How To Convert Python List To String? 8 Ways Explained (+Examples)
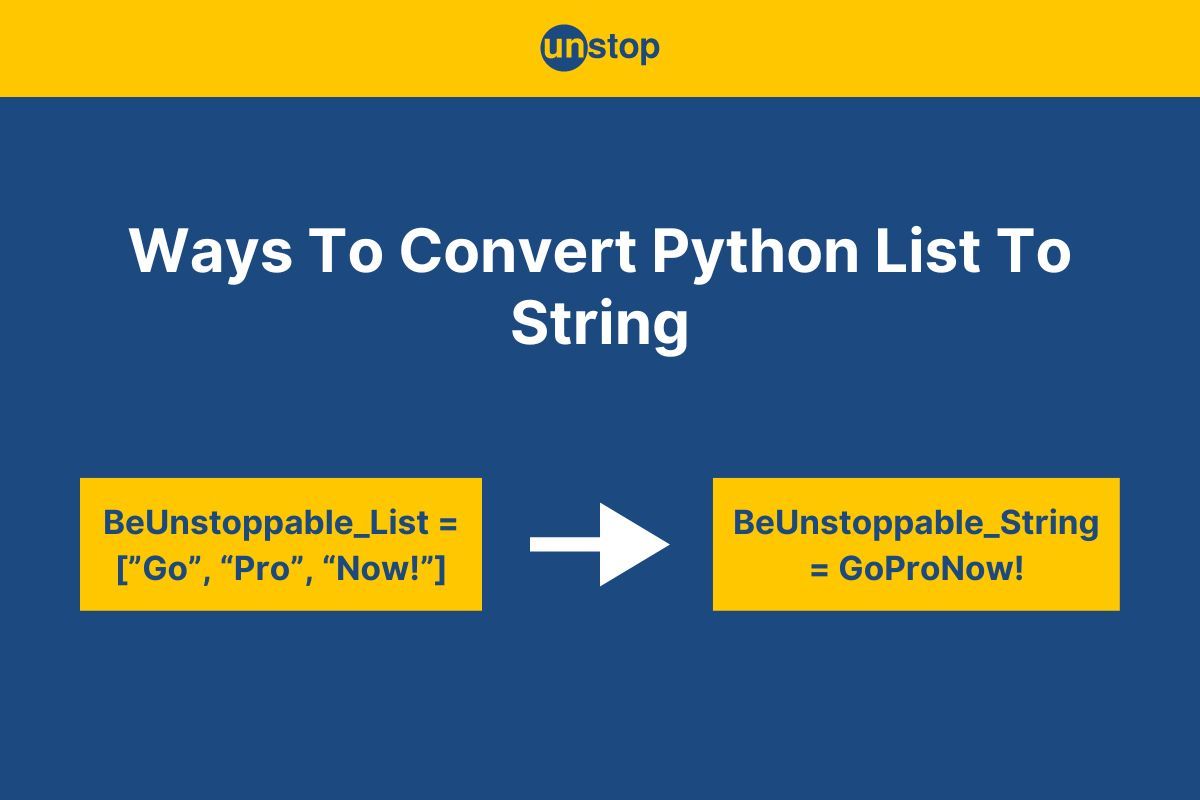
Lists are powerful data structures in Python language that allow users to store and manipulate the collection of items. While they are extremely useful, there are times when we might need to convert a list to a string for purposes like file handling, database querying, etc. Python provides multiple straightforward methods for transforming a list into a string format. In this article, we will explore all the Python list to string conversion methods along with detailed explanations and examples.
What Is A List In Python?
A list is a Python built-in collective data type that stores multiple heterogeneous values under a single variable name. This unique feature sets the Python lists apart from the other programming languages.
- The items stored in the list are ordered, which means they have a positional value they can easily access using their indices.
- We use square brackets [] to create lists in Python language. These brackets can also be used to retrieve the element in the list with its positional value inside the brackets.
- Lists are mutable, which means we can change the elements within a list by accessing them and directly assigning new values to them.
This shows that lists are versatile and widely used due to flexibility and ease of use. Some of the primary purposes of using lists that feed into the need to convert a Python list to string include organizing information, prioritizing tasks, memory recall, and more.
Syntax:
list = [item1,item2,item3,item4…]
Here,
- The list is a variable name that is used to store the values.
- [item1,item2,item3,item4…] are heterogeneous or homogeneous values.
What Is A String In Python?
A string is a sequence of characters, either as a literal constant or as a variable. Like most other programming languages, strings in Python are used to represent sequences of characters rather than numbers.
- The sequence of characters of a string is enclosed within either single quotes (‘...’) or double quotes (“....”) and may consist of letters, integer elements, symbols, and whitespaces.
- Strings are widely used for text processing tasks, including searching and manipulating text data.
- They are immutable, meaning a string cannot be modified once created. However, we can create a new individual string based on an original string by slicing it. We can also access and perform other operations on strings, like concatenating one string to another, indexing the substring, and formatting the string.
- We can modify string data whenever an update occurs, like the misplacement of a letter. In that case, we can use the replace method and split a string into multiple substrings based on the delimiter.
- Python strings also play a crucial role in reading and writing data from and to files.
- We can create common error messages that handle the exceptions in a human-readable format.
Syntax:
string_name = ’string_value’
string_name = "string_value"
Here, the name of the string is given by string_name, and the characters/ sequence of characters that make up the string are represented by string_value.
Now that we have a brief idea of what lists and strings are, let's move to discuss why we might need to convert a Python list to string.
To know more about string, read- Python Strings | Create, Format, Reassign & More (+Examples)
Why Convert Python List To String?
Converting a Python list to a string can be useful in various scenarios, depending on the program's requirements. Some common reasons for converting a Python list to a string include:
- Serialization: When we need to store or transmit data in a format that can be easily reconstructed later, converting a list to a string is useful.
- Printing and Logging: If we want to print or log the contents of a list, converting it to a string allows us to display the elements in a more readable format.
- Data Persistence: When saving data to a file or a database, we might need to convert the list to a string before writing it.
- Data Manipulation: In Some cases, we might want to manipulate the list’s elements as a single string, such as performing string operations on each element.
- Interoperability: When working with external systems or APIs that expect string inputs, we might need to convert a list to a string to facilitate communication.
- Custom Formatting: Sometimes, we may want to create a custom string representation of a list, possibly with specific delimiters or formatting, for display or other purposes.
Let us look at the various methods to convert a Python List into a string.
How To Convert List To String In Python?
There are multiple ways in which we can convert a Python list to a string. These methods include the following:
- The join() Method
- Iterative Approach
- List Comprehension
- The map() Function
- The format() Function
- Recursive Approach
- Enumeration Function
- Operator Module
We will discuss each of these approaches and look at their detailed code implementations in the sections ahead.
Also read- Python IDLE | The Ultimate Beginner's Guide With Images & Codes!
The join() Method To Convert Python List To String
The join() method in Python is a powerful tool for converting a list to a string. This method joins elements of an iterable, such as a list, into a single string using a specified separator. It is extremely easy to use this inbuilt method, the syntax for which is given below.
Syntax:
string_result = separator.join(list)
Here,
- The list is the parameter of the join() function. It is an iterable object with a collection of string values.
- The separator refers to the delimiter that separates the elements of the list in the resulting string.
- The outcome of the joining operation is stored in the string_result variable.
How Does The join() Function?
As we've mentioned above, the join() function can take an iterable object (i.e., list or tuple) as a parameter.
- Once the argument is passed to the join() function, iterates through each element in the list.
- During iteration, the elements are concatenated into a single string with a specifier delimiter.
- The function returns the concatenated string, thus successfully converting the Python list to string.
Code Example:
Output:
This@is@Unstop
Explanation:
In the simple Python program-
- We define a list called my_list and initialize the list with elements/ strings- This, is, and Unstop, using the double quotes method and square brackets.
- Next, we call the join() method by passing the my_list variable as an argument. We use the at-the-rate (@) character as the delimiter to concatenate the list of elements.
- The outcome of the join() operation is stored in the data variable, which we then print to the console using the print() function.
Convert Python List To String Through Iteration
Another commonly used method to convert a Python list to a string is the iteration approach. Here, we use a for loop to iterate every element in the list and connect them using a delimiter.
- To begin with, we create a list with elements or strings and then declare an empty string.
- We then use a for loop to traverse every element in the list and concatenate the element to the previously declared string using a delimiter.
- The outcome is stored in the same variable, and this process is repeated until the end of the loop.
- Finally, we get the list in string format by concatenating every element of the list to a single variable.
Code Example:
Output:
Hi, Good Morning Unstopppables.
Explanation:
In the example Python code-
- We create a list named the_list with 4 elements, i.e., "Hi", "Good", "Morning", and "Unstoppable.".
- Then, we create an empty string variable called string1, that is, there is no value inside the quotes.
- Next, we use a for loop to iterate through each element in the list. In each iteration, we use the arithmetic addition operator to concatenate the current element with a space character (' ') and then add it to the string1 variable.
- Once the loop is done with iterations, the string1 variable will contain the joined list.
- We then display the outcome of the Ptyhon list to string conversion operation using the print() function.
Convert Python List To String With List Comprehension
List comprehension is a way to create a list in Python. It allows us to create a new list by specifying the elements we want to include, which are compact and readable. It creates a new list much faster than other traversal methods. List comprehension provides a concise way to achieve list-to-string conversion as it combines the iteration and concatenation steps into a single line of code.
- Here, we use the join() method and the str() function along with list comprehension.
- In this method, we even change the no-string value of a list to a string value using list comprehension. we convert the list into a string.
- This method is best suited for a situation where we want to convert no-string type values in the list into a string.
Syntax:
string_result=separator.join([element for element in list])
Here,
- The separator is the delimiter that separates the elements of the list in the resulting string.
- The join() is the string method that converts the list into a string, and [element for element in list] is its parameter/ list comprehension that returns a list.
- The string_result is a variable that stores the resulting value.
Code Example:
Output:
Dare 2 Compete
Explanation:
In the Python code snippet-
- We define a list named my_list with elements "Dare", 2, and "Compete".
- Then, we use a list comprehension within the join() method to convert the Python list to string.
- This list comprehension iterates over each element in my_list and converts that element to a string using the str() function.
- Then, the join() method concatenates each string representation of the elements from the list comprehension with a space character (' ') in between them.
- The resulting string from the join() operation is stored in the variable result. which we then display to the console using the print() function.
The map() Function To Convert Python List To String
The map() function is a Python built-in method that applies a specified function to all elements in an iterable (like list, tuple, set) object and returns an iterator object that produces the results. In converting a Python list to a string, we can use map() to transform each element into a string and then join them using the join() method.
Syntax:
result_var=separator.join(map(function, iterator))
Here,
- The separator is a delimiter that separates the elements of the list in the resulting string, and join() is a string method that converts the list into a string.
- The function may be a built-in function like str() or a user-defined function.
- An iterator is a collection of values, which may be a list, tuple, or set, and result_var is a variable that stores the resulting value.
Code Example:
Output:
Lion King released in 1994
Explanation:
In the sample Python program-
- We begin by defining a list named list_data with elements: "Lion", "King", "released", "in", 1992, separated by commas.
- Next, we use the map() function to apply the str() function to each element in the data list. This converts each element into its string representation.
- Then, the join() method is called on the resulting mapped iterable. It concatenates each string representation of the elements from the mapped iterable with a space character (' ') between them.
- The resulting string from the join() operation is stored in the variable result.
- Finally, we print the value of the variable result using the print() function.
Convert Python List to String Using format() Function
The format() method is a bulti-in method in Python that is used for string formatting. It provides a way to concatenate elements within a string by replacing placeholders with corresponding values. We use {} as placeholders, which will be used to replace the values specified in the format() method.
In this context, we can use the format() function to convert the no-string value to a string and then the rest of the concept is the same as the join() method to concatenate every element of the list to a string.
Syntax:
separator.join(['{}'.format(element) for element in list])
Here,
- separator.join([...]): Concatenates elements from a list into a single string with a specified separator.
- ['{}'.format(element) for element in list]: Converts each element in the list to a string format using format(), creating a new list.
Code Example:
Output:
Future of 2024
Explanation:
In the Python code example-
- We define a list named my_list with elements "Future", "of", and 2024.
- Next, we use a list comprehension is used to iterate over each element in my_list. Within the list comprehension, we use the format() method to convert each element into its string representation.
- Then, we call the join() method on the resulting list from the list comprehension. It concatenates each string representation of the elements from the list comprehension with a space character (' ') between them.
- The resulting string from the join() operation is stored in the variable result which we diaply using print() function.
Convert Python List To String Using Recursion
Recursion is one of the important topics in programming concepts, where a function calls itself according to its definition. Recursive functions divide a big problem into smaller problems and similar sub-problems and solve until the base condition is reached. The base condition plays a major role in recursion, if the base condition is not defined the recursive function will fall into the infinite loop as it calls by itself.
We can use a recursive function to convert a Python list to string where the base condition must be- if the index and length of the list equal, return an empty string. Then, we add every element of the list as a one-string variable until the base condition returns false.
Syntax:
def list_to_string_recursive(lst):
if base_condition: # Base case
return base_value
else: # Recursive case
return list_to_string_recursive(modified_args)
Here,
- def list_to_string_recursive(lst): defines the recursive function named
- list_to_string_recursive: that takes a list lst as an argument.
- if base_condition: checks whether the base condition is met.
- return base_value: returns a value when the base condition is satisfied.
- else: denotes the start of the block for handling recursive cases.
- return list_to_string_recursive(modified_args): makes a recursive call to the function with modified arguments.
Code Example:
Output:
Recursive solution rocks
Explanation:
In the Python code above-
- We first define a function named convert_list_to_string, which takes two parameters: my_list (a list) and index (an integer with a default value of 0).
- Inside the function, there's a base case that checks if the index is equal to the length of the list my_list. If the case is met, then the function returns an empty string. This serves as the stopping condition for the recursive calls.
- If the base case is not met, the code proceeds to convert the current element to a string using the str() function.
- Then, it recursively calls the convert_list_to_string function with an incremented index (index + 1) and stores the result in the variable resulted_string.
- Finally, it concatenates the current element with a space character (' ') and the resulted_string obtained from the recursive call and returns this concatenated string.
- Outside the function, a list named my_list is defined with elements "Recursive", "solution", and "rocks".
- Then, we call the convert_list_to_string function with my_list as an argument, and the returned value is stored in the variable result.
- Finally, the content of the result variable is printed using the print() function.
Enumeration Function To Convert Python List To String
An enumeration function is also called an enumerator or enum in a programming concept. The enumerate() function in Python is the same as the iterator. It returns the value of the iterator as a tuple consisting of its index and the value of each element in the iterable object.
The enumerate() function can be employed to access both the index and value of each element in the list, facilitating a customized concatenation process. In this context, we have access to its index, and we use its index to append in string.
Syntax:
result_var=separator.join([ str(element) for i,element in enumerate(list)])
Here,
- The separator is a string that separates the elements of the list in the resulting string.
- The join() function is a string method that converts the list into a string.
- Str() is a function that converts the non-string value into a string value.
- Enumerate() is a function used to iterate over a sequence.
- result_var is a variable that stores the resulting value.
Now, let’s look at an example that shows how we can convert a Python list to string using the enumeration function.
Code Example:
Output:
0: Enumerate 1: with 2: care
Explanation:
In the Python example-
- We begin by defining a list named my_list with elements "Enumerate", "with", and "care".
- Then, we use the enumerate() function within a list comprehension to iterate over each element in my_list while also tracking the index of each element. The enumerate() function returns a tuple containing the index and the corresponding element.
- Within the list comprehension, an f-string is used to format each element along with its index. This creates strings in the format- index: element.
- The join() method is then called on the resulting list from the list comprehension. It concatenates each formatted string with a space character (' ') between them.
- The resulting string is stored in the variable result.
- Finally, the content of the result variable is printed using the print() function.
Convert Python List To String Using Operator Module
Python's operator module provides a concise way to concatenate elements of a list into a string. In the operator module, we have a function named methodcaller(), which is similar to the map() function, which takes the function and applies it to the element that it passes to.
Syntax:
result_var=separator. join(operator.methodcaller('__str__')element)
Here,
- The separator is a delimiter (here, a string) that separates the elements of the list in the resulting string.
- The join() function is a string method that converts the list into a string.
- Operator.methodcaller() is a function in the operator module that calls the method inside the parameter of it.
- __Str__ is a function that converts the non-string value into a string value.
- Enumerate() is a function used to iterate over a sequence.
- result_var is a variable that stores the resulting value.
Code Example:
Output:
Operator method magic!
Explanation:
In the above code,
- We begin by importing the operator module, which provides functions that implement the basic operations of the Python language.
- Next, we create a list named my_list with elements "Operator", "method", and "magic".
- Then, we use the operator.methodcaller('__str__') function within a generator expression. This function returns a callable object that calls the __str__ method on its operand.
- For each element in the my_list, the generator expression applies the callable object returned by operator.methodcaller('__str__') to convert each element into its string representation using the __str__ method.
- The join() method is then called on the resulting generator expression. It concatenates each string representation of the elements with a space character (' ') in between them.
- The resulting string is stored in the variable result and is printed to the console using the print() function.
Python Program To Convert String To List
As mentioned before, Python strings are immutable, i.e., they cannot be changed after creation. However, lists offer mutability, allowing for dynamic alterations to the data. When we need to modify or manipulate individual elements of a sequence, converting a string to a list becomes imperative.
Imagine dealing with a list of lists or names, and you want to sort them alphabetically or filter them based on specific criteria. Converting the string representation to a list grants you the flexibility to perform such operations effortlessly.
Converting Python String To List Using Split() Function
The split() function is a versatile tool for breaking strings into lists based on a specified delimiter.
- The parameters of the split function are separator and maxsplit.
- A separator may be a character or word that was present in the string, which we use to break the string into a list. By default, it will be a single space.
- Maxsplit is the maximum number of splits we need to do on the string. By default, it will be -1, which means no limit.
Syntax:
string.split(separator,maxsplit=-1)
Here,
- The separator is a string delimiter that separates the elements of the list in the resulting string. If the separator is not specified, then an empty space is used by default.
- Split() is a string function that splits the given string into a list based on the separator.
- Maxsplit is the maximum no.of splits to be performed on the string. By default, its value is -1.
Code Example:
Output:
['apple', 'orange', 'banana']
Explanation:
In the code snippet-
- We create a string variable named string_data with the value "apple,orange,banana".
- Then, we call the split() method on the string_data variable with a comma as the argument.
- This method splits the string into substrings whenever it encounters the specified separator, i.e., the comma, and returns a list of these substrings.
- The resulting list, containing substrings "apple", "orange", and "banana", is stored in the variable fruit_list.
- Finally, the content of the fruit_list variable is printed using the print() function.
Conclusion
Lists are a powerful concept in Python that can help store a wide range of values. In addition, they are changeable, organized, and easy to access. They serve critical roles in data organization, modification, and the generation of various data structures. String represents a list of characters and is widely used in text-processing tasks. However, once declared, strings are immutable in Python. Both the data types/ structures are integral to programming. It is, hence, important to understand how to manipulate them along with how to convert one to another and vice versa.
In this article, we have discussed multiple ways to convert a Python list to string. They include built-in methods like join(), map(), str(), format(), and other methods like recursive functions, operator modules, and more. There are various reasons why you might need to convert a Python list to a string, including encoding, printing, data persistence, data manipulation, interoperability, and customization. Choose any of the methods discussed depending upon your specific requirements.
Frequently Asked Questions
Q. How do we convert a list to a string in Python with commas?
To convert a Python list to string with commas, we can use the in-built string method join(). It takes an iterable object as a parameter, such as a list or a tuple, and returns the single string by concatenating every element of the iterable object. It also needs a specified separator to separate every element. For example, if we want a string with commas, we must pass the (,) as a separator before the join() function.
Code Example:
Output:
apple,banana,orange
Explanation:
- We first create a list named my_list that contains three items: 'apple', 'banana', and 'orange'.
- Then, we use the join() method to join the elements of my_list with a comma as a separator.
- The outcome is stored in the result_string variable, and the same is printed to the console using print().
Q. How do we print a string list in Python?
We can use the iterative approach to print a string list in Python. For that, we use a for loop to iterate over the elements in an iterable object and print them. Here, a string list is an iterable object which we can traverse using for loop.
Code Example:
Output:
Unstop
has
Mentors
Explanation:
We declare a list called data with 3 string elements, i.e., it is a string list that we traverse using a for a loop. The loop contains a print() statement which displays the respective element.
Q. How to convert a string to list string?
In Python, we can use the split() function to turn a string into a list of strings. This function divides a text into a list using a provided delimiter. If no delimiter is supplied, the string is divided based on whitespace by default.
Code Example:
Output:
['Good', 'Morning', 'Unstop']
Explanation:
We create a string variable called my_string and pass it to the split() function. The function converts the string to list of strings and stores it in the my_list variable. We print this list to the console using the print() function.
Q . What if our list contains a mix of sequence data types? Can we convert it to string?
Even if our list contains a mix of string data types, we can convert the list elements into a string type by converting every element of the list into a string and then joining them. For this, we can use map() and str() functions to first convert every element into a string. And then the map () function that applies a single function on the iterable object values. Str() function is a type cast function that converts the element into string type.
Code Example:
Output:
Is 3.14 the value of Pi ? True
Explanation:
- We declare the data variable with a list of values and use the map() function with parameters str() and data.
- The map() function applies the str() function on every element in the data list.
- Then, we use the join() method to join the list of all string-type values and store the result in the string variable.
- Lastly, we print output to the console.
Q. Can we customize the format when converting a list to a string?
Yes, we can customize the format by modifying the separator or including additional information. To customize the formatting, we use the f-string notation, which is one type of formatted string we use with the variable names in the curly bracket placeholders {}.In the string, the placeholder variable is replaced with its value.
Code Example:
Output:
Element: item1, Element: item2, Element: item3
Explanation:
In this example code in Python language, we first declare the data variable with a list of values. Then, we use f-string notation in list-comprehension to change the string into custom format. This is passed to the join() method for concatenation and its outcome is stored in the custom_string variable. Finally, we use print() to display the output.
Q. Can we convert a list of numbers to a string without spaces between them?
Yes, we can convert a list of numbers to a string without spaces in Python. For this, we need to use the join() method without any string separator.
- Since the numbers list is essentially a list of integers, all the elements are integer data type values, we first need to convert them into strings.
- For that, we use the map() function to apply the str type conversion on every element of the numbers list.
- Then, we simply use the join() method on the empty string to join all the elements of the list, which we have already converted.
Code Example:
Output:
12345
Q. What if our list contains special characters or non-string elements?
We can still convert a list with special characters or non-string elements to a string in Python. For this, we cannot use the join() method directly, which is a string method that only takes string values. So if we provide values other than string, it will pop the Type Error. However, we can typecast the non-string element to string, and then we pass it to the join() method.
- For this, we can convert the non-string elements to string literals using the map() with str() function.
- The map() function applies the function that is provided to it on all iterables that it passes to it. and the str() function is a type conversion function to convert strings.
- Once we have converted all the non-string items to string elements, we can use the join method to convert the list to a single string.
Code Example:
Output:
@#$123True
Explanation:
Initially, we declare the special_chars_list variable with elements that are mix type values. Then we use the map() function with parameters of str and list. Next, we use the join() method to convert the list of elements to strings and print its outcome in string_result to the console. This is how we can convert a Python list to string where the elements contain special characters.
Here are a few other insightful pieces you will love reading:
- Relational Operators In Python | 6 Types, Usage, Examples & More!
- 12 Ways To Compare Strings In Python Explained (With Examples)
- Python Logical Operators, Short-Circuiting & More (With Examples)
- Python Bitwise Operators | Positive & Negative Numbers (+Examples)
- How To Reverse A String In Python? 10 Easy Ways With Examples!
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment