Python Programming
Table of content:
- What Is Python? An Introduction
- What Is The History Of Python?
- Key Features Of The Python Programming Language
- Who Uses Python?
- Basic Characteristics Of Python Programming Syntax
- Why Should You Learn Python?
- Applications Of Python Language
- Advantages And Disadvantages Of Python
- Some Useful Python Tips & Tricks For Efficient Programming
- Python 2 Vs. Python 3: Which Should You Learn?
- Python Libraries
- Conclusion
- Frequently Asked Questions
- It's Python Basics Quiz Time!
Table of content:
- Python At A Glance
- Key Features of Python Programming
- Applications of Python
- Bonus: Interesting features of different programming languages
- Summing up...
- FAQs regarding Python
- Take A Quiz To Rehash Python's Features!
Table of content:
- What Is Python IDLE?
- What Is Python Shell & Its Uses?
- Primary Features Of Python IDLE
- How To Use Python IDLE Shell? Setting Up Your Python Environment
- How To Work With Files In Python IDLE?
- How To Execute A File In Python IDLE?
- Improving Workflow In Python IDLE Software
- Debugging In Python IDLE
- Customizing Python IDLE
- Code Examples
- Conclusion
- Frequently Asked Questions (FAQs)
- How Well Do You Know IDLE? Take A Quiz!
Table of content:
- What Is A Variable In Python?
- Creating And Declaring Python Variables
- Rules For Naming Python Variables
- How To Print Python Variables?
- How To Delete A Python Variable?
- Various Methods Of Variables Assignment In Python
- Python Variable Types
- Python Variable Scope
- Concatenating Python Variables
- Object Identity & Object References Of Python Variables
- Reserved Words/ Keywords & Python Variable Names
- Conclusion
- Frequently Asked Questions
- Rehash Python Variables Basics With A Quiz!
Table of content:
- What Is A String In Python?
- Creating String In Python
- How To Create Multiline Python Strings?
- Reassigning Python Strings
- Accessing Characters Of Python Strings
- How To Update Or Delete A Python String?
- Reversing A Python String
- Formatting Python Strings
- Concatenation & Comparison Of Python Strings
- Python String Operators
- Python String Functions
- Escape Sequences In Python Strings
- Conclusion
- Frequently Asked Questions
- Rehash Python Strings Basics With A Quiz!
Table of content:
- What Is Python Namespace?
- Lifetime Of Python Namespace
- Types Of Python Namespace
- The Built-In Namespace In Python
- The Global Namespace In Python
- The Local Namespace In Python
- The Enclosing Namespace In Python
- Variable Scope & Namespace In Python
- Python Namespace Dictionaries
- Changing Variables Out Of Their Scope & Python Namespace
- Best Practices Of Python Namespace
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Namespaces!
Table of content:
- What Are Logical Operators In Python?
- The AND Python Logical Operator
- The OR Python Logical Operator
- The NOT Python Logical Operator
- Short-Circuiting Evaluation Of Python Logical Operators
- Precedence of Logical Operators In Python
- How Does Python Calculate Truth Value?
- Final Note On How AND & OR Python Logical Operators Work
- Conclusion
- Frequently Asked Questions
- Python Logical Operators Quiz– Test Your Knowledge!
Table of content:
- What Are Bitwise Operators In Python?
- List Of Python Bitwise Operators
- AND Python Bitwise Operator
- OR Python Bitwise Operator
- NOT Python Bitwise Operator
- XOR Python Bitwise Operator
- Right Shift Python Bitwise Operator
- Left Shift Python Bitwise Operator
- Python Bitwise Operations And Negative Integers
- The Binary Number System
- Application of Python Bitwise Operators
- Python Bitwise Operator Overloading
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Bitwise Operators!
Table of content:
- What Is The Print() Function In Python?
- How Does The print() Function Work In Python?
- How To Print Single & Multi-line Strings In Python?
- How To Print Built-in Data Types In Python?
- Print() Function In Python For Values Stored In Variables
- Print() Function In Python With sep Parameter
- Print() Function In Python With end Parameter
- Print() Function In Python With flush Parameter
- Print() Function In Python With file Parameter
- How To Remove Newline From print() Function In Python?
- Use Cases Of The print() Function In Python
- Understanding Print Statement In Python 2 Vs. Python 3
- Conclusion
- Frequently Asked Questions
- Know The print() Function In Python? Take A Quiz!
Table of content:
- Working Of Normal Print() Function
- The New Line Character In Python
- How To Print Without Newline In Python | Using The End Parameter
- How To Print Without Newline In Python 2.x? | Using Comma Operator
- How To Print Without Newline In Python 3.x?
- How To Print Without Newline In Python With Module Sys
- The Star Pattern(*) | How To Print Without Newline & Space In Python
- How To Print A List Without Newline In Python?
- How To Remove New Lines In Python?
- Conclusion
- Frequently Asked Questions
- Think You Can Print Without a Newline in Python? Prove It!
Table of content:
- What Is A Python For Loop?
- How Does Python For Loop Work?
- When & Why To Use Python For Loops?
- Python For Loop Examples
- What Is Rrange() Function In Python?
- Nested For Loops In Python
- Python For Loop With Continue & Break Statements
- Python For Loop With Pass Statement
- Else Statement In Python For Loop
- Conclusion
- Frequently Asked Questions
- Think You Know Python's For Loop? Prove It!
Table of content:
- What Is Python While Loop?
- How Does The Python While Loop Work?
- How To Use Python While Loops For Iterations?
- Control Statements In Python While Loop With Examples
- Python While Loop With Python List
- Infinite Python While Loop in Python
- Python While Loop Multiple Conditions
- Nested Python While Loops
- Conclusion
- Frequently Asked Questions
- Mastered Python While Loop? Let’s Find Out!
Table of content:
- What Are Conditional If-Else Statements In Python?
- Types Of If-Else Statements In Python
- If Statement In Python
- If-Else Statement In Python
- Nested If-Else Statement In Python
- Elif Statement In Python
- Ladder If-Elif-Else Statement In Python
- Short Hand If-Statement In Python
- Short Hand If-Else Statement In Python
- Operators & If-Esle Statement In Python
- Other Statements With If-Else In Python
- Conclusion
- Frequently Asked Questions
- Quick If-Else Statement Quiz– Let’s Go!
Table of content:
- What Is Control Structure In Python?
- Types Of Control Structures In Python
- Sequential Control Structures In Python
- Decision-Making Control Structures In Python
- Repetition Control Structures In Python
- Benefits Of Using Control Structures In Python
- Conclusion
- Frequently Asked Questions
- Control Structures in Python – Are You the Master? Take A Quiz!
Table of content:
- What Are Python Libraries?
- How Do Python Libraries Work?
- Standard Python Libraries (With List)
- Important Python Libraries For Data Science
- Important Python Libraries For Machine & Deep Learning
- Other Important Python Libraries You Must Know
- Working With Third-Party Python Libraries
- Troubleshooting Common Issues For Python Libraries
- Python Libraries In Larger Projects
- Importance Of Python Libraries
- Conclusion
- Frequently Asked Questions
- Quick Quiz On Python Libraries – Let’s Go!
Table of content:
- What Are Python Functions?
- How To Create/ Define Functions In Python?
- How To Call A Python Function?
- Types Of Python Functions Based On Parameters & Return Statement
- Rules & Best Practices For Naming Python Functions
- Basic Types of Python Functions
- The Return Statement In Python Functions
- Types Of Arguments In Python Functions
- Docstring In Python Functions
- Passing Parameters In Python Functions
- Python Function Variables | Scope & Lifetime
- Advantages Of Using Python Functions
- Recursive Python Function
- Anonymous/ Lambda Function In Python
- Nested Functions In Python
- Conclusion
- Frequently Asked Questions
- Python Functions – Test Your Knowledge With A Quiz!
Table of content:
- What Are Python Built-In Functions?
- Mathematical Python Built-In Functions
- Python Built-In Functions For Strings
- Input/ Output Built-In Functions In Python
- List & Tuple Python Built-In Functions
- File Handling Python Built-In Functions
- Python Built-In Functions For Dictionary
- Type Conversion Python Built-In Functions
- Basic Python Built-In Functions
- List Of Python Built-In Functions (Alphabetical)
- Conclusion
- Frequently Asked Questions
- Think You Know Python Built-in Functions? Prove It!
Table of content:
- What Is A round() Function In Python?
- How Does Python round() Function Work?
- Python round() Function If The Second Parameter Is Missing
- Python round() Function If The Second Parameter Is Present
- Python round() Function With Negative Integers
- Python round() Function With Math Library
- Python round() Function With Numpy Module
- Round Up And Round Down Numbers In Python
- Truncation Vs Rounding In Python
- Practical Applications Of Python round() Function
- Conclusion
- Frequently Asked Questions
- Revisit Python’s round() Function – Take The Quiz!
Table of content:
- What Is Python pow() Function?
- Python pow() Function Example
- Python pow() Function With Modulus (Three Parameters)
- Python pow() Function With Complex Numbers
- Python pow() Function With Floating-Point Arguments And Modulus
- Python pow() Function Implementation Cases
- Difference Between Inbuilt-pow() And math.pow() Function
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python’s pow() Function!
Table of content:
- Python max() Function With Objects
- Examples Of Python max() Function With Objects
- Python max() Function With Iterable
- Examples Of Python max() Function With Iterables
- Potential Errors With The Python max() Function
- Python max() Function Vs. Python min() Functions
- Conclusion
- Frequently Asked Questions
- Think You Know Python max() Function? Take A Quiz!
Table of content:
- What Are Strings In Python?
- What Are Python String Methods?
- List Of Python String Methods For Manipulating Case
- List Of Python String Methods For Searching & Finding
- List Of Python String Methods For Modifying & Transforming
- List Of Python String Methods For Checking Conditions
- List Of Python String Methods For Encoding & Decoding
- List Of Python String Methods For Stripping & Trimming
- List Of Python String Methods For Formatting
- Miscellaneous Python String Methods
- List Of Other Python String Operations
- Conclusion
- Frequently Asked Questions
- Mastered Python String Methods? Take A Quiz!
Table of content:
- What Is Python String?
- The Need For Python String Replacement
- The Python String replace() Method
- Multiple Replacements With Python String.replace() Method
- Replace A Character In String Using For Loop In Python
- Python String Replacement Using Slicing Method
- Replace A Character At a Given Position In Python String
- Replace Multiple Substrings With The Same String In Python
- Python String Replacement Using Regex Pattern
- Python String Replacement Using List Comprehension & Join() Method
- Python String Replacement Using Callback With re.sub() Method
- Python String Replacement With re.subn() Method
- Conclusion
- Frequently Asked Questions
- Know How To Replace Python Strings? Prove It!
Table of content:
- What Is String Slicing In Python?
- How Indexing & String Slicing Works In Python
- Extracting All Characters Using String Slicing In Python
- Extracting Characters Before & After Specific Position Using String Slicing In Python
- Extracting Characters Between Two Intervals Using String Slicing In Python
- Extracting Characters At Specific Intervals (Step) Using String Slicing In Python
- Negative Indexing & String Slicing In Python
- Handling Out-of-Bounds Indices In String Slicing In Python
- The slice() Method For String Slicing In Python
- Common Pitfalls Of String Slicing In Python
- Real-World Applications Of String Slicing
- Conclusion
- Frequently Asked Questions
- Quick Python String Slicing Quiz– Let’s Go!
Table of content:
- Introduction To Python List
- How To Create A Python List?
- How To Access Elements Of Python List?
- Accessing Multiple Elements From A Python List (Slicing)
- Access List Elements From Nested Python Lists
- How To Change Elements In Python Lists?
- How To Add Elements To Python Lists?
- Delete/ Remove Elements From Python Lists
- How To Create Copies Of Python Lists?
- Repeating Python Lists
- Ways To Iterate Over Python Lists
- How To Reverse A Python List?
- How To Sort Items Of Python Lists?
- Built-in Functions For Operations On Python Lists
- Conclusion
- Frequently Asked Questions
- Revisit Python Lists Basics With A Quick Quiz!
Table of content:
- What Is List Comprehension In Python?
- Incorporating Conditional Statements With List Comprehension In Python
- List Comprehension In Python With range()
- Filtering Lists Effectively With List Comprehension In Python
- Nested Loops With List Comprehension In Python
- Flattening Nested Lists With List Comprehension In Python
- Handling Exceptions In List Comprehension In Python
- Common Use Cases For List Comprehensions
- Advantages & Disadvantages Of List Comprehension In Python
- Best Practices For Using List Comprehension In Python
- Performance Considerations For List Comprehension In Python
- For Loops & List Comprehension In Python: A Comparison
- Difference Between Generator Expression & List Comprehension In Python
- Conclusion
- Frequently Asked Questions
- Rehash Python List Comprehension Basics With A Quiz!
Table of content:
- What Is A List In Python?
- How To Find Length Of List In Python?
- For Loop To Get Python List Length (Naive Approach)
- The len() Function To Get Length Of List In Python
- The length_hint() Function To Find Length Of List In Python
- The sum() Function To Find The Length Of List In Python
- The enumerate() Function To Find Python List Length
- The Counter Class From collections To Find Python List Length
- The List Comprehension To Find Python List Length
- Find The Length Of List In Python Using Recursion
- Comparison Between Ways To Find Python List Length
- Conclusion
- Frequently Asked Questions
- Know How To Get Python List Length? Prove it!
Table of content:
- List of Methods To Reverse A Python List
- Python Reverse List Using reverse() Method
- Python Reverse List Using the Slice Operator ([::-1])
- Python Reverse List By Swapping Elements
- Python Reverse List Using The reversed() Function
- Python Reverse List Using A for Loop
- Python Reverse List Using While Loop
- Python Reverse List Using List Comprehension
- Python Reverse List Using List Indexing
- Python Reverse List Using The range() Function
- Python Reverse List Using NumPy
- Comparison Of Ways To Reverse A Python List
- Conclusion
- Frequently Asked Questions
- Time To Test Your Python List Reversal Skills!
Table of content:
- What Is Indexing In Python?
- The Python List index() Function
- How To Use Python List index() To Find Index Of A List Element
- The Python List index() Method With Single Parameter (Start)
- The Python List index() Method With Start & Stop Parameters
- What Happens When We Use Python List index() For An Element That Doesn't Exist
- Python List index() With Nested Lists
- Fixing IndexError Using The Python List index() Method
- Python List index() With Enumerate()
- Real-world Examples Of Python List index() Method
- Difference Between find() And index() Method In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python List Indexing? Take A Quiz!
Table of content:
- How To Remove Elements From List In Python?
- The remove() Method To Remove Element From Python List
- The pop() Method To Remove Element From List In Python
- The del Keyword To Remove Element From List In Python
- The clear() Method To Remove Elements From Python List
- List Comprehensions To Conditionally Remove Element From List In Python
- Key Considerations For Removing Elements From Python Lists
- Why We Need to Remove Elements From Python List
- Performance Comparison Of Methods To Remove Element From List In Python
- Conclusion
- Frequently Asked Questions
- Quiz– Prove You Know How To Remove Item From Python Lists!
Table of content:
- How To Remove Duplicates From A List In Python?
- The set() Function To Remove Duplicates From Python List
- Remove Duplicates From Python List Using For Loop
- Using List Comprehension Remove Duplicates From Python List
- Remove Duplicates From Python List Using enumerate() With List Comprehension
- Dictionary & fromkeys() Method To Remove Duplicates From Python List
- Remove Duplicates From Python List Using in, not in Operators
- Remove Duplicates From Python List Using collections.OrderedDict.fromkeys()
- Remove Duplicates From Python List Using Counter with freq.dist() Method
- The del Keyword Remove Duplicates From Python List
- Remove Duplicates From Python List Using DataFrame
- Remove Duplicates From Python List Using pd.unique and np.unipue
- Remove Duplicates From Python List Using reduce() function
- Comparative Analysis Of Ways To Remove Duplicates From Python List
- Conclusion
- Frequently Asked Questions
- Think You Know How to Remove Duplicates? Take A Quiz!
Table of content:
- What Is Python List & How To Access Elements?
- What Is IndexError: List Index Out Of Range & Its Causes In Python?
- Understanding Indexing Behavior In Python Lists
- How to Prevent/ Fix IndexError: List Index Out Of Range In Python
- Handling IndexError Gracefully Using Try-Except
- Debugging Tips For IndexError: List Index Out Of Range Python
- Conclusion
- Frequently Asked Questions
- Avoiding ‘List Index Out of Range’ Errors? Take A Quiz!
Table of content:
- What Is the Python sort() List Method?
- Sorting In Ascending Order Using The Python sort() List Method
- How To Sort Items In Descending Order Using Python sort() List Method
- Custom Sorting Using The Key Parameter Of Python sort() List Method
- Examples Of Python sort() List Method
- What Is The sorted() List Method In Python
- Differences Between sorted() And sort() List Methods In Python
- When To Use sorted() & When To Use sort() List Method In Python
- Conclusion
- Frequently Asked Questions
- Take A Quick Python's sort() Quiz!
Table of content:
- What Is A List In Python?
- What Is A String In Python?
