How To Reverse A String In Python? 10 Easy Ways With Examples!
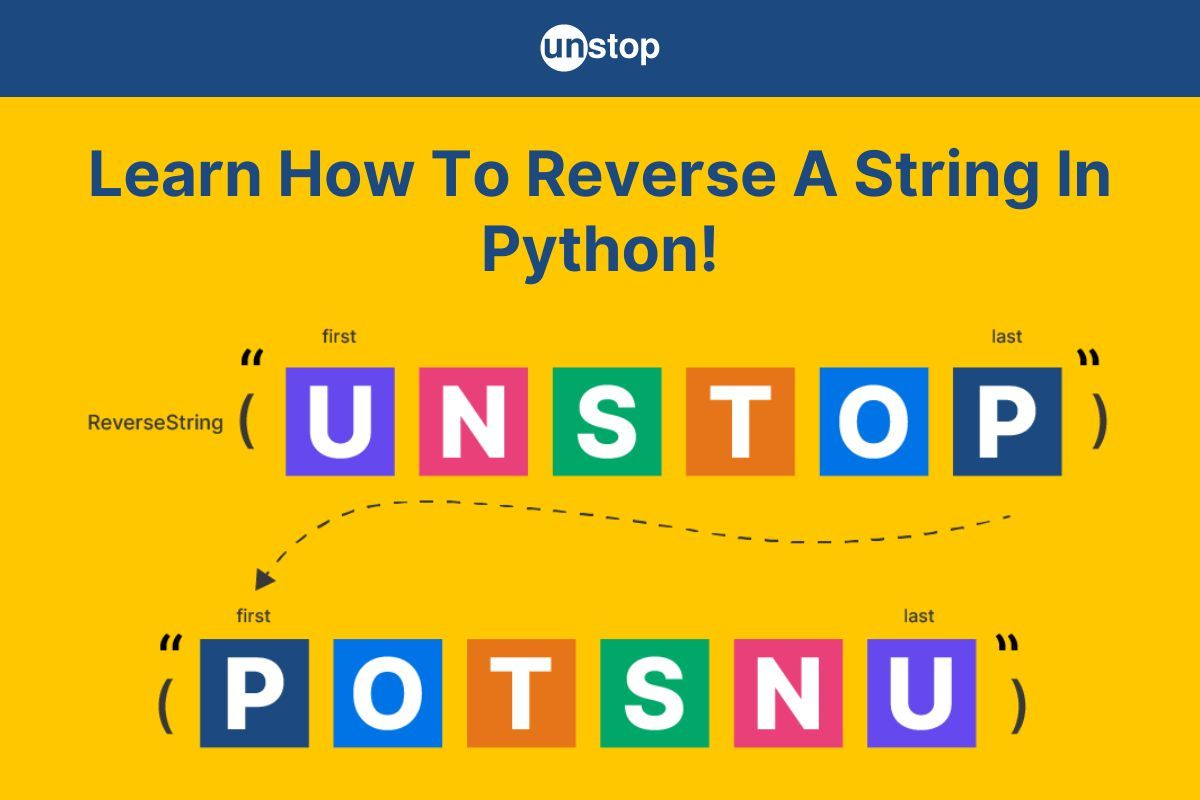
Strings are an essential data type in Python programming language that allows us to represent and manipulate textual data. There are multiple ways to manipulate and transform these strings, and one such interesting operation is the reversal of a string. This involves reversing the order of the characters in a string. In this article, we will explore how to reverse a string in Python, that is, the different approaches for string reversal, along with examples and their use cases.
Reversing strings can be useful in various scenarios, such as data analysis, text processing, and algorithmic problem-solving. Whether you're a beginner learning Python or an experienced developer looking to refresh your knowledge, this guide will provide you with a comprehensive understanding of how to reverse a string in Python effectively.
What Is A String In Python?
Before we dive into the intricacies of Python reverse strings, let's quickly recap what strings are in the first place. A string in Python is an immutable sequence of characters that can include letters, numbers, symbols, and even spaces. This immutability of strings means that once created, they cannot be altered. But they sure can be updated and manipulated, something we will discuss in this article.
The Python strings are declared by enclosing the characters within either single ('), double ("), or triple(""") quotation marks. You can perform various operations on strings, including concatenation, slicing, and formatting.
For example:
str1 = 'Hello, World!' #Here we are creating a Python string using single quotes
str2 = "Python is awesome" #Creating Python string using double quotes
str3 = '''Learning Python with Unstop''' #Python string with triple/ three single quotes
str4 = """I am Unstoppable!""" #Python string with triple/ three double quotes
Why Do We Need To Reverse Strings In Python?
Reversing a string in Python is an important programming concept with multiple applications across domains. Some of the most important reasons why you must know how to reverse a string in Python are:
- Palindrome Detection: Python string reversal in Ptyhon is a handy tool that allows us to check if it reads the same forwards and backward, aiding in palindrome detection.
- Encryption and cryptography: In certain encryption algorithms or cryptographic techniques, reversing a string can be a step in the process to enhance security or obfuscate the data.
- Algorithmic Problem Solving: Many coding challenges require reversing strings as part of their solution.
- String Encryption and Hashing: Some encryption or hashing algorithms involve reversing or manipulating strings in a specific way. While this is not a common practice or the most efficient technique for secure encryption, it can be an element in certain algorithms or techniques.
- User Interface (UI) and Display: In certain cases, you might want to display information in a user interface or output it in a reversed order for visual or formatting purposes. In such cases, knowing how to reverse these immutable data types can prove beneficial.
Challenges Of Reversing A String In Python
Reversing a string in Python programming may seem like a simple task, but there are certain string processing challenges and considerations that can arise. Especially when dealing with different scenarios or requirements. Here are some challenges we have to keep in mind when learning how to reverse a string in Python-
- String immutability: As we've mentioned before, strings in Python are immutable. This means that every time we reverse a string, we have to create a new string to hold the reversed version of our current string.
- Efficiency: The efficiency of the reverse string program will vary depending on which of the approaches to string reversal you choose. This will further impact the time taken in reversing strings, especially for larger strings.
- Algorithm Selection: Different algorithms vary in readability, complexity, and memory usage. It is, hence, crucial to choose the right algorithm/ reverse string methods for reversing strings in Python.
- Preserving Whitespace and Formatting: The reverse string methods in Python might not handle whitespace and formatting as expected. For instance, if there are leading or trailing spaces, reversing strings may not produce the desired result.
- Handling Unicode Characters: Strings in Python can contain Unicode characters. So, when reversing a string that includes such characters, it is important to ensure that the reversal maintains the correct order of characters, including any special or multibyte characters.
For Loop Method | How To Reverse String In Python?
One of the simplest methods to reverse a string in Python is by using a simple for loop. The for loop is a control flow statement that allows us to iterate over a sequence of elements such as lists, strings, or other iterable objects. Its versatility and simplicity make it a favorite choice for many programmers.
You can reverse a string in Python using a for loop with the following steps-
- Initialize an empty string to store the reversed version.
- Initiate a loop for iteration on string characters, i.e., characters of the initial string in reverse order using the range() function and len() function.
- Append each character to the reversed string.
Code Example:
Output:
Original String: Hello, World!
Reversed String: !dlroW ,olleH
Code Explanation:
In the Python program above-
- We first declare a variable called original_string (this is the string we want to reverse) and assign a value to it using double quotes.
- Then, we declare a second string variable called reversed_string and initialize it as empty using single quotes. This will hold the reversed string.
- Next, we initiate a for loop for iteration over the string and calculate the range for the string using the built-in functions range() and len().
- The inbuilt function len() gives us the length of the list/ string. The range() function generates a sequence of numbers that correspond to the indices of characters in the original string in reverse order. Its parameters are-
- The first parameter is set to len(original_string) - 1, which represents the last index of the original_string and marks the start of the range.
- The second parameter marks where to stop the iteration/ range, which is set to -1(exclusive). This indicates that the loop will continue until the index reaches 0, which is the first character of the string.
- The last parameter is the step, set to -1, indicating that the loop will decrease the index by 1 in each iteration.
- Inside the loop, we access each character of the original_string using the index i and concatenate it to the reversed_string. By doing this in reverse order, we gradually build the reversed version of the original string.
- We then use the print() statements to output both the strings to the console.
Time Complexity: O(n).
While Loop | How To Reverse A String In Python?
Another approach to reverse a string is by utilizing a while loop method. A while loop is a control flow structure in Python that repeatedly executes a block of code as long as a given condition is true. It allows us to create dynamic loops when the number of iterations is not known beforehand but depends on the evaluated condition. Let’s look at the steps to reverse a string using a while loop:
- Initialize an empty string to store the reversed version.
- Initialize a variable index to the length of the string minus one.
- Iterate through the string characters using the index variable, decrementing it after each iteration.
- Append each character to the reversed string.
Code Snippet:
Output:
Original String: Hello, World!
Reversed String: !dlroW ,olleH
Code Explanation:
In this example-
- We begin by declaring a variable original_string and initializing it with the string value- Hello, World!, using double quotes.
- Next, we declare another variable, reversed_string, and initialize it as an empty string. This will store the reversed version of our original/ current string.
- Then, we declare a third variable called index and initialize it to the value of the last position in the string. For this, we find the length of the string using the len() function and deduct 1 from it.
- After that, we define a while loop to iterate through the characters of the original string in reverse order and assign the result to the reversed_string variable.
- In each iteration, the current character is added to the reversed string, and the index is decremented to move to the previous character.
- After the loop completes iterations, we use the print() function to display both the original and reversed strings to the console.
Time Complexity : O(n)
Slicing Operator | How To Reverse A String In Python?
We've learned how to reverse a string in Python using explicit loops. In this section, we will discuss reversing strings in Python using a slicing operator. The slicing operator allows you to create a reversed copy of the string without explicitly using loops. Slicing a string in Python refers to extracting a portion or a substring from a given string.
Python's slicing operator, [::-1], provides an elegant and concise way to reverse a string. Given below is its syntax, followed by a proper code example with an explanation.
Slice Syntax:
reversed_string = original_string[::-1]
Here,
- original_string: This is the original string that you want to reverse.
- [::-1]: This is the slicing notation.
- The result of this slicing operation is a new string (reversed_string) that contains the characters of the original string in reverse order.
Code:
Output:
Original String: Hello, World!
Reversed String: !dlroW ,olleH
Code Explanation:
- The sample Python program above begins with the declaration of a variable original_string. We initialize this variable with the string value- Hello, World!.
- We define a second variable called reversed_string and use Python's slicing notation on original_string to initialize. This means-
- The slicing operator [::-1] is used to reverse the order of characters in the initial string.
- It starts the slicing operation of the original_string from the end of the string and moves to the beginning.
- In the process, it captures each character in the reverse order and stores the result in the reserved_string variable.
- Once the string slicing method finishes execution, we use two print() statements to display the original string as well as the reversed string.
Time complexity: O(n)
Slice Function | How To Reverse A String In Python?
The slice function in Python allows us to extract a portion of a sequence, such as a string or a list, by specifying the start, stop, and step values. It is a built-in function, and its functionality is synonymous with the slice operator. When reversing a string, we can leverage the slice function with a step value of -1 to create a reversed version of the original string.
Here are the steps to use the slice function:
- Start by defining the original string that you want to reverse.
- Create a slice object with a step value of -1 to indicate a reverse traversal of the entire string.
- Use the slice object as the index to the original string.
- Assign the reversed string to a new variable.
- Print the reversed string as the final output.
The syntax for the slice function is also referred to as the extended slice syntax because it is an extended/ elaborate version of the slice operator syntax.
The Slice Function Syntax is:
slice(start, stop, step)
Here,
- The function is represented by slice().
- The parameters of the function are- start, stop, and step, which represent the starting point/ index of the range, the endpoint/ index, and the decrement index, respectively.
Code Example:
Output:
!dlroW ,olleH
Code Explanation:
- We begin the example by defining a variable, original_string, and assign a string value to it using double quotes.
- Next, we declare another variable, reversed_string, which is used to store the reversed version of our initial string. We initialize this second string as follows-
- We use the slice() function to traverse the original string in reverse order.
- The parameters of the function are None, None, and -1. The None values indicate that we slice from the beginning to the end, and the -1 step value ensures a reverse order.
- This way, we retrieve the characters of the original string based on the reversed indices stored in the slice object.
- After the reversed string is assigned to the reversed_string variable, we use the print() function to display the result to the console.
Time complexity: O(n)
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
How To Reverse A String In Python With Recursion?
The concept of recursion is a powerful technique in programming, and it can also be employed to reverse a string. For this, we can define a function and recursively call it to add the last index character of the string on a loop. Then, store the result in a new string variable to get the reversed version of the string.
Here's how the recursive approach works:
- Define a function that recursively iterates on the string for its length.
- Base Case: If the length of the string is 0 or 1, return the string itself.
- Recursive Step: Call the function recursively with the substring excluding the first character and append the first character at the end.
Code Example:
Output:
!elbappotsnU ,olleH
Code Explanation:
- The code defines a function reverse_string_recursion that takes a string as input.
- In the function, it checks if the length of the input string is less than or equal to 1. If true, it returns the string as is.
- In the recursive case, the function calls itself with a substring starting from the second character and appends the first character at the end. This process continues until the base case is met.
- The example uses the recursive function to reverse the string 'Hello, Unstoppable!' and assigns the result to reversed_string.
- Finally, the reversed string is printed to the console using print(reversed_string).
Time Complexity: O(n). However, recursive methods have additional overhead due to function calls.
How To Reverse A String In Python Using Stack?
A stack is an abstract data type that follows the Last-In-First-Out (LIFO) principle. Imagine a stack of books placed on a table. The topmost book is easily accessible, while the books underneath cannot be reached directly.
Similarly, a stack data structure can be visualized as a vertical arrangement of elements, with only the topmost element accessible for operations. This makes stacks ideal for managing elements in a specific order. A stack supports two primary operations:
- Push: Adding an element to the stack.
- Pop: Removing the topmost element from the stack.
Here's how we can reverse a string using a Stack:
- Create an empty stack.
- Push each character of the string onto the stack.
- Pop each character from the stack and append it to a new string.
Code Example:
Output:
Original String: Hello, World!
Reversed String using Stack: !dlroW ,olleH
Code Explanation:
- We first define a variable, original_string, and assign the string value- Hello, World!, to it.
- Next, we define two variables, i.e., stack, which uses a list (stack) to simulate a stack data structure, and reversed_string, which we initialize as an empty string to store the reversed string.
- We then initiate a for loop, where each character from the original string is appended to the stack using the append() function.
- After that, we define a while-loop, which runs as long as the stack is not empty.
- Since the stack follows the Last-In-First-Out (LIFO) principle, inside this loop, characters are popped from the top of the stack and added to the reversed_string, effectively reversing the order of characters.
- We use the pop() function to pop the characters and the addition assignment operator to assign them to the reversed_string variable.
- Lastly, we use the print() statements in the code to print both the original and reversed strings.
Time Complexity: The time complexity of this approach is O(n), where n is the length of the string.
The reversed() Method | How To Reverse A String In Python?
In this section, we will discuss how to use the reversed() function to reverse a Python string. This inbuilt function can reverse any iterable object, including strings. But since strings in Python are immutable, we use this function indirectly. Here's how it can be applied:
- Define and assign a string variable.
- Use the built-in reversed() function on the original string to obtain a reverse iterator.
- Join the reversed characters using the join() method.
Code Example:
Output:
!dlroW ,olleH
Code Explanation:
- We declare and initialize a variable original_string with the value- Hello, World! This variable will be used as input for the reversed() function.
- Next, we declare another variable, reserved_function, and assign an empty string value to it. As mentioned in the code comments, this value is filled using the join() and reversed() function, as follows-
- The reversed() function returns a reverse iterator that allows us to iterate over the characters of the string in reverse order.
- To obtain the reversed string, we convert the reverse iterator to a list and then join the characters using the join() method.
- The join() method concatenates the characters without any separator between them, resulting in the reversed string.
- Finally, we print it using the print() statement.
Time Complexity: The time complexity of this approach for reversing strings is O(n), where n is the length of the string.
List Comprehension | How To Reverse A String In Python?
List comprehension provides a concise and expressive way to reverse a string. Here's how it can be utilized:
- Create a list comprehension that iterates through the characters of the string in reverse order.
- Join the reversed characters using the join() method.
Code Example:
Output:
Original String: Hi, I am Shivani!
Reversed String: !inavihS ma I ,iH
Code Explanation:
- We begin the code above by defining a function called reverse_string_list_comprehension that takes a string as input. Inside the function-
- A list comprehension is used to generate a list of characters from the original string in reverse order.
- In that, we use a for loop with the range() and length() functions to iterate over each character of the string in reverse order.
- This loop iterates over the characters and creates a list of these characters in reversed order.
- The reversed list of characters is then joined into a single string using the join() method.
- The reversed string is returned by the function.
- Then, we initialize a variable, original_string, with the string value- Hi, I am Shivani!.
- The function is called with the original string as an argument, and the result is assigned to reversed_string.
- The print statements output both the original and reversed strings.
Time Complexity: O(n)
Reverse A Python String Using Reverse() & Join() Functions
The built-in reverse() function, combined with the join() method, provides another way to reverse a string in Python. The reverse() function allows you to reverse the order of elements in a list, or more specifically, it reverses the elements in a sequence. When applied to a list, it modifies the original list in place, reversing the order of its elements.
The join() function is also a built-in method available for string objects in Python. It allows you to concatenate elements of an iterable, such as a list or a tuple, into a single string. It takes the iterable as an argument and returns a new string with the elements joined together with the specified separator.
The steps to use them for string reversal in Python are-
- Convert the string into a list using the list() function.
- Apply the reverse() function to reverse the list in-place.
- Use the join() method to concatenate the reversed characters.
Code Example:
Output:
Original String: Hello, Unstop!
Reversed String: !potsnU ,olleH
Code Explanation:
- We begin the code above by defining a function reverse_string_reverse_join that takes a string as input. Inside the function-
- A reversed_string variable is defined that applies the list() function on the original input string. This creates a list of the characters in this original string.
- Next, the elements of the list are reversed using the reverse() function.
- The list of characters is then joined and converted to a string using the join() function.
- This way, the function returns the reversed version of the original input string.
- Next, we declare and initialize the original_string variable with the value- Hello, Unstop!
- We then call the function we define, with the original_string as an argument, and the result is assigned to the reversed_string variable.
- Finally, we print both the strings using print() statements.
Time Complexity: O(n)
The reduce() Function | How To Reverse A String In Python?
We can use the reduction function, i.e., the reduce() function in Python, to reverse a string. For this, you have to import the reduce from functools. From there, you must use the functions.reduce() function along with a lambda function to concatenate the characters in reverse order. Here's an example:
Code:
Output:
Original String: Hello, Unstop Pro!
Reversed String using reduce(): !orP potsnU ,olleH
Code Explanation:
- We begin the example by importing the reduce function from the functools module.
- Then, we define the original_string variable, which we will be reversing.
- Next, we define the reversed_string variable to store the reversed version. Here-
- We use the reduce() function with a lambda function to concatenate characters in reverse order.
- The lambda function takes two arguments, i.e., acc, which represents the accumulated result, and char, which refers to the current character.
- The initial value for accumulation is an empty string, and the result is the reversed string.
- After the process is done, we output the values of both strings using print() statements.
Time Complexity: O(n)
Sorting Python Strings In Reverse Order
Apart from just reversing, sorting is also a common operation in programming that allows us to arrange elements in a specific order. In Python, sorting the characters of a string in reverse order can be accomplished using the built-in sorted() function. Here are the steps to sort the characters of a string-
- Define the original string that you want to sort in reverse order.
- Use the sorted() function with the reverse parameter set to true, to sort the characters of the string in descending order and store the result in a new variable.
- Convert the reversed list of characters into a string using the join() method, which concatenates the characters.
- Assign the reversed string to a new variable.
- Print the reversed string as the final output.
Code Example:
Output:
wroolllhed
Code Explanation:
- We begin by declaring a variable called original_string and assign the string value- hello world, to it.
- Next, we declare another variable called sorted_chars, which is used to store the list of characters from the initial string.
- It uses the sorted() function to create a sorted list of characters from the original_string in reverse order.
- The reverse=True parameter in sorted() ensures that the characters are sorted in descending order.
- The sorted characters are then joined into a single string using the join() method.
- The result is a reversed string obtained by sorting the characters in reverse order and sorted in descending order.
- The reversed string is printed using the print() statement. Note that the string is not completely reversed but reversed in descending order.
Time Complexity: In Python, the sorted() function has a time complexity of O(n log n), where n is the length of the input string.
Creating A Custom Reversible String In Python
We have already discussed a variety of ways to reverse a string in Python. Alternatively, you can also create a custom class to represent a reversible string object. Creating a custom reversible string in Python involves defining a class that extends a string-like class (e.g., str or UserString) and then implementing a method to reverse the string. Here's an example implementation:
Code:
Output:
!dlroW ,olleH
Code Explanation:
- Here, we import the UserString class from the collection module, as it contains all the functionality of a normal string.
- After that, we move on to define a class, ReversibleString, that inherits from UserString. In this class-
- We define a method called the reverse() function, which takes a string as input.
- The reverse method uses the slicing technique to reverse the characters of the input string (i.e., (self.data[::-1])) stored in the data attribute of the UserString base class.
- Next, we declare a variable, original_string, with the string value- Hello, World!.
- Then, we create an instance of the ReversibleString class, called reversible_string, and initialize it with the original_string.
- We then call the reverse() method on the reversible_string instance, and the result is assigned to the variable reversed_string.
- Finally, the reversed string is printed to the console using the print(reversed_string) statement.
Time Complexity: O(n)
Conclusion
There are multiple approaches for string reversal in Python, with each offering its own advantages and considerations. From using loops and recursion to leveraging built-in functions and string slicing methods, there are multiple ways to tackle this task. By understanding these methods, you can wield the power to manipulate strings in a versatile and efficient manner. So go ahead, embrace the art of reversing strings, and unlock new possibilities in your coding journey!
Frequently Asked Questions
Q. Can I reverse a string in-place in Python?
No. Python strings are immutable. This means they cannot be modified once declared. Therefore, reversing a string in place is not possible. However, a string can be reversed using several methods (such as loops, inbuilt function, extended string slicing, etc.) and assigned to the original string variable itself.
Q. Are there any limitations or considerations when reversing very long strings?
There are no limitations to reversing a string in Python, as such. But while reversing a long string, the following aspects have to be kept in mind-
- Memory: Processing long strings may require a lot of memory. Make sure your system meets the minimum memory requirements to perform such a reversal.
- Performance: Reversing a long string might also be computationally expensive.
These aspects have to be kept in mind when dealing with large-scale data and time-sensitive applications
Q. What is string reversal?
String reversal refers to the process of rearranging the characters of a string in reverse order. It involves flipping the order of the characters, so the first character becomes the last, the second character becomes the second-to-last, and so on.
Q. How do you reverse a string using reversed() in Python?
The built-in reversed() function in Python takes in a string as input and returns a reverse iterator of the string. This reverse iteration object can then be joined back to a single string using the join function. By doing so, we indirectly use the reversed() function to reverse a function, since we cannot directly reverse strings as they are immutable. Here is an example-
original_string = "Hello, World!"
reversed_string = ''.join(reversed(original_string))
Output:
!dlroW ,olleH
Q. Does reverse() work on strings?
Strings in Python are immutable, which means their content cannot be modified after creation. Therefore, there is no reverse() method directly available for strings in Python. The reverse() method is specifically a list method, and it reverses the elements of a list in place. If you want to reverse a string, there are several ways, like slicing, using a loop, using reversed(), and join() with which you can achieve the desired results.
Here is an example:
Output:
!dlroW ,olleH
In the example above, we have created a custom reverse function inside a class and then used that to reverse the string. However, you can also use the reverse list function indirectly to reverse a string. For that, you will first have to convert the string to a list, then reverse the list using the reverse list function. This reversed list can then, again, be converted to a string.
Q. What does the in-built function reversed() do in Python?
In Python, the reversed() function is an in-built function that returns a reverse iterator of a given iterable. It does not modify the original iterable but instead provides a way to iterate over its elements in reverse order. We use various methods like the slicing operator, slice function, extended slice technique, etc., to use the reversed() function on a string in Python.
Here's a simple example:
Q. How do you print in reverse order in Python?
To print a string or a sequence in reverse order in Python, you can use the easiest method i.e. slicing. Here's an example:
Output:
!nohtyP ,olleH
Q. How do you reverse a list in Python?
To reverse a list in Python, you can use the built-in Python reverse function, which reverses the order of elements in the list in-place. Alternatively, you can use the [::-1] slicing notation to slice string, and create a new reversed list.
Here are examples of both approaches:
Output:
[5, 4, 3, 2, 1]
Q. How do you reverse a number in Python?
One way to reverse a number in Python is to convert it to a string, reverse the string, and then convert it back to an integer. Here's a Python code for the same:
Output:
54321
Q. What is the fastest algorithm to reverse a string in Python?
In Python, the fastest algorithm to reverse a string is using slicing with [::-1]. This approach has an average time complexity of O(n), where n is the length of the string. It provides an efficient way to reverse strings without any additional overhead or complexity.
Code Snippet:
This method takes advantage of Python's powerful slicing capabilities and is optimized for string reversal.
By now, you must know all about how to reverse a string in Python. Here are a few other interesting topics you will surely enjoy reading:
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment