Python Programming
Table of content:
- What Is Python? An Introduction
- What Is The History Of Python?
- Key Features Of The Python Programming Language
- Who Uses Python?
- Basic Characteristics Of Python Programming Syntax
- Why Should You Learn Python?
- Applications Of Python Language
- Advantages And Disadvantages Of Python
- Some Useful Python Tips & Tricks For Efficient Programming
- Python 2 Vs. Python 3: Which Should You Learn?
- Python Libraries
- Conclusion
- Frequently Asked Questions
- It's Python Basics Quiz Time!
Table of content:
- Python At A Glance
- Key Features of Python Programming
- Applications of Python
- Bonus: Interesting features of different programming languages
- Summing up...
- FAQs regarding Python
- Take A Quiz To Rehash Python's Features!
Table of content:
- What Is Python IDLE?
- What Is Python Shell & Its Uses?
- Primary Features Of Python IDLE
- How To Use Python IDLE Shell? Setting Up Your Python Environment
- How To Work With Files In Python IDLE?
- How To Execute A File In Python IDLE?
- Improving Workflow In Python IDLE Software
- Debugging In Python IDLE
- Customizing Python IDLE
- Code Examples
- Conclusion
- Frequently Asked Questions (FAQs)
- How Well Do You Know IDLE? Take A Quiz!
Table of content:
- What Is A Variable In Python?
- Creating And Declaring Python Variables
- Rules For Naming Python Variables
- How To Print Python Variables?
- How To Delete A Python Variable?
- Various Methods Of Variables Assignment In Python
- Python Variable Types
- Python Variable Scope
- Concatenating Python Variables
- Object Identity & Object References Of Python Variables
- Reserved Words/ Keywords & Python Variable Names
- Conclusion
- Frequently Asked Questions
- Rehash Python Variables Basics With A Quiz!
Table of content:
- What Is A String In Python?
- Creating String In Python
- How To Create Multiline Python Strings?
- Reassigning Python Strings
- Accessing Characters Of Python Strings
- How To Update Or Delete A Python String?
- Reversing A Python String
- Formatting Python Strings
- Concatenation & Comparison Of Python Strings
- Python String Operators
- Python String Functions
- Escape Sequences In Python Strings
- Conclusion
- Frequently Asked Questions
- Rehash Python Strings Basics With A Quiz!
Table of content:
- What Is Python Namespace?
- Lifetime Of Python Namespace
- Types Of Python Namespace
- The Built-In Namespace In Python
- The Global Namespace In Python
- The Local Namespace In Python
- The Enclosing Namespace In Python
- Variable Scope & Namespace In Python
- Python Namespace Dictionaries
- Changing Variables Out Of Their Scope & Python Namespace
- Best Practices Of Python Namespace
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Namespaces!
Table of content:
- What Are Logical Operators In Python?
- The AND Python Logical Operator
- The OR Python Logical Operator
- The NOT Python Logical Operator
- Short-Circuiting Evaluation Of Python Logical Operators
- Precedence of Logical Operators In Python
- How Does Python Calculate Truth Value?
- Final Note On How AND & OR Python Logical Operators Work
- Conclusion
- Frequently Asked Questions
- Python Logical Operators Quiz– Test Your Knowledge!
Table of content:
- What Are Bitwise Operators In Python?
- List Of Python Bitwise Operators
- AND Python Bitwise Operator
- OR Python Bitwise Operator
- NOT Python Bitwise Operator
- XOR Python Bitwise Operator
- Right Shift Python Bitwise Operator
- Left Shift Python Bitwise Operator
- Python Bitwise Operations And Negative Integers
- The Binary Number System
- Application of Python Bitwise Operators
- Python Bitwise Operator Overloading
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Bitwise Operators!
Table of content:
- What Is The Print() Function In Python?
- How Does The print() Function Work In Python?
- How To Print Single & Multi-line Strings In Python?
- How To Print Built-in Data Types In Python?
- Print() Function In Python For Values Stored In Variables
- Print() Function In Python With sep Parameter
- Print() Function In Python With end Parameter
- Print() Function In Python With flush Parameter
- Print() Function In Python With file Parameter
- How To Remove Newline From print() Function In Python?
- Use Cases Of The print() Function In Python
- Understanding Print Statement In Python 2 Vs. Python 3
- Conclusion
- Frequently Asked Questions
- Know The print() Function In Python? Take A Quiz!
Table of content:
- Working Of Normal Print() Function
- The New Line Character In Python
- How To Print Without Newline In Python | Using The End Parameter
- How To Print Without Newline In Python 2.x? | Using Comma Operator
- How To Print Without Newline In Python 3.x?
- How To Print Without Newline In Python With Module Sys
- The Star Pattern(*) | How To Print Without Newline & Space In Python
- How To Print A List Without Newline In Python?
- How To Remove New Lines In Python?
- Conclusion
- Frequently Asked Questions
- Think You Can Print Without a Newline in Python? Prove It!
Table of content:
- What Is A Python For Loop?
- How Does Python For Loop Work?
- When & Why To Use Python For Loops?
- Python For Loop Examples
- What Is Rrange() Function In Python?
- Nested For Loops In Python
- Python For Loop With Continue & Break Statements
- Python For Loop With Pass Statement
- Else Statement In Python For Loop
- Conclusion
- Frequently Asked Questions
- Think You Know Python's For Loop? Prove It!
Table of content:
- What Is Python While Loop?
- How Does The Python While Loop Work?
- How To Use Python While Loops For Iterations?
- Control Statements In Python While Loop With Examples
- Python While Loop With Python List
- Infinite Python While Loop in Python
- Python While Loop Multiple Conditions
- Nested Python While Loops
- Conclusion
- Frequently Asked Questions
- Mastered Python While Loop? Let’s Find Out!
Table of content:
- What Are Conditional If-Else Statements In Python?
- Types Of If-Else Statements In Python
- If Statement In Python
- If-Else Statement In Python
- Nested If-Else Statement In Python
- Elif Statement In Python
- Ladder If-Elif-Else Statement In Python
- Short Hand If-Statement In Python
- Short Hand If-Else Statement In Python
- Operators & If-Esle Statement In Python
- Other Statements With If-Else In Python
- Conclusion
- Frequently Asked Questions
- Quick If-Else Statement Quiz– Let’s Go!
Table of content:
- What Is Control Structure In Python?
- Types Of Control Structures In Python
- Sequential Control Structures In Python
- Decision-Making Control Structures In Python
- Repetition Control Structures In Python
- Benefits Of Using Control Structures In Python
- Conclusion
- Frequently Asked Questions
- Control Structures in Python – Are You the Master? Take A Quiz!
Table of content:
- What Are Python Libraries?
- How Do Python Libraries Work?
- Standard Python Libraries (With List)
- Important Python Libraries For Data Science
- Important Python Libraries For Machine & Deep Learning
- Other Important Python Libraries You Must Know
- Working With Third-Party Python Libraries
- Troubleshooting Common Issues For Python Libraries
- Python Libraries In Larger Projects
- Importance Of Python Libraries
- Conclusion
- Frequently Asked Questions
- Quick Quiz On Python Libraries – Let’s Go!
Table of content:
- What Are Python Functions?
- How To Create/ Define Functions In Python?
- How To Call A Python Function?
- Types Of Python Functions Based On Parameters & Return Statement
- Rules & Best Practices For Naming Python Functions
- Basic Types of Python Functions
- The Return Statement In Python Functions
- Types Of Arguments In Python Functions
- Docstring In Python Functions
- Passing Parameters In Python Functions
- Python Function Variables | Scope & Lifetime
- Advantages Of Using Python Functions
- Recursive Python Function
- Anonymous/ Lambda Function In Python
- Nested Functions In Python
- Conclusion
- Frequently Asked Questions
- Python Functions – Test Your Knowledge With A Quiz!
Table of content:
- What Are Python Built-In Functions?
- Mathematical Python Built-In Functions
- Python Built-In Functions For Strings
- Input/ Output Built-In Functions In Python
- List & Tuple Python Built-In Functions
- File Handling Python Built-In Functions
- Python Built-In Functions For Dictionary
- Type Conversion Python Built-In Functions
- Basic Python Built-In Functions
- List Of Python Built-In Functions (Alphabetical)
- Conclusion
- Frequently Asked Questions
- Think You Know Python Built-in Functions? Prove It!
Table of content:
- What Is A round() Function In Python?
- How Does Python round() Function Work?
- Python round() Function If The Second Parameter Is Missing
- Python round() Function If The Second Parameter Is Present
- Python round() Function With Negative Integers
- Python round() Function With Math Library
- Python round() Function With Numpy Module
- Round Up And Round Down Numbers In Python
- Truncation Vs Rounding In Python
- Practical Applications Of Python round() Function
- Conclusion
- Frequently Asked Questions
- Revisit Python’s round() Function – Take The Quiz!
Table of content:
- What Is Python pow() Function?
- Python pow() Function Example
- Python pow() Function With Modulus (Three Parameters)
- Python pow() Function With Complex Numbers
- Python pow() Function With Floating-Point Arguments And Modulus
- Python pow() Function Implementation Cases
- Difference Between Inbuilt-pow() And math.pow() Function
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python’s pow() Function!
Table of content:
- Python max() Function With Objects
- Examples Of Python max() Function With Objects
- Python max() Function With Iterable
- Examples Of Python max() Function With Iterables
- Potential Errors With The Python max() Function
- Python max() Function Vs. Python min() Functions
- Conclusion
- Frequently Asked Questions
- Think You Know Python max() Function? Take A Quiz!
Table of content:
- What Are Strings In Python?
- What Are Python String Methods?
- List Of Python String Methods For Manipulating Case
- List Of Python String Methods For Searching & Finding
- List Of Python String Methods For Modifying & Transforming
- List Of Python String Methods For Checking Conditions
- List Of Python String Methods For Encoding & Decoding
- List Of Python String Methods For Stripping & Trimming
- List Of Python String Methods For Formatting
- Miscellaneous Python String Methods
- List Of Other Python String Operations
- Conclusion
- Frequently Asked Questions
- Mastered Python String Methods? Take A Quiz!
Table of content:
- What Is Python String?
- The Need For Python String Replacement
- The Python String replace() Method
- Multiple Replacements With Python String.replace() Method
- Replace A Character In String Using For Loop In Python
- Python String Replacement Using Slicing Method
- Replace A Character At a Given Position In Python String
- Replace Multiple Substrings With The Same String In Python
- Python String Replacement Using Regex Pattern
- Python String Replacement Using List Comprehension & Join() Method
- Python String Replacement Using Callback With re.sub() Method
- Python String Replacement With re.subn() Method
- Conclusion
- Frequently Asked Questions
- Know How To Replace Python Strings? Prove It!
Table of content:
- What Is String Slicing In Python?
- How Indexing & String Slicing Works In Python
- Extracting All Characters Using String Slicing In Python
- Extracting Characters Before & After Specific Position Using String Slicing In Python
- Extracting Characters Between Two Intervals Using String Slicing In Python
- Extracting Characters At Specific Intervals (Step) Using String Slicing In Python
- Negative Indexing & String Slicing In Python
- Handling Out-of-Bounds Indices In String Slicing In Python
- The slice() Method For String Slicing In Python
- Common Pitfalls Of String Slicing In Python
- Real-World Applications Of String Slicing
- Conclusion
- Frequently Asked Questions
- Quick Python String Slicing Quiz– Let’s Go!
Table of content:
- Introduction To Python List
- How To Create A Python List?
- How To Access Elements Of Python List?
- Accessing Multiple Elements From A Python List (Slicing)
- Access List Elements From Nested Python Lists
- How To Change Elements In Python Lists?
- How To Add Elements To Python Lists?
- Delete/ Remove Elements From Python Lists
- How To Create Copies Of Python Lists?
- Repeating Python Lists
- Ways To Iterate Over Python Lists
- How To Reverse A Python List?
- How To Sort Items Of Python Lists?
- Built-in Functions For Operations On Python Lists
- Conclusion
- Frequently Asked Questions
- Revisit Python Lists Basics With A Quick Quiz!
Table of content:
- What Is List Comprehension In Python?
- Incorporating Conditional Statements With List Comprehension In Python
- List Comprehension In Python With range()
- Filtering Lists Effectively With List Comprehension In Python
- Nested Loops With List Comprehension In Python
- Flattening Nested Lists With List Comprehension In Python
- Handling Exceptions In List Comprehension In Python
- Common Use Cases For List Comprehensions
- Advantages & Disadvantages Of List Comprehension In Python
- Best Practices For Using List Comprehension In Python
- Performance Considerations For List Comprehension In Python
- For Loops & List Comprehension In Python: A Comparison
- Difference Between Generator Expression & List Comprehension In Python
- Conclusion
- Frequently Asked Questions
- Rehash Python List Comprehension Basics With A Quiz!
Table of content:
- What Is A List In Python?
- How To Find Length Of List In Python?
- For Loop To Get Python List Length (Naive Approach)
- The len() Function To Get Length Of List In Python
- The length_hint() Function To Find Length Of List In Python
- The sum() Function To Find The Length Of List In Python
- The enumerate() Function To Find Python List Length
- The Counter Class From collections To Find Python List Length
- The List Comprehension To Find Python List Length
- Find The Length Of List In Python Using Recursion
- Comparison Between Ways To Find Python List Length
- Conclusion
- Frequently Asked Questions
- Know How To Get Python List Length? Prove it!
Table of content:
- List of Methods To Reverse A Python List
- Python Reverse List Using reverse() Method
- Python Reverse List Using the Slice Operator ([::-1])
- Python Reverse List By Swapping Elements
- Python Reverse List Using The reversed() Function
- Python Reverse List Using A for Loop
- Python Reverse List Using While Loop
- Python Reverse List Using List Comprehension
- Python Reverse List Using List Indexing
- Python Reverse List Using The range() Function
- Python Reverse List Using NumPy
- Comparison Of Ways To Reverse A Python List
- Conclusion
- Frequently Asked Questions
- Time To Test Your Python List Reversal Skills!
Table of content:
- What Is Indexing In Python?
- The Python List index() Function
- How To Use Python List index() To Find Index Of A List Element
- The Python List index() Method With Single Parameter (Start)
- The Python List index() Method With Start & Stop Parameters
- What Happens When We Use Python List index() For An Element That Doesn't Exist
- Python List index() With Nested Lists
- Fixing IndexError Using The Python List index() Method
- Python List index() With Enumerate()
- Real-world Examples Of Python List index() Method
- Difference Between find() And index() Method In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python List Indexing? Take A Quiz!
Table of content:
- How To Remove Elements From List In Python?
- The remove() Method To Remove Element From Python List
- The pop() Method To Remove Element From List In Python
- The del Keyword To Remove Element From List In Python
- The clear() Method To Remove Elements From Python List
- List Comprehensions To Conditionally Remove Element From List In Python
- Key Considerations For Removing Elements From Python Lists
- Why We Need to Remove Elements From Python List
- Performance Comparison Of Methods To Remove Element From List In Python
- Conclusion
- Frequently Asked Questions
- Quiz– Prove You Know How To Remove Item From Python Lists!
Table of content:
- How To Remove Duplicates From A List In Python?
- The set() Function To Remove Duplicates From Python List
- Remove Duplicates From Python List Using For Loop
- Using List Comprehension Remove Duplicates From Python List
- Remove Duplicates From Python List Using enumerate() With List Comprehension
- Dictionary & fromkeys() Method To Remove Duplicates From Python List
- Remove Duplicates From Python List Using in, not in Operators
- Remove Duplicates From Python List Using collections.OrderedDict.fromkeys()
- Remove Duplicates From Python List Using Counter with freq.dist() Method
- The del Keyword Remove Duplicates From Python List
- Remove Duplicates From Python List Using DataFrame
- Remove Duplicates From Python List Using pd.unique and np.unipue
- Remove Duplicates From Python List Using reduce() function
- Comparative Analysis Of Ways To Remove Duplicates From Python List
- Conclusion
- Frequently Asked Questions
- Think You Know How to Remove Duplicates? Take A Quiz!
Table of content:
- What Is Python List & How To Access Elements?
- What Is IndexError: List Index Out Of Range & Its Causes In Python?
- Understanding Indexing Behavior In Python Lists
- How to Prevent/ Fix IndexError: List Index Out Of Range In Python
- Handling IndexError Gracefully Using Try-Except
- Debugging Tips For IndexError: List Index Out Of Range Python
- Conclusion
- Frequently Asked Questions
- Avoiding ‘List Index Out of Range’ Errors? Take A Quiz!
Table of content:
- What Is the Python sort() List Method?
- Sorting In Ascending Order Using The Python sort() List Method
- How To Sort Items In Descending Order Using Python sort() List Method
- Custom Sorting Using The Key Parameter Of Python sort() List Method
- Examples Of Python sort() List Method
- What Is The sorted() List Method In Python
- Differences Between sorted() And sort() List Methods In Python
- When To Use sorted() & When To Use sort() List Method In Python
- Conclusion
- Frequently Asked Questions
- Take A Quick Python's sort() Quiz!
Table of content:
- What Is A List In Python?
- What Is A String In Python?
- Why Convert Python List To String?
- How To Convert List To String In Python?
- The join() Method To Convert Python List To String
- Convert Python List To String Through Iteration
- Convert Python List To String With List Comprehension
- The map() Function To Convert Python List To String
- Convert Python List to String Using format() Function
- Convert Python List To String Using Recursion
- Enumeration Function To Convert Python List To String
- Convert Python List To String Using Operator Module
- Python Program To Convert String To List
- Conclusion
- Frequently Asked Questions
- Convert Lists To Strings Like A Pro! Take A Quiz
Table of content:
- What Is Inheritance In Python?
- Python Inheritance Syntax
- Parent Class In Python Inheritance
- Child Class In Python Inheritance
- The __init__() Method In Python Inheritance
- The super() Function In Python Inheritance
- Method Overriding In Python Inheritance
- Types Of Inheritance In Python
- Special Functions In Python Inheritance
- Advantages & Disadvantages Of Inheritance In Python
- Common Use Cases For Inheritance In Python
- Best Practices for Implementing Inheritance in Python
- Avoiding Common Pitfalls in Python Inheritance
- Conclusion
- Frequently Asked Questions
- 💡 Python Inheritance Quiz – Are You Ready?
Table of content:
- What Is The Python List append() Method?
- Adding Elements To A Python List Using append()
- Populate A Python List Using append()
- Adding Different Data Types To Python List Using append()
- Adding A List To Python List Using append()
- Nested Lists With Python List append() Method
- Practical Use Cases Of Python List append() Method
- How append() Method Affects List Performance
- Avoiding Common Mistakes When Using Python List append()
- Comparing extend() With append() Python List Method
- Conclusion
- Frequently Asked Questions
- 🧠 Think You Know Python List append()? Take A Quiz!
Table of content:
- What Is A Linked List In Python?
- Types Of Linked Lists In Python
- How To Create A Linked List In Python
- How To Traverse A Linked List In Python & Retrieve Elements
- Inserting Elements In A Linked List In Python
- Deleting Elements From A Linked List In Python
- Update A Node Of Linked List In Python
- Reversing A Linked List In Python
- Calculating Length Of A Linked List In Python
- Comparing Arrays And Linked Lists In Python
- Advantages & Disadvantages Of Linked List In Python
- When To Use Linked Lists Over Other Data Structures
- Practical Applications Of Linked Lists In Python
- Conclusion
- Frequently Asked Questions
- 🔗 Linked List Logic: Can You Ace This Quiz?
Table of content:
- What Is Extend In Python?
- Extend In Python With List
- Extend In Python With String
- Extend In Python With Tuple
- Extend In Python With Set
- Extend In Python With Dictionary
- Other Methods To Extend A List In Python
- Difference Between append() and extend() In Python
- Conclusion
- Frequently Asked Questions
- Think You Know extend() In Python? Prove It!
Table of content:
- What Is Recursion In Python?
- Key Components Of Recursive Functions In Python
- Implementing Recursion In Python
- Recursion Vs. Iteration In Python
- Tail Recursion In Python
- Infinite Recursion In Python
- Advantages Of Recursion In Python
- Disadvantages Of Recursion In Python
- Best Practices For Using Recursion In Python
- Conclusion
- Frequently Asked Questions
- Recursive Thinking In Python: Test Your Skills!
Table of content:
- What Is Type Conversion In Python?
- Types Of Type Conversion In Python
- Implicit Type Conversion In Python
- Explicit Type Conversion In Python
- Functions Used For Explicit Data Type Conversion In Python
- Important Type Conversion Tips In Python
- Benefits Of Type Conversion In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Type Conversion? Take A Quiz!
Table of content:
- What Is Scope In Python?
- Local Scope In Python
- Global Scope In Python
- Nonlocal (Enclosing) Scope In Python
- Built-In Scope In Python
- The LEGB Rule For Python Scope
- Python Scope And Variable Lifetime
- Best Practices For Managing Python Scope
- Conclusion
- Frequently Asked Questions
- Think You Know Python Scope? Test Yourself!
Table of content:
- Understanding The Continue Statement In Python
- How Does Continue Statement Work In Python?
- Python Continue Statement With For Loops
- Python Continue Statement With While Loops
- Python Continue Statement With Nested Loops
- Python Continue With If-Else Statement
- Difference Between Pass and Continue Statement In Python
- Practical Applications Of Continue Statement In Python
- Conclusion
- Frequently Asked Questions
- Python 'continue' Statement Quiz: Can You Ace It?
Table of content:
- What Are Control Statements In Python?
- Types Of Control Statements In Python
- Conditional Control Statements In Python
- Loop Control Statements In Python
- Control Flow Altering Statements In Python
- Exception Handling Control Statements In Python
- Conclusion
- Frequently Asked Questions
- Mastering Control Statements In Python – Take the Quiz!
Table of content:
- Difference Between Mutable And Immutable Data Types in Python
- What Is Mutable Data Type In Python?
- Types Of Mutable Data Types In Python
- What Are Immutable Data Types In Python?
- Types Of Immutable Data Types In Python
- Key Similarities Between Mutable And Immutable Data Types In Python
- When To Use Mutable Vs Immutable In Python?
- Conclusion
- Frequently Asked Questions
- Quiz Time: Mutable vs. Immutable In Python!
Table of content:
- What Is A List?
- What Is A Tuple?
- Difference Between List And Tuple In Python (Comparison Table)
- Syntax Difference Between List And Tuple In Python
- Mutability Difference Between List And Tuple In Python
- Other Difference Between List And Tuple In Python
- List Vs. Tuple In Python | Methods
- When To Use Tuples Over Lists?
- Key Similarities Between Tuples And Lists In Python
- Conclusion
- Frequently Asked Questions
- 🧐 Lists vs. Tuples Quiz: Test Your Python Knowledge!
Table of content:
- Introduction to Python
- Downloading & Installing Python, IDLE, Tkinter, NumPy & PyGame
- Creating A New Python Project
- How To Write Python Hello World Program In Python?
- Way To Write The Hello, World! Program In Python
- The Hello, World! Program In Python Using Class
- The Hello, World! Program In Python Using Function
- Print Hello World 5 Times Using A For Loop
- Conclusion
- Frequently Asked Questions
- 👋 Python's 'Hello, World!'—How Well Do You Know It?
Table of content:
- Algorithm Of Python Program To Add To Numbers
- Standard Program To Add Two Numbers In Python
- Python Program To Add Two Numbers With User-defined Input
- The add() Method In Python Program To Add Two Numbers
- Python Program To Add Two Numbers Using Lambda
- Python Program To Add Two Numbers Using Function
- Python Program To Add Two Numbers Using Recursion
- Python Program To Add Two Numbers Using Class
- How To Add Multiple Numbers In Python?
- Add Multiple Numbers In Python With User Input
- Time Complexities Of Python Programs To Add Two Numbers
- Conclusion
- Frequently Asked Questions
- 💡 Quiz Time: Python Addition Basics!
Table of content:
- Swapping in Python
- Swapping Two Variables Using A Temporary Variable
- Swapping Two Variables Using The Comma Operator In Python
- Swapping Two Variables Using The Arithmetic Operators (+,-)
- Swapping Two Variables Using The Arithmetic Operators (*,/)
- Swapping Two Variables Using The XOR(^) Operator
- Swapping Two Variables Using Bitwise Addition and Subtraction
- Swap Variables In A List
- Conclusion
- Frequently Asked Questions (FAQs)
- Quiz To Test Your Variable Swapping Knowledge
Table of content:
- What Is A Quadratic Equation? How To Solve It?
- How To Write A Python Program To Solve Quadratic Equations?
- Python Program To Solve Quadratic Equations Directly Using The Formula
- Python Program To Solve Quadratic Equations Using The Complex Math Module
- Python Program To Solve Quadratic Equations Using Functions
- Python Program To Solve Quadratic Equations & Find Number Of Solutions
- Python Program To Plot Quadratic Functions
- Conclusion
- Frequently Asked Questions
- Quadratic Equations In Python Quiz: Test Your Knowledge!
Table of content:
- What Is Decimal Number System?
- What Is Binary Number System?
- What Is Octal Number System?
- What Is Hexadecimal Number System?
- Python Program to Convert Decimal to Binary, Octal, And Hexadecimal Using Built-In Function
- Python Program To Convert Decimal To Binary Using Recursion
- Python Program To Convert Decimal To Octal Using Recursion
- Python Program To Convert Decimal To Hexadecimal Using Recursion
- Python Program To Convert Decimal To Binary Using While Loop
- Python Program To Convert Decimal To Octal Using While Loop
- Python Program To Convert Decimal To Hexadecimal Using While Loop
- Convert Decimal To Binary, Octal, And Hexadecimal Using String Formatting
- Python Program To Convert Binary, Octal, And Hexadecimal String To A Number
- Complexity Comparison Of Python Programs To Convert Decimal To Binary, Octal, And Hexadecimal
- Conclusion
- Frequently Asked Questions
- 💡 Decimal To Binary, Octal & Hex: Quiz Time!
Table of content:
- What Is A Square Root?
- Python Program To Find The Square Root Of A Number
- The pow() Function In Python Program To Find The Square Root Of Given Number
- Python Program To Find Square Root Using The sqrt() Function
- The cmath Module & Python Program To Find The Square Root Of A Number
- Python Program To Find Square Root Using The Exponent Operator (**)
- Python Program To Find Square Root With A User-Defined Function
- Python Program To Find Square Root Using A Class
- Python Program To Find Square Root Using Binary Search
- Python Program To Find Square Root Using NumPy Module
- Conclusion
- Frequently Asked Questions
- 🤓 Think You Know Square Roots In Python? Take A Quiz!
Table of content:
- Understanding the Logic Behind the Conversion of Kilometers to Miles
- Steps To Write Python Program To Convert Kilometers To Miles
- Python Program To Convert Kilometer To Miles Without Function
- Python Program To Convert Kilometer To Miles Using Function
- Python Program to Convert Kilometer To Miles Using Class
- Tips For Writing Python Program To Convert Kilometer To Miles
- Conclusion
- Frequently Asked Questions
- 🧐 Mastered Kilometer To Mile Conversion? Prove It!
Table of content:
- Why Build A Calculator Program In Python?
- Prerequisites To Writing A Calculator Program In Python
- Approach For Writing A Calculator Program In Python
- Simple Calculator Program In Python
- Calculator Program In Python Using Functions
- Creating GUI Calculator Program In Python Using Tkinter
- Conclusion
- Frequently Asked Questions
- 🧮 Calculator Program In Python Quiz!
Table of content:
- The Calendar Module In Python
- Prerequisites For Writing A Calendar Program In Python
- How To Write And Print A Calendar Program In Python
- Calendar Program In Python To Display A Month
- Calendar Program In Python To Display A Year
- Conclusion
- Frequently Asked Questions
- Calendar Program In Python – Quiz Time!
Table of content:
- What Is The Fibonacci Series?
- Pseudocode Code For Fibonacci Series Program In Python
- Generating Fibonacci Series In Python Using Naive Approach (While Loop)
- Fibonacci Series Program In Python Using The Direct Formula
- How To Generate Fibonacci Series In Python Using Recursion?
- Generating Fibonacci Series In Python With Dynamic Programming
- Fibonacci Series Program In Python Using For Loop
- Generating Fibonacci Series In Python Using If-Else Statement
- Generating Fibonacci Series In Python Using Arrays
- Generating Fibonacci Series In Python Using Cache
- Generating Fibonacci Series In Python Using Backtracking
- Fibonacci Series In Python Using Power Of Matix
- Complexity Analysis For Fibonacci Series Programs In Python
- Applications Of Fibonacci Series In Python & Programming
- Conclusion
- Frequently Asked Questions
- 🤔 Think You Know Fibonacci Series? Take A Quiz!
Table of content:
- Different Ways To Write Random Number Generator Python Programs
- Random Module To Write Random Number Generator Python Programs
- The Numpy Module To Write Random Number Generator Python Programs
- The Secrets Module To Write Random Number Generator Python Programs
- Understanding Randomness and Pseudo-Randomness In Python
- Common Issues and Solutions in Random Number Generation
- Applications of Random Number Generator Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python's Random Module? Prove It!
Table of content:
- What Is A Factorial?
- Algorithm Of Program To Find Factorial Of A Number In Python
- Pseudocode For Factorial Program in Python
- Factorial Program In Python Using For Loop
- Factorial Program In Python Using Recursion
- Factorial Program In Python Using While Loop
- Factorial Program In Python Using If-Else Statement
- The math Module | Factorial Program In Python Using Built-In Factorial() Function
- Python Program to Find Factorial of a Number Using Ternary Operator(One Line Solution)
- Python Program For Factorial Using Prime Factorization Method
- NumPy Module | Factorial Program In Python Using numpy.prod() Function
- Complexity Analysis Of Factorial Programs In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Factorials In Python? Take A Quiz!
Table of content:
- What Is Palindrome In Python?
- Check Palindrome In Python Using While Loop (Iterative Approach)
- Check Palindrome In Python Using For Loop And Character Matching
- Check Palindrome In Python Using The Reverse And Compare Method (Python Slicing)
- Check Palindrome In Python Using The In-built reversed() And join() Methods
- Check Palindrome In Python Using Recursion Method
- Check Palindrome In Python Using Flag
- Check Palindrome In Python Using One Extra Variable
- Check Palindrome In Python By Building Reverse, One Character At A Time
- Complexity Analysis For Palindrome Programs In Python
- Real-World Applications Of Palindrome In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Palindromes? Take A Quiz!
Table of content:
- Best Python Books For Beginners
- Best Python Books For Intermediate Level
- Best Python Books For Experts
- Best Python Books To Learn Algorithms
- Audiobooks of Python
- Best Books To Learn Python And Code Like A Pro
- To Learn Python Libraries
- Books To Provide Extra Edge In Python
- Python Project Ideas - Reference
- Quiz To Rehash Your Knowledge Of Python Books!
Python Libraries | Standard, Third-Party & More (Lists + Examples)
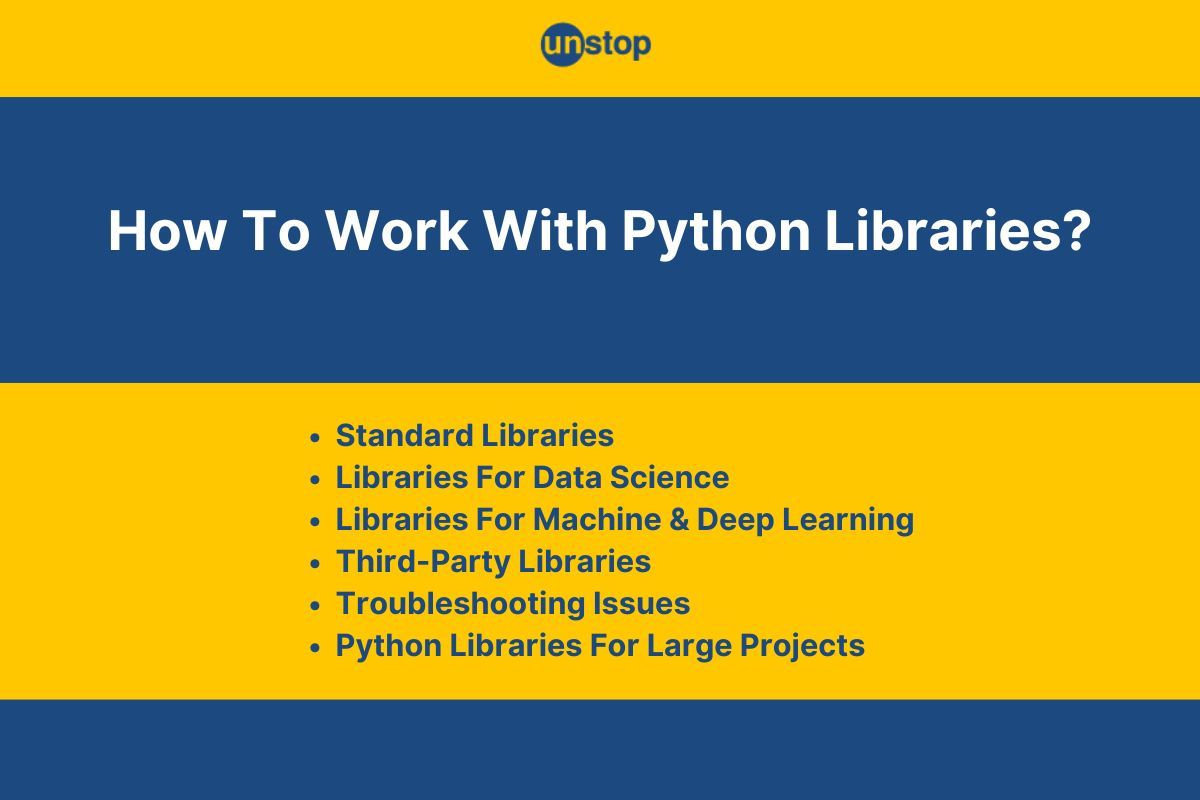
Python is one of the most popular and versatile programming languages out there, and one of the key reasons for its widespread appeal is the huge number of libraries available. But what exactly are Python libraries, and why should you care about them? Simply put, a Python library is a collection of pre-written code that you can use to perform common tasks without having to reinvent the wheel. Libraries make it easier to work with everything from data analysis and machine learning to web development and image processing. Let’s dive deeper into what makes these libraries so powerful and how they work!
What Are Python Libraries?
In Python programming, a library is a bundle of code that provides various functionalities, modules, and tools that you can use in your programs. These libraries contain collections of modules, which are individual files that group related functions, classes, and variables together.
Think of a library as a toolbox that contains many smaller tools (modules), each designed to perform specific tasks. For example, the math library provides a set of functions for performing mathematical operations such as square roots, trigonometric functions, and logarithms. The datetime library, on the other hand, provides tools for handling and manipulating dates and times. You don’t have to write the code for these operations from scratch—just import the library, and you are good to go.
Python libraries can be categorized into two main types:
- Standard Libraries: These come pre-installed with Python and include common functionalities that you can use out of the box (e.g., math, datetime, os).
- Third-Party Libraries: These are developed by the Python community or organizations and can be installed using a package manager like pip (e.g., NumPy, Pandas, TensorFlow).
In essence, Python libraries are your best friends when it comes to speeding up development, making your code cleaner, and leveraging pre-written, optimized solutions.
How Do Python Libraries Work?
Python libraries offer pre-written code, i.e., reusable functions, classes, and modules that you can import and utilize in your Python applications. Here’s how Python libraries generally work in a step-by-step process:
Step 1: Importing Libraries
The first step in using a Python library is importing it into your script. You can import an entire library or specific modules/functions depending on your needs. For example, to import the entire math library, you would write the following:
import math
Step 2: Accessing/ Using Functions and Classes
Once a library is imported, you can access and use the functions, classes, and constants it contains using dot notation. For example, you can use the built-in function sqrt() to find the square root of a number as follows:
math.sqrt(16) # Uses the sqrt function from the math library
Step 3: Interacting with Returned Objects
Many Python functions return objects that can be further manipulated. For example, some functions may return data structures like lists, and you can call other methods on them. For example, you can use the factorial() function to find the factorial of a number and then print what it returns as follows:
data = math.factorial(5) # Returns the factorial of 5
print(data) # Outputs 120
Step 4: Customization and Configuration
Some libraries allow you to customize or configure their behavior to suit your needs. You might use specific arguments when calling a function or configure global settings. For example:
data = pandas.DataFrame(data=my_data)
data.describe() # Provides statistical details about the DataFrame
Step 5: Handling Exceptions and Errors
Python libraries often raise exceptions when something goes wrong (e.g., invalid input). To handle such cases, you can use try and except blocks. For example:
try:
data_frame.dropna()
except ValueError as e:
print("An error occurred:", e)
Step 6: Performance Optimization
Some libraries are optimized for performance through features like native code or parallel processing. This is why libraries like NumPy are faster than writing custom Python code for numerical operations—they use optimized, low-level implementations for speed.
In essence, Python libraries encapsulate reusable code that handles common tasks, making your development process faster and more efficient. You import the library, access its functions, use them in your project, and handle errors appropriately, all while taking advantage of the performance optimizations built into the libraries themselves.
Importing & Using Python Libraries
To use any functionality from a library, you first need to import it into your code. The process of importing and using Python libraries is straightforward. Here's how it typically works:
Import the Library: You begin by using the import keyword to bring the library into your script. If you'd like a shorter name for the library, you can use the as keyword to alias it. For example:
import math # Imports the math library
Use the Library: Once imported, you can use the functions, classes, or variables provided by the library. The syntax for using an imported library typically follows the pattern:
library_name.function_name() or library_name.class_name().
Example: Let's say you want to calculate the square root of 25 and display the value of pi. Here is how this would look in code:
import math
print(math.sqrt(25)) # Output: 5.0
print(math.pi) # Output: 3.141592653589793
Importing specific items from a library module
Sometimes, you may not need the entire library, just specific functions or classes. In such cases, Python allows you to import only what you need, reducing memory usage and avoiding unnecessary imports.
Importing Specific Items: You can use the from keyword to import specific functions or variables directly from a module. This way, you don’t have to prefix the library name each time you call them. For example:
from math import sqrt, pi # Importing only sqrt and pi from math
Using the Imported Functions: Once imported, you can directly use the functions or classes without needing to prefix them with the library name. For example:
print(sqrt(25)) # Directly using sqrt function
Example: If you want to generate a random number between 1 and 10 and pick a random item from a list:
from random import randint, choice
print(randint(1, 10)) # Generates a random integer between 1 and 10
print(choice(['apple', 'banana', 'orange'])) # Picks a random item from the list
Standard Python Libraries (With List)
The Standard Library is a treasure trove of pre-built modules and packages bundled with every Python installation. These Python libraries are integral to the langauge and provide a broad range of functionality, from basic operations to advanced system tasks.
- Note that you don’t need to install them separately—they are readily available as part of the Python standard library.
- Standard libraries include built-in modules that can help you perform a variety of tasks such as file handling, mathematical operations, system interactions, data processing, and more.
- These libraries are tested, reliable, and widely used, making them an essential part of the Python ecosystem.
Some popular standard Python libraries include math, datetime, os, sys, and json, which offer utilities for specific tasks like working with numbers, managing files, and handling JSON data.
List Of Essential Standard Python Libraries
The table below lists some of the most commonly used standard libraries in Python, along with their descriptions and use cases.
Library Name |
Description |
Use Case/Applications |
math |
Provides mathematical functions like trigonometric operations, logarithms, etc. |
Advanced mathematical calculations. |
datetime |
Supplies classes for manipulating dates and times. |
Date and time manipulation, formatting, and arithmetic. |
os |
Interacts with the operating system, allowing file operations and process management. |
File and directory management, environment variable handling. |
sys |
Provides access to system-specific parameters and functions. |
Accessing command-line arguments and manipulating Python runtime. |
json |
Allows parsing of JSON data into Python objects and vice versa. |
Reading/writing JSON for web applications, APIs, and configuration files. |
collections |
Implements specialized container datatypes like deque, Counter, OrderedDict, and defaultdict. |
Handling advanced data structures, counting elements, and managing order. |
random |
Implements pseudo-random number generators and various randomization functions. |
Random number generation, shuffling, sampling data. |
pickle |
Serializes and deserializes Python objects for storage and transfer. |
Storing/loading Python objects, saving machine learning models. |
urllib |
Provides functions for parsing URLs and working with HTTP requests. |
Web scraping, making HTTP requests, downloading files. |
re |
Supports regular expressions for text searching, matching, and manipulation. |
Pattern matching, string validation, and text extraction. |
cgi |
Supports the development of web applications through HTTP request and response handling. |
Creating web applications using CGI scripts. |
socket |
Enables communication between computers using the TCP/IP protocol. |
Developing network-based applications like chat clients and servers. |
statistics |
Provides functions for statistical calculations. |
Mean, median, mode, variance, standard deviation calculations. |
csv |
Facilitates reading and writing CSV (Comma-Separated Values) files. |
Data import/export from spreadsheets and databases. |
functools |
Higher-order functions for functional programming. |
Memoization, partial function application, decorators. |
itertools |
Implements efficient looping constructs. |
Combinatorial constructs like permutations, combinations, and infinite iterators. |
threading |
Provides a way to run multiple threads (tasks) concurrently. |
Multithreading to improve performance in I/O-bound tasks. |
multiprocessing |
Enables the spawning of processes using an interface similar to threading. |
Parallel processing for CPU-bound tasks. |
sqlite3 |
Provides a lightweight database engine built into Python. |
Database management for small to medium-sized applications. |
argparse |
Helps parse command-line arguments passed to scripts. |
Creating user-friendly command-line interfaces. |
logging |
Provides a flexible framework for emitting log messages. |
Tracking application flow, debugging, and error reporting. |
Example Of Standard Python Library os– Directory Management
The simple Python program example below illustrates how to use the os standard Python library to to work with an existing directory and make a new one.
Code Example:
# Step 1: Import the os module
import os
# Step 2: Get the current working directory
current_directory = os.getcwd()
# Step 3: Create a new directory
os.mkdir('new_folder')
# Step 4: List all files and directories in the current directory
directory_contents = os.listdir()
# Step 5: Print the results
print("Current Directory:", current_directory)
print("Directory Contents:", directory_contents)
Output:
Current Directory: /home
Directory Contents: ['main.py', 'new_folder']
Explanation:
In the basic Python program example,
- We begin by importing the os module using the import statement.
- Then, we use the built-in function getcwd() to fetch the current working directory.
- Next, we create a new directory named new_folder using the mkdir() function, i.e., os.mkdir().
- We then use the lstdir() function on the current directors, which lists all files and directories in the current directory, i.e., os.listdir().
- After that, we use the print() function to display the current directory and its contents.
Check out this amazing course to become the best version of the Python programmer you can be.
Important Python Libraries For Data Science
Data Science is where Python shines, thanks to its extensive library ecosystem. These libraries simplify data manipulation, visualization, and advanced analytics. Python's data science libraries provide a robust framework for handling everything from basic data operations to advanced machine learning. Below is a breakdown of the most important Python libraries for data science, their descriptions, and typical use cases.
Library |
Description |
Use Case/Applications |
Pandas |
Provides data structures like DataFrames for easy data manipulation. |
Data cleaning, wrangling, and analysis. |
NumPy |
Supports large, multi-dimensional arrays and matrices, along with mathematical functions. |
Numerical computations and array operations. |
Matplotlib |
A plotting library for creating static, interactive, and animated visualizations. |
Data visualization and creating charts/graphs. |
Seaborn |
Built on Matplotlib, it provides a high-level interface for drawing attractive statistical graphics. |
Advanced visualization, heatmaps, and pair plots. |
SciPy |
Provides modules for optimization, integration, and linear algebra. |
Scientific computing and solving differential equations. |
Statsmodels |
Enables users to explore data and estimate statistical models. |
Statistical testing and model building. |
Scikit-learn |
Offers simple and efficient tools for data mining and machine learning. |
Classification, regression, clustering. |
PyTorch |
An open-source machine learning library based on the Torch library. |
Deep learning and tensor computations. |
Eli5 |
Explains predictions from machine learning models. |
Debugging and understanding ML model outputs. |
LightGBM |
A gradient boosting framework designed for fast and accurate predictions. |
Large-scale data classification and ranking. |
Example Of Pandas Python Library –Data Analysis
The Python program example below illustrates how to use the pandas Python library and its contents to analyse a dataset.
Code Example:
# Step 1: Import the Pandas library
import pandas as pd
# Step 2: Create a simple DataFrame (no external CSV required)
data = {
'Name': ['Shivani', 'Anisha', 'Tara', 'Ananya', 'Srishti'],
'Age': [24, 27, 22, 32, 29],
'City': ['Delhi', 'Bengalore', 'Coimbatore', 'Kolkata', 'Patna']
}
# Create a DataFrame from the dictionary
df = pd.DataFrame(data)
# Step 3: Display the first few rows of the dataset
print("First 3 rows of the dataset:")
print(df.head(3))
# Step 4: Get a summary of the dataset
print("\nSummary of the dataset:")
print(df.describe())
Output:
First 3 rows of the dataset:
Name ... City
0 Shivani ... Delhi
1 Anisha ... Bengalore
2 Tara ... Coimbatore
[3 rows x 3 columns]
Summary of the dataset:
Age
count 5.000000
mean 26.800000
std 3.962323
min 22.000000
25% 24.000000
50% 27.000000
75% 29.000000
max 32.000000
Explanation:
In the Python code example, we demonstrate the use of Pandas, one of the most widely used Python libraries for data manipulation and analysis.
- We start by importing the pandas library, commonly abbreviated as pd, so we can use its functions in our code.
- Then, using pd.read_csv('sample_data.csv'), we read a CSV file named 'sample_data.csv' into a Pandas DataFrame. The DataFrame is essentially a table-like structure, which makes it easy to handle and analyze data in a structured format (rows and columns).
- By calling data.head(), we display the first 5 rows of the DataFrame. This function is helpful to quickly inspect the data, ensuring it was loaded correctly and get an initial sense of the structure.
- Finally, we use data.describe() to get the summary of the dataset, including statistical details like the mean, standard deviation, min, max, and quartiles for each numerical column. This helps us understand the distribution and range of the data at a glance.
By following these steps, we use Pandas to easily load, inspect, and summarize data, which are critical tasks in data science workflows. This flow illustrates how Pandas facilitates the efficient handling of data, empowering data scientists to focus on analysis and model building without worrying about raw data manipulation. The same applies to other Python libraries.
Important Python Libraries For Machine & Deep Learning
Python's dominance in machine learning (ML) and deep learning (DL) stems from its versatile libraries. These libraries simplify tasks such as building predictive models, handling big data, and deploying neural networks. Whether you're training a simple regression model or developing state-of-the-art neural networks, Python's ML and DL libraries have you covered.
In the table below, we have listed some of the most important Python libraries for this segment, along with their functionalities and applications.
List Of Python Libraries For Machine & Deep Learning
Library |
Description |
Use Case/Applications |
Scikit-learn |
A go-to library for traditional ML algorithms. |
Classification, regression, clustering, preprocessing. |
TensorFlow |
A library for high-performance numerical computations and machine learning, developed by Google. |
Neural networks, deep learning, and scalable ML solutions. |
Keras |
High-level API for building neural networks, running on top of TensorFlow. |
Rapid prototyping and deep learning research. |
PyTorch |
Flexible, dynamic deep learning framework from Facebook. |
Tensors, neural networks, and dynamic computational graphs. |
LightGBM |
Gradient boosting framework optimized for speed and accuracy. |
Large-scale data, ranking, and classification tasks. |
Eli5 |
Simplifies model explanation and debugging. |
Explaining predictions from ML models. |
Theano |
Fast numerical computations, especially for deep learning. |
Custom neural network development. |
XGBoost |
Optimized gradient boosting library. |
Regression, classification, and ranking tasks. |
CatBoost |
Yandex’s open-source gradient boosting on decision trees. |
Handling categorical data efficiently. |
MXNet |
Scalable deep learning framework, supported by Amazon. |
Distributed training and model deployment. |
Theano |
Allows efficient numerical computations, especially for deep learning. |
Mathematical computations and neural network development. |
Note: All the libraries listed in this section are third-party libraries. We will discuss how to work with these third-party Python libraries in a later section, including installation and usage methods, so you can easily integrate them into your projects.
Example Of Scikit-learn Python Library– Simple Linear Regression
In the basic Python code example below, we have illustrated how to use the Scikit-learn library to build and evaluate a simple linear regression model.
Code Example:
from sklearn.linear_model import LinearRegression
import numpy as np
# Data (independent and dependent variables)
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([1.5, 3.7, 2.8, 4.9, 5.2])
# Step 1: Create a Linear Regression model
model = LinearRegression()
# Step 2: Fit the model with the data
model.fit(X, y)
# Step 3: Make a prediction
predicted_value = model.predict([[6]])
# Step 4: Print coefficients and predicted value
print("Slope (Coefficient):", model.coef_[0])
print("Intercept:", model.intercept_)
print("Prediction for X=6:", predicted_value[0])
Output:
Slope (Coefficient): 0.75
Intercept: 1.1
Prediction for X=6: 5.6
Explanation:
In the example Python program,
- We start by importing LinearRegression from Scikit-learn third-party Python library and the numpy library as np.
- Then, we prepare a simple dataset with independent and dependent variables. The dataset contains five data points where X is a 2D array representing our features, and y is a 1D array representing the target values.
- Next, we initialize a LinearRegression object, which serves as our model.
- The model is then fitted to the dataset using model.fit(X, y), allowing it to learn the relationship between X and y.
- After training, we make a prediction using model.predict([[6]]). This predicts the output for a new input value of X=6.
- Finally, we print the model's slope (coefficient), intercept, and predicted value, providing insight into the linear relationship it has learned.
Important Note: Before running this code, ensure that the Scikit-learn library is installed in your Python environment. If it's not installed, you'll encounter an error similar to this:
ModuleNotFoundError: No module named 'sklearn'
To resolve this, you can install Scikit-learn by running the following command in your terminal or command prompt (we will discuss this in detail in a later section):
pip install scikit-learn
If you're working within a Python IDE, the IDE may prompt you to install the required library, or you may need to install it manually in your terminal before executing the code.
Other Important Python Libraries You Must Know
While Python's most popular libraries are often those tied to specific fields like data science, machine learning, or web development, there are numerous other libraries that are essential for everyday tasks, making your life as a developer easier and more productive.
In the table below, we have listed the Python libraries covering a wide range of uses, from handling HTTP requests to working with databases or automating repetitive tasks.
Library Name |
Description |
Use Case / Applications |
Requests |
A simple yet elegant HTTP library for sending HTTP requests. |
API interaction, web scraping, and making HTTP requests to web servers. |
SQLAlchemy |
A SQL toolkit and Object-Relational Mapping (ORM) library for Python. |
Database manipulation, creating and querying databases. |
BeautifulSoup |
A library for parsing HTML and XML documents, commonly used in web scraping. |
Extracting data from websites and web scraping projects. |
Pillow |
A Python Imaging Library that adds image processing capabilities. |
Image manipulation, creating thumbnails, editing image files. |
Scrapy |
A powerful web scraping framework used to extract data from websites. |
Web scraping, crawling websites, gathering data for analysis. |
PyGame |
A set of Python modules designed for writing video games. |
Game development, building 2D games, simulations. |
Flask |
A lightweight web framework for building web applications. |
Developing web applications, APIs, and web servers. |
Django |
A high-level Python web framework that encourages rapid development and clean design. |
Full-fledged web applications, REST APIs, and backend development. |
pySerial |
A library to communicate with devices through the serial port. |
Interfacing with hardware and building IoT applications. |
pytest |
A testing framework for Python that simplifies writing tests. |
Unit testing, test automation, and ensuring code reliability. |
Paramiko |
A library for working with SSH, making it easier to automate server access. |
Automating server administration, SSH access, and file transfers. |
Celery |
A distributed task queue for handling asynchronous tasks. |
Background task management and scheduling tasks in web apps. |
Example For Requests Python Library
The following example demonstrates how to make a simple GET request to a website and handle the response.
Code Example:
# Step 1: Import the Requests library
import requests
# Step 2: Make a GET request to a website (e.g., fetching Python's official homepage)
response = requests.get('https://www.python.org')
# Step 3: Check if the request was successful (status code 200 means success)
if response.status_code == 200:
print("Request was successful!")
# Step 4: Print the first 500 characters of the HTML content
print(response.text[:500])
else:
print("Failed to retrieve the website.")
Explanation:
In the example Python code,
- We first import the requests library so we can make HTTP requests easily.
- Then, we use requests.get() to send a GET request to Python’s official website. The response object contains all the information returned by the server.
- Next, we use an if-statement to check if the status_code of the response is 200, which indicates that the request was successful. If successful, we proceed to print part of the HTML content.
- If the request is successful, the first 500 characters of the response text (the HTML content of the website) are printed. If not, an error message is displayed.
This simple example demonstrates how to fetch data from a website using the Requests library and handle responses effectively, which is useful in scenarios like web scraping, API interaction, or interacting with web servers in general. Note that this is also a third-party library, so check out the next section to learn how to work with these Python libraries.
Level up your coding skills with the 100-Day Coding Sprint at Unstop and get the bragging rights, now!
Working With Third-Party Python Libraries
Third-party libraries in Python are those that are not included in the standard Python distribution but offer valuable functionality. They are created by other developers and are made available for public use.
- These libraries extend Python's capabilities, allowing you to avoid reinventing the wheel for common (and complex) tasks.
- Examples include libraries like requests for HTTP operations, matplotlib for plotting, and scikit-learn for machine learning.
To use these libraries in your Python projects, you need to install them (since they are not a part of the default Python environment) and then import them into your code.
Installing Third-Party Python Libraries
While Python provides a large set of standard libraries, third-party libraries need to be installed separately, as they are not bundled with Python by default. Installing third-party libraries ensures that you can access extra functionality that is useful for specialized tasks such as data science, machine learning, web scraping, and more.
Import vs Installation: Importing brings a library into your current environment, but you can only import a library if it’s already installed. Without installation, Python won’t know where to find the library.
Here are the most common methods to install third-party libraries:
- Using pip (Python Package Installer): The most widely used tool for installing third-party libraries in Python is pip. It downloads and installs the library from the Python Package Index (PyPI). To install a library, simply run the following command in your terminal:
pip install library_name
pip install requests #This installs the requests library
- Using conda: If you use Anaconda (a popular distribution for data science and machine learning), you can use the conda command to install third-party libraries. This method is especially useful when you're managing a data science environment where compatibility with certain libraries is critical.
To install a library using conda:
conda install library_name
conda install numpy # This installs the numpy library
- Installing from a Local File or URL: If the library isn't available on PyPI or conda, or if you have a custom version, you can install it from a local file or GitHub URL. Use the following command to install from a local file:
pip install /path/to/library.tar.gz
Or, to install directly from a GitHub repository:
pip install git+https://github.com/username/repository.git
- Installing Multiple Libraries at Once: If you have several libraries to install, you can list them all in a requirements.txt file. This is especially useful for projects where you want to ensure that every team member is using the same set of libraries. Here’s how you do it:
- Create a requirements.txt file with the names of the libraries you need.
- Run the command:
pip install -r requirements.txt
This will install all the libraries listed in the file.
Creating Your Own Python Libraries
Creating your own third-party libraries allows you to share reusable code with others or within your team. When creating a Python library, you typically want it to be modular, well-documented, and easy to install.
Here’s how you can create your own Python library:
- Create a New Directory for Your Library: Create a new folder and name it after your library. For example, if you are making a library called mylibrary, create a directory named mylibrary.
- Add a Python File: Inside this directory, create a Python file (e.g., mylibrary.py). Here’s an example of a simple function:
# mylibrary.py
def greet(name):
return f"Hello, {name}!"
- Add a Setup File: To make your library installable, add a setup.py file. This file contains metadata about your library, such as its name and version.
from setuptools import setup
setup(
name="mylibrary",
version="0.1",
description="A simple greeting library",
py_modules=["mylibrary"],
install_requires=[],
)
- Install Your Library Locally: Once you’ve set up your library, you can install it locally by running:
pip install .
This command installs your library in the current environment, and you can now use it by importing it like any other Python library.
Troubleshooting Common Issues For Python Libraries
Even though Python libraries are incredibly useful, you may run into problems during their installation, import, or usage. In this section, we will discuss the two most common issues you might encounter when working with Python libraries and provide solutions to resolve them.
Python Library Import Errors
One of the most common issues when working with Python libraries is import errors. This happens when Python cannot find the library you're trying to import or if the library isn’t installed correctly.
Common Causes:
- The library is not installed.
- You are trying to import a module or function that doesn’t exist in the library.
- There are naming conflicts, such as using the same name for a variable and a module.
Example of Import Error:
import nonexistent_library
Output:
ModuleNotFoundError: No module named 'nonexistent_library'
Solution:
- Ensure that the library is installed using pip install library_name or conda install library_name.
- Double-check that you’ve spelt the library name correctly.
- Make sure the library is available in the current Python environment. If you’re using a virtual environment, activate it before running your code.
Best Practice: Use try-except blocks to handle import errors gracefully
try:
import numpy
except ImportError:
print("Numpy is not installed. Installing now...")
!pip install numpy
Version Conflicts When Using Python Libraries
Sometimes, you may encounter version conflicts between different libraries or between a library and the Python version you're using. This often happens when multiple versions of a library exist or when an outdated version is incompatible with the other libraries in your project.
Example of Version Conflict:
pip install numpy==1.18
pip install pandas==1.0
Output (potential conflict message):
ERROR: Could not find a version that satisfies the requirement pandas==1.0 (from versions: 1.1, 1.2, ...)
Solution:
- Use pip freeze to check the installed versions of libraries and check if the versions are compatible.
- You can create a requirements.txt file to pin exact versions of libraries that work well together:
pip freeze > requirements.txt
- Use a virtual environment to avoid conflicts between global and project-specific libraries.
- Upgrade or downgrade libraries using:
pip install library_name==version_number
Python Libraries In Larger Projects
When working on larger projects, managing multiple Python libraries and dependencies can become complicated. It's crucial to ensure that all libraries are compatible and easy to manage as your project grows.
Best Practices For Managing Python Libraries In Larger Projects
- Use a Virtual Environment: Isolate your project’s libraries from the global Python environment to avoid version conflicts. You can create a virtual environment using:
python -m venv myenv
- And activate it using:
source myenv/bin/activate # on macOS/Linux
myenv\Scripts\activate # on Windows
- Use requirements.txt: Keep track of the libraries required for your project in a requirements.txt file. This makes it easy to recreate the environment in the future or share the project with others:
pip freeze > requirements.txt
- Use Docker: For more advanced setups, you can containerize your project using Docker, ensuring that the entire development environment (including libraries) is consistent across different machines and operating systems.
Looking for guidance? Find the perfect mentor from select experienced coding & software experts here.
Importance Of Python Libraries
Python libraries play a crucial role in simplifying programming tasks and enhancing productivity. Here’s why they matter:
- Simplify Complex Tasks: Libraries provide pre-built functions and tools, allowing you to execute complex tasks with minimal effort.
Example: numpy simplifies numerical operations, while pandas streamlines data manipulation. - Save Time and Effort: Instead of writing code from scratch, you can leverage libraries to achieve results faster.
Example: Plotting data using matplotlib takes seconds compared to manually coding the entire visualization. - Enhance Code Efficiency: Libraries are optimized for performance, often outperforming custom solutions.
Example: Machine learning tasks are significantly faster using scikit-learn or TensorFlow. - Ensure Code Reusability: By using well-tested libraries, you can avoid duplicating work and focus on the unique aspects of your project.
Example: Web development frameworks like Flask or Django offer reusable components for building scalable applications. - Access to Cutting-Edge Technologies: Libraries provide easy access to advanced features like deep learning, big data analysis, and real-time applications.
Example: PyTorch and Keras allow even beginners to build neural networks for deep learning. - Community Support and Reliability: Popular libraries are maintained by large communities, ensuring regular updates, bug fixes, and extensive documentation.
Example: The requests is a highly reliable library for handling HTTP requests, with a robust support network.
Conclusion
In this article, we've explored the power and versatility of Python libraries, from understanding their role in simplifying code to diving deep into installation, usage, and troubleshooting. Libraries are at the heart of what makes Python an efficient and powerful tool for developers, whether you're analyzing data, building machine learning models, or automating tasks. We’ve also learned about the installation of third-party libraries and how to create and manage your own, ensuring you’re fully equipped to harness the full potential of Python’s ecosystem.
By now, you should have a solid understanding of how to import and use both standard and third-party Python libraries, troubleshoot common issues, and structure your Python projects for maximum efficiency.
Frequently Asked Questions
Q1. What is a module in Python?
A Python module is a file containing Python definitions and statements. It can define functions, classes, and variables. A module allows you to organize your code into reusable sections, making your projects more manageable and easier to maintain.
Q2. What's the difference between a module and a library in Python?
A module is a single file of Python code, whereas a library is a collection of modules that provides additional functionality to your code. Python libraries are often made up of multiple modules that handle different aspects of functionality.
Q3. Can I use Python libraries without installing them?
Yes, many Python libraries come pre-installed with the standard Python distribution, so you can directly import them into your code without installing them. However, third-party libraries need to be installed using tools like pip or conda.
Q4. How do I check which libraries are installed in my environment?
You can use the following command to list all installed libraries:
pip list
Q5. What is the purpose of using virtual environments in Python?
Virtual environments help isolate project-specific libraries from the global Python environment. This ensures that dependencies for one project do not interfere with another and makes it easier to manage different versions of Python libraries.
Q. How do I resolve an ImportError?
An ImportError typically occurs when a library or module isn't installed or isn't found by Python. You can resolve this by ensuring the library is installed with pip or conda and that it's available in the correct environment.
Q. How can I upgrade or downgrade a library?
You can use the following commands to upgrade or downgrade libraries:
To upgrade:
pip install --upgrade library_name
To downgrade to a specific version:
pip install library_name==version_number
Q. Can I create my own Python libraries?
Yes, you can create your own Python libraries by organizing your code into modules and packaging them for reuse. Once packaged, your library can be shared with others or used in different projects.
Quick Quiz On Python Libraries – Let’s Go!
Here are a few other Python topics you must explore:
- Find Length Of List In Python | 8 Ways (+Examples) & Analysis
- Python Namespace & Variable Scope Explained (With Code Examples)
- Convert Int To String In Python | Learn 6 Methods With Examples
- Python Bitwise Operators | Positive & Negative Numbers (+Examples)
- Python max() Function With Objects & Iterables (+Code Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Anish Malik 19 hours ago
Ujjwal Sharma 1 day ago
Pranjul Srivastav 3 days ago
Anjali Nimesh 1 week ago
Anjali Nimesh 6 days ago
T S ADITYA 1 week ago
kavita Prajapati 3 weeks ago