Python max() Function With Objects & Iterables (+Code Examples)
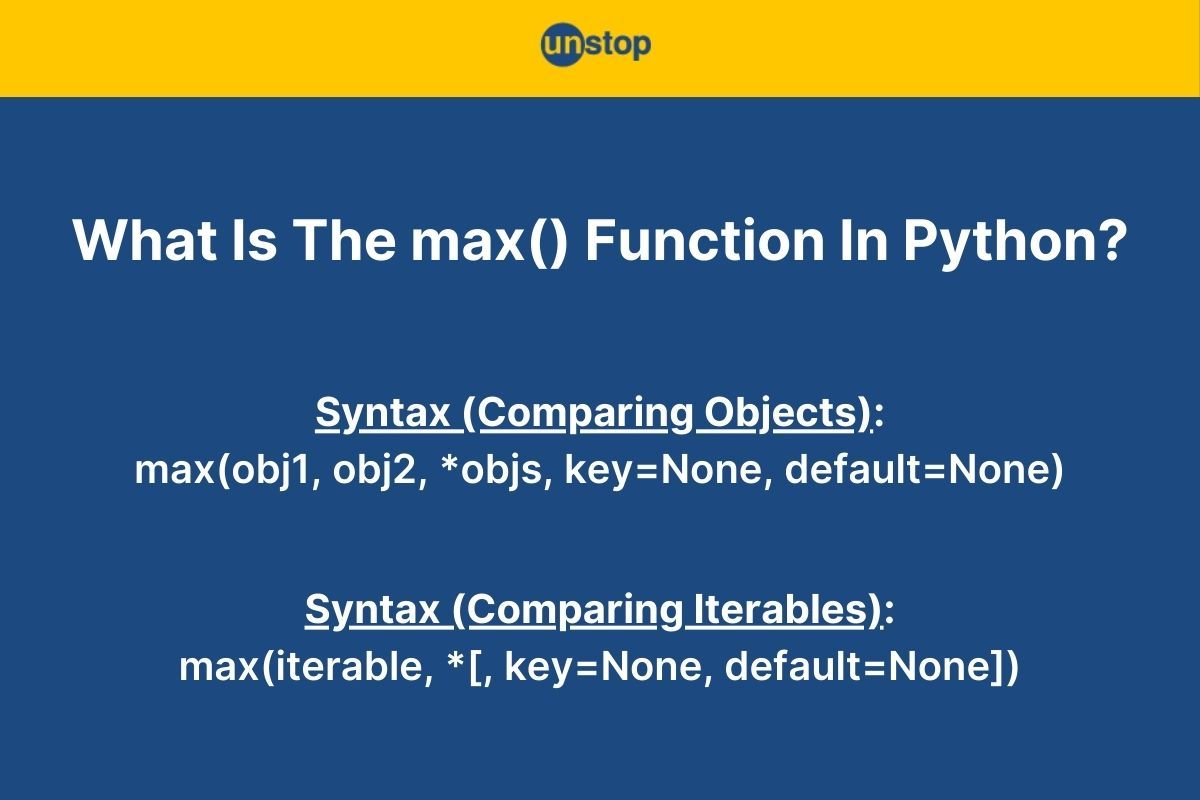
When working with data in Python, you often need to determine the largest value among a group of items. This is where the Python max() function comes into play. As one of Python’s built-in functions, max() efficiently identifies the maximum value/ largest element from a given set of inputs. Whether you're comparing numbers, strings, or other objects, max() is your go-to solution.
In this article, we’ll discuss the max function, covering its two primary forms—one for comparing objects or variables and another for working with iterable arguments. By the end, you’ll be equipped with a solid understanding of how to harness the Python max() function for various practical use cases, from simple comparisons to advanced data manipulation.
Python max() Function With Objects
In Python programming, objects are instances of classes that can hold data and have specific attributes and methods. The max function can be used to compare such objects to find the one with the maximum value/ largest item based on specified criteria. It evaluates the provided objects and returns the one considered the largest according to Python's comparison rules.
Syntax Of Python max() Function With Object
Here’s the basic syntax when using the Python max function with objects:
max(obj1, obj2, *objs, key=None, default=None)
Parameters Of Python max() Function With Objects
- Objects (obj1, obj2, ...): The input objects you want to compare to find the largest/ maximum element.
- key parameter: The key function that transforms each input before comparison (e.g., finding the object with the longest length by setting len as key argument).
- default: The (optional)- default value is used when no arguments are provided or an empty iterable is passed. This prevents errors by returning the specified default value.
Using Key & Default Parameters With Python max() Function
The key parameter customizes the comparison. For instance:
max("apple", "banana", "cherry", key=len) # Returns 'banana'
Here, the comparison is based on string length, not alphabetical order.
The default parameter provides a fallback:
max([], default="No items") # Returns 'No items'
Here, there are no iterable objects to compare, i.e., the Python list is empty. So the Python max() function returns the default value– "No items".
Return Values Of Python max() Function With Objects
The built-in Python function returns the object with the highest value among the provided inputs. If a key is specified, the returned value is based on the transformed comparison.
Exceptions Of Of Python max() Function With Objects
- TypeError: Raised when objects of incompatible types are compared (e.g., comparing a string and an integer).
- ValueError: Raised when max() is called on an empty sequence without a default value. (More on these later)
You now have a basic understanding of how the max function operates with individual objects. Now, let’s see some simple Python program examples.
Examples Of Python max() Function With Objects
In this section, we'll explore how the Python max() function operates with various object types. Each example demonstrates a different use case of the function to help you grasp its versatility.
Python max() Function To Find Maximum Of 4 Integer Variables
In this simple Python code example, we will pass four integer variables to the max() function and retrieve the largest item among them.
Code Example:
Output:
The maximum value is: 25
Explanation:
In the simple Python program,
- We define four integer variables and use the max() function to compare them.
- The function finds and returns the maximum integer value (25), which we store in the max_value variable.
- Then, we use the print() function to display it to the console.
Note: Since no additional parameters are provided, the function compares the numbers directly and selects the maximum.
Python max() Function To Find Maximum Of 4 String Variables Lexicographically
When working with strings, the max() function can be used to find the "largest" string based on lexicographical (or alphabetical) order. This means the strings are compared character by character, just like how words are arranged in a dictionary.
In this basic Python program example, we have illustrated how to use the max() function to compare strings lexicographically (alphabetical order) and find the maximum string.
Code Example:
Output:
The maximum string is: upskill
Explanation:
In this example,
- The Python max() function evaluates the string variables alphabetically and returns "upskill" because it comes last when sorted in lexicographical order.
- The comparison is based on the Unicode value of each character, and the string with the highest value (the one that would appear last in a dictionary) is returned.
Python max() Function To Find Maximum Of 4 String Variables By Length
The max() function in Python can be used to find the "largest" string from a set of strings based on different criteria. By default, max() compares the values themselves, but when we want to compare strings by a specific property—such as their length—we can use the key parameter.
In the basic Python code example below, we have illustrated how to compare four strings by proving the key parameter and determining the maximum based on length.
Code Example:
Output:
The longest string is: get hired
Explanation:
Here, max() compares the lengths of the string variables instead of their lexicographical order. The key argument set to length (key=len) directs max() to prioritize the length of each string. Since "get hired" has the most characters among the given mixture of strings, it is returned as the longest one.
Python max() Function With Exception
The max() function in Python will raise a ValueError if you attempt to find the maximum value of an empty iterable. To handle this scenario gracefully, you can use the default parameter, which allows you to specify a fallback value if the iterable is empty, thus avoiding an exception.
The Python example code below illustrates how max() handles exceptions when no valid input is provided and how the default parameter can prevent errors.
Code Example:
Output:
The maximum value is: No values
Explanation:
In this Python code example,
- We call max() on an empty list. Without a default value, this would raise a ValueError.
- However, by passing the default="No values" argument, we prevent the error and instead get the specified fallback value.
- The max() function returns "No values" because the iterable is empty. This ensures that our program runs smoothly even when no values are provided.
Check out this amazing course to become the best version of the Python programmer you can be.
Python max() Function With Floating-Point Values
Just like in the example where we compared integers, here we will use floating-point values and find the maximum number.
Code Example:
Output:
The maximum float is: 3.14
In this Python program example, the max() function compares the floating-point numbers and returns 3.14 as the highest value.
Python max() Function With Iterable
In Python, an iterable is any object that can return its elements one at a time, such as strings, dictionaries, sets, lists, and tuples. The max() function works seamlessly with iterables, allowing you to find the maximum value within them based on various criteria.
The function compares all elements in the iterable and returns the one with the highest value. You can also customize the comparison using the key parameter.
Syntax Of Python max() Function With Iterables
max(iterable, *[, key=None, default=None])
Parameters Of Python max() Function With Iterables
- iterable: The sequence (like a list or tuple) whose elements will be compared.
- key parameter: A function that applies the transformation to each element before comparison. For instance, key=str.lower would make comparisons case-insensitive.
- default parameter: A fallback value when the iterable is empty. If not provided and the iterable is empty, a ValueError is raised
Return Values Of Python max() Function With Iterables
The function returns the element with the maximum value in the iterable. If a key is provided, it returns the element with the maximum transformed value according to the key function.
Examples Of Python max() Function With Iterables
In this section, we will see how the max() function in Python works with various iterables and in various scenarios.
Python max() Function To Get Largest Item In A List
Let's see how to use the max() function to find the largest values from a list of integers.
Code Example:
Output:
The largest number in the list is: 19
Explanation:
In the example Python program, the max() function compares all the elements in the list iterable object and returns 19 as the largest value.
Python max() Function To Get Largest String In A List
When applied to strings, max() compares them lexicographically (alphabetically). We've already seen this in the example where we compared individual string objects. Let's see the implementation when working with a list of strings.
Code Example:
Output:
The lexicographically largest string is: upskill
Explanation:
In the example Python code,
- The max() function compares strings lexicographically, meaning it checks their characters' Unicode values one by one.
- In this list of strings, "upskill" comes last in lexicographical order because the letter "u" has a higher Unicode value than the starting letters of the other words.
Python max() Function In Dictionaries
When applied to a dictionary, the Python max() function, by default, performs comparisons on its keys. This means that if you directly pass a dictionary to max(), it doesn't consider the values—it only compares the keys lexicographically (i.e., based on their alphabetical or Unicode order). This is particularly useful when your focus is on sorting or finding extremes among dictionary keys rather than values.
However, if you want to compare values or even transform the comparison logic (e.g., the highest value in the dictionary), you can leverage the key parameter for customized behavior. The sample Python program below illustrates how the default mechanism works.
Code Example:
Output:
The lexicographically largest key is: Srishti
Explanation:
In this example, the max() function focuses on comparing the keys—"Shivani", "Shreeya", and "Srishti". It evaluates the keys lexicographically (i.e., in alphabetical order based on their Unicode values).
- "Shivani" and "Shreeya" both start with "S", so it moves to the next character. "h" (Shivani) equals "h" (Shreeya), so it continues further.
- "Srishti" comes later than both due to "r" in the second position, which has a higher Unicode value than "h".
- Thus, max() identifies "Srishti" as the lexicographically largest key.
Python max() Function To Get Highest Value Name (With Tie Handling)
The Python max() function, when combined with the key parameter, allows for tailored comparisons beyond the default behavior. In the case of a dictionary, this lets you evaluate values rather than just keys. When two or more values are tied for the maximum, max() resolves the tie by comparing their corresponding keys lexicographically (i.e., in alphabetical order). This is particularly handy when you need to extract the "best" key based on a combination of value and alphabetical precedence.
Code Example:
Output:
The name with the highest score is: Shivani
Explanation:
In this Python example program,
- We first compare the elements of the dictionary based on the score (defined in lambda function).
- Both "Shivani" and "Shreeya" have the highest score of 92. When there's a tie in values, max() relies on the keys' lexicographical order to decide.
- Here, the function picks "Shivani" because it is lexicographically larger than "Shreeya" (based on Unicode values for characters).
Python max() Function To Find Highest Value Item In Tuple
Here, we’ll use the max() function to find the tuple with the highest value in a list of tuples. By default, max() compares the first element of each tuple. If the first elements are equal, it compares the second element, and so on.
Code Example:
Output:
The tuple with the highest value is: (3, 7)
Explanation:
In the Python program sample, the max() function compares the tuples lexicographically:
- (2, 5) vs. (3, 7) → (3, 7) is larger since 3 > 2.
- Then, (3, 7) is compared with (1, 8) → (3, 7) remains larger because 3 > 1.
- Finally, (3, 7) is compared with (3, 5) → (3, 7) is larger because 7 > 5.
Thus, (3, 7) is returned as the tuple with the highest value.
Python max() Function To Find Largest Character In A String Lexicographically
In this example, we’ll use the max() function to find the lexicographically largest character in a string. When you apply max() to a string, it compares all the characters based on their Unicode values and returns the one with the highest value.
Code Example:
Output:
The largest character is: y
Explanation:
In this example, max() returns "y" because it has the highest lexicographical value among the characters in the string "python". The max() function compares characters based on their Unicode values, and "y" has a higher value than all the other characters in the string.
Level up your coding skills with the 100-Day Coding Sprint at Unstop and get the bragging rights, now!
Potential Errors With The Python max() Function
While the max() function is quite versatile, there are certain scenarios where it may raise errors. Understanding these potential pitfalls can help you avoid issues in your code.
1. ValueError for Empty Iterable
If you attempt to pass an empty iterable (like an empty list, tuple, or dictionary) to max(), it will raise a ValueError since there’s no item to compare.
Code Example:
Output:
Error: max() arg is an empty sequence
Explanation:
Since the list is empty, the max() function doesn't have any elements to compare, leading to a ValueError. To avoid this, you can either provide a default value using the default parameter (like we did in an example above) or check if the iterable is empty before calling max().
2. TypeError for Incompatible Data Types
The max() function in Python compares elements based on their order, and if the elements are of incompatible types, Python will raise a TypeError. For instance, if you try to compare strings and integers, you'll get an error.
Code Example:
Output:
Error: '>' not supported between instances of 'str' and 'int'
Explanation:
Here, Python doesn't know how to compare a string ('apple') with an integer (5 or 10), leading to a TypeError. You can avoid this by ensuring the elements being compared are of the same type.
3. Handling Ties Using the key Parameter
In the case of ties—where two or more elements are equally "max" based on the comparison—the max() in Python will return the first one it encounters. However, if you want to customize tie-breaking behavior, you can use the key parameter.
Code Example:
Output:
Max score holder: Shivani
Explanation:
Here, max() returns 'Shivani' because she is encountered first in the dictionary, even though both Shreeya and Shivani share the same score. If you want a different tie-breaking logic, like choosing the lexicographically larger key, you can adjust the key function.
Looking for guidance? Find the perfect mentor from select experienced coding & software experts here.
Python max() Function Vs. Python min() Functions
Both max() and min() are built-in Python functions that allow you to find the largest or smallest item (respectively) in an iterable or among multiple input values. Although they are conceptually similar, there are a few key differences in how they work and what they are used for.
The table below highlights the difference between the min() and the max() functions in Python.
Feature | max() | min() |
---|---|---|
Purpose |
Returns the largest value |
Returns the smallest value |
Default Behavior |
Finds the maximum item in an iterable or among values |
Finds the minimum item in an iterable or among values |
Functionality |
Compares values based on their natural ordering (e.g., numbers, lexicographical for strings) |
Compares values based on their natural ordering (e.g., numbers, lexicographical for strings) |
Common Use Case |
Used when finding the largest item in a collection |
Used when finding the smallest item in a collection |
Key Parameter |
Can use the key parameter to customize the comparison logic |
Can use the key parameter to customize the comparison logic |
Return Value |
The largest value based on comparison criteria |
The smallest value based on comparison criteria |
Code Example:
Output:
Max value: 25
Min value: 5
In this example, max() returns the highest value from the list, while min() finds the lowest value, demonstrating how these functions can be used to retrieve extremes from a collection.
Note: While both functions are designed to find extremes in data, they are complementary in that max() finds the largest element and min() finds the smallest. Both allow for advanced customization using the key parameter, making them highly versatile for a variety of tasks.
Conclusion
The Python max() function is a powerful tool for finding the largest element in an iterable or among multiple values. Whether you're working with numbers, strings, or dictionaries, max() simplifies the task of identifying maximum values. By leveraging the optional key parameter, you can even tailor the comparison logic to suit your needs—whether you're comparing by length, custom attributes, or handling ties. However, it's important to understand potential pitfalls, like handling empty iterables or comparing incompatible types. With these concepts in mind, you can confidently use max() in various scenarios, enhancing your Python programming skills.
Frequently Asked Questions
Q1. What happens if the iterable passed to max() is empty?
If you pass an empty iterable to max(), a ValueError will be raised. To avoid this, you can either provide a default value using the default parameter or check if the iterable is empty before calling max().
Q2. Can max() compare values of different types?
No, max() can only compare values of the same type. If you try to compare integers with strings, Python will raise a TypeError.
Q3. How does the key parameter work in max()?
The key parameter allows you to specify a function that modifies the comparison logic. For example, you can use it to compare elements based on their length, values in a dictionary, or any other custom criteria.
Q4. What happens if there is a tie between values when using max()?
If multiple elements have the same maximum value, max() will return the first one it encounters. You can adjust this behavior by using the key parameter to define custom tie-breaking rules.
Q5. How does the lambda function work with the max() function?
The lambda function allows you to define custom comparison logic for the elements in the iterable. For example, you can use a lambda function to compare elements based on a specific attribute, such as the length of strings or scores in a dictionary, rather than the default behavior of comparing the elements directly. This makes max() more flexible and powerful for complex comparisons.
Q6. Can max() be used on dictionaries?
Yes, when used on a dictionary, max(), by default, compares the dictionary keys lexicographically. If you want to compare values, you can use the key parameter to specify how the values should be compared.
You are now equipped to make the max of the Python max() function. Do check the following out:
- Relational Operators In Python | All 6 Types With Code Examples
- Fibonacci Series In Python & Nth Term | Generate & Print (+Codes)
- Python Bitwise Operators | Positive & Negative Numbers (+Examples)
- Python Logical Operators, Short-Circuiting & More (With Examples)
- Python Namespace & Variable Scope Explained (With Code Examples)
- How To Convert Python List To String? 8 Ways Explained (+Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment