Python Assert Keyword | Types, Uses, Best Practices (+Code Examples)
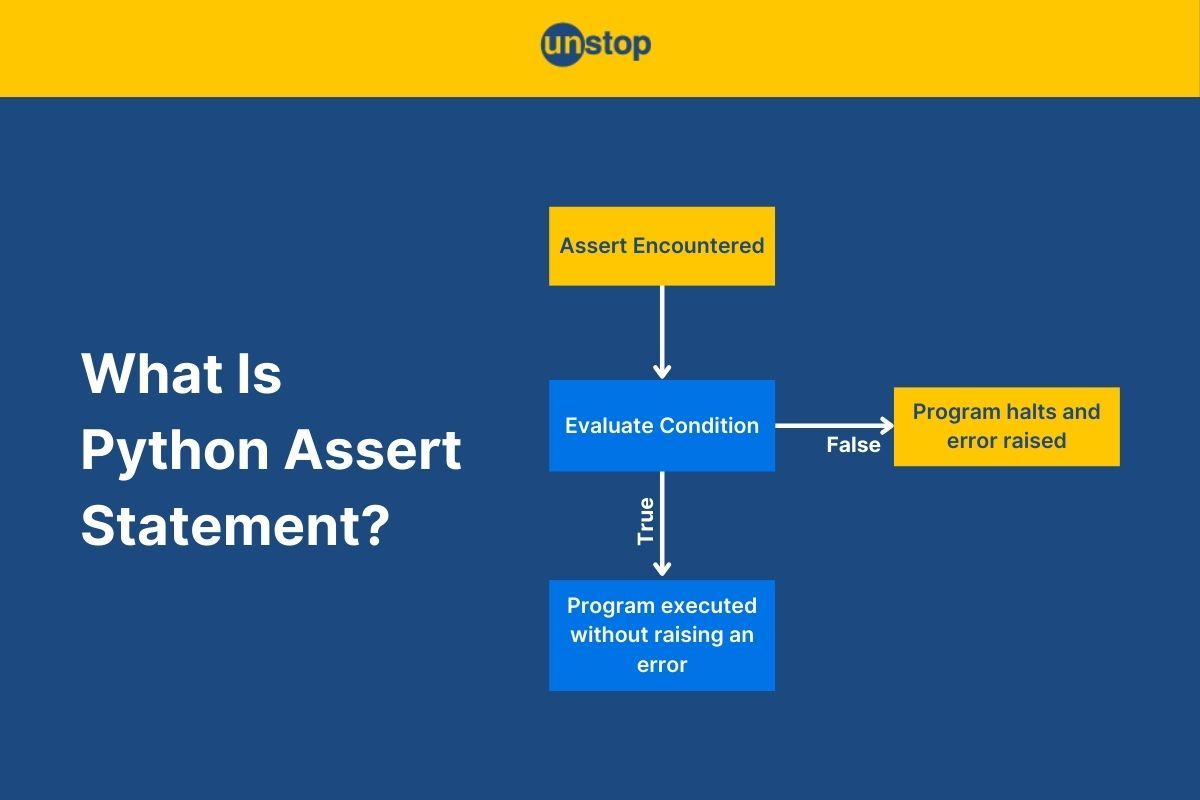
The Python assert keyword/ statement is a debugging tool that allows developers to test various assumptions in their code. Imagine debugging a complex program without a safety net—it’s like walking a tightrope without a harness. This is where Python assert statements come in. They act as checkpoints, ensuring that the program runs as expected by halting execution when conditions are not met.
In this article, we will discuss the Python assert keyword/ statement in depth, including its syntax, types, use cases, and best practices.
What Is Assert Keyword/ Statement In Python?
In Python, the assert statement is a built-in debugging aid. Think of it as a guardian that checks if certain conditions hold true during code execution.
- If the condition is true, the program continues to run smoothly.
- If not, an AssertionError is raised, stopping the program and signalling that something has gone off the rails.
For example, imagine you’re writing a function to calculate the square root of a number. You can use the Python assert keyword to ensure the input is non-negative. If someone tries to calculate the square root of -5, an assertion will immediately flag this as invalid input, preventing unexpected behavior later.
Python Assertions As Contracts
Assertions serve as implicit contracts between different parts of your code in Python programming. They verify that inputs, intermediate results, and outputs align with your expectations. Unlike exceptions, which handle known errors, assertions are for catching bugs during development, ensuring your assumptions are correct. (We will discuss the difference between the two in a later section.)
Everyday Examples Of Python Assertions
- Banking App: Ensuring the balance is never negative after a transaction.
- Game Development: Validating that player stats (e.g., health points) remain within acceptable bounds.
- Data Processing: Checking that a dataset is not empty before performing operations.
These are just a few scenarios where the Python assert keyword helps maintain the integrity of your code during development.
Syntax For Python Assert Statement
The syntax of the assert statement is straightforward but powerful.
assert condition, error_message
Here,
- The assert keyword marks the beginning of the statement.
- The condition is a boolean expression that evaluates to True or False. If True, the program continues execution. If False, an AssertionError is raised.
- The error_message (optional) is a string displayed as the error message if the assertion fails. This message provides context about what went wrong.
The assert statements check a condition and optionally provide an error message if the condition evaluates to False. Let’s look at a basic Python program example illustrating the implementation of the Python assert keyword/ statement.
Code Example: (Python assert without an error message)
In the basic Python code example, we use the assert keyword to check if x is greater than 0. Since the condition is True, the program continues running.
Note:
- Assertions are not a substitute for regular error handling. They’re designed to catch developer errors during testing and debugging.
- By default, Python disables assert statements when running in optimized mode (using the -O flag). This means assertions won’t run in production, making them a development-time tool. (More on these later đ)
How Does Python Assert Keyword/ Statement Work?
The Python assert statement follows a simple yet crucial workflow:
- Step 1: The flow moves to evaluate the condition with the assertion.
- Step 2a: If the condition is True, the flow moves to proceed with the next line of code.
- Step 2b: If the condition is False, the Python assert statement raises an AssertionError.
This logical flow ensures that assumptions in your code are consistently validated.
Code Example:
Output:
84.33333333333333
Traceback (most recent call last):
File "/home/main.py", line 7, in <module>
print(calculate_average([])) # Raises AssertionError with message: "The list of scores cannot be empty"
Explanation:
In the Python example program, we have demonstrated two cases, one to show valid assertions and the other for invalid.
- We first define a function called calculate_average(), which accepts a list called score.
- It uses the len() function to check the length of the list and then verifies that it is greater than zero using the assert keyword.
- It then calculates and returns the average of the list items by first getting the total using the built-in Python function sum() and dividing it by the length.
- In the valid case list, scores contains three elements. The assertion len(scores) > 0 evaluates to True because the list has a length greater than 0. Therefore, the function returns the average (84.33), which we then print to the console.
- Next, in the invalid case, we pass an empty list, so len(scores) > 0 evaluates to False. The assertion fails, and an AssertionError is raised with the specified message.
Check out this amazing course to become the best version of the Python programmer you can be.
Types Of Python Assert Statements
Assertions in Python come in various types, each suited for specific scenarios. Additionally, the assert statement can be formatted differently to suit your debugging needs. In this section, we will discuss the various types of Python assert keyword/ statement.
Value Python Assert Statement
Value assertions ensure that a variable holds a value within an expected range or satisfies specific conditions. These Python assert statements are particularly useful for validating user inputs or outputs of a function.
Why Use Them: Imagine you’re calculating the age of a user. It doesn’t make sense for the age to be negative or unrealistically high. Think of scenarios like verifying user age in a registration form. You wouldn't want to allow ages like -5 or 200, right? A value assertion helps enforce such logical constraints early.
Look at the simple Python program example below that illustrates this type of Python assert.
Code Example:
Explanation:
In the Python code example, we create a variable age and use assertions to check if that age lies between 0 and 120.
- If the condition is True, the program continues.
- If it’s False, an AssertionError is raised, printing the message "Age must be between 0 and 120".
- This ensures that invalid data (like a negative age) is caught immediately, preventing potential downstream errors.
- Since here, the condition is satisfied, and the program runs without raising an error/ generating an output.
Use Case: Value assertions are ideal for data validation tasks, such as ensuring a score is within valid bounds or a temperature reading is realistic.
Type Python Assert Statement
Type assertions validate the data type of a variable. Python is dynamically typed, meaning variables can change type at runtime, which sometimes leads to unexpected errors. This is why the type Python assert is especially useful.
Why Use Them: To avoid errors like passing a string to a function expecting a number, you can use type assertions to enforce the correct type.
Code Example:
Explanation:
In the example Python program, we have used the type assert to check if the name is of type str.
- We first create a variable name and assign the value “Unstop” to it.
- Then, we use the isinstance() function to check if the variable is of type string. The function returns True if the name is of type str; otherwise False.
- If False, the assert statement raises an AssertionError with the message "Name must be a string".
- Since the variable here is of type string, the program runs without generating an error.
Use Case: This type of Python assert statement is particularly helpful when working with functions that depend on specific data types to operate correctly. For instance, if a function expects a string but receives an integer, it could lead to runtime errors. Type assertions help enforce type safety.
Collection/ Membership Python Assert Statement
These Python assertions help check whether an element exists within a collection, such as a list, tuple, or dictionary. This is especially useful for validating configurations or ensuring that required items are present.
Why Use Them: When working with collections, it’s common to assume that certain key elements are present. Membership assertions can verify this assumption.
Code Example:
Explanation:
In the example Python code,
- We first create a list called fruits, containing three fruit names.
- Then, we use the membership Python assert to check if "apple" is a member of the fruits list.
- If "apple" is missing, an AssertionError is raised with the message "Apple is not in the fruits list".
- Since here the given value is contained in the list, the program runs without raising an error.
Use Case: This type of Python assert statement is useful in scenarios like verifying user permissions (checking if a user is in a list of admins) or validating keys in a configuration dictionary. For example, ensuring that a specific product exists in an inventory list before proceeding with a sale.
Exception Python Assert Statement
These assertions ensure that specific exceptions are avoided by validating critical conditions, such as division by zero or invalid file operations.
Why Use Them: They act as a safeguard, ensuring that your program only operates under valid conditions.
Code Example:
Explanation:
In the Python example code,
- We first define a function called divide which accepts two variables a and b.
- Inside the function, we use an assert statement to ensure that the divisor b is not zero, i.e., condition b != 0 (with the not equal to relational operator).
- If b equals zero, an AssertionError is raised with the message "Division by zero is not allowed".
- In the main part, we call the divide() function with the values 10 and 2. Since the divisor is not zero, the program runs without raising an error.
Use Case: This type of Python assert statement is ideal for preventing critical runtime errors and ensuring that preconditions for safe operation are met. For instance, division by zero is a common mathematical error that can crash your program. An assertion can help you catch such errors early.
Boolean Python Assert Statement
These assertions directly validate boolean conditions or flags that control program logic. They’re typically used in logical checks to ensure the program’s state is valid.
Why Use Them: In many cases, certain operations should only proceed if a specific condition is met, like ensuring a user is authenticated before accessing a resource.
Code Example:
Explanation:
In the Python program example,
- The assert checks if is_logged_in is True.
- If False, an AssertionError is raised with the message "User must be logged in to access this page".
Use Case: Boolean Python assert statements are useful in managing access control, feature toggles, and ensuring proper state in critical sections of the code. For instance, ensuring that a user is logged in before accessing a restricted page.
Level up your coding skills with the 100-Day Coding Sprint at Unstop and get the bragging rights, now!
Different Formats Of Python Assert Statement
The assert statement in Python can be used in several formats depending on the use case. Whether you want to provide a simple check or a detailed error message, the assert statement offers flexibility in handling different scenarios.
Python Assert Keyword Without Error Message
This is the simplest form of the Python assert statement. It checks if a given condition is True, and if not, raises an AssertionError. However, no additional message is provided to explain why the assertion failed.
Why Use It: This format is ideal when the condition being tested is clear enough that you don’t need to add extra context in case of failure.
Code Example:
Explanation:
In the sample Python code,
- We first create an age variable with a value of 30 and then use an assert statement to check if the value of age is greater than 0, i.e., condition age > 0.
- If the age is 0 or negative, the assertion fails, and an AssertionError is raised without any message. This is a basic check with no extra information.
Python Assert Keyword With Error Message
This format is an enhancement of the previous one, where you provide a custom error message that will be displayed if the assertion fails.
Why Use It: Providing a message gives more context on why the assertion failed, which can be especially helpful during debugging.
Code Example:
Explanation:
In the Python program sample,
- We create an age variable with a value of -5 and use an assert statement to check if the age is greater than 0.
- If age is negative, an AssertionError is raised with the message "Age must be a positive number".
- This form of the Python assert statement helps clarify the reason behind the failure, which can speed up debugging.
Python Assert Inside A Function
Assertions can be placed inside functions to validate inputs or intermediate results. This ensures that the function behaves as expected and that conditions are met before proceeding further.
Why Use It: This is particularly useful for validating parameters and ensuring that a function's input is within the expected range or type.
Code Example:
Explanation:
In the Python example code,
- We define a function called calculate_area, which accepts a variable radius.
- Inside the function, the assertion asserts radius > 0, "Radius must be positive" checks whether radius is positive.
- If the radius is negative, an AssertionError is raised with the message "Radius must be positive".
- This ensures that only valid input is processed in the function, helping prevent errors further down the line.
Python Assert To Check Dictionary Values
You can assert the presence of specific keys in a dictionary or validate the values associated with them. This format helps ensure that dictionaries contain valid data before proceeding with further operations.
Why Use It: This form of the Python assert statement is needed when you want to ensure the integrity of data stored in dictionaries, particularly when using dictionary values for further logic.
Code Example:
Explanation:
In the example,
- We use the Python assert keyword to check two conditions:
- Whether the key "age" exists in the user_info dictionary, i.e., “age” in user_info.
- Whether the value of "age" is greater than 0, i.e., user_info[“age”]>0.
- If either condition fails, an AssertionError is raised with the message "Invalid age value".
- This ensures that the dictionary contains valid data, which is especially helpful in applications where the dictionary’s values directly impact the program flow.
The different formats of the assert statement offer varying levels of error checking, from basic condition validation to detailed error messages. Choosing the right format depends on your specific use case and how much information you need to provide when an assertion fails. Each format serves to enhance the clarity, reliability, and maintainability of your code by ensuring that conditions are met before proceeding.
What Does the Assertion Error Do To Our Code?
An AssertionError is raised when a Python assert statement fails — that is when the condition specified by the assertion evaluates to False. This error is a built-in exception that interrupts the normal flow of the program, helping to identify problems early in the development process.
How Does Python Assertion Error Work?
- Assertion Evaluation: When a Python assert statement is encountered, the expression provided (the condition) is evaluated.
- Assertion Failure: If the condition is False, Python raises an AssertionError. The optional error message (if provided) will be included in the raised error to give more context about why the assertion failed.
- Program Halting/ Interruption: The program execution is halted, and the error traceback is shown, pointing to the location where the assertion failed.
Code Example:
Explanation:
In the sample Python program,
- The assert b != 0 statement checks that the denominator is not zero before performing division.
- When b == 0, the assertion fails, and an AssertionError is raised with the message "Denominator cannot be zero".
- The program is stopped at this point, preventing a division by zero error.
Assertion Error Details:
- When an assertion fails, the error message (if provided) appears with the AssertionError.
- A traceback is shown, pinpointing the location of the failure in the code.
Example Output:
AssertionError: Denominator cannot be zero
Here, the traceback highlights where the assertion failed in the program, helping you locate and fix the issue quickly.
When & Why Use Python Assert Statement?
Assertions are most beneficial when used during development and testing to validate assumptions. They provide a way to ensure that your code behaves as expected by verifying conditions at runtime. However, they are not intended to handle runtime errors in production environments. Here's when and why you should use assertions:
1. Checking Preconditions & Postconditions With Python Assert
Assertions should be used to check conditions before and after a function executes, ensuring the integrity of the program’s logic. For example, checking if inputs are within a valid range or if the output of a function is as expected. For example:
def process_order(order):
assert isinstance(order, dict), "Order must be a dictionary"
assert 'status' in order, "Order must have a status"
# Process order
2. Verifying Critical Assumptions In Code With Python Assert
Assertions are useful when you want to verify assumptions that your code depends on. These assumptions could be about the input types, the values, or the state of variables at certain points in the code. For example:
def calculate_discount(price, discount):
assert price >= 0, "Price cannot be negative"
assert 0 <= discount <= 100, "Discount should be between 0 and 100"
return price * (1 - discount / 100)
3. Catching "Can't Happen" Situations With Python Assert
If a situation should never occur in normal program execution (e.g., handling an unexpected input case), you can use assertions to catch these situations during development. For example:
def handle_exception(exception):
assert isinstance(exception, ValueError), "Only ValueError exceptions are allowed"
When Not To Use Python Assert? (Common Pitfalls)
While assertions are useful in development, there are several situations where they should be avoided to prevent issues like performance overhead, unexpected behavior, or improper error handling.
1. Avoid Using Python Assertions For User Input Validation
Python assert statements are meant for debugging and ensuring code correctness, not for handling expected user behavior. If you rely on assertions for input validation, they may be skipped when running Python in optimized mode (-O), leading to potential vulnerabilities or bugs.
For example:
# BAD PRACTICE: Using assertions to validate user input
user_age = int(input("Enter your age: "))
assert user_age > 0, "Age must be a positive number"
In this case, we use the input() function to take the value of age from the user and then use an assertion to validate it. It's better to use an if statement to validate input so it won't be skipped in production.
2. Python Assertions Should Not Be In Production Code
Assertions can be disabled globally in Python with the -O flag, meaning that any code relying on assertions for critical checks might fail silently. Therefore, you should never use assertions to check critical conditions in production environments. For example:
# BAD PRACTICE: Using assertions for critical runtime checks
def open_file(filename):
assert filename.endswith('.txt'), "File must be a .txt file"
# open file code
For runtime checks like verifying file extensions or error handling, use try-except blocks instead.
3. Do Not Rely On Python Assertions For Non-Exceptional Conditions
Assertions should not be used for conditions that can occur as part of normal program execution. If the situation is something that could regularly happen (e.g., user input errors, file not found), it's better to handle those with proper error handling mechanisms like exceptions. For example:
# BAD PRACTICE: Using assertions for expected behavior
items = [1, 2, 3]
assert len(items) == 3, "Expected 3 items" # Redundant check
The Python assert statement above, adds no real value because the length of the list is likely controlled in the program logic. Instead, simply handle these cases logically without the need for assertions.
4. Assertions Should Not Be Used for Complex Data Validations
For validating complex data structures like large lists or deeply nested objects, assertions can lead to performance overhead and reduce efficiency. These validations should be done through appropriate validation functions, not assertions. For example:
# BAD PRACTICE: Checking a large structure with assertions
assert len(data_structure) > 0, "Data structure cannot be empty" # Performance overhead
Instead of relying on assertions, ensure the code is robust and properly handles various cases with more efficient methods (such as using exception handling or validating the data before making complex operations).
Best Practices For Python Assert Statement
Now that we know when (and when not) to use Python assertions let’s discuss how to use them effectively. These best practices can help ensure assertions are used appropriately without cluttering the code or introducing performance issues:
- Use Assertions for Internal Logic & Development Checks: Assertions are intended to test internal logic assumptions during development. They should help you identify bugs and incorrect assumptions in your code. For example, validating input in a function, checking the state of a variable, or ensuring that some conditions hold true during execution (e.g., ensuring indices are within bounds).
- Use Assertions to Document Code: Assertions can serve as a form of documentation. They make it clear what conditions your code expects at certain points. For example, if you're writing a function that processes only positive integers, an assertion at the beginning of the function could document that expectation.
- Include Informative Error Messages: If an assertion fails, the error message should explain what went wrong. This helps you quickly identify why a certain condition was violated and debug more efficiently.
- Avoid Excessive Assertions: Using assertions for every small condition or in every line can clutter the code and make it harder to maintain. Limit the use of Python assert keyword/ statements to conditions that are critical for program correctness.
How Is Python Assert Different From Exception Handling?
While both assertions and exception handling help manage errors, they serve different purposes and are used in different contexts. The table below highlights the difference between Python assert keyword/ statement and exception handling.
Aspect |
Python Assert |
Python Exception Handling |
Purpose |
Used to verify conditions that should never fail during execution (internal logic checks). |
Used to handle runtime errors and exceptional situations, ensuring smooth program execution. |
When to Use |
Primarily used in the development and debugging stages to catch logic errors. |
Used for handling expected or unexpected runtime errors (e.g., file not found, invalid input). |
Behavior During Optimized Mode |
Can be disabled with the -O (optimize) flag, meaning assertions will not be evaluated. |
Never disabled, even in optimized mode; exceptions are always handled. |
Syntax |
assert condition, "Error message" |
try: ... except Exception as e: |
Control Flow |
If the condition fails, an AssertionError is raised, which usually halts the program. |
Exceptions provide a way to recover from errors (e.g., using try-except blocks to handle errors). |
Error Message |
Optional error message (if provided, displayed with the assertion error). |
Typically includes detailed error messages to describe what went wrong. |
Performance Impact |
Minimal overhead, used for internal logic checks and debugging. |
Can introduce overhead, especially if handling errors in complex blocks or retrying operations. |
Handling |
Doesn't allow for recovery. It’s meant for debugging and development purposes. |
Allows for recovery from errors by catching and handling them. Can include retries, fallbacks, etc. |
Use Case Example |
Checking the correctness of an index within a loop: assert i < len(list) |
Handling an I/O error when a file cannot be opened: try: open(file) except IOError: handle_error() |
Common Pitfalls |
Shouldn’t be used for user input validation or production code. |
Can lead to overuse or unnecessary handling of non-exceptional situations, leading to poor code design. |
Looking for guidance? Find the perfect mentor from select experienced coding & software experts here.
Conclusion
The Python assert statement is a powerful debugging tool that helps verify that certain conditions hold true during development.
- By using assertions, you can enforce assumptions about your code's behavior, catch potential errors early, and improve the readability and maintainability of your code.
- However, assertions are not a substitute for proper error handling. They are best suited for development and debugging, not for production environments, where explicit error handling through exceptions is preferred.
- Understanding when and where to use Python assert keyword, along with recognizing their limitations, is key to using them effectively.
It is also important to follow best practices, avoid overuse, and steer clear of using assertions for user input validation or critical runtime conditions. By balancing assertions with other error-handling techniques, you can create more robust, reliable Python programs.
Frequently Asked Questions
Q1. What is the purpose of the assert statement in Python?
The assert statement is used to test if a given condition is true. If the condition is false, it raises an AssertionError. It's typically used for debugging and validating assumptions about the code's behavior during development.
Q2. When should I use the Python assert statement?
You should use the Python assert keyword to check conditions that should always be true during the program's execution. For example, checking if an index is within bounds, verifying that a variable holds a specific value, or validating internal logic assumptions. Avoid using assertions for user input validation or error handling in production code.
Q3. Can I use Python assert for error handling?
No, Python assert is not meant for error handling. It is intended for debugging and development to catch logic errors early. For handling expected or unexpected errors during runtime (such as file I/O or invalid input), use Python's exception handling mechanisms like try-except.
Q4. What happens when I run Python with the -O flag?
When Python is run with the -O (optimize) flag, assertions are disabled. This means the conditions specified in assert statements are not checked, which can improve performance but may skip important internal logic checks. Therefore, avoid using assertions for critical checks in production environments.
Q5. What should I do if a Python assertion fails?
When an assertion fails, it raises an AssertionError and optionally displays the associated error message. This indicates that a condition you assumed to be true was not, and you should fix the underlying issue in the code.
Q6. Are there any performance concerns when using Python assertions?
Assertions generally have minimal overhead, but if used excessively or in performance-critical parts of your code, they can slow down execution. Since they are primarily a development tool, they should be used sparingly and removed or disabled in production code.
Q7. Can assertions help in debugging my code?
Yes, assertions are useful for identifying issues early by testing conditions that should hold true. They help in catching logic errors and provide immediate feedback, which is especially valuable during the development and debugging phases.
Q8. Can I disable assertions in my code?
Yes, you can disable assertions by running the script with the -O flag (optimize mode). This will skip all Python assert statements, which can be useful for improving performance in production environments but should not be used for critical error handling.
Do check the following out:
- Python max() Function With Objects & Iterables (+Code Examples)
- 12 Ways To Compare Strings In Python Explained (With Examples)
- Control Statement In Python | Types, Importance & Code Examples
- How To Convert Python List To String? 8 Ways Explained (+Examples)
- Python Namespace & Variable Scope Explained (With Code Examples)
- Python IDLE | The Ultimate Beginner's Guide With Images & Codes
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment