Table of content:
- What Are Control Statements In Python?
- Types Of Control Statements In Python
- Conditional Control Statements In Python
- Loop Control Statements In Python
- Control Flow Altering Statements In Python
- Exception Handling Control Statements In Python
- Conclusion
- Frequently Asked Questions
Control Statement In Python | Types, Importance & Code Examples
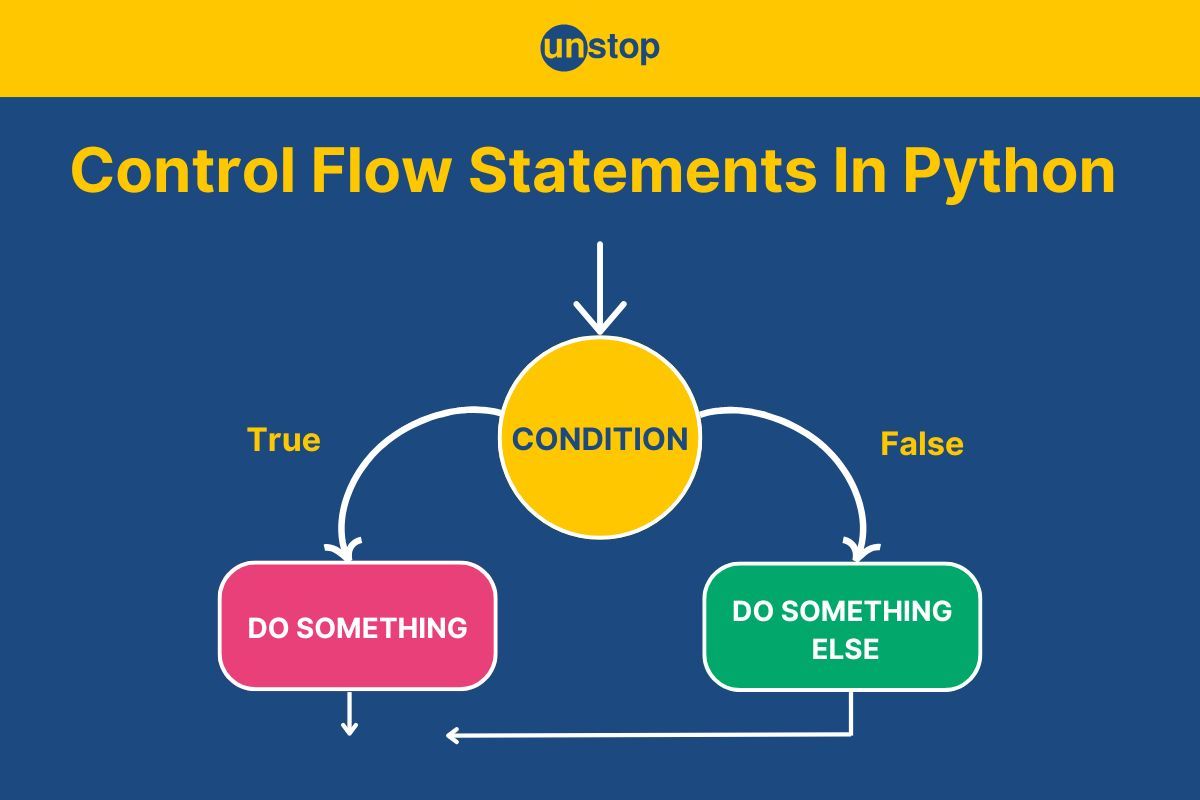
Control statements are fundamental components of programming that enable us to dictate the flow of execution within a program. In Python, these statements allow us to make decisions, repeat actions, and manage the sequence of operations based on specific conditions.
In this article, we will explore the different types of control statements in Python, including conditional statements like if, elif, and else, as well as loop constructs such as for and while. We will discuss their syntax, functionality, and practical examples to illustrate how they can be effectively employed in real-world programming tasks.
What Are Control Statements In Python?
Control statements are specific keywords used within control structures to modify the flow of execution. They enable us to dictate how a program behaves based on certain conditions or the occurrence of specific events. By utilising these statements, we can execute different code blocks under varying circumstances, effectively creating dynamic and responsive programs.
Importance Of Control Statements In Python
Control statements in Python programming are crucial for several reasons:
- Decision Making: They allow programs to make decisions based on user input or other variables, enabling more interactive applications.
- Code Efficiency: By using loops and conditional statements, we can avoid redundant code, leading to cleaner and more efficient programs.
- Flexibility: Control statements provide the ability to adapt the behaviour of a program in response to changing conditions or inputs, enhancing its versatility.
- Error Handling: Exception handling is vital for managing unexpected errors and controlling program flow during these situations, helping to maintain robustness in applications.
Types Of Control Statements In Python
There are primarily four types of control statements in Python:
- Conditional Control Statements: These statements, including if, elif, and else, allow us to execute certain code based on whether a specified condition is true or false.
- Looping Control Statements: The for and while loops enable us to repeat a block of code multiple times, making it easier to handle repetitive tasks.
- Control Flow Altering Statements: Statements like break, continue, and pass can alter the flow within loops, providing further control over how iterations are executed.
- Exception Handling Control Statements: Using try, except, and finally, these statements allow us to manage errors and exceptions that may occur during program execution, ensuring the program can continue running or fail gracefully.
Explore this amazing course and master all the key concepts of Python programming effortlessly!
Conditional Control Statements In Python
Conditional control statements are fundamental constructs that allow a program to execute certain blocks of code based on specified conditions. They enable decision-making capabilities within the code, allowing it to respond differently depending on the circumstances. Python provides several types of conditional statements, including if, if-else, and if-elif-else.
If Statement
The simplest form of a conditional statement is the if statement. It checks a condition and executes the associated block of code if the condition is true.
Syntax:
if condition:
# code to execute if condition is true
Here:
- if: The keyword that initiates the conditional statement.
- condition: An expression that evaluates to True or False.
Code Example:
YWdlID0gMTgKCmlmIGFnZSA+PSAxODoKICAgIHByaW50KCJZb3UgYXJlIGVsaWdpYmxlIHRvIHZvdGUuIikK
Output:
You are eligible to vote.
Explanation:
In the above code example-
- We start by defining a variable named age and assign it a value of 18.
- Next, we use an if statement to check if the value of age is greater than or equal to 18.
- If the condition is true (which it is, since age is 18), the code inside the if block will execute.
- In this case, we will print the message "You are eligible to vote." to the console, indicating that the person meets the age requirement for voting.
If-Else Statement
The if-else statement extends the if statement by providing an alternative block of code to execute when the condition is false.
Syntax:
if condition:
# code to execute if condition is true
else:
# code to execute if condition is false
Here:
- if: The keyword that initiates the conditional statement.
- condition: An expression that evaluates to True or False.
- else: The keyword that provides an alternative block.
Code Example:
YWdlID0gMTYKCmlmIGFnZSA+PSAxODoKICAgIHByaW50KCJZb3UgYXJlIGVsaWdpYmxlIHRvIHZvdGUuIikKZWxzZToKICAgIHByaW50KCJZb3UgYXJlIG5vdCBlbGlnaWJsZSB0byB2b3RlLiIpCg==
Output:
You are not eligible to vote.
Explanation:
In the above code example-
- We begin by defining a variable called age and assign it a value of 16.
- Then, we use an if statement to check if the value of age is greater than or equal to 18.
- Since the condition is false (16 is not greater than or equal to 18), the code inside the if block will not execute.
- Instead, we will move to the else block, which will execute when the if condition is false.
- As a result, we print the message "You are not eligible to vote." to the console, indicating that the person does not meet the age requirement for voting.
If-Elif-Else Statement
The if-elif-else statement allows us to check multiple conditions in sequence. If the first condition is false, it evaluates the next condition in the elif block and continues until it finds a true condition or reaches the else block.
Syntax:
if condition1:
# code to execute if condition1 is true
elif condition2:
# code to execute if condition2 is true
else:
# code to execute if all conditions are false
Here:
- if: The keyword that initiates the first conditional statement.
- condition1: An expression that evaluates to True or False.
- elif: The keyword that allows for an additional condition to be checked.
- condition2: Another expression that evaluates to True or False.
- else: The keyword that provides an alternative block if none of the previous conditions were true.
Code Example:
YWdlID0gMjAKCmlmIGFnZSA8IDEzOgogICAgcHJpbnQoIllvdSBhcmUgYSBjaGlsZC4iKQplbGlmIGFnZSA8IDIwOgogICAgcHJpbnQoIllvdSBhcmUgYSB0ZWVuYWdlci4iKQplbHNlOgogICAgcHJpbnQoIllvdSBhcmUgYW4gYWR1bHQuIikK
Output:
You are an adult.
Explanation:
In the above code example-
- We start by defining a variable named age and assign it a value of 20.
- Next, we use an if statement to check if age is less than 13.
- Since this condition is false (20 is not less than 13), the code inside the if block will not execute.
- Next, we encounter an elif (else if) statement that checks if age is less than 20.
- This condition is also false (20 is not less than 20), so the code inside the elif block will not execute either.
- Since both previous conditions are false, the program moves to the else block.
- The code inside the else block executes, printing the message "You are an adult." to the console, indicating that the person is categorized as an adult based on their age.
Loop Control Statements In Python
Looping control statements are constructs that allow us to execute a block of code repeatedly, based on a specified condition. These statements are essential for automating repetitive tasks, making our Python programs more efficient and concise. Python provides two primary types of looping control statements: for loops and while loops.
For Loop
The for loop is used to iterate over a sequence (like a list, tuple, or string) or a range of numbers. It executes the block of code for each item in the sequence.
Syntax:
for variable in sequence:
# code to execute for each item in the sequence
Here:
- for: The keyword that initiates the loop.
- variable: A temporary variable that takes the value of each item in the sequence during each iteration.
- sequence: A collection of items (e.g., list, tuple, string, or range).
Code Example:
ZnJ1aXRzID0gWyJhcHBsZSIsICJiYW5hbmEiLCAiY2hlcnJ5Il0KCmZvciBmcnVpdCBpbiBmcnVpdHM6CiAgICBwcmludChmcnVpdCkK
Output:
apple
banana
cherry
Explanation:
In the above code example-
- We begin by defining a list named fruits, which contains three elements: "apple," "banana," and "cherry."
- Next, we use a for loop to iterate over each element in the fruits list.
- For each iteration, the current element is assigned to the variable fruit.
- Inside the loop, we execute the print() function to display the value of fruit in the console.
While Loop
The while loop continues to execute a block of code as long as a specified condition is true. It is particularly useful when the number of iterations is not known beforehand.
Syntax:
while condition:
# code to execute as long as the condition is true
Here:
- while: The keyword that initiates the loop.
- condition: An expression that evaluates to True or False.
Code Example:
Y291bnQgPSAwCgp3aGlsZSBjb3VudCA8IDU6CiAgICBwcmludCgiQ291bnQgaXM6IiwgY291bnQpCiAgICBjb3VudCArPSAxCg==
Output:
Count is: 0
Count is: 1
Count is: 2
Count is: 3
Count is: 4
Explanation:
In the above code example-
- We start by defining a variable named count and assign it an initial value of 0.
- We then use a while loop that continues to execute as long as the condition count < 5 is true.
- Inside the loop, we use the print function to display the message "Count is:" followed by the current value of count.
- After printing, we increment the value of count by 1 using the count += 1 statement.
- This process repeats until count reaches 5, at which point the condition count < 5 becomes false.
- As a result, the program will print the values of count from 0 to 4.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Control Flow Altering Statements In Python
Control flow altering statements allow us to change the normal sequence of execution within loops or conditional blocks. These statements include break, continue, and pass, and they provide powerful ways to manipulate loops and conditionals by either exiting, skipping, or doing nothing in a block of code.
Break Statement
The break statement immediately terminates the enclosing loop, stopping further iterations and exiting the loop.
Syntax:
break
Code Example:
Zm9yIG51bWJlciBpbiByYW5nZSgxLCA2KToKICAgIGlmIG51bWJlciA9PSAzOgogICAgICAgIGJyZWFrCiAgICBwcmludCgiTnVtYmVyOiIsIG51bWJlcikK
Output:
Number: 1
Number: 2
Explanation:
In the above code example-
- We start with a for loop that will iterate through numbers generated by range(1, 6), which produces the values 1, 2, 3, 4, and 5.
- For each iteration, the current number is assigned to the variable number.
- Inside the loop, we have an if statement that checks if number is equal to 3.
- When number is 3, the break statement is executed, which immediately stops the loop.
- If number is not 3, the loop continues, and the program prints "Number:" followed by the current value of number.
Continue Statement
The continue statement skips the current iteration and moves directly to the next one. It’s useful when you want to skip certain conditions within a loop.
Syntax:
continue
Code Example:
Zm9yIG51bWJlciBpbiByYW5nZSgxLCA2KToKICAgIGlmIG51bWJlciA9PSAzOgogICAgICAgIGNvbnRpbnVlCiAgICBwcmludCgiTnVtYmVyOiIsIG51bWJlcikK
Output:
Number: 1
Number: 2
Number: 4
Number: 5
Explanation:
In the above code example-
- We start with a for loop that iterates over numbers generated by range(1, 6), which produces the values 1, 2, 3, 4, and 5.
- For each iteration, the current number is assigned to the variable number.
- Inside the loop, we use an if statement to check if number is equal to 3.
- When number is 3, the continue statement is executed, which skips the rest of the loop’s code for that iteration and moves to the next number.
- If number is not 3, the loop proceeds to the print function, which displays "Number:" followed by the current value of number.
Pass Statement
The pass statement acts as a placeholder that does nothing and allows the code to move to the next line. It is often used in situations where syntax requires a statement but you have no code to execute yet.
Syntax:
pass
Code Example:
Zm9yIG51bWJlciBpbiByYW5nZSgxLCA2KToKICAgIGlmIG51bWJlciA9PSAzOgogICAgICAgIHBhc3MKICAgIHByaW50KCJOdW1iZXI6IiwgbnVtYmVyKQo=
Output:
Number: 1
Number: 2
Number: 3
Number: 4
Number: 5
Explanation:
In the above code example-
- We start with a for loop that iterates over numbers generated by range(1, 6), which produces the values 1, 2, 3, 4, and 5.
- For each iteration, the current number is assigned to the variable number.
- Inside the loop, we use an if statement to check if number is equal to 3.
- When number is 3, the pass statement is executed, which does nothing and simply acts as a placeholder.
- Since pass has no effect, the loop continues normally, moving on to the print function.
- The program then prints "Number:" followed by the current value of number for each iteration.
Exception Handling Control Statements In Python
They provide a structured way to handle errors or exceptions that occur during program execution. By managing exceptions, we can prevent our programs from crashing unexpectedly and ensure they respond gracefully to unforeseen issues. Python offers several control statements for handling exceptions: try, except, and finally.
Try-Except Statement
The try-except statement allows us to test a block of code for potential errors. If an exception occurs within the try block, the program will jump to the except block to handle the error.
Syntax:
try:
# code that might cause an exception
except ExceptionType:
# code to handle the exception
Here:
- try: A keyword that starts the block where exceptions may occur.
- except: A keyword that defines a block of code to execute if an exception occurs.
- ExceptionType (optional): The type of exception to catch (e.g., ValueError, ZeroDivisionError).
Code Example:
dHJ5OgogICAgcmVzdWx0ID0gMTAgLyAwCmV4Y2VwdCBaZXJvRGl2aXNpb25FcnJvcjoKICAgIHByaW50KCJFcnJvcjogRGl2aXNpb24gYnkgemVybyBpcyBub3QgYWxsb3dlZC4iKQo=
Output:
Error: Division by zero is not allowed.
Explanation:
In the above code example-
- First, we start with a try block, where we place code that might cause an error.
- Now inside the try block, we attempt to calculate result by dividing 10 by 0.
- Since dividing by zero is mathematically undefined, this operation raises a ZeroDivisionError.
- We then immediately moves to the except block, which catches the ZeroDivisionError.
- In the except block, we use the print function to display the message "Error: Division by zero is not allowed."
- As a result, instead of stopping the program with an error, the program gracefully handles it and displays the error message.
Finally Statement
The finally statement provides a block of code that will run regardless of whether an exception was raised. It’s often used for cleanup tasks, like closing files or releasing resources.
Syntax:
try:
# code that might cause an exception
except ExceptionType:
# code to handle the exception
finally:
# code that always executes, regardless of exceptions
Here:
- try: The keyword that starts the block where exceptions may occur.
- except: The keyword to catch and handle specific exceptions.
- finally: A keyword for code that always executes, regardless of whether an exception occurred.
Code Example:
dHJ5OgogICAgcmVzdWx0ID0gMTAgLyAwCmV4Y2VwdCBaZXJvRGl2aXNpb25FcnJvcjoKICAgIHByaW50KCJFcnJvcjogRGl2aXNpb24gYnkgemVybyBpcyBub3QgYWxsb3dlZC4iKQpmaW5hbGx5OgogICAgcHJpbnQoIkV4ZWN1dGlvbiBjb21wbGV0ZWQuIikK
Output:
Error: Division by zero is not allowed.
Execution completed.
Explanation:
In the above code example-
- We begin with a try block, where we place code that could potentially raise an error.
- Inside the try block, we try to calculate result by dividing 10 by 0.
- Dividing by zero is undefined, so this operation raises a ZeroDivisionError.
- The program immediately moves to the except block, which catches the ZeroDivisionError.
- In the except block, we use the print function to display the message "Error: Division by zero is not allowed."
- After the except block, we have a finally block, which always executes, regardless of whether an error occurred or not.
- Inside the finally block, we print "Execution completed." to indicate that the program has finished running this section of code.
- As a result, the program displays the error message and then confirms that execution has completed, providing a final message even when an error occurs.
Searching for someone to answer your programming-related queries? Find the perfect mentor here.
Conclusion
In conclusion, control statements are essential building blocks in Python programming language that allow us to guide how our code runs based on certain conditions. By using if, else, elif, and loops like for and while, we can create programs that respond intelligently to different situations, making our code more dynamic and interactive.
As you continue your journey in Python, mastering control statements will help you tackle a wide range of programming challenges. They not only make your code more flexible but also enhance your problem-solving skills. So, explore, practice, and see how these control statements can transform your coding experience!
Frequently Asked Questions
Q. Why are control statements important?
Control statements make code more efficient and readable. They allow developers to implement logic, which makes programs dynamic—meaning they can respond to user input or changes in data.
Q. What are control statements in Python?
Control statements in Python guide the flow of execution in a program based on conditions. These statements include if, else, elif, loops (for, while), and keywords like break, continue, and pass to manage loop execution.
Q. How does the if statement work in Python?
The if statement checks a condition. If the condition is True, it executes the code within its block. If False, the code is skipped. For example:
x = 5
if x > 3:
print("x is greater than 3") # This will execute since 5 > 3.
Q. What’s the difference between break and continue in loops?
Here are the key differences between break and continue statements in loops:
Feature |
Break Statement |
Continue Statement |
---|---|---|
Purpose |
Exits the loop entirely. |
Skips the current iteration and moves to the next one. |
Effect on Loop |
Stops the loop immediately, ending further execution within it. |
Skips remaining code for the current iteration only. |
Usage Example |
Typically used when a specific condition requires terminating the loop. |
Used when a condition requires skipping certain iterations. |
Placement |
Often placed inside an if statement to break based on a condition. |
Typically within an if statement to skip specific cases. |
After Effect |
Loop ends, and control moves to the statement after the loop. |
Loop continues with the next iteration. |
Example Code |
if i == 3: break |
if i == 3: continue |
Q. Can I nest control statements in Python?
Yes, you can nest control statements. That’s where putting one if statement inside another if statement comes in, allowing for more complex decision-making structures. Make sure it is correctly indented so it’s clear. For Example:
for i in range(5):
if i % 2 == 0:
print(f"{i} is even")
else:
print(f"{i} is odd")
In this example, each iteration of the for loop checks if i is even or odd.
Q. What is the purpose of the pass statement?
The pass statement does nothing and acts as a placeholder. It is useful when a statement is syntactically required, but no action is needed:
if condition:
pass # Placeholder for future code
With this, we conclude our discussion on control statements in Python. Here are a few other topics that you might be interested in reading:
- Difference Between Java And Python Decoded
- Difference Between C and Python | C or Python - Which One Is Better?
- Python IDLE | The Ultimate Beginner's Guide With Images & Codes
- Python Logical Operators, Short-Circuiting & More (With Examples)
- Python Bitwise Operators | Positive & Negative Numbers (+Examples)
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment