Random Number Generator Python Program (16 Ways + Code Examples)
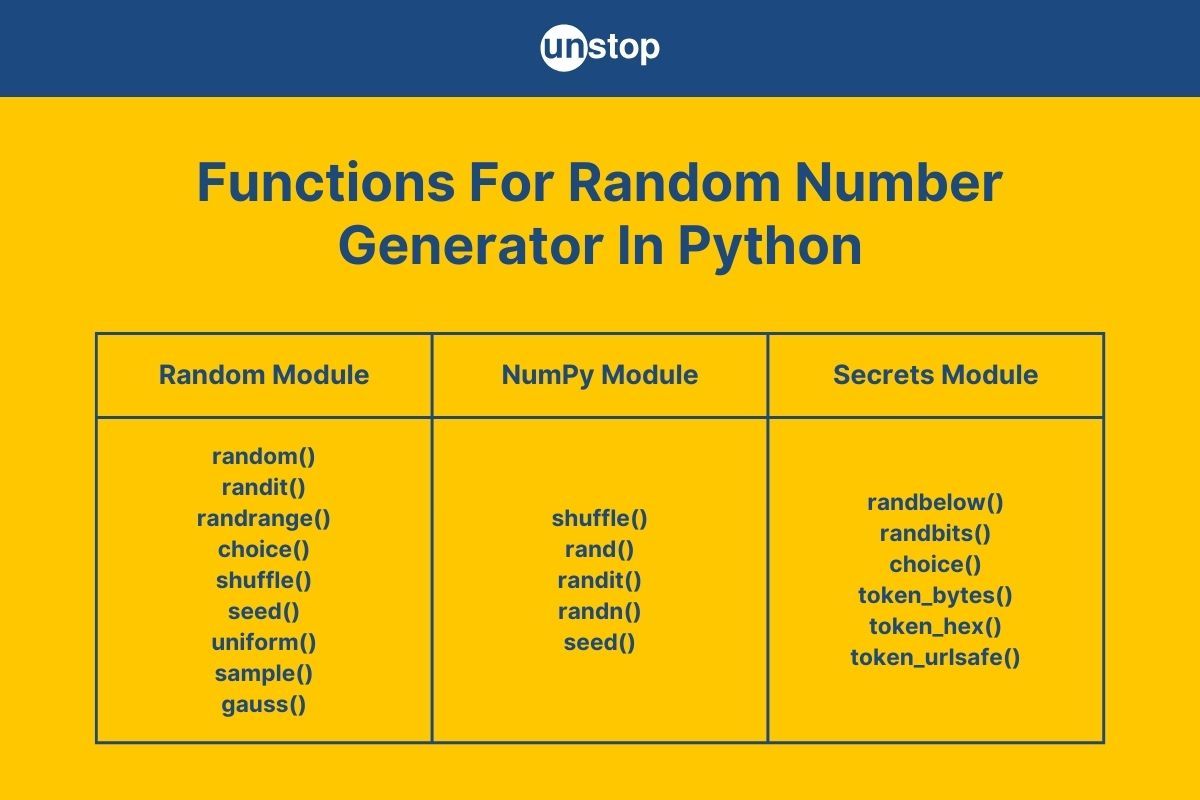
Random numbers play a crucial role in various applications, from cryptography to simulations and gaming. Writing the random number generator Python program is pretty straightforward, thanks to the robust standard library in Python language. In this article, we will guide you through creating random number generators in Python, covering the built-in random module and more advanced topics.
Different Ways To Write Random Number Generator Python Programs
As we've mentioned, random number generation is essential in various fields, such as simulations, cryptography, statistical sampling, gaming, and procedural generation in computer graphics. It allows for the creation of unpredictable results, ensuring fairness, security, and diversity in these applications.
There are three primary modules used for random number generation in Python:
- The Random Module: This is a versatile module included in Python's standard library.
- The NumPy Library/ Module: It is a powerful library for numerical computations, offering efficient random number generation.
- The Secrets Module: A module designed for cryptographically secure random numbers.
We will explore each of these modules and the built-in function that can be used to write random number generator Python programs in detail.
Random Module To Write Random Number Generator Python Programs
The random module in Python provides tools to generate pseudo-random numbers for various distributions and perform random operations on sequences. This module is part of the Python Standard Library, meaning no additional installations are required to use it. To use the random module and its random number generation-related functions, you must simply import it at the beginning of your Python script.
import random
The table below lists the different inbuilt functions available in the random module in Python. We can use them to write code to generate random numbers in Python.
Function | Description |
---|---|
random.random() | Generates a random float between 0.0 and 1.0. |
random.randint(a, b) | Generates a random integer between a and b (inclusive). |
random.randrange(start, stop, step) | Generates a random integer from start to stop-1 with a specified step. |
random.choice(seq) | Selects a random item from a non-empty sequence seq. |
random.seed(a=None) | Initializes the random number generator with a seed value a. |
random.shuffle(seq) | Shuffles the elements of a list seq in place. |
random.uniform(a, b) | Generates a random float between a and b. |
random.sample(pop, k) | Returns a k length list of unique elements chosen from pop. |
random.gauss(mu, sigma) | Generates a random float based on the Gaussian (normal) distribution with mean mu and standard deviation sigma. |
In the sections ahead, we will explore how to use every built-in Python function above to write random number generator Python programs, with examples.
Python Program To Generate Random Numbers Using random() Function
The random() function in Python's random module is a fundamental tool for generating pseudo-random floating-point numbers.
- When called, it returns a random float in the range [0.0, 1.0), where 0.0 is inclusive and 1.0 is exclusive.
- The random() function is commonly used to introduce randomness into various scenarios, such as generating probabilities, simulating natural phenomena, or normalizing data in statistical analysis.
Given below is the syntax for the function, followed by a simple Python program example that illustrates how to use it.
Syntax:
random.random()
Code Example:
Output:
Random float between 0.0 and 1.0: 0.6234567890123456
Scaled random float between 1.0 and 10.0: 8.123456789012345
List of random floats: [0.1234567890123456, 0.2345678901234567, 0.3456789012345678, 0.4567890123456789, 0.5678901234567890]
Explanation:
In the simple Python code example, we first import the random module to access its random number generation functions.
- As mentioned in the code comment, we generate a random floating number between 0.0 and 1.0 by calling the random.random() function and store it in variable random_float.
- Then, we print the generated random float with a descriptive string message using the print() function and f-strings.
- Next, we generate a random float using the pseudo-random number generator function random() once again.
- Here, we call random.random() and scale it to be between 1.0 and 10.0 by multiplying by 9 and adding 1, i.e., random.random() * 9 + 1.
- The function generates a random floating value between 0.0 and 1.0, multiplies it by 9, and scales the range between 0.0 and 9.0. Then, adding 1, it shifts the range to be between 1.0 and 10.0.
- Following this, we print the scaled random float with a descriptive message.
- Next, we generate multiple random floats using a list comprehension with the random function, i.e., [random.random() for _ in range(5)]. This generates 5 random floats and stores them in the list named random_floats.
- We also print the list of random floats with a descriptive message to the console.
Time Complexity: O(1)
Space Complexity: O(1)
Random Number Generator Python Program Using randit() Function
The randint() function in Python's random module is used to generate a random integer between two specified values, inclusive. This is particularly useful in applications requiring random selections, such as simulations, games, and random sampling.
Syntax:
random.randint(a, b)
Here, parameters a and b are the lower and upper bounds of the range (inclusive range), respectively. The example Python program below illustrates the use of this random generator function.
Code Example:
Output:
Random integer between 1 and 10: 8
Random integer between -5 and 5: 2
Random integer between 100 and 200: 183
Explanation:
In the example Python code-
- We first declare a variable random_int and assign it a value using the randit() function. That is, we generate a random integer between 1 and 10, i.e., random.randint(1, 10).
- Then, we print this value to the console using the print() function. Note that output will differ every time you run this Python program since we are generating random numbers.
- Next, we call the randit() function again to generate a random integer between -5 and 5, i.e., random.randint(-5, 5). We store the outcome in variable random_int_negative and print it to the console.
- After that, we generate a random integer between 100 and 200 using randit, i.e., random.randint(100, 200), store it in the variable random_int_large and print the same.
- In this example, we have demonstrated the use of the randit() function to generate random numbers in Python for positive, negative and large ranges.
Time Complexity: O(1)
Space Complexity: O(1)
Random Number Generator Python Program Using For-Loop & randit() To Create List Of Random Integers
We can generate a list of random integers by using the randint() function within a Python for-loop. This approach is particularly useful when you need a collection of random values for tasks such as initializing arrays, creating random datasets, or simulating random events in programs. Given below is a sample Python program illustrating this approach.
Code Example:
Output:
List of 5 random integers between 1 and 10: [10, 4, 1, 10, 9]
Explanation:
In the sample Python code-
- We initialize three integer variables, start, end, and count, with the values 1, 10, and 15, respectively. These numbers define the range and the number of random integers we want to generate.
- Then, we use list comprehension with the randit() function and a for loop to generate a list of random integers. The outcome is stored in the list random_integers.
- Here, the function call random.randint(start, end) is repeated count number of times due to the for loop.
- We print the list of generated random integers with a descriptive message.
Time Complexity: O(n)
Space Complexity: O(n)
The randrange() Function To Write Random Number Generator Python Program
The randrange() function in the random module allows for a more flexible generation of random integers, offering control over the step between values in addition to the range. This function is particularly useful when you need random integers within a range that are not necessarily consecutive. The syntax for this function is given ahead, followed by a Python program sample showcasing its usage.
Syntax:
random.randrange(start, stop, step)
Here,
- The parameters start and stop refer to the starting value of the range (inclusive) and the end value of the range (exclusive), respectively.
- The parameter step signifies the difference between each consecutive value in the range.
Code Example:
Output:
Random integer between 0 and 9: 7
Random integer between 1 and 10: 3
Random even integer between 0 and 10: 8
Explanation:
In the Python code sample-
- We use the randrange() function to first generate a random integer between 0 and 9, i.e., random.randrange(10). Here, we mention only the stop argument/ bound of the range.
- The outcome is stored in the variable random_int_default_step and printed to the console using the print() function.
- Next, we generate a random integer between 1 and 10 using the function call random.randrange(1, 11). Here, we specify the start and stop arguments for the range.
- We store the number generated in the variable random_int_range and print it with a descriptive message.
- After that, we generate a random even integer between 0 and 10 using a function call where we specify the start, stop, and step arguments, i.e., random.randrange(0, 11, 2).
- We store the outcome in the random_int_step variable and print the same to the console with a string message.
Time Complexity: O(1)
Space Complexity: O(1)
Random Number Generator Python Program Using choice() Function
The choice() function in Python's random module selects a random element from a non-empty sequence, such as a string, list, or tuple. This basic function is especially useful for making random selections from a predefined set of elements. Below is the syntax for the function, followed by a basic Python program example, where we use characters instead of integers.
Syntax:
random.choice(seq)
Here, the seq parameter is a non-empty sequence (list, tuple, string, etc.) from which to choose a random element.
Code Example:
Output:
Random fruit: banana
Random color: blue
Random letter: k
Explanation:
In the basic Python code example-
- We first create a list called fruits and initialize it with elements- apple, banana, cherry, and date.
- Then, we initialize a tuple called colors with elements- red, green, blue and yellow. And string called letters with the 'abcdefghijklmnopqrstuvwxyz'.
- Next, we use the choice() function to select a random element from each container, as follows-
- We select a random fruit from the list fruits and store it in the variable random_fruit.
- Then, we select a random color from the tuple colors and store it in the random_color variable.
- Next, we select a random letter from the string letters and store it in the random_letter variable.
- We use the print() function with f-string to print each randomly selected element with a descriptive message.
Time Complexity: O(1)
Space Complexity: O(1)
The seed() Function In Random Number Generator Python Program
The seed() function in Python's random module initialises the random number generator with a seed value. Setting the seed ensures that the sequence of random numbers generated is deterministic, meaning that the same seed will always produce the same sequence of random numbers.
This function can be particularly useful when you need reproducible results, such as in testing or debugging scenarios. Below is its syntax, followed by a Python program example to illustrate its usage.
Syntax:
random.seed(a=None)
Here, the parameter a is the seed value. If None, the current system time is used as the seed.
Code Example:
Output:
Random integer with seed 42: 81
Random integer with seed 42 again: 81
Explanation:
In the Python code example-
- We first set the seed to 42 using the random() function, i.e., random.seed(42). This ensures that the sequence of random numbers generated thereafter will be the same every time the code is run.
- Then, we generate a random integer between 1 and 100 with the randit() function, i.e., random.randint(1, 100). We store the outcome in random_int and print it with a descriptive message.
- After that, we reset the seed to 42 again using random.seed(42) to ensure the same sequence of random numbers.
- Next, we generate another random integer between 1 and 100 using the same function call random.randint(1, 100) and store it in random_int_again.
- We print the generated random integer with a descriptive message. Since the seed is set to 42 again, this random integer will be the same as random_int.
Time Complexity: O(1)
Space Complexity: O(1)
The shuffle() Function In Random Number Generator Python Program
The shuffle() function in Python's random module is used to randomly reorder the elements of a list in place. This function is useful for scenarios such as randomizing the order of items in a list, shuffling cards in a game, or creating random permutations.
Syntax:
random.shuffle(seq)
Here, the parameter seq refers to the list we want to shuffle.
Code Example:
Output:
Shuffled list: [3, 1, 5, 2, 4]
Shuffled list of words: ['date', 'apple', 'cherry', 'banana']
Explanation:
In the code-
- We define a list of numbers called numbers with the values [1, 2, 3, 4, 5].
- Then, we use the shuffle() function inside the print() function, passing the list numbers as an argument.
- The function call random.shuffle(numbers) randomly rearranges the elements of the list, and the same is printed with a descriptive message.
- Next, we define a list of strings called words and initialize it with elements- 'apple', 'banana', 'cherry', and 'date'.
- After that, we use the random() function to rearrange the elements of the list randomly, i.e., random.shuffle(words).
- The outcome is printed to the console with a descriptive message.
Time Complexity: O(n)
Space Complexity: O(1)
The uniform() Function To Write Random Number Generator Python Program
The uniform() function from the random module generates a random floating-point number between two specified values. It takes two parameters, i.e., the lower and the upper bound of the range.
This function is particularly useful for simulations, random sampling, and other applications where a continuous range of values is required.
Syntax:
random.uniform(a, b)
Here, parameters a and b represent the lower and upper bounds of the range from which we want to generate a random number.
Code Example:
Output:
Random float between 1.0 and 10.0: 7.38573242597399
Random float between -5.0 and 5.0: 2.34534598372458
Random float between 0.0 and 1.0: 0.67384948354739
Explanation:
In the code example-
- We generate a random floating value between the range 1.0 and 10.0 using the uniform() function, store it in the variable random_float and then print it to the console.
- In the function call, we specify both the upper and lower bounds, i.e., random.uniform(1.0, 10.0).
- Next, we generate a random floating value between the range -5.0 and 5.0 using the uniform() function, i.e., random.uniform(-5.0, 5.0).
- We store it in random_float_negative variable and print the generated random float with a descriptive message.
- After that, we generate a random float between the range 0.0 and 1.0 using the function call- random.uniform(0.0, 1.0).
- We store the outcome in the variable random_float_small and print the same with a descriptive message.
Time Complexity: O(1)
Space Complexity: O(1)
The sample() Function To Write Random Number Generator Python Program
The sample() function in Python's random module selects a specified number of unique elements from a population or sequence. This function is useful for tasks such as random sampling, creating test datasets, or selecting random subsets of data.
Syntax:
random.sample(population, k)
Here, the parameters population and k represent the sequence or set from which to sample and the number of unique elements to sample.
Code Example:
Output:
Sample of 3 unique numbers: [2, 5, 8]
Sample of 2 unique fruits: ['banana', 'date']
Sample of 4 unique letters: ['e', 'r', 'p', 'm']
Explanation:
In the code-
- We create and initialize a list of integers called numbers, a list of strings called fruits and a string of letters called letters.
- Then, we use the sample() function to select unique elements from the containers as follows:
- First, we sample 3 elements from the numbers list with the function call random.sample(numbers, 3). We store the outcome in random_sample_numbers.
- Then, we select 2 unique elements from the list fruits with the function call random.sample(fruits, 2). We store this in the new list random_sample_fruits.
- Lastly, we select 4 unique characters from the string letters, with function call random.sample(letters, 4) and store them in random_sample_letters.
- We print the sample selections to the console using the print() function with a descriptive message.
Time Complexity: O(k)
Space Complexity: O(k)
The gauss() Function In Random Number Generator Python Program
The gauss() function in Python's random module generates random floating-point numbers according to a Gaussian (normal) distribution. It is characterized by a mean (mu) and a standard deviation (sigma). This function is useful in simulations, statistical modelling, and any application requiring normally distributed random values.
Syntax:
random.gauss(mu, sigma)
Here, the parameters mu and sigma refer to the mean and standard deviation of the distribution, respectively.
Code Example:
Output:
Random Gaussian float (mu=0, sigma=1): 0.13659036620192342
Random Gaussian float (mu=10, sigma=2): 8.563287658990876
Explanation:
In the code-
- We generate a random float number from a Gaussian distribution with a mean of 0 and a standard deviation of 1 using the gauss() function, i.e., random.gauss(0, 1).
- The outcome is stored in the random_gaussian variable and printed to the console.
- Next, we use the gauss() function to generate a random float with a mean of 10 and a standard deviation of 2, i.e., random.gauss(10, 2) and store it in variable random_gaussian_custom.
- We finally print the generated Gaussian random float value to the console using the print() function.
Time Complexity: O(1)
Space Complexity: O(1)
The Numpy Module To Write Random Number Generator Python Programs
NumPy is a powerful Python library used for numerical computations. It provides a collection of mathematical functions to operate on data structures like arrays, matrices, etc. One of the significant advantages of NumPy Library is its efficiency and speed, which come from its underlying implementation in C and its ability to perform vectorized operations.
The library also contains a random module with many functions that we can use to randomly shuffle containers and generate random numbers (as shown in the table below).
Functions In NumPy's Random Module To Write Random Number Generator Python Programs
Function | Description |
---|---|
numpy.random.shuffle(x) | Randomly shuffles the elements in the array x in-place. |
numpy.random.randn(d0, d1, ..., dn) | Generates an array of shapes (d0, d1, ..., dn) filled with samples from the standard normal distribution. |
numpy.random.randint(low, high=None, size=None, dtype=int) | Returns random integers from low (inclusive) to high (exclusive). If high is None, returns integers from 0 to low. |
numpy.random.rand(d0, d1, ..., dn) | Generates an array of shapes (d0, d1, ..., dn) filled with samples from a uniform distribution over [0, 1). |
numpy.random.seed(seed=None) | Sets the seed for the random number generator, ensuring reproducibility of the random numbers. |
Let's explore how to use these functions to write random number generator Python programs with examples and explanations.
Random Number Generator Python Program To Shuffle NumPy Array With shuffle() Function
The shuffle() function in NumPy's random module is used to randomly reorder the elements along the first axis of an array. This function is useful for tasks such as randomizing the order of items in a dataset, shuffling data before training machine learning models, or simply generating random permutations of data.
Syntax:
numpy.random.shuffle(arr)
Here, the arr parameter refers to the array to be shuffled. The function modifies the array in place.
Code Example:
Output:
Original 1D array: [1 2 3 4 5]
Shuffled 1D array: [3 1 5 2 4]
Original 2D array:
[[1 2 3]
[4 5 6]]
Shuffled 2D array:
[[4 5 6]
[7 8 9]]
Explanation:
In the code example, we first import the NumPy module as np.
- We create a 1D NumPy array called array_1d with elements [1, 2, 3, 4, 5] using the array function from NumPy, i.e., np.array(), and then print it to the console.
- Then, we use the shuffle() function to shuffle the elements of the 1D array in-place, i.e., np.random.shuffle(array_1d) and print the same to the console.
- Next, we create a 2D NumPy array called array_2d with elements [[1, 2, 3], [4, 5, 6]], i.e., np.array(), and print it.
- After that, we shuffle the 2D array along the first axis (rows) using np.random.shuffle(array_2d). This shuffles the rows of the 2D array in-place and we print the same.
Time Complexity: O(n)
Space Complexity: O(1)
Random Number Generator Python Program With randn() Function (Array Of Random Gaussian Values) 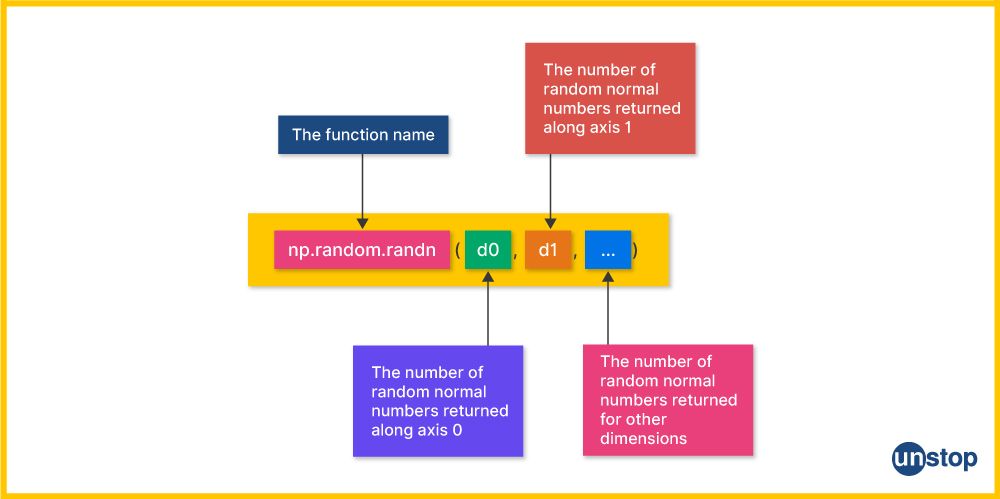
The randn() function in NumPy's random module generates samples from a standard normal distribution (mean 0, standard deviation 1). It is particularly useful for simulations, statistical modelling, and creating datasets that follow a normal distribution.
Syntax:
numpy.random.randn(d0, d1, ..., dn)
Here, the parameters (d0, d1, ..., dn) are the dimensions of the returned array. If no argument is given, a single float is returned.
Code Example:
Output:
Single random Gaussian value: 0.7326683480719926
1D array of random Gaussian values: [ 0.2502069 -0.49688522 1.14219565 -0.13421088 0.38374633]
2D array of random Gaussian values:
[[-1.51758685 -0.02937749 0.97405624 1.38784288]
[ 0.52708376 -0.38132584 0.59512134 -0.50929157]
[ 1.28778565 -1.02271012 0.0702896 -0.9390628 ]]
3D array of random Gaussian values:
[[[-1.06141024 -0.42251495 0.62187888 -0.14501411]
[ 1.10003565 0.34683267 -1.17410644 -0.2654551 ]
[ 0.67403271 -0.84637242 1.29284809 0.27001261]][[ 0.12857652 0.86641216 0.2162445 -1.5432281 ]
[-0.45122925 -0.19555358 0.82569083 -0.13040124]
[ 1.19058007 -0.78876419 0.41131967 -0.30957283]]]
Explanation:
In the code-
- We first generate a single random Gaussian value using np.random.randn(), store it in single_value variable and print it to the console using print() function.
- Then, we generate a 1D array called array_1d of 5 random Gaussian values using np.random.randn(5) and print it.
- Next, we generate a 2D array called array_2d of random Gaussian values with dimensions (3, 4) with the function call np.random.randn(3, 4) and print it.
- Lastly, we generate a 3D array called array_3d containing random Gaussian values with dimensions (2, 3, 4) using np.random.randn(2, 3, 4).
- We print the 3D array of random Gaussian values using the print() function.
Time Complexity: O(n)
Space Complexity: O(n)
Random Number Generator Python Program & The randit() Function (Array Of Random Integer Values)
The randit() function in NumPy's random module generates random integers within a specified range, inclusive of both the lower and upper bounds. This function is useful for creating arrays of random integer values, which can be used in simulations, testing, or any application requiring random integer datasets.
Syntax:
numpy.random.randint(low, high=None, size=None, dtype=int)
Here,
- Parameters low and high refer to the lower (inclusive) and the upper bounds (exclusive) of the range, respectively. If the high parameter is equated to None, the range is [0, low).
- The parameter size and type refer to the dimension/ shape and the desired data type of the output array, respectively. The default desired data type is integer (int).
Code Example:
Output:
Single random integer between 1 and 10: 1
1D array of random integers between 1 and 10: [ 5 8 10 1 5]
2D array of random integers between 0 and 20:
[[ 5 7 8]
[14 10 1]]
3D array of random integers between -10 and 10:
[[[-9 7 -8 2]
[ 4 1 5 6]
[-8 8 7 -7]][[-9 9 6 1]
[ 9 5 -8 -2]
[ 6 -8 2 -2]]]
Explanation:
In the code-
- We generate a single random integer between 1 and 10 with the function call np.random.randint(1, 11), store it in single_value variable and print it to the console using the print() function.
- Then, we generate a 1D array called array_1d containing 5 random integers between 1 and 10 using np.random.randint(1, 11, size=5) and print it.
- Next, we generate a 2D array called array_2d containing random integers between 0 and 20 with shape (2, 3) using np.random.randint(0, 20, size=(2, 3)) and print it.
- Lastly, we generate a 3D array called array_3d, containing random integers between -10 and 10 with shape (2, 3, 4) using np.random.randint(-10, 10, size=(2, 3, 4)) and print the 3D array to the console.
Time Complexity: O(n)
Space Complexity: O(n)
The rand() Function & Random Number Generator Python Program (Array Of Random Floating-Point Values)
The rand() function in NumPy's random module generates an array of random floating-point values in the half-open interval [0.0, 1.0). This function is particularly useful for generating random data for simulations, statistical modelling, and other applications where uniformly distributed random numbers are required.
Syntax:
numpy.random.rand(d0, d1, ..., dn)
Here, parameters (d0, d1, ..., dn) give the dimensions of the output array.
Code Example:
Output:
Single random floating-point value: 0.36810772666663927
The 1D array of random floating-point values: [0.16177422 0.63924101 0.05440232 0.43455883 0.18793935]
The 2D array of random floating-point values:
[[0.32867084 0.70515914]
[0.30365872 0.68890806]]
The 3D array of random floating-point values:
[[[0.1159709 0.56142271 0.29046816 0.04102852]
[0.36418783 0.66500143 0.00626299 0.54011616]][[0.35953867 0.44741456 0.72165408 0.04353927]
[0.36590939 0.36144806 0.9162406 0.69689063]]]
Explanation:
In the above code-
- We generate a single random floating-point value using np.random.rand(), store it in the single_value variable and print the same with the print() function.
- Then, we generate a 1D array named array_1d, consisting of 5 random floating-point values using np.random.rand(5) and print it.
- Next, we generate a 2D array named array_2d containing random floating-point values with shape (2, 2) using np.random.rand(2, 2) and print the 2D array.
- We generate a 3D array named array_3d of random floating-point values with shape (2, 2, 4) using np.random.rand(2, 2, 4) and print the same to the output console.
Note: The ANSI escape sequence \033[1m and \033[0m inside the f-strings is used to bold mark the enclosed section of the string.
Time Complexity: O(n)
Space Complexity: O(n)
Random Number Generator In Python With Numpy & The seed() Function
The seed() function in NumPy's random module initialises the random number generator with a seed value. This allows you to generate the same sequence of random numbers every time the code is run, which is useful for reproducibility in experiments or simulations.
Syntax:
numpy.random.seed(seed=None)
Here, the parameter seed is the seed value used to initialize the random number generator. If None, the seed is initialized based on the system time.
Code Example:
Output:
Random integers: [6 3 7 4 6]
Random floats: [0.37454012 0.95071431 0.73199394 0.59865848 0.15601864]
Same random integers: [6 3 7 4 6]
Same random floats: [0.37454012 0.95071431 0.73199394 0.59865848 0.15601864]
Explanation:
In the code above-
- We first set the seed to a specific value, 42, using np.random.seed(42). This ensures that the sequence of random numbers generated thereafter will be the same every time the code is run.
- Then, we generate a random array of integers called random_integers with values between 0 and 10 using np.random.randint(0, 10, size=5) and print it.
- Next, we generate a random array of floating-point values between 0.0 and 1.0 called random_floats using np.random.rand(5) and print it.
- After that, we reset the seed again to the same value, 42, using np.random.seed(42). This ensures that the sequence of random numbers generated from this point will be the same as before.
- Following this, we again generate the same random array of integers using np.random.randint(0, 10, size=5), store it in same_random_integers and print it.
- Lastly, we generate the same random array of floating-point values using np.random.rand(5), store it in same_random_floats and print the same to the console using print() function.
Time Complexity: O(1)
Space Complexity: O(1)
NumPy Vs. Random Module To Write Random Number Generator Python Programs
The random module in Python provides tools for generating random numbers and performing random operations. However, it is designed for simpler use cases and smaller datasets.
In contrast, NumPy's random functionalities, provided by numpy.random, are more suited for large-scale numerical computations and scientific applications. It offers a wider range of distributions and faster performance for generating large arrays of random numbers.
The Secrets Module To Write Random Number Generator Python Programs
The secrets module in Python is designed to generate cryptographically strong random numbers suitable for managing data such as passwords, account authentication, security tokens, and related secrets. This module provides higher security than the random module, which is not suitable for cryptographic purposes.
Random Number Generator Python Program Functions In Secrets Module
Function | Description |
---|---|
secrets.randbelow(n) | Returns a random integer in the range [0, n). |
secrets.randbits(k) | Returns a random integer with k random bits. |
secrets.choice(sequence) | Returns a randomly chosen element from a non-empty sequence. |
secrets.token_bytes(n) | Returns a random byte string containing n bytes. |
secrets.token_hex(n) | Returns a random text string in hexadecimal, with n bytes converted to two hex digits each. |
secrets.token_urlsafe(n) | Returns a random URL-safe text string, containing n bytes Base64 encoded. |
The sample Python code below illustrates the use of these functions.
Code Example:
Output:
Random integer with 8 bits: 153
Random choice from list: banana
Random byte string: b'\xdc\x85\x86\xed\xc7zW\xc8_>'
Random hex string: 3f92182c47ffa2349161fa67a926afba
Random URL-safe string: wR_-ODbq9LJoOC0rLX8AQw
Explanation:
In the code snippet above, we first import the secrets module to generate cryptographically secure random numbers and strings.
- Then, we generate a random integer below 10 called rand_int, using secrets.randbelow(10) and then print the random integer using the print() function.
- Next, we generate a random integer with 8 bits called rand_bits using secrets.randbits(8) and print the random integer.
- We then define a list named my_list containing ['apple', 'banana', 'cherry'].
- After that, we generate a random element stored in rand_choice from the list using secrets.choice(my_list) and print the random choice.
- Then, we generate a random byte string of 10 bytes stored in the rand_bytes variable, using secrets.token_bytes(16) and print the random byte string.
- Next, we generate a random hex string of 16 bytes stored in rand_hex, using secrets.token_hex(16) and print the random hex string using the print() function.
- Lastly, we generate a random URL-safe string of 16 bytes stored in rand_urlsafe, using secrets.token_urlsafe(16) and print it.
Time Complexity: O(1)
Space Complexity: O(1)
Understanding Randomness and Pseudo-Randomness In Python
What Is Randomness?
Randomness refers to the lack of pattern or predictability in events. In the context of computing, randomness is often required for various applications such as simulations, games, cryptography, and randomized algorithms.
- True randomness can be obtained from physical processes, such as radioactive decay or thermal noise, which are inherently unpredictable.
- However, true random number generators (TRNGs) are often not practical for most computing tasks due to their dependency on physical phenomena and their relative slowness.
- Instead, most applications use pseudo-random number generators (PRNGs), which use algorithms to produce sequences of numbers that approximate the properties of random numbers.
What Is Pseudo-Randomness In Python?
Pseudo-randomness refers to the generation of a sequence of numbers that appears to be random but is actually produced by a deterministic process.
- In Python, the random module is used to generate pseudo-random numbers. This module relies on algorithms to produce sequences of numbers that simulate randomness.
- Despite being deterministic, pseudo-random numbers can be sufficient for many applications if the algorithm used is robust enough to provide a good distribution and period.
For cryptographic purposes, however, stronger randomness guarantees are required, which are provided by modules like secrets.
The Underlying Algorithm Of Python's Random Module
Python's random module uses the Mersenne Twister algorithm, which is a widely used and well-tested pseudo-random number generator. The Mersenne Twister has several desirable properties:
- Periodicity: It has a very long period of 219937−12^{19937} - 1 , which means it will generate a vast sequence of numbers before repeating.
- Equidistribution: It provides a uniform distribution of numbers in a high-dimensional space, which makes it suitable for many statistical applications.
- Speed: The algorithm is relatively fast, making it efficient for general-purpose use.
The Mersenne Twister algorithm maintains an internal state, which is updated as random numbers are generated. The state is initialized using a seed value, which can be set using the seed() function in the random module. If the same seed is used, the sequence of random numbers generated will be identical, which is useful for debugging and testing.
Common Issues and Solutions in Random Number Generation
Random number generation is a crucial aspect of many applications in computing, from simulations and games to cryptography and data analysis. However, several common issues can arise when working with random numbers. Understanding these issues and their solutions is essential for effectively utilizing randomness and writing random number generator Python programs.
1. Pseudo-Randomness and Predictability
Issue: Pseudo-random number generators (PRNGs) produce sequences of numbers that, while appearing random, are generated by deterministic algorithms. This determinism means that if the internal state (seed) of the PRNG is known, the entire sequence can be predicted. This predictability is problematic for applications requiring high security, such as cryptography.
Solution: For cryptographic purposes, use the secrets module instead of the random module. The secrets module generates cryptographically secure random numbers that are suitable for security-sensitive applications.
Example:
import secrets
# Generate a cryptographically secure random integer below 10
secure_rand_int = secrets.randbelow(10)
print(f"Secure random integer below 10: {secure_rand_int}")
2. Reproducibility with Random Seed
Issue: In scientific research, simulations, and testing, it's often necessary to reproduce results. Without setting a seed, each run of the program will produce different sequences of random numbers, making it difficult to replicate results.
Solution: Use random.seed() to initialize the PRNG with a specific seed value. This ensures that the sequence of random numbers generated will be the same every time the program is run with that seed, aiding reproducibility.
Example:
import random
# Set the seed for reproducibility
random.seed(42)# Generate reproducible random numbers
rand_float = random.random()
rand_int = random.randint(1, 10)
print(f"Reproducible random float: {rand_float}")
print(f"Reproducible random integer: {rand_int}")
3. Quality of Randomness
Issue: The quality of randomness can vary between PRNGs. Some applications, like simulations and modelling, require high-quality randomness to ensure accurate and unbiased results.
Solution: Use well-tested and widely accepted PRNG algorithms, such as the Mersenne Twister used by Python's random module. For most purposes, this algorithm provides a good balance of speed and quality of randomness.
Example:
import random
# Generate a sequence of random numbers using Mersenne Twister
random_numbers = [random.random() for _ in range(5)]
print(f"Random numbers: {random_numbers}")
4. Performance and Efficiency
Issue: Generating random numbers can be computationally expensive, especially in large-scale simulations or real-time applications.
Solution: Optimize the use of random number generation by generating numbers in bulk when possible and reusing them. Additionally, consider the performance characteristics of different PRNGs and choose one that balances quality and speed for your specific application.
Example:
import random
# Generate a bulk of random numbers at once
random_numbers = random.sample(range(1000), 10)
print(f"Bulk random numbers: {random_numbers}")
5. Uniformity and Bias
Issue: Poorly implemented PRNGs can produce biased or non-uniform distributions, affecting the fairness and accuracy of simulations and models.
Solution: Use established libraries and fundamental functions to ensure uniformity. Python’s random module and numpy.random module provide functions that generate uniformly distributed random numbers.
Example:
import numpy as np
# Generate a uniform distribution of random numbers using NumPy
uniform_random_numbers = np.random.uniform(low=0.0, high=1.0, size=5)
print(f"Uniform random numbers: {uniform_random_numbers}")
Applications of Random Number Generator Python
Random number generators (RNGs) play a crucial role in various fields due to their ability to produce unpredictable and unbiased results. Here are some key applications:
- Cryptography: In cryptography, RNGs are essential for generating secure keys, initialization vectors, nonces, salts, and other cryptographic parameters. Security depends on the unpredictability of these values to prevent unauthorized access.
- Simulations and Modeling: RNGs are used in simulations to model complex systems and processes, such as weather forecasting, financial markets, and physical phenomena. Monte Carlo simulations, which rely heavily on random sampling, are a common example.
- Gaming: RNGs are used in gaming to ensure fair play and unpredictability. They determine outcomes like dice rolls, card shuffling, loot drops, and procedural generation of game content.
- Machine Learning and Data Science: In machine learning, RNGs are used for initializing weights, splitting datasets into training and testing sets, and performing cross-validation. Ensuring reproducibility in experiments often involves setting seeds.
- Random Sampling and Permutations: RNGs are used for randomly sampling subsets of data, which is useful in statistics, surveys, and experiments to avoid bias and ensure representative samples.
- Testing and Debugging: RNGs are used to create random test data for software testing and debugging. This helps ensure that programs can handle a wide range of input scenarios.
- Lottery and Gambling: RNGs are fundamental to lottery games, slot machines, and other forms of gambling to ensure fairness and randomness in the outcomes.
- Procedural Content Generation: RNGs are used to create procedural content in games and simulations, such as terrain, levels, characters, and quests, to provide unique experiences every time.
Conclusion
Random number generation is a fundamental aspect of many computational tasks. There are numerous ways of writing random number generator Python programs included in the random, NumPy (random) and secrets modules. Each module serves distinct purposes, ranging from general-purpose random number generation to cryptographically secure random numbers for security-sensitive applications.
Understanding the underlying principles of randomness and pseudo-randomness, as well as the strengths and limitations of different random number generation methods, is crucial for effectively utilizing these tools. Python's comprehensive support for random number generation makes it a powerful language for a wide array of applications, including simulations, machine learning, gaming, cryptography, and procedural content generation.
Frequently Asked Questions
Q. What is the difference between the random, numpy.random, and secrets modules in Python?
All three Python modules, i.e., random, numpy.random and secrets, consist of many functions that are used when writing random number generator Python programs. But certain functionalities of these modules lead to major differentiating factors, i.e.:
- The random module provides random functions for generating pseudo-random numbers for general purposes.
- The numpy.random module offers similar functionality but is optimized for performance with arrays and matrices, making it suitable for scientific computing and data analysis.
- The secrets module is designed for generating cryptographically secure random numbers, which are essential for security-sensitive applications like password generation and cryptographic keys.
Q. Why should I use secrets instead of random for cryptographic applications?
The random module uses pseudo-random number generation, which can be predictable if the internal state (seed) is known. This predictability makes it unsuitable for cryptographic purposes where unpredictability is crucial. The secrets module, on the other hand, provides a series of functions that generate cryptographically secure random numbers, ensuring that they are less predictable and more secure against attacks.
Hence, it is better to use the secrets module when writing a random number generator Python program for cryptographic applications.
Q. How can I ensure reproducibility in my random number generation?
You can set a seed for the random number generator using the random.seed() function to ensure reproducibility. This initializes the random number generator with a specific seed value, so the random sequence of numbers generated will be the same each time the program runs with that seed. This is particularly useful for debugging, testing, and scientific experiments where consistent results are required.
Q. What are some common issues when using random number generators, and how can I avoid them?
Common issues include predictability in pseudo-random number generators, the need for reproducibility in scientific experiments, and ensuring the quality and performance of random number generation. To address these issues, you can use the secrets module for cryptographic applications, set seeds for reproducibility, and choose appropriate algorithms and libraries like numpy.random for performance and quality. Additionally, understanding the underlying principles and limitations of each naive method helps in selecting the right tool for your specific application.
Q. Can I generate random numbers from a specific statistical distribution?
Yes, both the random and numpy.random Python modules provide functions to generate random numbers from specific statistical distributions. For example, you can use random.gauss(mu, sigma) to generate numbers from a Gaussian distribution or numpy.random.uniform(low, high, size) for a uniform distribution. These functions can conveniently be used when writing random number generator Python programs for simulations, modelling, and statistical analysis.
Do check out the following:
- Convert Int To String In Python | Learn 6 Methods With Examples
- How To Print Without Newline In Python? (Mulitple Ways + Examples)
- Python Bitwise Operators | Positive & Negative Numbers (+Examples)
- How To Convert Python List To String? 8 Ways Explained (+Examples)
- Python While Loop | Types With Control Statements (Code Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment