Convert Int To String In Python | Learn 6 Methods With Examples
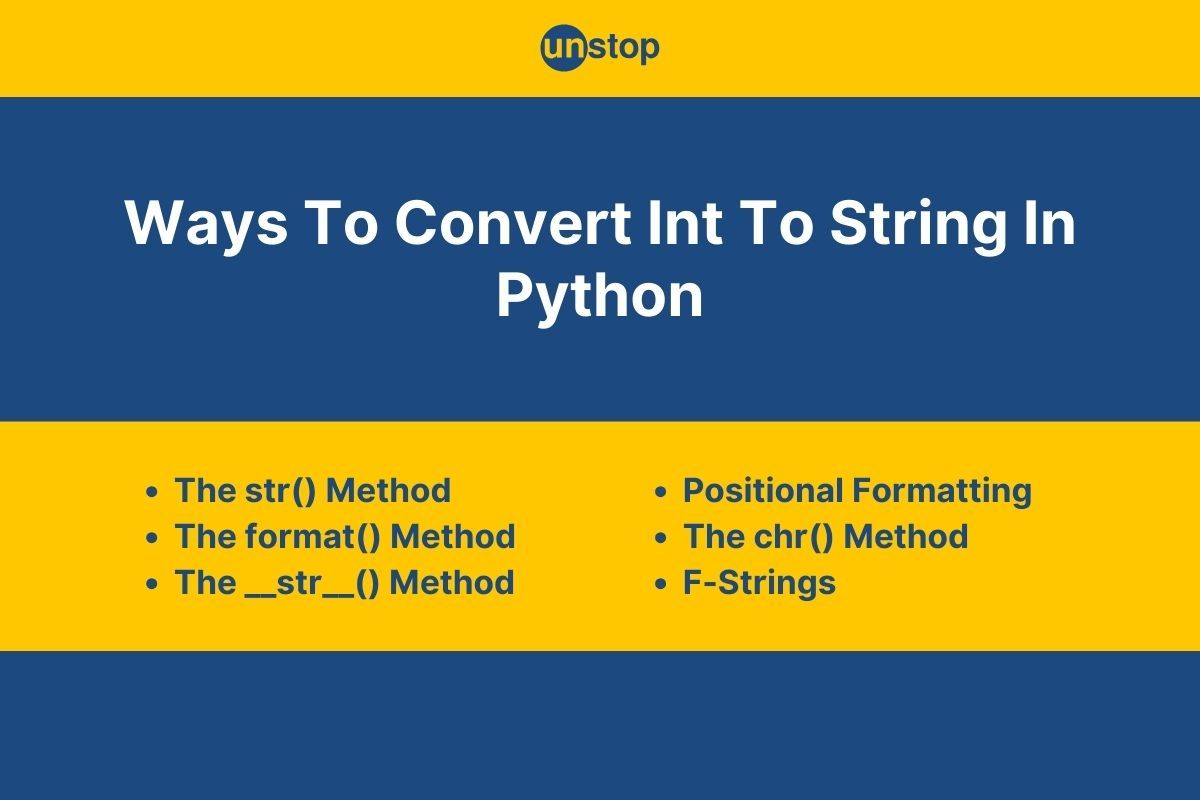
Python, renowned for its simplicity and versatility, offers numerous functionalities that make programming tasks a breeze. One such fundamental operation is converting integers to strings, a task that might seem straightforward but holds nuances that can trip up even seasoned developers in their daily programming progress.
Understanding how to effectively convert integers to strings is crucial for various applications, from data manipulation to user interface interactions and mathematical operations. In this article, we will learn about how to convert int to string in Python language and various methods used for this purpose like str() function, string formatting, format() method, F-strings, positional formatting, __str__() method and chr() function with examples.
Introduction to Integer In Python
Integer is a data type in Python programming that consists of whole numbers, including positive, negative, and zero. However, it does not include fractional, decimal, or floating-point numbers.
- There is no limit to the length of an integer in Python, meaning that it can be of any length.
- Integers are immutable in nature, i.e., their value cannot be changed after creation.
- Integer values can be created by simply initializing a variable with any list of integer values.
- Integers can be binary, octal, or hexadecimal too. If a number has 0b in its front, it is a binary number. If a number has 0o in front of it, it is an octal number. If a number has 0x in front of it, it is a hexadecimal number.
Code Example:
Output:
6
85
354152
Explanation:
In this Python example-
- We first initialize a variable a with binary value 0b0110 and display the value to the console using the print() function.
- Similarly, we declare and initialize variable b with an octal value of 0o125 and display it.
- At last, we initialize variable c with hexadecimal value 0x56768 and also display it on the output window.
What Are Strings In Python?
Strings in Python are represented as a sequence of characters enclosed by single quotes, double quotes, or triple quotes.
- Single and double quotes are considered equivalent in Python and triple quotes are used to represent multi-line strings.
- Initial strings are immutable in nature. It means the characters of strings cannot be changed once the string is created.
- Strings can be created by simply assigning a sequence of characters to a variable. It can contain alphabetic letters, numbers, spaces, symbols and all other characters.
Code Example:
Output:
String in Python
String in Python
This is a
multiline string.
Explanation:
In this sample Python program-
- As mentioned in the code comments, a variable s1 is initialized with a string value enclosed in single quotes i.e., ‘String in Python’.
- Then, a variable s2 is initialized with a string value enclosed in double quotes “String in Python”.
- After that, a variable s3 is initialized with a multiline string value enclosed in triple quotes.
- Then, we call the print() function on all three of the variables one by one to print their values as output.
How To Convert Int To String In Python?
In Python, converting an integer to a string is a fundamental operation frequently encountered in programming tasks. This type conversion can be accomplished through various built-in methods, each offering its own advantages and use cases.
One common approach to convert int to string in Python is to use built-in functions like str(), which directly converts an integer to its string representation. For instance, str(123) returns the string '123'. Another method involves string formatting techniques such as the %s keyword/ format specifier or the format() method. All of them are based on a general algorithm that is followed to complete the conversion.
The general algorithm to convert int to string in Python is as follows:
Step 1- Initialize the variable: Initialize a variable with the integer value that you want to convert into string.
Step 2- Call the function: There are various methods to convert integer to string in Python which can be called according to their syntax. The user can choose any function according to their preference. The most common function used for this purpose is the str() function. It is called by passing the integer value to be converted into a string inside the function as an argument.
Step 3- Store Result: Store the output of the function in another variable.
Step 4- Print the string: Print the output string stored in the resultant variable
Convert Int To String In Python Using The str() Function
Python has a built-in function called str() function that can convert any data type to a string. Integers, floats, lists, tuples, and other data types can easily undergo string conversions using the str() method. So we can use it to convert int to string in Python. The str() function is often used in combination with the print() function to display the output to the user.
Syntax:
str(integer_value)
Here,
- str(): It is the name of the function used for int conversion to string.
- integer_value: It is the integer value that is passed as an argument to the str() function to convert into string.
Here is a sample program to convert int to string in Python language using str() function:
Code Example:
Output:
468
<class ‘str’>
Explanation:
In the simple Python program-
- Firstly, we initialized an integer variable a with value 468.
- Then, we call the str() function on variable a and stored the output in another variable s.
- We then print the value of th string using the print() function.
- The value of s was printed in the output which was the converted string version of the value stored in a.
- At last, we use the type() function to verify the identify that the value of s is a string value.
Convert Int To String In Python Using format() Method
The format() method is one of the methods to convert int to string in Python using string formatting. It takes any variable as an input and converts it into a string if it is not already one. In this method, the curly braces {} are used as placeholders for the integer value and the format() method is called on the desired string to replace the placeholders with that integer value.
Syntax:
‘{}’.format(integer)
Here,
- {}: The curly brackets are used to define the placeholder.
- format(): It is the name of the method to convert int to string.
- integer: It is the integer value that is passed in the format() method as an argument to be converted into string.
Here’s an example of how to convert integers into strings in Python using the string format() method:
Code Example:
Output:
The value of n is 567.
Explanation:
In the Python code example-
- We start by initializing a variable n with the value 567.
- Then, we create a string s where curly braces are the placeholder for the value of n.
- Next, we call the format() method on the string s, passing the value of n as an argument. This method replaces the {} placeholder in the string with the provided value.
- When we display the value of variable s using the print() function, the outcome is the value of n inserted inside the string.
Convert Int To String In Python Using F-strings
The f-strings, i.e., Formatted String Literal, offer a concise and readable syntax for string formatting, including the conversion of integers to strings.
- Using f-strings, we can embed expressions directly within string literals by prefixing the string with the letter f.
- Inside the string, we can place curly braces {} to enclose expressions, including integer variables, that will be evaluated and replaced with their string representations at runtime.
- This allows for seamless integration of integer values into strings without the need for explicit conversion functions like str().
- F-strings are highly versatile, supporting various formatting options and expressions, making them a powerful tool for string manipulation.
Syntax:
f’{integer}’
Here,
- f: It is the prefix to be attached in this method.
- integer: It is the integer value which is to be converted to string. It is placed inside curly brackets enclosed in quotes after the prefix f.
Here is an example of how to convert int to string using f-strings.
Code Example:
Output:
The integer value is: 123
Explanation:
In the Python program example-
- We define an integer variable integer_value with a value of 123.
- Using an f-string (f""), we create a string literal where {integer_value} represents the expression to be evaluated and replaced with its string representation.
- When the f-string is evaluated, integer_value is converted to its string representation and substituted into the string.
- Finally, we print the resulting string, which contains the integer value converted to a string.
Convert Int To String In Python Using %s Keyword (Positional Formatting)
The %s keyword is another method to convert int to string in Python. This method is also known as positional formatting. The %s keyword is a format specifier which indicates that the formatted value should be treated as a string. This method allows us to embed integer values directly into a string and have them automatically converted to their string representations during formatting.
Syntax:
“%s” % integer
Here,
- %s: It is written inside quotes and is used as a placeholder.
- %integer: The % symbol is written, and then the integer value is written, which is to be converted into a string.
The example below explains how to convert int to string in Python using positional formatting.
Code Example:
Output:
The value of n is 67.
Explanation:
In the example Python code-
- We assign the value 67 to the variable n.
- Next, we create a string variable s using string formatting. The %s placeholder in the string indicates that we'll replace it with the value of n.
- We use the % operator to perform string formatting, where % n indicates that the value of n will be inserted into the string at the placeholder %s.
- Then, we print the formatted string s using the print() function.
Convert Int To String In Python Using __str__() Method
In Python, the __str__() method is a special method that defines the string representation of an object. By overriding this method in a custom class, you can define how instances of that class should be converted to strings. While this method is primarily used within class definitions, it can also be leveraged to convert integers to strings by first encapsulating the integer within a custom class and then defining the __str__() method to return its string representation.
Syntax:
integer.__str__()
Here,
- integer: It is the integer value that is to be converted into a string.
- __str__(): It is the name of the function used to convert integer to string.
Code Example:
Output:
259
<class ‘str’>
Explanation:
In the above code-
- We initialize a variable n with the value 259.
- Next, we call the __str__() method on the variable n. This method converts the integer n into its string representation.
- Then, we print the value of s, which now holds the string representation of the integer n.
- After that, we print the type of s using the type() function to determine the data type of the variable s.
- In this case, since we've converted n to a string, the output would be the string '259'. The type of s would be <class 'str'>, indicating that s is of type string.
Convert Int To String In Python Using chr() Function
The chr() function in Python takes an integer argument representing a Unicode code point and returns the corresponding character. It can take values from 0 to 1,114,111 numbers. For instance, chr(65) returns the character 'A', as 65 represents the Unicode code point for the uppercase letter 'A'.
To convert an integer to a string using the chr() function, you can simply pass the integer value as an argument to chr(). However, it's important to note that this method works effectively for integers that correspond to valid ASCII values. For integers outside the ASCII range, you may encounter errors or unexpected behaviour.
Working Mechanism: To convert int to string in Python using chr(), we-
- First, add the ASCII code of character 0 to the integer.
- Then, it is passed to the chr() function which provides the corresponding character to the resultant ASCII code.
- This process is repeated until all numbers of the integer are converted to string.
Syntax:
chr(integer)
Here,
- chr(): It is the name of the function used to convert int to string.
- integer: It is the integer value passed as an argument to chr() function to be converted to string.
Here is an example of how to convert int to string using chr() function:
Code Example:
Output:
Integer 65 converted to string: A
Explanation:
In the above code,
- We define a function int_to_string which takes an integer num as input.
- Inside the function, we attempt to convert the integer num into its corresponding ASCII character using the chr() function.
- If the conversion is successful, we assign the result to the variable string_num.
- If the conversion encounters a ValueError, indicating that the integer value is out of the range of valid ASCII characters, we return an error message string.
- Finally, we return either the converted string or an error message.
- There's an example usage provided where an integer value 65 is passed to the int_to_string function, and the result is printed out. In this example, the integer 65 corresponds to the ASCII character 'A', so the output of the example would be "Integer 65 converted to string: A".
Conclusion
Mastering the conversion of integers to strings in Python is a fundamental skill that every developer should possess. In this article, we have discussed how to convert int to string in Python in various ways. These range from use of built-in functions like str() to techniques like f-strings and string formatting. Understanding these techniques equips you with the flexibility to handle diverse scenarios efficiently, whether you're working on data processing tasks, building user interfaces, or developing web applications.
Frequently Asked Questions
Q. Does Python automatically convert int to string?
No, Python does not convert int to string automatically. There are many languages that automatically convert int to string while performing string operations, but Python is not one of them. There are various methods that describe how to convert int to string in Python like str() function, format() method, f-strings, positional formatting, __str__() method and chr() function.
The str() function is the most common method among them all. It takes integer value as an argument and converts it into string.
Q. How to use toString() in Python?
Unlike other languages, there is no specific method named toString() in Python. The main function of the toString() method is to convert any value to string. This can be accomplished in Python using the built-in str() function. The str() function converts any value to string.
Q. What is the easiest way to convert an integer to string in Python?
The easiest way to convert int to string in Python is by implementing the str() function of Python. The str() function takes an integer value as input and converts it into string value automatically using its built-in mechanism. It is called the easiest of all methods because its syntax is very simple and also its functioning is easy to understand unlike other methods.
Code Example:
Output:
12
<class ‘int’>
12
<class ‘str’>
Explanation:
The code initializes variable x with the value 12, prints its value (12) and type (int), then converts x into a string y using str() function, prints its value ('12'), and its type (str).
Q. What is type() function in Python?
The type() function is a built-in function in Python which is used to return the type of variable or value inputted as its argument. It is an easy-to-use function which is beneficial in determining the type of a variable or value. It returns the name of the class to which the given object belongs.
Syntax:
type(object)
Example:
print(type(10)) # This will return int type, i.e., Output: <class 'int'>
Q. How to convert string to integer in Python?
The method to convert string to integer in Python is as easy as converting an int to string in Python. The function used to convert strings to integer in Python is int() method. It takes a string as its argument and converts that string to integer. Then, we can perform the desired operations on that numeric value.
Syntax:
int(string value)
Example:
Output:
<class ‘str’>
<class ‘int’>
Explanation:
The code first initializes a variable s with the string value "4567", then prints its type (str). Next, it converts s into an integer using int() function and assigns the result back to s, and prints its type (int).
Q. How to change datatype in Python?
There are various ways to convert or change one datatype to another datatype. Just like in the article, we learnt about how to convert integer to string in Python. In the similar manner, other string data types can also be type casted.
The string can be changed to integer using int() function, integer can be changed in string using str() function, integer can be changed into float using built-in float() function. All these methods are discussed in detail in the article.
There are other methods too for conversion between tuples and lists like tuple() function and list() function.
Q. How to convert integer to binary string in Python?
A binary string is a string in Python which consists of only 1 or 0 as characters. If it contains any other character then it is classified as non-binary string.There are two methods to convert integer to binary string in Python – bin() method and format() method.
The bin() method takes an integer value as input and generates its corresponding binary string with 0b prefix.
Syntax:
bin(integer)
Example:
print(bin(956))
Output:
0b1110111100
Another solution is the format() method, which takes an integer and its value specifier as input, which is b for binary string, and then generates the corresponding binary string for the number.
Syntax:
format(value, value specifier)
Example:
print(format(358, 'b'))
Output:
101100110
We now know about the various techniques we can employ to convert int to string in Python. Here are a few other interesting topics you must read:
- Python IDLE | The Ultimate Beginner's Guide With Images & Codes!
- How To Reverse A String In Python? 10 Easy Ways With Examples!
- How To Convert Python List To String? 8 Ways Explained (+Examples)
- Python Logical Operators, Short-Circuiting & More (With Examples)
- 12 Ways To Compare Strings In Python Explained (With Examples)
- Relational Operators In Python | 6 Types, Usage, Examples & More!
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment