Python Variables | A Comprehensive Guide With Code Examples
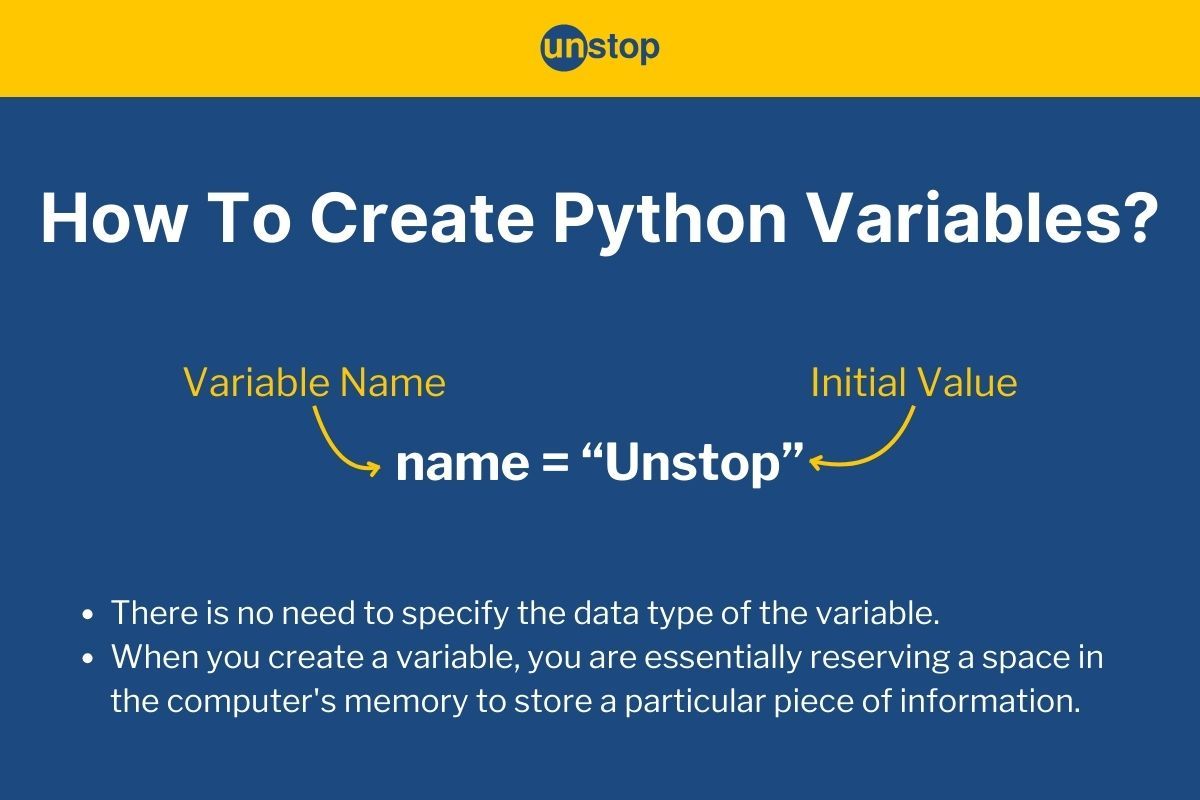
Embarking on the journey of Python programming opens the door to a world of possibilities, and at its core lies the concept of variables. These unassuming entities are the building blocks of code, the vessels that store and manipulate data. In this article, we delve into the fundamentals of Python variables, understanding how they work, exploring their dynamic nature, and gaining insights into best practices. Whether you're a novice coder or a seasoned developer, grasping the essence of Python variables lays a solid foundation for unlocking the language's potential.
What Is A Variable In Python?
In Python, a variable is a symbolic name or identifier that represents a memory location containing data, such as a numeric value, a string, or any other type of information. Unlike some other programming languages, Python is a dynamically typed language, meaning you don't need to explicitly declare the type of a variable. Instead, the type is inferred based on the value assigned to it.
- Variables play a crucial role in programming by allowing developers to store and manipulate data in their programs.
- When you create a variable, you are essentially reserving a space in the computer's memory to store a particular piece of information.
- The name given to the variable serves as a reference to access and modify this stored data.
- Variables can be reassigned to different values during the execution of a program, making Python a flexible and dynamic language.
For example, you might use a variable named count to store the number of items in a list or an integer object/ variable named age to represent a person's age. The ability to use variables makes it easier to write code that is both readable and adaptable to different situations.
Creating And Declaring Python Variables
A Python variable self-assigns the best possible datatype as you assign a value to it. You can simply type the name of the variable, say x equals 3, and it will assign a suitable data type (int in this case) to it. In case you want to change the variable type yourself, it's discussed later in the article.
Let’s take a look at an example of how to declare a variable in Python.
Code Example:
Output:
3
Explanation:
- We begin by assigning the variable x a value of 3. Note that we are not using any data type keywords since there is no need to do so in Python.
- Then, we use the print() function to display the value of x, which also automatically determines the datatype to print.
You can even assign other values, like words or decimal values, to a Python variable in a similar way.
Code Example:
Output:
Unstop
3.1415
Explanation:
- We begin the sample Python code above by declaring and initializing two variables, i.e., word with the value Unstop and decimal with the value 3.1415.
- We know Python automatically adjusts the variable datatype according to need. So, the data type of the variable word would be a string, and that of decimal would be floating-point/ float.
- Next, we use the print() statement to display the values of these variables. The print function can print any value of any built-in datatype just by passing the variable as a parameter.
Rules For Naming Python Variables
While the Python language automatically determines the data types of variables, there are a few rules we must adhere to when naming them. This is essential to creating valid variables. The rules are as follows:
- Valid Sequences of Characters: Variable names can consist of letters (both uppercase and lowercase), digits, and the underscore character (_). However, they cannot start with a digit or any special characters.
- Case-Sensitivity: Python is case-sensitive, so myVariable and myvariable would be considered different variables.
- Reserved Words: Avoid using Python reserved words (keywords) as variable names. Examples of reserved words include if, else, for, while, class, etc.
- No Spaces: Variable names cannot contain spaces. However, you can use underscores or camel case for readability (e.g., my_variable or myVariable). Camel case is where instead of using spaces, you capitalize every next word after the first one.
- Avoid Built-in Names: Avoid using names that are already used for built-in functions or objects in Python (e.g., print, str, list). Overriding built-in names can lead to unexpected behavior.
- Meaningful and Descriptive: Choose variable names that are meaningful and descriptive of the variable's purpose. This improves code readability, especially in complex codes.
- Snake Case for Variables: It's a common convention in Python to use snake case for variable names (e.g., my_variable). This means words are lowercase and separated by underscores.
- Camel Case for Classes: For class names, use the Pascal case, which is similar to the camel case. But the difference is that in the former, each word, including the first, is capitalized, i.e., starts with capital letters (e.g., MyClass).
- Length Limitation: While there's no strict limit, it's good practice to keep variable names reasonably short and descriptive. Long, descriptive names are preferred over short and cryptic ones.
How To Print Python Variables?
You can print the value of a Python variable by just using the print function. In Python, you do not need to specify any parameters in the print function (such as format specifiers or escape sequences). This is because the function automatically identifies the datatype and then prints it similarly to the assignment.
- You can also print any type of data type, including pictures, which, using the print function, gives a matrix.
- However, you can use custom print functions from relevant libraries to do it however you want.
- Also note that in Python, the print function automatically prints on the next line.
- You can also use the display function in case you are working on a jupyter notebook.
Let's take a look at an example where we print the values of variables of different data types.
Code Example:
Output:
5
10.213
Unstoppable
Explanation:
- We begin the simple Python program by declaring three variables, i.e., x, y, and z, of different data types.
- Of these, x is an integer variable with the value 5, y is a float variable with a value of 10.213, and z is a string variable with the value Unstoppable.
- The print(x), print(y), and print(z) statements output the values of x, y, and z respectively.
- None of the print statements contain any element other than the variable name. Since Python is dynamically typed, the function does the job despite the fact that the variables hold different types of data.
How To Delete A Python Variable?
The Python language has a built-in function called the del() function, which can be used to delete a variable from the memory. This delete function was basically built up for the purpose of resolving the insufficient memory issue that may be caused due to unnecessary variable values Python can be holding.
- It's specifically useful when dealing with large amounts of data.
- It can be used not only with variables but also for lists, arrays, object types, or even certain parts of lists and arrays.
- It throws an error if you try to print or use the variable again as it has been cleared from the memory, similar to how you delete any image permanently from your phone and you cannot access it anymore.
Below is an example to show the implementation of the same.
Code Example:
Output:
ERROR!
4
Traceback (most recent call last):
NameError: name 'var' is not defined.
Explanation:
- We start by setting up a variable called var and assigning it the value 4. Think of this like a container holding the value 4.
- Then, we use the print() function with var as a parameter to display its value on the terminal.
- After that, we use the del() function with var as a parameter. This tells the program to delete the variable var and clear its value from memory.
- Next, as mentioned in the code comments, when we again try to print the value of var, we get an error because we have already deleted it. The error message says that var is not defined, meaning it no longer exists.
Various Methods Of Variables Assignment In Python
In Python, you can assign values to variables using the assignment operator (=). The variable assignment allows you to store data of various types, such as numbers, strings, lists, or even complex objects like images or even complex machine learning models. It is made to ease the process of dealing with Python variables so one can focus more on the actual task or algorithm quickly.
Code Example:
Output:
[10, 3.14, 'Pradeep Goyal', 'Hello, world!', True, False, [1, 2, 3, 4, 5], ['apple', 'banana', 'orange'], 13.14, 'Dare2 Compete']
Explanation:
In the above code-
- We declare variables of different data types using the simple assignment operator as follows:
- Numeric variables (x and y) hold an integer and a float value, respectively.
- String variables (name and message) store text using both single and double quotes.
- Boolean variables (is_valid and has_error) represent truth values.
- List variables (numbers and fruits) store sequences of elements.
- Then, we combine all the variables created thus far in a list variable called whole_list.
- Next, we use the print() function to display the list to the console.
Here, we can see the versatile and adaptive nature of Python variables, especially lists. In that, they can store any variable of any datatype, no matter if it's a list itself mixed with other datatypes. Unlike other languages, python makes it so easy to work with this kind of variable with ease. We can even print them similar to a list without even writing a different function for printing each of them separately.
Declaration Without Initialization Of Python Variables
We have already seen how to declare and initialize Python variables in the section above. But note that there, we simultaneously declared and then initialized the variables. But what if you only want to declare a variable without giving it any initial value?
We know that Python is a dynamically typed language where we do not declare variables with an integer type. So, one way to get this done is by simply declaring the variable you want and initializing it with 0. However, it is not advised to use zero since it might lead to logical errors if the variable is not used properly.
The more sensible and technically correct process is using the word None to initialize a variable. It is a reserved keyword in Python, which means nothing is stored in it. And you can redeclare/ initialize the variable with the correct value whenever you want in the program.
Redeclaring Variables in Python
We already know that there is no need to explicitly declare variable type when changing the value to a different datatype. Python automatically adopts the datatype provided, and the declaration works even though it was done vaguely.
Code Example:
Output:
Unstop
Explanation:
- In the example above, we first assign a variable x with the value of 5. So, x initially holds the integer object/ value 5.
- We then change the value assigned to x, i.e., redeclare it and assign the string value Unstop to it.
- This is possible because, in Python, variables can hold different types of values at different points in the code. So, x now contains the string Unstop.
- Finally, we print the value of x using the print() function. Since x was last assigned the value Unstop, that is what will be printed as the output.
Assigning Same Value To Multiple Python Variables
You can assign the same value to multiple variables by just equating the variable to the other variable you want to copy the value from, for example, x=y=z=10. Doing so will reference the value of x, y, and z to the same address, so they will take much less space compared to storing them separately. Although the address will change as soon as you change the value. Below is an example of how this can be done in a program.
Code Example:
Output:
10
10
10
Explanation:
In the code above-
- We assign the integer value 10 to three variables, x, y, and z, simultaneously, using the basic assignment operator three times.
- This is possible because the assignment operator (=) in Python returns the assigned value, allowing for chained assignments.
- Then, we use three print() statements to display the values of x, y, and z, all of which are 10.
Assigning Different Values to Multiple Variables at Once
There are 2 methods for initializing different values to multiple variables in the same line. The first is that you can type the variable's names by separating them with commas and then equate them (assignment operator) with the values you want to assign, separated in the same way. Below is an example to help you understand how this is done.
Code Example:
Output:
10 20 30
Explanation:
- In this example, we simultaneously assign the values 10, 20, and 30 to three variables x, y, and z, respectively. As you can see, the values and variable names are separated by a comma.
- This is a form of multiple assignment in Python, where each variable on the left side gets assigned the corresponding value from the right side.
- After the assignment, we use print() statements to display the values of the variables to the console.
In the second method, we can use a list to assign variables with their respective values. Simply equate Python variables, separated by commas, to a list that contains the values you want to assign in the right order. Note that you can also equate different values too.
Code Example:
Output:
Dare 2 Compete
Explanation:
- In this example, we use the lists method of multiple assignments to assign values from the list, i.e., ["Dare", 2, "Compete"], to the variables x, y, and z, respectively.
- As a result, the variable x is assigned the value Dare, y is assigned the value 2, and z is assigned the value Compete.
- Next, we use a single print() statement with variable names separated by commas to display their values. Since there is a print function, the values are all printed in a single line.
Multiple assignment is a convenient way to unpack values from a list into individual variables. You can also create one of your own and try using any type of combination with lists and variables.
Also read- Advantages and Disadvantages of Python
Python Variable Types
Python variables can hold many types of values/ data, from numeric values to arrays to even multi-dimensional arrays. It can even be a complex data structure like graphs, maps, binary trees, etc. Some common datatypes are:
1. Numeric Types:
- int: Represents integer values (e.g., 5, -10, 0).
- float: Represents floating-point numbers with decimal places (e.g., 3.14, -2.5, 0.0).
- complex: Represents complex numbers in the form of a + bj, where a and b are real numbers and j is the iota used to represent imaginary numbers (e.g., 2+3j).
2. Sequence Types:
- str: String represents a text or a word enclosed in single quotes ('') or double quotes ("") (e.g., "Unstop", 'variable', "text").
- list: Represents an ordered collection of items enclosed in square brackets ([]), where each item can be of any datatype (e.g., [1, 2, 3], ['a', 'b', 'c'], [1, 'two', 3.0]).
- tuple: Similar to lists but immutable, meaning their elements cannot be modified once defined. They are enclosed in parentheses (()) (e.g., (1, 2, 3), ('a', 'b', 'c'), (1, 'two', 3.0)).
4. Mapping Type:
- dict: Represents an unordered collection of key-value pairs enclosed in curly braces ({}). You can access any value if you have the other one. Keys are unique and used to access values (e.g., {'name': 'John', 'age': 30}).
5. Set Types:
- Set: It represents an unordered collection of unique elements enclosed in curly braces ({}). Duplicates are automatically removed. Eg: set_variable = {1, 2, 3, "cherry", True}
- frozenset: Similar to sets but immutable. Once defined, its elements cannot be modified (e.g., frozenset({1, 2, 3}), frozenset({'a', 'b', 'c'})).
6. Boolean Type:
It represents the truth values, True or False. You can even use 0 and 1 in their places where 0 represents False, and 1 represents True. Eg: boolean_variable = True
7. None Type:
It represents the absence of a value or a null value. Eg: none_variable = None
Python Variable Scope
Python variable scope refers to a location inside the program where the respective variable is recognized, is accessible, can be modified, and can referenced within the code. For example, the variables in a function are temporary as we might need to call the function many times, even simultaneously in a loop, and storing these variable values globally can cause errors if the same function is running parallel.
Python variable scope can be customarily used for many specific applications while defining functions in your code. There are four types of variable scopes:
1. Local Python Variables
Variables defined within a function or class are of local scope and cannot be accessed outside of the function. Local variables are created when the function is called and are destroyed when the function completes execution. So, they are basically bound inside the function and have a life span till the function is running.
Each time a function is called, it creates a new instance of the variable. If the same function is running simultaneously, then also the local variable for both of them is stored in different memories as different occurrences.
Code Example:
Output:
10
Explanation:
- We begin the example above by defining a function named function inside which we create a local variable named local_variable with a value of 10.
- Note that this variable is local to the function and exists only within the function's scope.
- Next, we use the print() statement within the function to output the value of the local variable.
- After that, we call the function(), which then prints the value of the local variable defined within its active scope. The local variable is not accessible outside the function.
- This variable is local as this would be deleted as soon as the compiler (the computer program that understands the code and provides output) exits the function.
2. Global Python Variables
Variables that are defined outside of any function or class have a global scope. This means they can be accessed from anywhere in the code, including within functions and classes. One must avoid using these in functions if not required. If you still need to, then it is best to store some constant values that do not hamper the operation of the function if run parallelly at the same time. You can also define a global variable within a function or class, but for this, you must use the global keyword before initialization.
Code Example:
Output:
5
10
Explanation:
- We begin by defining a global variable x with a value of 5 outside any function, making it accessible throughout the entire script.
- Then, we define a function named function, which contains a print(x) statement that outputs the value of the global variable x. As is evident, we can access the global variable x from inside this function.
- We define another global variable named variable inside the function, with a value of 10 using the global keyword.
- Another print(variable) statement is included in the function, which outputs the value of the global variable variable.
- Lastly, we call the function(), and it prints the values of both global variables x and variable.
3. Nonlocal (Enclosing) Python Variables
Nonlocal variables in Python are variables declared within a nested function such that it does not belong to just that function but also to the enclosing function. It allows you to modify and access variables from the immediate outer scope within a nested function.
You might sometimes be required to make the Python variable accessible to just outside functions, for which you can create it outside and pass it as a parameter instead. However, nonlocal variables provide more flexibility and hence are preferred in some instances.
Code Example:
Output:
Inner function: 15
Outer function: 15
Explanation:
- We first define an outer function named outer_function, where we initialize the variable x with the value 10.
- Inside this function, we define an inner function named inner_function, inside which-
- We define another variable, x, by using the nonlocal keyword to indicate that x refers to the variable defined in the outer scope (outer_function).
- Then, using the increment operator, we increase the value of x by 5 within inner_function.
- It also has a print() statement, i.e., print("Inner function:", x), which outputs the modified value of x within the inner function.
- Then, we call the inner_function from inside the outer function and also have a print() statement to display the value of x from the outer function.
- That is, print("Outer function:", x), which outputs the value of x within the outer function, demonstrating that the modification in the inner function affects the variable in the outer scope due to the use of the nonlocal keyword.
Note: Alternatively, if we did not use the nonlocal keyword inside the inner_function, and only redeclared x to the value of 5. Then, print("Inner function:', x) would output the value 5, and print("Outer function:", x) would output 10, indicating that changing x in the inner scope does not affect x in the outer scope.
4. Python Variables With Built-In Scope
The built-in scope of Python variables includes the ones in the namespace containing names that are preassigned in Python. These names are always available without the need for explicit imports. They include functions like print(), len(), data types (like int, str, etc.), and other commonly used elements. Here's a simple code example demonstrating the use of the built-in scope Python variables.
Code Example:
Output:
Hello, World!
Length of 'Python': 6
Approximate value of pi: 3.14
0
1
2
3
4
Explanation:
In this example, we demonstrate the use of various built-in functions, data types, and operations in Python.
- We first use the built-in functions print(), len(), int(), float(), and str() for different purposes, as mentioned in the code comments.
- Then, we use abs() and max() functions, where the former gives the absolute value of the parameter and later returns the max value from amongst its parameters.
- Next, we use the round() function, which rounds up the values passed as parameters, like the constant pi in this case.
- We also use the type() function, which determines the data types of variables passed as parameters.
- Then, we create sequences, i.e., lists and tuples, using built-in list() and tuple() functions, respectively.
- Next, we create a dictionary using the dict() and the zip() functions to combine two lists.
- We then employ the range() function to create a sequence of numbers for iteration inside a for-loop.
- After that, we use the built-in logical operators (and, or, not) and comparison/ relational operators (==, !=) to perform a comparison of input values/ integer objects.
- The code demonstrates the versatility of built-in functionalities without the need for explicit imports.
Concatenating Python Variables
Concatenation means combining two or more Python variables like strings or lists, etc. We generally use the addition arithmetic operator for this purpose. However, concatenation cannot be referred to as simple addition; that is, if we say we concat 4 and 5, we mean it would form 45, not 9.
We can use the same Python addition operator for both concatenation and addition operations, based on which datatype you use. But if you want to resolve any conflict that arises from using the addition arithmetic operator for concatenation, an alternative you can use is the inbuilt concat() function. It helps perform concatenation whenever required.
Code Example:
Output:
Ashima is 25 years old.
Explanation:
- We begin by initializing a string variable name with the value Ashima and then an integer variable age with the value 25.
- Next, use the str() function to convert the integer age to a string so that it can be concatenated with the rest of the string variables.
- This is used inside the concatenation method, where we use the addition operator to connect the initial variables with string terms- is and years old, with spaces in the right places.
- The outcome of this concatenation operation on variables is stored in the variable result.
- Then, we use the print(result) statement to display the final string to the console, as shown above.
As an alternative to the str() method, you can also use the f-strings, which allow us to directly insert variables into the string using curly braces {}. In this method, the variables are automatically converted to strings and concatenated with the rest of the variables/ parameters.
Code Example:
Output:
Rahul is 28 years old.
Explanation:
- We begin this Python example by creating a variable name to store the string value Rahul and a variable age to store an integer number 25.
- Next, we create a new variable result, which is assigned a value using the f-strings.
- Inside this f-string, we insert the names of Python variables we want to concatenate into the string within curly brackets.
- The outcome will be a concatenated string with the variables, name, age, and other terms.
- We then use the print() function to display the whole sentence to the output console.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Object Identity & Object References Of Python Variables
In Python, every piece of data is treated as an object. Objects have a unique identity, a value, and a type. When you create a variable and assign it a value, you are essentially creating a reference to an object. Understanding object identity and references is crucial for grasping how Python variables work.
Object Identity
In Python, every object has a unique identity, which serves as a way to distinguish it from other objects. The object identity is represented by a unique integer, and it remains constant throughout the object's lifetime. The id() function is used to obtain the identity of an object.
Example:
x = 42
print(id(x))
In this example, x is assigned the integer value 42, and id(x) returns the memory address of the object representing the integer 42.
Object References
An object reference is the association between a variable and the memory location (object) where the value is stored. When you create a variable and assign it a value, the variable is essentially a reference to the object in memory. Note that multiple variables can refer to the same object.
Example:
x = [1, 2, 3] # Creates a list and assigns it to variable 'x'
y = x # Creates a new reference 'y' pointing to the same list object
print(x)
print(y)
Here, both x and y reference the same list object in memory. Any modification made to the list through either x or y will affect the same underlying object.
Reserved Words/ Keywords & Python Variable Names
In Python, reserved words, also known as keywords, are specific words that have predefined meanings within the language. These words are reserved for specific purposes and cannot be used as identifiers (such as variable names or function names) in your code. Using these reserved words as variable names would lead to syntax errors and confusion. You can check these keywords anytime by simply typing help(“keywords”) on your Python interpreter, which is basically your terminal when you are coding in a Python ide. The table below gives a brief overview of some common Python keywords.
Table Of Python Keywords:
False |
None |
True |
and |
as |
assert |
async |
await |
break |
class |
continue |
def |
del |
elif |
else |
except |
finally |
for |
from |
global |
if |
import |
in |
is |
lambda |
nonlocal |
not |
or |
pass |
raise |
return |
try |
while |
with |
yield |
These keywords are case-sensitive. If you assign the variable name as these keywords, then it will throw syntax errors. Below is an example of the same.
Code Example:
Output:
False=4
^^^^^
SyntaxError: cannot assign to False
Explanation:
In the code above-
- We first create a variable called False and assign the value of 4 to it. Note that False is a reserved keyword used as a return value for boolean operations.
- Then, we use the print() method to display the value of this variable to the console.
- When we do this, we simply get a syntax error stating that the value cannot be assigned to False as it is a reserved keyword.
Conclusion
Understanding Python variables is foundational to writing effective and efficient code. Whether you are a beginner or an experienced developer, grasping the nuances of variables in Python is crucial for creating robust and maintainable programs. By following best practices and embracing the dynamic nature of Python variables, you can write code that is not only functional but also elegant and easy to comprehend. So, go ahead, experiment with variables, and unlock the full potential of Python programming!
Frequently Asked Questions
Q. What is a variable in Python?
A variable in Python is a symbolic name that references a value or an integer object/ string object. It provides a way to store and manipulate data in a program. Python variables are created using an assignment single statement, and their values can be changed during the execution of the program.
Q. Can we use the same name for different types of Python variables?
Yes, in Python, you can use the same name for variables of different types. Unlike some statically typed languages, Python is dynamically typed, which means you don't have to declare the type of a variable explicitly. The variable's type is determined at runtime based on the value it is assigned.
Here's an example:
In this example, the variable x is used for three different types. i.e., first as an integer, then as a string, and finally as a list. Python allows this flexibility, making it easy to work with different types of data using the same variable name.
Q. How do variable types work in Python?
Python is dynamically typed, meaning you don't need to declare the type of a Python variable explicitly. The interpreter infers the type based on the assigned value. Also these Python variables can hold different types, and their types can change during runtime.
Q. What is the scope of a variable in Python?
The concept of scope in Python variables refers to the region of the program from where a variable can be accessed. The different Python variable scopes include local, enclosing, global, and built-in Python. Understanding variable scope is crucial for writing maintainable and bug-free code.
Q. Can variables be used in string manipulation in Python?
Yes, variables can be used in string manipulation in Python. You can embed Python variables in strings using various methods like string concatenation, string formatting with % or format(), and f-strings. This allows for dynamic and flexible construction of strings based on Python variable values.
Q. What is the naming convention for Python variables?
The recommended naming convention for Python variables follows the guidelines outlined in PEP 8, the official style guide for Python code. Variables should use snake_case, where words are in lowercase and separated by underscores, promoting readability and consistency. Descriptive and clear names are encouraged to convey the purpose of the variable, avoiding single-letter names unless used as loop counters. It's crucial to avoid using Python-reserved words as variable names and maintain consistency in naming throughout the codebase. Ambiguous abbreviations should be avoided in favor of more explicit and understandable names.
Q. How are variables different from constants in Python?
Python variables are symbols that represent values and can change during program execution, while constants are values that do not change during program execution. In Python, there is no strict concept of constants, but conventionally, Python variables with uppercase names are considered constants and are not intended to be modified.
Here are a few more interesting topics you must read:
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment