Table of content:
- What Is Python? An Introduction
- What Is The History Of Python?
- Key Features Of The Python Programming Language
- Who Uses Python?
- Basic Characteristics Of Python Programming Syntax
- Why Should You Learn Python?
- Applications Of Python Language
- Advantages And Disadvantages Of Python
- Some Useful Python Tips & Tricks For Efficient Programming
- Python 2 Vs. Python 3: Which Should You Learn?
- Python Libraries
- Conclusion
- Frequently Asked Questions
- It's Python Basics Quiz Time!
Table of content:
- Python At A Glance
- Key Features of Python Programming
- Applications of Python
- Bonus: Interesting features of different programming languages
- Summing up...
- FAQs regarding Python
- Take A Quiz To Rehash Python's Features!
Table of content:
- What Is Python IDLE?
- What Is Python Shell & Its Uses?
- Primary Features Of Python IDLE
- How To Use Python IDLE Shell? Setting Up Your Python Environment
- How To Work With Files In Python IDLE?
- How To Execute A File In Python IDLE?
- Improving Workflow In Python IDLE Software
- Debugging In Python IDLE
- Customizing Python IDLE
- Code Examples
- Conclusion
- Frequently Asked Questions (FAQs)
- How Well Do You Know IDLE? Take A Quiz!
Table of content:
- What Is A Variable In Python?
- Creating And Declaring Python Variables
- Rules For Naming Python Variables
- How To Print Python Variables?
- How To Delete A Python Variable?
- Various Methods Of Variables Assignment In Python
- Python Variable Types
- Python Variable Scope
- Concatenating Python Variables
- Object Identity & Object References Of Python Variables
- Reserved Words/ Keywords & Python Variable Names
- Conclusion
- Frequently Asked Questions
- Rehash Python Variables Basics With A Quiz!
Table of content:
- What Is A String In Python?
- Creating String In Python
- How To Create Multiline Python Strings?
- Reassigning Python Strings
- Accessing Characters Of Python Strings
- How To Update Or Delete A Python String?
- Reversing A Python String
- Formatting Python Strings
- Concatenation & Comparison Of Python Strings
- Python String Operators
- Python String Functions
- Escape Sequences In Python Strings
- Conclusion
- Frequently Asked Questions
- Rehash Python Strings Basics With A Quiz!
Table of content:
- What Is Python Namespace?
- Lifetime Of Python Namespace
- Types Of Python Namespace
- The Built-In Namespace In Python
- The Global Namespace In Python
- The Local Namespace In Python
- The Enclosing Namespace In Python
- Variable Scope & Namespace In Python
- Python Namespace Dictionaries
- Changing Variables Out Of Their Scope & Python Namespace
- Best Practices Of Python Namespace
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Namespaces!
Table of content:
- What Are Logical Operators In Python?
- The AND Python Logical Operator
- The OR Python Logical Operator
- The NOT Python Logical Operator
- Short-Circuiting Evaluation Of Python Logical Operators
- Precedence of Logical Operators In Python
- How Does Python Calculate Truth Value?
- Final Note On How AND & OR Python Logical Operators Work
- Conclusion
- Frequently Asked Questions
- Python Logical Operators Quiz– Test Your Knowledge!
Table of content:
- What Are Bitwise Operators In Python?
- List Of Python Bitwise Operators
- AND Python Bitwise Operator
- OR Python Bitwise Operator
- NOT Python Bitwise Operator
- XOR Python Bitwise Operator
- Right Shift Python Bitwise Operator
- Left Shift Python Bitwise Operator
- Python Bitwise Operations And Negative Integers
- The Binary Number System
- Application of Python Bitwise Operators
- Python Bitwise Operator Overloading
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Bitwise Operators!
Table of content:
- What Is The Print() Function In Python?
- How Does The print() Function Work In Python?
- How To Print Single & Multi-line Strings In Python?
- How To Print Built-in Data Types In Python?
- Print() Function In Python For Values Stored In Variables
- Print() Function In Python With sep Parameter
- Print() Function In Python With end Parameter
- Print() Function In Python With flush Parameter
- Print() Function In Python With file Parameter
- How To Remove Newline From print() Function In Python?
- Use Cases Of The print() Function In Python
- Understanding Print Statement In Python 2 Vs. Python 3
- Conclusion
- Frequently Asked Questions
- Know The print() Function In Python? Take A Quiz!
Table of content:
- Working Of Normal Print() Function
- The New Line Character In Python
- How To Print Without Newline In Python | Using The End Parameter
- How To Print Without Newline In Python 2.x? | Using Comma Operator
- How To Print Without Newline In Python 3.x?
- How To Print Without Newline In Python With Module Sys
- The Star Pattern(*) | How To Print Without Newline & Space In Python
- How To Print A List Without Newline In Python?
- How To Remove New Lines In Python?
- Conclusion
- Frequently Asked Questions
- Think You Can Print Without a Newline in Python? Prove It!
Table of content:
- What Is A Python For Loop?
- How Does Python For Loop Work?
- When & Why To Use Python For Loops?
- Python For Loop Examples
- What Is Rrange() Function In Python?
- Nested For Loops In Python
- Python For Loop With Continue & Break Statements
- Python For Loop With Pass Statement
- Else Statement In Python For Loop
- Conclusion
- Frequently Asked Questions
- Think You Know Python's For Loop? Prove It!
Table of content:
- What Is Python While Loop?
- How Does The Python While Loop Work?
- How To Use Python While Loops For Iterations?
- Control Statements In Python While Loop With Examples
- Python While Loop With Python List
- Infinite Python While Loop in Python
- Python While Loop Multiple Conditions
- Nested Python While Loops
- Conclusion
- Frequently Asked Questions
- Mastered Python While Loop? Let’s Find Out!
Table of content:
- What Are Conditional If-Else Statements In Python?
- Types Of If-Else Statements In Python
- If Statement In Python
- If-Else Statement In Python
- Nested If-Else Statement In Python
- Elif Statement In Python
- Ladder If-Elif-Else Statement In Python
- Short Hand If-Statement In Python
- Short Hand If-Else Statement In Python
- Operators & If-Esle Statement In Python
- Other Statements With If-Else In Python
- Conclusion
- Frequently Asked Questions
- Quick If-Else Statement Quiz– Let’s Go!
Table of content:
- What Is Control Structure In Python?
- Types Of Control Structures In Python
- Sequential Control Structures In Python
- Decision-Making Control Structures In Python
- Repetition Control Structures In Python
- Benefits Of Using Control Structures In Python
- Conclusion
- Frequently Asked Questions
- Control Structures in Python – Are You the Master? Take A Quiz!
Table of content:
- What Are Python Libraries?
- How Do Python Libraries Work?
- Standard Python Libraries (With List)
- Important Python Libraries For Data Science
- Important Python Libraries For Machine & Deep Learning
- Other Important Python Libraries You Must Know
- Working With Third-Party Python Libraries
- Troubleshooting Common Issues For Python Libraries
- Python Libraries In Larger Projects
- Importance Of Python Libraries
- Conclusion
- Frequently Asked Questions
- Quick Quiz On Python Libraries – Let’s Go!
Table of content:
- What Are Python Functions?
- How To Create/ Define Functions In Python?
- How To Call A Python Function?
- Types Of Python Functions Based On Parameters & Return Statement
- Rules & Best Practices For Naming Python Functions
- Basic Types of Python Functions
- The Return Statement In Python Functions
- Types Of Arguments In Python Functions
- Docstring In Python Functions
- Passing Parameters In Python Functions
- Python Function Variables | Scope & Lifetime
- Advantages Of Using Python Functions
- Recursive Python Function
- Anonymous/ Lambda Function In Python
- Nested Functions In Python
- Conclusion
- Frequently Asked Questions
- Python Functions – Test Your Knowledge With A Quiz!
Table of content:
- What Are Python Built-In Functions?
- Mathematical Python Built-In Functions
- Python Built-In Functions For Strings
- Input/ Output Built-In Functions In Python
- List & Tuple Python Built-In Functions
- File Handling Python Built-In Functions
- Python Built-In Functions For Dictionary
- Type Conversion Python Built-In Functions
- Basic Python Built-In Functions
- List Of Python Built-In Functions (Alphabetical)
- Conclusion
- Frequently Asked Questions
- Think You Know Python Built-in Functions? Prove It!
Table of content:
- What Is A round() Function In Python?
- How Does Python round() Function Work?
- Python round() Function If The Second Parameter Is Missing
- Python round() Function If The Second Parameter Is Present
- Python round() Function With Negative Integers
- Python round() Function With Math Library
- Python round() Function With Numpy Module
- Round Up And Round Down Numbers In Python
- Truncation Vs Rounding In Python
- Practical Applications Of Python round() Function
- Conclusion
- Frequently Asked Questions
- Revisit Python’s round() Function – Take The Quiz!
Table of content:
- What Is Python pow() Function?
- Python pow() Function Example
- Python pow() Function With Modulus (Three Parameters)
- Python pow() Function With Complex Numbers
- Python pow() Function With Floating-Point Arguments And Modulus
- Python pow() Function Implementation Cases
- Difference Between Inbuilt-pow() And math.pow() Function
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python’s pow() Function!
Table of content:
- Python max() Function With Objects
- Examples Of Python max() Function With Objects
- Python max() Function With Iterable
- Examples Of Python max() Function With Iterables
- Potential Errors With The Python max() Function
- Python max() Function Vs. Python min() Functions
- Conclusion
- Frequently Asked Questions
- Think You Know Python max() Function? Take A Quiz!
Table of content:
- What Are Strings In Python?
- What Are Python String Methods?
- List Of Python String Methods For Manipulating Case
- List Of Python String Methods For Searching & Finding
- List Of Python String Methods For Modifying & Transforming
- List Of Python String Methods For Checking Conditions
- List Of Python String Methods For Encoding & Decoding
- List Of Python String Methods For Stripping & Trimming
- List Of Python String Methods For Formatting
- Miscellaneous Python String Methods
- List Of Other Python String Operations
- Conclusion
- Frequently Asked Questions
- Mastered Python String Methods? Take A Quiz!
Table of content:
- What Is Python String?
- The Need For Python String Replacement
- The Python String replace() Method
- Multiple Replacements With Python String.replace() Method
- Replace A Character In String Using For Loop In Python
- Python String Replacement Using Slicing Method
- Replace A Character At a Given Position In Python String
- Replace Multiple Substrings With The Same String In Python
- Python String Replacement Using Regex Pattern
- Python String Replacement Using List Comprehension & Join() Method
- Python String Replacement Using Callback With re.sub() Method
- Python String Replacement With re.subn() Method
- Conclusion
- Frequently Asked Questions
- Know How To Replace Python Strings? Prove It!
Table of content:
- What Is String Slicing In Python?
- How Indexing & String Slicing Works In Python
- Extracting All Characters Using String Slicing In Python
- Extracting Characters Before & After Specific Position Using String Slicing In Python
- Extracting Characters Between Two Intervals Using String Slicing In Python
- Extracting Characters At Specific Intervals (Step) Using String Slicing In Python
- Negative Indexing & String Slicing In Python
- Handling Out-of-Bounds Indices In String Slicing In Python
- The slice() Method For String Slicing In Python
- Common Pitfalls Of String Slicing In Python
- Real-World Applications Of String Slicing
- Conclusion
- Frequently Asked Questions
- Quick Python String Slicing Quiz– Let’s Go!
Table of content:
- Introduction To Python List
- How To Create A Python List?
- How To Access Elements Of Python List?
- Accessing Multiple Elements From A Python List (Slicing)
- Access List Elements From Nested Python Lists
- How To Change Elements In Python Lists?
- How To Add Elements To Python Lists?
- Delete/ Remove Elements From Python Lists
- How To Create Copies Of Python Lists?
- Repeating Python Lists
- Ways To Iterate Over Python Lists
- How To Reverse A Python List?
- How To Sort Items Of Python Lists?
- Built-in Functions For Operations On Python Lists
- Conclusion
- Frequently Asked Questions
- Revisit Python Lists Basics With A Quick Quiz!
Table of content:
- What Is List Comprehension In Python?
- Incorporating Conditional Statements With List Comprehension In Python
- List Comprehension In Python With range()
- Filtering Lists Effectively With List Comprehension In Python
- Nested Loops With List Comprehension In Python
- Flattening Nested Lists With List Comprehension In Python
- Handling Exceptions In List Comprehension In Python
- Common Use Cases For List Comprehensions
- Advantages & Disadvantages Of List Comprehension In Python
- Best Practices For Using List Comprehension In Python
- Performance Considerations For List Comprehension In Python
- For Loops & List Comprehension In Python: A Comparison
- Difference Between Generator Expression & List Comprehension In Python
- Conclusion
- Frequently Asked Questions
- Rehash Python List Comprehension Basics With A Quiz!
Table of content:
- What Is A List In Python?
- How To Find Length Of List In Python?
- For Loop To Get Python List Length (Naive Approach)
- The len() Function To Get Length Of List In Python
- The length_hint() Function To Find Length Of List In Python
- The sum() Function To Find The Length Of List In Python
- The enumerate() Function To Find Python List Length
- The Counter Class From collections To Find Python List Length
- The List Comprehension To Find Python List Length
- Find The Length Of List In Python Using Recursion
- Comparison Between Ways To Find Python List Length
- Conclusion
- Frequently Asked Questions
- Know How To Get Python List Length? Prove it!
Table of content:
- List of Methods To Reverse A Python List
- Python Reverse List Using reverse() Method
- Python Reverse List Using the Slice Operator ([::-1])
- Python Reverse List By Swapping Elements
- Python Reverse List Using The reversed() Function
- Python Reverse List Using A for Loop
- Python Reverse List Using While Loop
- Python Reverse List Using List Comprehension
- Python Reverse List Using List Indexing
- Python Reverse List Using The range() Function
- Python Reverse List Using NumPy
- Comparison Of Ways To Reverse A Python List
- Conclusion
- Frequently Asked Questions
- Time To Test Your Python List Reversal Skills!
Table of content:
- What Is Indexing In Python?
- The Python List index() Function
- How To Use Python List index() To Find Index Of A List Element
- The Python List index() Method With Single Parameter (Start)
- The Python List index() Method With Start & Stop Parameters
- What Happens When We Use Python List index() For An Element That Doesn't Exist
- Python List index() With Nested Lists
- Fixing IndexError Using The Python List index() Method
- Python List index() With Enumerate()
- Real-world Examples Of Python List index() Method
- Difference Between find() And index() Method In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python List Indexing? Take A Quiz!
Table of content:
- How To Remove Elements From List In Python?
- The remove() Method To Remove Element From Python List
- The pop() Method To Remove Element From List In Python
- The del Keyword To Remove Element From List In Python
- The clear() Method To Remove Elements From Python List
- List Comprehensions To Conditionally Remove Element From List In Python
- Key Considerations For Removing Elements From Python Lists
- Why We Need to Remove Elements From Python List
- Performance Comparison Of Methods To Remove Element From List In Python
- Conclusion
- Frequently Asked Questions
- Quiz– Prove You Know How To Remove Item From Python Lists!
Table of content:
- How To Remove Duplicates From A List In Python?
- The set() Function To Remove Duplicates From Python List
- Remove Duplicates From Python List Using For Loop
- Using List Comprehension Remove Duplicates From Python List
- Remove Duplicates From Python List Using enumerate() With List Comprehension
- Dictionary & fromkeys() Method To Remove Duplicates From Python List
- Remove Duplicates From Python List Using in, not in Operators
- Remove Duplicates From Python List Using collections.OrderedDict.fromkeys()
- Remove Duplicates From Python List Using Counter with freq.dist() Method
- The del Keyword Remove Duplicates From Python List
- Remove Duplicates From Python List Using DataFrame
- Remove Duplicates From Python List Using pd.unique and np.unipue
- Remove Duplicates From Python List Using reduce() function
- Comparative Analysis Of Ways To Remove Duplicates From Python List
- Conclusion
- Frequently Asked Questions
- Think You Know How to Remove Duplicates? Take A Quiz!
Table of content:
- What Is Python List & How To Access Elements?
- What Is IndexError: List Index Out Of Range & Its Causes In Python?
- Understanding Indexing Behavior In Python Lists
- How to Prevent/ Fix IndexError: List Index Out Of Range In Python
- Handling IndexError Gracefully Using Try-Except
- Debugging Tips For IndexError: List Index Out Of Range Python
- Conclusion
- Frequently Asked Questions
- Avoiding ‘List Index Out of Range’ Errors? Take A Quiz!
Table of content:
- What Is the Python sort() List Method?
- Sorting In Ascending Order Using The Python sort() List Method
- How To Sort Items In Descending Order Using Python sort() List Method
- Custom Sorting Using The Key Parameter Of Python sort() List Method
- Examples Of Python sort() List Method
- What Is The sorted() List Method In Python
- Differences Between sorted() And sort() List Methods In Python
- When To Use sorted() & When To Use sort() List Method In Python
- Conclusion
- Frequently Asked Questions
- Take A Quick Python's sort() Quiz!
Table of content:
- What Is A List In Python?
- What Is A String In Python?
- Why Convert Python List To String?
- How To Convert List To String In Python?
- The join() Method To Convert Python List To String
- Convert Python List To String Through Iteration
- Convert Python List To String With List Comprehension
- The map() Function To Convert Python List To String
- Convert Python List to String Using format() Function
- Convert Python List To String Using Recursion
- Enumeration Function To Convert Python List To String
- Convert Python List To String Using Operator Module
- Python Program To Convert String To List
- Conclusion
- Frequently Asked Questions
- Convert Lists To Strings Like A Pro! Take A Quiz
Table of content:
- What Is Inheritance In Python?
- Python Inheritance Syntax
- Parent Class In Python Inheritance
- Child Class In Python Inheritance
- The __init__() Method In Python Inheritance
- The super() Function In Python Inheritance
- Method Overriding In Python Inheritance
- Types Of Inheritance In Python
- Special Functions In Python Inheritance
- Advantages & Disadvantages Of Inheritance In Python
- Common Use Cases For Inheritance In Python
- Best Practices for Implementing Inheritance in Python
- Avoiding Common Pitfalls in Python Inheritance
- Conclusion
- Frequently Asked Questions
- 💡 Python Inheritance Quiz – Are You Ready?
Table of content:
- What Is The Python List append() Method?
- Adding Elements To A Python List Using append()
- Populate A Python List Using append()
- Adding Different Data Types To Python List Using append()
- Adding A List To Python List Using append()
- Nested Lists With Python List append() Method
- Practical Use Cases Of Python List append() Method
- How append() Method Affects List Performance
- Avoiding Common Mistakes When Using Python List append()
- Comparing extend() With append() Python List Method
- Conclusion
- Frequently Asked Questions
- 🧠 Think You Know Python List append()? Take A Quiz!
Table of content:
- What Is A Linked List In Python?
- Types Of Linked Lists In Python
- How To Create A Linked List In Python
- How To Traverse A Linked List In Python & Retrieve Elements
- Inserting Elements In A Linked List In Python
- Deleting Elements From A Linked List In Python
- Update A Node Of Linked List In Python
- Reversing A Linked List In Python
- Calculating Length Of A Linked List In Python
- Comparing Arrays And Linked Lists In Python
- Advantages & Disadvantages Of Linked List In Python
- When To Use Linked Lists Over Other Data Structures
- Practical Applications Of Linked Lists In Python
- Conclusion
- Frequently Asked Questions
- 🔗 Linked List Logic: Can You Ace This Quiz?
Table of content:
- What Is Extend In Python?
- Extend In Python With List
- Extend In Python With String
- Extend In Python With Tuple
- Extend In Python With Set
- Extend In Python With Dictionary
- Other Methods To Extend A List In Python
- Difference Between append() and extend() In Python
- Conclusion
- Frequently Asked Questions
- Think You Know extend() In Python? Prove It!
Table of content:
- What Is Recursion In Python?
- Key Components Of Recursive Functions In Python
- Implementing Recursion In Python
- Recursion Vs. Iteration In Python
- Tail Recursion In Python
- Infinite Recursion In Python
- Advantages Of Recursion In Python
- Disadvantages Of Recursion In Python
- Best Practices For Using Recursion In Python
- Conclusion
- Frequently Asked Questions
- Recursive Thinking In Python: Test Your Skills!
Table of content:
- What Is Type Conversion In Python?
- Types Of Type Conversion In Python
- Implicit Type Conversion In Python
- Explicit Type Conversion In Python
- Functions Used For Explicit Data Type Conversion In Python
- Important Type Conversion Tips In Python
- Benefits Of Type Conversion In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Type Conversion? Take A Quiz!
Table of content:
- What Is Scope In Python?
- Local Scope In Python
- Global Scope In Python
- Nonlocal (Enclosing) Scope In Python
- Built-In Scope In Python
- The LEGB Rule For Python Scope
- Python Scope And Variable Lifetime
- Best Practices For Managing Python Scope
- Conclusion
- Frequently Asked Questions
- Think You Know Python Scope? Test Yourself!
Table of content:
- Understanding The Continue Statement In Python
- How Does Continue Statement Work In Python?
- Python Continue Statement With For Loops
- Python Continue Statement With While Loops
- Python Continue Statement With Nested Loops
- Python Continue With If-Else Statement
- Difference Between Pass and Continue Statement In Python
- Practical Applications Of Continue Statement In Python
- Conclusion
- Frequently Asked Questions
- Python 'continue' Statement Quiz: Can You Ace It?
Table of content:
- What Are Control Statements In Python?
- Types Of Control Statements In Python
- Conditional Control Statements In Python
- Loop Control Statements In Python
- Control Flow Altering Statements In Python
- Exception Handling Control Statements In Python
- Conclusion
- Frequently Asked Questions
- Mastering Control Statements In Python – Take the Quiz!
Table of content:
- Difference Between Mutable And Immutable Data Types in Python
- What Is Mutable Data Type In Python?
- Types Of Mutable Data Types In Python
- What Are Immutable Data Types In Python?
- Types Of Immutable Data Types In Python
- Key Similarities Between Mutable And Immutable Data Types In Python
- When To Use Mutable Vs Immutable In Python?
- Conclusion
- Frequently Asked Questions
- Quiz Time: Mutable vs. Immutable In Python!
Table of content:
- What Is A List?
- What Is A Tuple?
- Difference Between List And Tuple In Python (Comparison Table)
- Syntax Difference Between List And Tuple In Python
- Mutability Difference Between List And Tuple In Python
- Other Difference Between List And Tuple In Python
- List Vs. Tuple In Python | Methods
- When To Use Tuples Over Lists?
- Key Similarities Between Tuples And Lists In Python
- Conclusion
- Frequently Asked Questions
- 🧐 Lists vs. Tuples Quiz: Test Your Python Knowledge!
Table of content:
- Introduction to Python
- Downloading & Installing Python, IDLE, Tkinter, NumPy & PyGame
- Creating A New Python Project
- How To Write Python Hello World Program In Python?
- Way To Write The Hello, World! Program In Python
- The Hello, World! Program In Python Using Class
- The Hello, World! Program In Python Using Function
- Print Hello World 5 Times Using A For Loop
- Conclusion
- Frequently Asked Questions
- 👋 Python's 'Hello, World!'—How Well Do You Know It?
Table of content:
- Algorithm Of Python Program To Add To Numbers
- Standard Program To Add Two Numbers In Python
- Python Program To Add Two Numbers With User-defined Input
- The add() Method In Python Program To Add Two Numbers
- Python Program To Add Two Numbers Using Lambda
- Python Program To Add Two Numbers Using Function
- Python Program To Add Two Numbers Using Recursion
- Python Program To Add Two Numbers Using Class
- How To Add Multiple Numbers In Python?
- Add Multiple Numbers In Python With User Input
- Time Complexities Of Python Programs To Add Two Numbers
- Conclusion
- Frequently Asked Questions
- 💡 Quiz Time: Python Addition Basics!
Table of content:
- Swapping in Python
- Swapping Two Variables Using A Temporary Variable
- Swapping Two Variables Using The Comma Operator In Python
- Swapping Two Variables Using The Arithmetic Operators (+,-)
- Swapping Two Variables Using The Arithmetic Operators (*,/)
- Swapping Two Variables Using The XOR(^) Operator
- Swapping Two Variables Using Bitwise Addition and Subtraction
- Swap Variables In A List
- Conclusion
- Frequently Asked Questions (FAQs)
- Quiz To Test Your Variable Swapping Knowledge
Table of content:
- What Is A Quadratic Equation? How To Solve It?
- How To Write A Python Program To Solve Quadratic Equations?
- Python Program To Solve Quadratic Equations Directly Using The Formula
- Python Program To Solve Quadratic Equations Using The Complex Math Module
- Python Program To Solve Quadratic Equations Using Functions
- Python Program To Solve Quadratic Equations & Find Number Of Solutions
- Python Program To Plot Quadratic Functions
- Conclusion
- Frequently Asked Questions
- Quadratic Equations In Python Quiz: Test Your Knowledge!
Table of content:
- What Is Decimal Number System?
- What Is Binary Number System?
- What Is Octal Number System?
- What Is Hexadecimal Number System?
- Python Program to Convert Decimal to Binary, Octal, And Hexadecimal Using Built-In Function
- Python Program To Convert Decimal To Binary Using Recursion
- Python Program To Convert Decimal To Octal Using Recursion
- Python Program To Convert Decimal To Hexadecimal Using Recursion
- Python Program To Convert Decimal To Binary Using While Loop
- Python Program To Convert Decimal To Octal Using While Loop
- Python Program To Convert Decimal To Hexadecimal Using While Loop
- Convert Decimal To Binary, Octal, And Hexadecimal Using String Formatting
- Python Program To Convert Binary, Octal, And Hexadecimal String To A Number
- Complexity Comparison Of Python Programs To Convert Decimal To Binary, Octal, And Hexadecimal
- Conclusion
- Frequently Asked Questions
- 💡 Decimal To Binary, Octal & Hex: Quiz Time!
Table of content:
- What Is A Square Root?
- Python Program To Find The Square Root Of A Number
- The pow() Function In Python Program To Find The Square Root Of Given Number
- Python Program To Find Square Root Using The sqrt() Function
- The cmath Module & Python Program To Find The Square Root Of A Number
- Python Program To Find Square Root Using The Exponent Operator (**)
- Python Program To Find Square Root With A User-Defined Function
- Python Program To Find Square Root Using A Class
- Python Program To Find Square Root Using Binary Search
- Python Program To Find Square Root Using NumPy Module
- Conclusion
- Frequently Asked Questions
- 🤓 Think You Know Square Roots In Python? Take A Quiz!
Table of content:
- Understanding the Logic Behind the Conversion of Kilometers to Miles
- Steps To Write Python Program To Convert Kilometers To Miles
- Python Program To Convert Kilometer To Miles Without Function
- Python Program To Convert Kilometer To Miles Using Function
- Python Program to Convert Kilometer To Miles Using Class
- Tips For Writing Python Program To Convert Kilometer To Miles
- Conclusion
- Frequently Asked Questions
- 🧐 Mastered Kilometer To Mile Conversion? Prove It!
Table of content:
- Why Build A Calculator Program In Python?
- Prerequisites To Writing A Calculator Program In Python
- Approach For Writing A Calculator Program In Python
- Simple Calculator Program In Python
- Calculator Program In Python Using Functions
- Creating GUI Calculator Program In Python Using Tkinter
- Conclusion
- Frequently Asked Questions
- 🧮 Calculator Program In Python Quiz!
Table of content:
- The Calendar Module In Python
- Prerequisites For Writing A Calendar Program In Python
- How To Write And Print A Calendar Program In Python
- Calendar Program In Python To Display A Month
- Calendar Program In Python To Display A Year
- Conclusion
- Frequently Asked Questions
- Calendar Program In Python – Quiz Time!
Table of content:
- What Is The Fibonacci Series?
- Pseudocode Code For Fibonacci Series Program In Python
- Generating Fibonacci Series In Python Using Naive Approach (While Loop)
- Fibonacci Series Program In Python Using The Direct Formula
- How To Generate Fibonacci Series In Python Using Recursion?
- Generating Fibonacci Series In Python With Dynamic Programming
- Fibonacci Series Program In Python Using For Loop
- Generating Fibonacci Series In Python Using If-Else Statement
- Generating Fibonacci Series In Python Using Arrays
- Generating Fibonacci Series In Python Using Cache
- Generating Fibonacci Series In Python Using Backtracking
- Fibonacci Series In Python Using Power Of Matix
- Complexity Analysis For Fibonacci Series Programs In Python
- Applications Of Fibonacci Series In Python & Programming
- Conclusion
- Frequently Asked Questions
- 🤔 Think You Know Fibonacci Series? Take A Quiz!
Table of content:
- Different Ways To Write Random Number Generator Python Programs
- Random Module To Write Random Number Generator Python Programs
- The Numpy Module To Write Random Number Generator Python Programs
- The Secrets Module To Write Random Number Generator Python Programs
- Understanding Randomness and Pseudo-Randomness In Python
- Common Issues and Solutions in Random Number Generation
- Applications of Random Number Generator Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python's Random Module? Prove It!
Table of content:
- What Is A Factorial?
- Algorithm Of Program To Find Factorial Of A Number In Python
- Pseudocode For Factorial Program in Python
- Factorial Program In Python Using For Loop
- Factorial Program In Python Using Recursion
- Factorial Program In Python Using While Loop
- Factorial Program In Python Using If-Else Statement
- The math Module | Factorial Program In Python Using Built-In Factorial() Function
- Python Program to Find Factorial of a Number Using Ternary Operator(One Line Solution)
- Python Program For Factorial Using Prime Factorization Method
- NumPy Module | Factorial Program In Python Using numpy.prod() Function
- Complexity Analysis Of Factorial Programs In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Factorials In Python? Take A Quiz!
Table of content:
- What Is Palindrome In Python?
- Check Palindrome In Python Using While Loop (Iterative Approach)
- Check Palindrome In Python Using For Loop And Character Matching
- Check Palindrome In Python Using The Reverse And Compare Method (Python Slicing)
- Check Palindrome In Python Using The In-built reversed() And join() Methods
- Check Palindrome In Python Using Recursion Method
- Check Palindrome In Python Using Flag
- Check Palindrome In Python Using One Extra Variable
- Check Palindrome In Python By Building Reverse, One Character At A Time
- Complexity Analysis For Palindrome Programs In Python
- Real-World Applications Of Palindrome In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Palindromes? Take A Quiz!
Table of content:
- Best Python Books For Beginners
- Best Python Books For Intermediate Level
- Best Python Books For Experts
- Best Python Books To Learn Algorithms
- Audiobooks of Python
- Best Books To Learn Python And Code Like A Pro
- To Learn Python Libraries
- Books To Provide Extra Edge In Python
- Python Project Ideas - Reference
- Quiz To Rehash Your Knowledge Of Python Books!
Fix Indexerror (List Index Out Of Range) In Python +Code Examples
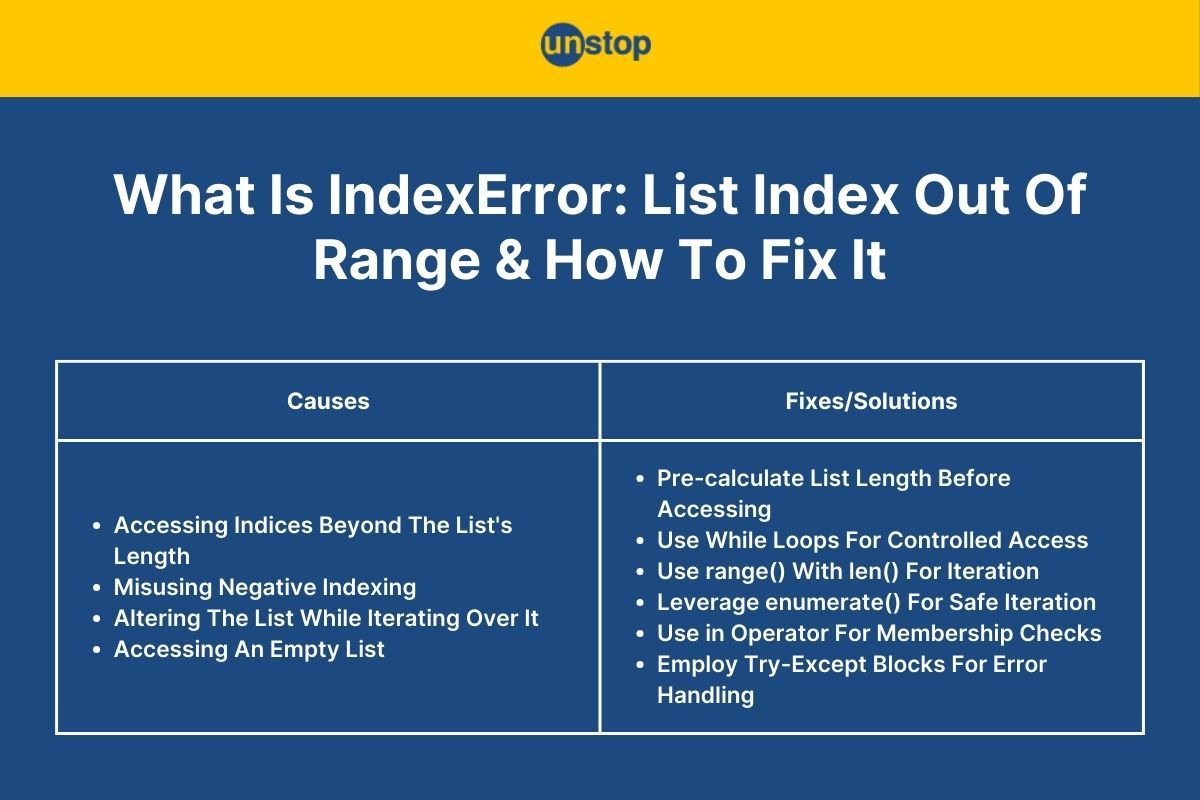
A Python list is a versatile data structure that allows you to store and manipulate a collection of elements, such as numbers, strings, or other objects, in an ordered manner. While working with lists, you might encounter a common error known as IndexError: list index out of range. This error occurs when you try to access an index that doesn't exist within the bounds of the list.
In this article, we'll explore the causes of this error, understand Python language’s indexing behavior, and learn how to prevent and handle it gracefully.
What Is Python List & How To Access Elements?
A Python list is essentially a container that holds a sequence of items, making it easy to manage and access elements through their indices. Indices start from 0 for the first element and can also be negative to count from the end of the list.
Below is a simple Python program example demonstrating the creation of lists and how to access elements using their index value.
Code Example:
# List creation
Unstop = ["upskill", "learn", "practice", "get hired"]
# Accessing elements
print(Unstop[0])Â # Output: upskill
print(Unstop[-1])Â # Output: get hired
In the example, we first create a list, Unstop, with four string elements separated by commas.
- Then, we use the index values to access the elements and print their values using the print() function.
- Here, Unstop[0] provides access to the first element, "upskill", and Unstop[-1] provides access to the last element, "get hired".
This straightforward way of accessing list elements lays the foundation for understanding how indices work, which is key to avoiding errors like IndexError: list index out of range.
Also read: Python List | Everything You Need To Know (With Detailed Examples)
What Is IndexError: List Index Out Of Range & Its Causes In Python?
The IndexError: list index out of range occurs when you attempt to access a position in a list that doesn’t exist. In Python language, lists have defined boundaries, and trying to access elements beyond these boundaries leads to this error.
In this section, we will discuss the common scenarios that trigger this error, along with illustrative examples.
1. Accessing Indices Beyond The List's Length
Every Python list has a fixed number of elements, and each element is assigned an index starting from 0. When you try to access an index greater than the last valid index (length of the list minus one), Python raises an IndexError.
This typically happens when there's an incorrect assumption about the list's size or when iterating beyond its range without proper bounds checking. The basic Python program example below illustrates this scenario.
Code Example:
# Creating the list
Unstop = ["upskill", "learn", "practice", "get hired"]
# Accessing an out-of-range index
print(Unstop[4]) # IndexError: list index out of range
Output:
Traceback (most recent call last):
File "/home/main.py", line 4, in <module>
print(Unstop[4]) # IndexError: list index out of range
IndexError: list index out of range
Explanation:
In this example, the list Unstop has 4 elements, with indices ranging from 0 to 3. Attempting to access the element at position 4 (i.e., 5th element) using Unstop[4] exceeds this range, triggering the IndexError.
2. Misusing Negative Indexing
Negative indexing allows you to access elements starting from the end of the list, with -1 referring to the last element. However, this flexibility comes with boundaries: the smallest valid negative index corresponds to the length of the list multiplied by -1.
Going beyond this range is equivalent to stepping out of the list’s bounds, leading to an IndexError. Look at the basic Python code example below to understand how this works.
Code Example:
# Creating list with four elements
Unstop = ["upskill", "learn", "practice", "get hired"]
# Misusing negative indexing
print(Unstop[-5])
Output:
Traceback (most recent call last):
File "/home/main.py", line 4, in <module>
print(Unstop[-5])
IndexError: list index out of range
Explanation:
In this example, we use the same list as before. The valid negative indices for this list range from -1 to -4. So, the negative index -5 exceeds the range, resulting in an error.
3. Altering The List While Iterating Over It
Iterating over a list while simultaneously modifying it (e.g., adding, removing, or changing elements) can disrupt the iteration process. This is because the list's structure changes during the loop, and indices that were initially valid may no longer exist. This mismatch between the iteration's expectation and the actual list state often results in an out-of-range error.
Code Example:
# List creation
Unstop = ["upskill", "learn", "practice", "get hired"]
# Altering the list during iteration
for i in range(len(Unstop)):
Unstop.pop(0) # Removing elements
print(Unstop[i])
Output:
get hired
print(Unstop[i]) # IndexError: list index out of range
IndexError: list index out of range
Explanation:
In the Python program example, we use a for loop to iterate through the list, remove the elements. This shortens the list but the iteration continues with the original length, causing indices to go out of range.
4. Accessing An Empty List
An empty list has no elements, so any attempt to access an index—positive or negative—will always be invalid. This often occurs when lists are unintentionally left empty due to programming logic errors or improper initialization, leading to an IndexError when access is attempted.
Code Example:
# Empty list
Unstop = []
# Accessing an index
print(Unstop[0]) # IndexError: list index out of range
Output:
print(Unstop[0]) # IndexError: list index out of range
IndexError: list index out of range
Explanation:
In the simple Python code example above, we create an empty list Unstop, i.e., it does not have any elements. So when we access the element at index 0, i.e., Unstop[0] inside the print function in Python, it generates an error since it is invalid.
These causes highlight the importance of understanding and managing indices carefully while working with lists.
Understanding Indexing Behavior In Python Lists
Python lists are indexed containers, meaning each element is assigned a unique position (index) within the list. These indices are crucial for accessing and manipulating list elements efficiently. Understanding how Python interprets these indices can help avoid errors like IndexError: list index out of range.
Positive vs. Negative Indexing
In Python, indices can be both positive and negative:
- Positive Indices: Start from 0 and move forward. For example, in a list Unstop = ["upskill", "learn", "practice", "get hired"], Unstop[0] corresponds to "upskill", while Unstop[3] corresponds to "get hired".
- Negative Indices: Start from -1 and move backward. Here, Unstop[-1] points to "get hired", and Unstop[-2] points to "practice".
This dual-indexing system offers flexibility, but improper use, such as going beyond the valid range of positive or negative indices, leads to errors.
Check out this amazing course to become the best version of the Python programmer you can be.
How to Prevent/ Fix IndexError: List Index Out Of Range In Python
Avoiding the IndexError requires careful handling of lists, ensuring you’re always accessing valid indices. In this section, we will discuss the practical strategies to prevent or fix this error, complete with code examples and explanations.
1. Pre-calculate List Length Before Accessing
Using len() to check the length of a list before attempting to access an index ensures that you’re always working within the valid range. This method is simple yet effective because it explicitly prevents accessing an index beyond the list’s boundaries.
By validating the index against the length of the list, you ensure that the program won’t attempt to access nonexistent elements, thereby preventing the error.
Code Example:
Unstop = ["upskill", "learn", "practice", "get hired"]
# Accessing an element only if the index is valid
index = 3
if index < len(Unstop):
print(Unstop[index]) # Output: get hired
else:
print("Index out of range")
Output:
get hired
Explanation:
In the Python code example, we begin with the list Unstop and then declare a variable index with value 3 (representing the maximum index limit). Then, we use an if-else statement to access the list elements.
- In the if condition, i.e., index < len(Unstop), we first use the built-in Python function len() to calculate the length of the list.
- Then, we use the less than relational operator to ensure the index (3) is within the list's length.
- If the condition is true, then the if-block executes, printing the element to the console.
- If the condition is false, the else block executes, printing a message– Index out of range.
- The if-else block thus prevents accessing invalid indices, eliminating the risk of an IndexError.
2. Use While Loops for Controlled Access
When iterating over a list with a while loop, you can control the condition under which the loop continues. By incorporating a check that compares the loop counter with the list’s length, you make sure the loop terminates before attempting to access an invalid index.
This method gives you more flexibility and control over the iteration process, reducing the risk of out-of-bounds access compared to traditional for loops. The example Python program below illustrates how this method works.
Code Example:
Unstop = ["upskill", "learn", "practice", "get hired"]
# Using while loop to iterate safely
i = 0
while i < len(Unstop):
print(Unstop[i], end=' ')
i += 1
Output:
upskill learn practice get hired
Explanation:
In the example, we use a while loop to continue only while i (index value) is less than the list's length, avoiding any out-of-bound access. Till the time the loop condition is met, the print() function inside prints the value to the console.
Note that here, we have used the end parameter space so that all the elements are printed in the same line separated by spaces.
3. Use range() With len() For Iteration
Combining range() with len() in a for loop is a foolproof way to iterate through a list without risking an out-of-range error. In this approach, you generate a sequence of indices that precisely match the valid indices of the list, ensuring that every index is within the list’s bounds.
This method eliminates the possibility of accidentally accessing an index that doesn’t exist, thus preventing the IndexError. Look at the example Python code below, which illustrates this method.
Code Example:
Unstop = ["upskill", "learn", "practice", "get hired"]
# Using range() with len()
for i in range(len(Unstop)):
print(Unstop[i])
Output:
upskill
learn
practice
get hired
Explanation:
In the example, we use a for loop to iterate through the Unstop list.
- In the loop condition, we use the range() and len() functions to generate indices from 0 to len(Unstop) - 1, ensuring safe iteration.
- That is, the len() function provides the length of the list, and range() defines the range in which the loop iterates.
4. Leverage enumerate() For Safe Iteration
The enumerate() function provides both the index and the value during iteration, allowing you to avoid manual index handling. This method reduces the risk of errors by automatically keeping track of the current index and element, ensuring that indices are accessed in a valid order. It also improves code readability and maintainability by removing the need for separate counter variables.
Code Example:
Unstop = ["upskill", "learn", "practice", "get hired"]
# Using enumerate()
for index, value in enumerate(Unstop):
print(f"Index {index}: {value}")
Output:
Index 0: upskill
Index 1: learn
Index 2: practice
Index 3: get hired
Explanation:
In the sample Python program, we use a for loop with the enumerate() function in its condition to avoid manual handling of indices, reducing errors and improving readability.
- The enumerate() function ensures that we get the right index and value in the list Unstop.
- As long as the loop condition is met, the print() function displays the element with the respective index.
5. Use in Operator for Membership Checks
Before attempting to access an element in the list, using the in operator checks if the element exists. This prevents errors that arise when trying to access an element that may not be present in the list. Although this method doesn't directly deal with indices, it helps avoid errors that might occur when accessing elements that are missing or when accessing by incorrect values. It ensures that the program operates safely and logically by first verifying element existence.
Code Example:
Unstop = ["upskill", "learn", "practice", "get hired"]
# Check membership before access
item = "practice"
if item in Unstop:
print(f"Found: {item}")
else:
print("Item not found")
Output:
Found: practice
Explanation:
In the sample Python code, we begin with the same list, create a variable item, and assign the value “practice” to it.
- Then, we use the in operator to set the condition for an if-else statement.
- The condition checks if the item is contained in the Unstop list.
- If the condition is true, i.e., the item is found, then the if-block prints its value using formatted strings.
- If the value is not found, i.e., the condition is false, the else block prints the message– Item not found.
This is how the in operator verifies if the item exists in the list before attempting any operation, eliminating invalid accesses.
Level up your coding skills with the 100-Day Coding Sprint at Unstop and get the bragging rights now!
Handling IndexError Gracefully Using Try-Except
Even with preventive measures in place, errors may occasionally occur in dynamic or unpredictable scenarios. Handling IndexError gracefully ensures that your program doesn’t crash abruptly, and it helps you identify and debug issues effectively.
Employ Try-Except Blocks For Error Handling
The try-except block in Python allows you to handle errors gracefully, preventing your program from crashing when an IndexError occurs. By catching the exception, you can provide a more user-friendly response or attempt an alternative action.
Code Example:
Unstop = ["upskill", "learn", "practice", "get hired"]
index = 5
try:
print(Unstop[index])
except IndexError:
print("Oops! The index you tried to access is out of range.")
Output:
Oops! The index you tried to access is out of range.
Explanation:
In the Python program sample, we start with the Unstop list and initialize a variable index with value 5.
- We then use a try-except block, wherein inside try, we use print() to access and display the value at the position given by the index.
- In case we encounter an IndexError in try-block, the flow moves to the except block, which displays an error message.
Here, we attempt to access the list using an invalid index (5). But instead of crashing, the try-except block catches the IndexError and prints a custom error message, allowing the program to continue running smoothly.
Note: Python’s error messages often include useful details, such as the index that caused the problem or the operation being performed. Analyzing these messages helps pinpoint the root cause of the error and guides you toward a solution.
Debugging Tips For IndexError: List Index Out Of Range Python
Effectively debugging IndexError requires understanding what went wrong in your code and identifying the problematic index or operation. Here are some practical tips:
- Utilize Print Statements for Troubleshooting
Insert print statements in your code to display the indices being accessed and the list's length during execution. This helps you identify when an index goes out of bounds and what led to the issue.
- Use a Debugger Tool to Step Through Your Code
Modern Python IDEs and tools provide debuggers that let you step through your code line by line. By inspecting variable values and the program's flow, you can pinpoint exactly where and why the IndexError occurs.
Looking for guidance? Find the perfect mentor from select experienced coding & software development experts here.
Conclusion
The IndexError: list index out of range is a common error encountered when working with lists in Python. It usually occurs due to accessing indices that are outside the valid range of the list.
- Understanding Python's indexing behavior, carefully handling list operations, and implementing preventive measures can significantly reduce the chances of encountering this error.
- By using robust coding practices, such as validating indices with len(), iterating safely with range() or enumerate(), and employing try-except blocks for graceful error handling, you can make your code more reliable and error-resistant.
- Additionally, debugging tools and techniques help you quickly identify and fix the root causes of any issues.
With these strategies, you’re better equipped to handle the IndexError (list index out of range) and use lists effectively, ensuring your Python programs run smoothly without unexpected interruptions.
Frequently Asked Questions
Q1. What is an IndexError (list index out of range) in Python?
An IndexError occurs when you try to access an index in a list (or other indexable data types) that is out of the valid range. The error specifically happens when you reference an index that doesn’t exist, either because it’s too large or negative beyond the available elements.
Q2. How do I prevent an IndexError (list index out of range) in Python?
To prevent an IndexError, always ensure you're working within the valid range of the list. You can:
- Check the list length using len().
- Use try-except blocks to handle errors gracefully.
- Iterate using range() or enumerate() to stay within valid index bounds.
Q3. What happens when I access an index that doesn’t exist in a list?
When you try to access an index that doesn't exist (i.e., it is beyond the list’s boundaries), Python raises an IndexError (list index out of range). This exception tells you that the requested index is invalid.
Q4. Can I use negative indices in Python?
Yes, negative indices in Python refer to elements from the end of the list. For example, -1 accesses the last element, -2 accesses the second-to-last element, and so on. However, if you use a negative index that’s too large (i.e., smaller than -len(list)), it will raise an IndexError (list index out of range).
Q5. What are some common causes of IndexError?
Some common causes of IndexError (list index out of range) include:
- Accessing an index that’s larger than the list’s length.
- Misusing negative indices that exceed the list’s range.
- Modifying a list while iterating over it can change the indices.
- Trying to access an element from an empty list.
Some common ways to avoid the IndexError (list index out of range) in Python are: pre-calculate the list length or use len(), range(), enumerate(), etc., functions for safe iterations.
Avoiding ‘List Index Out of Range’ Errors? Take A Quiz!
Do check out the following Python topics:
- Remove Duplicates From Python List | 12 Ways With Code Examples
- Random Number Generator Python Program (16 Ways + Code Examples)
- Python Libraries | Standard, Third-Party & More (Lists + Examples)
- Python input() Function (+Input Casting & Handling With Examples)
- Python Reverse List | 10 Ways & Complexity Analysis (+Examples)
- Python max() Function With Objects & Iterables (+Code Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Divyansh Shrivastava 3 weeks ago
Pranjul Srivastav 1 month ago
Ujjwal Sharma 1 month ago
niyati m singh 1 month ago
Pardha Venkata Sai Patnam 1 month ago
A Vishnuvardhan 1 month ago