Python type() Function | Syntax, Applications & More (+Examples)
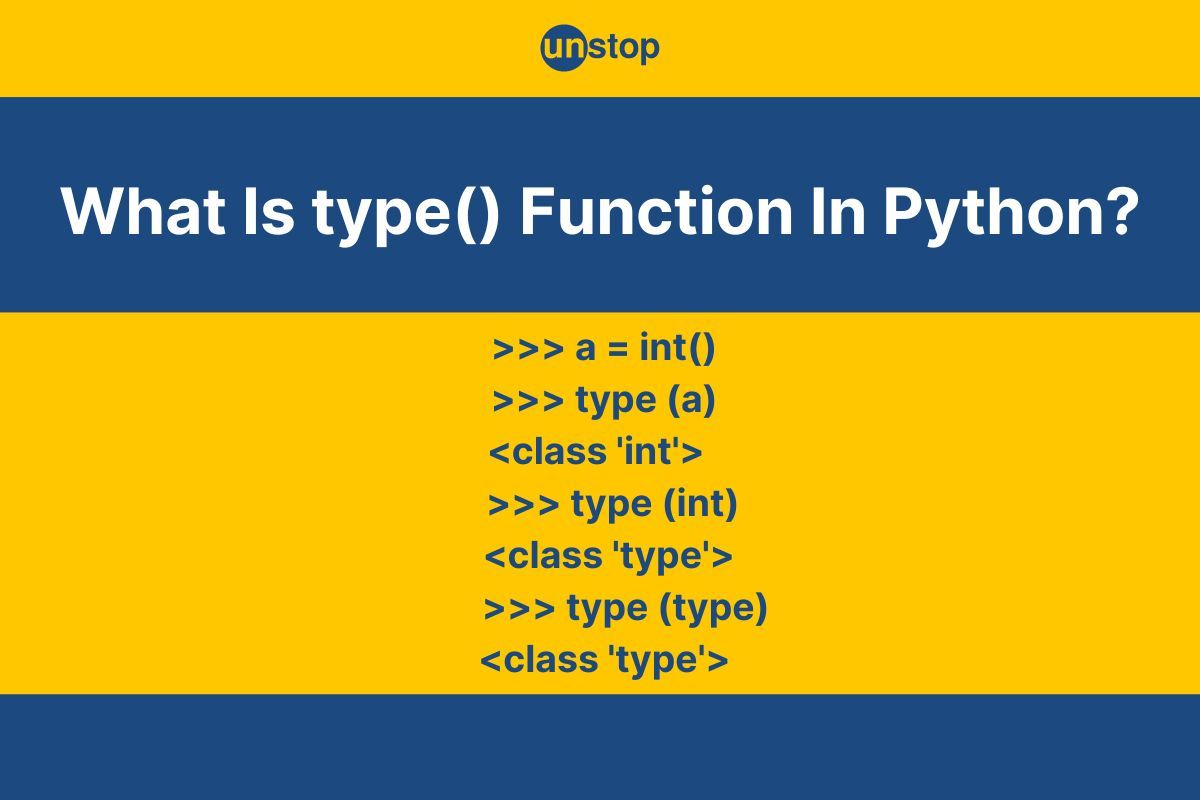
In Python, everything is an object, from numbers to strings, lists, and even functions. To effectively work with these objects, it's essential to know their types. This is where the type() function comes into play.
The Python type() function is a built-in utility that returns the type of a given object. It's a fundamental tool for understanding and manipulating data in Python programming. In this article, we'll explore how the Python type() function works, explore various examples of its usage, and discuss some practical applications where it can enhance your code.
What Is Python type() Function?
As discussed above, the type() function in Python is a built-in function used to identify the type of an object or to dynamically create new types (classes). It serves two main purposes:
- Identifying the type of an object: It allows you to check the class or type of an object during runtime, helping developers determine what kind of data they are working with.
- Creating new types: It can also be used to create new types (classes) dynamically by passing the appropriate arguments, although this is a more advanced use case.
The Python type() function works as follows:
- Pass an object: First, we provide the type() function with any Python object as an argument.
- Returns the type: The function then determines the data type of that object and returns it as a result.
Python type() Function Syntax:
There are two different ways in which we can use the Python type() function:
1. To Get The Type Of An Object (Single Argument):
type(object)
Here, object refers to the object whose type is to be identified. This parameter is essential and can be a value, a variable name, etc.
2. To Create A New Type/ Class (Three Arguments):
type(name, bases, dict)
Here,
- name is the name/identifier of the new class we want to create.
- base is a tuple containing the base classes from which the new class will inherit (can be empty).
- dict refers to a dictionary containing attributes and methods that define the new class.
Return Value Of Python type() Function:
- When passing a single object as an argument, the return value of the type() function is the type of that object. For Example-
type(10) # Output: <class 'int'>
- When passing name, bases, and dict as arguments, the return value of the type() function is a new type (class) object. For Example-
MyClass = type('MyClass', (object,), {'x': 5})
print(MyClass) # Output: <class '__main__.MyClass'>
Now, we will explore different examples to understand how the type() function in Python works:
Python type() To Find The Object Type
We can use the Python type() function to find the type of any Python object. For example, it can help us identify whether the argument passed is an int, str, list, or another data type.
Code Example:
Output:
<class 'int'>
<class 'float'>
<class 'str'>
<class 'list'>
Explanation:
In the above code example-
- We start by initializing four variables: x is set to 10 (an integer), y to 3.14 (a float), z to "Hello, World!" (a string), and a (list type) containing the numbers 1, 2, and 3.
- Next, we use the type() function along with the print() function to display the type of each variable.
- The first line, print(type(x)), checks the type of x, which returns <class 'int'>, indicating that x is an integer.
- The second line, print(type(y)), checks the type of y, resulting in <class 'float'>, confirming that y is a float.
- The third line, print(type(z)), checks the type of z, which returns <class 'str'>, indicating that z is of string data type.
- The last line, print(type(a)), checks the type of a, and returns <class 'list'>, which tells us that a is a list.
Python type() To Check If An Object Is Of A Specific Type
We can also use the Python type() function to compare an object's type against a specific. It helps us confirm what type the object belongs to.
Code Example:
Output:
x is a list.
y is a string.
z is an integer.
Explanation:
In the above code example-
- We begin by initializing three variables: x is a list containing the numbers 1, 2, and 3; y is a string set to "Hello"; and z is an integer data type with a value of 42.
- Next, we use the type() function and an if statement to check the type of the variable x. We use the equality operator (==) to see if the type of x is equal to list. If it is, we print "x is a list." using the print() function.
- Similarly, in the next if statement, we check the type of the variable y. If it is equal to str, we print "y is a string."
- Finally, in the last if statement, we check the type of the variable z. If it is equal to int, we print "z is an integer."
Python type() With Conditional Statement
We can use the Python type() function within conditional statements to execute different blocks of code based on the type of an object.
Code Example:
Output:
x is a float.
Explanation:
In the above code example-
- We start by initializing the variable x with the float value of 3.14.
- Next, we use an if statement to check if the type of x is equal to int. If this condition is true, we print "x is an integer." Since x is not an integer, this condition is skipped.
- Then we move to the elif statement that checks if the type of x is equal to float. Since x is a float, we print "x is a float."
- If neither of the previous conditions is satisfied, we check another elif statement to see if the type of x is equal to str. But since x is not a string, this condition is also skipped.
- Finally, if none of the conditions are met, the else block will execute, printing "x is of another type," which acts as a catch-all for any other types not specifically checked. However, in this case, it doesn't run because the float condition is met.
Python type() With 3 Parameters
The Python type() function can also be used to dynamically create a new class when provided with three parameters: the class name, base classes, and attributes.
Code Example:
Output:
100
Explanation:
In the above code example-
- We create a class called NewClass using the type() function.
- This function takes three arguments: the name of the class as a string, a tuple containing the base class (in this case, object), and a dictionary of attributes, which defines an attribute attr with a value of 100.
- We then instantiate an object of NewClass by calling NewClass(), which creates a new instance named obj.
- Finally, we use print() function to access the attr attribute of obj, which outputs the value 100, demonstrating that the attribute was successfully created and can be accessed from the object.
Python type() For A Class Object
The Python type() function can be used to determine the type of an object created from a class, allowing us to confirm the class to which the object belongs.
Code Example:
Output:
<class '__main__.MyClass'>
Explanation:
In the above code example-
- We start by defining a class called MyClass using the class keyword. The pass statement indicates that the class has no body.
- Next, we create an instance of MyClass by calling MyClass(), which initializes a new object and assigns it to the variable obj.
- We then use the print() function along with the type() function to check the type of the object obj. The output confirms that obj is an instance of MyClass, allowing us to verify the object's type.
Applications Of Python type() Function
Here are some common applications of the Python type() function:
- Type Checking: We can use Python type() to verify the type of a variable or object, ensuring it matches an expected data type during debugging or validation. For Example-
x = 5
if type(x) == int:
print("x is an integer.")
- Dynamic Type Checking: In dynamically typed languages like Python, it’s useful to check the type of variables at runtime to adjust behaviour accordingly. For Example-
def check_type(data):
if type(data) == str:
print("It's a string.")
elif type(data) == list:
print("It's a list.")
- Class Type Identification: When working with objects, Python type() can be used to identify which current class an object belongs to. For Example-
class MyClass:
pass
obj = MyClass()
print(type(obj)) # Output: <class '__main__.MyClass'>
- Object Creation And Metaprogramming: The type() function can be used in advanced Python programming to dynamically create new types or classes with attributes. Essentially, it acts like a constructor for creating new classes at runtime. For Example-
MyNewClass = type('MyNewClass', (object,), {'attribute': 42})
obj = MyNewClass()
print(obj.attribute) # Output: 42
- Function Parameter Validation: It is often used to ensure we use the correct type of function parameters before performing operations on them. For Example-
def add_numbers(a, b):
if type(a) == int and type(b) == int:
return a + b
else:
return "Invalid input"
- Exploring Python's Built-In Types: The Python type() allows us to explore and experiment with various built-in types, which helps us understand the data structure or object we are working with. For Example-
print(type(3.14)) # Output: <class 'float'>
print(type("Hello")) # Output: <class 'str'>
Conclusion
The type() function in Python programming language is a powerful feature for both identifying an object's type and dynamically creating new classes. Mastering its use not only aids in debugging and improving code readability but also enables more flexible and dynamic programming techniques. By using Python type(), developers can ensure their code handles different data types efficiently and behaves as expected.
Frequently Asked Questions
Q. What is the purpose of the type() function in Python?
The type() function serves two main purposes:
- Identifying the type of an object: It allows developers to determine the type of any object in Python. This is particularly useful for debugging or when working with functions that may accept different types of input. For example, if you're unsure whether a variable is a string or a list, you can use type(variable) to confirm its type.
- Creating new types/classes: When called with three arguments, type() can create new class types dynamically. This feature is especially useful in metaprogramming, where classes can be defined and modified at runtime.
Q. How do I use type() to check the variable types?
You can use type(variable_name) in Python to check the variable type. It returns the class type of the specified object, allowing you to determine whether a variable is an integer, float, string, list, or any other type. For example, if you have a variable x = 10, calling type(x) will return <class 'int'>, indicating that x is an integer.
Q. Can I use type() with multiple arguments?
Yes, the type() function can be called with three arguments to create a new dynamic class body. The syntax is type(name, bases, dict), where name is the class name, bases is a tuple of base classes, and dict is a dictionary data type of attribute values and methods.
Q. What happens if I pass an invalid object to type()?
If you pass an invalid object to type(), Python will raise a TypeError. For instance, calling type() without any arguments will result in the following error diagnosis:
print(type()) # Raises TypeError: type() missing 1 required positional argument: 'obj'
However, passing None is valid and will return <class 'NoneType'>:
print(type(None)) # Output: <class 'NoneType'>
Q. Can I use type() to check if an object is an instance of a specific class?
While type function can help identify an object's type, it is often more appropriate to use the isinstance() function for type checking. This is because isinstance() not only checks if an object is an instance of a specified class but also considers inheritance, allowing for more flexible and accurate type checks. For example-
class Animal:
passclass Dog(Animal):
passdog = Dog()
# Using type()
print(type(dog) == Dog) # True
print(type(dog) == Animal) # False# Using isinstance()
print(isinstance(dog, Dog)) # True
print(isinstance(dog, Animal)) # True
In this source code, type() only checks if the object is exactly of the specified type, while isinstance() also accounts for inheritance.
Q. Is type() a built-in function?
Yes, type() is a built-in function in Python, meaning it is readily available for use without the need to import any external libraries or modules. Built-in functions like type() are part of the core Python language, providing essential functionalities for everyday programming tasks. You can call it at any time in your Python code without additional setup.
Here are a few other topics that you might be interested in:
- Swap Two Variables In Python- Different Ways | Codes + Explanation
- Python Bitwise Operators | Positive & Negative Numbers (+Examples)
- Python Program To Add Two Numbers | 8 Techniques With Code Examples
- Difference Between C and Python | Definition & Feature Explained
- Calculator Program In Python - Explained With Code Examples
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment