Python Programming
Table of content:
- What Is Python? An Introduction
- What Is The History Of Python?
- Key Features Of The Python Programming Language
- Who Uses Python?
- Basic Characteristics Of Python Programming Syntax
- Why Should You Learn Python?
- Applications Of Python Language
- Advantages And Disadvantages Of Python
- Some Useful Python Tips & Tricks For Efficient Programming
- Python 2 Vs. Python 3: Which Should You Learn?
- Python Libraries
- Conclusion
- Frequently Asked Questions
- It's Python Basics Quiz Time!
Table of content:
- Python At A Glance
- Key Features of Python Programming
- Applications of Python
- Bonus: Interesting features of different programming languages
- Summing up...
- FAQs regarding Python
- Take A Quiz To Rehash Python's Features!
Table of content:
- What Is Python IDLE?
- What Is Python Shell & Its Uses?
- Primary Features Of Python IDLE
- How To Use Python IDLE Shell? Setting Up Your Python Environment
- How To Work With Files In Python IDLE?
- How To Execute A File In Python IDLE?
- Improving Workflow In Python IDLE Software
- Debugging In Python IDLE
- Customizing Python IDLE
- Code Examples
- Conclusion
- Frequently Asked Questions (FAQs)
- How Well Do You Know IDLE? Take A Quiz!
Table of content:
- What Is A Variable In Python?
- Creating And Declaring Python Variables
- Rules For Naming Python Variables
- How To Print Python Variables?
- How To Delete A Python Variable?
- Various Methods Of Variables Assignment In Python
- Python Variable Types
- Python Variable Scope
- Concatenating Python Variables
- Object Identity & Object References Of Python Variables
- Reserved Words/ Keywords & Python Variable Names
- Conclusion
- Frequently Asked Questions
- Rehash Python Variables Basics With A Quiz!
Table of content:
- What Is A String In Python?
- Creating String In Python
- How To Create Multiline Python Strings?
- Reassigning Python Strings
- Accessing Characters Of Python Strings
- How To Update Or Delete A Python String?
- Reversing A Python String
- Formatting Python Strings
- Concatenation & Comparison Of Python Strings
- Python String Operators
- Python String Functions
- Escape Sequences In Python Strings
- Conclusion
- Frequently Asked Questions
- Rehash Python Strings Basics With A Quiz!
Table of content:
- What Is Python Namespace?
- Lifetime Of Python Namespace
- Types Of Python Namespace
- The Built-In Namespace In Python
- The Global Namespace In Python
- The Local Namespace In Python
- The Enclosing Namespace In Python
- Variable Scope & Namespace In Python
- Python Namespace Dictionaries
- Changing Variables Out Of Their Scope & Python Namespace
- Best Practices Of Python Namespace
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Namespaces!
Table of content:
- What Are Relational Operators In Python?
- Type Of Relational Operators In Python
- Equal-To Relational Operator In Python
- Greater Than Relational Operator In Python
- Less-Than Relational Operator In Python
- Not Equal-To Relational Operator In Python
- Greater-Than Or Equal-To Relational Operator In Python
- Less-Than Or Equal-To Relational Operator In Python
- Why And When To Use Relational Operators?
- Precedence & Associativity Of Relational Operators In Python
- Advantages & Disadvantages Of Relational Operators In Python
- Real-World Applications Of Relational Operators In Python
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Logical Operators In Python?
- The AND Python Logical Operator
- The OR Python Logical Operator
- The NOT Python Logical Operator
- Short-Circuiting Evaluation Of Python Logical Operators
- Precedence of Logical Operators In Python
- How Does Python Calculate Truth Value?
- Final Note On How AND & OR Python Logical Operators Work
- Conclusion
- Frequently Asked Questions
- Python Logical Operators Quiz– Test Your Knowledge!
Table of content:
- What Is The Print() Function In Python?
- How Does The print() Function Work In Python?
- How To Print Single & Multi-line Strings In Python?
- How To Print Built-in Data Types In Python?
- Print() Function In Python For Values Stored In Variables
- Print() Function In Python With sep Parameter
- Print() Function In Python With end Parameter
- Print() Function In Python With flush Parameter
- Print() Function In Python With file Parameter
- How To Remove Newline From print() Function In Python?
- Use Cases Of The print() Function In Python
- Understanding Print Statement In Python 2 Vs. Python 3
- Conclusion
- Frequently Asked Questions
- Know The print() Function In Python? Take A Quiz!
Table of content:
- Working Of Normal Print() Function
- The New Line Character In Python
- How To Print Without Newline In Python | Using The End Parameter
- How To Print Without Newline In Python 2.x? | Using Comma Operator
- How To Print Without Newline In Python 3.x?
- How To Print Without Newline In Python With Module Sys
- The Star Pattern(*) | How To Print Without Newline & Space In Python
- How To Print A List Without Newline In Python?
- How To Remove New Lines In Python?
- Conclusion
- Frequently Asked Questions
- Think You Can Print Without a Newline in Python? Prove It!
Table of content:
- What Is A Python For Loop?
- How Does Python For Loop Work?
- When & Why To Use Python For Loops?
- Python For Loop Examples
- What Is Rrange() Function In Python?
- Nested For Loops In Python
- Python For Loop With Continue & Break Statements
- Python For Loop With Pass Statement
- Else Statement In Python For Loop
- Conclusion
- Frequently Asked Questions
- Think You Know Python's For Loop? Prove It!
Table of content:
- What Is Python While Loop?
- How Does The Python While Loop Work?
- How To Use Python While Loops For Iterations?
- Control Statements In Python While Loop With Examples
- Python While Loop With Python List
- Infinite Python While Loop in Python
- Python While Loop Multiple Conditions
- Nested Python While Loops
- Conclusion
- Frequently Asked Questions
- Mastered Python While Loop? Let’s Find Out!
Table of content:
- Understanding Python Infinite Loops
- How To Write An Infinite Loop In Python
- When Are Infinite Loops In Python Necessary?
- Types Of Infinite Loops In Python
- Python Infinite Loop With Control Statements
- Finite Vs Infinite Loops In Python
- How To Avoid Python Infinite Loops?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Conditional If-Else Statements In Python?
- Types Of If-Else Statements In Python
- If Statement In Python
- If-Else Statement In Python
- Nested If-Else Statement In Python
- Elif Statement In Python
- Ladder If-Elif-Else Statement In Python
- Short Hand If-Statement In Python
- Short Hand If-Else Statement In Python
- Operators & If-Esle Statement In Python
- Other Statements With If-Else In Python
- Conclusion
- Frequently Asked Questions
- Quick If-Else Statement Quiz– Let’s Go!
Table of content:
- What Is Control Structure In Python?
- Types Of Control Structures In Python
- Sequential Control Structures In Python
- Decision-Making Control Structures In Python
- Repetition Control Structures In Python
- Benefits Of Using Control Structures In Python
- Conclusion
- Frequently Asked Questions
- Control Structures in Python – Are You the Master? Take A Quiz!
Table of content:
- What Are Python Libraries?
- How Do Python Libraries Work?
- Standard Python Libraries (With List)
- Important Python Libraries For Data Science
- Important Python Libraries For Machine & Deep Learning
- Other Important Python Libraries You Must Know
- Working With Third-Party Python Libraries
- Troubleshooting Common Issues For Python Libraries
- Python Libraries In Larger Projects
- Importance Of Python Libraries
- Conclusion
- Frequently Asked Questions
- Quick Quiz On Python Libraries – Let’s Go!
Table of content:
- What Are Python Functions?
- How To Create/ Define Functions In Python?
- How To Call A Python Function?
- Types Of Python Functions Based On Parameters & Return Statement
- Rules & Best Practices For Naming Python Functions
- Basic Types of Python Functions
- The Return Statement In Python Functions
- Types Of Arguments In Python Functions
- Docstring In Python Functions
- Passing Parameters In Python Functions
- Python Function Variables | Scope & Lifetime
- Advantages Of Using Python Functions
- Recursive Python Function
- Anonymous/ Lambda Function In Python
- Nested Functions In Python
- Conclusion
- Frequently Asked Questions
- Python Functions – Test Your Knowledge With A Quiz!
Table of content:
- What Are Python Built-In Functions?
- Mathematical Python Built-In Functions
- Python Built-In Functions For Strings
- Input/ Output Built-In Functions In Python
- List & Tuple Python Built-In Functions
- File Handling Python Built-In Functions
- Python Built-In Functions For Dictionary
- Type Conversion Python Built-In Functions
- Basic Python Built-In Functions
- List Of Python Built-In Functions (Alphabetical)
- Conclusion
- Frequently Asked Questions
- Think You Know Python Built-in Functions? Prove It!
Table of content:
- What Is A round() Function In Python?
- How Does Python round() Function Work?
- Python round() Function If The Second Parameter Is Missing
- Python round() Function If The Second Parameter Is Present
- Python round() Function With Negative Integers
- Python round() Function With Math Library
- Python round() Function With Numpy Module
- Round Up And Round Down Numbers In Python
- Truncation Vs Rounding In Python
- Practical Applications Of Python round() Function
- Conclusion
- Frequently Asked Questions
- Revisit Python’s round() Function – Take The Quiz!
Table of content:
- What Is Python pow() Function?
- Python pow() Function Example
- Python pow() Function With Modulus (Three Parameters)
- Python pow() Function With Complex Numbers
- Python pow() Function With Floating-Point Arguments And Modulus
- Python pow() Function Implementation Cases
- Difference Between Inbuilt-pow() And math.pow() Function
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python’s pow() Function!
Table of content:
- Python max() Function With Objects
- Examples Of Python max() Function With Objects
- Python max() Function With Iterable
- Examples Of Python max() Function With Iterables
- Potential Errors With The Python max() Function
- Python max() Function Vs. Python min() Functions
- Conclusion
- Frequently Asked Questions
- Think You Know Python max() Function? Take A Quiz!
Table of content:
- What Are Strings In Python?
- What Are Python String Methods?
- List Of Python String Methods For Manipulating Case
- List Of Python String Methods For Searching & Finding
- List Of Python String Methods For Modifying & Transforming
- List Of Python String Methods For Checking Conditions
- List Of Python String Methods For Encoding & Decoding
- List Of Python String Methods For Stripping & Trimming
- List Of Python String Methods For Formatting
- Miscellaneous Python String Methods
- List Of Other Python String Operations
- Conclusion
- Frequently Asked Questions
- Mastered Python String Methods? Take A Quiz!
Table of content:
- What Is Python String?
- The Need For Python String Replacement
- The Python String replace() Method
- Multiple Replacements With Python String.replace() Method
- Replace A Character In String Using For Loop In Python
- Python String Replacement Using Slicing Method
- Replace A Character At a Given Position In Python String
- Replace Multiple Substrings With The Same String In Python
- Python String Replacement Using Regex Pattern
- Python String Replacement Using List Comprehension & Join() Method
- Python String Replacement Using Callback With re.sub() Method
- Python String Replacement With re.subn() Method
- Conclusion
- Frequently Asked Questions
- Know How To Replace Python Strings? Prove It!
Table of content:
- What Is String Slicing In Python?
- How Indexing & String Slicing Works In Python
- Extracting All Characters Using String Slicing In Python
- Extracting Characters Before & After Specific Position Using String Slicing In Python
- Extracting Characters Between Two Intervals Using String Slicing In Python
- Extracting Characters At Specific Intervals (Step) Using String Slicing In Python
- Negative Indexing & String Slicing In Python
- Handling Out-of-Bounds Indices In String Slicing In Python
- The slice() Method For String Slicing In Python
- Common Pitfalls Of String Slicing In Python
- Real-World Applications Of String Slicing
- Conclusion
- Frequently Asked Questions
- Quick Python String Slicing Quiz– Let’s Go!
Table of content:
- Introduction To Python List
- How To Create A Python List?
- How To Access Elements Of Python List?
- Accessing Multiple Elements From A Python List (Slicing)
- Access List Elements From Nested Python Lists
- How To Change Elements In Python Lists?
- How To Add Elements To Python Lists?
- Delete/ Remove Elements From Python Lists
- How To Create Copies Of Python Lists?
- Repeating Python Lists
- Ways To Iterate Over Python Lists
- How To Reverse A Python List?
- How To Sort Items Of Python Lists?
- Built-in Functions For Operations On Python Lists
- Conclusion
- Frequently Asked Questions
- Revisit Python Lists Basics With A Quick Quiz!
Table of content:
- What Is List Comprehension In Python?
- Incorporating Conditional Statements With List Comprehension In Python
- List Comprehension In Python With range()
- Filtering Lists Effectively With List Comprehension In Python
- Nested Loops With List Comprehension In Python
- Flattening Nested Lists With List Comprehension In Python
- Handling Exceptions In List Comprehension In Python
- Common Use Cases For List Comprehensions
- Advantages & Disadvantages Of List Comprehension In Python
- Best Practices For Using List Comprehension In Python
- Performance Considerations For List Comprehension In Python
- For Loops & List Comprehension In Python: A Comparison
- Difference Between Generator Expression & List Comprehension In Python
- Conclusion
- Frequently Asked Questions
- Rehash Python List Comprehension Basics With A Quiz!
Table of content:
- What Is A List In Python?
- How To Find Length Of List In Python?
- For Loop To Get Python List Length (Naive Approach)
- The len() Function To Get Length Of List In Python
- The length_hint() Function To Find Length Of List In Python
- The sum() Function To Find The Length Of List In Python
- The enumerate() Function To Find Python List Length
- The Counter Class From collections To Find Python List Length
- The List Comprehension To Find Python List Length
- Find The Length Of List In Python Using Recursion
- Comparison Between Ways To Find Python List Length
- Conclusion
- Frequently Asked Questions
- Know How To Get Python List Length? Prove it!
Table of content:
- List of Methods To Reverse A Python List
- Python Reverse List Using reverse() Method
- Python Reverse List Using the Slice Operator ([::-1])
- Python Reverse List By Swapping Elements
- Python Reverse List Using The reversed() Function
- Python Reverse List Using A for Loop
- Python Reverse List Using While Loop
- Python Reverse List Using List Comprehension
- Python Reverse List Using List Indexing
- Python Reverse List Using The range() Function
- Python Reverse List Using NumPy
- Comparison Of Ways To Reverse A Python List
- Conclusion
- Frequently Asked Questions
- Time To Test Your Python List Reversal Skills!
Table of content:
- What Is Indexing In Python?
- The Python List index() Function
- How To Use Python List index() To Find Index Of A List Element
- The Python List index() Method With Single Parameter (Start)
- The Python List index() Method With Start & Stop Parameters
- What Happens When We Use Python List index() For An Element That Doesn't Exist
- Python List index() With Nested Lists
- Fixing IndexError Using The Python List index() Method
- Python List index() With Enumerate()
- Real-world Examples Of Python List index() Method
- Difference Between find() And index() Method In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python List Indexing? Take A Quiz!
Table of content:
- How To Remove Elements From List In Python?
- The remove() Method To Remove Element From Python List
- The pop() Method To Remove Element From List In Python
- The del Keyword To Remove Element From List In Python
- The clear() Method To Remove Elements From Python List
- List Comprehensions To Conditionally Remove Element From List In Python
- Key Considerations For Removing Elements From Python Lists
- Why We Need to Remove Elements From Python List
- Performance Comparison Of Methods To Remove Element From List In Python
- Conclusion
- Frequently Asked Questions
- Quiz– Prove You Know How To Remove Item From Python Lists!
Table of content:
- How To Remove Duplicates From A List In Python?
- The set() Function To Remove Duplicates From Python List
- Remove Duplicates From Python List Using For Loop
- Using List Comprehension Remove Duplicates From Python List
- Remove Duplicates From Python List Using enumerate() With List Comprehension
- Dictionary & fromkeys() Method To Remove Duplicates From Python List
- Remove Duplicates From Python List Using in, not in Operators
- Remove Duplicates From Python List Using collections.OrderedDict.fromkeys()
- Remove Duplicates From Python List Using Counter with freq.dist() Method
- The del Keyword Remove Duplicates From Python List
- Remove Duplicates From Python List Using DataFrame
- Remove Duplicates From Python List Using pd.unique and np.unipue
- Remove Duplicates From Python List Using reduce() function
- Comparative Analysis Of Ways To Remove Duplicates From Python List
- Conclusion
- Frequently Asked Questions
- Think You Know How to Remove Duplicates? Take A Quiz!
Table of content:
- What Is Python List & How To Access Elements?
- What Is IndexError: List Index Out Of Range & Its Causes In Python?
- Understanding Indexing Behavior In Python Lists
- How to Prevent/ Fix IndexError: List Index Out Of Range In Python
- Handling IndexError Gracefully Using Try-Except
- Debugging Tips For IndexError: List Index Out Of Range Python
- Conclusion
- Frequently Asked Questions
- Avoiding ‘List Index Out of Range’ Errors? Take A Quiz!
Table of content:
- What Is the Python sort() List Method?
- Sorting In Ascending Order Using The Python sort() List Method
- How To Sort Items In Descending Order Using Python sort() List Method
- Custom Sorting Using The Key Parameter Of Python sort() List Method
- Examples Of Python sort() List Method
- What Is The sorted() List Method In Python
- Differences Between sorted() And sort() List Methods In Python
- When To Use sorted() & When To Use sort() List Method In Python
- Conclusion
- Frequently Asked Questions
- Take A Quick Python's sort() Quiz!
Table of content:
- What Is A List In Python?
- What Is A String In Python?
- Why Convert Python List To String?
- How To Convert List To String In Python?
- The join() Method To Convert Python List To String
- Convert Python List To String Through Iteration
- Convert Python List To String With List Comprehension
- The map() Function To Convert Python List To String
- Convert Python List to String Using format() Function
- Convert Python List To String Using Recursion
- Enumeration Function To Convert Python List To String
- Convert Python List To String Using Operator Module
- Python Program To Convert String To List
- Conclusion
- Frequently Asked Questions
- Convert Lists To Strings Like A Pro! Take A Quiz
Table of content:
- What Is The Python List append() Method?
- Adding Elements To A Python List Using append()
- Populate A Python List Using append()
- Adding Different Data Types To Python List Using append()
- Adding A List To Python List Using append()
- Nested Lists With Python List append() Method
- Practical Use Cases Of Python List append() Method
- How append() Method Affects List Performance
- Avoiding Common Mistakes When Using Python List append()
- Comparing extend() With append() Python List Method
- Conclusion
- Frequently Asked Questions
- 🧠 Think You Know Python List append()? Take A Quiz!
Table of content:
- What Is A Linked List In Python?
- Types Of Linked Lists In Python
- How To Create A Linked List In Python
- How To Traverse A Linked List In Python & Retrieve Elements
- Inserting Elements In A Linked List In Python
- Deleting Elements From A Linked List In Python
- Update A Node Of Linked List In Python
- Reversing A Linked List In Python
- Calculating Length Of A Linked List In Python
- Comparing Arrays And Linked Lists In Python
- Advantages & Disadvantages Of Linked List In Python
- When To Use Linked Lists Over Other Data Structures
- Practical Applications Of Linked Lists In Python
- Conclusion
- Frequently Asked Questions
- 🔗 Linked List Logic: Can You Ace This Quiz?
Table of content:
- What Is Extend In Python?
- Extend In Python With List
- Extend In Python With String
- Extend In Python With Tuple
- Extend In Python With Set
- Extend In Python With Dictionary
- Other Methods To Extend A List In Python
- Difference Between append() and extend() In Python
- Conclusion
- Frequently Asked Questions
- Think You Know extend() In Python? Prove It!
Table of content:
- What Is Recursion In Python?
- Key Components Of Recursive Functions In Python
- Implementing Recursion In Python
- Recursion Vs. Iteration In Python
- Tail Recursion In Python
- Infinite Recursion In Python
- Advantages Of Recursion In Python
- Disadvantages Of Recursion In Python
- Best Practices For Using Recursion In Python
- Conclusion
- Frequently Asked Questions
- Recursive Thinking In Python: Test Your Skills!
Table of content:
- What Is Type Conversion In Python?
- Types Of Type Conversion In Python
- Implicit Type Conversion In Python
- Explicit Type Conversion In Python
- Functions Used For Explicit Data Type Conversion In Python
- Important Type Conversion Tips In Python
- Benefits Of Type Conversion In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Type Conversion? Take A Quiz!
Table of content:
- What Is Scope In Python?
- Local Scope In Python
- Global Scope In Python
- Nonlocal (Enclosing) Scope In Python
- Built-In Scope In Python
- The LEGB Rule For Python Scope
- Python Scope And Variable Lifetime
- Best Practices For Managing Python Scope
- Conclusion
- Frequently Asked Questions
- Think You Know Python Scope? Test Yourself!
Table of content:
- Understanding The Continue Statement In Python
- How Does Continue Statement Work In Python?
- Python Continue Statement With For Loops
- Python Continue Statement With While Loops
- Python Continue Statement With Nested Loops
- Python Continue With If-Else Statement
- Difference Between Pass and Continue Statement In Python
- Practical Applications Of Continue Statement In Python
- Conclusion
- Frequently Asked Questions
- Python 'continue' Statement Quiz: Can You Ace It?
Table of content:
- What Are Control Statements In Python?
- Types Of Control Statements In Python
- Conditional Control Statements In Python
- Loop Control Statements In Python
- Control Flow Altering Statements In Python
- Exception Handling Control Statements In Python
- Conclusion
- Frequently Asked Questions
- Mastering Control Statements In Python – Take the Quiz!
Table of content:
- Understanding Python Flow Control
- Break Statement In Python
- Examples Of Break Statements In Python
- Continue Statement In Python
- Examples Of Continue Statements In Python
- Using Break and Continue Statements In A Single Program
- Difference Between Break And Continue Statements In Python
- When To Use Which Statement In Python?
- Conclusion
- Frequently Asked Questions
Table of content:
- Difference Between Mutable And Immutable Data Types in Python
- What Is Mutable Data Type In Python?
- Types Of Mutable Data Types In Python
- What Are Immutable Data Types In Python?
- Types Of Immutable Data Types In Python
- Key Similarities Between Mutable And Immutable Data Types In Python
- When To Use Mutable Vs Immutable In Python?
- Conclusion
- Frequently Asked Questions
- Quiz Time: Mutable vs. Immutable In Python!
Table of content:
- What Is A List?
- What Is A Tuple?
- Difference Between List And Tuple In Python (Comparison Table)
- Syntax Difference Between List And Tuple In Python
- Mutability Difference Between List And Tuple In Python
- Other Difference Between List And Tuple In Python
- List Vs. Tuple In Python | Methods
- When To Use Tuples Over Lists?
- Key Similarities Between Tuples And Lists In Python
- Conclusion
- Frequently Asked Questions
- 🧐 Lists vs. Tuples Quiz: Test Your Python Knowledge!
Table of content:
- What Is Inheritance In Python?
- Python Inheritance Syntax
- Parent Class In Python Inheritance
- Child Class In Python Inheritance
- The __init__() Method In Python Inheritance
- The super() Function In Python Inheritance
- Method Overriding In Python Inheritance
- Types Of Inheritance In Python
- Special Functions In Python Inheritance
- Advantages & Disadvantages Of Inheritance In Python
- Common Use Cases For Inheritance In Python
- Best Practices for Implementing Inheritance in Python
- Avoiding Common Pitfalls in Python Inheritance
- Conclusion
- Frequently Asked Questions
- 💡 Python Inheritance Quiz – Are You Ready?
Table of content:
- Introduction to Python
- Downloading & Installing Python, IDLE, Tkinter, NumPy & PyGame
- Creating A New Python Project
- How To Write Python Hello World Program In Python?
- Way To Write The Hello, World! Program In Python
- The Hello, World! Program In Python Using Class
- The Hello, World! Program In Python Using Function
- Print Hello World 5 Times Using A For Loop
- Conclusion
- Frequently Asked Questions
- 👋 Python's 'Hello, World!'—How Well Do You Know It?
Table of content:
- Algorithm Of Python Program To Add To Numbers
- Standard Program To Add Two Numbers In Python
- Python Program To Add Two Numbers With User-defined Input
- The add() Method In Python Program To Add Two Numbers
- Python Program To Add Two Numbers Using Lambda
- Python Program To Add Two Numbers Using Function
- Python Program To Add Two Numbers Using Recursion
- Python Program To Add Two Numbers Using Class
- How To Add Multiple Numbers In Python?
- Add Multiple Numbers In Python With User Input
- Time Complexities Of Python Programs To Add Two Numbers
- Conclusion
- Frequently Asked Questions
- 💡 Quiz Time: Python Addition Basics!
Table of content:
- Swapping in Python
- Swapping Two Variables Using A Temporary Variable
- Swapping Two Variables Using The Comma Operator In Python
- Swapping Two Variables Using The Arithmetic Operators (+,-)
- Swapping Two Variables Using The Arithmetic Operators (*,/)
- Swapping Two Variables Using The XOR(^) Operator
- Swapping Two Variables Using Bitwise Addition and Subtraction
- Swap Variables In A List
- Conclusion
- Frequently Asked Questions (FAQs)
- Quiz To Test Your Variable Swapping Knowledge
Table of content:
- What Is A Quadratic Equation? How To Solve It?
- How To Write A Python Program To Solve Quadratic Equations?
- Python Program To Solve Quadratic Equations Directly Using The Formula
- Python Program To Solve Quadratic Equations Using The Complex Math Module
- Python Program To Solve Quadratic Equations Using Functions
- Python Program To Solve Quadratic Equations & Find Number Of Solutions
- Python Program To Plot Quadratic Functions
- Conclusion
- Frequently Asked Questions
- Quadratic Equations In Python Quiz: Test Your Knowledge!
Table of content:
- What Is Decimal Number System?
- What Is Binary Number System?
- What Is Octal Number System?
- What Is Hexadecimal Number System?
- Python Program to Convert Decimal to Binary, Octal, And Hexadecimal Using Built-In Function
- Python Program To Convert Decimal To Binary Using Recursion
- Python Program To Convert Decimal To Octal Using Recursion
- Python Program To Convert Decimal To Hexadecimal Using Recursion
- Python Program To Convert Decimal To Binary Using While Loop
- Python Program To Convert Decimal To Octal Using While Loop
- Python Program To Convert Decimal To Hexadecimal Using While Loop
- Convert Decimal To Binary, Octal, And Hexadecimal Using String Formatting
- Python Program To Convert Binary, Octal, And Hexadecimal String To A Number
- Complexity Comparison Of Python Programs To Convert Decimal To Binary, Octal, And Hexadecimal
- Conclusion
- Frequently Asked Questions
- 💡 Decimal To Binary, Octal & Hex: Quiz Time!
Table of content:
- What Is A Square Root?
- Python Program To Find The Square Root Of A Number
- The pow() Function In Python Program To Find The Square Root Of Given Number
- Python Program To Find Square Root Using The sqrt() Function
- The cmath Module & Python Program To Find The Square Root Of A Number
- Python Program To Find Square Root Using The Exponent Operator (**)
- Python Program To Find Square Root With A User-Defined Function
- Python Program To Find Square Root Using A Class
- Python Program To Find Square Root Using Binary Search
- Python Program To Find Square Root Using NumPy Module
- Conclusion
- Frequently Asked Questions
- 🤓 Think You Know Square Roots In Python? Take A Quiz!
Table of content:
- Understanding the Logic Behind the Conversion of Kilometers to Miles
- Steps To Write Python Program To Convert Kilometers To Miles
- Python Program To Convert Kilometer To Miles Without Function
- Python Program To Convert Kilometer To Miles Using Function
- Python Program to Convert Kilometer To Miles Using Class
- Tips For Writing Python Program To Convert Kilometer To Miles
- Conclusion
- Frequently Asked Questions
- 🧐 Mastered Kilometer To Mile Conversion? Prove It!
Table of content:
- Why Build A Calculator Program In Python?
- Prerequisites To Writing A Calculator Program In Python
- Approach For Writing A Calculator Program In Python
- Simple Calculator Program In Python
- Calculator Program In Python Using Functions
- Creating GUI Calculator Program In Python Using Tkinter
- Conclusion
- Frequently Asked Questions
- 🧮 Calculator Program In Python Quiz!
Table of content:
- The Calendar Module In Python
- Prerequisites For Writing A Calendar Program In Python
- How To Write And Print A Calendar Program In Python
- Calendar Program In Python To Display A Month
- Calendar Program In Python To Display A Year
- Conclusion
- Frequently Asked Questions
- Calendar Program In Python – Quiz Time!
Table of content:
- What Is The Fibonacci Series?
- Pseudocode Code For Fibonacci Series Program In Python
- Generating Fibonacci Series In Python Using Naive Approach (While Loop)
- Fibonacci Series Program In Python Using The Direct Formula
- How To Generate Fibonacci Series In Python Using Recursion?
- Generating Fibonacci Series In Python With Dynamic Programming
- Fibonacci Series Program In Python Using For Loop
- Generating Fibonacci Series In Python Using If-Else Statement
- Generating Fibonacci Series In Python Using Arrays
- Generating Fibonacci Series In Python Using Cache
- Generating Fibonacci Series In Python Using Backtracking
- Fibonacci Series In Python Using Power Of Matix
- Complexity Analysis For Fibonacci Series Programs In Python
- Applications Of Fibonacci Series In Python & Programming
- Conclusion
- Frequently Asked Questions
- 🤔 Think You Know Fibonacci Series? Take A Quiz!
Table of content:
- Different Ways To Write Random Number Generator Python Programs
- Random Module To Write Random Number Generator Python Programs
- The Numpy Module To Write Random Number Generator Python Programs
- The Secrets Module To Write Random Number Generator Python Programs
- Understanding Randomness and Pseudo-Randomness In Python
- Common Issues and Solutions in Random Number Generation
- Applications of Random Number Generator Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python's Random Module? Prove It!
Table of content:
- What Is A Factorial?
- Algorithm Of Program To Find Factorial Of A Number In Python
- Pseudocode For Factorial Program in Python
- Factorial Program In Python Using For Loop
- Factorial Program In Python Using Recursion
- Factorial Program In Python Using While Loop
- Factorial Program In Python Using If-Else Statement
- The math Module | Factorial Program In Python Using Built-In Factorial() Function
- Python Program to Find Factorial of a Number Using Ternary Operator(One Line Solution)
- Python Program For Factorial Using Prime Factorization Method
- NumPy Module | Factorial Program In Python Using numpy.prod() Function
- Complexity Analysis Of Factorial Programs In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Factorials In Python? Take A Quiz!
Table of content:
- What Is Palindrome In Python?
- Check Palindrome In Python Using While Loop (Iterative Approach)
- Check Palindrome In Python Using For Loop And Character Matching
- Check Palindrome In Python Using The Reverse And Compare Method (Python Slicing)
- Check Palindrome In Python Using The In-built reversed() And join() Methods
- Check Palindrome In Python Using Recursion Method
- Check Palindrome In Python Using Flag
- Check Palindrome In Python Using One Extra Variable
- Check Palindrome In Python By Building Reverse, One Character At A Time
- Complexity Analysis For Palindrome Programs In Python
- Real-World Applications Of Palindrome In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Palindromes? Take A Quiz!
Table of content:
- Best Python Books For Beginners
- Best Python Books For Intermediate Level
- Best Python Books For Experts
- Best Python Books To Learn Algorithms
- Audiobooks of Python
- Best Books To Learn Python And Code Like A Pro
- To Learn Python Libraries
- Books To Provide Extra Edge In Python
- Python Project Ideas - Reference
- Quiz To Rehash Your Knowledge Of Python Books!
Calculator Program In Python - Explained With Code Examples
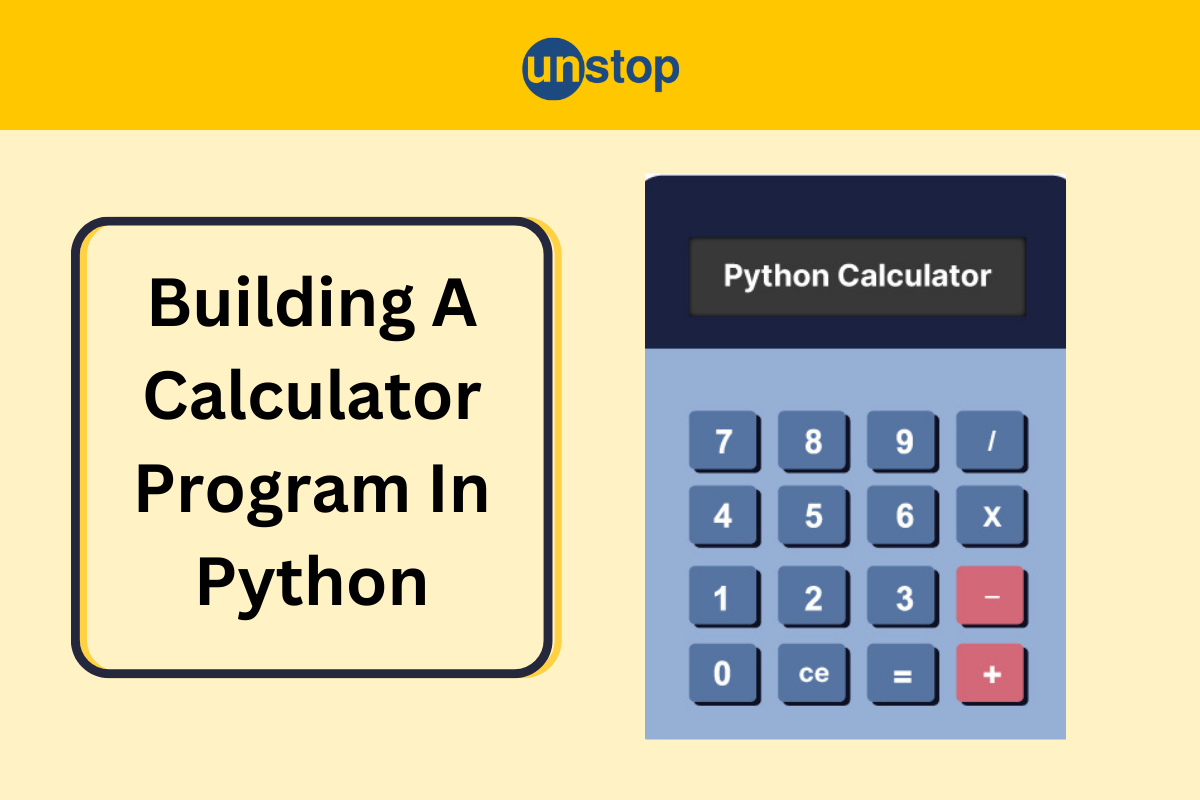
Python is a versatile programming language known for its simplicity and readability, making it ideal for a wide range of applications from web development to scientific computing. Learning Python opens up a world of possibilities for programmers, and creating a fully functional calculator program in Python is a great way to get started. In this article, we will do just that. You will learn how to write a Python program that can be used as a simple calculator.
Let's first understand the fundamental function of a calculator in computing, a tool that goes beyond simple arithmetic, before diving into the calculator code.
Why Build A Calculator Program In Python?
For those who are new to Python programming, building a Python calculator provides an engaging and instructive experience that allows for customization and additional development as one's abilities advance. Here are some of the benefits you’ll get from building a calculator program in Python:
- Learning Programming Concepts: For novice programmers, creating a calculator in Python can be a great way to acquire the essentials of the language. It allows practice with variables, functions, and control structures like loops and conditional statements. It covers basic arithmetic operations (addition, subtraction, multiplication, and division).
- Practical Exercise: Using a calculator allows you to put your theoretical knowledge to use in a real-world project. It aids in comprehending how to use Python for computations, handle user input, and organize code.
- Customization and Improvement: After you've made a simple calculator, you can add more features to increase its usefulness. For instance, you could use libraries like Tkinter to create a graphical user interface (GUI), handle errors gracefully, implement scientific functions (trigonometry, exponentiation, and logarithms), and design an intuitive user interface.
Put your tech skills to the test! Sharpen your computer fundamentals with Unstop
Prerequisites To Writing A Calculator Program In Python
In order to develop a simple calculator in Python, you'll need to know about or check the following:
- Installation of Python: Verify that Python is installed on your computer. From the official website, python.org, you can download and install Python. Select the most recent stable version that is currently accessible. Python 2 and Python 3 can both be used to build the calculator, but Python 3 is advised due to its continuous support and enhancements.
- A text editor or Integrated Development Environment (IDE): To write and run Python code, you'll need a coding environment. You can use IDEs such as VSCode, PyCharm, or Python IDLE (the built-in IDE for Python). Alternatively, all you need to write Python code is a basic text editor such as Notepad++, Atom, or Sublime Text.
- Basic Syntax Understanding: Become acquainted with the syntax, variables, data types, operators, control structures (loops, if statements), and functions of Python. To help you understand these ideas, there are online tutorials, documentation, and learning resources for Python available.
- Handling Input/Output: Recognize how to use Python to manage input from users and display output. Users can provide input using the input() function, and output can be shown on the console using the print() function.
Approach For Writing A Calculator Program In Python
Writing a calculator program in Python generally entails the following steps:
- Recognize requirements: Clearly define the features that the calculator must have. Select the basic arithmetic operations (multiplication, division, addition, and subtraction) that the calculator will execute. It's also possible to think about adding features like exponentiation, square root, trigonometric functions, etc.
- Create the Program Structure: Make a plan for your program's structure. Choose the functions, variables, and control flow that you will require. To keep your code organized, you could, for example, write different functions for each arithmetic operation.
- Gather User Input: To gather user input, use Python's input() function. Request that the user input the desired operation and a number.
- Carry Out Calculations: Using Python's built-in arithmetic operators (+, -, *, /), carry out the necessary arithmetic operation based on the user's input. Make sure to gracefully handle situations such as division by zero or invalid inputs.
- Present the Result: After the computation is finished, use Python's print() function to present the result to the user.
- Add More Functionalities (Optional): If you'd like, you can incorporate additional features like managing decimals, carrying out scientific computations, or designing an intuitive user interface (graphical or command-line).
- Error Handling: Use error handling to deal with unforeseen inputs and potential calculation errors. Try and except blocks can be used to catch exceptions and show informative error messages.
Simple Calculator Program In Python
We'll be breaking down the process of writing the Python calculator program into easy steps. To aid in a thorough understanding of the concepts, a basic calculator program in Python that can carry out addition, subtraction, multiplication, and division—all of which depend on the input provided by the user—should be created.
Step 1: Create a File for the Calculator
To begin writing a calculator program in Python, we first need to create a file. The method to get this done is given below.
Method Description:
- Creating a Python File: Open a text editor or an IDE and create a new file with a ".py" extension. For example, name it calculator.py.
- Write Python Code: Inside the file, start writing Python code to build the calculator.
Code Example (calculator.py):
# Simple Calculator Program in Python
# Function to add two numbers
def add(x, y):
return x + y# Function to subtract two numbers
def subtract(x, y):
return x - y# Function to multiply two numbers
def multiply(x, y):
return x * y# Function to divide two numbers
def divide(x, y):
if y == 0:
return "Cannot divide by zero"
return x / y# Display operations to the user
print("Select operation:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
Step 2: Prompt for User Input
The next step is to prompt the user to provide us with valid input. The method for the same and an example code is given below.
Method Description:
- Use input() Function: Prompt the user to select an operation and enter numbers for calculation.
- Assign Inputs to Variables: Read user input and assign the input values to variables.
Code Example (continuation in calculator.py):
# Get user input for operation choice
choice = input("Enter choice (1/2/3/4): ")# Get user input for numbers
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
Step 3: Put Calculation Logic & Implement Conditional Statements
The user is prompted to select an operation for further calculation. The method for the same and an example code is given below.
Method Description:
- Once the user submits the input, we use the if-elif statement to execute the respective function.
- In addition to the four options, we also have an else block, in case the user inputs irrelevant data.
- We pass the variables input by the user into the function. The statement thus returns the outcome of the operations.
Code Example (continuation in calculator.py):
# Based on the user's choice, perform the arithmetic operations
if choice == '1':
result = num1 + num2
elif choice == '2':
result = num1 - num2
elif choice == '3':
result = num1 * num2
elif choice == '4':
if num2 == 0:
result = "Cannot divide by zero"
else:
result = num1 / num2
else:
result = "Invalid input"
Step 4: Check & Print the Output
In this step, conditional statements are used to analyse if the outcome is a valid result of calculations, or something went wrong.
Method Description:
- We use an if-else statement with equality relational operator and OR logical operator. Here we check if the outcome is either 'Invalid Input' or 'Cannot Divide by zero'
- If this condition is true, then we display the same message. If false, then we display the actual outcome of the calculation.
Code Example (continuation in calculator.py):
# Provide feedback for invalid input
if result == "Invalid input" or result == "Cannot divide by zero":
print(result)
else:
print("Result:", result)
Now that we know the different segments of how to write the calculator program in Python language, let's take a look at a complete example. It will help you understand how all of the components discussed above come into play.
Calculator Program In Python Using Functions
For using functions to encapsulate arithmetic operations (addition, subtraction, multiplication, and division), the method entails writing a Python calculator program. Functions facilitate the modularization of the code, improving its organization, readability, and reusability.
General approach followed in coding a calculator using functions:
- Define Functions: For every arithmetic operation (addition, subtraction, multiplication, and division), create a distinct function.
- Display Operations: Inform the user of the available arithmetic operations.
- Accept User Input: Ask the user to select an action and enter a number.
- Carry Out Calculations: Select the appropriate function and call it to carry out the calculation.
- Display Result: Show the user the computation's outcome.
Now, let's code this to get a better understanding of the given approach:
Code Example:
IyBGdW5jdGlvbnMgZm9yIGFyaXRobWV0aWMgb3BlcmF0aW9ucw0KZGVmIGFkZCh4LCB5KToNCiAgcmV0dXJuIHggKyB5DQoNCmRlZiBzdWJ0cmFjdCh4LCB5KToNCiAgcmV0dXJuIHggLSB5DQoNCmRlZiBtdWx0aXBseSh4LCB5KToNCiAgcmV0dXJuIHggKiB5DQoNCmRlZiBkaXZpZGUoeCwgeSk6DQogIGlmIHkgPT0gMDoNCiAgIHJldHVybiAiQ2Fubm90IGRpdmlkZSBieSB6ZXJvIg0KICByZXR1cm4geCAvIHkNCg0KIyBEaXNwbGF5IG9wZXJhdGlvbnMgdG8gdGhlIHVzZXINCnByaW50KCJTZWxlY3Qgb3BlcmF0aW9uOiIpDQpwcmludCgiMS4gQWRkIikNCnByaW50KCIyLiBTdWJ0cmFjdCIpDQpwcmludCgiMy4gTXVsdGlwbHkiKQ0KcHJpbnQoIjQuIERpdmlkZSIpDQoNCiMgR2V0IHVzZXIgaW5wdXQgZm9yIG9wZXJhdGlvbiBjaG9pY2UgYW5kIG51bWJlcnMNCmNob2ljZSA9IGlucHV0KCJFbnRlciBjaG9pY2UgKDEvMi8zLzQpOiAiKQ0KbnVtMSA9IGZsb2F0KGlucHV0KCJFbnRlciBmaXJzdCBudW1iZXI6ICIpKQ0KbnVtMiA9IGZsb2F0KGlucHV0KCJFbnRlciBzZWNvbmQgbnVtYmVyOiAiKSkNCg0KIyBCYXNlZCBvbiB0aGUgdXNlcidzIGNob2ljZSwgcGVyZm9ybSB0aGUgYXJpdGhtZXRpYyBvcGVyYXRpb25zDQppZiBjaG9pY2UgPT0gJzEnOg0KICByZXN1bHQgPSBhZGQobnVtMSwgbnVtMikNCmVsaWYgY2hvaWNlID09ICcyJzoNCiAgcmVzdWx0ID0gc3VidHJhY3QobnVtMSwgbnVtMikNCmVsaWYgY2hvaWNlID09ICczJzoNCiAgcmVzdWx0ID0gbXVsdGlwbHkobnVtMSwgbnVtMikNCmVsaWYgY2hvaWNlID09ICc0JzoNCiAgcmVzdWx0ID0gZGl2aWRlKG51bTEsIG51bTIpDQplbHNlOg0KICByZXN1bHQgPSAiSW52YWxpZCBpbnB1dCINCg0KIyBQcm92aWRlIGZlZWRiYWNrIGZvciBpbnZhbGlkIGlucHV0DQppZiByZXN1bHQgPT0gIkludmFsaWQgaW5wdXQiIG9yIHJlc3VsdCA9PSAiQ2Fubm90IGRpdmlkZSBieSB6ZXJvIjoNCiAgcHJpbnQocmVzdWx0KQ0KZWxzZToNCiAgcHJpbnQoIlJlc3VsdDoiLCByZXN1bHQp
Output:
Select operation:
1. Add
2. Subtract
3. Multiply
4. Divide
Enter choice (1/2/3/4): 3
Enter first number: 4
Enter second number: 5
Result: 20.0
The user is prompted to choose an operation and enter numbers when the code runs. Once the options and numbers have been entered, the code executes the selected action and shows the outcome or the relevant error message in the event that the input is incorrect.
Explanation:
1. The code begins by defining four functions, each representing a basic arithmetic operation, i.e., add(x, y), subtract(x, y), multiply(x, y), and divide(x, y).
2. Each function uses the respective arithmetic operator to make calculations on input variables and gives the outcome stored in variable result.
3. Then, we use a set of print() statements to display the available operations (addition, subtraction, multiplication, and division).
4. Next, we use the input() function to prompt the user to select one of the operations by providing a number between 1 to 4, indicating the preferred operation.
5. Again, using the input() functions, we prompt the user to provide values for two variables, num1 and num2.
6. We then use the conditional if-elif statement to determine which operation must be executed depending on user input and provide the result thereof. Inside the statement-
- If the user selects option 1, then num1 and num2 are passed to the add() function, which adds the numbers and returns the outcome.
- For option 2, num1 and num2 are passed to the subtract() function. The function subtracts num2 from num1 and returns the outcome to the result variable.
- For option 3, num1 and num2 are passed to the multiply() function, which returns the product of the variable.
- For option 4, num1 and num2 are passed to the divide() function. The function first checks if the denominator is equal to 0. If true, it returns the string- Cannot divide by 0. If not, then it returns the outcome of the division.
- If none of the above options are picked, then the else block returns 'Invalid output'.
7. We then employ an if-else statetment to print the outcome. It first checks if the outcome is either 'Invalid Input' or 'Cannot be divided by zero' from option 4. If this is true, the same is printed to the console using print() method.
8. If it is false, then the else block is executed which prints the result along with the numerical value.
Creating GUI Calculator Program In Python Using Tkinter
Building a graphical user interface (GUI) application that allows users to do arithmetic operations through a visual interface is part of creating a GUI calculator using Tkinter in Python. Python's built-in GUI library, Tkinter, offers tools for creating windows, buttons, labels, and text fields. Here’s what a simple GUI calculator will look like:
Before diving into the code let’s take a look at the general approach that needs to be followed for coding this calculator:
- Import Tkinter: To create the GUI elements, import the Tkinter module.
- Build GUI Window: Construct the calculator application's main window.
- Facilitate user interaction: Add buttons, labels, and input fields to the user interface design to facilitate user interaction.
- Explain Functions: Make functions to carry out mathematical operations.
- Connect UI Functions: To execute an operation, connect the functions to the buttons.
- Launch the program: Initiate the main event loop in order to manage user input and display the GUI.
Now, let us try to understand this with an example code:
aW1wb3J0IHRraW50ZXIgYXMgdGsNCg0KZGVmIGNsaWNrX2J1dHRvbihvcGVyYXRpb24pOg0KICAgIHRyeToNCiAgICAgICAgbnVtMSA9IGZsb2F0KGVudHJ5MS5nZXQoKSkNCiAgICAgICAgbnVtMiA9IGZsb2F0KGVudHJ5Mi5nZXQoKSkNCiAgICAgICAgDQogICAgICAgIGlmIG9wZXJhdGlvbiA9PSAnKyc6DQogICAgICAgICAgICByZXN1bHQgPSBudW0xICsgbnVtMg0KICAgICAgICBlbGlmIG9wZXJhdGlvbiA9PSAnLSc6DQogICAgICAgICAgICByZXN1bHQgPSBudW0xIC0gbnVtMg0KICAgICAgICBlbGlmIG9wZXJhdGlvbiA9PSAnKic6DQogICAgICAgICAgICByZXN1bHQgPSBudW0xICogbnVtMg0KICAgICAgICBlbGlmIG9wZXJhdGlvbiA9PSAnLyc6DQogICAgICAgICAgICBpZiBudW0yID09IDA6DQogICAgICAgICAgICAgICAgcmVzdWx0ID0gIkNhbm5vdCBkaXZpZGUgYnkgemVybyINCiAgICAgICAgICAgIGVsc2U6DQogICAgICAgICAgICAgICAgcmVzdWx0ID0gbnVtMSAvIG51bTINCiAgICAgICAgZWxzZToNCiAgICAgICAgICAgIHJlc3VsdCA9ICJJbnZhbGlkIG9wZXJhdGlvbiINCiAgICAgICAgICAgIGxhYmVsX3Jlc3VsdC5jb25maWcodGV4dD0iUmVzdWx0OiAiICsgc3RyKHJlc3VsdCkpDQoNCiAgICBleGNlcHQgVmFsdWVFcnJvcjoNCiAgICAgICAgbGFiZWxfcmVzdWx0LmNvbmZpZyh0ZXh0PSJJbnZhbGlkIGlucHV0IikNCg0Kcm9vdCA9IHRrLlRrKCkNCnJvb3QudGl0bGUoIlNpbXBsZSBDYWxjdWxhdG9yIikNCg0KbGFiZWxfbnVtMSA9IHRrLkxhYmVsKHJvb3QsIHRleHQ9IkVudGVyIGZpcnN0IG51bWJlcjoiKQ0KbGFiZWxfbnVtMS5wYWNrKCkNCg0KZW50cnkxID0gdGsuRW50cnkocm9vdCkNCmVudHJ5MS5wYWNrKCkNCg0KbGFiZWxfbnVtMiA9IHRrLkxhYmVsKHJvb3QsIHRleHQ9IkVudGVyIHNlY29uZCBudW1iZXI6IikNCmxhYmVsX251bTIucGFjaygpDQoNCmVudHJ5MiA9IHRrLkVudHJ5KHJvb3QpDQplbnRyeTIucGFjaygpDQoNCmJ1dHRvbl9hZGQgPSB0ay5CdXR0b24ocm9vdCwgdGV4dD0iKyIsIGNvbW1hbmQ9bGFtYmRhOiBjbGlja19idXR0b24oJysnKSkNCmJ1dHRvbl9zdWJ0cmFjdCA9IHRrLkJ1dHRvbihyb290LCB0ZXh0PSItIiwgY29tbWFuZD1sYW1iZGE6IGNsaWNrX2J1dHRvbignLScpKQ0KYnV0dG9uX211bHRpcGx5ID0gdGsuQnV0dG9uKHJvb3QsIHRleHQ9IioiLCBjb21tYW5kPWxhbWJkYTogY2xpY2tfYnV0dG9uKCcqJykpDQpidXR0b25fZGl2aWRlID0gdGsuQnV0dG9uKHJvb3QsIHRleHQ9Ii8iLCBjb21tYW5kPWxhbWJkYTogY2xpY2tfYnV0dG9uKCcvJykpDQoNCmJ1dHRvbl9hZGQucGFjayhzaWRlPXRrLkxFRlQpDQpidXR0b25fc3VidHJhY3QucGFjayhzaWRlPXRrLkxFRlQpDQpidXR0b25fbXVsdGlwbHkucGFjayhzaWRlPXRrLkxFRlQpDQpidXR0b25fZGl2aWRlLnBhY2soc2lkZT10ay5MRUZUKQ0KDQpsYWJlbF9yZXN1bHQgPSB0ay5MYWJlbChyb290LCB0ZXh0PSIiKQ0KbGFiZWxfcmVzdWx0LnBhY2soKQ0KDQpyb290Lm1haW5sb29wKCkNCg==
Output:
When this code is executed, a GUI window that looks like a basic calculator interface will appear. The calculator's buttons allow users to enter numbers, carry out arithmetic operations, clear their input, and obtain the result by clicking the "Equal" button.
Explanation:
The provided code snippet explains the steps involved in building a basic Tkinter calculator as well as its features and components.
- We begin by importing the tinker module which helps create a window/ make graphical interfaces. This window will be our calculator with buttons, input boxes, and labels.
- After that, the code uses a try-execpt block with if-elif conditional statement to define click_button() function and set up functions that will be called when any of the operation buttons ('+', '-', '*', '/') is clicked.
- It attempts to extract numerical values from entry1 and entry2 fields, representing the user input.
- Finally, it updates the label_result text to display the calculated result or an error message.
- Now using, GUI Creation using Tkinter creates a Tkinter window (root) with the title "Simple Calculator". The idea is to create labels and widgets for user to input values.
- Labels (label_num1, label_num2) to prompt users for input of the first and second numbers.
- Creates entry widgets (entry1, entry2) where users can input numerical values.
- Next, the code sets up buttons (button_add, button_subtract, button_multiply, button_divide) for addition, subtraction, multiplication, and division operations, respectively.
- After that, it packs these widgets and buttons into the window, arranging them side by side using the pack() method with the side parameter.
- Finally, an empty label_result label is used to display the result of the arithmetic operation performed.
- The buttons have commands associated with them, calling click_button() with the respective operation when clicked. Users can input numbers into the entry1 and entry2 fields and choose an operation by clicking the buttons.
Conclusion
A calculator program in Python can be designed to perform mathematical operations like addition, subtraction, multiplication, and division efficiently, offering users a convenient tool for computational tasks. With its user-friendly interface and precise functionality, it simplifies complex calculations with just a few lines of code.
Creating a basic calculator with a graphical user interface (GUI) using the Tkinter library in Python offers an accessible way to perform simple arithmetic operations. The calculator lets users enter numbers, select arithmetic operations, and examine the answers instantaneously by utilizing Tkinter's features.
This calculator software illustrates the basic concepts of managing user input, carrying out mathematical operations, and presenting results in a graphical user interface. With the use of buttons that depict addition, subtraction, multiplication, and division operations, users may easily complete simple math calculations.
Additionally, the program uses error handling to handle situations like division by zero or invalid inputs, guaranteeing a seamless user experience by presenting the proper error messages as needed.
Overall, this straightforward calculator shows how to integrate user interaction, arithmetic operations, and error handling into a graphical environment using Tkinter. It is meant to serve as an introduction to the process of constructing a basic graphical application in Python.
Frequently Asked Questions
Q1. What are the calculation functions in Python?
The most popular Python calculation functions are as follows:
- add() is a function that combines two numbers.
- subtract(): Two numbers are subtracted using this function.
- multiply(): This function performs a multiplication of two numbers.
- divide(): This function splits a pair of numbers in half.
- pow(): A number is raised to a power using this function.
- sqrt(): This function yields a number's square root.
- abs(): This yields a number's absolute value.
- round(): This function rounds an integer to the number of decimal places that you specify.
- ceil(): Yields the smallest integer that is either greater than or equal to a given number.
- floor(): Yields the largest integer that is either equal to or less than a given number.
Q2. What are the basic math calculations in Python?
In Python programs, we can perform multiple mathematical operations and calculations on variables, expressions, etc.. We use elements called operators to perform these manipulations. Some of the most common/ basic mathematical calculations and their operators are-
- Addition: The addition operator (+) to add two numbers or variables and calculate the sum.
- Subtraction: The subtraction operator (-) is used to subtract one number/ variable from another.
- Multiplication: The multiplication operator (*) is used to multiply one variable/ number by another.
- Division: The division operator (/) is used to divide one variable/ number (the dividend) by another (the divisor).
- Floor division: When two numbers are divided by each other, the floor division operator (//) eliminates any fractional part and returns the whole number portion of the quotient or the division's outcome. For instance, 10 / 3 = 3. It divides integers and rounds to the next whole number every time.
- Modulus: The remainder of the division of one number by another is returned by the modulus operator (%). For example, 10% of 3 is equal to 1, since 10% of 3 is less than 1. It's frequently used to find out what's left over after division and whether a given number is divisible by another.
- Exponentiation: A number is raised to the power of another number using the exponentiation operator (**). For instance, since 2 raised to the power of 3 equals 8, 2 ** 3 equals 8. For the specified exponent, it denotes repeatedly multiplying the base number by itself.
These operators can be used to manipulate numbers in simple mathematical operations.
Q3. How do I make Python calculate faster?
There exist multiple methods for enhancing Python computation speed in order to maximize efficiency. Here are some pointers:
- Make use of effective algorithms and data Structures: Make use of data structures and algorithms that are appropriate for the given task. Generally, efficient algorithms work better for certain problems.
- Libraries such as NumPy provide optimized algorithms and data structures for numerical computations in mathematics.
- Make Use of Compiled Libraries: For data manipulation and numerical calculations, make use of compiled libraries like Pandas, sciPy, and numpy. Their use of low-level languages, such as Fortran and C/C++, speeds up computations.
- Reduce Looping and Vectorize Operations: Reduce the amount of time you spend looping over elements by using the vectorized operations that libraries like NumPy provide. Vectorized operations increase efficiency by executing calculations on whole arrays or matrices at once.
- Utilize iterators and generators: Iterators and generators can expedite operations and use less memory, particularly when working with large datasets. Rather than putting all of the data into memory, they enable the processing of the data in smaller portions.
- Optimization and Profiling: Locate performance bottlenecks in your code by using profiling tools such as line_profiler or cProfile. Refactor or rewrite the identified slow sections to make them more efficient.
- Cythonize Important Code Segments: Superset Cython translates Python code to C code, which is subsequently compiled into machine code. Important portions of the code can be substantially sped up in this way.
Q4. Is Calculate a function in Python?
In Python, calculate is not a built-in function like print(), len(), or input(). However, calculate can be a function if it is defined within the Python code. For instance, if you define a function named calculate in your Python code, it becomes a user-defined function that can perform a specific task or computation based on the code written within its block.
For example: Following code is defining a function named calculate that adds two numbers and returns the result:
def calculate(a, b):
result = a + b
return result
In this example, calculate is a function that takes two parameters (a and b), performs addition on them within the function block, and returns the result. You can call this function and pass different arguments to perform addition using the calculate function.
For example,
# Using the calculate function to add numbers
sum_result = calculate(5, 3)print(sum_result) # Output will be 8
Here, the calculate function adds 5 and 3 together and returns the result, which is then printed to the console.
Q5. How do I start learning Python?
Learning Python is an excellent choice due to its simplicity and versatility. Here's a step-by-step guide on how to start learning Python:
- Establish Specific Goals: Recognize your motivation for learning Python. A goal will direct your learning path, whether it is for machine learning, web development, data analysis, etc.
- Install Python: Go to the official website, download, and install Python. Select the most recent stable version. Basic tools and an interpreter are included with Python.
- Select educational materials:
- Online Education: Coursera, Udemy, and Unstop are online platforms that provide beginner-friendly structured courses.
- Books: "Automate the Boring Stuff with Python" by Al Sweigart and "Python Crash Course" by Eric Matthes are two suggestions.
- Tutorials and Documentation: Additional helpful resources include Python documentation, online tutorials from sites like W3Schools, and YouTube video tutorials.
- Recognize fundamental ideas:
- Learn about the fundamental structure, indentation, and syntax of Python.
- Know how to use variables and the many forms of data (integers, floats, strings, lists, tuples, and dictionaries).
- Study functions, for-and while-loops, and if expressions.
- Always, always, always practice: Regular coding will help you to really comprehend things. You can use resources like LeetCode, HackerRank, or CodeSignal to solve coding puzzles on a daily basis.
- Implementing what you learned: What better way to test your concepts than by creating your own projects? Create little projects to put what you've learned to use. This will increase not only your in-depth knowledge of the language but also help you grasp the concept a little better.
- Investigate Specialized Areas: As you gain confidence with the fundamentals of Python, investigate particular areas based on your interests, such as web development (using Flask or Django), data analysis (using libraries like Pandas), machine learning (using TensorFlow or PyTorch).
- Involve the Community: To ask questions and pick up tips from others, join Python forums (such as r/learnpython on Reddit), communities (such as Stack Overflow), or go to Python meetups or events.
- Learn from Others: Assist other students, look for mentorship, or work in pairs with a more seasoned individual.
- Personal initiatives: Focus on your interest-based personal initiatives. Creating something useful will help you stay motivated and reinforce what you've learned.
- Keep Up to Date: Python is always changing. Stay current with libraries, best practices, and new features.
Q6. What is return in Python?
The return keyword in Python is used to deliver a value back to the caller within a function. Upon encountering a return statement in a called function, the interpreter promptly ends the function and returns any provided value to the caller.
Principal Aspects of Python's Return Type:
- Leaving a Position: No matter where in the function the return statement is located, it always results in the instant termination of the function's execution.
- Passing Values: The caller may optionally receive a value or the result of an expression via the return statement. As an illustration:
def add(a, b):
return a + b
- Returning None: Python returns None implicitly if the return statement is missing or contains no value.
- Several Returns: Python functions are capable of having several return statements under certain circumstances. The function terminates, and the accompanying return statement is executed when a condition is satisfied.
- Returning Early: Functions can return a value and terminate early if a condition is met by using return in conditional statements. It facilitates the control of flow inside a function.
🧮 Calculator Program In Python Quiz!
You may also like to read:
I am a biotechnologist-turned-content writer and try to add an element of science in my writings wherever possible. Apart from writing, I like to cook, read and travel.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Deepak Kaura 1 day ago
A Vishnuvardhan 1 day ago
Aishwarya Manikandan 1 day ago
Apoorva Gupta 1 day ago