Python String.Replace() And 8 Other Ways Explained (+Examples)
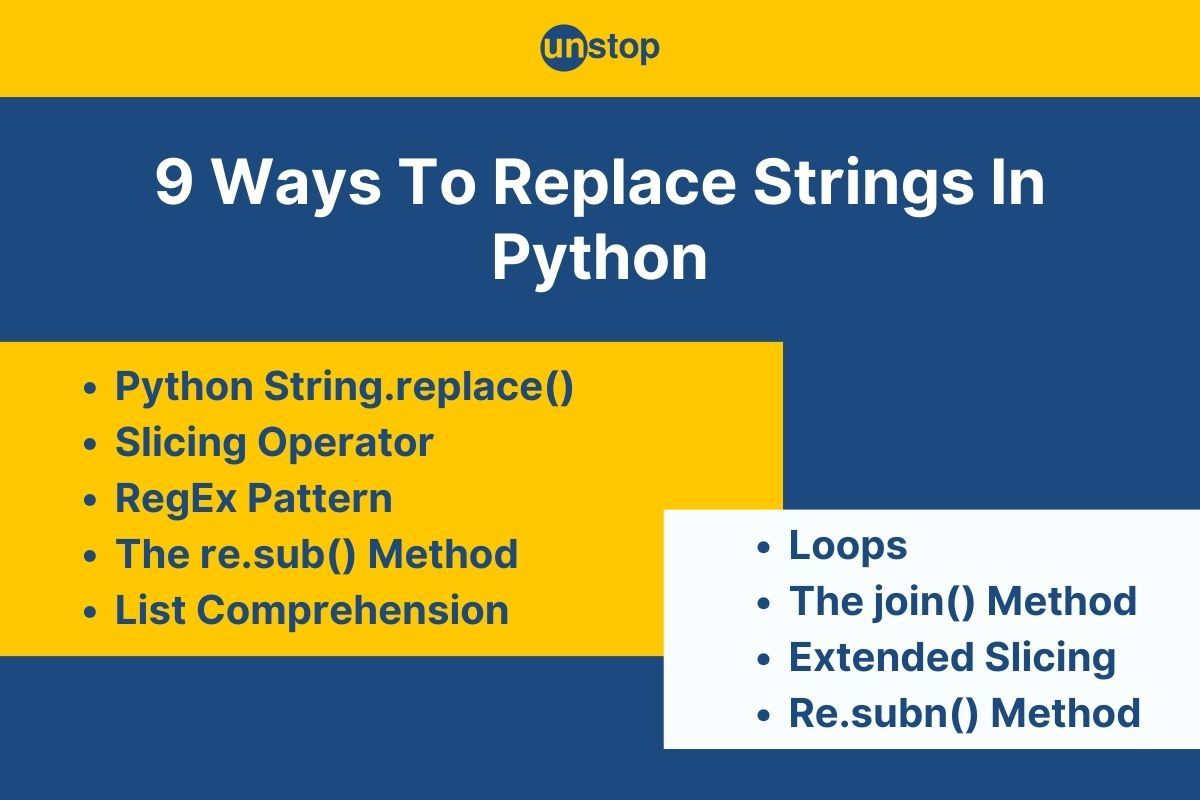
Python is a versatile and widely used powerful coding language that provides users with a rich set of tools such as strings. The strings are one of the most essential data types in any programming language since they allow us to work with textual data. One of the fundamental operations when working with strings is replacement. The Python programming language offers multiple methods to carry out the replacement operations. In this article we will explore them in detail, including the Python string replace() along with other useful/ advanced concepts and best practices.
What Is Python String?
In general programming, strings refer to collections of Unicode values or collections of characters. Many programming languages don't provide a string as their built-in data type and instead provide the string as an array of characters.
However, in Python, strings are a built-in data type that is also an immutable sequence of Unicode values. That is, a Python string, once created, can not be altered. But, it can be modified by creating a new string. This implies, that we can replace the string and store it in a new variable, but the original string can not be changed because of the immutable nature of the string.
The Need For Python String Replacement
Replacing a current string is a common operation in programming and can be necessary for various reasons. Here are some scenarios where string replacement in Python is commonly used:
- Text Manipulation and Transformation: The string replacement approach is often employed to transform or manipulate text data. For example, you might need to replace certain words, phrases, or patterns in a text document to modify its content.
- Data Cleaning and Preprocessing: You might encounter inconsistencies or unwanted special characters when working with data, especially user-generated or external data. In such cases, the Python string replacement methods can help clean and preprocess the data to make it more consistent and usable.
- Code Refactoring: In software development, you may need to refactor code, which involves restructuring or modifying existing code without changing its external behavior. Replacement strings can be used in such instances to update variable names, function calls, or other identifiers in the code.
- Template Rendering: In web development or other contexts where templates are used, a tuple of string replacement is often employed to substitute placeholders with actual values.
- Data Masking and Redaction: In scenarios where sensitive information needs to be protected, string replacement can be used to mask or redact certain parts of the data.
- Text Search and Highlighting: Python's string replacement methods can also be used to search for or mark specific occurrences of a substring within a larger text.
The Python String replace() Method
The Python string replace() is a built-in string method that returns a copy of a new string with all occurrences of substring- old replaced with a new substring. In other words, this string replace method is used to create a new string that contains the replacement of a substring from the initial input string. Note that the original string will be the same before and after the Python string replace method has been applied.
The Syntax for Python String Replace() is:
String.replace(Old, New, Count)
Here,
- The term string refers to the string variable on which the replacement is being applied.
- The replace() refers to the built-in Python function.
- The content inside the brackets are the parameters of the replace() function.
Parameters Of Python String.replace() Method
- Old: It is an old substring that you want to replace in the original single string.
- New: It refers to the new substring that you want to replace the old with. That is, this substring will be inserted in the place of the old input string.
- Count (optional): It specifies the maximum no. of times/ occurrences of substring that needs to be replaced with the new substring. However, this is an optional parameter. That is-
- The value is -1 by default, meaning all occurrences of the old substring will be replaced.
- If a count argument is given, then the first count occurrences are replaced with a new substring.
Return Value Of Python String.replace() Method
This function, when applied to a string, returns a new string that is similar to the old string but contains a new substring in place of the specific old substring.
When To Use Python String.replace() Method?
The replace() method in Python is used to replace occurrences of a specified substring with another substring in a given string. You might want to use the replace() method in various situations, such as:
- Replace Substring: Use Python string.replace() to substitute occurrences of a specified substring with another substring in a given string.
- Remove Substring: Employ replace() to eliminate a specific substring from a string.
- Case-insensitive Replacement: Make case-insensitive replacements by using the optional argument count to limit the number of occurrences replaced.
- Replace Multiple Substrings: Chain multiple replace() calls to replace or modify different substrings in a string.
- Conditional Replacement: Use replace() for conditional string replacement based on specific conditions or variables.
- Whitespace Removal: Utilize replace() to eliminate or modify whitespace in a string, such as removing spaces.
- HTML or XML Parsing: When working with HTML or XML strings, Python string replace() method can be handy for modifying or cleaning up specific tags or attributes.
Replace All Instances Of A Substring Using Python String.replace() Method
By now, you are familiar with the syntax for the Python string.replace() method and also how to use it. In this section, we learn how to replace all the instances of a substring with a new substring in a variable. In this case, we will not pass any value for the optional argument count. The count argument then will, by default be -1, which means all occurrences of the old string will be replaced.
Code:
Output:
Hello Unstop, hello Unstop!
Explanation:
In the sample Python program above-
- We first declare and initialize a variable called data, with the string value- Hello world, hello world! using the single quotes method.
- Here, we want to replace the substring- world, with the substring- 'Unstop'.
- So, we declare another variable called data_replaced and use the Python string.replace() method to assign value to it. Here-
- We call the replace() method on the data variable and pass parameters world (old) and Unstop (new) to it (i.e., data.replace()).
- Note that the count argument is missing since we want to replace all instances of the selected substring.
- The string replace() method returnsEvery occurrence of 'world' in the original string with 'Unstop'.
- After the replacement is done, the new string is assigned to the data_replaced variable.
- Next, we use the print() statement to display the resulting string to the console.
Replace Some Instances Of Substring Using Python String replace() Method
In this case, we pass the count argument along with the old and new variables as the parameters to the Python string replace() method. As a result of this, only a first-count number (i.e., the first count occurrences, where count refers to a specific number) of old substring instances will be replaced with new substrings.
Code:
Output:
Hello Unstop, hello Python!
Explanation:
The example Python program above shows how to replace a specific number of occurrences of a substring.
- We declare and initialize a variable, data, with the string value- Hello Python, hello Python!.
- Next, we declare another variable called data_replaced and assign the value to is as follows-
- We call the replace() function on the data variable where we want to replace a substring with a new one.
- The substring that we want to replace is Python, so it becomes the old parameter, and the substring with which we want to replace is Unstop, so that becomes our new parameter.
- The count of replacement we want to make is 1, so we pass the third count parameter as 1.
- Only the first occurrence of 'Python' is replaced with 'Unstop', and subsequent occurrences are not affected due to the specified limit of 1.
- The result of the string.replace() method is stored in the data_replaced variable.
- Finally, we print this value to the console using the print() function.
Replacing All Instances Of A Single Character With Python String.replace() Method
In this section, we will see how we can use the Python string.replace() method to replace all occurrences of a single character inside a string. We will pass the single character we want to replace as the old parameter and the new character as the new parameter.
Code:
Output:
Hellr Prrgram!
Explanation:
- In the Python code example above, we create a data variable and initialize it with the value- Hello Program!.
- Then, we create another variable, new_data, and assign the old string to it after replacing a single character. This is how it is done-
- We call the replace() method on the data variable by passing the characters o and r to it as parameters.
- Here, the character (o) is the old parameter since it is to be replaced by the character (r), which is the new parameter for the function.
- The count of replacement is equal to all occurrences, so we don't include the optional argument count.
- After replacement is done, the new string is stored in the new_data variable.
- Next, we use the print() statement to display this string to the console.
Multiple Replacements With Python String.replace() Method
We have already seen how to use the Python string.replace () method to replace a single character in a string and all the instances of a substring, as well as only a few instances of a substring. This brings about the question- is it possible to do multiple replacements in a string in a single instance?
Well, the answer is yes. We can use the replace method multiple times to replace the multiple words (substrings) in a string. Below is an example of the same.
Code:
Output:
Hello Unstoppables, Good Afternoon to all!
Explanation:
- We begin the Python program example above by creating and initializing the variable data with the string value- Hello Program, Good Morning to all!
- Here, we want to replace the word/ substring Unstop with the word Unstoppables on the generated string. And also, want to replace the substring- Morning with the substring- Afternoon.
- To do what is stated above, we declare a variable called data_replaced and assign it the string value by applying the built-in method replace() to the original string twice. In that-
- We first call the replace() method on the data variable and pass parameters Unstop (old) and Unstoppable (new) to it. This invokes the function which replaces the substring.
- After the first replacement is done, we call the function again, but this applies to the string generated after the first call. Here, we pass Morning (old) and Afternoon (old) as parameters to the function.
- When the whole replacement process is done, the new string is stored in the data_replaced variable.
- Lastly, we use the print() statement to display the new string with replaced substrings to the output window.
This section covers the various ways in which we can apply the Python string.replace() method. While this is the most commonly used method, it is not the only one. In fact, Python has numerous string replacement methods, which we will explore in the sections ahead.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Replace A Character In String Using For Loop In Python
A for loop in programming is used to iterate over any sequence structure (such as a string, list, tuple, set, etc.) until a condition is satisfied. You can make use of these loops for string replacement in Python.
For this, you must first declare a new empty string and then traverse through the string, one character at a time. If the character you want to replace is found, you can replace it with another character and concatenate it to the new string. Alternatively, you can also concatenate the same character to the new string.
Code:
Output:
Hellk, Wkrld!
Explanation:
In the sample Python program above, we use the for loop with an if-else statement for string replacement. Here-
- We begin by declaring a variable called data and assign the string value- Hello, World! to it.
- Then, we declare another variable of data type string called new_data and initialize it as an empty string.
- Now, say we want to replace every o character in the string with the character k. We create a for loop to get this done, which contains an if-else statement to mention the conditions. Here-
- The loop iterates over every character (represented by i) in the string.
- If the character being traversed is equal to o, then the if block is implemented, and the character is replaced by k.
- However, if the character is not equal to o, then the else block is implemented, which replaces the character with itself.
- The characters are concatenated to a new string after every iteration.
- The new string resulting after the loop completes execution is stored in the new_data variable.
- Finally, we print the new string to the console using the print() function. As you can see, the string printed is- Hellk, Wkrld!, which is the replacement string, i.e., the initial string, with one character replaced everywhere.
Python String Replacement Using Slicing Method
Another string replacement approach that we can use to replace substring in a string is the string slicing method. In this method, we extract some/all portions of the string using the slicing technique and then replace the snipped section with the desired substring.
That is, we extract the section before the word (substring) that we want to replace, add the new substring, and then extract the substring after the word we want to replace. Take a look at the example below, which will provide you with an explanation of the string slicing technique for string replacement in Python.
Code:
Output:
Hello Unstop, Good Morning!
Explanation:
- In the example, we first declare a string variable, data, and initialize it with a string value.
- We declare another string variable, new_data, and initialize it as follows-
- Using the slicing technique, we first extract the substring from index values 0 to 6, i.e., data[:6]. This retrieves the substring (Hello) from the original.
- Next, we concatenate it with a new substring (Unstop). This is the substring we want to replace (insert) into the old string.
- After that, we once again use the slicing operator and extract the substring from index value 11 to the end. This retrieves the substring (,Good Morning!), which we then concatenate with the rest of the string.
- This process generates a new string with the needed replacement, and it is stored in the new_data variable.
- Lastly, we print the new string to the console using the print() function.
Replace A Character At a Given Position In Python String
It is possible to replace a particular character at a given position in a string with a new character. For this, you will first have to convert the string to a list and then replace the character at the given position/ index value. After that is done, you can again convert the list to a string by using the built-in method join() in Python.
Code:
Output:
Helio
Explanation:
- We begin by initializing three variables, i.e., a string variable data with the value- Hello, an integer variable position with the value of 3, and a new_char variable with the character as a string value (i).
- The variable position represents the index position whose character we want to replace, and the character we want to replace it with is given by the new_char variable.
- Next, we convert the data string to a list using the list() function in Python and store it in the new_list variable. This generates a list where each character of the string is an element.
- After that, we use the indices feature of the list to replace the character at position value with the new character. This new list is updated in the new_list variable.
- Finally, we use the join() method, to join the elements of the list and convert it to a string. This new string is stored in the new_data variable.
- Lastly, we print it using the print() statement.
Replace Multiple Substrings With The Same String In Python
We already know how to replace multiple substrings in a string with the Python string.replace() method. But that is not the only way to make multiple replacements. Another way to get this done is by using the for loop. Here, we can replace multiple substrings with the same string by using a for loop where in each iteration, we replace the substring with the same string.
Code:
Output:
Hello program, this is program be program!
Explanation:
- We declare a variable data and initialize it with a string value, in this example.
- Next, we initialize a list of strings called substrings and add substrings from the data string to it. These include- world, Unstop, and ready.
- Then, we create a for loop, which takes an element of the substring list (represented by the character i) and traverses the data string.
- Inside the loop, we use the Python string.replace() method with two parameters, i.e., character i and a string program.
- When encountered with an element from the substring list in the main string, the loop replaces it with the word program.
- The loop results in a replacement string where a few substrings of the original string, are replaced with the string- program. This is stored back in the data variable.
- Finally, we print the value of the data variable using the print() function.
Python String Replacement Using Regex Pattern
In Python, regular expressions (regex) are a powerful tool for pattern matching and manipulation of strings. The re module provides support for regular expressions in Python. String replacement using regex patterns can be done using the re.sub() function, which replaces occurrences of a pattern in a string with a specified replacement.
The basic syntax of re.sub() method is:
re.sub(pattern, replacement, string)
Here,
- pattern: The regular expression pattern to search for in the string.
- replacement: The string to replace the matched pattern with.
- string: The input string in which the pattern will be searched and replaced.
Let's take a look at an example to see how the regex pattern is used for Python string replacement.
Code:
Output:
The price of the book is $XX. The price of the pen is $XX.
Explanation:
At the beginning of the Python code example, we import the Python re module to use the re.sub() function.
- We define a variable text and assign a string value (sentence) to it using the double quotes method.
- Next, as mentioned in the code comments, we define the regex (regular expression) pattern. Here, the character r indicates that the following text is a pattern.
- The pattern looks for dollar amounts in the format of a dollar sign followed by one or more digits (e.g., $20). That is, \$\d+, where d+ refers to one or more digits.
- Then, we define the replacement string variable, indicating that we want to replace the matched dollar amounts with the value $XX.
- Next, we call the re.sub() function and pass the three variables created above as parameters to it. Whenever this function finds any dollar amount in the text variable, it replaces it with '$XX'.
- The output of the re.sub() function is stored in the result variable.
- Finally, we use the print() statement to display the new string with replaced values to the console.
Python String Replacement Using List Comprehension & Join() Method
In Python, you can achieve string replacement using list comprehension and the join() method. This method is particularly useful when you want to perform the replacement on specific conditions or patterns in the string.
List Comprehension and Join Method
-
List Comprehension: You can use list comprehension to iterate over the characters of the string and conditionally replace certain patterns.
-
Join Method: The join() method is then used to concatenate the modified characters back into a string.
Code:
Output:
The c@t @nd the b@t @re t@king @ n@p on the m@t.
Explanation:
- In the sample Python code, we define a variable text and assign a string value to it.
- Then, we define two more variables, old_char, and new_char, and assign character values to them using single quotes. Here, the former is the character we want to replace, and the latter is one we want to replace the original with.
- Next, we use the list comprehension (i.e., []) and join() methods to iterate over each character in the text string. In that-
- We use an if-else statement inside [] to traverse the list of string's characters one by one.
- If the program encounters the value of old_character, it is replaced with the value of new_charcater.
- All other characters are left as is in the string.
- Then, the join() method concatenates the characters back into a string.
- The result of this operation is stored in the modified_text variable, which is displayed to the console using a print() statement.
Python String Replacement Using Callback With re.sub() Method
In Python, you can perform string replacement using a callback function with the re.sub() method. This approach allows you to define a function that takes a match object as an argument and returns the replacement string. The re.sub() method can accept a callback function as the replacement parameter. The callback function is called for every non-overlapping occurrence of the pattern, and it determines the replacement for each match.
Here's the basic syntax:
re.sub(pattern, callback_function, string)
where:
- pattern: The regular expression pattern to search for in the string.
- callback_function: A function that takes a match object as an argument and returns the replacement string.
- string: The input string in which the pattern will be searched and replaced.
Code:
Output:
ThIs Is A sAmplE strIng wIth sOmE vOwEls.
Explanation:
- We begin by importing a module called re that helps with working with regular expressions in Python.
- Next, we declare the text variable and assign a sentence to it that contains some vowels.
- Then, we create a function called replace_vowels(), which takes a match object as an argument and returns the upper version of the matched vowel. Inside this function-
- The match() method is used to check the string for specified characters.
- If a match for the characters is found, then the value is stored in the vowel variable inside the function.
- The function then returns the upper case of the respective vowel using the upper() method.
- The replace_vowels function hence returns the upper case version of the matched vowel. It will be passed as a parameter to the re.sub() function for Python string replacement.
- Next, we define a variable pattern, to which we assign a pattern value that looks for lowercase vowels (a, e, i, o, or u).
- We then call the re.sub() function to the text, passing the pattern, replace_vowels function, and the text variable as parameters.
- Whenever it finds a lowercase vowel, it calls our replace_vowels function to replace it with the uppercase version. The outcome of this operation is stored in the result variable.
- We then print the modified text, which now has all the lowercase vowels replaced with their uppercase versions, using the print() statement.
Python String Replacement With re.subn() Method
In Python, the re.subn() method is similar to re.sub() in that it looks for a regular expression pattern in the input string and replaces it with the specified substring. However, there is a difference between the two. Which is the re.subn() method of Python string replacement also returns the number of substitutions made in addition to the modified string.
It takes five parameters, with three similar to the ones in the re.sub() method. This function can be especially useful when you want to know how many replacements were performed. Let's look at its syntax and an example for a better understanding of the same.
The re.subn() method has the following syntax:
re.subn(pattern, replacement, string, count=0, flags=0)
Here,
- pattern: The regular expression pattern to search for in the string.
- replacement: The string to replace the matched pattern with.
- string: The input string in which the pattern will be searched and replaced.
- count (optional): Maximum number of occurrences to replace. If not specified or 0, all occurrences will be replaced.
- flags (optional): Additional flags that modify the behavior of the regular expression.
Code:
Output:
Modified string: The price of the book is $XX. The price of the pen is $XX.
Number of replacements: 2
Explanation:
The example above is similar to the one we discussed in the Regex pattern topic. However, there are a few additions.
- We begin by importing the Python re module and then initialize the text variable just like we did earlier.
- Then, we define a pattern variable and a replacement variable and assign them the values of the patterns we are looking for in the initial string and the replacement for it, respectively.
- The pattern (\$\d+) defines the rule (pattern), which states to find a dollar sign followed by one or more digits.
- Next, we call the re.subn() method on the text variable. This function-
- Searches for the pattern in the text variable.
- Whenever it finds any of the money amounts, it replaces them with the replacement variable, i.e., $XX.
- We display the new version of our sentence with the changes and also find out how many changes were made.
- The new string is stored in the result variable, and the number of replacements is stored in the num_repalcements variable.
- We then use the print() function twice to print the new string and the number of replacements (i.e., 2) to the console.
Conclusion
Understanding and mastering string replacement in Python is crucial for effective string manipulation in your programs. One of the best and most efficient ways to do this is the Python string.replace() method, using which we can replace a single character, multiple characters, a single substring, and multiple substrings in the original string. Other methods used for string replacement in a Pythonic way include the for loop, the regex pattern, the re.sub() method, the slicing technique, list comprehension, etc. Whether you're making simple substitutions or tackling more complex patterns with regular expressions, Python's string replacement capabilities have you covered!
Frequently Asked Questions
Q. What is the fastest way to replace a substring in Python?
One of the fastest ways to replace a substring within a raw string is by using the Python string replace() method. This method is a built-in string formatting method that efficiently replaces all occurrences of an old substring with a new substring. It is highly optimized and efficient for simple substring replacements.
Example:
original_string = "This is a sample string."
new_string = original_string.replace("sample", "modified")
Q. How do you replace only one occurrence of a string in Python?
To replace only one occurrence of a string in Python, you can use the string.replace() method with the optional count parameter set to 1. The count parameter specifies the maximum number of occurrences to replace. Here's an example:
Code:
Output:
This is an example string. Replacing only 1 occurrence.
Explanation:
- In this example, only the first occurrence of the substring one is replaced with the character string 1 because the count parameter is set to 1.
- If you set the count to a higher number, that number of occurrences would be replaced. If you omit the count parameter, all occurrences will be replaced.
Q. Can we replace a string by using its index, like string[1]=’a’?
No, you cannot replace a character in a string by directly accessing its index and assigning a new value. This is because strings in Python are immutable, which means their individual characters cannot be modified after the string is created. When you attempt to modify a character using the index assignment, it will result in a TypeError.
But there are many string replacement approaches that you can take, including the Python string replace() method, a for loop with an if-else statement, the join() method, the regex pattern, etc.
Q. How do you replace a number with a string in Python?
You can replace a number with a string/ substring by using the Python string replace() method. Note that here, you will treat the number as a character string instead of an integer variable. Below is an example of the same.
Code:
Output:
This is number nine
Q. How to replace substring with regex?
To replace a substring using the regex pattern/ expression, you first need to import the re-module in Python. This module consists of various functions, of which we will be using the re.sub() function. This function replaces all occurrences of the given regex pattern with the specified string.
The syntax for the regex pattern is:
re.sub(pattern, new, old)
Here,
- pattern- It is the regex pattern that we want to replace
- new- It is the new substring we want to replace with.
- old- It is the original string whose substring(s) we want to replace with the new one.
Q. What is an efficient way to replace multiple characters in a string?
One of the most efficient ways to replace multiple characters in a string is using the translate() method. This method allows you to replace multiple alphanumeric characters in a string. This method will use the translation table created from a dictionary to carry out Python string replacement.
Syntax:
string.translate(translation_table)
Code:
Output:
Hills Pzsgzam
Q. How can we replace a substring only if it occurs at the start or end of a string?
We can replace a substring, even if it occurs at the start or end of a string, by using two of the many Python string replacement methods. These are the startswith() and endswith() functions. The former checks if a given substring is at the start of the string, and the latter checks if the substring is at the end of the string. If and when these functions encounter the given substring, we can use the replace() method to replace the desired string.
Code Example:
Output:
Hi Unstop!
Q. What happens if we use the replace method on the non-string variables?
If you attempt to use the replace method on a non-string variable, Python will raise an AttributeError. The Python string replace method is specifically a string method, that is, it can only be used on string objects. Here's an example to illustrate this:
number = 42
# Attempting to use replace on a non-string variable will result in an AttributeError
# Uncommenting the line below will raise an error: 'int' object has no attribute 'replace'
# new_number = number.replace("2", "3")
In this example, the number is an integer variable, and calling replace on it will result in an error because the Python string replace() method is not defined for integers or other non-string types.
You might also be interested in reading the following:
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment