Table of content:
- What Is Python? An Introduction
- What Is The History Of Python?
- Key Features Of The Python Programming Language
- Who Uses Python?
- Basic Characteristics Of Python Programming Syntax
- Why Should You Learn Python?
- Applications Of Python Language
- Advantages And Disadvantages Of Python
- Some Useful Python Tips & Tricks For Efficient Programming
- Python 2 Vs. Python 3: Which Should You Learn?
- Python Libraries
- Conclusion
- Frequently Asked Questions
- It's Python Basics Quiz Time!
Table of content:
- Python At A Glance
- Key Features of Python Programming
- Applications of Python
- Bonus: Interesting features of different programming languages
- Summing up...
- FAQs regarding Python
- Take A Quiz To Rehash Python's Features!
Table of content:
- What Is Python IDLE?
- What Is Python Shell & Its Uses?
- Primary Features Of Python IDLE
- How To Use Python IDLE Shell? Setting Up Your Python Environment
- How To Work With Files In Python IDLE?
- How To Execute A File In Python IDLE?
- Improving Workflow In Python IDLE Software
- Debugging In Python IDLE
- Customizing Python IDLE
- Code Examples
- Conclusion
- Frequently Asked Questions (FAQs)
- How Well Do You Know IDLE? Take A Quiz!
Table of content:
- What Is A Variable In Python?
- Creating And Declaring Python Variables
- Rules For Naming Python Variables
- How To Print Python Variables?
- How To Delete A Python Variable?
- Various Methods Of Variables Assignment In Python
- Python Variable Types
- Python Variable Scope
- Concatenating Python Variables
- Object Identity & Object References Of Python Variables
- Reserved Words/ Keywords & Python Variable Names
- Conclusion
- Frequently Asked Questions
- Rehash Python Variables Basics With A Quiz!
Table of content:
- What Is A String In Python?
- Creating String In Python
- How To Create Multiline Python Strings?
- Reassigning Python Strings
- Accessing Characters Of Python Strings
- How To Update Or Delete A Python String?
- Reversing A Python String
- Formatting Python Strings
- Concatenation & Comparison Of Python Strings
- Python String Operators
- Python String Functions
- Escape Sequences In Python Strings
- Conclusion
- Frequently Asked Questions
- Rehash Python Strings Basics With A Quiz!
Table of content:
- What Is Python Namespace?
- Lifetime Of Python Namespace
- Types Of Python Namespace
- The Built-In Namespace In Python
- The Global Namespace In Python
- The Local Namespace In Python
- The Enclosing Namespace In Python
- Variable Scope & Namespace In Python
- Python Namespace Dictionaries
- Changing Variables Out Of Their Scope & Python Namespace
- Best Practices Of Python Namespace
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Namespaces!
Table of content:
- What Are Logical Operators In Python?
- The AND Python Logical Operator
- The OR Python Logical Operator
- The NOT Python Logical Operator
- Short-Circuiting Evaluation Of Python Logical Operators
- Precedence of Logical Operators In Python
- How Does Python Calculate Truth Value?
- Final Note On How AND & OR Python Logical Operators Work
- Conclusion
- Frequently Asked Questions
- Python Logical Operators Quiz– Test Your Knowledge!
Table of content:
- What Are Bitwise Operators In Python?
- List Of Python Bitwise Operators
- AND Python Bitwise Operator
- OR Python Bitwise Operator
- NOT Python Bitwise Operator
- XOR Python Bitwise Operator
- Right Shift Python Bitwise Operator
- Left Shift Python Bitwise Operator
- Python Bitwise Operations And Negative Integers
- The Binary Number System
- Application of Python Bitwise Operators
- Python Bitwise Operator Overloading
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Bitwise Operators!
Table of content:
- What Is The Print() Function In Python?
- How Does The print() Function Work In Python?
- How To Print Single & Multi-line Strings In Python?
- How To Print Built-in Data Types In Python?
- Print() Function In Python For Values Stored In Variables
- Print() Function In Python With sep Parameter
- Print() Function In Python With end Parameter
- Print() Function In Python With flush Parameter
- Print() Function In Python With file Parameter
- How To Remove Newline From print() Function In Python?
- Use Cases Of The print() Function In Python
- Understanding Print Statement In Python 2 Vs. Python 3
- Conclusion
- Frequently Asked Questions
- Know The print() Function In Python? Take A Quiz!
Table of content:
- Working Of Normal Print() Function
- The New Line Character In Python
- How To Print Without Newline In Python | Using The End Parameter
- How To Print Without Newline In Python 2.x? | Using Comma Operator
- How To Print Without Newline In Python 3.x?
- How To Print Without Newline In Python With Module Sys
- The Star Pattern(*) | How To Print Without Newline & Space In Python
- How To Print A List Without Newline In Python?
- How To Remove New Lines In Python?
- Conclusion
- Frequently Asked Questions
- Think You Can Print Without a Newline in Python? Prove It!
Table of content:
- What Is A Python For Loop?
- How Does Python For Loop Work?
- When & Why To Use Python For Loops?
- Python For Loop Examples
- What Is Rrange() Function In Python?
- Nested For Loops In Python
- Python For Loop With Continue & Break Statements
- Python For Loop With Pass Statement
- Else Statement In Python For Loop
- Conclusion
- Frequently Asked Questions
- Think You Know Python's For Loop? Prove It!
Table of content:
- What Is Python While Loop?
- How Does The Python While Loop Work?
- How To Use Python While Loops For Iterations?
- Control Statements In Python While Loop With Examples
- Python While Loop With Python List
- Infinite Python While Loop in Python
- Python While Loop Multiple Conditions
- Nested Python While Loops
- Conclusion
- Frequently Asked Questions
- Mastered Python While Loop? Let’s Find Out!
Table of content:
- What Are Conditional If-Else Statements In Python?
- Types Of If-Else Statements In Python
- If Statement In Python
- If-Else Statement In Python
- Nested If-Else Statement In Python
- Elif Statement In Python
- Ladder If-Elif-Else Statement In Python
- Short Hand If-Statement In Python
- Short Hand If-Else Statement In Python
- Operators & If-Esle Statement In Python
- Other Statements With If-Else In Python
- Conclusion
- Frequently Asked Questions
- Quick If-Else Statement Quiz– Let’s Go!
Table of content:
- What Is Control Structure In Python?
- Types Of Control Structures In Python
- Sequential Control Structures In Python
- Decision-Making Control Structures In Python
- Repetition Control Structures In Python
- Benefits Of Using Control Structures In Python
- Conclusion
- Frequently Asked Questions
- Control Structures in Python – Are You the Master? Take A Quiz!
Table of content:
- What Are Python Libraries?
- How Do Python Libraries Work?
- Standard Python Libraries (With List)
- Important Python Libraries For Data Science
- Important Python Libraries For Machine & Deep Learning
- Other Important Python Libraries You Must Know
- Working With Third-Party Python Libraries
- Troubleshooting Common Issues For Python Libraries
- Python Libraries In Larger Projects
- Importance Of Python Libraries
- Conclusion
- Frequently Asked Questions
- Quick Quiz On Python Libraries – Let’s Go!
Table of content:
- What Are Python Functions?
- How To Create/ Define Functions In Python?
- How To Call A Python Function?
- Types Of Python Functions Based On Parameters & Return Statement
- Rules & Best Practices For Naming Python Functions
- Basic Types of Python Functions
- The Return Statement In Python Functions
- Types Of Arguments In Python Functions
- Docstring In Python Functions
- Passing Parameters In Python Functions
- Python Function Variables | Scope & Lifetime
- Advantages Of Using Python Functions
- Recursive Python Function
- Anonymous/ Lambda Function In Python
- Nested Functions In Python
- Conclusion
- Frequently Asked Questions
- Python Functions – Test Your Knowledge With A Quiz!
Table of content:
- What Are Python Built-In Functions?
- Mathematical Python Built-In Functions
- Python Built-In Functions For Strings
- Input/ Output Built-In Functions In Python
- List & Tuple Python Built-In Functions
- File Handling Python Built-In Functions
- Python Built-In Functions For Dictionary
- Type Conversion Python Built-In Functions
- Basic Python Built-In Functions
- List Of Python Built-In Functions (Alphabetical)
- Conclusion
- Frequently Asked Questions
- Think You Know Python Built-in Functions? Prove It!
Table of content:
- What Is A round() Function In Python?
- How Does Python round() Function Work?
- Python round() Function If The Second Parameter Is Missing
- Python round() Function If The Second Parameter Is Present
- Python round() Function With Negative Integers
- Python round() Function With Math Library
- Python round() Function With Numpy Module
- Round Up And Round Down Numbers In Python
- Truncation Vs Rounding In Python
- Practical Applications Of Python round() Function
- Conclusion
- Frequently Asked Questions
- Revisit Python’s round() Function – Take The Quiz!
Table of content:
- What Is Python pow() Function?
- Python pow() Function Example
- Python pow() Function With Modulus (Three Parameters)
- Python pow() Function With Complex Numbers
- Python pow() Function With Floating-Point Arguments And Modulus
- Python pow() Function Implementation Cases
- Difference Between Inbuilt-pow() And math.pow() Function
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python’s pow() Function!
Table of content:
- Python max() Function With Objects
- Examples Of Python max() Function With Objects
- Python max() Function With Iterable
- Examples Of Python max() Function With Iterables
- Potential Errors With The Python max() Function
- Python max() Function Vs. Python min() Functions
- Conclusion
- Frequently Asked Questions
- Think You Know Python max() Function? Take A Quiz!
Table of content:
- What Are Strings In Python?
- What Are Python String Methods?
- List Of Python String Methods For Manipulating Case
- List Of Python String Methods For Searching & Finding
- List Of Python String Methods For Modifying & Transforming
- List Of Python String Methods For Checking Conditions
- List Of Python String Methods For Encoding & Decoding
- List Of Python String Methods For Stripping & Trimming
- List Of Python String Methods For Formatting
- Miscellaneous Python String Methods
- List Of Other Python String Operations
- Conclusion
- Frequently Asked Questions
- Mastered Python String Methods? Take A Quiz!
Table of content:
- What Is Python String?
- The Need For Python String Replacement
- The Python String replace() Method
- Multiple Replacements With Python String.replace() Method
- Replace A Character In String Using For Loop In Python
- Python String Replacement Using Slicing Method
- Replace A Character At a Given Position In Python String
- Replace Multiple Substrings With The Same String In Python
- Python String Replacement Using Regex Pattern
- Python String Replacement Using List Comprehension & Join() Method
- Python String Replacement Using Callback With re.sub() Method
- Python String Replacement With re.subn() Method
- Conclusion
- Frequently Asked Questions
- Know How To Replace Python Strings? Prove It!
Table of content:
- What Is String Slicing In Python?
- How Indexing & String Slicing Works In Python
- Extracting All Characters Using String Slicing In Python
- Extracting Characters Before & After Specific Position Using String Slicing In Python
- Extracting Characters Between Two Intervals Using String Slicing In Python
- Extracting Characters At Specific Intervals (Step) Using String Slicing In Python
- Negative Indexing & String Slicing In Python
- Handling Out-of-Bounds Indices In String Slicing In Python
- The slice() Method For String Slicing In Python
- Common Pitfalls Of String Slicing In Python
- Real-World Applications Of String Slicing
- Conclusion
- Frequently Asked Questions
- Quick Python String Slicing Quiz– Let’s Go!
Table of content:
- Introduction To Python List
- How To Create A Python List?
- How To Access Elements Of Python List?
- Accessing Multiple Elements From A Python List (Slicing)
- Access List Elements From Nested Python Lists
- How To Change Elements In Python Lists?
- How To Add Elements To Python Lists?
- Delete/ Remove Elements From Python Lists
- How To Create Copies Of Python Lists?
- Repeating Python Lists
- Ways To Iterate Over Python Lists
- How To Reverse A Python List?
- How To Sort Items Of Python Lists?
- Built-in Functions For Operations On Python Lists
- Conclusion
- Frequently Asked Questions
- Revisit Python Lists Basics With A Quick Quiz!
Table of content:
- What Is List Comprehension In Python?
- Incorporating Conditional Statements With List Comprehension In Python
- List Comprehension In Python With range()
- Filtering Lists Effectively With List Comprehension In Python
- Nested Loops With List Comprehension In Python
- Flattening Nested Lists With List Comprehension In Python
- Handling Exceptions In List Comprehension In Python
- Common Use Cases For List Comprehensions
- Advantages & Disadvantages Of List Comprehension In Python
- Best Practices For Using List Comprehension In Python
- Performance Considerations For List Comprehension In Python
- For Loops & List Comprehension In Python: A Comparison
- Difference Between Generator Expression & List Comprehension In Python
- Conclusion
- Frequently Asked Questions
- Rehash Python List Comprehension Basics With A Quiz!
Table of content:
- What Is A List In Python?
- How To Find Length Of List In Python?
- For Loop To Get Python List Length (Naive Approach)
- The len() Function To Get Length Of List In Python
- The length_hint() Function To Find Length Of List In Python
- The sum() Function To Find The Length Of List In Python
- The enumerate() Function To Find Python List Length
- The Counter Class From collections To Find Python List Length
- The List Comprehension To Find Python List Length
- Find The Length Of List In Python Using Recursion
- Comparison Between Ways To Find Python List Length
- Conclusion
- Frequently Asked Questions
- Know How To Get Python List Length? Prove it!
Table of content:
- List of Methods To Reverse A Python List
- Python Reverse List Using reverse() Method
- Python Reverse List Using the Slice Operator ([::-1])
- Python Reverse List By Swapping Elements
- Python Reverse List Using The reversed() Function
- Python Reverse List Using A for Loop
- Python Reverse List Using While Loop
- Python Reverse List Using List Comprehension
- Python Reverse List Using List Indexing
- Python Reverse List Using The range() Function
- Python Reverse List Using NumPy
- Comparison Of Ways To Reverse A Python List
- Conclusion
- Frequently Asked Questions
- Time To Test Your Python List Reversal Skills!
Table of content:
- What Is Indexing In Python?
- The Python List index() Function
- How To Use Python List index() To Find Index Of A List Element
- The Python List index() Method With Single Parameter (Start)
- The Python List index() Method With Start & Stop Parameters
- What Happens When We Use Python List index() For An Element That Doesn't Exist
- Python List index() With Nested Lists
- Fixing IndexError Using The Python List index() Method
- Python List index() With Enumerate()
- Real-world Examples Of Python List index() Method
- Difference Between find() And index() Method In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python List Indexing? Take A Quiz!
Table of content:
- How To Remove Elements From List In Python?
- The remove() Method To Remove Element From Python List
- The pop() Method To Remove Element From List In Python
- The del Keyword To Remove Element From List In Python
- The clear() Method To Remove Elements From Python List
- List Comprehensions To Conditionally Remove Element From List In Python
- Key Considerations For Removing Elements From Python Lists
- Why We Need to Remove Elements From Python List
- Performance Comparison Of Methods To Remove Element From List In Python
- Conclusion
- Frequently Asked Questions
- Quiz– Prove You Know How To Remove Item From Python Lists!
Table of content:
- How To Remove Duplicates From A List In Python?
- The set() Function To Remove Duplicates From Python List
- Remove Duplicates From Python List Using For Loop
- Using List Comprehension Remove Duplicates From Python List
- Remove Duplicates From Python List Using enumerate() With List Comprehension
- Dictionary & fromkeys() Method To Remove Duplicates From Python List
- Remove Duplicates From Python List Using in, not in Operators
- Remove Duplicates From Python List Using collections.OrderedDict.fromkeys()
- Remove Duplicates From Python List Using Counter with freq.dist() Method
- The del Keyword Remove Duplicates From Python List
- Remove Duplicates From Python List Using DataFrame
- Remove Duplicates From Python List Using pd.unique and np.unipue
- Remove Duplicates From Python List Using reduce() function
- Comparative Analysis Of Ways To Remove Duplicates From Python List
- Conclusion
- Frequently Asked Questions
- Think You Know How to Remove Duplicates? Take A Quiz!
Table of content:
- What Is Python List & How To Access Elements?
- What Is IndexError: List Index Out Of Range & Its Causes In Python?
- Understanding Indexing Behavior In Python Lists
- How to Prevent/ Fix IndexError: List Index Out Of Range In Python
- Handling IndexError Gracefully Using Try-Except
- Debugging Tips For IndexError: List Index Out Of Range Python
- Conclusion
- Frequently Asked Questions
- Avoiding ‘List Index Out of Range’ Errors? Take A Quiz!
Table of content:
- What Is the Python sort() List Method?
- Sorting In Ascending Order Using The Python sort() List Method
- How To Sort Items In Descending Order Using Python sort() List Method
- Custom Sorting Using The Key Parameter Of Python sort() List Method
- Examples Of Python sort() List Method
- What Is The sorted() List Method In Python
- Differences Between sorted() And sort() List Methods In Python
- When To Use sorted() & When To Use sort() List Method In Python
- Conclusion
- Frequently Asked Questions
- Take A Quick Python's sort() Quiz!
Table of content:
- What Is A List In Python?
- What Is A String In Python?
- Why Convert Python List To String?
- How To Convert List To String In Python?
- The join() Method To Convert Python List To String
- Convert Python List To String Through Iteration
- Convert Python List To String With List Comprehension
- The map() Function To Convert Python List To String
- Convert Python List to String Using format() Function
- Convert Python List To String Using Recursion
- Enumeration Function To Convert Python List To String
- Convert Python List To String Using Operator Module
- Python Program To Convert String To List
- Conclusion
- Frequently Asked Questions
- Convert Lists To Strings Like A Pro! Take A Quiz
Table of content:
- What Is Inheritance In Python?
- Python Inheritance Syntax
- Parent Class In Python Inheritance
- Child Class In Python Inheritance
- The __init__() Method In Python Inheritance
- The super() Function In Python Inheritance
- Method Overriding In Python Inheritance
- Types Of Inheritance In Python
- Special Functions In Python Inheritance
- Advantages & Disadvantages Of Inheritance In Python
- Common Use Cases For Inheritance In Python
- Best Practices for Implementing Inheritance in Python
- Avoiding Common Pitfalls in Python Inheritance
- Conclusion
- Frequently Asked Questions
- 💡 Python Inheritance Quiz – Are You Ready?
Table of content:
- What Is The Python List append() Method?
- Adding Elements To A Python List Using append()
- Populate A Python List Using append()
- Adding Different Data Types To Python List Using append()
- Adding A List To Python List Using append()
- Nested Lists With Python List append() Method
- Practical Use Cases Of Python List append() Method
- How append() Method Affects List Performance
- Avoiding Common Mistakes When Using Python List append()
- Comparing extend() With append() Python List Method
- Conclusion
- Frequently Asked Questions
- 🧠 Think You Know Python List append()? Take A Quiz!
Table of content:
- What Is A Linked List In Python?
- Types Of Linked Lists In Python
- How To Create A Linked List In Python
- How To Traverse A Linked List In Python & Retrieve Elements
- Inserting Elements In A Linked List In Python
- Deleting Elements From A Linked List In Python
- Update A Node Of Linked List In Python
- Reversing A Linked List In Python
- Calculating Length Of A Linked List In Python
- Comparing Arrays And Linked Lists In Python
- Advantages & Disadvantages Of Linked List In Python
- When To Use Linked Lists Over Other Data Structures
- Practical Applications Of Linked Lists In Python
- Conclusion
- Frequently Asked Questions
- 🔗 Linked List Logic: Can You Ace This Quiz?
Table of content:
- What Is Extend In Python?
- Extend In Python With List
- Extend In Python With String
- Extend In Python With Tuple
- Extend In Python With Set
- Extend In Python With Dictionary
- Other Methods To Extend A List In Python
- Difference Between append() and extend() In Python
- Conclusion
- Frequently Asked Questions
- Think You Know extend() In Python? Prove It!
Table of content:
- What Is Recursion In Python?
- Key Components Of Recursive Functions In Python
- Implementing Recursion In Python
- Recursion Vs. Iteration In Python
- Tail Recursion In Python
- Infinite Recursion In Python
- Advantages Of Recursion In Python
- Disadvantages Of Recursion In Python
- Best Practices For Using Recursion In Python
- Conclusion
- Frequently Asked Questions
- Recursive Thinking In Python: Test Your Skills!
Table of content:
- What Is Type Conversion In Python?
- Types Of Type Conversion In Python
- Implicit Type Conversion In Python
- Explicit Type Conversion In Python
- Functions Used For Explicit Data Type Conversion In Python
- Important Type Conversion Tips In Python
- Benefits Of Type Conversion In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Type Conversion? Take A Quiz!
Table of content:
- What Is Scope In Python?
- Local Scope In Python
- Global Scope In Python
- Nonlocal (Enclosing) Scope In Python
- Built-In Scope In Python
- The LEGB Rule For Python Scope
- Python Scope And Variable Lifetime
- Best Practices For Managing Python Scope
- Conclusion
- Frequently Asked Questions
- Think You Know Python Scope? Test Yourself!
Table of content:
- Understanding The Continue Statement In Python
- How Does Continue Statement Work In Python?
- Python Continue Statement With For Loops
- Python Continue Statement With While Loops
- Python Continue Statement With Nested Loops
- Python Continue With If-Else Statement
- Difference Between Pass and Continue Statement In Python
- Practical Applications Of Continue Statement In Python
- Conclusion
- Frequently Asked Questions
- Python 'continue' Statement Quiz: Can You Ace It?
Table of content:
- What Are Control Statements In Python?
- Types Of Control Statements In Python
- Conditional Control Statements In Python
- Loop Control Statements In Python
- Control Flow Altering Statements In Python
- Exception Handling Control Statements In Python
- Conclusion
- Frequently Asked Questions
- Mastering Control Statements In Python – Take the Quiz!
Table of content:
- Difference Between Mutable And Immutable Data Types in Python
- What Is Mutable Data Type In Python?
- Types Of Mutable Data Types In Python
- What Are Immutable Data Types In Python?
- Types Of Immutable Data Types In Python
- Key Similarities Between Mutable And Immutable Data Types In Python
- When To Use Mutable Vs Immutable In Python?
- Conclusion
- Frequently Asked Questions
- Quiz Time: Mutable vs. Immutable In Python!
Table of content:
- What Is A List?
- What Is A Tuple?
- Difference Between List And Tuple In Python (Comparison Table)
- Syntax Difference Between List And Tuple In Python
- Mutability Difference Between List And Tuple In Python
- Other Difference Between List And Tuple In Python
- List Vs. Tuple In Python | Methods
- When To Use Tuples Over Lists?
- Key Similarities Between Tuples And Lists In Python
- Conclusion
- Frequently Asked Questions
- 🧐 Lists vs. Tuples Quiz: Test Your Python Knowledge!
Table of content:
- Introduction to Python
- Downloading & Installing Python, IDLE, Tkinter, NumPy & PyGame
- Creating A New Python Project
- How To Write Python Hello World Program In Python?
- Way To Write The Hello, World! Program In Python
- The Hello, World! Program In Python Using Class
- The Hello, World! Program In Python Using Function
- Print Hello World 5 Times Using A For Loop
- Conclusion
- Frequently Asked Questions
- 👋 Python's 'Hello, World!'—How Well Do You Know It?
Table of content:
- Algorithm Of Python Program To Add To Numbers
- Standard Program To Add Two Numbers In Python
- Python Program To Add Two Numbers With User-defined Input
- The add() Method In Python Program To Add Two Numbers
- Python Program To Add Two Numbers Using Lambda
- Python Program To Add Two Numbers Using Function
- Python Program To Add Two Numbers Using Recursion
- Python Program To Add Two Numbers Using Class
- How To Add Multiple Numbers In Python?
- Add Multiple Numbers In Python With User Input
- Time Complexities Of Python Programs To Add Two Numbers
- Conclusion
- Frequently Asked Questions
- 💡 Quiz Time: Python Addition Basics!
Table of content:
- Swapping in Python
- Swapping Two Variables Using A Temporary Variable
- Swapping Two Variables Using The Comma Operator In Python
- Swapping Two Variables Using The Arithmetic Operators (+,-)
- Swapping Two Variables Using The Arithmetic Operators (*,/)
- Swapping Two Variables Using The XOR(^) Operator
- Swapping Two Variables Using Bitwise Addition and Subtraction
- Swap Variables In A List
- Conclusion
- Frequently Asked Questions (FAQs)
- Quiz To Test Your Variable Swapping Knowledge
Table of content:
- What Is A Quadratic Equation? How To Solve It?
- How To Write A Python Program To Solve Quadratic Equations?
- Python Program To Solve Quadratic Equations Directly Using The Formula
- Python Program To Solve Quadratic Equations Using The Complex Math Module
- Python Program To Solve Quadratic Equations Using Functions
- Python Program To Solve Quadratic Equations & Find Number Of Solutions
- Python Program To Plot Quadratic Functions
- Conclusion
- Frequently Asked Questions
- Quadratic Equations In Python Quiz: Test Your Knowledge!
Table of content:
- What Is Decimal Number System?
- What Is Binary Number System?
- What Is Octal Number System?
- What Is Hexadecimal Number System?
- Python Program to Convert Decimal to Binary, Octal, And Hexadecimal Using Built-In Function
- Python Program To Convert Decimal To Binary Using Recursion
- Python Program To Convert Decimal To Octal Using Recursion
- Python Program To Convert Decimal To Hexadecimal Using Recursion
- Python Program To Convert Decimal To Binary Using While Loop
- Python Program To Convert Decimal To Octal Using While Loop
- Python Program To Convert Decimal To Hexadecimal Using While Loop
- Convert Decimal To Binary, Octal, And Hexadecimal Using String Formatting
- Python Program To Convert Binary, Octal, And Hexadecimal String To A Number
- Complexity Comparison Of Python Programs To Convert Decimal To Binary, Octal, And Hexadecimal
- Conclusion
- Frequently Asked Questions
- 💡 Decimal To Binary, Octal & Hex: Quiz Time!
Table of content:
- What Is A Square Root?
- Python Program To Find The Square Root Of A Number
- The pow() Function In Python Program To Find The Square Root Of Given Number
- Python Program To Find Square Root Using The sqrt() Function
- The cmath Module & Python Program To Find The Square Root Of A Number
- Python Program To Find Square Root Using The Exponent Operator (**)
- Python Program To Find Square Root With A User-Defined Function
- Python Program To Find Square Root Using A Class
- Python Program To Find Square Root Using Binary Search
- Python Program To Find Square Root Using NumPy Module
- Conclusion
- Frequently Asked Questions
- 🤓 Think You Know Square Roots In Python? Take A Quiz!
Table of content:
- Understanding the Logic Behind the Conversion of Kilometers to Miles
- Steps To Write Python Program To Convert Kilometers To Miles
- Python Program To Convert Kilometer To Miles Without Function
- Python Program To Convert Kilometer To Miles Using Function
- Python Program to Convert Kilometer To Miles Using Class
- Tips For Writing Python Program To Convert Kilometer To Miles
- Conclusion
- Frequently Asked Questions
- 🧐 Mastered Kilometer To Mile Conversion? Prove It!
Table of content:
- Why Build A Calculator Program In Python?
- Prerequisites To Writing A Calculator Program In Python
- Approach For Writing A Calculator Program In Python
- Simple Calculator Program In Python
- Calculator Program In Python Using Functions
- Creating GUI Calculator Program In Python Using Tkinter
- Conclusion
- Frequently Asked Questions
- 🧮 Calculator Program In Python Quiz!
Table of content:
- The Calendar Module In Python
- Prerequisites For Writing A Calendar Program In Python
- How To Write And Print A Calendar Program In Python
- Calendar Program In Python To Display A Month
- Calendar Program In Python To Display A Year
- Conclusion
- Frequently Asked Questions
- Calendar Program In Python – Quiz Time!
Table of content:
- What Is The Fibonacci Series?
- Pseudocode Code For Fibonacci Series Program In Python
- Generating Fibonacci Series In Python Using Naive Approach (While Loop)
- Fibonacci Series Program In Python Using The Direct Formula
- How To Generate Fibonacci Series In Python Using Recursion?
- Generating Fibonacci Series In Python With Dynamic Programming
- Fibonacci Series Program In Python Using For Loop
- Generating Fibonacci Series In Python Using If-Else Statement
- Generating Fibonacci Series In Python Using Arrays
- Generating Fibonacci Series In Python Using Cache
- Generating Fibonacci Series In Python Using Backtracking
- Fibonacci Series In Python Using Power Of Matix
- Complexity Analysis For Fibonacci Series Programs In Python
- Applications Of Fibonacci Series In Python & Programming
- Conclusion
- Frequently Asked Questions
- 🤔 Think You Know Fibonacci Series? Take A Quiz!
Table of content:
- Different Ways To Write Random Number Generator Python Programs
- Random Module To Write Random Number Generator Python Programs
- The Numpy Module To Write Random Number Generator Python Programs
- The Secrets Module To Write Random Number Generator Python Programs
- Understanding Randomness and Pseudo-Randomness In Python
- Common Issues and Solutions in Random Number Generation
- Applications of Random Number Generator Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python's Random Module? Prove It!
Table of content:
- What Is A Factorial?
- Algorithm Of Program To Find Factorial Of A Number In Python
- Pseudocode For Factorial Program in Python
- Factorial Program In Python Using For Loop
- Factorial Program In Python Using Recursion
- Factorial Program In Python Using While Loop
- Factorial Program In Python Using If-Else Statement
- The math Module | Factorial Program In Python Using Built-In Factorial() Function
- Python Program to Find Factorial of a Number Using Ternary Operator(One Line Solution)
- Python Program For Factorial Using Prime Factorization Method
- NumPy Module | Factorial Program In Python Using numpy.prod() Function
- Complexity Analysis Of Factorial Programs In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Factorials In Python? Take A Quiz!
Table of content:
- What Is Palindrome In Python?
- Check Palindrome In Python Using While Loop (Iterative Approach)
- Check Palindrome In Python Using For Loop And Character Matching
- Check Palindrome In Python Using The Reverse And Compare Method (Python Slicing)
- Check Palindrome In Python Using The In-built reversed() And join() Methods
- Check Palindrome In Python Using Recursion Method
- Check Palindrome In Python Using Flag
- Check Palindrome In Python Using One Extra Variable
- Check Palindrome In Python By Building Reverse, One Character At A Time
- Complexity Analysis For Palindrome Programs In Python
- Real-World Applications Of Palindrome In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Palindromes? Take A Quiz!
Table of content:
- Best Python Books For Beginners
- Best Python Books For Intermediate Level
- Best Python Books For Experts
- Best Python Books To Learn Algorithms
- Audiobooks of Python
- Best Books To Learn Python And Code Like A Pro
- To Learn Python Libraries
- Books To Provide Extra Edge In Python
- Python Project Ideas - Reference
- Quiz To Rehash Your Knowledge Of Python Books!
Swap Two Variables In Python- Different Ways | Codes + Explanation
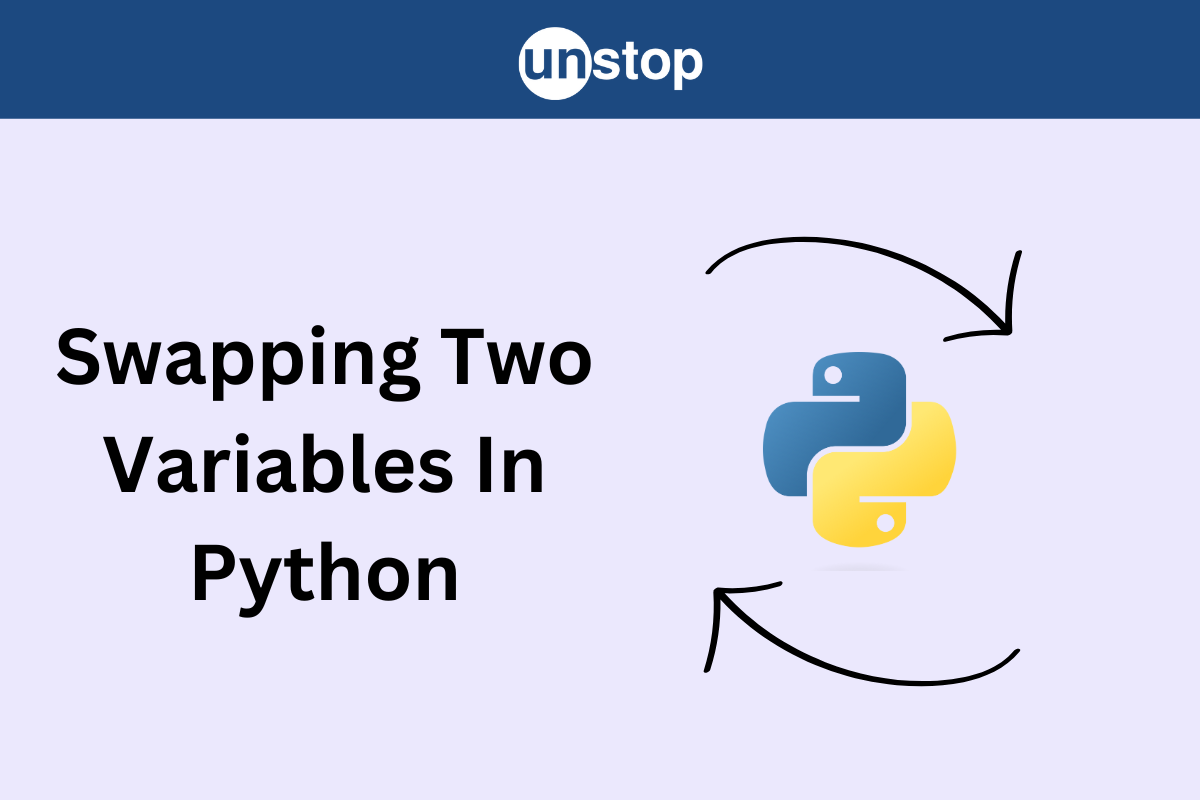
Variable swapping is a typical programming procedure that Python and other computer languages employ. The goal of this essay is to give readers a thorough understanding of the various Python variable-swapping methods. A temporary variable, arithmetic operators, the XOR operator, the comma operator, and bitwise addition and subtraction are a few of the techniques we will examine.
This article will walk you through each method step by step, regardless of your experience with Python development or your desire to improve your skills. Let's discover the ways to swap values of variables in Python.
Learn Python in 6 weeks! Check out this course on Unstop
Swapping in Python
Think about the case when the initial values of the two variables, a and b, are 5 and 10, respectively. The values of 'a' and 'b' should be swapped so that 'a' should be 10 and 'b' should be 5. This article will examine various methods for completing this swapping. These include swapping using a temporary variable, comma operator, arithmetic operators, etc. Each technique will be thoroughly described, giving the underlying idea a clear comprehension. Let's get started.
Swapping Two Variables Using A Temporary Variable
The most common method of swapping in Python is by using a temporary variable. In this method, we create a third temporary variable and assign the value of the first original variable to it. And then carry on a series of other assignments as mentioned in the points below:
- Say, the value of 'a' will be kept in a third variable that we'll refer to as a temporary variable.
- Then, we shall assign the value of b to a.
- Finally, we shall provide 'b' the temporary variable's value.
- We can then carry out the swap using a temporary storage site.
Code Example:
#Creating two variables
a = 5
b = 10
#Swapping using a temporary variable
temp = a
a = b
b = temp
print("After swapping:")
print("a =", a)
print("b =", b)
Output:
After swapping:
a = 10.0
b = 5.0
Explanation:
- First we created and declared two variables called a and b. Then, we assigned them values 5 and 10 respectively.
- Next, we create and declare a new variable called temp. This will be our temporary variable to help swap the values of a and b.
- Now we set temp equal to the value of a, which is 5.
- Then, we set its value equal to the value of b, which is 10.
- Now a's value is 10 instead of 5.
- Next, we set b's value equal to the value of temp, which is 5.
- So now b's value is 5 instead of 10.
- We swapped the values in a and b using the temporary variable temp.
- Finally, we print them to see the swapped values as output.
Swapping Two Variables Using The Comma Operator In Python
One way to swap two variables in Python using the comma operator is by assigning the values of the two variables to each other separated by a comma. This method does not require a third variable. We can use this operator for swapping in Python as follows:
- Let's call the initial two variables 'a' and 'b', which contain their corresponding values.
- We use the comma operator in a single line of code to switch the values of "a" and "b."
- We can assign several values in a single statement using the comma operator.
- The comma operator is used to concurrently assign the value of 'b' to 'a' and the value of 'a' to 'b'.
- The values of 'a' and 'b' are switched in this way. 'a' now has 'b''s original value, and 'b' now has 'a''s original value.
As is evident, there is no need for a temporary variable or any further actions for this switch to be accomplished in a single line of code. Let’s take a look at a code example that shows an implementation of this method of swapping two variables in Python.
Code Example:
#Initializing two variables
a = 5
b = 10
# Swapping variables using the comma operator
a, b = b, a
print("After swapping:")
print("a =", a)
print("b =", b)
Output:
After swapping:
a = 10.0
b = 5.0
Explanation:
- First we created and declared two variables called a and b, and then assign them values 5 and 10 respectively.
- Now we get to the swapping part i.e., a, b = b, a
Let's break this down:
- On the left side, we have a and b, and on the right side, we have b and a.
- The values on the right side are assigned to the variables on the left side.
- So b (which is 10) gets assigned to a.
- And a (which is 5) gets assigned to b.
- The values have been swapped.
- Finally, we print them to see the swapped values as output.
Swapping Two Variables Using The Arithmetic Operators (+,-)
The arithmetic operator is one of the common techniques used in Python to swap variables, where we use various arithmetic operators in pairs to swap variables like (+,-) and (*,/). Let us discuss here how will we use (+,-) arithmetic pair to swap variables:
- We shall change the values of "a" and "b" using addition and subtraction procedures.
- The total of 'a' and 'b' will be updated by adding 'a' and 'b'.
- Then, we will assign the difference to "b" by deducting it from the revised value of "a."
- Finally, we will allocate the difference to 'a' by deducting the updated value of 'a' (which now holds the original value of 'b') from 'b'.
- This technique employs mathematical processes to achieve the swap.
Code Example:
a = 5
b = 10
# Swapping variables using arithmetic operators
a = a + b
b = a - b
a = a - b
print("After swapping:")
print("a =", a)
print("b =", b)
Output:
After swapping:
a = 10.0
b = 5.0
Explanation:
- First we created and declared two variables called a and b, and then assign them values 5 and 10 respectively.
- Now we do : a = a + b
- This takes the original value of a (which is 5) and adds b (which is 10) to it. So now a becomes 5 + 10 = 15
- Next we do : b = a - b so, b gets set to the current value of a (which is 15) minus the original value of b (which is 10). So b becomes 15 - 10 = 5.
- Finally we do : a = a - b, so a gets set to its current value (15) minus the current value of b (5). So a becomes 15 - 5 = 10.
- The values have been swapped.
- Finally we print them out to see the swapped values.
Swapping Two Variables Using The Arithmetic Operators (*,/)
The arithmetic operator is also one of the common techniques used in Python to swap variables, where we use various arithmetic operators in pairs to swap variables like (+,-) and (*,/).
Let us discuss here how will we use the (*,/) arithmetic pair to swap variables:
- Get the variables started: Start by giving the two variables you want to swap their starting values. Suppose we have the variables 'a' and 'b'.
- Multiply 'a' by 'b' to get the answer.
- Give 'a' the outcome of the multiplication.
- Subtract the modified value of 'a' from 'b's' initial value.
- Assign 'b' the division's outcome.
- Subtract the updated value of 'b' from the updated value of 'a'.
- 'A' should be given the division's outcome.
- The values of 'a' and 'b' will be switched by carrying out these actions. Now, the initial value of 'b' will be saved in 'a,' and the initial value of 'a' will be stored in 'b'.
Code Example:
#Creating variables to swap
a = 5
b = 10
# Swapping variables using division and multiplication arithmetic operators
a = a * b
b = a / b
a = a / b
print("After swapping:")
print("a =", a)
print("b =", b)
Output:
After swapping:
a = 10.0
b = 5.0
Explanation:
- First, we created and declared two variables called a and b, and then assigned them values 5 and 10, respectively.
- Now we do : a = a * b
- This takes a (which is 5) and multiplies it by b (which is 10). So now a becomes 5 * 10 = 50.
- Next, we do: b = a / b, so b gets set to a (which is 50) divided by the original b (which is 10). So b becomes 50 / 10 = 5.
- Finally, we do: a = a / b, so a gets set to the current a (50) divided by the current b (5).
- So a becomes 50 / 5 = 10.
- The values have been swapped.
- Finally, we print out the swapped values.
Swapping Two Variables Using The XOR(^) Operator
Python allows you to swap two variables by using the bitwise XOR operator (). When comparing two operands, the XOR operator compares their corresponding bits and returns 1 if they differ and 0 if they are the same. Because of this, whenever the bits of the two variables differ, the outcome of an XOR operation on two variables will be a number with all of its bits set to 1.
For instance, when the digits 10 and 5 are XORed, the result is 11 (0b1010 ^ 0b0101 = 0b1111). This is because the result sets the bits that are different in the two numbers (1 and 0) to 1 while setting the same bits (0 and 0) to 0.
Let us now see how we can use this property of XOR operator to swap variables in Python :-
- Assume that we wish to swap the values of the two variables "a" and "b."
- Beginning with 'a,' assign 'b' the XOR operation between 'a' and 'b'. The value of 'a' and 'b' added together is temporarily stored thanks to this step.
- Then, give the updated value of 'a' (which now contains the combined value) and the original value of 'b' in the XOR operation. By doing this, the value of 'a' is essentially subtracted from the combined value, leaving only the original value of 'b'.
- Lastly, give 'a' the XOR operation between the updated values of 'a' and 'b'. By removing the original value of "b" from the combined value in this phase, the original value of "a" is obtained.
- Thus, we successfully swapped 'a' and 'b'.
Code Example:
# Swapping variables using the XOR operator
a = 5
b = 10
a = a ^ b
b = a ^ b
a = a ^ b
print("After swapping:")
print("a =", a)
print("b =", b)
Output:
After swapping:
a = 10.0
b = 5.0
Explanation:
- First, we created and declared two variables called a and b, and then assigned them values 5 and 10, respectively.
- Then, we start the swapping process by doing : a = a ^ b.
- This takes a (which is 5) and does an XOR with b (which is 10).
- 5 XOR 10 equals 15, so now a becomes 15 (binary 1010 ^ 0101).
- Next, we do : b = a ^ b, so b gets set to a XOR b. a is 15 and b is 10.
- 15 XOR 10 equals 5, so b becomes 5 (binary 1010 ^ 1010).
- Consequently, b now equals 5
- Finally, we do : a = a ^ b, so a gets set to a (which is 15) XOR b (which is 5).
- 15 XOR 5 equals 10. So a becomes 10 (binary 1010 ^ 0101).
- The values have been swapped.
- Lastly, we print them out the swapped values.
Swapping Two Variables Using Bitwise Addition and Subtraction
Bitwise operators, as you might know, operate on individual bits of binary numbers. In this section, we will look at bitwise addition and subtraction techniques, though they aren’t commonly used.
- Bitwise addition involves adding the matching bits of two binary numbers while accounting for carry values from earlier places. For example, the addition of 5 (101) and 3 (011) would result in 8 (1000) since 1+1 in the rightmost bit causes a carry of 1 to the following bit.
- Bitwise subtraction includes taking into account borrowed values from earlier positions as you subtract the bits of one binary integer from another. For example, if 3 (011) were subtracted from 5 (101), the result would be 2 (010), where 1 is taken from the leftmost bit position.
We can use bitwise addition and subtraction for swapping in Python programs by taking advantage of the fact that the sum of two numbers is the same as the complements of those numbers' differences. Let us now see how we can use this operator to swap variables in Python:
- Bitwise addition and subtraction operations between 'a' and 'b' allow us to accomplish the swap.
- We will update 'a' with the outcome by adding 'a' and 'b' bitwise.
- The difference will then be assigned to bitwise operand 'b' by deducting the initial value of 'b' from the revised value of 'a'.
- We will finally allocate the difference to bitwise divide the new value of 'b' from 'a'.
- This technique performs the swap using bitwise operations.
Code Example:
# Swapping variables using bitwise addition and subtraction
a = 5
b = 10
a = (a & b) + (a | b)
b = a + (~b) + 1
a = a + (~b) + 1
print("After swapping:")
print("a =", a)
print("b =", b)
Output:
After swapping:
a = 10
b = 5
Explanation:
- First, we created and declared two variables called a and b, then assigned them values 5 and 10, respectively.
- Now we start the swapping process by doing : a = (a & b) + (a | b)
- (a & b) does a bitwise AND of 5 (a) and 10 (b), which is 0.
- (a | b) does a bitwise OR of 5 (a) and 10 (b), which is 15.
- So we are setting a to 0 + 15 = 15.
- Next, we do b = a + (~b) + 1, so a is 15, ~b flips the bits in 10 to get -11, and 1 is added.
- So b becomes 15 + (-11) + 1 = 5
- Finally, we do: a = a + (~b) + 1, so a is 15, ~b flips 5 bits to get -6, and 1 is added.
- So a becomes 15 + (-6) + 1 = 10
- The values have been swapped.
- Finally, we print them out the swapped values.
Swap Variables In A List
Swapping variables in a list involves rearranging the values or elements within the list. This can be achieved using the following steps:
- We begin with a list of various items or values.
- We use a special mechanism to swap the values in the list.
- The indices of the two components that we want to swap are first determined.
- Let's assume that 'i' and 'j' are the indices.
- We set the value of element 'i' to a temporary variable before switching out the variables.
- The value of element 'j' is then assigned to the position of element 'i'.
- Finally, we place the temporary variable's value in the element 'j''s location.
Also Read: Difference between list and tuple in Python
Code Example:
# Swapping values in a list
my_list = [1, 2, 3, 4, 5]
i = 0
j = 1
my_list[i], my_list[j] = my_list[j], my_list[i]
print("After swapping:")
print("my_list =", my_list)
Output:
After swapping:
my_list = [2, 1, 3, 4, 5]
Explanation:
- We begin by creating a list called my_list with the entries [1, 2, 3, 4, 5].
- Next, we create a variable called i and set it equal to 0 : i = 0. This will represent the index of the first value we want to swap.
- Then we create a variable called j and set it to 1 : j = 1. This will represent the index of the second value we want to swap.
- Now we get to the swapping : my_list[i], my_list[j] = my_list[j], my_list[i]
- This uses the comma operator to swap the values at index i and index j in my_list.
- So my_list[0] (which is 1) gets swapped with my_list[1] (which is 2).
- After this, my_list will contain values as : [2, 1, 3, 4, 5]. So, the values are swapped.
- Finally, we print out my_list to see the swapped values in the list.
Conclusion
Swapping variables is a common programming task that involves exchanging the values stored in two variables. There are several techniques to swap variables in Python, each with their own advantages.
The key methods covered include using a temporary third variable, the comma operator, arithmetic operators like multiplication and division, bitwise XOR and bitwise AND/OR operators, and swapping values in lists by index. All these approaches allow efficient swapping without loss of data. The optimal technique depends on the specific context and programmer's preferences. But having an understanding of these versatile mechanisms gives Python programmers flexibility in interchanging values as needed. Happy Coding !!
Frequently Asked Questions (FAQs)
Q1. How to swap two elements in a string in Python?
Strings in Python are immutable, which means that their values cannot be changed directly. As a result, direct element swapping within a string is not possible. However, by changing the string into a list, switching the entries in the list, and then changing the list back to a string, you can accomplish a similar result. Here is how to swap two elements in a string step-by-step:
- Start with the string you wish to change.
- Using the list() method, which divides each character into distinct list elements, turn the string into a list.
- The indices of the two components you want to swap out should be determined.
- To swap the elements at the specified indices, use standard list element switching procedures.
- Convert the list back to a string using the join() function once the elements have been switched.
- Use the join() function to concatenate the components of the list back into a single string by specifying an empty string as the separator.
- The two elements will be switched in the final string.
Here is an instance to demonstrate the procedure:
# Original string
my_string = "Hello"
# Convert string to list
my_list = list(my_string)
# Identify indices to swap
index1 = 1
index2 = 3
# Swap elements in the list
my_list[index1], my_list[index2] = my_list[index2], my_list[index1]
# Convert list back to string
swapped_string = ''.join(my_list)
# Print the swapped string
print("Swapped string:", swapped_string)
Output:
Swapped string: Hlleo
The string "Hello" is where we begin in the aforementioned example. It is converted to a list, which is then changed to a string by switching the items at indices 1 and 3 ('e' and 'l'). "Hlelo" is the swapped string that results.
Remember that while using this method, you can swap items within a string without really changing the string itself. The desired elements are swapped into a new string that is then created.
Q2. How do you swap two lists in Python? Give an example.
You can use a temporary variable to hold one of the lists and then assign the data from one list to the other in Python to swap two lists. Here is an instance to demonstrate the procedure.
Code Example:
# Original lists
list1 = [1, 2, 3]
list2 = [4, 5, 6]
# Swapping lists using a temporary variable
temp = list1
list1 = list2
list2 = temp
# Print the swapped lists
print("Swapped list1:", list1)
print("Swapped list2:", list2)
Output :
Swapped list1: [4, 5, 6]
Swapped list2: [1, 2, 3]
Q3. How to Swap three numbers in Python? Give an example.
To swap three numbers in Python, we can utilize the same technique used for swapping two numbers i.e. using a temporary variable. Here's an example of how we can do it :
Code Example:
# Original values
a = 1
b = 2
c = 3
# Swapping three numbers using a temporary variable
temp = a
a = b
b = c
c = temp
# Print the swapped values
print("Swapped values:")
print("a =", a)
print("b =", b)
print("c =", c)
Output:
Swapped values:
a = 2
b = 3
c = 1
Three variables, a, b, and c, hold the initial values in the example above. These are the actions we take to exchange these values:
- Give a temporary variable called temp the value of a.
- Give the value of b.
- Give b the value of c.
- Give c the value of temp, which contains a's initial value.
- The values of a, b, and c have been rearranged as a result of the swap.
As a result, the values are switched, with a now holding b's original value, b's original value being held by c, and c's original value being held by a.
Q4. How to swap two numbers in Java using a temporary variable?
To swap two numbers in Java using a temporary variable is similar to Python, we can follow these steps:
- Set the integers you want to swap into a temporary variable called temp with the same data type.
- Give temp the value of a.
- Give a b value and vice versa.
- Give b the value of temp, which contains a's initial value.
- The values of a and b have been switched after the swap.
Because of this, a now has b's original value and b now has a's original value. Let us see an example of this :
import java.io.*;
public class NumberSwapper {
public static void main(String[] args) {
// Original values
int a = 5;
int b = 10;
// Swapping two numbers using a temporary variable
int temp = a;
a = b;
b = temp;
// Print the swapped values
System.out.println("Swapped values:");
System.out.println("a = " + a);
System.out.println("b = " + b);
}
}
Output :
Swapped values:
a = 10
b = 5
Q5. What does swap() mean in Python?
In Python, swap() typically refers to exchanging the values of two variables. However, it's important to note that Python does not have a built-in swap() function like other programming languages. Instead, you can achieve swapping using various techniques like a temporary variable, tuple unpacking, etc.
Q6. How to swap two variables in a single line in a Python program?
One of the most concise ways to swap two variables in a single line of code is by utilizing the comma operator. We have discussed this method above in this article. Let us see one more example of it.
Code Example:
# Swapping variables using the comma operator
a = 6
b = 11
#Swapping
a, b = b, a
print("After swapping:")
print("a =", a)
print("b =", b)
Output :
After swapping:
a = 11
b = 6
Q7. How to swap two strings without using a 3rd variable in Python?
As you may already be aware, Python has a built-in data type called a string that represents a group or stream of characters. We can use a variety of methods, including the comma operator, the temporary variable approach, and string concatenation with slicing, to swap two strings.
Combining string concatenation and slicing is one of the most widely used methods. While string slicing enables us to extract specific chunks of a string, string concatenation refers to the combining of two strings together. We can get the desired swapped version of the strings by combining these processes.
Code Example:
string1 = "Hello"
string2 = "World"
string1 = string1 + string2
string2 = string1[:len(string1) - len(string2)]
string1 = string1[len(string2):]
print("After swapping:")
print("string1 =", string1)
print("string2 =", string2)
Output:
After swapping:
string1 = World
string2 = Hello
Quiz To Test Your Variable Swapping Knowledge
You may be interested in reading:
I am a biotechnologist-turned-writer and try to add an element of science in my writings wherever possible. Apart from writing, I like to cook, read and travel.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Vishwajeet Singh 1 month ago
niyati m singh 1 month ago
Shradha Manure 1 month ago
Pardha Venkata Sai Patnam 1 month ago
PUJALA JYOTHIRMAYE 1 month ago
A Vishnuvardhan 1 month ago