- Why Convert Python List To String?
- How To Convert List To String In Python?
- The join() Method To Convert Python List To String
- Convert Python List To String Through Iteration
- Convert Python List To String With List Comprehension
- The map() Function To Convert Python List To String
- Convert Python List to String Using format() Function
- Convert Python List To String Using Recursion
- Enumeration Function To Convert Python List To String
- Convert Python List To String Using Operator Module
- Python Program To Convert String To List
- Conclusion
- Frequently Asked Questions
- Convert Lists To Strings Like A Pro! Take A Quiz
Table of content:
- What Is Inheritance In Python?
- Python Inheritance Syntax
- Parent Class In Python Inheritance
- Child Class In Python Inheritance
- The __init__() Method In Python Inheritance
- The super() Function In Python Inheritance
- Method Overriding In Python Inheritance
- Types Of Inheritance In Python
- Special Functions In Python Inheritance
- Advantages & Disadvantages Of Inheritance In Python
- Common Use Cases For Inheritance In Python
- Best Practices for Implementing Inheritance in Python
- Avoiding Common Pitfalls in Python Inheritance
- Conclusion
- Frequently Asked Questions
- 💡 Python Inheritance Quiz – Are You Ready?
Table of content:
- What Is The Python List append() Method?
- Adding Elements To A Python List Using append()
- Populate A Python List Using append()
- Adding Different Data Types To Python List Using append()
- Adding A List To Python List Using append()
- Nested Lists With Python List append() Method
- Practical Use Cases Of Python List append() Method
- How append() Method Affects List Performance
- Avoiding Common Mistakes When Using Python List append()
- Comparing extend() With append() Python List Method
- Conclusion
- Frequently Asked Questions
- 🧠 Think You Know Python List append()? Take A Quiz!
Table of content:
- What Is A Linked List In Python?
- Types Of Linked Lists In Python
- How To Create A Linked List In Python
- How To Traverse A Linked List In Python & Retrieve Elements
- Inserting Elements In A Linked List In Python
- Deleting Elements From A Linked List In Python
- Update A Node Of Linked List In Python
- Reversing A Linked List In Python
- Calculating Length Of A Linked List In Python
- Comparing Arrays And Linked Lists In Python
- Advantages & Disadvantages Of Linked List In Python
- When To Use Linked Lists Over Other Data Structures
- Practical Applications Of Linked Lists In Python
- Conclusion
- Frequently Asked Questions
- 🔗 Linked List Logic: Can You Ace This Quiz?
Table of content:
- What Is Extend In Python?
- Extend In Python With List
- Extend In Python With String
- Extend In Python With Tuple
- Extend In Python With Set
- Extend In Python With Dictionary
- Other Methods To Extend A List In Python
- Difference Between append() and extend() In Python
- Conclusion
- Frequently Asked Questions
- Think You Know extend() In Python? Prove It!
Table of content:
- What Is Recursion In Python?
- Key Components Of Recursive Functions In Python
- Implementing Recursion In Python
- Recursion Vs. Iteration In Python
- Tail Recursion In Python
- Infinite Recursion In Python
- Advantages Of Recursion In Python
- Disadvantages Of Recursion In Python
- Best Practices For Using Recursion In Python
- Conclusion
- Frequently Asked Questions
- Recursive Thinking In Python: Test Your Skills!
Table of content:
- What Is Type Conversion In Python?
- Types Of Type Conversion In Python
- Implicit Type Conversion In Python
- Explicit Type Conversion In Python
- Functions Used For Explicit Data Type Conversion In Python
- Important Type Conversion Tips In Python
- Benefits Of Type Conversion In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Type Conversion? Take A Quiz!
Table of content:
- What Is Scope In Python?
- Local Scope In Python
- Global Scope In Python
- Nonlocal (Enclosing) Scope In Python
- Built-In Scope In Python
- The LEGB Rule For Python Scope
- Python Scope And Variable Lifetime
- Best Practices For Managing Python Scope
- Conclusion
- Frequently Asked Questions
- Think You Know Python Scope? Test Yourself!
Table of content:
- Understanding The Continue Statement In Python
- How Does Continue Statement Work In Python?
- Python Continue Statement With For Loops
- Python Continue Statement With While Loops
- Python Continue Statement With Nested Loops
- Python Continue With If-Else Statement
- Difference Between Pass and Continue Statement In Python
- Practical Applications Of Continue Statement In Python
- Conclusion
- Frequently Asked Questions
- Python 'continue' Statement Quiz: Can You Ace It?
Table of content:
- What Are Control Statements In Python?
- Types Of Control Statements In Python
- Conditional Control Statements In Python
- Loop Control Statements In Python
- Control Flow Altering Statements In Python
- Exception Handling Control Statements In Python
- Conclusion
- Frequently Asked Questions
- Mastering Control Statements In Python – Take the Quiz!
Table of content:
- Difference Between Mutable And Immutable Data Types in Python
- What Is Mutable Data Type In Python?
- Types Of Mutable Data Types In Python
- What Are Immutable Data Types In Python?
- Types Of Immutable Data Types In Python
- Key Similarities Between Mutable And Immutable Data Types In Python
- When To Use Mutable Vs Immutable In Python?
- Conclusion
- Frequently Asked Questions
- Quiz Time: Mutable vs. Immutable In Python!
Table of content:
- What Is A List?
- What Is A Tuple?
- Difference Between List And Tuple In Python (Comparison Table)
- Syntax Difference Between List And Tuple In Python
- Mutability Difference Between List And Tuple In Python
- Other Difference Between List And Tuple In Python
- List Vs. Tuple In Python | Methods
- When To Use Tuples Over Lists?
- Key Similarities Between Tuples And Lists In Python
- Conclusion
- Frequently Asked Questions
- 🧐 Lists vs. Tuples Quiz: Test Your Python Knowledge!
Table of content:
- Introduction to Python
- Downloading & Installing Python, IDLE, Tkinter, NumPy & PyGame
- Creating A New Python Project
- How To Write Python Hello World Program In Python?
- Way To Write The Hello, World! Program In Python
- The Hello, World! Program In Python Using Class
- The Hello, World! Program In Python Using Function
- Print Hello World 5 Times Using A For Loop
- Conclusion
- Frequently Asked Questions
- 👋 Python's 'Hello, World!'—How Well Do You Know It?
Table of content:
- Algorithm Of Python Program To Add To Numbers
- Standard Program To Add Two Numbers In Python
- Python Program To Add Two Numbers With User-defined Input
- The add() Method In Python Program To Add Two Numbers
- Python Program To Add Two Numbers Using Lambda
- Python Program To Add Two Numbers Using Function
- Python Program To Add Two Numbers Using Recursion
- Python Program To Add Two Numbers Using Class
- How To Add Multiple Numbers In Python?
- Add Multiple Numbers In Python With User Input
- Time Complexities Of Python Programs To Add Two Numbers
- Conclusion
- Frequently Asked Questions
- 💡 Quiz Time: Python Addition Basics!
Table of content:
- Swapping in Python
- Swapping Two Variables Using A Temporary Variable
- Swapping Two Variables Using The Comma Operator In Python
- Swapping Two Variables Using The Arithmetic Operators (+,-)
- Swapping Two Variables Using The Arithmetic Operators (*,/)
- Swapping Two Variables Using The XOR(^) Operator
- Swapping Two Variables Using Bitwise Addition and Subtraction
- Swap Variables In A List
- Conclusion
- Frequently Asked Questions (FAQs)
- Quiz To Test Your Variable Swapping Knowledge
Table of content:
- What Is A Quadratic Equation? How To Solve It?
- How To Write A Python Program To Solve Quadratic Equations?
- Python Program To Solve Quadratic Equations Directly Using The Formula
- Python Program To Solve Quadratic Equations Using The Complex Math Module
- Python Program To Solve Quadratic Equations Using Functions
- Python Program To Solve Quadratic Equations & Find Number Of Solutions
- Python Program To Plot Quadratic Functions
- Conclusion
- Frequently Asked Questions
- Quadratic Equations In Python Quiz: Test Your Knowledge!
Table of content:
- What Is Decimal Number System?
- What Is Binary Number System?
- What Is Octal Number System?
- What Is Hexadecimal Number System?
- Python Program to Convert Decimal to Binary, Octal, And Hexadecimal Using Built-In Function
- Python Program To Convert Decimal To Binary Using Recursion
- Python Program To Convert Decimal To Octal Using Recursion
- Python Program To Convert Decimal To Hexadecimal Using Recursion
- Python Program To Convert Decimal To Binary Using While Loop
- Python Program To Convert Decimal To Octal Using While Loop
- Python Program To Convert Decimal To Hexadecimal Using While Loop
- Convert Decimal To Binary, Octal, And Hexadecimal Using String Formatting
- Python Program To Convert Binary, Octal, And Hexadecimal String To A Number
- Complexity Comparison Of Python Programs To Convert Decimal To Binary, Octal, And Hexadecimal
- Conclusion
- Frequently Asked Questions
- 💡 Decimal To Binary, Octal & Hex: Quiz Time!
Table of content:
- What Is A Square Root?
- Python Program To Find The Square Root Of A Number
- The pow() Function In Python Program To Find The Square Root Of Given Number
- Python Program To Find Square Root Using The sqrt() Function
- The cmath Module & Python Program To Find The Square Root Of A Number
- Python Program To Find Square Root Using The Exponent Operator (**)
- Python Program To Find Square Root With A User-Defined Function
- Python Program To Find Square Root Using A Class
- Python Program To Find Square Root Using Binary Search
- Python Program To Find Square Root Using NumPy Module
- Conclusion
- Frequently Asked Questions
- 🤓 Think You Know Square Roots In Python? Take A Quiz!
Table of content:
- Understanding the Logic Behind the Conversion of Kilometers to Miles
- Steps To Write Python Program To Convert Kilometers To Miles
- Python Program To Convert Kilometer To Miles Without Function
- Python Program To Convert Kilometer To Miles Using Function
- Python Program to Convert Kilometer To Miles Using Class
- Tips For Writing Python Program To Convert Kilometer To Miles
- Conclusion
- Frequently Asked Questions
- 🧐 Mastered Kilometer To Mile Conversion? Prove It!
Table of content:
- Why Build A Calculator Program In Python?
- Prerequisites To Writing A Calculator Program In Python
- Approach For Writing A Calculator Program In Python
- Simple Calculator Program In Python
- Calculator Program In Python Using Functions
- Creating GUI Calculator Program In Python Using Tkinter
- Conclusion
- Frequently Asked Questions
- 🧮 Calculator Program In Python Quiz!
Table of content:
- The Calendar Module In Python
- Prerequisites For Writing A Calendar Program In Python
- How To Write And Print A Calendar Program In Python
- Calendar Program In Python To Display A Month
- Calendar Program In Python To Display A Year
- Conclusion
- Frequently Asked Questions
- Calendar Program In Python – Quiz Time!
Table of content:
- What Is The Fibonacci Series?
- Pseudocode Code For Fibonacci Series Program In Python
- Generating Fibonacci Series In Python Using Naive Approach (While Loop)
- Fibonacci Series Program In Python Using The Direct Formula
- How To Generate Fibonacci Series In Python Using Recursion?
- Generating Fibonacci Series In Python With Dynamic Programming
- Fibonacci Series Program In Python Using For Loop
- Generating Fibonacci Series In Python Using If-Else Statement
- Generating Fibonacci Series In Python Using Arrays
- Generating Fibonacci Series In Python Using Cache
- Generating Fibonacci Series In Python Using Backtracking
- Fibonacci Series In Python Using Power Of Matix
- Complexity Analysis For Fibonacci Series Programs In Python
- Applications Of Fibonacci Series In Python & Programming
- Conclusion
- Frequently Asked Questions
- 🤔 Think You Know Fibonacci Series? Take A Quiz!
Table of content:
- Different Ways To Write Random Number Generator Python Programs
- Random Module To Write Random Number Generator Python Programs
- The Numpy Module To Write Random Number Generator Python Programs
- The Secrets Module To Write Random Number Generator Python Programs
- Understanding Randomness and Pseudo-Randomness In Python
- Common Issues and Solutions in Random Number Generation
- Applications of Random Number Generator Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python's Random Module? Prove It!
Table of content:
- What Is A Factorial?
- Algorithm Of Program To Find Factorial Of A Number In Python
- Pseudocode For Factorial Program in Python
- Factorial Program In Python Using For Loop
- Factorial Program In Python Using Recursion
- Factorial Program In Python Using While Loop
- Factorial Program In Python Using If-Else Statement
- The math Module | Factorial Program In Python Using Built-In Factorial() Function
- Python Program to Find Factorial of a Number Using Ternary Operator(One Line Solution)
- Python Program For Factorial Using Prime Factorization Method
- NumPy Module | Factorial Program In Python Using numpy.prod() Function
- Complexity Analysis Of Factorial Programs In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Factorials In Python? Take A Quiz!
Table of content:
- What Is Palindrome In Python?
- Check Palindrome In Python Using While Loop (Iterative Approach)
- Check Palindrome In Python Using For Loop And Character Matching
- Check Palindrome In Python Using The Reverse And Compare Method (Python Slicing)
- Check Palindrome In Python Using The In-built reversed() And join() Methods
- Check Palindrome In Python Using Recursion Method
- Check Palindrome In Python Using Flag
- Check Palindrome In Python Using One Extra Variable
- Check Palindrome In Python By Building Reverse, One Character At A Time
- Complexity Analysis For Palindrome Programs In Python
- Real-World Applications Of Palindrome In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Palindromes? Take A Quiz!
Table of content:
- Best Python Books For Beginners
- Best Python Books For Intermediate Level
- Best Python Books For Experts
- Best Python Books To Learn Algorithms
- Audiobooks of Python
- Best Books To Learn Python And Code Like A Pro
- To Learn Python Libraries
- Books To Provide Extra Edge In Python
- Python Project Ideas - Reference
- Quiz To Rehash Your Knowledge Of Python Books!
Python Logical Operators, Short-Circuiting & More (With Examples)
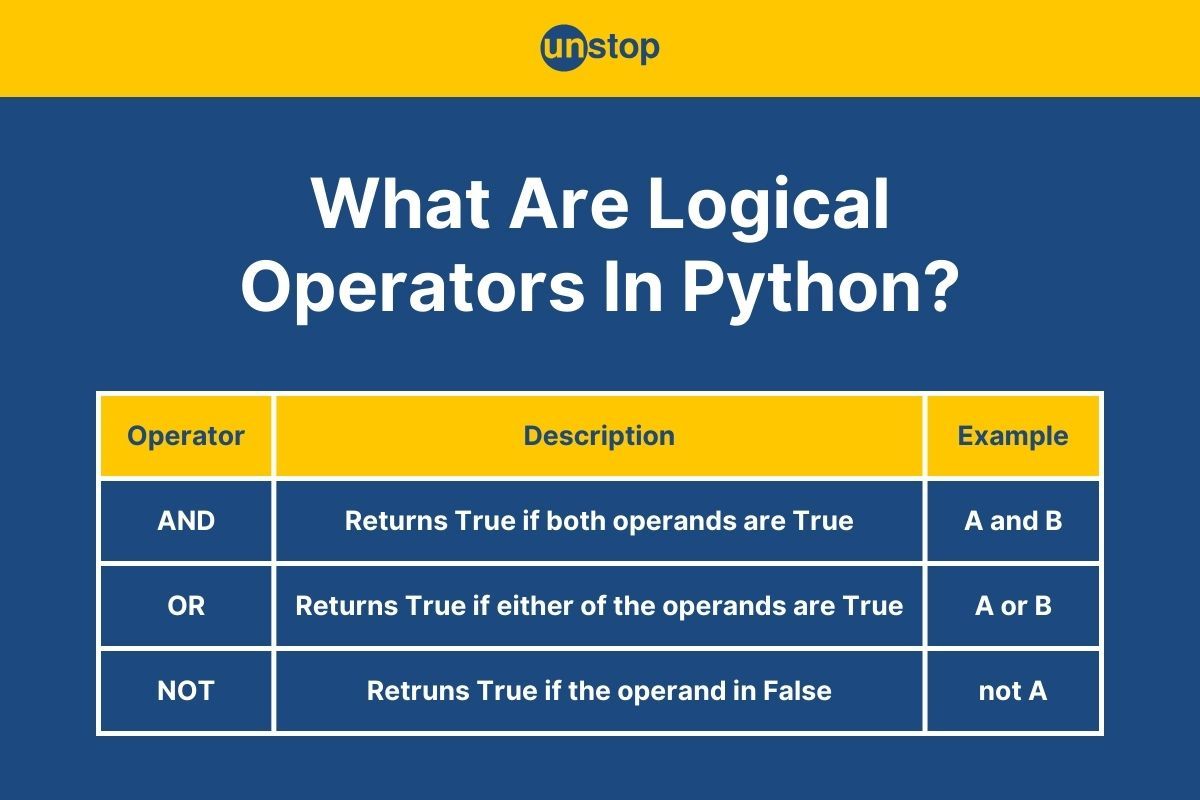
Logical operators in Python are essential components of the language's decision-making and control flow structures. These operators allow developers to perform logical operations on Boolean values, which are either True or False. Logical operators are crucial for creating conditional statements and expressions, enabling the implementation of complex decision-making processes within Python programs. In this article, we'll look at logical operators in Python programming, their purposes, syntax, and practical applications.
What Are Logical Operators In Python?
Python, like other programming languages, is built around Boolean logic. It enables programmers to do comparisons, conditional statements, and standard algorithms. Python comparison operators include the greater than (>) and equals to (==) symbols, while logical operators include AND, OR, and NOT. In this section, we will examine Boolean logic and expressions and how to utilize Python's Boolean operators.
Python's logical operators are essential for working with boolean data because they enable the execution of logical operations. Programmers use boolean data, which is represented by the values True or False, to make decisions. Logical operators improve the flexibility and complexity of logical expressions by offering a variety of ways to mix, edit, or examine boolean values.
Python has three main logical operators, i.e., and, or, and not. Here is a brief definition of their roles:
- AND (and) Operator: The and operator yields True if both operands are True; else, it returns False.
- OR (or) Operator: If at least one of the operands is True, the or operator returns True; otherwise, it returns False.
- NOT (not) Operator: The NOT operator returns the operand's inverse boolean value. If the operand is True, it does not return True; otherwise, it returns False.
Now, let's take a look at each of these Python logical operators individually and in greater detail.
The AND Python Logical Operator
Two boolean expressions can be combined in Python using the logical AND operator. It only returns True if both of the expressions it links are True; otherwise, it returns False. The AND Python logical operator is frequently used when you want to establish an expression that needs more than one of the conditions to be satisfied.
- Two boolean expressions, sometimes known as operands, are evaluated by the AND operator.
- Only in the event that both operands are True does it return True.
- The result is False if at least one operand is False.
Syntax Of AND Logical Operator In Python
result = operand1 and operand2
Here,
- The two boolean expressions or values you wish to combine are operand1 and operand2.
- The and keyword indicates that we are using the AND operator.
Working Of AND Python Logical Operator
In Python, you may combine two or more boolean statements with the AND logical operator. If any of the operands being combined with AND are not true, it evaluates to False and yields True. A detailed explanation of the AND operator's behavior is as follows:
1. Start: Start with a logical expression that uses the AND operator.
2. Assess the Initial Condition: Start by evaluating the first condition in the expression. The expression's overall result is False if the first condition is False, hence the other conditions don't need to be evaluated. If the result of the first expression is True, then evaluate the second expression.
3. Check for Short-Circuiting: The operator checks or verifies the following condition if the first condition is True. The total outcome is True if the second condition is likewise true. Short-circuiting happens if the second condition is False, and the interpreter doesn't evaluate the other conditions since the outcome will always be False.
4. Repeat for Additional Conditions: The procedure is repeated if the expression has more criteria. Each subsequent condition is assessed only when the preceding requirements are true.
5. End: When the logical action involving the application of the AND operator is finished, the flow comes to an end.
Truth Table Of AND Python Logical Operator
The truth table given below summarises the result the AND operator will give depending upon the result of the two operands/ expressions.
Input/ Operand A |
Input/ Operand B |
Output(A AND B) |
0 |
0 |
0 |
0 |
1 |
0 |
1 |
0 |
0 |
1 |
1 |
1 |
Code Example:
#Initializing a variable to store age, then using relational operator to check adulthood
age = 25
is_adult = age >= 18
#Initializing income variable and then checking employment status
income = 50000
has_job = income > 0
#Checking eligibility for load using AND operator
eligible_for_loan = is_adult and has_job
print(f"Is adult: {is_adult}")
print(f"Has job: {has_job}")
print(f"Eligible for loan: {eligible_for_loan}")
Output:
Is adult: True
Has job: True
Eligible for loan: True
Code Explanation:
In this Python example, we use several variables representing different aspects of a person's eligibility for a loan.
- As mentioned in code comments, we first create a variable age and initialize it with the value 25.
- Then, we use the greater than equals to comparison operator in a boolean expression to see if the value of age is more than or equal to 18. The outcome of this comparison is assigned to the is-adult variable.
- Next, we create a variable income to represent the person's income and give it an initial value of 50,000.
- Once again, we use a boolean expression to check if the person has a job or not, i.e., if income>0. The result of this comparison is assigned to the has-job variable.
- After that, we use the AND logical operator to combine the conditions represented by is_adult and has_job.
- The result, assigned to the eligible_for_loan variable, indicates whether the person meets both criteria for loan eligibility.
- Finally, we print out the values of is_adult, has_job, and eligible_for_loan to show whether the person is an adult, has a job, and is eligible for a loan, respectively.
The outcome of the logical AND operation between is_adult and has_job is set to the eligible_for_loan variable. Only when all requirements are satisfied (both variables are True) is the applicant qualified for a loan.
Since both requirements are satisfied in this instance, eligible_for_loan is True. This explains how the AND Python logical operator can be used to combine numerous criteria in a program.
Using Python Logical Operator AND With Boolean Expressions
In Python, the logical operator AND serves as a pivotal tool for constructing conditional statements based on multiple Boolean expressions.
- When applied to Boolean expressions, the and operator evaluates to True only when all the individual conditions are true.
- This means that each condition/ expression linked by AND must be satisfied for the entire expression to be considered true.
- This functionality allows developers to create more nuanced and precise conditions within their programs.
- For instance, within an if statement, the AND operator can be employed to ensure that multiple criteria, such as numeric ranges or string conditions, are met simultaneously before executing a specific block of code.
The use of AND fosters the creation of intricate decision-making structures, enhancing the robustness and reliability of Python programs by demanding the fulfillment of all specified conditions for the overall logical expression to be deemed true.
Code Example:
# Example using the AND operator with boolean expressions
age = 25
is_student = True
# Check if the person is both a student and their age is between 18 and 25
if is_student and (18 <= age <= 25):
print("This person is a student between the ages of 18 and 25.")
else:
print("This person does not meet the specified criteria.")
Output:
This person is a student between the ages of 18 and 25.
Explanation:
In this Python code example, we have a scenario involving a person's age and whether they are a student or not.
- We initialize the variable age with a value of 25, representing the age of an individual.
- Another variable, is_student, is set to True, indicating whether the person is currently a student.
- The subsequent if-else statement checks two conditions using the logical AND operator:
- The first condition (is_student) checks if the person is a student, and
- The second condition (18 <= age <= 25) verifies if the person's age falls between 18 and 25 (inclusive).
- If both conditions are true, the code inside the corresponding if block is executed, printing a string message- This person is a student between the ages of 18 and 25.
- If either of the conditions is false, the code inside the else block is executed, printing the message- This person does not meet the specified criteria.
The OR Python Logical Operator
In Python, the logical OR operator is employed to combine two boolean expressions. It returns True if at least one of the expressions it connects is True; otherwise, it returns False. The OR operator is commonly used when you want to establish an expression that requires at least one of the conditions to be satisfied.
- Two boolean expressions, also known as operands, are assessed by the OR operator.
- It returns True if at least one operand is True.
- The result is False only if both operands are False.
Syntax Of OR Python Logical Operator
result = operand1 or operand2
Here,
- The two boolean expressions or values you wish to combine are operand1 and operand2.
- The or keyword indicates that we are using the OR operator.
Note- Regardless of operand2's value, the complete expression evaluates to True if operand1 is True. The entire expression evaluates to the value of operand2 if operand1 is False. The whole expression evaluates to False if operand2 is likewise False; otherwise, it evaluates to True.
Working Of OR Python Logical Operator
As mentioned before, the logical OR operator returns true if either of the expressions is true. Given below is a detailed explanation of its working mechanism:
1. Start: Use the or operator in a logical phrase to get started.
2. Evaluate the First Condition: The expression's first condition is assessed. The expression's overall result is True if the first condition is true and the other conditions don't need to be evaluated.
3. Check for Short-Circuiting: In case the first condition is false, short-circuiting takes place. The OR operator recognizes that since at least one of the conditions is true, the overall expression will result in True regardless of the other conditions.
4. Skip Subsequent Conditions: The interpreter neglects to consider the remaining requirements as they are aware that the final outcome has already been decided. The main idea behind short-circuiting is to reduce calculation time when the outcome is obvious.
5. End: When the logical action involving the application of the or operator is finished, the flow comes to an end.
Truth Table For OR Python Logical Operator:
Input A |
Input B |
Output (A OR B) |
0 |
0 |
0 |
0 |
1 |
1 |
1 |
0 |
1 |
1 |
1 |
1 |
Now, let’s take a look at an example showing the implementation of the logical OR operator in Python.
Code Example:
#Declaring and initializing two integer variables
x = 5
y = 10
#Using Python logical operator OR to test two conditions simultaneously
result = (x > 3) or (y < 5)
print(result)
Output:
True
Code Explanation:
In the simple Python program-
- We assign values 5 and 10 to the integer variables x and y, respectively.
- Next, we combine two conditions, i.e., the first x>3 and the second y<5, using the logical OR operator.
- Since x is bigger than 3, the first condition is satisfied in this instance.
- The second criterion, which is false, determines if the value of y is smaller than 5.
- In this case, the outcome of the logical OR operation is assigned to the result variable, which is true. This is because the logical OR operator only needs one of the requirements to be true.
- Lastly, we print the value of the result variable using the print() method.
Using Python Logical Operator OR With Boolean Expressions
In Python, the logical operator OR is used to combine boolean expressions in a way that the overall expression evaluates to True if at least one of the individual boolean expressions is True.
- It returns False only when all the conditions are False.
- This allows for the creation of more complex conditions by checking multiple criteria simultaneously.
- For example, consider a situation where you want to determine whether a person is eligible for a discount at a store.
Using OR, you can create a boolean expression that checks if the person is a student or a senior citizen and if either condition is met, the overall expression evaluates to True, indicating eligibility for the discount.
Code Example:
age = 25
is_student = True
discount_eligible = is_student or age >= 60
if discount_eligible:
print("You are eligible for a discount!")
else:
print("Sorry, you are not eligible for a discount.")
Output:
You are eligible for a discount!
Explanation:
In the example Python program-
- We begin by defining two variables - age and is_student. The variable age is set to the value 25, representing the age of an individual.
- The variable is_student is assigned the value True, indicating whether the person is currently a student.
- Next, we use the OR logical operator to combine two conditions to determine if the person is eligible for a discount.
- The first condition is the outcome is_student.
- The second condition is age >= 60, which checks if the person is older than 60.
- This operation results in True if the person is a student (is_student is True) or if their age is 60 or higher (age >= 60).
- The outcome of the operation is assigned to the variable discount_eligible.
- Following that, we have an if statement that evaluates the value of discount_eligible. If it is True, the program prints a message indicating that the individual is eligible for a discount.
- Conversely, if discount_eligible is False, the program prints a message conveying that the person is not eligible for a discount.
In summary, this piece of code showcases the use of the OR Python logical operator to create a condition for discount eligibility.
The NOT Python Logical Operator
Python's logical NOT operator (not) is a unary operator that negates a boolean expression's truth value. It returns the opposite boolean value given a single boolean operand. Not returns False if the operand is False and True if the operand is True.
Syntax Of NOT Python Logical Operator
result = not operand
Here,
- The boolean phrase or value that you wish to negate is given by the term operand.
- The not keyword represents the NOT operator.
- The result variable is where we store the output of the not operation.
Working Of NOT Logical Operator In Python
The working mechanism of the NOT logical operator in Python programming language is as follows:
1. Start: Start with a condition or boolean expression that you wish to use the not operator to negate.
2. Evaluate the Condition: Analyze the first boolean scenario. For example, (x > 0), where x is a variable, may be this.
3. Apply not Operator: Apply the NOT operator to the boolean condition's outcome. Using not will provide False if the first condition was True. Using not will yield True if the initial condition was False.
4. Result: The original boolean condition is negated as a final outcome of applying not.
Truth Table For NOT Python Logical Operator:
Input A |
Output (NOT A) |
0 |
1 |
1 |
0 |
Code Example:
a = 10
b = 5
# Using the not operator with an expression
result = not (a > b)
print(result)
Output:
false
Code Explanation:
In the above code,
- We declare two variables a and b, and assign the values 10 and 5 to them, respectively.
- Then, we use the NOT operator along with the equation a>b. Since a exceeds b, the equation (a > b) evaluates to True.
- As a result, the NOT expression is evaluated as False since the operator applied to it.
- The outcome of the NOT logical operator is stored in the variable result.
- Lastly, we display the value of the result variable using the print() method.
Using Python Logical Operator NOT With Boolean Expressions
In Python, the logical operator NOT is used to negate the truth value of a boolean expression. It is a unary operator that reverses the boolean outcome of the expression it precedes. When applied to a true statement, it results in False, and vice versa. For instance, if you have a condition that evaluates to True, using not before the condition will make it False. Conversely, if the condition is initially False, applying not will make it True.
Code Example:
# Example using NOT with boolean expressions
is_sunny = True
# Applying NOT to reverse the truth value
is_raining = not is_sunny
print("Is it raining?", is_raining)
Output:
Is it raining? False
Explanation:
In this Python code example-
- We begin by assigning the boolean value True to the variable is_sunny, indicating that the weather is sunny.
- Following this assignment, the script utilizes the logical operator NOT to reverse the truth value of is_sunny.
- The result of this operation is then stored in a new variable named is_raining. Essentially, if is_sunny is initially True, applying not turns it into False, suggesting that it is not sunny, and hence, it is raining.
- Finally, a print statement is employed to display the question "Is it raining?" along with the value of is_raining.
When executed, the script outputs whether it is raining or not based on the logical inversion of the original sunny condition, providing a response such as: Is it raining? False, in the given example.
Short-Circuiting Evaluation Of Python Logical Operators
Short-circuiting is a behavior exhibited by logical operators in Python, including AND and OR, where the second operand is not evaluated if the outcome can be determined based on the evaluation of the first operand alone.
- This optimization is particularly useful for improving performance and efficiency in certain scenarios.
- For the AND operator, if the first operand is False, the overall expression will be False regardless of the value of the second operand.
- Therefore, the second operand is not evaluated, and the result is False.
- Similarly, for the OR operator, if the first operand is True, the overall expression will be True regardless of the value of the second operand.
- Consequently, the second operand is not evaluated, and the result is True.
This behavior can be advantageous when dealing with complex expressions or when the second operand involves computationally expensive operations. By short-circuiting, unnecessary computations are avoided, leading to improved performance. Below is an example to illustrate short-circuiting in Python logical operators.
Code Example:
# Short-circuiting with the AND operator
def expensive_function():
print("This function is expensive!")
return False
result_and = False and expensive_function() # Since the first operand is False, the second operand is not evaluated
print("Result with AND:", result_and)
# Output: Result with AND: False
# Short-circuiting with the OR operator
result_or = True or expensive_function() # Since the first operand is True, the second operand is not evaluated
print("Result with OR:", result_or)
# Output: Result with OR: True
Output:
Result with AND: False
Result with OR: True
Explanation:
- In the provided Python code, there are two main operations that demonstrate the short-circuiting behavior of logical operators and and or.
- We start by declaring a function named expensive_function() in the Python code. This function, when called, prints a message stating that it is an expensive operation and then returns the boolean value False.
- Next, we encounter the first operation using the and operator: False and expensive_function(). In this case, the first operand is False.
- According to the short-circuiting behavior of the and operator, the second operand (expensive_function()) is not evaluated since the overall result of the and operation is already known to be False when the first operand is False. Therefore, the function expensive_function() is not executed, and the result of the entire expression is determined to be False.
- Moving on to the second operation involving the or operator: True or expensive_function(). Here, the first operand is True.
- As per the short-circuiting principle of the or operator, the second operand (expensive_function()) is not evaluated because the overall result of the or operation is guaranteed to be True when the first operand is True. Consequently, the function expensive_function() is not called, and the result of the entire expression is established as True.
- Finally, the code prints the results of both operations. The output shows "Result with AND: False" and "Result with OR: True," affirming the expected outcomes based on the short-circuiting behavior of the logical operators.
Precedence of Logical Operators In Python
Logical operators are used in programming to execute logical operations on boolean data. In Python, logical operators have a specific precedence, which determines the order in which they are evaluated when used together in an expression. Understanding operator precedence is crucial for correctly interpreting and predicting how expressions will be evaluated. Given below is the precedence of logical operators in Python, from highest to lowest:
- NOT (!): The not operator has the highest precedence. It is a unary operator that negates the value of its operand.
- AND (&&): The and operator has a lower precedence than not. It is a binary operator that returns True only if both operands are True.
- OR (||): The or operator has the lowest precedence among logical operators. It is a binary operator that returns True if at least one of the operands is True.
When more than one operator is in use, Python ranks them according to a predetermined hierarchy:
Operator |
Precedence |
NOT (!) |
Highest Precedence |
AND (&&) |
Medium Precedence |
OR (||) |
Lowest Precedence |
The highest precedence operator is used to group expressions first, then the groupings for lesser precedent operators, and so on.
Note:
It's important to note that parentheses can be used to override the default precedence and explicitly specify the order of evaluation. Expressions within parentheses are evaluated first. If there are nested parentheses, the innermost expressions are evaluated first.
Let's consider two examples to illustrate the precedence of logical operators in Python. The first demonstrates normal precedence implementation, while in the second example, we see how to override the precedence hierarchy of Python's logical operators.
Code Example 1:
# Example with logical operator precedence
#Normal precedence hierachy is followed
result = not True and False or True and not False
print(result)
Output:
True
Explanation:
In this example, the expression involves NOT, AND, and OR operators. The NOT operator has the highest precedence, followed by AND and OR. The result will be True, as the expression evaluates as follows:
- not True is False (due to the NOT operator).
- False and False is False (due to the AND operator).
- True and not False is True (due to the AND and NOT operators).
- False or True is True (due to the OR operator).
Code Example 2:
# Example using parentheses to override precedence
result = not (True and False) or (True and not False)
print(result)
Output:
True
Explanation:
In this example, we use parentheses to override the default precedence and explicitly specify the order of evaluation. The expression inside the innermost parentheses is evaluated first, and then the outer ones are considered. The result will be True, as the expression evaluates as follows:
- True and False is False (inside the parentheses).
- not False is True (inside the parentheses).
- True and True is True.
- not (True and False) is True.
- True or True is True.
These examples highlight how understanding and using operator precedence, along with parentheses, can help control the order of evaluation in complex expressions.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
How Does Python Calculate Truth Value?
In Python, an expression or object's truth value depends on its boolean context. An item in boolean context is either regarded as "truthy" or "falsy." These truth values are necessary for conditional statements and boolean operations. It is essential to comprehend the Python truth value calculation process to write legible and efficient code. Python follows these steps to determine an expression's truth value:
1. Constants: The unchanging elements of predetermined truth values apply to True and False. For example:
x = 5
y = 0
result = not (x > 10) and (y > 10)
print(f"Result: {result}")
Output:
Result: True
Explanation:
In this code,
- We assign the value 5 to the variable x and the value 0 to the variable y.
- Then, we evaluate whether x is not greater than 10 (which is true) and whether y is greater than 10 (which is false).
- The expression results in True and False, which is False. Therefore, when we print the result, it outputs False.
2. Numeric Types: Numerical types have truth values. Examples of these include integers and floats. Any number greater than zero is regarded as true, and zero as false. For example:
# Define numerical values
integer_value = 10
float_value = 3.14
zero_value = 0
# Check truth values using bool() function
integer_truth = bool(integer_value)
float_truth = bool(float_value)
zero_truth = bool(zero_value)
# Print truth values
print(f"Integer Value: {integer_value}, Truth Value: {integer_truth}") # Output: Integer Value: 10, Truth Value: True
print(f"Float Value: {float_value}, Truth Value: {float_truth}") # Output: Float Value: 3.14, Truth Value: True
print(f"Zero Value: {zero_value}, Truth Value: {zero_truth}") # Output: Zero Value: 0, Truth Value: False
Output:
Integer Value: 10, Truth Value: True
Float Value: 3.14, Truth Value: True
Zero Value: 0, Truth Value: False
Explanation:
In the above code,
- We assign numerical values to variables integer_value, float_value, and zero_value (10, 3.14, and 0, respectively).
- Using the bool() function, we determine the truth values of these variables (integer_truth, float_truth, and zero_truth).
- Then, we print the original values and their corresponding truth values.
3. Sequences (Strings, Lists, Tuples, etc.): Sequences that are not empty are regarded as true, whereas those that are empty are regarded as false. For example:
# Define sequences
non_empty_string = "Hello"
empty_string = ""
non_empty_list = [1, 2, 3]
empty_list = []
non_empty_tuple = (1, 2, 3)
empty_tuple = ()
# Check truthiness using bool() function
non_empty_string_truth = bool(non_empty_string)
empty_string_truth = bool(empty_string)
non_empty_list_truth = bool(non_empty_list)
empty_list_truth = bool(empty_list)
non_empty_tuple_truth = bool(non_empty_tuple)
empty_tuple_truth = bool(empty_tuple)
# Print truth values
print(f"Non-empty String: {non_empty_string}, Truth Value: {non_empty_string_truth}") # Output: Non-empty String: Hello, Truth Value: True
print(f"Empty String: {empty_string}, Truth Value: {empty_string_truth}") # Output: Empty String: , Truth Value: False
print(f"Non-empty List: {non_empty_list}, Truth Value: {non_empty_list_truth}") # Output: Non-empty List: [1, 2, 3], Truth Value: True
print(f"Empty List: {empty_list}, Truth Value: {empty_list_truth}") # Output: Empty List: [], Truth Value: False
print(f"Non-empty Tuple: {non_empty_tuple}, Truth Value: {non_empty_tuple_truth}") # Output: Non-empty Tuple: (1, 2, 3), Truth Value: True
print(f"Empty Tuple: {empty_tuple}, Truth Value: {empty_tuple_truth}") # Output: Empty Tuple: (), Truth Value: False
Output:
Non-empty String: Hello, Truth Value: True
Empty String: , Truth Value: False
Non-empty List: [1, 2, 3], Truth Value: True
Empty List: [], Truth Value: False
Non-empty Tuple: (1, 2, 3), Truth Value: True
Empty Tuple: (), Truth Value: False
Explanation:
In the above code,
- We define various sequences, including non-empty and empty strings, lists, and tuples.
- We then use the bool() function in the code to check the truthiness of these sequences and print their original values along with their truth values.
4. Mappings (Dictionaries): A dictionary that is not empty is regarded as truthful, whereas one that is empty is regarded as false. For example:
# Define non-empty and empty dictionaries
non_empty_dict = {'key': 'value'}
empty_dict = {}
# Check truth values using bool() function
non_empty_truth = bool(non_empty_dict)
empty_truth = bool(empty_dict)
# Print truth values
print(f"Non-empty Dictionary: {non_empty_dict}, Truth Value: {non_empty_truth}") # Output: Non-empty Dictionary: {'key': 'value'}, Truth Value: True
print(f"Empty Dictionary: {empty_dict}, Truth Value: {empty_truth}") # Output: Empty Dictionary: {}, Truth Value: False
Output:
Non-empty Dictionary: {'key': 'value'}, Truth Value: True
Empty Dictionary: {}, Truth Value: False
Explanation:
In the above code block,
- We define a non-empty dictionary (non_empty_dict) with a key-value pair and an empty dictionary (empty_dict).
- The code then uses the bool() function to check the truthiness of these dictionaries and prints their original values along with their truth values.
- This code, therefore, demonstrates that a non-empty dictionary evaluates to True while an empty dictionary evaluates to False.
5. Custom Objects: By using the __bool__ or __len__ methods, custom objects can specify their truth value. The item is by default regarded as truthy if neither is declared. For example:
class TruthyObject:
def __bool__(self):
return True
class FalsyObject:
def __bool__(self):
return False
# Testing the truthiness of instances
print(bool(TruthyObject())) # Output: True
print(bool(FalsyObject())) # Output: False
Output:
True
False
Explanation:
In the above code block-
- We define two classes, TruthyObject and FalsyObject, both of which have a __bool__ method that determines their truth value.
- TruthyObject's __bool__ method always returns True and FalsyObject's __bool__ method always returns False.
- Then, we use the bool() function to check the truth values of instances of these classes and print the results.
- This demonstrates how custom classes in Python may specify how they behave for truth values.
6. Logical Operations: Depending on the truth value, the logical operations (and, or, not) return one of the operands. For example:
# Define operands
operand1 = True
operand2 = False
# Logical AND operation
result_and = operand1 and operand2 # True if both operands are True, otherwise False
# Logical OR operation
result_or = operand1 or operand2 # True if at least one operand is True, otherwise False
# Logical NOT operation
result_not_operand1 = not operand1 # True if operand1 is False, otherwise False
result_not_operand2 = not operand2 # True if operand2 is False, otherwise False
# Print results
print(f"Logical AND: {operand1} and {operand2} is {result_and}") # Output: Logical AND: True and False is False
print(f"Logical OR: {operand1} or {operand2} is {result_or}") # Output: Logical OR: True or False is True
print(f"Logical NOT of {operand1} is {result_not_operand1}") # Output: Logical NOT of True is False
print(f"Logical NOT of {operand2} is {result_not_operand2}") # Output: Logical NOT of False is True
Output:
Logical AND: True and False is False
Logical OR: True or False is True
Logical NOT of True is False
Logical NOT of False is True
Explanation:
In the above code block-
- We define two operands, operand1 and operand2, with boolean values (True and False).
- Then, we perform logical operations on these operands: logical AND, logical OR, and logical NOT.
- result_and is True only if both operand1 and operand2 are True, otherwise it is False.
- result_or is True if at least one of operand1 or operand2 is True, otherwise it is False.
- result_not_operand1 is the logical NOT of operand1, which is True if operand1 is False, and vice versa.
- result_not_operand2 is the logical NOT of operand2, which is True if operand2 is False, and vice versa.
- Finally, we print the results of these logical operations.
You can build more expressive and logical code by being able to foresee and influence how Python evaluates truth values by being aware of these processes. When the goal is not immediately evident, it is usually a good idea to utilize boolean comparisons since explicit is preferable to implicit.
Final Note On How AND & OR Python Logical Operators Work
The logical operators AND and OR in Python are fundamental and indispensable tools for combining and evaluating Boolean expressions within conditional statements. Understanding how these operators work is crucial for writing effective and expressive code.
Python Logical AND Operator (and)
The AND operator returns True only if all the individual conditions it combines are True. If any one of the conditions is False, the entire expression is evaluated as False. This means that all conditions must be satisfied for the overall expression to be considered True.
Procedure for Evaluation:
- The AND operator returns True only if all the conditions it combines are True.
- If any of the conditions is false, the entire expression evaluates to be false.
- It requires all conditions to be satisfied for the overall expression to be considered True.
Example:
x = 5 y = 10 result = (x > 0) and (y < 20) print(result)
Output:
True
Explanation: In this example, both conditions (x > 0) and (y < 20) are True, so the overall result is True.
2. Logical OR (or):
Conversely, the OR operator returns True if at least one of the individual conditions it combines is True. It evaluates to False only if all the conditions are False. This means that any one True condition is sufficient for the overall expression to be considered True.
Evaluation Procedure:
- The OR operator returns True if at least one of the conditions it combines is True.
- It evaluates to False only if all the conditions are False.
- The OR operator can be used to create conditions where only one of the multiple conditions needs to be True for the entire expression to be True.
Example:
a = 15 b = 30 result = (a > 10) or (b < 25) print(result)
Output:
True
Explanation: Here, the condition (b < 25) is False, even though (a > 10) is True. Since at least one condition is True, the overall result is True.
Conclusion
In conclusion, Python logical operators play a fundamental role in shaping the logical flow of programs and decision-making processes within a program. They allow developers to express complex conditions and control the execution of their code snippet based on the truth values of boolean expressions. The AND operator requires both operands to be True for the overall expression to be True, while the OR operator needs at least one operand to be True. On the other hand, the NOT operator negates the boolean value of its operand.
Combining these logical operators enables developers to create intricate conditional statements, making their code more flexible and robust. It is essential to understand short-circuit evaluation, a feature of logical operators in Python, where the second operand is only evaluated if necessary, optimizing performance and preventing unnecessary computations. By mastering Python logical operators, programmers can enhance the clarity and efficiency of their code, leading to more maintainable and readable applications.
Frequently Asked Questions
Q. What is == and != in Python?
The equality operator in Python is represented as ==. It's employed to compare two values and determine whether they are equal. The expression evaluates to True if the values on both sides of the operator are the same and to False otherwise. It can be explained with the following snippet:
5 == 5 # Evaluates to True because 5 is equal to 5
Conversely, the inequality operator is represented by !=. It is used to check whether two values are not equal. The expression evaluates to True if the values vary and to False if they are equal. It can be explained with the following snippet:
5 != 10 # Evaluates to True because 5 is not equal to 10
So, in summary, the equals to (==) operator checks for equality, while the not equal to (!=) operator checks for inequality.
Q. What is %= in Python?
The equation a = a % b can be shortened with the Python operator a %= b. This is a compound assignment operator that applies the modulus operation to both a and b and then returns the result to a. The remainder of dividing a by b is determined by the modulus operation. As an illustration, consider this:
x = 10
y = 3
x %= y # Equivalent to x = x % y
print(x)
Output:
1
Explanation:
In this example, x starts at 10, and the value of x is updated to reflect the remaining amount of x divided by y following the operation x %= y. This is because 10% 3 equals 1, and the %= operator is used to allocate the result back to x.
Q. What is 8 % 3 in Python?
The expression uses the modulo operator (%). It performs the modulus operation where the left operand (8) is divided by the right operand (3), and the residual is calculated. Thus, in Python:
result = 8 % 3
print(result)
#The output will be 2
This is because 8 divided by 3 is 2 with a remainder of 2. Therefore, the result of 8 % 3 is 2
Q. Is ++ allowed in Python?
No, Python does not support the increment operator, i.e., ++. The ++ operator is not available in Python to increase a variable by 1. For incrementing, you would instead use the += operator. As an illustration, consider the following:
x = 5
x += 1 # Increment x by 1
print(x)#The output will be 6
Explanation:
This is due to the fact that x starts at 5 and is subsequently increased by 1 via the += operator. As a result, x takes on the value 6, which is printed.
Q. What are logical operators and bitwise operators in Python?
In Python, many operations on binary integers and boolean values are carried out using two types of operators, i.e., bitwise operators and logical operators.
Logical operators: These are used to conduct logical conditions /operations on boolean variables. Common Python logical operators include:
- and: If both operands are True, then returns True.
- or: If at least one operand is True, returns True.
- not: Returns False in the case of a True operand and True in the case of a False operand.
Example:
x = True
y = Falseprint(x and y) # False
print(x or y) # True
print(not x) # False
Bitwise Operators: These operators work with the discrete bits that make up binary numbers. They are frequently employed in low-level programming and other scenarios requiring direct manipulation of binary data. In Python, the bitwise operators are:
- Bitwise AND (&): Executes an AND operation bitwise.
- Bitwise OR (|): Executes a bitwise OR procedure.
- Bitwise XOR (^): Executes an exclusive OR bitwise XOR operation.
- Bitwise NOT (~): Reverses the binary number's bits.
- Left Shift (<<): Shifts the bits of a binary number to the left.
- Right Shift (>>): Shifts the bits of a binary number to the right.
Example:
a = 5 # binary: 0101
b = 3 # binary: 0011
print(a & b) # 1 (binary: 0001)
print(a | b) # 7 (binary: 0111)
print(a ^ b) # 6 (binary: 0110)
print(~a) # -6 (binary: 11111010 in two's complement)
print(a << 1) # 10 (binary: 1010)
print(a >> 1) # 2 (binary: 0010)
Q. What does 3 dots mean in Python?
The three dots (...) are referred to as the Ellipsis literal in Python. The ellipsis has several applications and is frequently used as a placeholder in programming. The three dots (...) are a flexible and powerful tool that may be utilized in type hinting, multidimensional array representation, and slicing. The context in which they are employed determines their exact meaning. Typical applications include the following:
- Slice Notation: In slice notation, the ellipsis can be used to express a wide range when working with multidimensional objects or arrays. It means 'and so on' or 'omitted' in the context of indexing.
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9]
print(my_list[::2]) # Output: [1, 3, 5, 7, 9]
Ellipsis (...) can be used to succinctly describe the step of 2 in the slice indicated by the (::2) in the example above.
- Numpy and Other Libraries: The Ellipsis is used in libraries such as NumPy to indicate multiple colons in higher-dimensional array indexing.
import numpy as np
my_array = np.random.rand(3, 3, 3)
print(my_array[..., 0]) # Selects all elements along the last axis with index 0
Here, the shortcut... is used to indicate the number of colons required to access all dimensions other than the one that is designated.
- PEP 484 Type Hints: In type hinting, the 'Any' type is represented by the ellipsis.
def my_function(param: ...) -> ...:
# Function implementation
When you wish to say that the type is unknown or might be any type, you use this syntax.
Q. Which is not a logical operator?
The arithmetic operator is not a logical operator. Arithmetic operators perform mathematical operations, such as addition, subtraction, multiplication, and division. In Python, common arithmetic operators include addition (+), subtraction (-), multiplication (*), division (/), and others.
On the other hand, logical operators include AND, OR, and NOT. These Python logical operators are used to combine or manipulate boolean expressions in conditions and control flow statements.
Python Logical Operators Quiz– Test Your Knowledge!
Want to expand your understanding of Python concepts? Do read the following:
- 12 Ways To Compare Strings In Python Explained (With Examples)
- Python IDLE | The Ultimate Beginner's Guide With Images & Codes!
- Python String.Replace() And 8 Other Ways Explained (+Examples)
- How To Reverse A String In Python? 10 Easy Ways With Examples!
- Flask vs Django: Understand Which Python Framework You Should Choose
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Ujjwal Sharma 18 hours ago
Divyansh Shrivastava 1 week ago
Pranjul Srivastav 2 weeks ago
Anjali Nimesh 3 weeks ago
Vishwajeet Singh 1 month ago
SIRIVERU MAHESWARI 1 month ago