Angular Conversion Functions | Math Python Built-in Functions
This includes methods like math.radian() and math.degrees() for efficient inter-conversion of angular value among radian and degrees. These functions are particularly useful when dealing with trigonometric calculations, where angles are commonly expressed in both degrees and radians.
Python math.radians() Method
The Python built-in function maps an angle in degrees to the corresponding radians. Radians are a unit of measurement for angles, generally we use in mathematical and trigonometric calculations.
Code Example:
aW1wb3J0IG1hdGgKCnJhZGlhbnNfdmFsID0gbWF0aC5yYWRpYW5zKDQ1KQpwcmludCgiNDUgZGVncmVlcyBpbiByYWRpYW5zOiIsIGYne3JhZGlhbnNfdmFsOi40Zn0nKQ==
Output:
45 degrees in radians: 0.7854
In the code example, we convert 45 degrees to radians using the math.radians() method and print the result. This outputs the result 0.7854 (truncated to 4 decimal places) which is stored in the radians_val variable.
Python math.degrees() Method
The Python built-in function maps an angle in radians to the corresponding degrees. Degrees are a unit of measurement for angles that we commonly use in everyday contexts and graphical representations.
Code Example:
aW1wb3J0IG1hdGgKCmRlZ3JlZXNfdmFsID0gbWF0aC5kZWdyZWVzKDEpCnByaW50KCIxIHJhZGlhbiBpbiBkZWdyZWVzOiIsIGYne2RlZ3JlZXNfdmFsOi40Zn0nKQ==
Output:
1 radian in degrees: 57.2958
In the code example, we convert 1 radian to degrees using the math.degrees() method and print the result. This outputs the result 57.2958 (truncated to 4 decimal places), stored in the degrees_val variable.
Check out this amazing course to become the best version of the Python programmer you can be.
Exponential Functions | Math Python Built-in Functions
The Python built-in functions in this subcategory are particularly useful in scenarios where precise exponential calculations are required, especially when dealing with small values of x to avoid loss of precision. It includes methods like exp() and expm1() to calculate ex and ex-1, respectively.
Python math.exp() and math.expm1() Methods
The exp() and expm1() methods take a numeric value as exponent (say, x) and return the exponential value of the given number.
- The math.exp() returns ex while, math.expm1() returns ex-1.
- It is particularly useful in scenarios involving exponential growth or decay, such as financial modelling, population growth calculations, or scientific computations.
The basic Python code example below illustrates the use of these built-in functions to calculate the exponential value of 2.
Code Example:
aW1wb3J0IG1hdGgKCiNVc2luZyB0aGUgZXhwb25lbnRpYWwgZnVuY3Rpb25zCmV4cF92YWwgPSBtYXRoLmV4cCgyKQpleHBtMV92YWwgPSBtYXRoLmV4cG0xKDIpCgojUHJpbnRpbmcgdGhlIG91dGNvbWVzIG9mIHRoZSBvcGVyYXRpb25zCnByaW50KCJFeHBvbmVudGlhbCB2YWx1ZSBvZiAyOiIsIGV4cF92YWwpCnByaW50KCJFeHBvbmVudGlhbCB2YWx1ZSBvZiAyIG1pbnVzIDE6IiwgZXhwbTFfdmFsKQ==
Output:
Exponential value of 2: 7.38905609893065
Exponential value of 2 minus 1: 6.38905609893065
In the code example, we compute the e2 and e2-1 using the math.exp() and math.expm1() methods respectively and print the results. These functions return the exponential value of 2 with high precision.
Logarithmic Functions | Math Python Built-in Functions
This includes the log(), log10(), log2() and log1p() functions which provide various options for calculating logarithms in Python, catering to different use cases and requirements.
- Python math.log() Method : Returns the logarithm of a given number, say x to the given base. If base is not specified, it defaults to e (natural logarithm), i.e., loge(x).
- Python math.log10() Method: Returns the base-10 logarithm of x, i.e., log10(x).
- Python math.log1p() Method: Returns the natural logarithm of (1 + x), i.e., ln(1+x).
- Python math.log2() Method: Returns the base-2 logarithm of x, i.e., log2(x).
The example below illustrates the use of these built-in functions in Python.
Code Example:
aW1wb3J0IG1hdGgKCmxvZ192YWwgPSBtYXRoLmxvZygxMCkKbG9nMTBfdmFsID0gbWF0aC5sb2cxMCgxMDAwKQpsb2cxcF92YWwgPSBtYXRoLmxvZzFwKDAuNSkKbG9nMl92YWwgPSBtYXRoLmxvZzIoMTYpCgpwcmludCgiTmF0dXJhbCBsb2dhcml0aG0gb2YgMTA6IiwgbG9nX3ZhbCkKcHJpbnQoIkJhc2UtMTAgbG9nYXJpdGhtIG9mIDEwMDA6IiwgbG9nMTBfdmFsKQpwcmludCgiTmF0dXJhbCBsb2dhcml0aG0gb2YgKDEgKyAwLjUpOiIsIGxvZzFwX3ZhbCkKcHJpbnQoIkJhc2UtMiBsb2dhcml0aG0gb2YgMTY6IiwgbG9nMl92YWwp
Output:
Natural logarithm of 10: 2.302585092994046
Base-10 logarithm of 1000: 3.0
Natural logarithm of (1 + 0.5): 0.4054651081081644
Base-2 logarithm of 16: 4.0
In the code example we calculate the value of loge10 using the math.log() method, the value of log101000 using the math.log10() method, the value of loge(1+0.5) using the math.log1p() method, and the value of loge16 using the math.log2() method and print the results.
Trigonometric Functions | Math Python Built-in Functions
This includes functions like sin(), cos() and tan() to perform trigonometric computations in engineering and scientific applications where we analyse waveforms and periodic phenomena.
1. Python math.sin() Method: The Python built-in function from the math mdoule returns the sine of angle given in radians.
Code Example:
aW1wb3J0IG1hdGgKCnNpbl92YWwgPSBtYXRoLnNpbihtYXRoLnBpIC8gMikKcHJpbnQoIlNpbmUgb2Ygz4AvMiByYWRpYW5zOiIsIHNpbl92YWwp
Output:
Sine of π/2 radians: 1.0
In the code example, we calculate the sin of π/2 radians using the math.sin() method, passing π/2 as input, which yields 1.0 as output.
2. Python math.cos() Method: This built-in function returns the cosine of angle given in radians.
Code Example:
aW1wb3J0IG1hdGgKCmNvc192YWwgPSBtYXRoLmNvcyhtYXRoLnBpKQpwcmludCgiQ29zaW5lIG9mIM+AIHJhZGlhbnM6IiwgY29zX3ZhbCk=
Output:
Cosine of π radians: -1.0
In the example, we calculate the cosine of π radians using the math.cos() method, passing π as input, which yields -1.0 as cosine of π.
3. Python math.tan() Method: This Python built-in function returns the cosine of angle given in radians.
Code Example:
aW1wb3J0IG1hdGgKCnRhbl92YWwgPSBtYXRoLnRhbihtYXRoLnBpIC8gMykKcHJpbnQoIlRhbmdlbnQgb2Ygz4AvMyByYWRpYW5zOiIsIGYne3Rhbl92YWw6LjRmfScp
Output:
Tangent of π/3 radians: 1.7321
In the example, we calculate the tangent of π/3 radians using math.tan() method, passing π/3 as input, which yields 1.7321 as output.
Inverse Trigonometric Functions | Math Python Built-in Functions
The Python built-in functions in this subcategory have their use-case in engineering and scientific applications for calculating the angle in radians or degrees. It includes functions like:
- Python math.asin() Function: Calculates the inverse sine value of a given number.
- Python math.acos() Function: Calculates the inverse cosine value of a given number.
- Python math.atan() Function: Calculates the inverse tangent value of a given number.
The Python example code below illustrates the use of all these functions.
Code Example:
aW1wb3J0IG1hdGgKCiNVc2luZyBpbnZlcnNlIHRyaWcgZnVuY3Rpb25zIGluc2lkZSBwcmludCBzdGF0ZW1lbnRzIHRvIGNhbGN1bGF0ZSBhbmQgcHJpbnQgdGhlIHZhbHVlcwpwcmludCgiQXJjIHNpbmUgb2YgMToiLG1hdGguYXNpbigxKSkKcHJpbnQoIkFyYyBjb3NpbmUgb2YgLTE6IixtYXRoLmFjb3MoLTEpKQpwcmludCgiQXJjIHRhbmdlbnQgb2YgMToiLG1hdGguYXRhbigxKSk=
Output
Arc sine of 1: 1.5707963267948966
Arc cosine of -1: 3.141592653589793
Arc tangent of 1: 0.7853981633974483
In the code example, we print the inverse sine, cosine, and tangent values of the 1, -1, and 1, respectively. Which yields the respective angle value as output (in radians).
Hyperbolic Functions | Math Python Built-in Functions
These Python built-in functions are somewhat similar to trigonometric functions, but with a key difference. They help in hyperbolic calculation in scientific applications.
- Python math.sinh() Method: Returns the hyperbolic sine of a given number.
- Python math.cosh() Method: Returns the hyperbolic cosine of a given number.
- Python math.tanh() Method: Returns the hyperbolic tangent of a given number.
- Python math.asinh() Method: Returns the inverse hyperbolic sine (area hyperbolic sine) of a given number.
- Python math.acosh() Method: Returns the inverse hyperbolic cosine (area hyperbolic cosine) of a given number.
- Python math.atanh() Method: Returns the inverse hyperbolic tangent (area hyperbolic tangent) of a given number.
Code Example:
aW1wb3J0IG1hdGgKCnByaW50KCJIeXBlcmJvbGljIHNpbmUgb2YgMToiLCBtYXRoLnNpbmgoMSkpCnByaW50KCJIeXBlcmJvbGljIGNvc2luZSBvZiAxOiIsIG1hdGguY29zaCgxKSkKcHJpbnQoIkh5cGVyYm9saWMgdGFuZ2VudCBvZiAxOiIsIG1hdGgudGFuaCgxKSkKcHJpbnQoIkludmVyc2UgaHlwZXJib2xpYyBzaW5lIG9mIDE6IiwgbWF0aC5hc2luaCgxKSkKcHJpbnQoIkludmVyc2UgaHlwZXJib2xpYyBjb3NpbmUgb2YgMToiLCBtYXRoLmFjb3NoKDEpKQpwcmludCgiSW52ZXJzZSBoeXBlcmJvbGljIHRhbmdlbnQgb2YgMC41OiIsIG1hdGguYXRhbmgoMC41KSk=
Output:
Hyperbolic sine of 1: 1.1752011936438014
Hyperbolic cosine of 1: 1.5430806348152437
Hyperbolic tangent of 1: 0.7615941559557649
Inverse hyperbolic sine of 1: 0.881373587019543
Inverse hyperbolic cosine of 1: 0.0
Inverse hyperbolic tangent of 0.5: 0.5493061443340548
In the code example, we use the hyperbolic sine, cosine, and tangent functions, as well as inverse hyperbolic sine, cosine, and tangent functions, to calculate their respective hyperbolic values.
Python Built-In Functions For Strings
In Python, there are several built-in functions for handling strings. They help us perform various manipulations, calculations, conversions, etc. on Python strings. Some examples are len() and str(), as well as functions from the string module like str.upper(), str.lower(), str.join(), str.split(), str.capitalize(), etc.
In the table below, we have listed some of the most commonly used Python built-in functions with respect to string, along with a description and syntax.
Function/Method Name |
Syntax |
Description |
len() |
len(s) |
Returns the length of the string or sequence s. |
str() |
str(object) |
Returns a string representation of an object. |
upper() |
s.upper() |
Returns a copy of the string s converted to uppercase. |
lower() |
s.lower() |
Returns a copy of the string s converted to lowercase. |
split() |
s.split(del [, n]) |
Returns a list of words in the string s separated by the delimiter del. Optional value n, number of times to split the string. |
join() |
del.join(itr) |
Joins all the elements of an iterable itr into a single string with del as the delimiter. |
capitalize() |
s.capitalize() |
Capitalise the string s (convert first character to uppercase) |
center() |
s.center(len [,char]) |
Center align the string s, with specified length len and optional filler character char (space is default) |
count() |
s.count(value [, start, end]) |
Count the number of times a specified value occurs in a string s with optional start and end position specificity. |
endswith() |
s.endswith(value [, start, end]) |
Returns true if the string s ends with the specified value. Optional start and end positions of string. |
find() |
s.find(value [, start, end]) |
Searches the string s for a specified value and returns the position of where it was found. Optional start and end positions of string. |
isalnum() |
s.isalnum() |
If all characters in the string s are alphanumeric, it returns true, otherwise false. |
isalpha() |
s.isalpha() |
If all characters in the string s are in the alphabet, it returns true, otherwise false. |
isascii() |
s.isascii() |
If all characters in the string s are ascii characters, it returns true, otherwise false. |
isdecimal() |
s.isdecimal() |
If all characters in the string s are decimals, it returns true, otherwise false. |
isdigit() |
s.isdigit() |
If all characters in the string s are digits, it returns true, otherwise false. |
islower() |
s.islower() |
If all characters in the string s are lower case, it returns true, otherwise false. |
isnumeric() |
s.isnumeric() |
If all characters in the string s are numeric, it returns true, otherwise false. |
isprintable() |
s.isprintable() |
If all characters in the string s are printable, it returns true, otherwise false. |
isspace() |
s.isspace() |
If all characters in the string s are whitespaces, it returns true, otherwise false. |
isupper() |
s.isupper() |
If all characters in the string s are upper case, it returns true, otherwise false. |
ljust() |
s.ljust(len [, char]) |
Left align the string s with specified length len of final string. Optional filler character char. |
lstrip() |
s.lstrip([char]) |
Removes leading spaces of the string s. Optional characters char to trim. |
replace() |
s.replace(oldvalue, newvalue [, count]) |
Returns a string where a specified newvalue is replaced with a specified oldvalue with optional number of occurrences to replace. |
startswith() |
s.startswith(value [, start, end]) |
If the string s starts with the specified value, it returns true. Optional start and end position of the search string. |
strip() |
s.strip([chars]) |
Returns a trimmed version of the string s. Optional the characters chars to trim. |
swapcase() |
s.swapcase() |
Returns a string with all lower case becomes upper case and vice versa. |
title() |
s.title() |
Converts the first character of each word to uppercase in the string s. |
translate() |
s.translate() |
Returns a translated version of the string s |
zfill() |
s.zfill(len) |
Fills the 0’s at the beginning of the string s, until it reaches the specified length len. |
Now that you have an overview of all relevant Python built-in functions for strings, let’s discuss some key function in more detail.
Level up your coding skills with the 100-Day Coding Sprint at Unstop and claim the bragging rights, now!
Python len() function
The Python built-in function is used to determine the length of a sequence, such as a string, list, or tuple. It commonly returns the number of characters present in a string or how many elements are in a list or tuple.
Code Example:
I0NyZWF0aW5nIGEgc3RyaW5nClMgPSAiSGVsbG8sIFdvcmxkISIKCiNVc2luZyBsZW4oKSBmdW5jdGlvbiB0byBjYWxjdWxhdGUgdGhlIGxlbmd0aCBvZiBhIHN0cmluZwpsZW5ndGggPSBsZW4oUykKcHJpbnQoIkxlbmd0aCBvZiB0aGUgc3RyaW5nOiIsIGxlbmd0aCk=
Output:
Length of the string: 13
In the example above, we calculate the length of a string using the len() function by passing the string S to the len() function. The function calculates and returns the length of the string as output, stored in the variable length.
Python str() function
The Python built-in function converts an object to a string representation. This is useful when you need to ensure that an object is in string format for concatenation, printing, or other string operations. It takes an object as input and returns the string version of the object.
Code Example:
I0NyZWF0aW5nIGFuIGludGVnZXIgdmFyaWFibGUKbnVtYmVyID0gMTIzCgojQ29udmVydGluZyB0aGUgdmFyaWFibGUgdG8gc3RyaW5nCnN0cmluZ19udW1iZXIgPSBzdHIobnVtYmVyKQpwcmludCgiU3RyaW5nIHJlcHJlc2VudGF0aW9uOiIsIHN0cmluZ19udW1iZXIp
Output:
String representation: 123
In the example above, we use the str() function to convert an integer to a string. We pass the integer 123 to the str() function, which returns its string form as output.
Python upper() Method
The Python built-in function converts all characters in a string to uppercase. This is useful when you need to standardize or normalize text to a single case for comparison, storage, or display. It returns a new string with all characters converted to uppercase.
Code Example:
I0NyZWF0aW5nIGEgc3RyaW5nIGFsbCBpbiBsb3dlcmNhc2UKdGV4dCA9ICJoZWxsbyIKCiNDb252ZXJ0aW5nIGl0IHRvIHVwcGVyIGNhc2UKdXBwZXJfdGV4dCA9IHRleHQudXBwZXIoKQpwcmludCgiVXBwZXJjYXNlIHRleHQ6IiwgdXBwZXJfdGV4dCk=
Output:
Uppercase text: HELLO
In the code example, we call the upper() method by passing the string text as input. The function converts the string “hello” to uppercase and stores the output in the upper_text variable.
Python lower() Method
The Python built-in function converts all characters in a string to lowercase. This is useful when you need to standardize or normalize text to a single case for comparison, storage, or display. It returns a new string with all characters converted to lowercase.
Code Example:
I0NyZWF0aW5nIGEgc3RyaW5nIGFsbCBpbiB1cHBlcmNhc2UKdGV4dCA9ICJIRUxMTyIKCiNDb252ZXJ0aW5nIGl0IHRvIGxvd2VyIGNhc2UKbG93ZXJfdGV4dCA9IHRleHQubG93ZXIoKQpwcmludCgiTG93ZXJjYXNlIHRleHQ6IiwgbG93ZXJfdGV4dCk=
Output:
Lowercase text: hello
Here, we call the lower() method to the string “HELLO”, which converts the complete string to lowercase.
Python split() Method
The Python built-in function converts a string into a list of substrings based on a specified separator. It is useful for parsing strings, extracting data, or breaking down text into manageable parts. It returns a list of substrings.
Code Example:
I0NyZWF0aW5nIGEgc3RyaW5nCnRleHQgPSAiSGVsbG8gV29ybGQiCgojU3BsaXR0aW5nIHRoZSBzdHJpbmcgaW50byBhIGxpc3Qgb2Ygd29yZHMKd29yZHMgPSB0ZXh0LnNwbGl0KCkKcHJpbnQoIkxpc3Qgb2Ygd29yZHM6Iiwgd29yZHMp
Output:
List of words: ['Hello', 'World']
In the code example, we call the split() method by passing the string “Hello World” as an argument. It returns the list of substrings separated by whitespace.
Python join() Method
The Python built-in function concatenates the elements of an iterable (such as a list or tuple) into a single string, with each element separated by a specified delimiter. It is useful for constructing strings from collections of substrings.
Code Example:
I0NyZWF0aW5nIGEgbGlzdAp3b3JkcyA9IFsiSGVsbG8iLCAiV29ybGQiXQoKI0NvbnZlcnRpbmcgaXQgdG8gYSBzdHJpbmcvIGNvbmNhdGVuYXRpbmcgdGhlIGVsZW1lbnRzCnNlbnRlbmNlID0gIiAiLmpvaW4od29yZHMpCnByaW50KCJKb2luZWQgc2VudGVuY2U6Iiwgc2VudGVuY2Up
Output:
Joined sentence: Hello World
In the example, we join a list of words with a space using the join() method. We pass the list as an iterable and space(“ ”) as a delimiter.
Python capitalize() Method
The Python built-in function converts only the first character of a string to uppercase and the remaining characters to lowercase. It is useful for formatting text to ensure proper capitalization at the start of sentences or titles.
Code Example:
I0NyZWF0aW5nIGEgc3RyaW5nCnRleHQgPSAiaGVsbG8gd29ybGQiCgojQ29udmVydGluZyB0aGUgZmlyc3QgY2hhcmFjdGVyIGZyb20gbG93ZXIgdG8gdXBwZXJjYXNlCmNhcGl0YWxpemVkX3RleHQgPSB0ZXh0LmNhcGl0YWxpemUoKQpwcmludCgiQ2FwaXRhbGl6ZWQgdGV4dDoiLCBjYXBpdGFsaXplZF90ZXh0KQ==
Output:
Capitalized text: Hello world
In the example above, we capitalise a lowercase string using the capitalize() method. We call the capitalize() method to the string named text (“hello world”). It converts the string and returns the outcome to the capitalized_text variable.
Python count() Method
The Python built-in function converts the number of occurrences of a substring within a string. Generally, we use it to determine the frequency of a substring in a text, such as counting words, characters, or patterns.
Code Example:
dGV4dCA9ICJiYW5hbmEiCnN1YnN0cmluZyA9ICJhIgoKY291bnQgPSB0ZXh0LmNvdW50KHN1YnN0cmluZykKcHJpbnQoIkNvdW50IG9mICdhJyBpbiAnYmFuYW5hJzoiLCBjb3VudCk=
Output:
Count of 'a' in 'banana': 3
In the code example, we count the occurrences of the substring "a" in the string "banana". For this, we call the count() method by passing the substring (“a”) as an argument and print the same stored in the count variable.
Python find() Method
The Python built-in function locates the position of a substring within a string. It returns the index of the first occurrence of the substring, or -1 if the substring is not found. Generally, we use it for searching substrings within a text.
Code Example:
dGV4dCA9ICJoZWxsbyB3b3JsZCIKc3Vic3RyaW5nID0gIndvcmxkIgoKaW5kZXggPSB0ZXh0LmZpbmQoc3Vic3RyaW5nKQpwcmludCgiSW5kZXggb2YgJ3dvcmxkJzoiLCBpbmRleCk=
Output:
Index of 'world': 6
In the code example, we find the position of the substring "world" in the string "hello world" using the find() method.
Python replace() Method
The Python built-in function helps replace occurrences of a specified substring with another substring.
- This method accepts the substring to be replaced and the substring to replace the old one and returns a new string with the specified replacements.
- Generally, we use it to modify text by replacing words, phrases, or characters.
Code Example:
dGV4dCA9ICJoZWxsbyB3b3JsZCIKCnJlcGxhY2VkX3RleHQgPSB0ZXh0LnJlcGxhY2UoIndvcmxkIiwgIlB5dGhvbiIpCnByaW50KCJSZXBsYWNlZCB0ZXh0OiIsIHJlcGxhY2VkX3RleHQp
Output:
Replaced text: hello Python
In the code example, we replace all occurrences of the substring "world" with "Python" using the replace() method.
Input/ Output Built-In Functions In Python
Input/output (I/O) operations are essential in any programming language for interacting with the user and handling file operations. Python has built-in functions for performing various input/output tasks efficiently, such as input(), open(), print(), etc. We have listed these Python built-in functions in the table below, along with the syntax and a description.
Function Name |
Syntax |
Description |
input() |
input(prompt) |
Reads a line from input and returns it as a string. |
print() |
print(value, ...) |
Prints the given values to the standard output. |
open() |
open(file, mode) |
Opens a file and returns a file object. |
read() |
file.read(size=-1) |
Reads and returns a string from the file. |
write() |
file.write(string) |
Writes the string string to the file. |
Now, let’s discuss these Python built-in functions in slightly more detail and with a code example.
Python input() Function
The Python built-in function is used to take input from the user.
- It displays an optional prompt message and waits for the user to type some input and press Enter.
- We generally use this for interactive programs where user input is needed.
Code Example:
I1VzaW5nIHRoZSBpbnB1dCgpIGZ1bmN0aW9uIHRvIHJlYWQgaW5wdXQgZnJvbSB1c2VyCnVzZXJfaW5wdXQgPSBpbnB1dCgpCgojUHJpbnRpbmcgdGhlIGlucHV0IHByb3ZpZGVkCnByaW50KCJZb3UgZW50ZXJlZDoiLCB1c2VyX2lucHV0KQ==
Input:
Unstop
Output:
You entered: Unstop
Here, we use the input() function to get input from the user without a prompt. Assuming that the user enters the string/ word Unstop, we then print the same to show the functionality of the input() function.
Python print() Function
The Python built-in function is used to output text or other data to the console or another output stream.
- We generally use this function to display information to the user, debug, and log.
- This function, by default, contains a newline character, which means that if more than one print statement is used one after another, it will print the subsequent output in the next/ new line.
You have already seen this function in all the examples above, but let’s look at a short example.
Code Example:
I1VzaW5nIHRoZSBwcmludCBsaWJyYXJ5IGZ1bmN0aW9uIHRvIG91dHB1dCBhIHN0cmluZwpwcmludCgiSGVsbG8sIFdvcmxkISIp
Output:
Hello, World!
Python open() Function
The Python built-in function is used to open a file and return a corresponding file object.
- It is essential to use this function whenever we want to perform any operations on a file, i.e., to read from and write to files.
- The function is generally used with a mode to specify the purpose for opening the file, for example “r” for reading, “w” for writing, and so on.
Code Example:
I1VzaW5nIG9wZW4oKSB0byBhY2Nlc3MgYSBmaWxlIG5hbWVzIGV4YW1wbGUudHh0IGluIHRoZSByZWFkIG1vZGUKZmlsZSA9IG9wZW4oImV4YW1wbGUudHh0IiwgInIiKQoKI09uY2UgdGhlIGZpbGUgaXMgb3BlbiB3ZSBjYW4gcGVyZm9ybSBvdGhlciBvcGVyYXRpb25zIG9uIGl0LCBsaWtlIHJlYWQgYXMgZG9uZSBiZWxvdwpjb250ZW50ID0gZmlsZS5yZWFkKCkKCiNQcmludGluZyB0aGUgY29udGVudCB3ZSByZWFkIGZyb20gdGhlIGZpbGUKcHJpbnQoIkZpbGUgY29udGVudDoiLCBjb250ZW50KQoKI0Nsb3NpbmcgdGhlIGZpbGUgaXMgZXNzZW50aWFsIHRvIGF2b2lkIGFueSB1bmV4cGVjdGVkIGJlaGF2aW9yCmZpbGUuY2xvc2UoKQ==
Output:
File content: Unstop is best!
In the code example above, we open a file for reading using the open() function, read its content, and print it.
Note: This will throw an error as the directory or file should be available and should be accessible. Since, it was just a demonstration, “example.txt” was a local file created for it, and its content was printed above.
Python read() Method
The Python built-in functio method is used to read the contents of a file. It can read a specified number of bytes or the entire file.
Code Example:
ZmlsZSA9IG9wZW4oInVuc3RvcC50eHQiLCAiciIpCgojVXNpbmcgdGhlIHJlYWQoKSBmdW5jdGlvbiB0byByZWFkIGNvbnRlbnQgZnJvbSBhIGZpbGUKY29udGVudCA9IGZpbGUucmVhZCgpCgojUHJpbnRpbmcgdGhlIGNvbnRlbnQgYW5kIHRoZW4gY2xvc2luZyBpdApwcmludCgiRmlsZSBjb250ZW50OiIsIGNvbnRlbnQpCmZpbGUuY2xvc2UoKQ==
Output:
File content: Unstop is best!
In the code example above, we read the entire content of a file using the read() method.
Note: This will throw an error as the directory or file should be available and should be accessible. SInce, it was just a demonstration, “example.txt” was a local file created for it and its content was read and printed above.
Python write() Function
The Python built-in function is used to write a string to a file. It is commonly used for creating or modifying files. As you will see in the example below, when opening the file for writing, we must specify that in the mode when opening the file.
Code Example:
I09wZW5pbmcgdGhlIGZpbGUgaW4gd3JpdGUgbW9kZQpmaWxlID0gb3BlbigiZXhhbXBsZS50eHQiLCAidyIpCgojV3JpdGluZyBjb250ZW50IHRvIHRoZSBmaWxlIHVzaW5nIHRoZSB3cml0ZSgpIGZ1bmN0aW9uCmNoYXJhY3RlcnNfd3JpdHRlbiA9IGZpbGUud3JpdGUoIkhlbGxvLCBXb3JsZCEiKQoKI1ByaW50aW5nIHRoZSBudW1iZXIgb2YgY2hhcnJhY3RlcnMgYmVmb3JlIGNsb3NpbmcgdGhlIGZpbGUKcHJpbnQoIkNoYXJhY3RlcnMgd3JpdHRlbjoiLCBjaGFyYWN0ZXJzX3dyaXR0ZW4pCmZpbGUuY2xvc2UoKQ==
Output:
Characters written: 13
In the code example above, we use the write() method to write "Hello, World!" to a file and print the number of characters written.
List & Tuple Python Built-In Functions
In Python there are a number of built-in functions to let us manipulate and handle the lists and tuples easily and efficiently. Lists and tuples are versatile and immutable data structures in Python that play a crucial role in data handling. Listed in the table below are all relevant built-in functions in Python, used in conjunction with lists and tuples.
Function Name |
Syntax |
Description |
len() |
len(s) |
Returns the length of the list or tuple s. |
sorted() |
sorted(iterable) |
Returns a new sorted list from the elements of the iterable. |
append() |
list.append(x) |
Appends an item x to the end of the list. |
extend() |
list.extend(iterable) |
Extends the list by appending elements from the iterable. |
index() |
list.index(x) |
Returns the index of the first occurrence of item x in the list. |
Python len() Function
The Python built-in function is used to determine the length of a sequence, such as a string, list, or tuple. It takes a sequence as input and returns the number of characters present in a string or how many elements are in a list or tuple.
Code Example:
I0NyZWF0aW5nIGEgbGlzdApteV9saXN0ID0gWzEsIDIsIDMsIDQsIDVdCgojVXNpbmcgdGhlIGxlbigpIGZ1bmN0aW9uIHRvIGZpbmQgdGhlIG51bWJlciBvZiBjaGFyYWN0ZXJzCmxlbmd0aCA9IGxlbihteV9saXN0KQpwcmludCgiTGVuZ3RoIG9mIHRoZSBsaXN0OiIsIGxlbmd0aCk=
Output:
Length of the list: 5
In the example, we calculate the length of a list using the len() function. We pass the list my_list to the len() function, which returns the length of the list as output.
Python sorted() Function
The sorted() function is used to return a sorted list from the elements of any iterable.
- By default the function sorts the elments in an ascending order, however you can specify a sequence by adding an optional argument key.
- It is often used to sort lists, tuples, or any iterable based on a specific key or in reverse order.
Code Example:
I0NyZWF0aW5nIGEgbGlzdApudW1iZXJzID0gWzUsIDIsIDksIDEsIDUsIDZdCgojU29ydGluZyB0aGUgbGlzdCBpbiBkZWZhdWx0IG9yZGVyCnNvcnRlZF9udW1iZXJzID0gc29ydGVkKG51bWJlcnMpCnByaW50KCJTb3J0ZWQgbGlzdDoiLCBzb3J0ZWRfbnVtYmVycyk=
Output:
Sorted list: [1, 2, 5, 5, 6, 9]
In the code example above, we call the sorted() function to sort a list of six integers, called numbers, in the default ascending order.
Python append() Method
The Python built-in function is used to add a single item to the end of a list. Using it, conveniently, we can add new elements to an existing list.
Code Example:
I0NyZWF0aW5nIGEgbGlzdApudW1iZXJzID0gWzEsIDIsIDMsIDRdCgojVXNpbmcgYXBwZW5kKCkgdG8gYWRkIGFuIGVsZW1lbnQKbnVtYmVycy5hcHBlbmQoNSkKcHJpbnQoIlVwZGF0ZWQgbGlzdDoiLCBudW1iZXJzKQ==
Output:
Updated list: [1, 2, 3, 4, 5]
In the code example, we add the number 5 to the end of a list of four integer values called numbers. The append() method modifies the original list in place and hence does not return a new list.
File Handling Python Built-In Functions
File handling is a crucial part of programming. It allows you to read and write to a file on the disk. Python offers many built-in functions for performing various file operations easily and efficiently. We have listed these Python built-in functions in the table below with the syntax and description.
Function Name |
Syntax |
Description |
close() |
file.close() |
Closes the file. |
flush() |
file.flush() |
Flushes the internal buffer. |
seek() |
file.seek(offset[, whence]) |
Moves the file pointer to a specified position. |
tell() |
file.tell() |
Returns the current file position. |
truncate() |
file.truncate(size=None) |
Resizes the file to a specified size |
As you can see in the syntax column above, we have used the term “file” followed by a dot operator, before all the function names. This is common practice to indicate that we are using Python built-in function from the file object or for the purpose of carrying out file handling operations.
Python close() Method
The Python built-in function is used to close an open file. It is important to close a file after it has been used to free up system resources and to ensure that all buffered data is written to the file. Failing to close a file can lead to data loss or corruption.
Code Example:
I09wZW5pbmcgYSBmaWxlIGZvciB3cml0aW5nCmZpbGUgPSBvcGVuKCJleGFtcGxlLnR4dCIsICJ3IikKCiNVc2luZyB3cml0ZSgpIGZyb20gZmlsZSBvYmplY3QgdG8gYWRkIGNvbnRlbnQgdG8gdGhlIGZpbGUKZmlsZS53cml0ZSgiSGVsbG8sIFdvcmxkISIpCmZpbGUuY2xvc2UoKQ==
Output: In example.txt
Hello, World!
In the code example above, we open a file for writing and use the write() function to write the string/ content "Hello, World!" to it.
Python tell() Method
The Python built-in function is used to determine the current position of the file pointer within the file. It returns the byte offset from the beginning of the file, which allows you to keep track of where you are within the file.
Code Example:
I09wZW5pbmcgYSBmaWxlIGFuZCB3cml0aW5nIGEgdGV4dCB0byBpdApmaWxlID0gb3BlbigiZXhhbXBsZS50eHQiLCAidyIpCmZpbGUud3JpdGUoIkhlbGxvLCBXb3JsZCEiKQoKI1VzaW5nIHRlbGwoKSB0byBjaGVjayB0aGUgcG9pc2l0b24gb2YgdGhlIGN1cnNvciBhZnRlciB3cml0aW5nCnBvc2l0aW9uID0gZmlsZS50ZWxsKCkKcHJpbnQoIkZpbGUgcG9pbnRlciBwb3NpdGlvbjoiLCBwb3NpdGlvbikKZmlsZS5jbG9zZSgp
Output:
File pointer position: 13
In the code example, we open a file for writing and write "Hello, World!" to it. Then, we use the tell() function to the position of the file pointer, which should be at the end of the string. As shown in the output, this is 13, equivalent to the length of the text written.
Python truncate() Method
The Python built-in function is used to resize the file to a specified size.
- If the specified size is larger than the current size, the file is extended with null bytes.
- If the size is smaller, the file is truncated and any excess data is lost.
- It comes in handy when we want to control the size of a file.
Code Example:
I09wZW5pbmcgYSBmaWxlIGFuZCB3cml0aW5nIGEgdGV4dCB0aGUgbGVuZ3RoIG9mIDEzIGNoYXJhY3RlcnMgdG8gaXQKZmlsZSA9IG9wZW4oImV4YW1wbGUudHh0IiwgIncrIikKZmlsZS53cml0ZSgiSGVsbG8sIFdvcmxkISIpCgojVXNpbmcgdHJ1bmNhdGUgdG8gcmVzaXplIHRoZSBjb250ZW50IHRvIDUgYnl0ZXMKZmlsZS50cnVuY2F0ZSg1KQoKIyBNb3ZpbmcgdGhlIGN1cnNvciB0byB0aGUgc3RhcnQgb2YgdGhlIGZpbGUgYW5kIHJlYWRpbmcgY29udGVudCB0byBjaGVjayBpZiB0cnVuY2F0ZWQKZmlsZS5zZWVrKDApCmNvbnRlbnQgPSBmaWxlLnJlYWQoKQpwcmludCgiVHJ1bmNhdGVkIGNvbnRlbnQ6IiwgY29udGVudCkKZmlsZS5jbG9zZSgp
Output:
Truncated content: Hello
In the code example, we open a file for writing and reading and write "Hello, World!" to it. Then, we use the truncate() function to truncate the file to 5 bytes. We then read the truncated content, which results in "Hello".
Python Built-In Functions For Dictionary
Dictionaries are powerful data structures in Python that hold and retrieve data in the form of key-value pairs. Python provides many built-in functions for working with dictionaries, enabling developers to manipulate and process data effectively.
Listed in the table below are the commonly used Python built-in functions for working with dictionaries, with a description and the syntax.
Function Name |
Syntax |
Description |
len() |
len(d) |
Returns the number of items in the dictionary d. |
keys() |
d.keys() |
Returns a view of the dictionary's keys. |
values() |
d.values() |
Returns a view of the dictionary's values. |
items() |
d.items() |
Returns a view of the dictionary's key-value pairs. |
get() |
d.get(key[, default]) |
Returns the value for key if key is in the dictionary, else default. |
Like in other categories, here, we also use a character and dot operator before the function name. Here, the character “d” indicates that the function belongs to the dict class and that we are working with dictionary operations.
Python len() Function
The Python built-in function has already been featured in some other sections. In the case of dictionaries, it is used to determine the number of key-value pairs in a dictionary. We generally use it to check the size of the dictionary, which can be helpful in loops, conditions, or simply to understand the amount of data stored.
Code Example:
I0NyZWF0aW5nIGEgZGljdGlvbmFyeSByZXByZXNlbnRpbmcgc3R1ZGVudHMgYW5kIHRoZWlyIGFnZXMKc3R1ZGVudF9hZ2VzID0geyJTcmlzaCI6IDIxLCAiU2hyZWV5YSI6IDIyLCAiU2hpdmkiOiAyM30KCiNVc2luZyB0aGUgbGVuKCkgZnVuY3Rpb24gdG8gZmluZCB0aGUgbnVtYmVyIG9mIHBhaXJzIGluIHRoZSBkaWN0aW9uYXJ5Cmxlbmd0aCA9IGxlbihzdHVkZW50X2FnZXMpCnByaW50KCJOdW1iZXIgb2Ygc3R1ZGVudHM6IiwgbGVuZ3RoKQ==
Output:
Number of students: 3
In the code example, we create a dictionary with three key-value paris and then use the len() function on it to find the number of these value pairs.
Python keys() Method
The Python built-in function is used to obtain all the keys in a dictionary. This can be useful when you need to iterate over keys or check if a certain key exists in the dictionary.
Code Example:
c3R1ZGVudF9hZ2VzID0geyJTcmlzaCI6IDIxLCAiU2hyZWV5YSI6IDIyLCAiU2hpdmkiOiAyM30KCiNFeHRyYWN0aW5nIGp1c3QgdGhlIGtleXMgZnJvbSBwYWlycwprZXlzID0gc3R1ZGVudF9hZ2VzLmtleXMoKQoKcHJpbnQoIktleXM6Iiwga2V5cyk=
Output:
Keys: dict_keys([Srish, Shreeya, Shivi])
Just like in the previous example, we have the same dictionary on which we use the keys() function to get all the keys. The function returns the keys in the form of a special view object.
Python values() Method
In contrast to the keys() function, this Python built-in function is used to obtain all the values in a dictionary. This can be useful when you need to process or analyze the values stored in the dictionary.
Code Example:
c3R1ZGVudF9hZ2VzID0geyJTcmlzaCI6IDIxLCAiU2hyZWV5YSI6IDIyLCAiU2hpdmkiOiAyM30KCiNVc2luZyB2YWx1ZXMoKSB0byBnZXQgdGhlIHZhbHVlIGZyb20gdGhlIHBhaXJzCnZhbHVlcyA9IHN0dWRlbnRfYWdlcy52YWx1ZXMoKQpwcmludCgiVmFsdWVzOiIsIHZhbHVlcyk=
Output:
Values: dict_values([21, 22, 23])
In the code example, we use the same list as before and use the values() method to get all the values returned as a special view object.
Type Conversion Python Built-In Functions
Type conversion is the process of converting the data type of one variable or object to another. It is important for various operations, including input/output handling and performing mathematical calculations. Python provides many built-in functions for type conversion, as shown in the table below.
Function Name |
Syntax |
Description |
int() |
int(x) |
Converts x to an integer. |
float() |
float(x) |
Converts x to a floating-point number. |
str() |
str(object) |
Converts object to a string. |
list() |
list(iterable) |
Converts iterable to a list. |
tuple() |
tuple(iterable) |
Converts iterable to a tuple. |
bin() |
bin(x) |
Converts an integer, x to a binary string. |
oct() |
oct(x) |
Converts an integer, x to an octal string. |
hex() |
hex(x) |
Converts an integer, x to a hexadecimal string. |
bool() |
bool(argument) |
Converts an argument to a boolean value. |
chr() |
chr(x) |
Returns the character for the integer unicode number, x. |
ord() |
ord(c) |
Returns the integer unicode value of a character c. |
bytearray() |
bytearray() |
It creates an object of bytearray class. |
bytes() |
bytes() |
It creates an immutable object of the bytes class. |
Python int() Function
The Python built-in function is used to convert a number or a string to an integer. It is useful for type conversion when you need to perform arithmetic operations or store numbers in integer format.
Code Example:
c3RyaW5nX251bWJlciA9ICIxMjMiCgppbnRlZ2VyX251bWJlciA9IGludChzdHJpbmdfbnVtYmVyKQpwcmludCgiSW50ZWdlciB2YWx1ZToiLCBpbnRlZ2VyX251bWJlcik=
Output:
Integer value: 123
Here, we convert a string (“123”) to an integer (123) using the int() function and then print it.
Python float() Function
The Python built-in function is used to convert a number or a string to a floating-point number. Generally, we use it for type conversion when you need to perform arithmetic operations involving decimal points.
Code Example:
c3RyaW5nX251bWJlciA9ICIxMjMuNDUiCgpmbG9hdF9udW1iZXIgPSBmbG9hdChzdHJpbmdfbnVtYmVyKQpwcmludCgiRmxvYXQgdmFsdWU6IiwgZmxvYXRfbnVtYmVyKQ==
Output:
Float value: 123.45
Here, we convert a string (“123.45”) to a floating-point value (123.45) using the float() function and print it.
Python str() Function
The Python built-in function is used to convert an object to a string representation. Generally, we put it to use when you need to ensure that an object is in string format for concatenation, printing, or other string operations.
Code Example:
bnVtYmVyID0gMTIzCgpzdHJpbmdfbnVtYmVyID0gc3RyKG51bWJlcikKcHJpbnQoIlN0cmluZyByZXByZXNlbnRhdGlvbjoiLCBzdHJpbmdfbnVtYmVyKQ==
Output:
String representation: 123
Here, we convert an integer (123) to a string (“123”) using the str() function and print it.
Python list() Function
The Python built-in function is used to convert an iterable to a list. We put it to use for type conversion when you need to manipulate or access the elements of the iterable in list format.
Code Example:
bXlfdHVwbGUgPSAoMSwgMiwgMykKCmxpc3RfZnJvbV90dXBsZSA9IGxpc3QobXlfdHVwbGUpCnByaW50KCJMaXN0IGZyb20gdHVwbGU6IiwgbGlzdF9mcm9tX3R1cGxlKQ==
Output:
List from tuple: [1, 2, 3]
Here, we convert a tuple (my_tuple) to a list of characters (list_from_tuple) using the list() function.
Python tuple() Function
The Python built-in function is used to convert an iterable to a tuple. It is useful for type conversion when you need to ensure that the elements are in an immutable sequence.
Code Example:
bXlfbGlzdCA9IFsxLCAyLCAzXQoKdHVwbGVfZnJvbV9saXN0ID0gdHVwbGUobXlfbGlzdCkKcHJpbnQoIlR1cGxlIGZyb20gbGlzdDoiLCB0dXBsZV9mcm9tX2xpc3Qp
Output:
Tuple from list: (1, 2, 3)
Here, we convert a list (my_list) to a tuple (tuple_from_list) using the tuple() function.
Basic Python Built-In Functions
In this section, we'll cover some of the fundamental built-in functions, their use-cases, the module they belong to (if applicable), and provide example snippets for better understanding. Basic Python built-in functions as listed in the table below, have a wide range of functionalities, from mathematical operations to type conversion and more.
Function Name |
Syntax |
Description |
type() |
type(object) |
Returns the type of an object. |
help() |
help([object]) |
Invokes the built-in help system. |
dir() |
dir([object]) |
Returns a list of valid attributes for the object. |
id() |
id(object) |
Returns the identity of an object. |
callable() |
callable(object) |
Checks if the object appears callable. |
Python type() Function
The Python built-in function is used to determine the type of an object. Generally, we use it for debugging, type checking, and understanding the nature of the objects you are working with.
Code Example:
I0NyZWF0aW5nIGFuIGludGVnZXIgdmFyaWFibGUKbnVtYmVyID0gMTIzCgojQ2hlY2tpbmcgaXRzIHR5cGUKcHJpbnQoIlR5cGUgb2YgbnVtYmVyOiIsIHR5cGUobnVtYmVyKSk=
Output:
Type of number: <class 'int'>
Here, we use the type() function to get the type of an integer variable called number.
Python dir() Function
The Python built-in function is used to get a list of the attributes and methods of an object. It is useful for introspection, debugging, and exploring the capabilities of an object.
Code Example:
I0NyZWF0aW5nIGEgbGlzdMKgCm15X2xpc3QgPSBbMSwgMiwgM10KCiMgQ2hlY2tpbmcgYW5kIGRpc3BsYXlpbmcgdGhlIGxpc3QgYXR0cmlidXRlcyB1c2luZyBkaXIoKSBpbnNpZGUgcHJpbnQoKQpwcmludCgiQXR0cmlidXRlcyBhbmQgbWV0aG9kcyBvZiBteV9saXN0OiIsIGRpcihteV9saXN0KSk=
Output:
Attributes and methods of my_list: ['__add__', '__class__', '__class_getitem__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__gt__', '__hash__', '__iadd__', '__imul__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__rmul__', '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'append', 'clear', 'copy', 'count', 'extend', 'index', 'insert', 'pop', 'remove', 'reverse', 'sort']
Here, we use the dir() function to get the attributes and methods of a list, as shown in the output window.
Python id() Function
The Python built-in function is used to get the unique identifier of an object. This is useful for understanding object references, memory management, and ensuring that objects are distinct.
Code Example:
bnVtYmVyID0gMTIzCgojVXNpbmcgaWQoKSBpbnNpZGUgcHJpbnQgdG8gY2hlY2sgYW5kIGRpc3BsYXkgdGhlIHVuaXF1ZSBpZGVudGlmaWVyIGZvciB0aGUgdmFyaWFibGUKcHJpbnQoIklEIG9mIG51bWJlcjoiLCBpZChudW1iZXIpKQ==
Output:
ID of number: 124363478470704
Here, we use the id() function to get the unique identifier of an integer, as shown in the output window.
List Of Python Built-In Functions (Alphabetical)
Listed in the table below are any advanced or less frequently used built-in functions that might not fall into the categories discussed above.
Function Name |
Syntax |
Description |
all() |
all(iterable) |
Returns True if all elements of the iterable are true (or if the iterable is empty). |
any() |
any(iterable) |
Returns True if any element of the iterable is true. If the iterable is empty, returns False. |
ascii() |
ascii(object) |
Returns a string containing a printable representation of an object, escaping non-ASCII characters. |
bin() |
bin(x) |
Converts an integer number to a binary string prefixed with "0b". |
bool() |
bool([x]) |
Converts a value to a Boolean, using the standard truth testing procedure. |
bytearray() |
bytearray([source[, encoding[, errors]]]) |
Returns a new array of bytes. |
bytes() |
bytes([source[, encoding[, errors]]]) |
Returns a new "bytes" object, which is an immutable sequence of integers in the range 0 <= x < 256. |
chr() |
chr(i) |
Returns the string representing a character whose Unicode code point is the integer i. |
classmethod() |
classmethod(function) |
Converts a method into a class method. |
compile() |
compile(source, filename, mode, flags=0, dont_inherit=False, optimize=-1) |
Compiles a source into a code or AST object. |
complex() |
complex([real[, imag]]) |
Creates a complex number. |
delattr() |
delattr(object, name) |
Deletes the named attribute from the object. |
dict() |
dict(**kwargs) |
Creates a new dictionary. |
divmod() |
divmod(a, b) |
Takes two numbers and returns a pair of numbers (a // b, a % b). |
enumerate() |
enumerate(iterable, start=0) |
Returns an enumerate object, which yields pairs containing a count and a value yielded by the iterable. |
eval() |
eval(expression, globals=None, locals=None) |
Evaluates the given expression within the provided globals and locals. |
exec() |
exec(object[, globals[, locals]]) |
Executes the given object within the provided globals and locals. |
format() |
format(value[, format_spec]) |
Converts a value to a formatted string according to format_spec. |
frozenset() |
frozenset([iterable]) |
Returns a new frozenset object, which is an immutable set. |
getattr() |
getattr(object, name[, default]) |
Returns the value of the named attribute of an object. If not found, returns the default value if provided. |
globals() |
globals() |
Returns a dictionary representing the current global symbol table. |
hasattr() |
hasattr(object, name) |
Returns True if the object has the named attribute. |
hash() |
hash(object) |
Returns the hash value of the object. |
hex() |
hex(x) |
Converts an integer number to a lowercase hexadecimal string prefixed with "0x". |
isinstance() |
isinstance(object, classinfo) |
Returns True if the object is an instance or subclass of classinfo. |
issubclass() |
issubclass(class, classinfo) |
Returns True if class is a subclass (directly or indirectly) of classinfo. |
iter() |
iter(object[, sentinel]) |
Returns an iterator object. |
locals() |
locals() |
Updates and returns a dictionary representing the current local symbol table. |
memoryview() |
memoryview(obj) |
Returns a memory view object from the given object. |
next() |
next(iterator[, default]) |
Retrieves the next item from the iterator by calling its __next__() method. If default is given, it is returned if the iterator is exhausted. |
object() |
object() |
Returns a new featureless object. |
oct() |
oct(x) |
Converts an integer number to an octal string prefixed with "0o". |
ord() |
ord(c) |
Returns an integer representing the Unicode code point of the given Unicode character. |
property() |
property(fget=None, fset=None, fdel=None, doc=None) |
Returns a property attribute. |
range() |
range(start, stop[, step]) |
Generates a sequence of numbers from start to stop (exclusive) by step. |
repr() |
repr(object) |
Returns a string containing a printable representation of an object. |
reversed() |
reversed(seq) |
Returns an iterator that accesses the given sequence in reverse order. |
round() |
round(number[, ndigits]) |
Rounds a number to a given precision in decimal digits. |
set() |
set([iterable]) |
Returns a new set object. |
setattr() |
setattr(object, name, value) |
Sets the named attribute on the given object to the specified value. |
slice() |
slice(start, stop[, step]) |
Returns a slice object representing the set of indices specified by range(start, stop, step). |
staticmethod() |
staticmethod(function) |
Converts a method into a static method. |
super() |
super([type[, object-or-type]]) |
Returns a proxy object that delegates method calls to a parent or sibling class. |
vars() |
vars([object]) |
Returns the __dict__ attribute of the given object. |
zip() |
zip(*iterables) |
Returns an iterator of tuples, where the i-th tuple contains the i-th element from each of the argument iterables. |
Looking for guidance? Find the perfect mentor from select experienced coding & software experts here.
Let’s discuss three of the more common Python built-in function from the table above, with the help of examples.
Python all() Function
The Python built-in function is used to check if all elements in an iterable satisfy a certain condition. It returns True if all elements in the iterable are true (or if the iterable is empty); otherwise returns False.
Code Example:
bnVtYmVycyA9IFsxLCAyLCAzLCA0LCA1XQoKcmVzdWx0ID0gYWxsKG51bSA+IDAgZm9yIG51bSBpbiBudW1iZXJzKQpwcmludChyZXN1bHQp
Output:
True
Here, we use the all() function to check if all numbers in the list are greater than 0.
Python any() Function
The Python built-in function is used to check if any element in an iterable satisfies a certain condition. It returns True if any elements in the iterable are true (or if the iterable is empty), otherwise returns False.
Code Example:
bnVtYmVycyA9IFswLCAxLCAyLCAzLCA0XQoKcmVzdWx0ID0gYW55KG51bSA+IDIgZm9yIG51bSBpbiBudW1iZXJzKQpwcmludChyZXN1bHQp
Output:
True
Here, we use the any() function to check if any numbers in the list are greater than 2.
Python ascii() Function
The Python built-in function is used to get a string representation of an object with non-ASCII characters escaped. It returns a string containing a printable representation of an object, escaping non-ASCII characters.
Code Example:
I0NyZWF0aW5nIGEgc3RyaW5nIHZhcmlhYmxlIHRoYXQgY29udGFpbnMgYW4gZW1vamkKdGV4dCA9ICJQeXRob24gaXMgZnVuISDwn5iKIgoKI0ZpbmRpbmcgYW5kIHByaW50aW5nIHRoZSBhc2NpaSB2YWx1ZSBvZiB0aGUgZW1vamkKcmVzdWx0ID0gYXNjaWkodGV4dCkKcHJpbnQocmVzdWx0KQ==
Output:
'Python is fun! \U0001f60a'
Here, we convert the emoji to its Unicode escape sequence using the ascii() function.
Conclusion
Python’s built-in functions are the backbone of efficient and effective coding, empowering developers to tackle everything from simple data manipulations to complex computations with ease. Mastering these built-in functions across categories—whether for mathematical operations, string handling, file manipulation, and more—is essential for writing efficient Python programs.
Familiarity with these powerful tools not only simplifies problem-solving but also opens doors to writing cleaner, more readable code. Whether you're just starting or are a seasoned Pythonista, these functions are key to unlocking the language's full potential and enhancing your coding prowess.
Frequently Asked Questions
Q1. How do I find the list of all built-in functions in Python?
To view all built-in functions in Python, you can use the dir() function on the builtins module. This will return a list of all attributes, including functions, exceptions, and other objects.
Code Example:
aW1wb3J0IGJ1aWx0aW5zCgpidWlsdF9pbl9mdW5jdGlvbnMgPSBkaXIoYnVpbHRpbnMpCnByaW50KGJ1aWx0X2luX2Z1bmN0aW9ucyk=
This will print a list of all the available constants, exceptions and built-in functions in Python.
Q2. Can I override Python built-in functions?
Yes, you can override built-in functions by defining a function with the same name within the current scope. However, this practice is generally discouraged as it may lead to confusion and unexpected behavior in your code.
Q3. What is the difference between all() and any() functions?
The all() function returns True if all elements in the iterable are true, while any() function returns True if any element in the iterable is true.
Q4. How many built-in functions does Python have?
Python has over 70 built-in functions, covering a wide range of functionalities from basic operations to more complex tasks.
Q5. Give an example of the zip() function?
The zip() function combines elements from multiple iterables into pairs, as shown in the example below.
Code Example:
I1VzaW5nIHppcCBvbiBpdGVyYWJsZXMKI0FuZCB0aGVuIGNvbnZlcnRpbmcgaXQgdG8gYSBsaXN0IGJlZm9yZSBwcmludGluZwpwcmludChsaXN0KHppcChbMSwgMiwgM10sIFsnYScsICdiJywgJ2MnXSkpKQ==
Output:
[(1, 'a'), (2, 'b'), (3, 'c')]
In the code example above, we combine two lists into a single list using the zip() function.
Q6. How does enumerate() work and why is it useful?
The enumerate() function adds a counter to an iterable, returning an enumerate object. This is useful for accessing both index and value in a for loop:
Code Example:
Zm9yIGluZGV4LCB2YWx1ZSBpbiBlbnVtZXJhdGUoWydhJywgJ2InLCAnYyddKToKICBwcmludChpbmRleCwgdmFsdWUp
Output:
0 a
1 b
2 c
In the code example above, we use the enumerate() function to print the index of each value inside the list.
Q7. What are Python's built-in functions?
Built-in functions in Python arе prе-dеfinеd functions that are readily available and accеssiblе without rеquiring any import statеmеnts or additional sеtups. Thеsе functions arе part of thе Python standard library and offеr a widе rangе of functionalitiеs to perform various tasks.
Q8. How do classmethod() and staticmethod() differ?
The classmethod() converts a method into a class method, which receives the class (cls) as an implicit first argument. The staticmethod() on the other hand, converts a method into a static method, which does not receive an implicit first argument.
Q9. How can I create a range of numbers in Python?
You can use the Python built-in function, range() to create a range of numbers. For example, range(1, 10) generates numbers from 1 to 9.
Q10. How does the eval() function work? Explain with an example.
The eval() function evaluates a string as a Python expression and returns the result. It can also execute code dynamically.
Code Example:
eCA9IDEwCmV4cHJlc3Npb24gPSAieCAqIDIgKyA1IgoKcmVzdWx0ID0gZXZhbChleHByZXNzaW9uKQpwcmludChyZXN1bHQp
Output:
25
In the code example above, we use eval() function to evaluate the string "x * 2 + 5" as if it were a Python expression, using the current value of x and prints the result.
This compiles our discussion on the Python built-in functions. Here are a few more Python topics you must explore:
- Python Logical Operators, Short-Circuiting & More (With Examples)
- Python Bitwise Operators | Positive & Negative Numbers (+Examples)
- 12 Ways To Compare Strings In Python Explained (With Examples)
- Python Namespace & Variable Scope Explained (With Code Examples)
- How To Reverse A String In Python? 10 Easy Ways With Examples
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment