If-Else Statement In Python | All Conditional Statements + Examples
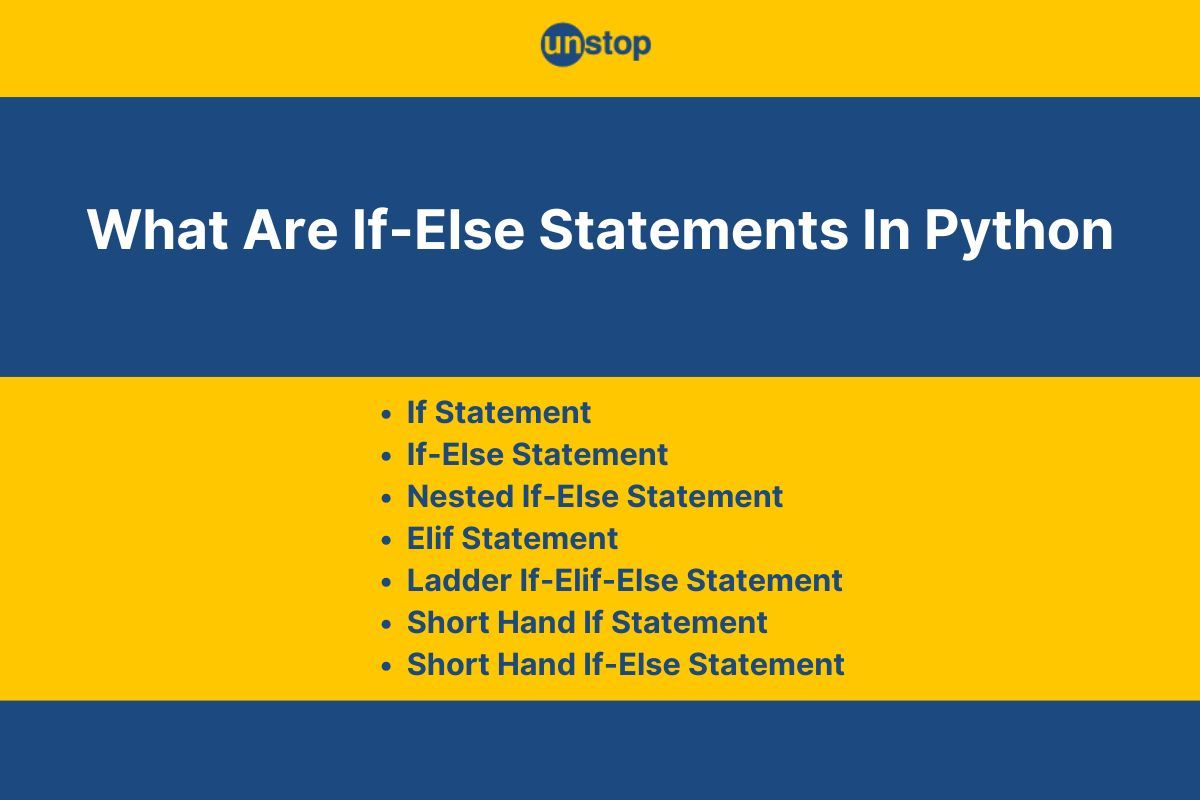
Python is celebrated for its simplicity and versatility, making it a top choice for developers and beginners alike. One of its core strengths lies in its ability to manage control flow, allowing programs to make decisions based on given conditions. At the heart of this decision-making process are conditional statements, which enable your code to react differently under various scenarios.
In this article, we will discuss the if-else statements in Python, their forms and uses with examples. Whether you're controlling simple program flow or building complex logic, understanding these statements is a must.
What Are Conditional If-Else Statements In Python?
Conditional statements are the decision-makers in your Python code. They allow you to dictate how your program should respond under specific circumstances. At their core, these statements evaluate a condition—something that can either be True or False. Based on the outcome, Python will execute one of the predefined code blocks.
It enables your program to act differently depending on the situation. For instance, you might want your code to display a special message when a user logs in successfully or alert them if their credentials are incorrect. Without these decision-making constructs, your Python programs would simply run from start to finish without any flexibility and be unable to adapt to changing inputs or environments.
Indentation In Python If-Else Statement
Unlike many other programming languages that use braces {} or keywords to define code blocks, Python relies on indentation to separate different blocks of code. This indentation isn’t just for readability; it’s syntactically required. Failing to indent properly can lead to IndentationError, a common stumbling block for beginners.
For example:
if condition:
# This block is indented and will run if the condition is True
print("Condition is True")
else:
# This block runs if the condition is False
print("Condition is False")
Here, the if-block is indented inside the line of code with the if keyword and the else-block is indented inside the line containing the else keyword.
Types Of If-Else Statements In Python
Python offers a variety of if-else structures to handle different scenarios. Each type allows developers to implement decision-making logic tailored to specific needs. Here are the different types of conditional/ if-else statements in Python programming:
- If Statement: Executes a specified block of code only when the specified condition is True.
- If-Else Statement: Provides an alternative block of code to execute when the condition is False. (In addition to the block when the condition is True)
- Nested If-Else Statement: Allows for an if-else statement inside another, enabling more complex decision trees.
- Ladder If-Elif-Else Statement: Evaluate multiple conditions sequentially using elif (else-if), executing the block of the first condition that evaluates to True.
- Short Hand If Statement: A compact form of the if statement, useful for simple, single-line condition checks.
- Short Hand If-Else Statement: Also called the ternary operator, it enables one-line conditional expressions for both True and False outcomes.
Each of these types offers flexibility to control the flow of your Python program efficiently. Let's look at each, starting with the if statement.
If Statement In Python
The if statement is the simplest form of a conditional statement in Python. It allows you to execute a block of code only if a specified condition evaluates to True. If the condition is False, the code block is skipped entirely, and the flow moves to the next line in the code.
Flowchart Of If-Statement In Python
The image above shows the flow of control through an if-statement in Python language. Now, let’s look at an example illustrating its implementation.
Syntax Of If-Statement In Python
if condition:
# Block of code to execute if the condition is True
Here,
- The if keyword marks the beginning of the conditional check.
- The condition refers to an expression that evaluates to True or False.
- The indented block contains the code to execute when the condition is True.
Example Of If-Statement In Python
Output:
The number is greater than 5.
Explanation:
In the simple Python code example, we have showcased two alternatives.
- In the first case, we first create a variable number with the value 10. Then, we use an if-statement with the condition number > 5, which evaluates to True.
- As a result, the if-block is executed, with the print() function displaying the respective message.
- In the second case, we assign the value 3 to the variable number and then again use an if-statement with the condition number>5. Here, the condition evaluates to False, which is why the if-block is skipped and no output is produced.
Check out this amazing course to become the best version of the Python programmer you can be.
If-Else Statement In Python
The if-else statement extends the basic if statement by providing an alternative block of code to execute when the condition evaluates to False. This ensures that one of the two blocks runs, depending on the result of the condition.
In short, the if-else statement in Python is a straightforward way to control program flow:
- If Statement: Executes a block of code when the condition is True.
- Else Statement: Executes an alternative block of code when the condition is False.
The simple Python program example in the next sections illustrates the implementation of the if-else statement.
Flowchart Of If-Else Statement In Python
Syntax Of If-Else Statement In Python
if condition:
# Block of code to execute if the condition is True
else:
# Block of code to execute if the condition is False
Here,
- The if keyword marks the beginning of the conditional statement.
- The else keyword marks the alternative block to execute when the condition is False.
- Both the blocks of code are indented inside the respective keywords.
Example Of If-Else Statement In Python
Output:
You are eligible to vote.
You are not eligible to vote.
Explanation:
In this Python program example, we again illustrate two cases to show how both the blocks of an if-else statement are executed alternatively.
- In the first scenario, we create an age variable (18) and use an if-else statement to check the condition age >= 18.
- Since the condition is True, the if block executes, displaying the string message– "You are eligible to vote." to the console.
- In the second scenario, we assign the value 16 to the age variable and then use an if-else statement with the same condition.
- Since the condition is False, the else-block executes, printing "You are not eligible to vote."
- This setup ensures that the program always provides a response, regardless of the condition’s outcome.
Nested If-Else Statement In Python
A nested if-else statement is when you place one if-else statement inside another. This structure allows you to check multiple conditions and respond accordingly, enabling more complex decision-making in your code. While you can nest more than one if-else statement, for this section, we will cover only a set of nested statements.
Flowchart Of Nested If-Else Statement In Python
Syntax Of Nested If-Else Statement In Python
if outer_condition:
if inner_condition:
# Block executed if both conditions are True
else:
# Block executed if outer_condition is True and inner_condition is False
else:
# Block executed if outer_condition is False
Here,
- The outer if-else statement begins with the first if keyword and marks the first decision level.
- The inner if-else begins with the second if keyword and marks the second decision level.
Note: The inner statement will executed only if the outer condition is True. If the outer condition is false, the outer else-block will be executed, skipping the inner statement completely.
Example Of Nested If-Else Statement In Python
Output:
Grade: B
Explanation:
In the example Python program,
- First, the outer if-else statement checks whether the score is at least 50. Since this is True, the inner if checks whether the score is 90 or more.
- Since this second condition is False, the else block of the inner statement executes, printing "Grade: B."
- If the outer condition had been False, the program would have skipped the inner block and directly printed "Grade: F."
Elif Statement In Python
The elif statement (short for "else if") allows you to check multiple conditions sequentially. It executes its block of code if its condition evaluates to True and no previous conditions were True. This helps in scenarios where you want to implement mutually exclusive conditions efficiently.
Syntax Of Elif Statement In Python
if condition1:
# Block executed if condition1 is True
elif condition2:
# Block executed if condition2 is True
else:
# Block executed if neither condition is True
Here,
- The if keyword marks the first condition, and the elif keyword marks the next condition, which is evaluated only if the first condition is False.
- The block indented inside the respective block is executed if the preceding condition is true.
- Multiple elif blocks can be used to handle multiple conditions.
Example Of Elif Statement In Python
Output:
The number is negative.
Explanation:
In the example Python code,
- We first create a variable called number with the value -3 and then use an elif statement to check two different conditions sequentially with only one else-block.
- The first if-condition checks if the number is greater than 0, which is False.
- As a result, the flow moves to the elif condition (number < 0), which is True.
- So, the corresponding if-block is executed printing the message– "The number is negative." to the console.
- If all if and elif conditions were False, the else block would have executed.
When To Use Elif In Python Programs
- Multiple Conditions: Use elif when you need to check more than two conditions sequentially.
- Mutual Exclusivity: Only one block in an if-elif-else chain will execute, making it efficient for decision-making.
Level up your coding skills with the 100-Day Coding Sprint at Unstop and get the bragging rights, now!
Ladder If-Elif-Else Statement In Python
The if-elif-else statement, often referred to as a ladder, is used when multiple conditions need to be evaluated in sequence. It allows you to check several conditions one after another, and the first condition that evaluates to True will have its corresponding block executed. If none of the conditions are True, the else block (if present) will execute.
Flowchart Of Ladder If-Elif-Else Statement In Python
Syntax of Ladder If-Elif-Else Statement in Python
if condition1:
# Block executed if condition1 is True
elif condition2:
# Block executed if condition2 is True
elif condition3:
# Block executed if condition3 is True
...
else:
# Block executed if none of the above conditions are True
Here,
- The if keyword marks the beginning of the ladder.
- The elif keyword allows for additional conditions to be checked, if the previous condition evaluates to false.
- The else keyword marks the block of code to be executed if none of the preceding conditions are met.
Example Of If-Statement In Python
Output:
The number is zero.
Explanation:
In the Python code example,
- We create a variable number with a value of 0 and use a ladder if-elif-else statement to check two conditions sequentially.
- The first if-condition checks if the number is greater than zero, i.e., number > 0.
- Since the condition is False, the flow moves to the next elif to check if the number < 0.
- This condition is also False, so the else block executes, printing the message– "The number is zero."
- This structure ensures that only one block is executed, even if multiple conditions could potentially be True.
Short Hand If-Statement In Python
The short-hand if statement offers a concise way to write a simple if statement on a single line. This is especially useful for quick checks or assignments where only one statement needs to be executed if the condition is True.
Syntax Of Short Hand If Statement In Python
if condition: expression
Here, the if keyword marks the statement with the condition referring to the boolean expression that evaluates to True or False, and the expression referring to the single statement to execute if the condition is True.
Example Of Shorthand If-Statement In Python
Output:
Number is greater than 5
Explanation:
In the Python program sample,
- We assign the value 10 to the variable number and then use the short-hand if-statement.
- The if-condition checks if the number is greater than 5, which evaluates to True.
- So, the built-in function print() executes on the same line as the if.
- This shorthand format keeps the code compact and is handy for straightforward checks.
Short Hand If-Else Statement In Python
The short-hand if-else statement, also known as the ternary operator, allows you to write a simple if-else block in a single line. It’s useful for quick decision-making and inline value assignment.
Syntax Of Short Hand If-Else Statement In Python
value_if_true if condition else value_if_false
Here,
- The condition refers to the statement condition/ boolean expression to evaluate.
- The terms value_if_true and value_if_false refer to the result if the condition is True or False, respectively.
Example Of Short Hand If-Else Statement In Python
Output:
Odd
Explanation:
In the basic Python program example,
- The condition checks if the number is even by dividing it by 2 and equating the remainder to zero, i.e., number % 2 == 0.
- Since it evaluates to False for 7, the value "Odd" is assigned to the result variable, which is then printed to the console.
- This compact syntax is perfect for quick evaluations and assignments in a single line.
Looking for guidance? Find the perfect mentor from select experienced coding & software engg. experts here.
Operators & If-Esle Statement In Python
Conditional statements in Python often work in tandem with operators to evaluate complex conditions. Logical operators like AND, OR, and NOT allow you to combine multiple conditions within an if-else structure, providing more flexibility and control over the program's flow.
Logical Operators In Python
- AND: Returns True if both conditions are True.
- OR: Returns True if at least one condition is True.
- NOT: Reverses the truth value of a condition.
Example Of Logical Operators & If-Else Statement In Python
Output:
Eligible for admission
Explanation:
In the Python code sample,
- The if condition uses the AND operator to ensure both age >= 18 and score >= 80 are True.
- Since both conditions hold, "Eligible for admission" is printed.
- If one or both conditions were False, the program would evaluate the elif statement using the OR operator to print the appropriate message.
- Finally, the else block serves as a fallback for unexpected scenarios.
Other Statements With If-Else In Python
In addition to the basic if-else structures, Python provides some specialized statements that work seamlessly with conditional logic. These statements enhance code readability and functionality by offering unique use cases for specific scenarios.
Pass With If-Else Statement In Python
The pass statement acts as a placeholder. It allows you to write an if-else block without executing any operation, which can be helpful when you're planning to implement logic later or need to maintain code structure.
Code Example:
Explanation:
In the sample Python program,
- We create a variable number with a value of 5 and use an if-statement with the condition checks if the number > 0.
- The condition evaluates to True, but the pass statement tells Python to do nothing and move on.
- This can be useful when sketching out a program's structure without breaking its execution.
Lambda Function With If Else Statement In Python
A lambda function is a small, anonymous function in Python that can take any number of arguments but only one expression. When combined with an if-else statement, it allows you to write compact, single-line conditionals for quick evaluations or inline computations.
Why Use Lambda If-Else Statement?
- Conciseness: Ideal for simple operations where defining a full function might feel excessive.
- Inline Use: Frequently used in contexts like sorting, filtering, or mapping, where brevity is key.
- Readability: Keeps the code clean and to the point when the logic is simple.
Syntax of Lambda If-Else Statement In Python
lambda arguments: expression_if_true if condition else expression_if_false
Here,
- arguments: Inputs to the lambda function.
- if condition: The condition to evaluate.
- expression_if_true: The value returned if the condition is true.
- expression_if_false: The value returned if the condition is false.
The sample Python code below illustrates the use of the lambda function with if-else statement in Python.
Code Example:
Output:
Even
Odd
Explanation:
In the basic Python code example, we define a lambda function that takes an input x. It evaluates x % 2 == 0:
- If True, it returns "Even."
- If False, it returns "Odd."
Key Benefits Of Lambda If-Else Statement In Python
- Quick Evaluations: Perfect for small conditional checks.
- Enhanced Productivity: Allows embedding logic directly in high-order functions like map(), filter(), or sorted().
- Avoids Boilerplate: Reduces the need for multiple lines of code for simple decisions.
When To Avoid Lambda If-Else Statement In Python
- Complex Logic: If the logic involves multiple conditions or becomes hard to read, a standard function is preferable.
- Debugging Needs: Lambdas are harder to debug since they lack a function name.
Conclusion
The if-else statements in Python are the backbone of decision-making in programming. Starting from the basic if statements to more advanced structures like nested if-else and ladder if-elif-else, there are a wide range of conditional statements offering ways to control the flow of your programs. Alternatively, you can also use the shorthand if-else statement for compact logic and powerful constructs like lambda if-else for concise inline operations.
Understanding these conditional statements is crucial for writing efficient, readable, and dynamic code. With this foundational knowledge, you will be able to handle complex conditions in Python like a pro.
Frequently Asked Questions
Q1. What is the purpose of if-else statements in Python?
If-else statements are used to control the flow of a program by executing specific blocks of code based on conditions. They enable decision-making, allowing the program to respond differently depending on the input or state.
Q2. What is the difference between if, if-else, and if-elif-else?
The difference between the various types of conditional/ if-else statements is:
- The if statement: Executes a block of code if the condition is true.
- The if-else statement: Executes one block if the condition is true, and another if it’s false.
- The if-elif-else statement: Adds multiple conditions to test sequentially, executing the first block where the condition is true.
Q3. Why is indentation important in Python if-else statements?
Python uses indentation to define the blocks of code that belong to each condition. Without proper indentation, the interpreter cannot determine which lines of code to execute for a given condition, leading to syntax errors.
Q4. Can we have multiple else statements in a single if-else block?
No, a single if block can only have one else clause. However, you can chain multiple conditions using elif to form a sequence of conditions and statements to execute.
Q5. What is the difference between a lambda if-else and a regular if-else?
A lambda if-else is a compact, one-liner function for simple conditions, while a regular if-else can handle more complex logic with multiple statements and conditions.
Q6. What happens if the condition in an if statement is not true?
If the condition evaluates to False, the program skips the block of code associated with that if statement. If there’s an else block, it executes the code there instead.
Q7. Can an if-else statement be written in a single line?
Yes, you can use the short-hand if-else statement in Python, allowing you to write conditional logic in a single line for simple expressions.
Check out the following Python topics:
- Python Assert Keyword | Types, Uses, Best Practices (+Code Examples)
- Python While Loop | Types With Control Statements (Code Examples)
- Python input() Function (+Input Casting & Handling With Examples)
- Python max() Function With Objects & Iterables (+Code Examples)
- Python Break Statement Explained (With All Loops + Code Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment