Python Program To Add Two Numbers | 8 Techniques With Code Examples
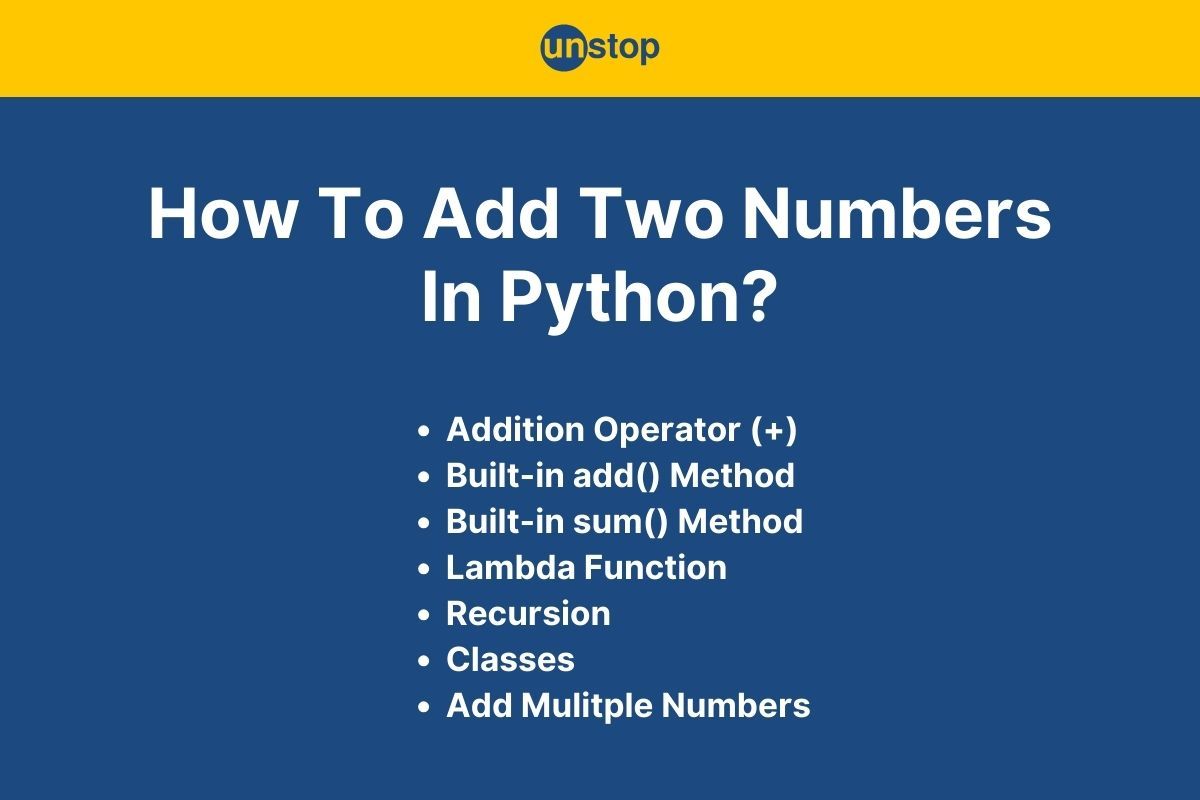
Python is a versatile programming language that allows users to simplify complex functions and tasks, given the simplicity of its syntax. This makes it easier to understand and write Python programs for complex problems. In this article, we embark on a journey to uncover the various ways in which we can add two numbers in Python programming.
The techniques Python offers for this range from the fundamental approach of using operators to more advanced methods leveraging functions and data structures. Whether you're a beginner seeking to grasp the essentials or an experienced coder looking to refine your skills, join us as we unravel the power and flexibility of Python in tackling the seemingly simple task of adding two numbers.
Algorithm Of Python Program To Add To Numbers
Before exploring the various techniques and constructs we can use to write a Python program to add two numbers, it is important to understand the basic logic and mechanism behind the code. In this section, we will discuss the algorithm for a Python program that adds two numbers in a step-by-step format.
Step 1- Start: The program execution begins.
Step 2- Define An Addition Function: Define a function add_numbers that takes two parameters (num1 and num2).
- Inside the function, calculate the sum of num1 and num2 and store it in a variable (sum_result).
- Return the sum_result.
Step 3- Input: Prompt the user to enter the first and second numbers. Read and store the input as number1 and number2.
Step 4- Function Call: The next step is to call the addition function with the two numbers as inputs. It stores the outcome in a variable (result).
Step 5- Output: Print the result, indicating the sum of number1 and number2.
Step 6- The program execution concludes.
Now that we know the general structure of the algorithm to write a Python program to add two numbers, let's take a look at a Python code example that shows how to implement this algorithm.
Code Example:
Output:
Enter the first number: 19
Enter the second number: 4
The sum of 19.0 and 4.0 is: 23.0
Explanation:
In this simple Python program-
- We begin by defining a function named add_numbers that takes two parameters, num1 and num2.
- Inside this function, we use the addition arithmetic operator to calculate the sum of num1 and num2 and assign the result to the variable sum_result.
- Subsequently, we ensure that the function returns the value stored in sum_result.
- Next, we use the input() method to prompt the user to input the two values and store them in variables num1 and num2, respectively.
- We also use the float() function along with input() to convert the input value into floating-point values.
- Then, we call the add_numbers function to perform the addition with the arguments number1 and number2. The outcome of the operation is stored in the variable result.
- Finally, we print the result using the print() function with a formatted string. Inside the string, we insert the values for both the numbers and the sum inside curly braces.
Also read- Python IDLE | The Ultimate Beginner's Guide With Images & Codes!
Standard Program To Add Two Numbers In Python
The standard program to add two numbers in Python is a fundamental example that showcases the simplicity of arithmetic operations in the language. This program typically involves the use of two variables to store the numbers, a straightforward addition operation, and finally, the display of the result. User input or pre-defined values can be used for the numbers. This method provides a clear illustration of how to perform a common mathematical operation within the Python programming language.
Syntax:
result=A+B
Here,
- A and B are two variables with integer or float values.
- The plus sign (+) is a mathematical operator used to perform the addition operation.
- The result is a variable that stores the output value.
Now, let's look at two code examples: one where we perform addition on two numbers of integer data type, and the other where we perform addition on two floating-point numbers.
1. Python Program To Add Two Integers
Code Example:
Output:
11
Explanation:
- We begin by initializing two variables, A and B, with the values 5 and 6, respectively.
- Next, we perform the addition operation on the two variables using the addition operator. The outcome of the addition is stored in the sum variable.
- We then use the print() function to display the output in the window.
2. Python Program To Add Two Floating Point Numbers
Code Example:
Output:
11.8
Explanation:
- As mentioned in code comments, we begin the Python code by assigning values 5.5 and 6.3 to two variables, A and B, respectively.
- Then, we implement the addition operation on the two variables, i.e., A+ B. The outcome is stored in the sum variable.
- We then use the print() function to display the output in the window.
Python Program To Add Two Numbers With User-defined Input
The method for adding two numbers in Python by using user-defined input involves the incorporation of user interaction to input two numbers. This interactive approach allows users to input values, typically through the built-in function input(), making the program more dynamic. The program then proceeds to convert these user-provided inputs into numeric values, performs the addition operation, and displays the result. This method is beneficial for scenarios where the numbers can vary, offering flexibility and user engagement in providing input.
Code Example:
Output:
Enter A value:5
Enter B value:6
11
Explanation:
- We begin the sample Python program by declaring two variables, A and B. Instead of initializing the variables, we assign user-defined input.
- For this, we use the input() function to prompt users to provide values and then use the int() function to convert the provided input to the integer type.
- Next, we calculate the sum of A and B and store the result in the variable sum.
- Finally, we display the computed sum using the print() statement.
This concise code prompts users to input values for A and B, calculates their sum, and then prints the result.
The add() Method In Python Program To Add Two Numbers
In this method, we use the inbuilt function in Python, i.e., add() to add two numbers. This function is typically applied to sets and is used to add numbers to the set. However, we can also use it on numeric types to directly add two given numbers.
The program encapsulates the two numbers within the add() method, and the result is the sum of the provided values. It is equivalent to using the addition operator, but it offers a concise and readable way to carry out addition without the need for explicit arithmetic operators.
Syntax:
result=operator.add(A, B)
Here,
- A and B are variables that contain Integer or float values.
- The function operator.add() will perform the addition of two numbers.
- The result variable is where we store the outcome of the function.
Code Example:
Output:
30
Explanation:
We begin the Python code example by importing the operator module.
- After this, we assign a value of 10 to variable A and a value of 20 to variable B.
- Next, we call the add() function from the operator module to get the sum of A and B. We use the dot operator to access the function.
- The outcome of the add() function is stored in the variable sum.
- Lastly, we print the computed sum using the print() function.
In summary, this code utilizes the operator module to perform addition, allowing us to add the values of A and B and display the result.
Python Program To Add Two Numbers Using Lambda
Lambda python function is an anonymous function that takes any number of values as parameters but only returns a single value. We can use this function to add two numbers. For that, we need to define a lambda function that takes 2 parameters and returns its addition. To perform the addition of two numbers, we need to call the lambda function that we defined previously.
Syntax:
sum=lambda x,y:x+y
Here,
- The variables x and y are two values that are the parameters for the lambda function.
- The expression x+y is the addition of operation, which returns the addition of two values.
- Sum is the name of the lambda function. We will use it to call the function to add two numbers.
Code:
Output:
30
Explanation:
- We start by assigning a value of 10 to variable A and a value of 20 to variable B.
- Next, we define a lambda function with the name sum to perform addition on its parameters, i.e., sum=lambda X, Y: X+Y.
- This lambda function takes two parameters, X and Y, and returns their sum.
- Next, we call the lambda function by passing A and B as arguments A and B. The outcome of addition is stored in the result variable ans.
- Finally, we print the computed sum using print().
Python Program To Add Two Numbers Using Function
Under this method, the way to write the Python program to add two numbers is to define a basic function that takes two parameters. For example, we define the basic function that takes two variables and returns the addition of those two numbers. To perform addition, we need to call the function by passing arguments to the function.
Syntax:
result=sum(A, B)
Here,
- A and B are two variables that contain integer or float values.
- The sum() function is a function, which will take two arguments.
- The result variable stores the output value
Code Example:
Output:
30
Explanation:
- We begin by defining a function named sum that takes two parameters, X and Y. It returns their sum using the expression return X+Y.
- Then, we create two variables, A and B, and assign them values 10 and 20, respectively.
- Next, we call the sum function with the arguments A and B. The outcome is stored in the variable result.
- Finally, we display the computed sum using the print() method.
Using The In-Built Sum() Function To Add Two Numbers
Alternatively, we can also use the built-in sum() function in Python. This function works with iterable objects, such as lists, tuples, etc., of numeric type. We can apply this function to a list with two elements if we want to use it in writing a Python program to add two numbers.
Syntax:
result=sum(iterable)
Here,
- The term iterable refers to the iterable object, which is the parameter for the sum() function.
- The identifier result is the name given to the variable where we store the output value.
Code Example:
Output:
30
Explanation:
- In the example, we begin by declaring a list called lst with two elements, i.e., 10 and 20.
- Next, we call the sum() method by passing the list as an argument. We store the outcome in the result variable.
- We then use the print() function to display the output in the window.
Python Program To Add Two Numbers Using Recursion
Recursion function is a technique where a function calls itself to in order to solve the problem. It is a process where a function solves smaller instances of the same problem until it reaches a base case. It is commonly used in algorithms to solve problems that can be broken down into smaller subproblems.
To add two integers, we write a recursive function that takes two values, and the recursive function calls that increments one and decrements the other.
Syntax:
result=sum(A, B)
Here,
- A and B are two variables which can have integer or float values.
- The term sum() is the name given to the recursion function.
- The term result is the name of the variable which stores the output value.
Code Example:
Output:
30
Explanation:
- We start by defining a recursive function named sum that takes two parameters, A and B.
- Inside this function, we have an if-statement that checks if B is equal to 0, using the equality relational operator.
- If the condition is true, we return the current value of A.
- If it is false, we recursively call the sum function with incremented A and decremented B.
- After defining the function, we assign a value of 10 to variable A and a value of 20 to variable B.
- Next, we call the sum function with the arguments A and B and store the sum in the variable result.
- Finally, we print the computed result using the print() statement.
Python Program To Add Two Numbers Using Class
We can use two numbers using a class wherein we need to implement the sum function, which will be responsible for adding two numbers. So, we define a class and create a constructor method for handling two arguments and a sum method. Then, we call the class by passing two values and then call the sum method of that class to get the addition of two numbers.
Code Example:
Output:
30
Explanation:
- We begin by defining a class named numbers. The class has an __init__ method, which serves as the constructor.
- The constructor takes three parameters, i.e., self (a reference to the instance), A, and B. Inside the constructor, we assign the values of A and B to the instance variables self.A and self.B, respectively.
- Additionally, the class contains a sum() method. This method takes no external parameters but utilizes the instance variables self.A and self.B to calculate their sum, returning the result.
- Next, we create a class instance called values, i.e., values= numbers(10,20). This invokes the constructor, initializing the instance variables A and B with values 10 and 20.
- We then call the sum() method on the instance of class values using the dot operator. The outcome of this operation is stored in the variable result.
- Finally, we print the computed result using print().
How To Add Multiple Numbers In Python?
So far, we have discussed various techniques to use when writing a Python to add two numbers. But what if we want to add multiple numbers? Well, there are various methods we can employ to add multiple numbers in Python. We will discuss two of the most common approaches for the same in this section.
1. Using Python Built-in sum() Method To Add Mulitple Numbers
In this method, we store the multiple values in a iterable type variable such as a list or a tuple. After that, we use the sum() method by passing the iterable variable to the sum function. This will return the summation of all the values in that iterable variable.
Code Example:
Output:
100
Explanation:
- First, we create a list construct called numbers and add values 10, 20, 30, and 40 to it.
- We then call the sum() method, passing the list to it as arguments.
- The function adds the multiple numbers in the list and stores the output in the result variable.
- We then use the print() function to display the output in the window.
2. Using A For Loop To Add Mulitple Numbers In Python
In this method, we store the multiple values in an iterable type variable, such as a list or a tuple. Then, we use a for loop to traverse the iterable object and successively add each element. The code given below will help you understand how this is implemented.
Code Example:
Output:
100
Explanation:
- We start with a list named numbers containing the values [10, 20, 30, 40].
- Next, we initialize a variable named result with the value 0.
- We then enter a for loop that iterates over each element (i) in the list numbers.
- Inside the loop, we increment the result variable by adding the current value of i.
- By the end of all iterations, the loop will return the sum of all elements on the list. This is stored in the result variable.
- After completing the loop, we display the final value of the result variable using the print() function.
In summary, this code utilizes a for loop to iterate through each element in the list and accumulate their sum in the result variable, which is then printed. The final output represents the sum of the numbers in the given list.
Add Multiple Numbers In Python With User Input
In Python, adding multiple numbers by taking user input can be achieved through a concise and readable approach using list comprehension. The program prompts the user to input a sequence of numbers separated by spaces, which is then split into individual strings. List comprehension converts each string element into an integer, creating a list of integers. Finally, the sum() function is utilized to calculate the sum of these integers.
Code Example:
Output:
Enter multiple numbers: 10 20 30 40
100
Explanation:
- We use list comprehension to create a list named numbers.
- We have the input() method inside the list to prompt the user to input multiple numbers as a space-separated sequence.
- The split() method separates the input into individual numbers. The int(i) part ensures that each input is converted to an integer before being added to the list.
- Next, we call the sum() function to calculate the sum of all the numbers in the list numbers.
- Finally, we display the result to the console using the print() function.
Therefore, this code efficiently handles user input of multiple numbers, converts them to integers, calculates their sum, and prints the result.
Time Complexities Of Python Programs To Add Two Numbers
Below is a table comparing the time complexities of every method that we have discussed above:
Method | Complexity |
---|---|
Method 1: Standard program | O(1) |
Method 2: User-defined input | O(n) |
Method 3: .add() method | O(1) |
Method 4: Lambda function | O(1) |
Method 5: Python's sum() function | O(1) |
Method 6: Recursion | O(n) |
Method 7: Class | O(1) |
Method 8: Multiple numbers in a list | O(n) |
Method 9: Multiple numbers in a list with user input | O(n) |
Conclusion
In conclusion, creating a Python program to add two numbers is a straightforward and fundamental exercise that provides a hands-on introduction to the language's syntax and basic functionalities. As you continue your journey with Python programming, you can explore additional features, such as error handling, optimization techniques, and modular programming.
Whether you are a programming novice or looking to refresh your skills, mastering the basics, like adding two numbers, sets the stage for more advanced programming challenges. So, take this foundational knowledge and build upon it to unlock the full potential of Python for your future coding endeavors.
Frequently Asked Questions
Q. How do we add 1 to 10 in Python?
We can add 1 to 10 numbers using a for loop and range function. We use the loop to traverse from 1 to 10 numbers and add each number to the result variable. The result variable is initially set to 0 value.
Code Example:
Output:
55
Q. What will be the output of 10+True?
The output of 10+True is 11, 10 is integer, and True is a boolean value which represents 1 in integer format. So, the True value is converted to 1 and added to the 10 value, resulting in a final output of 11.
Code Example:
print(10+True)
Output:
11
Q. What happens if we add two string variables?
If we try to add two string variables with the addition operator (+), the two strings are concatenated. This results in a new string as the string before the (+) operator follows with the string after the operator.
Code Example:
Output:
Hello Unstop
Q. What will be the output of 10+” str” in Python?
In Python, attempting to concatenate an integer (10) with a string ("str") using the addition operator (+) will result in a TypeError. This is because Python does not allow direct concatenation of different data types without explicit conversion. To concatenate or add these values, you need to convert the integer to a string explicitly using the str() function, as in the example below.
result = str(10) + "str"
print(result)
#Here, the result will be 10str
Q. How do we add two sets in Python?
We have two primary approaches to adding two sets in Python. These are the use of the union operator and union() method.
Add two sets using the union operator (|): Sets are unordered collections of distinct items, and joining two sets entails combining their distinct elements into a new set. In this technique, we merge the members of the two sets using the union operator (|). The final set will include all of the distinct components from both sets.
Code Example:
Output:
{1,2,3,4}
Add two sets using the union() method: We can use the union() method on set1 and pass set2 as the parameter in this method. The union() function returns a new set containing all of the distinct members from both sets.
Code Example:
Output:
{1,2,3,4}
Q. What does the __ add __ () do in Python?
The __add__() function in Python is a specific method that determines the behavior of the addition operator (+) for class objects. You may customize the addition operation when using the + operator with instances of your class.
When the addition operator (+) is used between two objects, Python executes the __add__() method of the left operand and sends the right operand as an argument. The __add__() function should be defined within the class and return the result of the addition.
Code:
Output:
30
Explanation:
We first define a Number class with an __init__ method to initialize an instance with a given value. The class also contains the __add__ method, which is implemented to enable the addition of two Number objects, resulting in a new Number instance with the sum of their values. The __str__ method converts the instance to a string for printing.
Instances num1 and num2 are created with values 10 and 20. The addition operator (+) is then overloaded by the __add__ method, and the result is a new Number instance with the value 30. This result is printed, yielding the output 30.
Q. What is digit sum in Python?
In Python, digit sum refers to the process of computing the sum of each digit inside a given integer. It entails removing each digit from the integer and combining them together.
Code Example:
Output:
15
Explanation:
The digit_sum function calculates the sum of the digits of a given number using a while loop and modular arithmetic. It iteratively extracts the last digit of the number, adds it to the sum_of_digits variable, and removes that digit from the number until the number becomes 0. The result of applying this function to the number 12345 is then printed, resulting in the output 15 (1 + 2 + 3 + 4 + 5).
This compiles our discussion on how to write Python programs to add two numbers. Here are a few other articles you must read:
- Flask vs Django: Understand Which Python Framework You Should Choose
- How To Reverse A String In Python? 10 Easy Ways With Examples!
- Python String.Replace() And 8 Other Ways Explained (+Examples)
- 12 Ways To Compare Strings In Python Explained (With Examples)
- Python Logical Operators, Short-Circuiting & More (With Examples)
- Python Bitwise Operators | Positive & Negative Numbers (+Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment